function varargout = MainFrame(varargin)
% MAINFRAME MATLAB code for MainFrame.fig
% MAINFRAME, by itself, creates a new MAINFRAME or raises the existing
% singleton*.
%
% H = MAINFRAME returns the handle to a new MAINFRAME or the handle to
% the existing singleton*.
%
% MAINFRAME('CALLBACK',hObject,eventData,handles,...) calls the local
% function named CALLBACK in MAINFRAME.M with the given input arguments.
%
% MAINFRAME('Property','Value',...) creates a new MAINFRAME or raises the
% existing singleton*. Starting from the left, property value pairs are
% applied to the GUI before MainFrame_OpeningFcn gets called. An
% unrecognized property name or invalid value makes property application
% stop. All inputs are passed to MainFrame_OpeningFcn via varargin.
%
% *See GUI Options on GUIDE's Tools menu. Choose "GUI allows only one
% instance to run (singleton)".
%
% See also: GUIDE, GUIDATA, GUIHANDLES
% Edit the above text to modify the response to help MainFrame
% Last Modified by GUIDE v2.5 30-Apr-2017 06:35:20
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @MainFrame_OpeningFcn, ...
'gui_OutputFcn', @MainFrame_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before MainFrame is made visible.
function MainFrame_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to MainFrame (see VARARGIN)
% Choose default command line output for MainFrame
handles.output = hObject;
clc;
handles.videoFilePath = 0;
handles.videoInfo = 0;
handles.videoImgList = 0;
handles.videoStop = 1;
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes MainFrame wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = MainFrame_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --- Executes on button press in pushbuttonPlay.
function pushbuttonPlay_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonPlay (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 播放按钮
set(handles.pushbuttonPause, 'Enable', 'On');
set(handles.pushbuttonPause, 'tag', 'pushbuttonPause', 'String', '暂停');
set(handles.sliderVideoPlay, 'Max', handles.videoInfo.NumberOfFrames, 'Min', 0, 'Value', 1);
set(handles.editSlider, 'String', sprintf('%d/%d', 0, handles.videoInfo.NumberOfFrames));
% 循环载入视频帧图像并显示
for i = 1 : handles.videoInfo.NumberOfFrames
waitfor(handles.pushbuttonPause,'tag','pushbuttonPause');
I = imread(fullfile(pwd, sprintf('video_images\\%03d.jpg', i)));
try
imshow(I, [], 'Parent', handles.axesVideo);
% 设置进度条
set(handles.sliderVideoPlay, 'Value', i);
set(handles.editSlider, 'String', sprintf('%d/%d', i, handles.videoInfo.NumberOfFrames));
catch
return;
end
drawnow;
end
% 控制暂停按钮
set(handles.pushbuttonPause, 'Enable', 'Off');
% --- Executes on button press in pushbuttonOpenVideoFile.
function pushbuttonOpenVideoFile_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonOpenVideoFile (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 打开视频文件按钮
videoFilePath = OpenVideoFile();
if videoFilePath == 0
return;
end
set(handles.editVideoFilePath, 'String', videoFilePath);
msgbox('打开视频文件成功!', '提示信息');
handles.videoFilePath = videoFilePath;
guidata(hObject, handles);
% --- Executes on button press in pushbuttonImageList.
function pushbuttonImageList_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonImageList (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 获取图像序列按钮
if handles.videoInfo == 0
msgbox('请先获取视频信息', '提示信息');
return;
end
Video2Images(handles.videoFilePath);
msgbox('获取图像序列成功!', '提示信息');
% --- Executes on button press in pushbuttonStopCheck.
function pushbuttonStopCheck_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonStopCheck (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 视频分析
[s, sx, sy] = MotionAnalysis();
handles.s = s;
handles.sx = sx;
handles.sy = sy;
% Update handles structure
guidata(hObject, handles);
% --- Executes on button press in pushbuttonPause.
function pushbuttonPause_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonPause (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 暂停按钮
% 获取响应标记
str = get(handles.pushbuttonPause, 'tag');
if strcmp(str, 'pushbuttonPause') == 1
set(handles.pushbuttonPause, 'tag', 'pushbuttonContinue', 'String', '继续');
pause on;
else
set(handles.pushbuttonPause, 'tag', 'pushbuttonPause', 'String', '暂停');
pause off;
end
% --- Executes on button press in pushbuttonStop.
function pushbuttonStop_Callback(hObject, eventdata, handles)
% hObject handle to pushbuttonStop (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% 停止按钮
axes(handles.axesVideo); cla; axis on; box on;
set(gca, 'XTick', [], 'YTick', [], ...
'XTickLabel', '', 'YTickLabel', '', 'Color', [0.7020 0.7804 1.0000]);
set(handles.editSlider, 'String', '0/0');
set(handles.sliderVideoPlay, 'Value', 0);
set(handles.pushbuttonPause, 'tag', 'pushbuttonContinue', 'String', '继续');
set(handles.pushbuttonPause, 'Enable', 'Off');
set(handles.pushbuttonPause, 'String', '暂停');
function editFrameNum_Callback(hObject, eventdata, handles)
% hObject handle to editFrameNum (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Hints: get(hObject,'String') returns contents of editFrameNum as text
% str2double(get(hObject,'String')) returns contents of editFrameNum as a double
% --- Executes during object creation, after setting all properties.
function editFrameNum_CreateFcn(hObject, eventdata, handles)
% hObject handle to editFrameNum (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles empty - handles not created until after all CreateFcns called
% Hint: edit controls usually have a white background on Windows.
% See ISPC and COMPUTER.
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function editFrameWidth_Callback(hObject, eventdata, handles)
% hObject handle to editFrameWidth (see GCBO)
% eventdata reserved - to be defined in a future version of MATLA
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
MATLAB是MathWorks公司出品的商业数学软件,用于数据分析、无线通信、深度学习、图像处理与计算机视觉、信号处理、量化金融与风险管理、机器人,控制系统等领域。 【主页资源】 遗传算法、免疫算法、退火算法、粒子群算法、鱼群算法、蚁群算法和神经网络算法等常用智能算法的MATLAB实现,包含TSP、LQR控制器、结合量子算法、多目标优化、粒子群等matlab程序。 MATLAB计算机视觉与深度学习实战项目:直方图优化去雾技术、基于形态学的权重自适应图像去噪、多尺度形态学提取眼前节组织、基于分水岭算法的肺癌分割诊断、基于harris 的角点检测(可以直接用matlab自带的函数)、基于K均值的据类算法分割(算法时间有点久)、 区域生长算法进行肝部肿瘤分割(原始分割精度不高)、matlab编写的图像处理相关算法代码及算法原理等等。
资源推荐
资源详情
资源评论
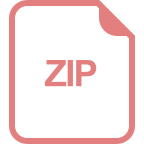
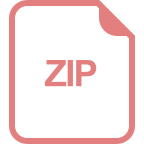
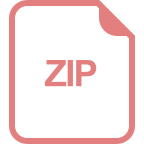
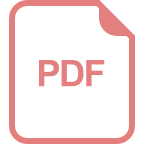
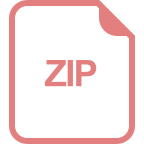
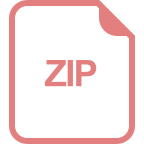
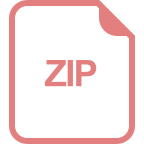
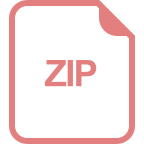
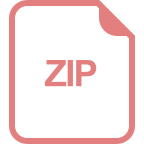
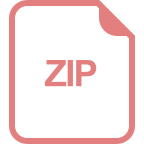
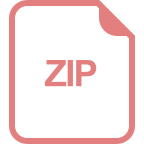
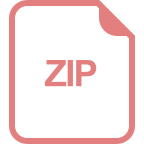
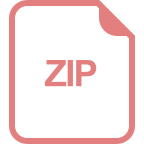
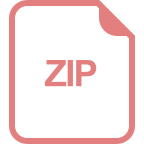
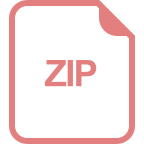
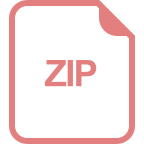
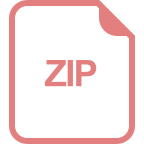
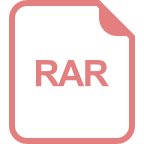
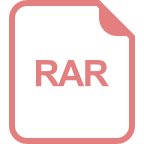
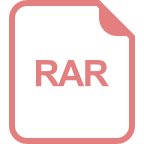
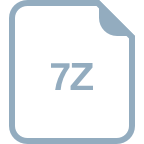
收起资源包目录

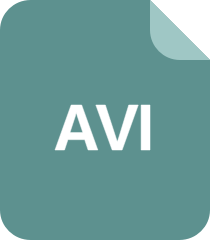
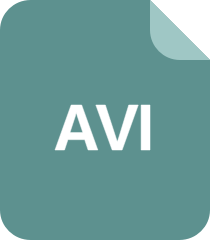
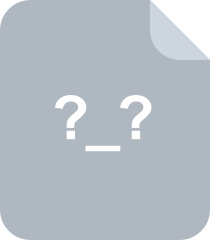
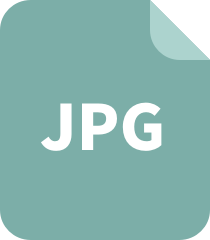
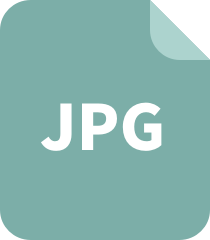
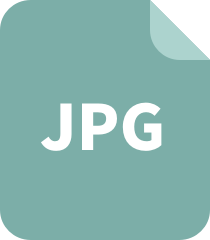
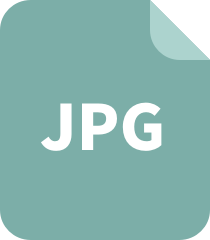
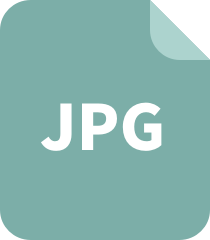
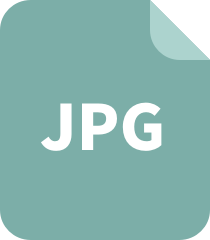
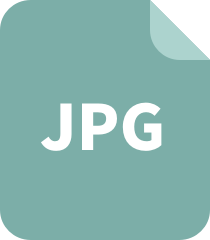
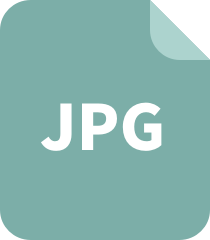
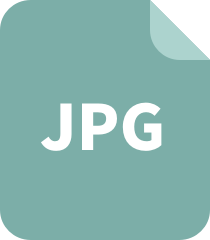
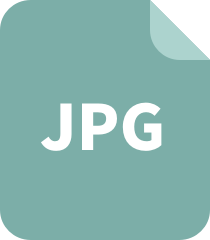
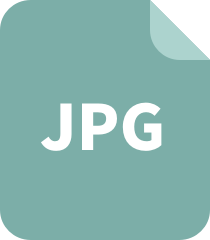
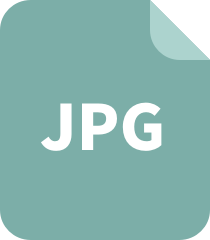
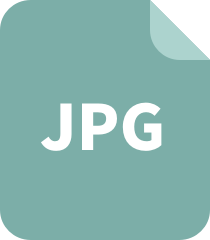
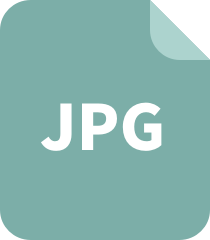
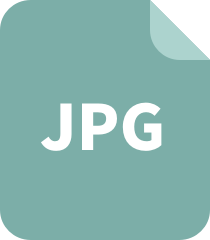
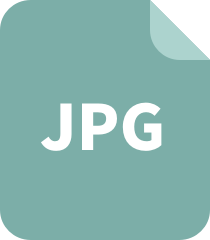
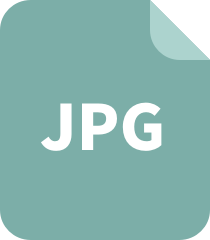
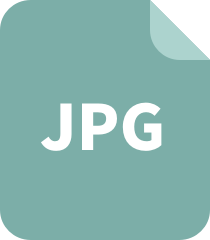
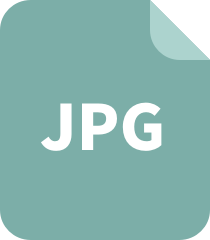
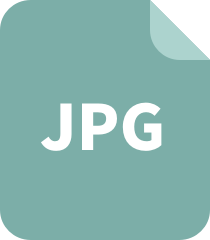
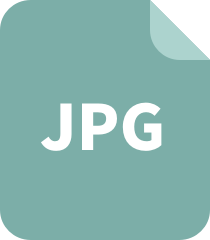
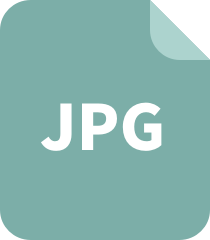
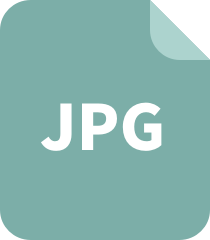
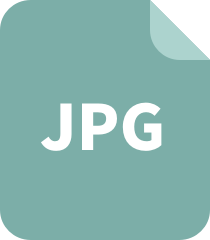
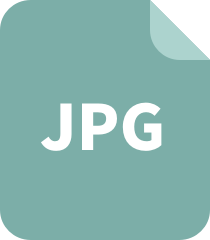
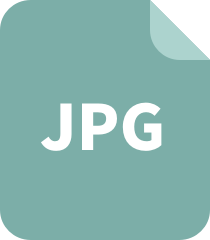
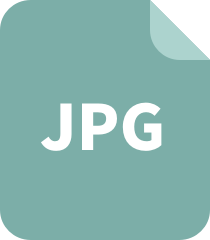
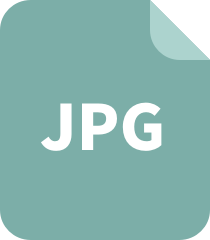
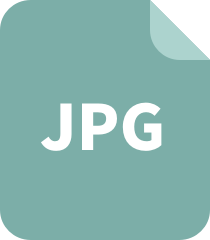
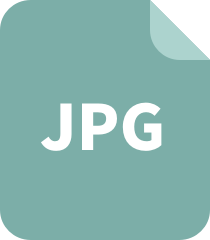
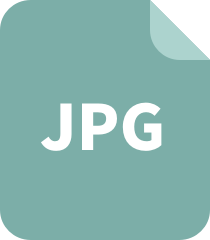
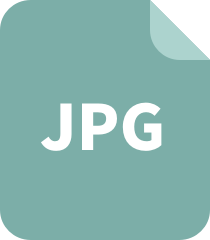
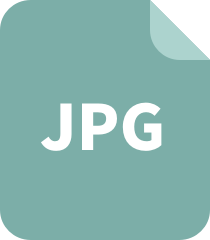
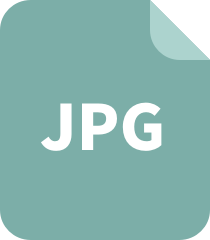
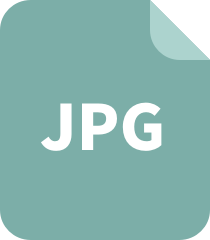
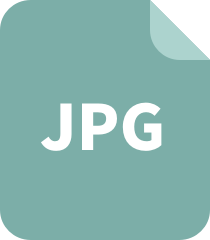
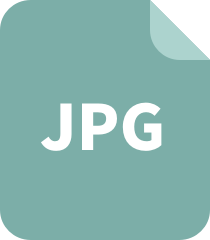
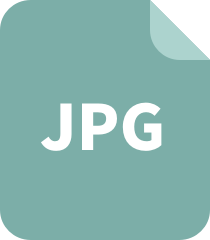
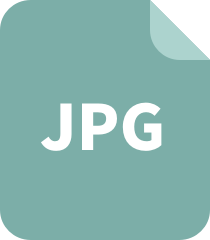
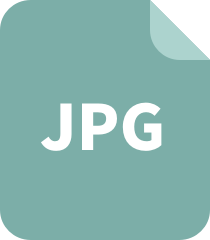
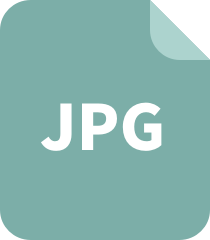
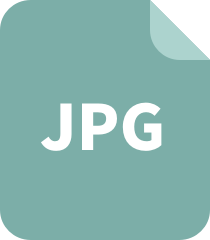
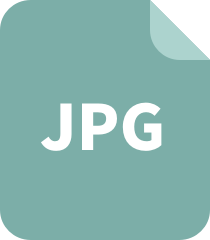
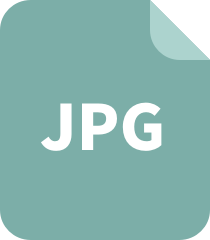
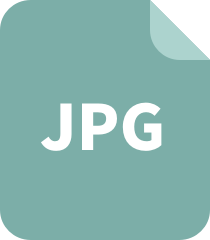
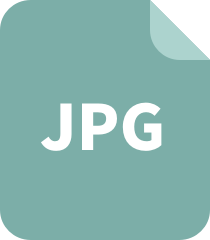
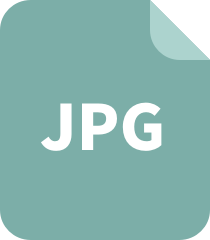
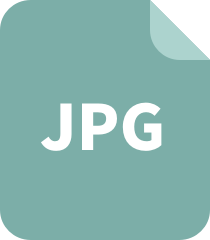
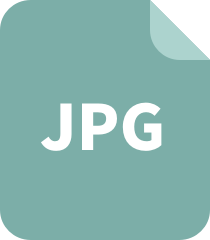
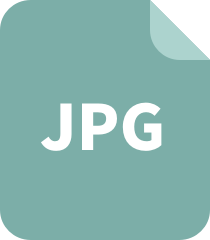
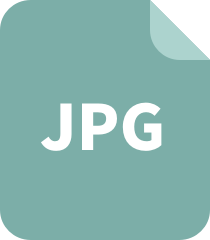
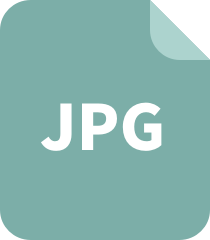
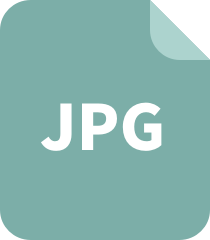
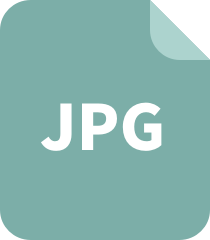
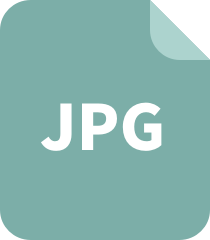
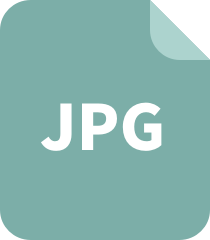
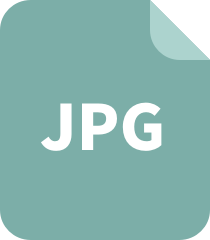
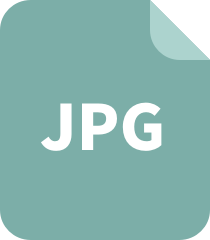
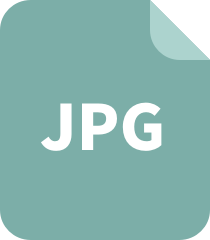
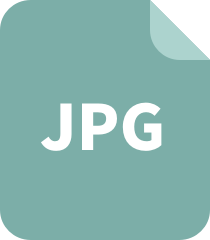
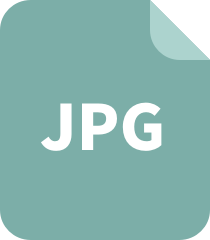
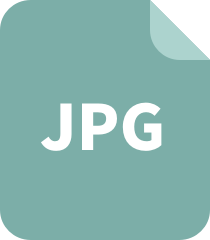
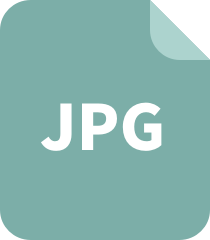
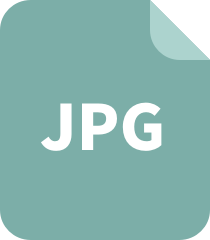
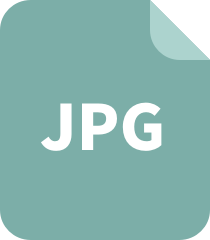
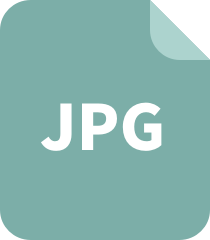
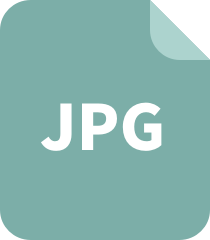
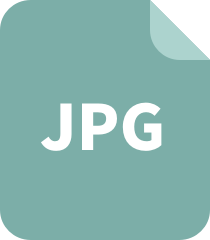
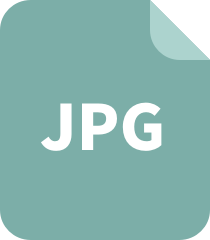
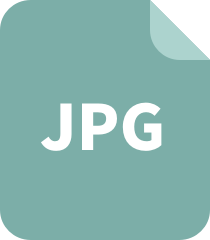
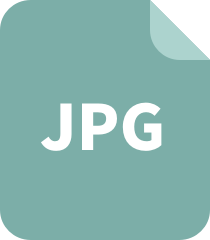
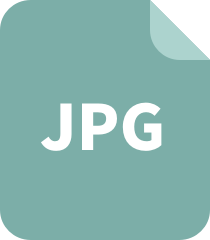
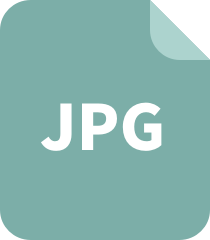
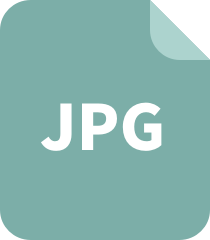
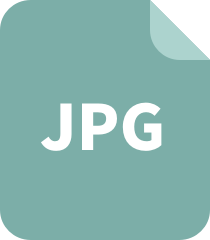
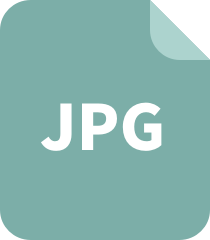
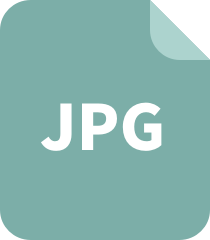
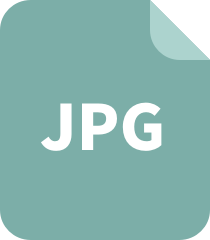
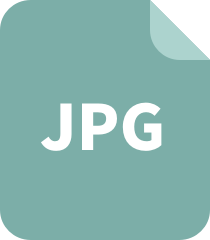
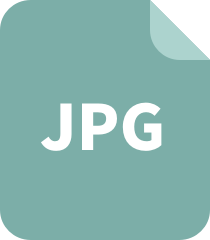
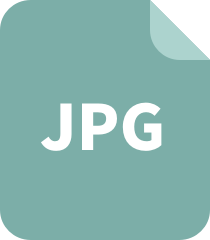
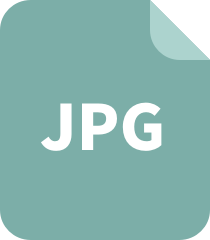
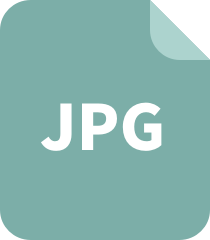
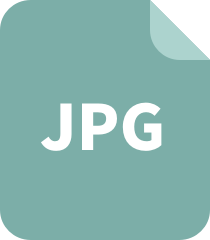
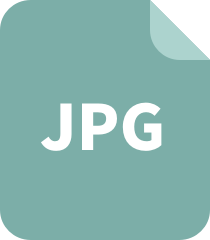
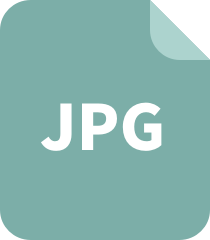
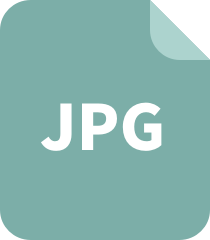
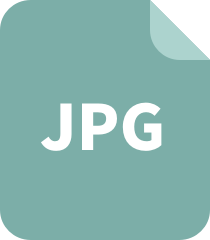
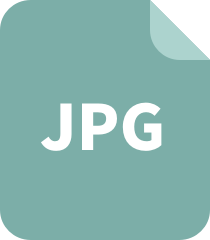
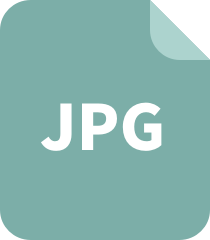
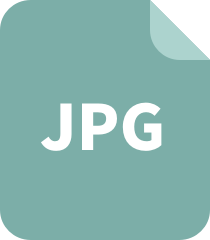
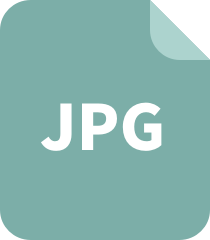
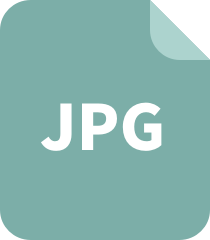
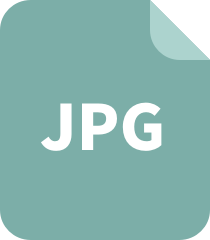
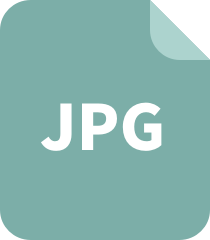
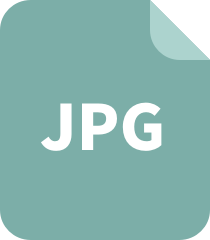
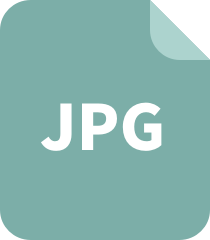
共 127 条
- 1
- 2
资源评论
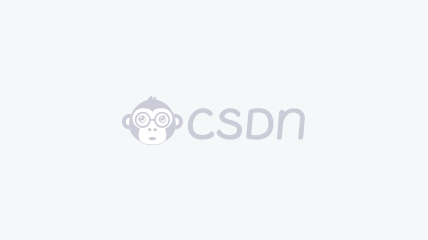

枫蜜柚子茶
- 粉丝: 7542
- 资源: 5155
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

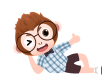
安全验证
文档复制为VIP权益,开通VIP直接复制
