export_fig
==========
A toolbox for exporting figures from MATLAB to standard image and document formats nicely.
### Overview
Exporting a figure from MATLAB the way you want it (hopefully the way it looks on screen), can be a real headache for the unitiated, thanks to all the settings that are required, and also due to some eccentricities (a.k.a. features and bugs) of functions such as `print`. The first goal of export_fig is to make transferring a plot from screen to document, just the way you expect (again, assuming that's as it appears on screen), a doddle.
The second goal is to make the output media suitable for publication, allowing you to publish your results in the full glory that you originally intended. This includes embedding fonts, setting image compression levels (including lossless), anti-aliasing, cropping, setting the colourspace, alpha-blending and getting the right resolution.
Perhaps the best way to demonstrate what export_fig can do is with some examples.
### Examples
**Visual accuracy** - MATLAB's exporting functions, namely `saveas` and `print`, change many visual properties of a figure, such as size, axes limits and ticks, and background colour, in unexpected and unintended ways. Export_fig aims to faithfully reproduce the figure as it appears on screen. For example:
```Matlab
plot(cos(linspace(0, 7, 1000)));
set(gcf, 'Position', [100 100 150 150]);
saveas(gcf, 'test.png');
export_fig test2.png
```
generates the following:
| Figure: | test.png: | test2.png: |
|:-------:|:---------:|:----------:|
|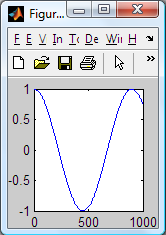|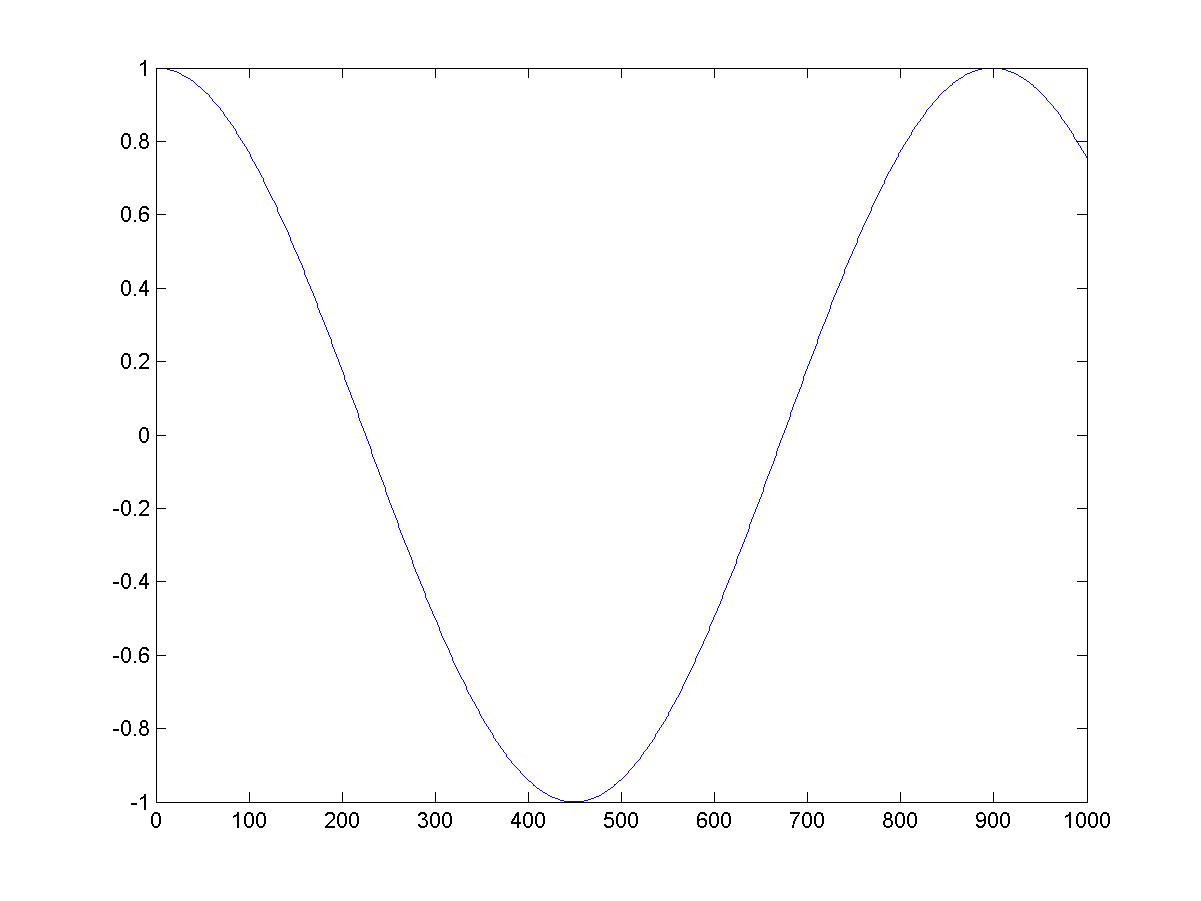||
Note that the size and background colour of test2.png (the output of export_fig) are the same as those of the on screen figure, in contrast to test.png. Of course, if you want the figure background to be white (or any other colour) in the exported file then you can set this prior to exporting using:
```Matlab
set(gcf, 'Color', 'w');
```
Notice also that export_fig crops and anti-aliases (smooths, for bitmaps only) the output by default. However, these options can be disabled; see the Tips section below for details.
**Resolution** - by default, export_fig exports bitmaps at screen resolution. However, you may wish to save them at a different resolution. You can do this using either of two options: `-m<val>`, where <val> is a positive real number, magnifies the figure by the factor <val> for export, e.g. `-m2` produces an image double the size (in pixels) of the on screen figure; `-r<val>`, again where <val> is a positive real number, specifies the output bitmap to have <val> pixels per inch, the dimensions of the figure (in inches) being those of the on screen figure. For example, using:
```Matlab
export_fig test.png -m2.5
```
on the figure from the example above generates:
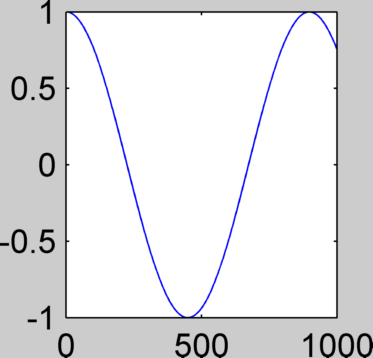
Sometimes you might have a figure with an image in. For example:
```Matlab
imshow(imread('cameraman.tif'))
hold on
plot(0:255, sin(linspace(0, 10, 256))*127+128);
set(gcf, 'Position', [100 100 150 150]);
```
generates this figure:

Here the image is displayed in the figure at resolution lower than its native resolution. However, you might want to export the figure at a resolution such that the image is output at its native (i.e. original) size (in pixels). Ordinarily this would require some non-trivial computation to work out what that resolution should be, but export_fig has an option to do this for you. Using:
```Matlab
export_fig test.png -native
```
produces:
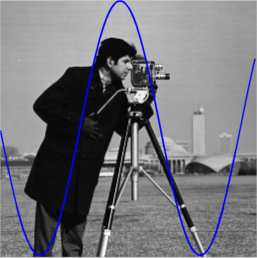
with the image being the size (in pixels) of the original image. Note that if you want an image to be a particular size, in pixels, in the output (other than its original size) then you can resize it to this size and use the `-native` option to achieve this.
All resolution options (`-m<val>`, `-q<val>` and `-native`) correctly set the resolution information in PNG and TIFF files, as if the image were the dimensions of the on screen figure.
**Shrinking dots & dashes** - when exporting figures with dashed or dotted lines using either the ZBuffer or OpenGL (default for bitmaps) renderers, the dots and dashes can appear much shorter, even non-existent, in the output file, especially if the lines are thick and/or the resolution is high. For example:
```Matlab
plot(sin(linspace(0, 10, 1000)), 'b:', 'LineWidth', 4);
hold on
plot(cos(linspace(0, 7, 1000)), 'r--', 'LineWidth', 3);
grid on
export_fig test.png
```
generates:
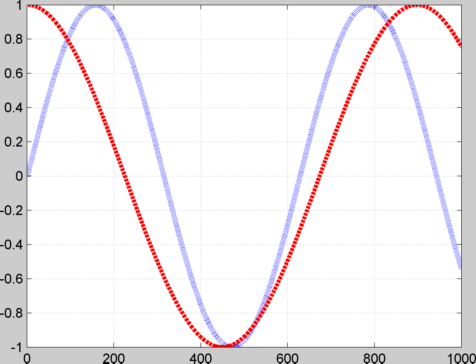
This problem can be overcome by using the painters renderer. For example:
```Matlab
export_fig test.png -painters
```
used on the same figure generates:

Note that not only are the plot lines correct, but the grid lines are too.
**Transparency** - sometimes you might want a figure and axes' backgrounds to be transparent, so that you can see through them to a document (for example a presentation slide, with coloured or textured background) that the exported figure is placed in. To achieve this, first (optionally) set the axes' colour to 'none' prior to exporting, using:
```Matlab
set(gca, 'Color', 'none'); % Sets axes background
```
then use export_fig's `-transparent` option when exporting:
```Matlab
export_fig test.png -transparent
```
This will make the background transparent in PDF, EPS and PNG outputs. You can additionally save fully alpha-blended semi-transparent patch objects to the PNG format. For example:
```Matlab
logo;
alpha(0.5);
```
generates a figure like this:
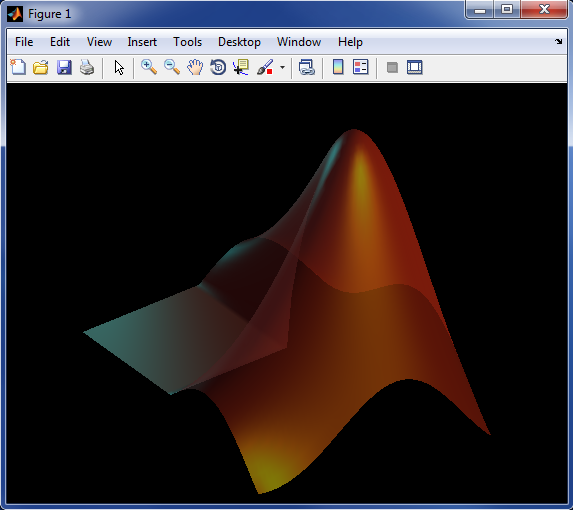
If you then export this to PNG using the `-transparent` option you can then put the resulting image into, for example, a presentation slide with fancy, textured background, like so:

and the image blends seamlessly with the background.
**Image quality** - when publishing images of your results, you want them to look as good as possible. By default, when outputting to lossy file formats (PDF, EPS and JPEG), export_fig uses a high quality setting, i.e. low compression, for images, so little information is lost. This is in contrast to MATLAB's print and saveas functions, whose default quality settings are poor. For example:
```Matlab
A = im2double(imread('peppers.png'));
B = randn(ceil(size(A, 1)/6), ceil(size(A, 2)/6), 3) * 0.1;
B = cat(3, kron(B(:,:,1), ones(6)), kron(B(:,:,2), ones(6)), kron(B(:,:,3), ones(6)));
B = A + B(1:size(A, 1),1:size(A, 2),:);
imshow(B);
print -dpdf test.pdf
```
generates a PDF file, a sub-window of which looks (when zoomed in) like this:
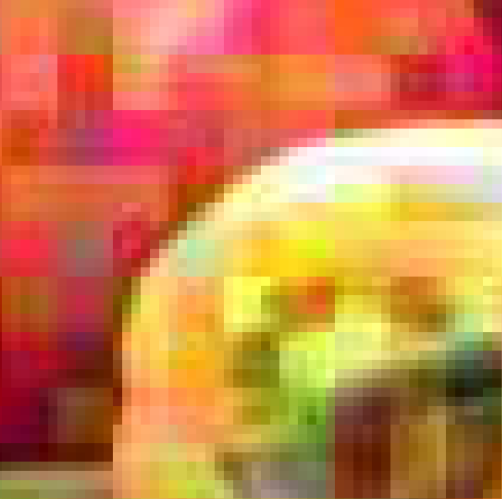
while the command
```Matlab
export_fig test.pdf
```
on the same figure produces this:

While much better, the image still contains some compression artifacts (see the low level noise around the edge of the pepper). You may prefer to export with no artifacts at all, i.e. lossless compression. Alternatively, you might need a smaller file, and be willing to accept more compression. Either way, export_fig has an option that can suit your needs: `-q<val>`, where <val> is a number from 0-100, will set the level of lossy image compression (again in PDF, EPS and JPEG outputs only; other formats are lossless), from high compression (0) to low compression/high quality (100). If you want lossless compression in any of those formats then specify a <val> greater than 100. For examp
没有合适的资源?快使用搜索试试~ 我知道了~
goGPS-MATLAB
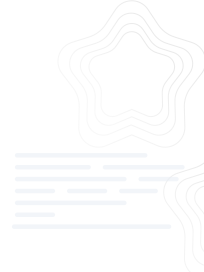
共1159个文件
m:820个
png:115个
txt:43个

需积分: 5 3 下载量 5 浏览量
2024-02-29
18:57:14
上传
评论
收藏 113.29MB ZIP 举报
温馨提示
goGPS是一款用于处理GNSS原始数据的软件。它最初是专门为GPS单频低成本接收机编写的,但现在它可以充分利用多星座,多频率,多跟踪观测。goGPS实现了多种算法来分析数据,目前这些算法包括两个主要的最小二乘(LS)引擎:一个用于可观测值的组合(例如,无电离层观测),另一个能够使用所有记录的频率和跟踪,而不执行任何组合(电离层延迟是正常方程的参数)。组合和不组合的引擎都可以计算精确点定位(PPP)解决方案和网络调整(NET)。 内容索引: 介绍 一点历史 关于 安装 需求 安装程序 goGPS目录 执行 设置 目录索引 菜单 侧边栏 选项卡 先进的 资源 命令 数据源 接收方的信息 预处理 处理 参数化购买力平价 参数化网络 大气 底栏 命令语言 目录索引 接收机数据组织 最小的脚本 推出 如何选择会议 如何选择接收器 主要命令 负载 PREPRO 购买力平价 网。 接收器上的循环 并行执行 PINIT PKILL 票面价值 地块及出口 显示 出口 验证 验证 电离层插值 SEID SID REMIONO 多路径管理 国会议员 其他命令 重命名 空 EMPTYWORK EMPTYOU
资源推荐
资源详情
资源评论
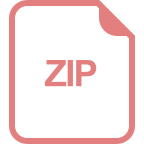
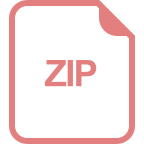
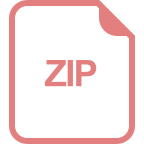
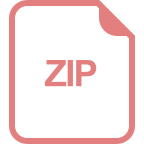
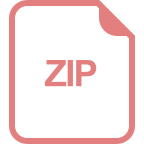
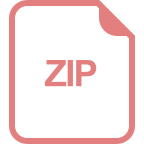
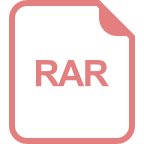
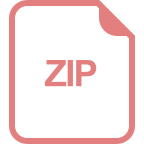
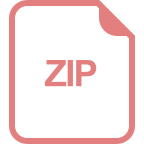
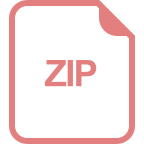
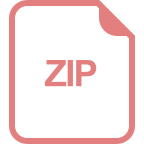
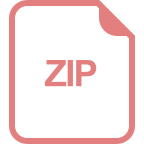
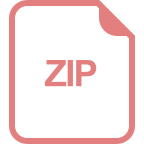
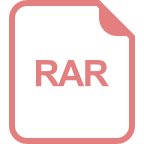
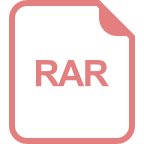
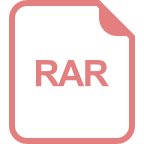
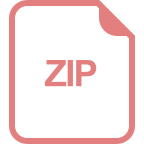
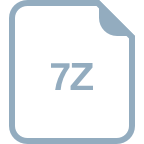
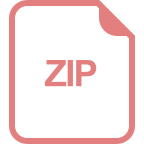
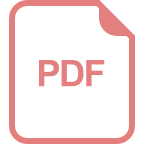
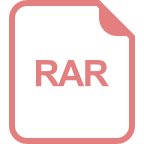
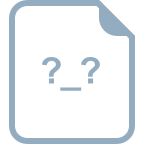
收起资源包目录

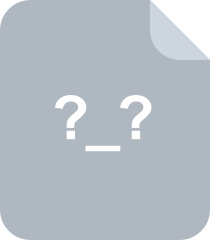
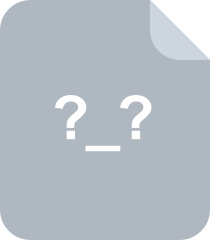
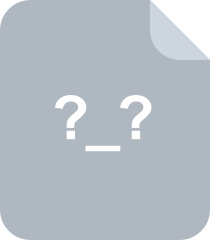
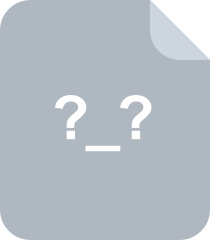
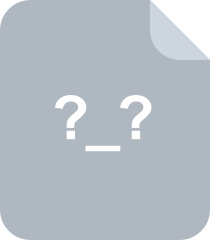
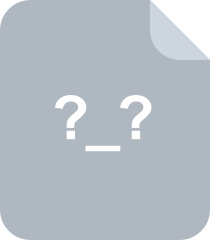
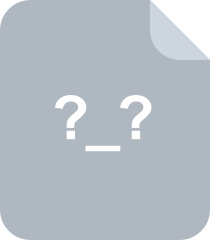
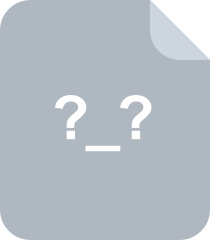
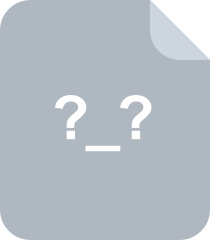
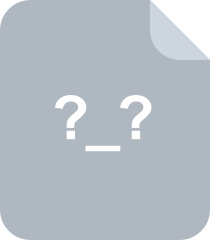
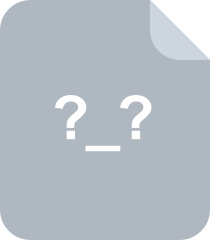
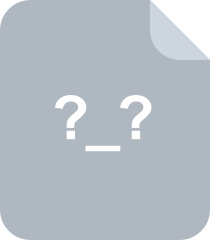
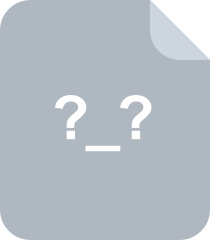
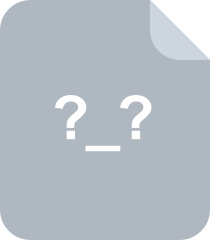
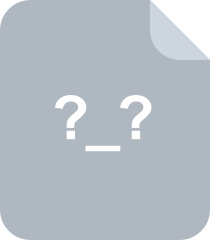
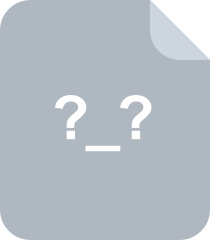
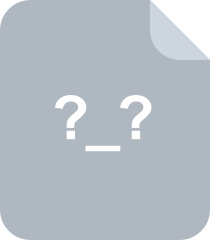
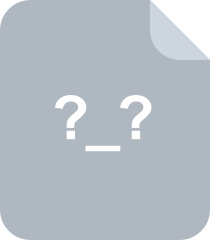
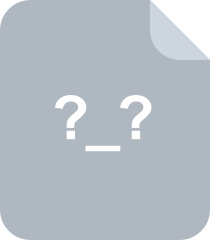
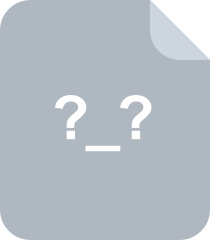
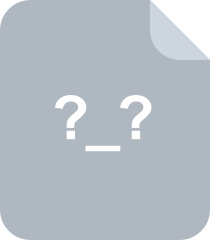
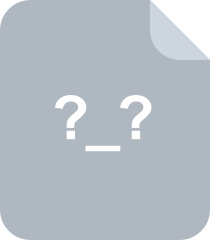
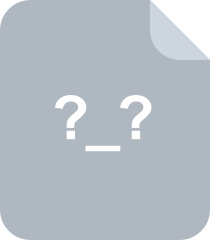
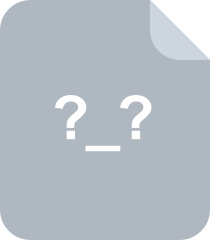
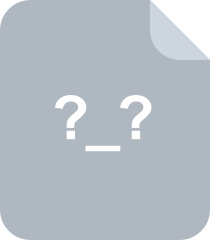
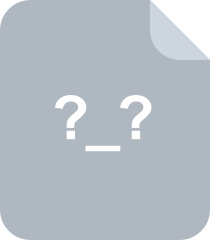
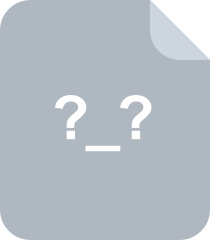
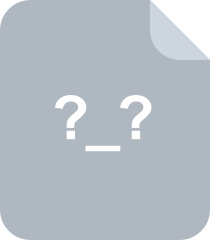
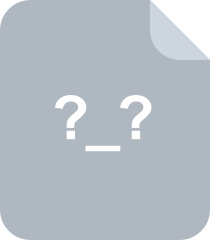
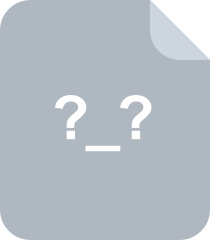
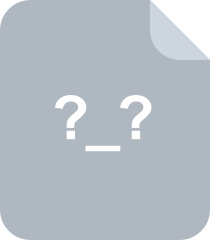
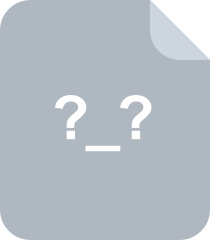
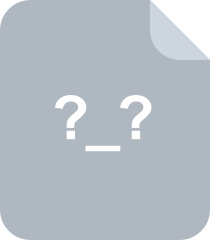
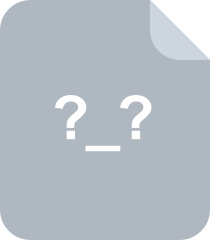
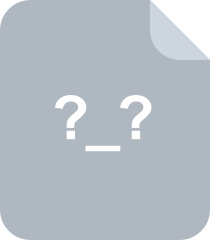
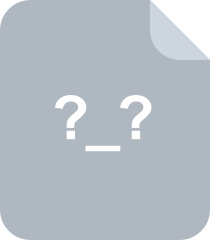
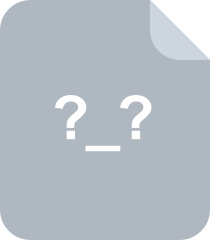
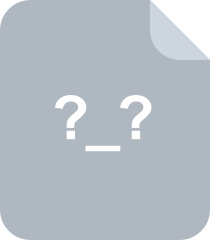
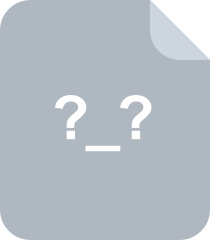
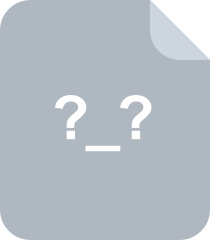
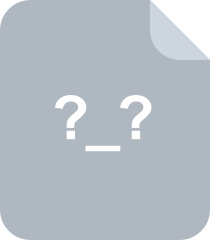
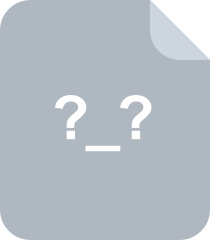
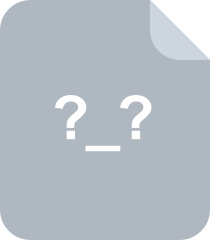
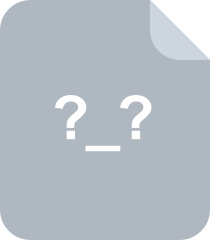
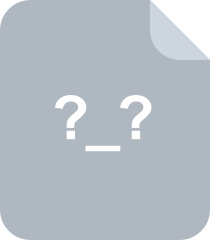
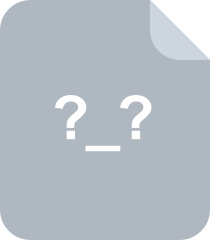
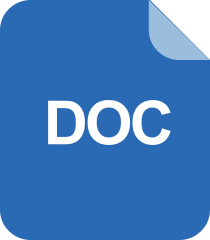
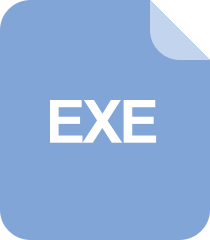
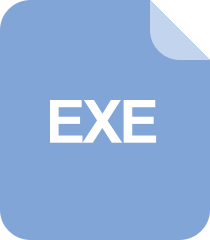
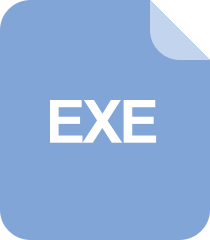
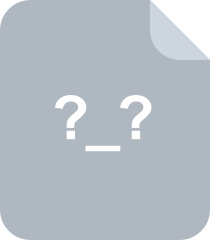
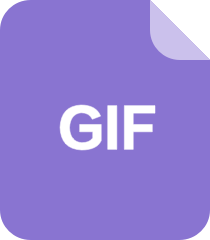
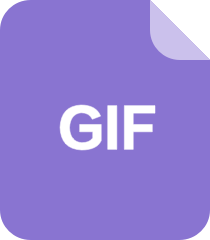
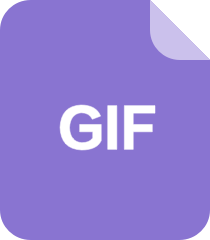
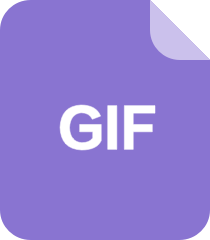
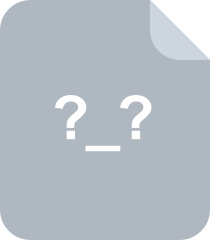
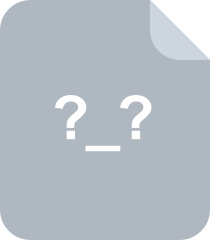
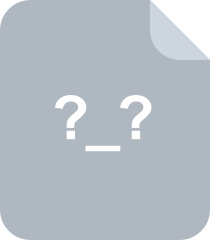
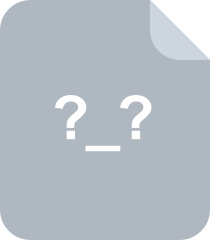
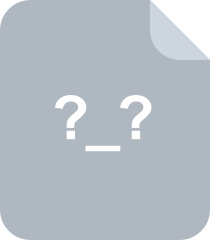
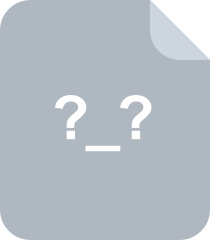
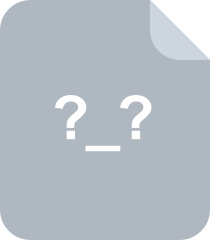
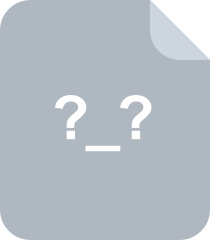
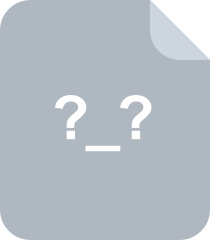
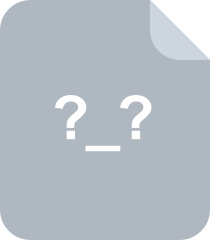
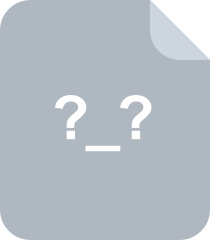
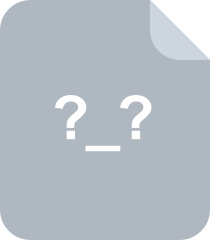
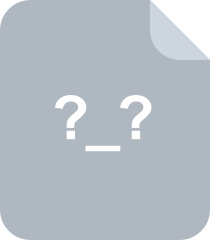
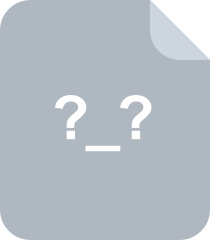
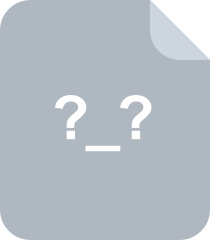
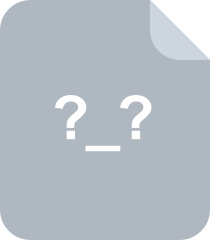
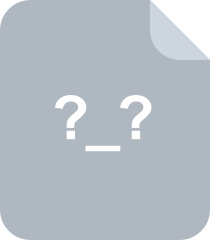
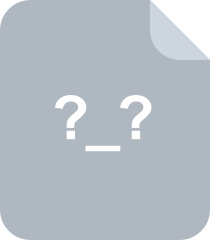
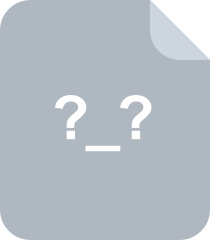
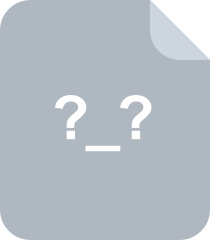
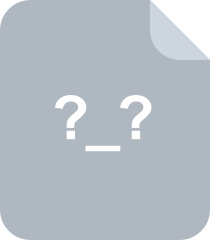
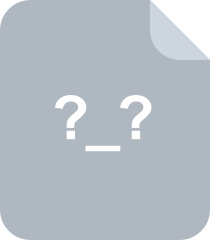
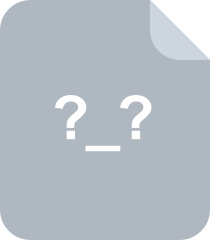
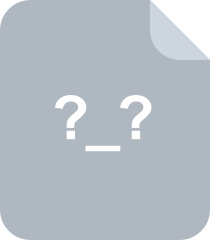
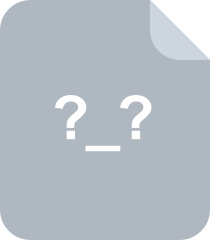
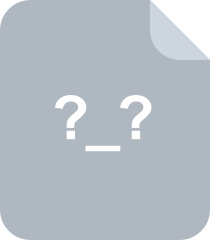
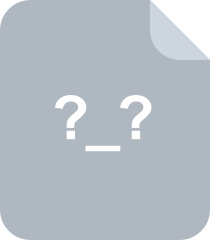
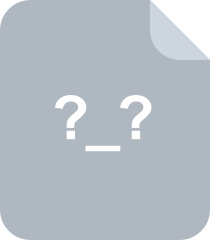
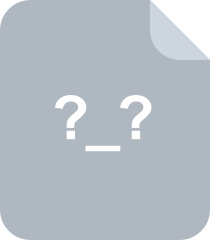
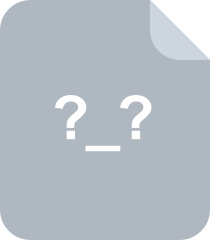
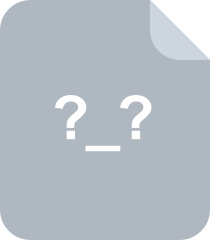
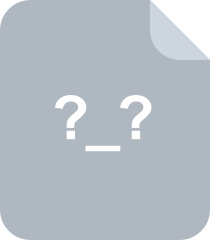
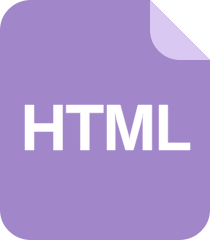
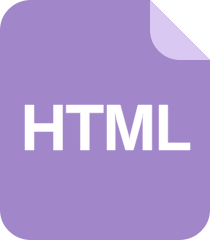
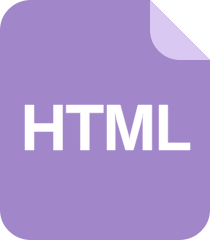
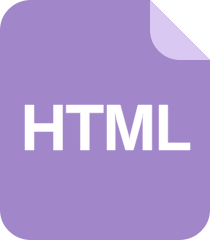
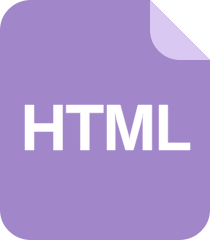
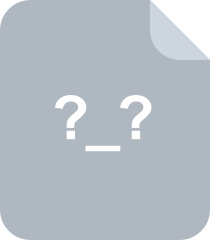
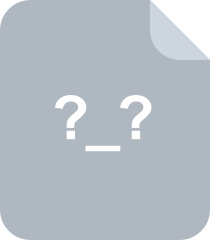
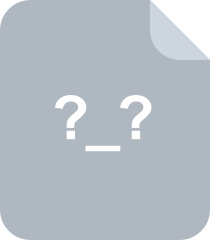
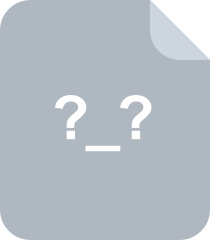
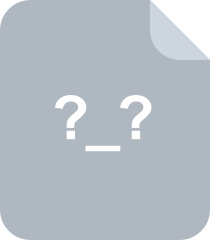
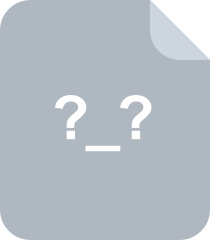
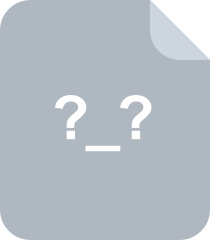
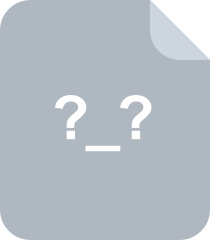
共 1159 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
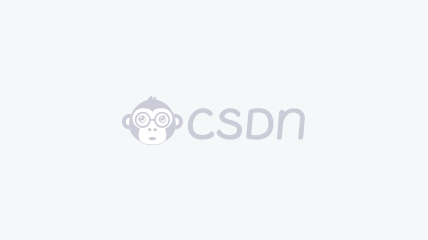

阿齐Archie
- 粉丝: 3w+
- 资源: 2473
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

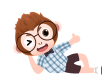
最新资源
- 基于python和协同过滤算法的电影推荐系统
- 国际象棋棋子检测3-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- Python毕业设计基于知识图谱的电影推荐系统源码(完整项目代码)
- 基于C++的简易图书管理系统(含exe可执行文件)
- 使用python爬取数据并采用Django搭建系统的前后台,使用Spark进行数据处理并进行电影推荐项目源码
- 商城蛋糕数据库sql源码
- 基于Spark的电影推荐系统源码(毕设)
- NET综合解决工具,windows平台必备
- ZZU 面向对象Java实验报告
- 2024年秋学季-C#课程的信息系统大作业winform
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


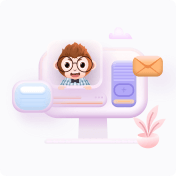
安全验证
文档复制为VIP权益,开通VIP直接复制
