function [channel_estimate] = LTE_channel_estimator(LTE_params,ChanMod, UE, rx_ref_symbols, RefSym, RefMapping, perfect_channel, sigma_n2, y_tx_assembled_genie, y_rx_assembled)
% LTE channel estimator - to filter the output of the transmitter.
% [chan_output] = LTE_channel_model(BS_output, SNR)
% Author: Michal Simko, msimko@nt.tuwien.ac.at
% (c) by INTHFT
% www.nt.tuwien.ac.at
%
% input : ChanMod ... struct -> include info about filtering type, channel autocorrelation matrix
% channel_estimation_method ... string -> type of channel estimation
% channel_interpolation_method... string -> type of channel interpolation
% rx_ref_symbols ... [ x ] matrix of recieved reference symbols
% RefSym ... [ x ] matrix of transmitted reference symbols
% RefMapping ... [ x ] logical matrix of positions of reference symbols
% perfect_channel ... [ x ] coefficients of perfect channel
% sigma_n2 ... [1x1] double noise estimate
% output: channel_estimate ... [ x ] matrix of estimate of channel coefficients
%
% date of creation: 2009/04/06
% last changes: 2008/12/12 Simko
% global LTE_params;
%% Channel estimator
channel_estimate = nan(size(perfect_channel));
interpolation = false;
channel_estimation_method = UE.channel_estimation_method;
channel_interpolation_method = UE.channel_interpolation_method;
switch ChanMod.filtering
case 'BlockFading'
switch channel_estimation_method
case 'corrMMSE'
[position_freq,position_time] = find(RefMapping(:,:,1));
pilots_num = length(position_freq);
H_ls_matrix = nan(pilots_num/2,ChanMod.nRX,ChanMod.nTX);
for tt = 1:ChanMod.nTX
for rr = 1:ChanMod.nRX
H_ls_one_channel = rx_ref_symbols(:,:,tt,rr)./RefSym(:,:,tt);
[position_freq,position_time] = find(RefMapping(:,:,tt)); %positons of reference symbols
if(tt>2)
%position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
[notused reference_mapping] = sort(position_freq); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,length(position_freq),2);
H_ls_one_channel(:,2) = [];
else
position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
[notused reference_mapping] = sort(position(:,1)); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,length(position_freq)/2,2);
end
H_ls_one_channel = mean(H_ls_one_channel,2);
H_ls_matrix(:,rr,tt) = H_ls_one_channel(reference_mapping);
end
end
H_ls_vector = H_ls_matrix(:);
channel_autocorrelation = UE.channel_autocorrelation_matrix;
antenna_correlation = kron(squeeze(ChanMod.corrRX(1,:,:)) , squeeze(ChanMod.corrTX(1,:,:)));
channel_autocorrelation_full = kron(antenna_correlation,channel_autocorrelation);
permutation_matrix = zeros(LTE_params.Ntot*ChanMod.nTX*ChanMod.nRX);
permutation_vector = 1:LTE_params.Ntot*ChanMod.nTX*ChanMod.nRX;
pilot_pos = repmat(notused,ChanMod.nRX*ChanMod.nTX,1) + kron((0:(ChanMod.nRX*ChanMod.nTX-1))',ones(LTE_params.Ntot/3,1)*LTE_params.Ntot);
pilots_num = length(pilot_pos);
permutation_vector(pilot_pos) = [];
permutation_vector = [pilot_pos;permutation_vector'];
for pos_i = 1:LTE_params.Ntot*ChanMod.nRX*ChanMod.nTX
permutation_matrix(pos_i,permutation_vector(pos_i)) = 1;
end
channel_autocorrelation_full_permutated = permutation_matrix * channel_autocorrelation_full * permutation_matrix';
channel_estimate_permutated = channel_autocorrelation_full_permutated(:,1:pilots_num)*inv( channel_autocorrelation_full_permutated(1:pilots_num,1:pilots_num) + sigma_n2*eye(pilots_num)) * H_ls_vector;
channel_estimate_help = permutation_matrix'*channel_estimate_permutated;
channel_estimate_help2 = reshape(channel_estimate_help,LTE_params.Ntot,ChanMod.nRX,ChanMod.nTX);
channel_estimate(:,1,:,:) = channel_estimate_help2;
channel_estimate = repmat(channel_estimate(:,1,:,:),[1,LTE_params.Nsub,1,1]);
end
end
for tt = 1:ChanMod.nTX
for rr = 1:ChanMod.nRX
switch ChanMod.filtering
case 'BlockFading'
switch channel_estimation_method
case 'PERFECT'
channel_estimate = perfect_channel; % perfect channel knowledge
case {'LS', 'GENIE'} %LS for block fading
H_ls_one_channel = rx_ref_symbols(:,:,tt,rr)./RefSym(:,:,tt);
[position_freq,position_time] = find(RefMapping(:,:,tt)); %positons of reference symbols
if(tt>2)
%position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
[notused reference_mapping] = sort(position_freq); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,length(position_freq),2);
H_ls_one_channel(:,2) = [];
else
position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
[notused reference_mapping] = sort(position(:,1)); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,length(position_freq)/2,2);
end
H_ls_one_channel = mean(H_ls_one_channel,2);
H_ls_one_channel = H_ls_one_channel(reference_mapping);
interpolation = true;
case 'MMSE'
%first LS estimate on teh pilots positions
H_ls_one_channel = rx_ref_symbols(:,:,tt,rr)./RefSym(:,:,tt);
[position_freq,position_time] = find(RefMapping(:,:,tt)); %positons of reference symbols
if(tt>2)
%position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
pilots_num = length(position_freq);
[notused reference_mapping] = sort(position_freq); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,pilots_num,2);
H_ls_one_channel(:,2) = [];
else
position = reshape(position_freq,length(position_freq)/2,2); %form the position of reference symbols to appropriate matrix
pilots_num = length(position_freq)/2;
[notused reference_mapping] = sort(position(:,1)); %find the real order of reference symbols in frequency
H_ls_one_channel = reshape(H_ls_one_channel,pilots_num,2);
end
H_ls_one_channel = mean(H_ls_one_channel,2);
H_ls_one_ch
没有合适的资源?快使用搜索试试~ 我知道了~
基于MATLAB实现的lte物理层仿真信道建模,平台搭建 函数功能具有详细描述+使用说明文档.zip
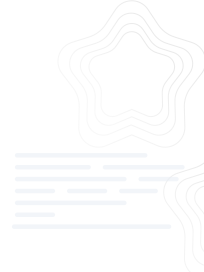
共83个文件
m:33个
mexw32:13个
mexa64:13个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 188 浏览量
2024-05-23
09:42:26
上传
评论 1
收藏 209KB ZIP 举报
温馨提示
CSDN IT狂飙上传的代码均可运行,功能ok的情况下才上传的,直接替换数据即可使用,小白也能轻松上手 【资源说明】 基于MATLAB实现的lte物理层仿真信道建模,平台搭建 函数功能具有详细描述+使用说明文档.zip 1、代码压缩包内容 主函数:main.m; 调用函数:其他m文件;无需运行 运行结果效果图; 2、代码运行版本 Matlab 2020b;若运行有误,根据提示GPT修改;若不会,私信博主(问题描述要详细); 3、运行操作步骤 步骤一:将所有文件放到Matlab的当前文件夹中; 步骤二:双击打开main.m文件; 步骤三:点击运行,等程序运行完得到结果; 4、仿真咨询 如需其他服务,可后台私信博主; 4.1 期刊或参考文献复现 4.2 Matlab程序定制 4.3 科研合作 功率谱估计: 故障诊断分析: 雷达通信:雷达LFM、MIMO、成像、定位、干扰、检测、信号分析、脉冲压缩 滤波估计:SOC估计 目标定位:WSN定位、滤波跟踪、目标定位 生物电信号:肌电信号EMG、脑电信号EEG、心电信号ECG 通信系统:DOA估计、编码译码、变分模态分解、管道泄漏、滤波器、数字信号处理+传输+分析+去噪、数字信号调制、误码率、信号估计、DTMF、信号检测识别融合、LEACH协议、信号检测、水声通信 5、欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
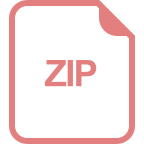
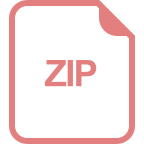
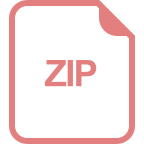
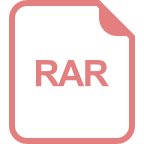
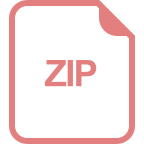
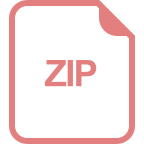
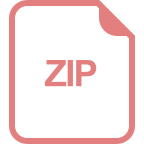
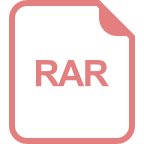
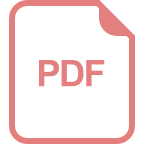
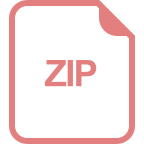
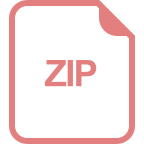
收起资源包目录


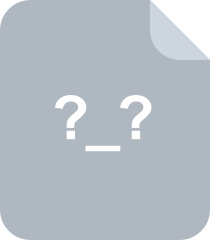
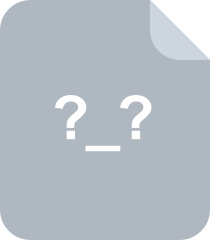
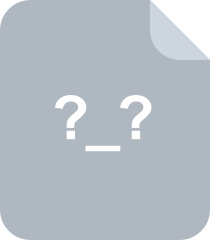
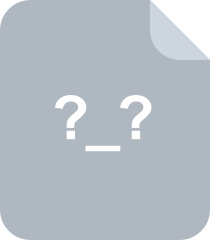
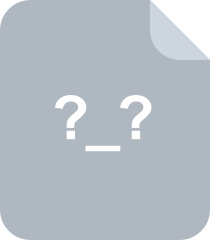
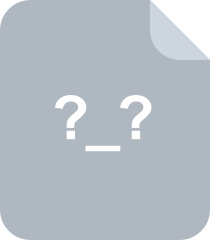
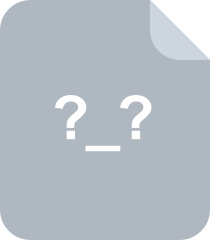
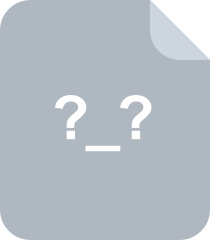
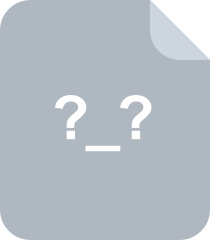
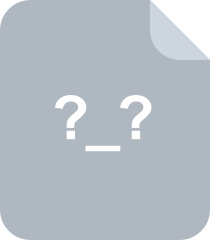
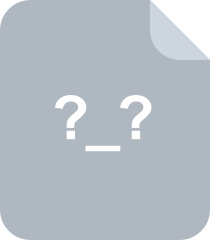
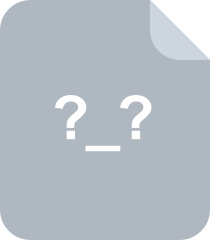
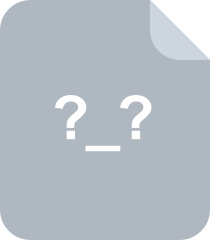
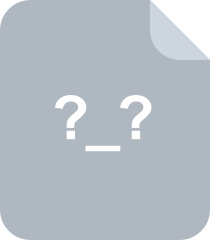
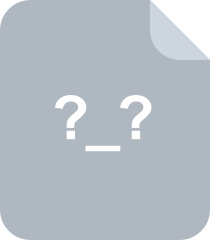
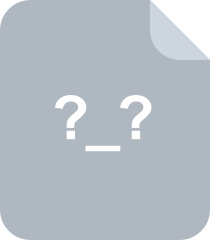
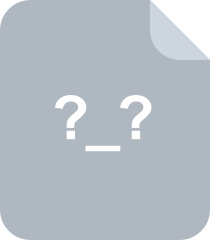
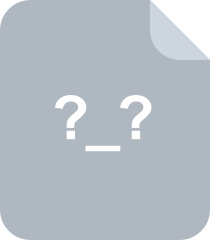
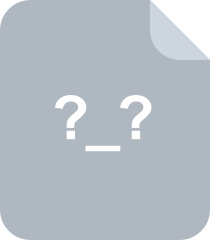
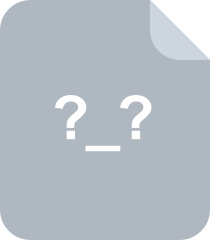
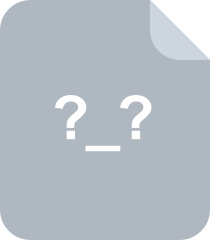
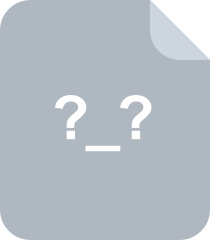
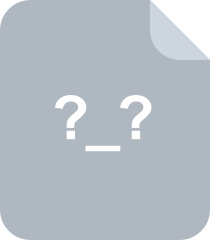
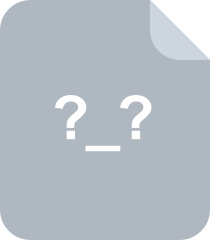
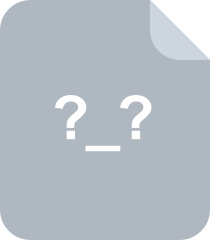
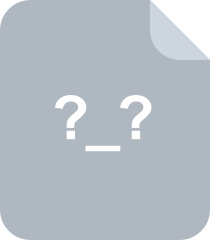
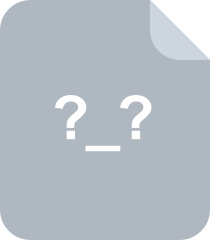
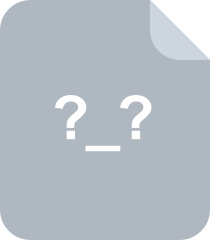
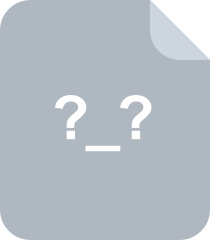
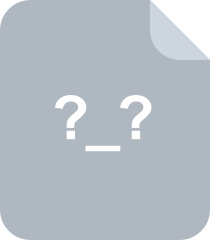
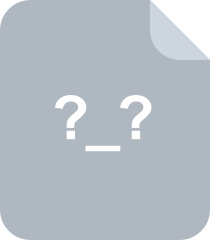
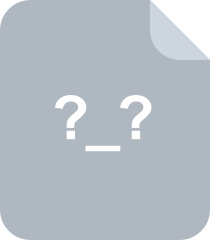
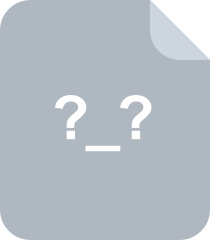
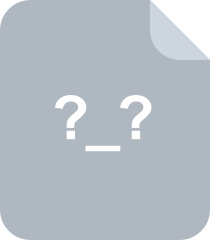
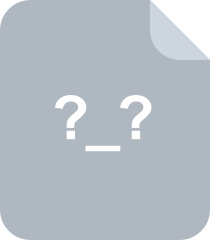
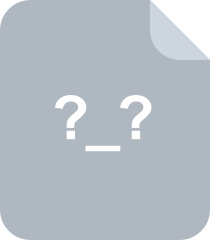
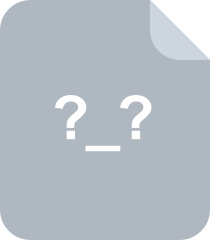
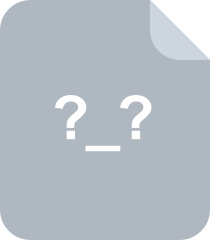
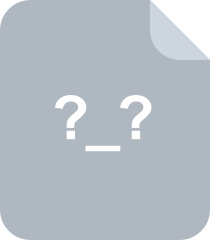
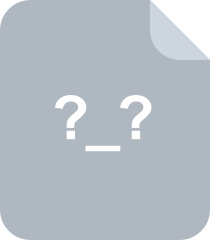
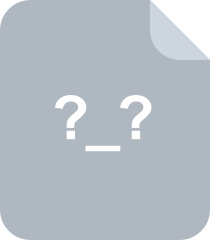
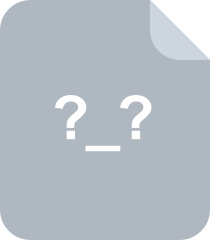
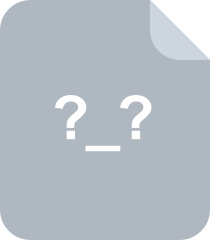
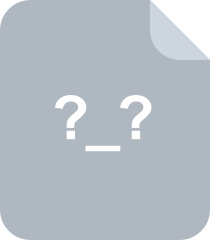
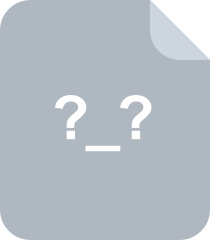
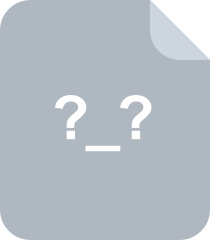
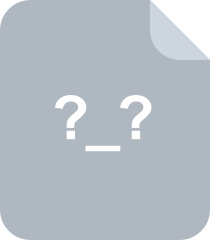
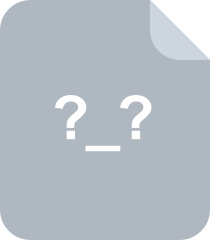
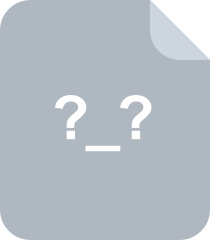
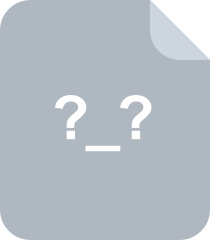
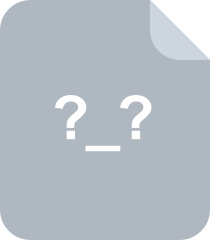
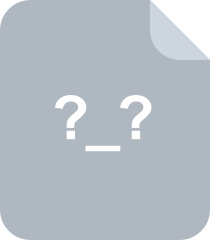
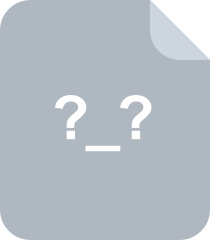
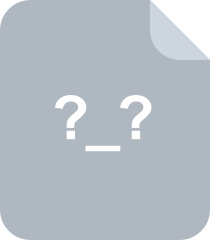
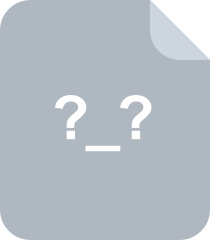
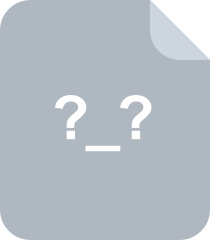
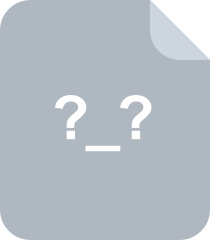
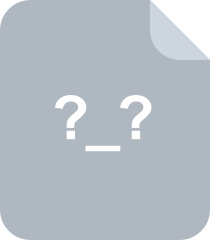
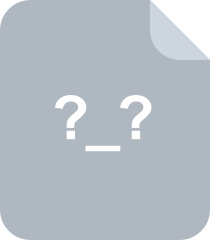
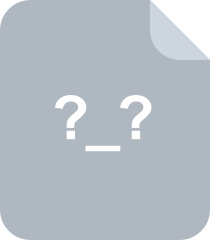
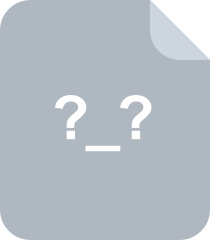
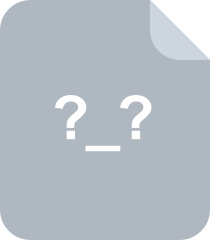
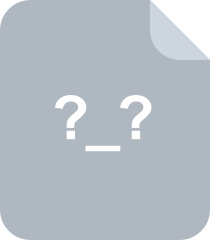
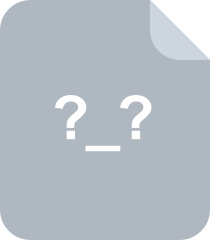
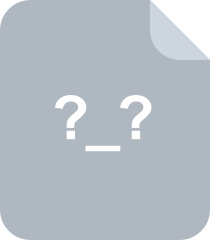
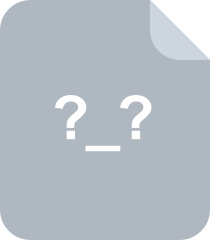
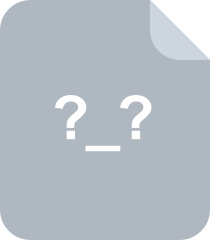
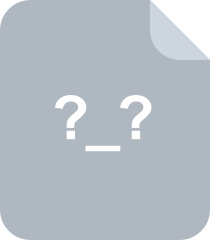
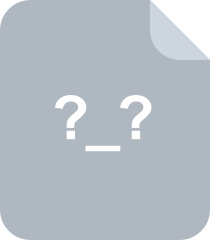
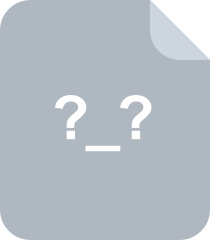
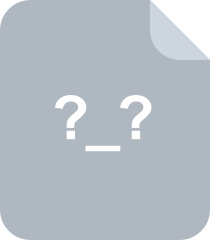
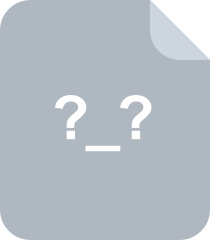
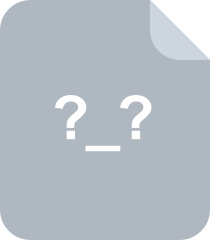
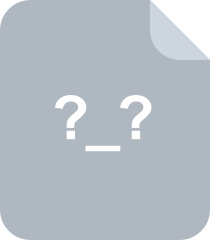
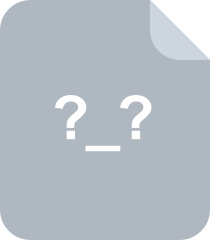
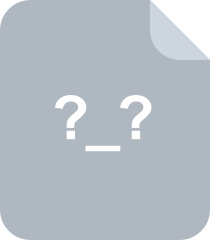
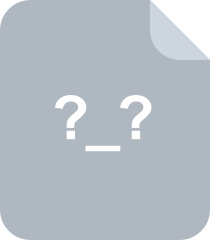
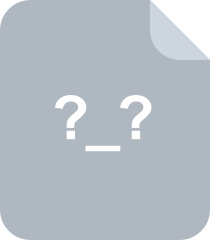
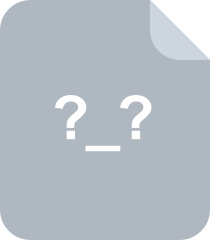
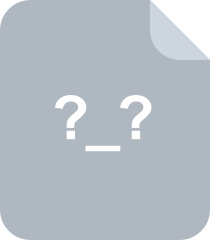
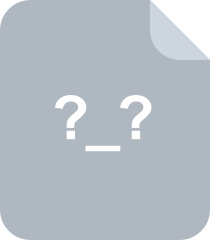
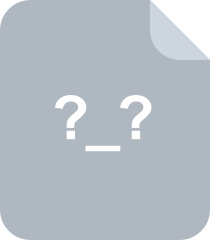
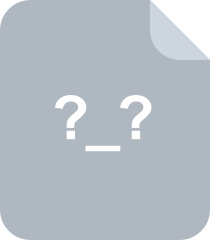
共 83 条
- 1
资源评论
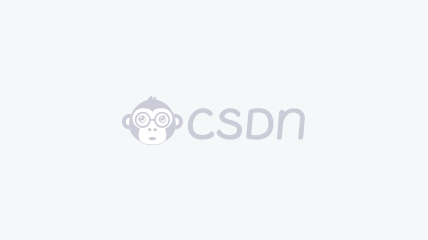

IT狂飙
- 粉丝: 4842
- 资源: 2650

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

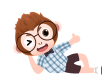
最新资源
- 梯度下降算法稀疏建模MATLAB库103版.zip
- 通过Matlab R2016b创建的CleanRepair eps PostScript矢量文件.zip
- 华为企业网络仿真平台(eNSP):设备仿真、图形化操作及应用场景解析
- 0-ANSWER.html
- 推荐系统用matlab编写的代码.zip
- 通过SRPPHAT等实现麦克风声源定位.zip
- 为那些用MATLAB绘图的人准备的备忘单.zip
- 我的matlab作业文件.zip
- 为学生学习MATLAB Simulink提供了一个很棒的有用资源列表,其中包括技巧、教程、视频、备忘单和学习MATLA.zip
- 无人机的MATLAB轨迹跟踪控制.zip
- 物理信息动态模态分解的MATLAB代码piDMD.zip
- 无线传感器网络中节点定位的MATLAB脚本.zip
- 稀疏表示分类器应用于高光谱图像分类的MATLAB代码实现仿真论文地址如下.zip
- 稀疏表示问题的MATLAB库.zip
- 先进PID控制MATLAB仿真 4th MATLAB PID算法 仿真 学习.zip
- 相关向量机的MATLAB代码使用SB2_Release_200.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


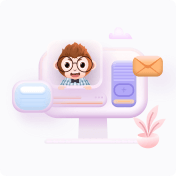
安全验证
文档复制为VIP权益,开通VIP直接复制
