% Binary Distillation Column from
%
% Hahn, J. and T.F. Edgar, An improved method for nonlinear model reduction using balancing of
% empirical gramians, Computers and Chemical Engineering, 26, pp. 1379-1397, (2002)
%
% t -- time (not used)
% x -- mole fraction of A at each stage
% xdot -- state derivatives
function xdot = distill(t,x)
global u
% Inputs (1):
% Reflux Ratio (L/D)
rr=u;
% States (32):
% x(1) - Reflux Drum Liquid Mole Fraction of Component A
% x(2) - Tray 1 - Liquid Mole Fraction of Component A
% .
% .
% .
% x(17) - Tray 16 - Liquid Mole Fraction of Component A (Feed Location)
% .
% .
% .
% x(31) - Tray 30 - Liquid Mole Fraction of Component A
% x(32) - Reboiler Liquid Mole Fraction of Component A
% Parameters
% Feed Flowrate (mol/min)
Feed = 24.0/60.0;
% Mole Fraction of Feed
x_Feed = 0.5;
% Distillate Flowrate (mol/min)
D=0.5*Feed;
% Flowrate of the Liquid in the Rectification Section (mol/min)
L=rr*D;
% Vapor Flowrate in the Column (mol/min)
V=L+D;
% Flowrate of the Liquid in the Stripping Section (mol/min)
FL=Feed+L;
% Relative Volatility = (yA/xA)/(yB/xB) = KA/KB = alpha(A,B)
vol=1.6;
% Total Molar Holdup in the Condenser
atray=0.25;
% Total Molar Holdup on each Tray
acond=0.5;
% Total Molar Holdup in the Reboiler
areb=1.0;
% Vapor Mole Fractions of Component A
% From the equilibrium assumption and mole balances
% 1) vol = (yA/xA) / (yB/xB)
% 2) xA + xB = 1
% 3) yA + yB = 1
y(1)=x(1)*vol/(1+(vol-1)*x(1));
y(2)=x(2)*vol/(1+(vol-1)*x(2));
y(3)=x(3)*vol/(1+(vol-1)*x(3));
y(4)=x(4)*vol/(1+(vol-1)*x(4));
y(5)=x(5)*vol/(1+(vol-1)*x(5));
y(6)=x(6)*vol/(1+(vol-1)*x(6));
y(7)=x(7)*vol/(1+(vol-1)*x(7));
y(8)=x(8)*vol/(1+(vol-1)*x(8));
y(9)=x(9)*vol/(1+(vol-1)*x(9));
y(10)=x(10)*vol/(1+(vol-1)*x(10));
y(11)=x(11)*vol/(1+(vol-1)*x(11));
y(12)=x(12)*vol/(1+(vol-1)*x(12));
y(13)=x(13)*vol/(1+(vol-1)*x(13));
y(14)=x(14)*vol/(1+(vol-1)*x(14));
y(15)=x(15)*vol/(1+(vol-1)*x(15));
y(16)=x(16)*vol/(1+(vol-1)*x(16));
y(17)=x(17)*vol/(1+(vol-1)*x(17));
y(18)=x(18)*vol/(1+(vol-1)*x(18));
y(19)=x(19)*vol/(1+(vol-1)*x(19));
y(20)=x(20)*vol/(1+(vol-1)*x(20));
y(21)=x(21)*vol/(1+(vol-1)*x(21));
y(22)=x(22)*vol/(1+(vol-1)*x(22));
y(23)=x(23)*vol/(1+(vol-1)*x(23));
y(24)=x(24)*vol/(1+(vol-1)*x(24));
y(25)=x(25)*vol/(1+(vol-1)*x(25));
y(26)=x(26)*vol/(1+(vol-1)*x(26));
y(27)=x(27)*vol/(1+(vol-1)*x(27));
y(28)=x(28)*vol/(1+(vol-1)*x(28));
y(29)=x(29)*vol/(1+(vol-1)*x(29));
y(30)=x(30)*vol/(1+(vol-1)*x(30));
y(31)=x(31)*vol/(1+(vol-1)*x(31));
y(32)=x(32)*vol/(1+(vol-1)*x(32));
% Compute xdot
xdot(1) = 1/acond*V*(y(2)-x(1));
xdot(2) = 1/atray*(L*(x(1)-x(2))-V*(y(2)-y(3)));
xdot(3) = 1/atray*(L*(x(2)-x(3))-V*(y(3)-y(4)));
xdot(4) = 1/atray*(L*(x(3)-x(4))-V*(y(4)-y(5)));
xdot(5) = 1/atray*(L*(x(4)-x(5))-V*(y(5)-y(6)));
xdot(6) = 1/atray*(L*(x(5)-x(6))-V*(y(6)-y(7)));
xdot(7) = 1/atray*(L*(x(6)-x(7))-V*(y(7)-y(8)));
xdot(8) = 1/atray*(L*(x(7)-x(8))-V*(y(8)-y(9)));
xdot(9) = 1/atray*(L*(x(8)-x(9))-V*(y(9)-y(10)));
xdot(10) = 1/atray*(L*(x(9)-x(10))-V*(y(10)-y(11)));
xdot(11) = 1/atray*(L*(x(10)-x(11))-V*(y(11)-y(12)));
xdot(12) = 1/atray*(L*(x(11)-x(12))-V*(y(12)-y(13)));
xdot(13) = 1/atray*(L*(x(12)-x(13))-V*(y(13)-y(14)));
xdot(14) = 1/atray*(L*(x(13)-x(14))-V*(y(14)-y(15)));
xdot(15) = 1/atray*(L*(x(14)-x(15))-V*(y(15)-y(16)));
xdot(16) = 1/atray*(L*(x(15)-x(16))-V*(y(16)-y(17)));
xdot(17) = 1/atray*(Feed*x_Feed+L*x(16)-FL*x(17)-V*(y(17)-y(18)));
xdot(18) = 1/atray*(FL*(x(17)-x(18))-V*(y(18)-y(19)));
xdot(19) = 1/atray*(FL*(x(18)-x(19))-V*(y(19)-y(20)));
xdot(20) = 1/atray*(FL*(x(19)-x(20))-V*(y(20)-y(21)));
xdot(21) = 1/atray*(FL*(x(20)-x(21))-V*(y(21)-y(22)));
xdot(22) = 1/atray*(FL*(x(21)-x(22))-V*(y(22)-y(23)));
xdot(23) = 1/atray*(FL*(x(22)-x(23))-V*(y(23)-y(24)));
xdot(24) = 1/atray*(FL*(x(23)-x(24))-V*(y(24)-y(25)));
xdot(25) = 1/atray*(FL*(x(24)-x(25))-V*(y(25)-y(26)));
xdot(26) = 1/atray*(FL*(x(25)-x(26))-V*(y(26)-y(27)));
xdot(27) = 1/atray*(FL*(x(26)-x(27))-V*(y(27)-y(28)));
xdot(28) = 1/atray*(FL*(x(27)-x(28))-V*(y(28)-y(29)));
xdot(29) = 1/atray*(FL*(x(28)-x(29))-V*(y(29)-y(30)));
xdot(30) = 1/atray*(FL*(x(29)-x(30))-V*(y(30)-y(31)));
xdot(31) = 1/atray*(FL*(x(30)-x(31))-V*(y(31)-y(32)));
xdot(32) = 1/areb*(FL*x(31)-(Feed-D)*x(32)-V*y(32));
xdot = xdot'; % xdot must be a column vector

天天Matlab代码科研顾问
- 粉丝: 3w+
- 资源: 2775
最新资源
- 基于java+ssm+mysql的校园二手物品交易平台开题报告.docx
- 疫苗预约小程序ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 英语互助小程序springboot-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 学习资料库小程序设计ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 基于java+ssm+mysql的校园兼职管理系统开题报告.docx
- 运动健康小程序SpringBoot-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- MATLAB代码:含电动汽车参与园区综合能源系统优化调度模型 关键词:电动汽车 改进粒子群 综合能源 优化调度 园区 参考文档:加好友获取 仿真平台:MATLAB 主要内容:代码主要做的是一个含有
- 基于YOLO的摔倒检测研究
- 使用python卷积神经网络做交通信号灯识别的自动驾驶,并在unity进行3d可视化 另有全景图像配准算法源码,改进yolov5目标检测识别源码,体感交互切水果等 计算机硕士
- 内容分发网络(CDN)的工作原理、应用场景与发展前景解析
- 基于STM32F407开发调试,Modbus TCP服务器源程序 采用LWIP网络通讯库,外部PHY采用LAN8720 使用 modbus poll工具调试通过 该工程可直接作为模板开发 源码
- MATLAB代码:基于蒙特卡洛抽样的电动汽车充电负荷计算 关键词:电动汽车 蒙特卡洛模拟 抽样 充放电负荷 参考文档:《主动配电网多源协同运行优化研究-乔珊》完全复现 仿真平台:MATLAB 优
- 《CDN技术分享》PDF
- simplorer与Maxwell电机联合仿真,包含搭建好的Simplorer电机场路耦合主电路与控制算法(矢量控制SVPWM),包含电路与算法搭建的详细教程视频 仿真文件可复制,可将教程中的电机模
- 四旋翼无人机PID模型,飞行器本体模型,位置控制,姿态控制,控制分配和电机控制
- 改进人工大猩猩优化算法(CGTO,2021年提出的优化算法较新),自己研究的改进方法,没有任何成果产出,有没有一款较新的智能算法且效果好一些的呢,那么今天它来了,CGTO通过改进勘探阶段公式,混沌映射
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


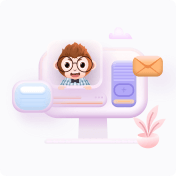