package org.lyh.common.service;
import com.baomidou.mybatisplus.core.metadata.TableInfo;
import com.baomidou.mybatisplus.core.metadata.TableInfoHelper;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.IService;
import org.lyh.common.domain.LyhChildrenData;
import org.lyh.common.domain.LyhChildrenDataGroup;
import org.lyh.common.domain.LyhQueryParameter;
import org.lyh.common.util.LyhLoggerUtils;
import org.lyh.common.util.LyhObjUtils;
import org.lyh.common.util.LyhSpringContextUtil;
import java.io.Serializable;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public interface LyhBaseService<T> extends IService<T> {
boolean saveOrUpdate(T entity);
Page<T> queryDataPageList(LyhQueryParameter queryParameter);
boolean removeByIds(T entity);
boolean removeById(Serializable pk);
List<T> list(T entity);
T getDataById(T entity);
boolean remove(T entity);
// 保存的操作
default boolean saveOrUpdateBefore(T entity) {
return true;
}
default boolean saveOrUpdateAfter(T entity) {
return true;
}
// 批量保存的操作
default boolean saveOrUpdateBatchBefore(Collection<T> entityList) {
return true;
}
default boolean saveOrUpdateBatchAfter(Collection<T> entityList) {
return true;
}
// 根据主键删除的前后逻辑
default boolean removeBefore(Serializable id) {
return true;
}
default boolean removeAfter(Serializable id) {
return true;
}
// 批量删除的操作
default boolean removeBatchBefore(Collection<? extends Serializable> idList) {
return true;
}
default boolean removeBatchAfter(Collection<? extends Serializable> idList) {
return true;
}
//根据实体 -> 获取表信息,主键名 -> 获取实体的主键数值
default Object buildIdVal(T queryData) {
Assert.notNull(queryData, "error: can not execute. because queryData is empty!", new Object[0]);
TableInfo tableInfo = TableInfoHelper.getTableInfo(queryData.getClass());
Assert.notNull(tableInfo, "error: can not execute. because can not find cache of TableInfo for entity!", new Object[0]);
String keyProperty = tableInfo.getKeyProperty();
Assert.notEmpty(keyProperty, "error: can not execute. because can not find column for id from entity!", new Object[0]);
Object idVal = tableInfo.getPropertyValue(queryData, tableInfo.getKeyProperty());
return idVal;
}
// 保存子表的操作
default void saveChildrenData(T entity) {
Object id = buildIdVal(entity);
if (LyhObjUtils.isNotEmpty(id)) {
LyhChildrenDataGroup annotation = entity.getClass().getAnnotation(LyhChildrenDataGroup.class);
if (LyhObjUtils.isNotEmpty(annotation)) {
LyhChildrenData[] lyhChildrenData = annotation.childDataList();
for (LyhChildrenData childrenData : lyhChildrenData) {
String masterKey = childrenData.masterKey();
String subKey = childrenData.subKey();
Class entityClazz = childrenData.entityClazz();
String fieldName = childrenData.fieldName();
String saveMethodName = childrenData.saveMethodName();
String serviceClazz = childrenData.serviceClazz();
try {
// 获取子表对应object数据
TableInfo tableInfo = TableInfoHelper.getTableInfo(entity.getClass());
Object propertyValue = tableInfo.getPropertyValue(entity, fieldName);
if (!(propertyValue instanceof Collection)) {
LyhLoggerUtils.error(this.getClass(), "propertyValue not instanceof Collection!");
throw new RuntimeException("propertyValue not instanceof Collection!");
}
List subDataList = (List) propertyValue;
// 将字段进行填充, 拿到masterKey的数值
Object masterKeyValue = tableInfo.getPropertyValue(entity, masterKey);
for(Object obj: subDataList) LyhObjUtils.setProperty(obj, subKey, masterKeyValue);
// 如何调用到 子表的service的bean 来调用定义的"方法名"
Object serviceBean = LyhSpringContextUtil.getBean(serviceClazz);
Method method = serviceBean.getClass().getMethod(saveMethodName, Collection.class);
Object res = method.invoke(serviceBean, subDataList);
if (res instanceof Boolean){
Boolean f = (Boolean)res;
if (!f) LyhLoggerUtils.error(this.getClass(), "保存子表数据未0条,注意正确性!");
}
} catch (NoSuchMethodException |InvocationTargetException | IllegalAccessException e) {
LyhLoggerUtils.error(this.getClass(), e.getMessage());
throw new RuntimeException(e);
}
}
}
}
}
// 查询子表的操作
default void queryChildrenData(T entity){
LyhChildrenDataGroup annotation = entity.getClass().getAnnotation(LyhChildrenDataGroup.class);
if (LyhObjUtils.isNotEmpty(annotation)){
LyhChildrenData[] lyhChildrenData = annotation.childDataList();
for (LyhChildrenData childrenData: lyhChildrenData){
String masterKey = childrenData.masterKey();
String subKey = childrenData.subKey();
String serviceClazz = childrenData.serviceClazz();
String queryMethodName = childrenData.queryMethodName();
String fieldName = childrenData.fieldName();
try {
Object masterKeyValue = LyhObjUtils.getProperty(entity, masterKey);
Class entityClazz = childrenData.entityClazz();
Object subEntity = entityClazz.newInstance();
LyhObjUtils.setProperty(subEntity, subKey, masterKeyValue);
Object serviceBean = LyhSpringContextUtil.getBean(serviceClazz);
Method method = serviceBean.getClass().getMethod(queryMethodName, Object.class);
Object subData = method.invoke(serviceBean, subEntity);
LyhObjUtils.setProperty(entity, fieldName, subData);
}catch (NoSuchMethodException | InvocationTargetException | IllegalAccessException |
InstantiationException e){
LyhLoggerUtils.error(this.getClass(), e.getMessage());
throw new RuntimeException(e);
}
}
}
}
// 查询子表的操作
default void queryChildrenData(List<T> dataList){
if (LyhObjUtils.isNotEmpty(dataList)){
T enclass = dataList.get(0);
LyhChildrenDataGroup annotation = enclass.getClass().getAnnotation(LyhChildrenDataGroup.class);
if (LyhObjUtils.isNotEmpty(annotation)){
LyhChildrenData[] lyhChildrenData = annotation.childDataList();
for (LyhChildrenData childrenData: lyhChildrenData){
Iterator<T> iterator = dataList.iterator();
while (iterator.hasNext()){
T entity = iterator.next();
String masterKey = childrenData.masterKey();
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该APP为基于Java和Vue框架开发的水稻病虫害检测应用程序,源码共计436个文件,涵盖97个CSS样式文件、96个Java类文件、52个Vue组件文件、51个JavaScript脚本文件、28个FTL模板文件、20个PNG图像文件、18个XML配置文件、15个JPEG图像文件、6个JSON数据文件以及6个JPG图像文件。此项目为作者的个人硕士毕业设计作品,旨在提供一个便捷的水稻病虫害检测工具。
资源推荐
资源详情
资源评论
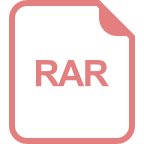
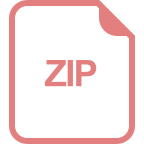
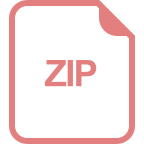
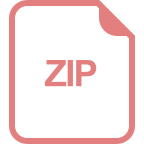
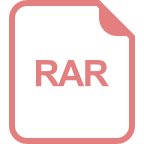
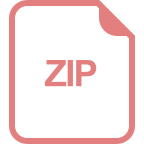
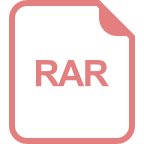
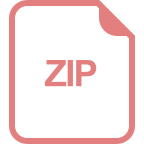
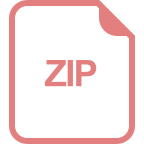
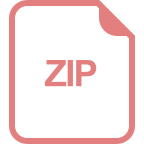
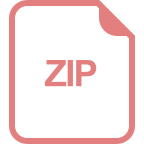
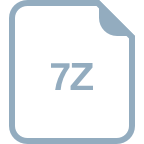
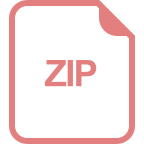
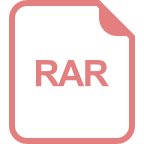
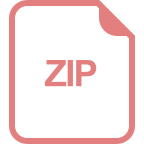
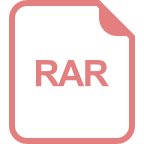
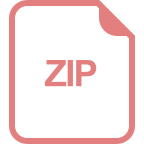
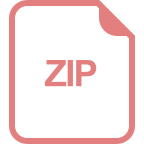
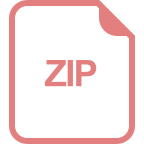
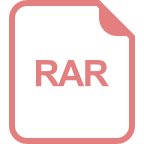
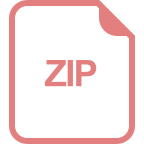
收起资源包目录

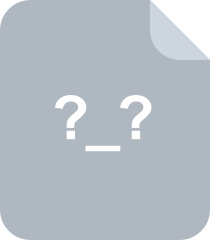
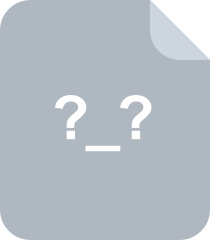
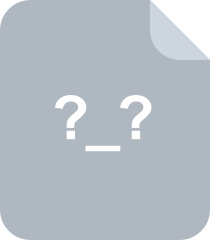
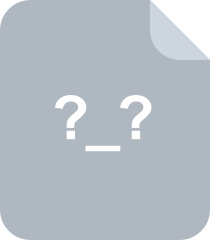
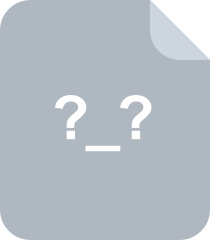
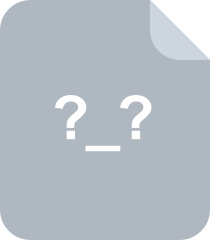
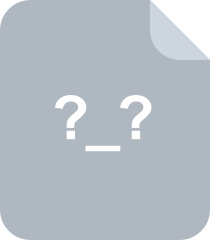
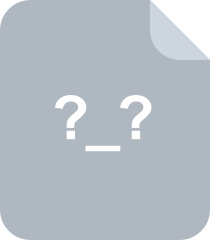
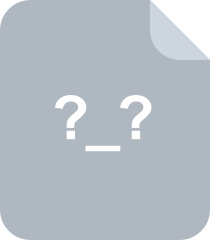
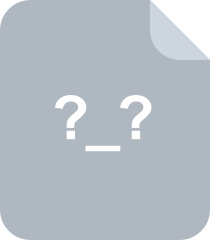
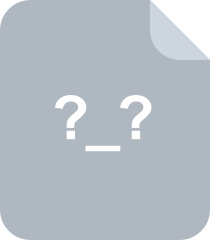
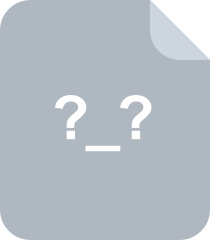
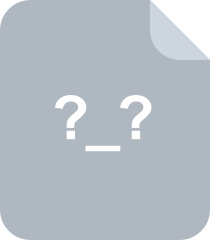
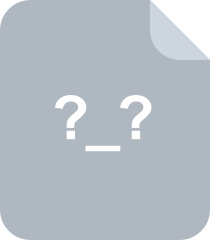
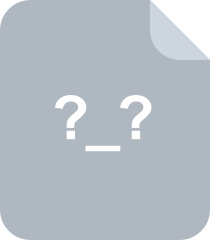
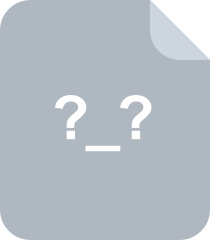
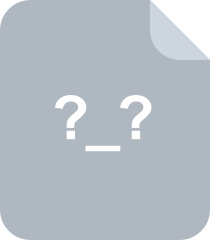
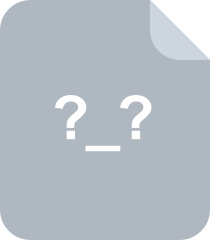
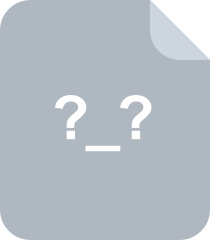
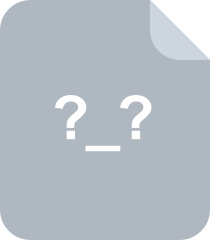
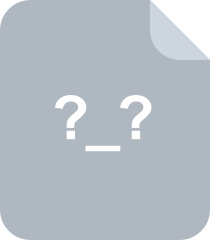
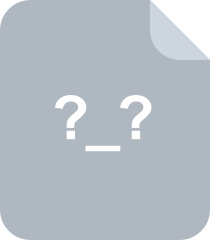
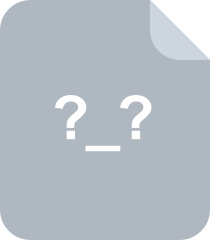
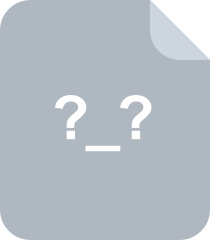
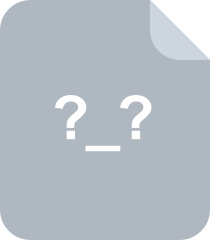
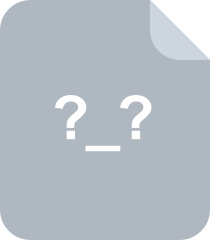
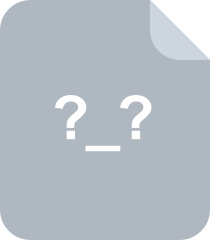
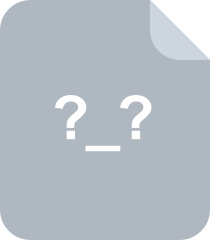
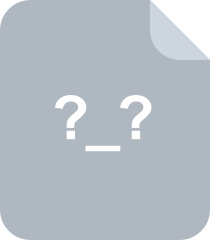
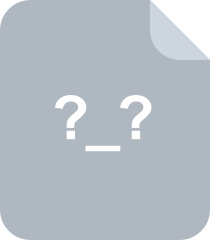
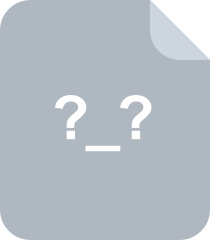
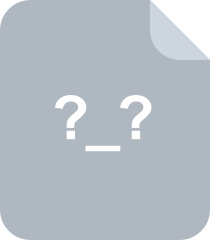
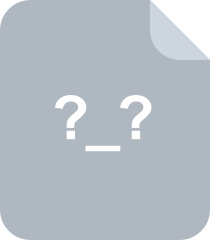
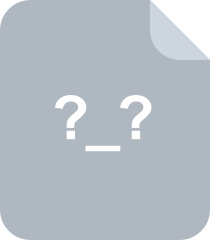
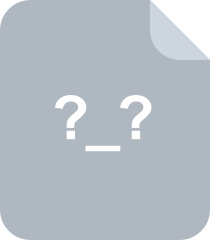
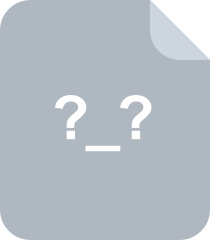
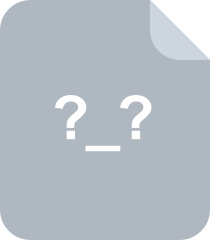
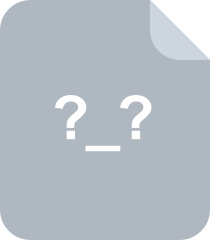
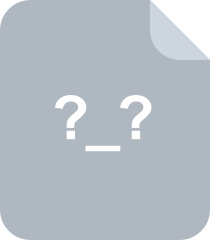
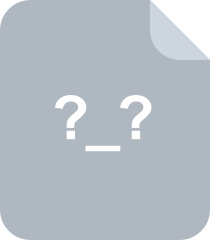
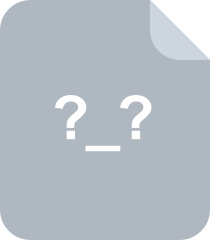
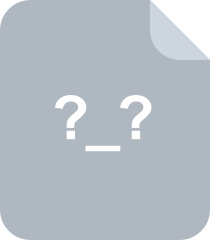
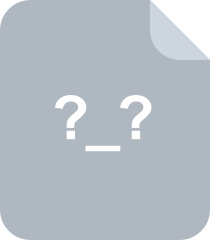
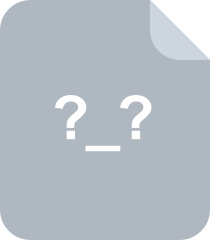
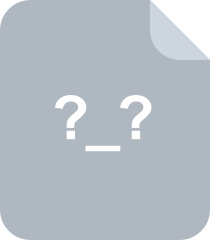
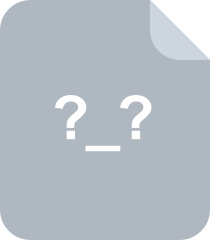
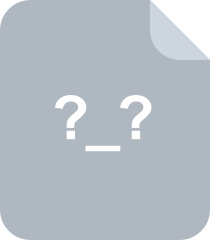
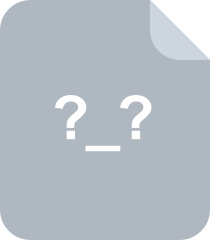
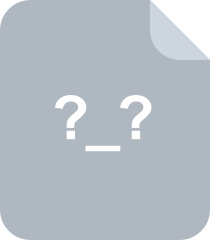
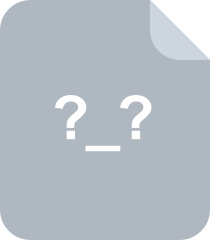
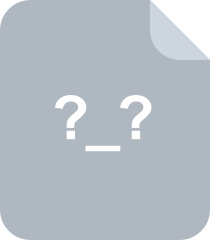
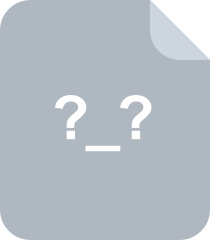
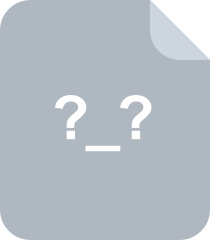
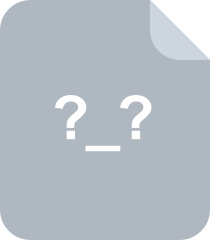
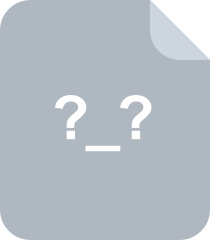
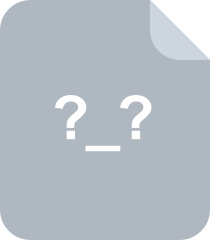
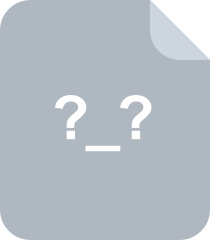
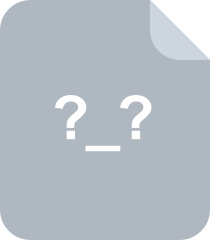
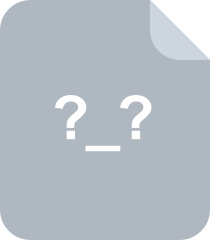
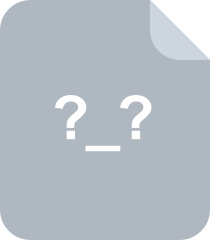
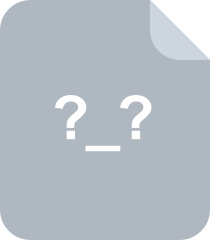
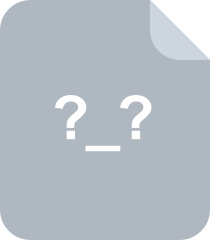
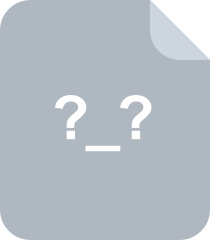
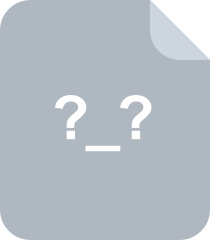
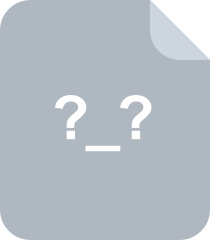
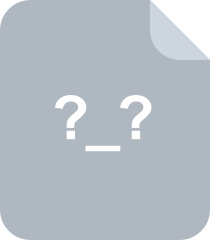
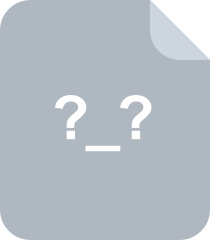
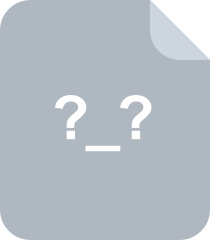
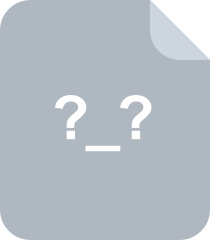
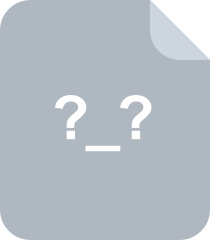
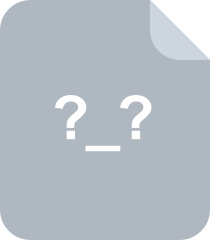
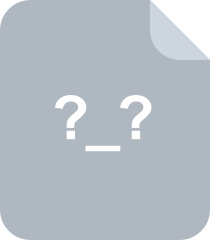
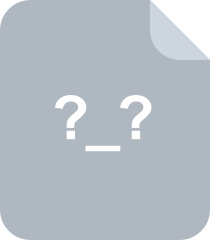
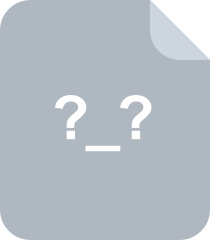
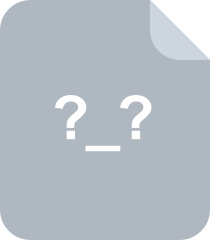
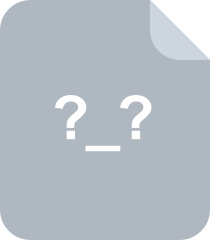
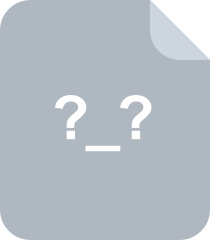
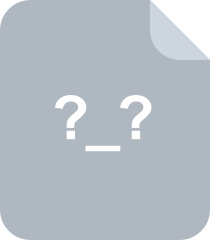
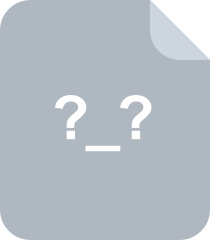
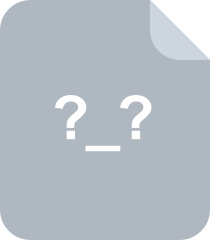
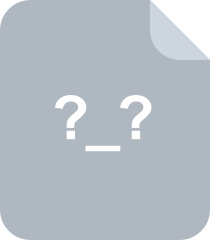
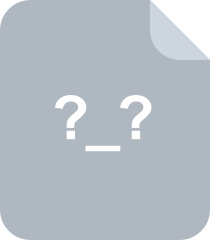
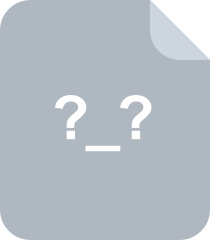
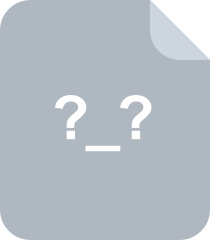
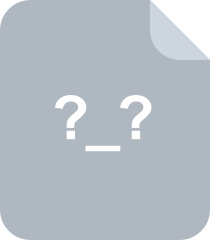
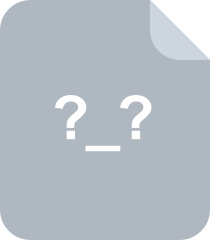
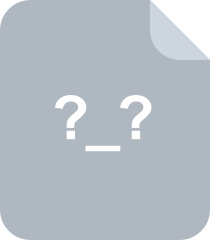
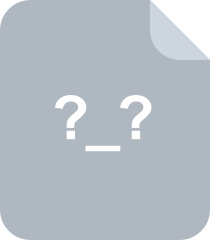
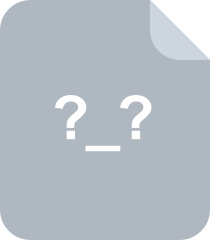
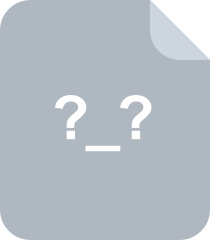
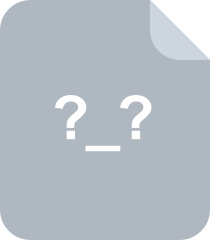
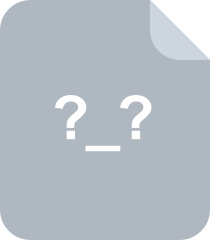
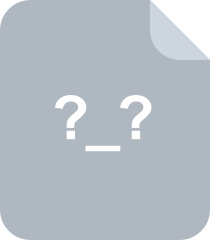
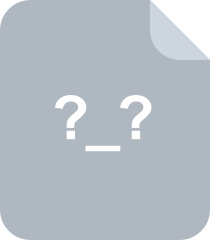
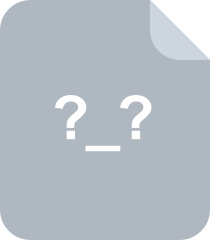
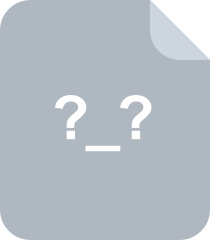
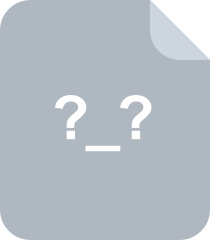
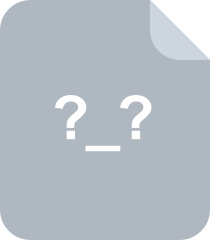
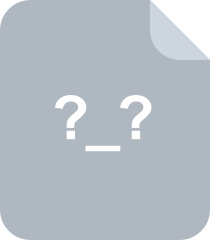
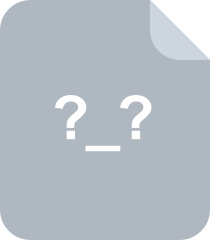
共 436 条
- 1
- 2
- 3
- 4
- 5
资源评论
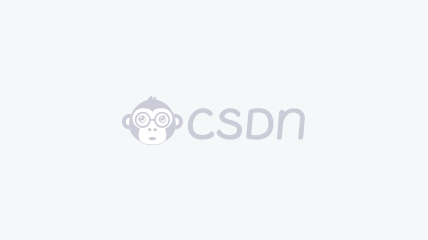

lsx202406
- 粉丝: 2434
- 资源: 5585
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

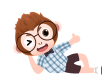
最新资源
- 日志文件:日志概念、LogBack日志技术的概述、使用、logback.xml配置文件详解
- 基于python使用Drl来解决多智能体卸载问题+源码(期末作业&课程设计&项目开发)
- 科学计算领域中的Fortran语言基础知识与应用
- 4.健身房预约课程-微信小程序.zip
- 小乌龟键盘控制源码111111
- 电赛2023年本科组电子电路设计比赛指南与任务解析
- Delphi 12 控件之dspack For Delphi 10.2 - 视频播放组件包e963a-main.zip
- delphi 12 控件之FB4D – The OpenSource Cross-Platform Library for FirebaseFB4D-master.zip
- Rust语言入门与进阶教程
- delphi 12 控件之Delphi开发的微信电脑版登录工具ec617-main.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


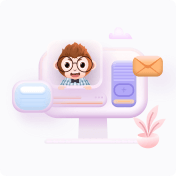
安全验证
文档复制为VIP权益,开通VIP直接复制
