%% Housekeeping
clearvars;
clc;
%% Define w and d
w = 1.8;
d = 0.45;
C = w/(2*d);
%% Get all possible nominal current combinations
I_nominal = [90 92 94 96 98 100 102 104 106 108 110].*10^(-9);
combinations = combvec(I_nominal, I_nominal, I_nominal, I_nominal); % Generate current combinations
%% Get alpha and beta for each combination of four nominal currents.
alpha_nom = zeros(1,length(combinations(1,:))); % initialize a vector to store alpha
alpha_currents_nom = zeros(4,length(combinations(1,:))); % vector to hold current combinations that yield particular alphas
beta_nom = zeros(1,length(combinations(1,:))); % initialize a vector to store beta
beta_currents_nom = zeros(4,length(combinations(1,:))); % vector to hold current combinations that yield particular betas
for i = 1:length(combinations(1,:)) % loop through the current combinations
currents = combinations(:,i); % store the currents for a particular combination
I_a = currents(1);
I_b = currents(2);
I_c = currents(3);
I_d = currents(4);
alpha_nom(i) = atand(C*((I_c + I_d - (I_a + I_b))/(I_c + I_d + I_a + I_b))); % calculate/store alpha
alpha_currents_nom(:,i) = [I_a; I_b; I_c; I_d]; % store the current combination
beta_nom(i) = atand(C*((I_b + I_c - (I_a + I_d))/(I_b + I_c + I_a + I_d))); % calculate/store beta
beta_currents_nom(:,i) = alpha_currents_nom(:,i); % store the current combination
end
%% Get medians from measured cal data
% Channel A
I_A_filename = "GRIDS_DIONE_CALIBRATION_12132022_1_A_90-110nA_L.xlsx";
I_A_sheet = "IDM Data (arrays)";
I_A_matrix = readmatrix(I_A_filename, "Sheet", I_A_sheet);
I_A = I_A_matrix(:,6);
I_A_90med = median(I_A(1:1288));
I_A_92med = median(I_A(1509:2632));
I_A_94med = median(I_A(2874:3860));
I_A_96med = median(I_A(4077:5036));
I_A_98med = median(I_A(5221:6685));
I_A_100med = median(I_A(6984:8039));
I_A_102med = median(I_A(8377:9188));
I_A_104med = median(I_A(9369:10200));
I_A_106med = median(I_A(10410:11344));
I_A_108med = median(I_A(11513:12324));
I_A_110med = median(I_A(12545:13320));
I_A_med = [I_A_90med I_A_92med I_A_94med I_A_96med I_A_98med I_A_100med I_A_102med I_A_104med I_A_106med I_A_108med I_A_110med];
% Channel B
I_B_filename = "GRIDS_DIONE_CALIBRATION_12142022_2_B_90-110nA_L.xlsx";
I_B_sheet = "IDM Data (arrays)";
I_B_matrix = readmatrix(I_B_filename, "Sheet", I_B_sheet);
I_B = I_B_matrix(:,7);
I_B_90med = median(I_B(1:1076));
I_B_92med = median(I_B(1206:2124));
I_B_94med = median(I_B(2309:3104));
I_B_96med = median(I_B(3309:4056));
I_B_98med = median(I_B(4317:5036));
I_B_100med = median(I_B(5297:5964));
I_B_102med = median(I_B(6205:7040));
I_B_104med = median(I_B(7197:7888));
I_B_106med = median(I_B(8065:8804));
I_B_108med = median(I_B(9007:9653));
I_B_110med = median(I_B(9853:10480));
I_B_med = [I_B_90med I_B_92med I_B_94med I_B_96med I_B_98med I_B_100med I_B_102med I_B_104med I_B_106med I_B_108med I_B_110med];
% Channel C
I_C_filename = "GRIDS_DIONE_CALIBRATION_12082022_3_C_90nA-110nA_L.xlsx";
I_C_sheet = "IDM Data (arrays)";
I_C_matrix = readmatrix(I_C_filename, "Sheet", I_C_sheet);
I_C = I_C_matrix(:,8);
I_C_90med = median(I_C(65:1208));
I_C_92med = median(I_C(1229:2336));
I_C_94med = median(I_C(2345:3466));
I_C_96med = median(I_C(3489:4504));
I_C_98med = median(I_C(4527:5366));
I_C_100med = median(I_C(5397:6448));
I_C_102med = median(I_C(6469:7416));
I_C_104med = median(I_C(7425:8731));
I_C_106med = median(I_C(8749:9800));
I_C_108med = median(I_C(9817:10788));
I_C_110med = median(I_C(10789:11160));
I_C_med = [I_C_90med I_C_92med I_C_94med I_C_96med I_C_98med I_C_100med I_C_102med I_C_104med I_C_106med I_C_108med I_C_110med];
% Channel D
I_D_filename = "GRIDS_DIONE_CALIBRATION_12142022_4_D_90-110nA_L.xlsx";
I_D_sheet = "IDM Data (arrays)";
I_D_matrix = readmatrix(I_D_filename, "Sheet", I_D_sheet);
I_D = I_D_matrix(:,9);
I_D_90med = median(I_D(33:740));
I_D_92med = median(I_D(929:1564));
I_D_94med = median(I_D(1805:2696));
I_D_96med = median(I_D(2906:3548));
I_D_98med = median(I_D(3769:4484));
I_D_100med = median(I_D(4713:5468));
I_D_102med = median(I_D(5661:6300));
I_D_104med = median(I_D(6473:7140));
I_D_106med = median(I_D(7361:7996));
I_D_108med = median(I_D(8188:8772));
I_D_110med = median(I_D(8961:9600));
I_D_med = [I_D_90med I_D_92med I_D_94med I_D_96med I_D_98med I_D_100med I_D_102med I_D_104med I_D_106med I_D_108med I_D_110med];
%% Replace current combination values with appropriate measured currents
combinations_measured = combinations;
% Collector A
for i = 1:length(combinations(1,:))
if combinations(1,i) - 90/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(1);
elseif combinations(1,i) - 92/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(2);
elseif combinations(1,i) - 94/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(3);
elseif combinations(1,i) - 96/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(4);
elseif combinations(1,i) - 98/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(5);
elseif combinations(1,i) - 100/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(6);
elseif combinations(1,i) - 102/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(7);
elseif combinations(1,i) - 104/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(8);
elseif combinations(1,i) - 106/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(9);
elseif combinations(1,i) - 108/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(10);
elseif combinations(1,i) - 110/10^9 <= 10^(-20)
combinations_measured(1,i) = I_A_med(11);
end
end
% Collector B
for i = 1:length(combinations(2,:))
if combinations(2,i) - 90/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(1);
elseif combinations(2,i) - 92/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(2);
elseif combinations(2,i) - 94/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(3);
elseif combinations(2,i) - 96/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(4);
elseif combinations(2,i) - 98/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(5);
elseif combinations(2,i) - 100/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(6);
elseif combinations(2,i) - 102/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(7);
elseif combinations(2,i) - 104/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(8);
elseif combinations(2,i) - 106/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(9);
elseif combinations(2,i) - 108/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(10);
elseif combinations(2,i) - 110/10^9 <= 10^(-20)
combinations_measured(2,i) = I_B_med(11);
end
end
% Collector C
for i = 1:length(combinations(3,:))
if combinations(3,i) - 90/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(1);
elseif combinations(3,i) - 92/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(2);
elseif combinations(3,i) - 94/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(3);
elseif combinations(3,i) - 96/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(4);
elseif combinations(3,i) - 98/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(5);
elseif combinations(3,i) - 100/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(6);
elseif combinations(3,i) - 102/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(7);
elseif combinations(3,i) - 104/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(8);
elseif combinations(3,i) - 106/10^9 <= 10^(-20)
combinations_measured(3,i) = I_C_med(9);
elseif combinations(3,i) - 10

Matlab科研辅导帮
- 粉丝: 3w+
- 资源: 7819
最新资源
- 数据资产目录管理平台建设方案(46页).pptx
- 数字化架构的演进和治理.pptx
- 智能制造与卓越运营业务体系设计(86页).pptx
- 基于S7-200 PLC与组态王技术的港口码头装卸料小车控制系统设计与应用,基于S7-200 PLC与组态王技术的港口码头装卸料小车智能控制系统研究与应用,No.942 基于S7-200 PLC和组态
- 《CNN 将行走模式识别为一种非自愿的识别行为》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《从轮廓步态图像中提取步态能量图像特征,然后使用树模型对这些特征进行分类》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《关于 Gait Recognition 的精彩内容集合步态识别》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《基于轮廓分析的步态识别进行人类身份识别》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《基于卷积神经网络的步态识别》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《基于时间部分的步态识》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- Deepseek写的贪吃蛇小游戏
- 《基于深度学习模型的步态识别系统》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《基于深度学习的步态识别系统》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- DeepSeek 资源,Deepseek-r1复现科普与资源汇总,Deepseek-r1复现科普与资源汇总,目前复现主要针对于R1蒸馏模型(领域模型或者自有SFT模型)和R1-Zero的复现
- 《来自毫米波雷达数据的基于步态的用户识别》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
- 《基于时间部分的步态识别模型》(毕业设计,源码,教程)简单部署即可运行。功能完善、操作简单,适合毕设或课程设计.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


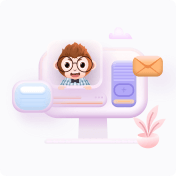