/*
* Trace Recorder for Tracealyzer v4.8.1
* Copyright 2023 Percepio AB
* www.percepio.com
*
* SPDX-License-Identifier: Apache-2.0
*
* The generic core of the trace recorder's snapshot mode.
*/
#include <trcRecorder.h>
#if (TRC_CFG_RECORDER_MODE == TRC_RECORDER_MODE_SNAPSHOT)
#if (TRC_USE_TRACEALYZER_RECORDER == 1)
#include <string.h>
#include <stdarg.h>
#include <stdint.h>
#ifndef TRC_CFG_RECORDER_DATA_INIT
#define TRC_CFG_RECORDER_DATA_INIT 1
#endif
#if ((TRC_HWTC_TYPE == TRC_CUSTOM_TIMER_INCR) || (TRC_HWTC_TYPE == TRC_CUSTOM_TIMER_DECR))
#error "CUSTOM timestamping mode is not (yet) supported in snapshot mode!"
#endif
/* DO NOT CHANGE */
#define TRACE_MINOR_VERSION 7
/* Keeps track of the task's stack low mark */
typedef struct {
void* tcb;
uint32_t uiPreviousLowMark;
} TaskStackMonitorEntry_t;
TraceKernelPortDataBuffer_t xKernelPortDataBuffer;
#if (TRC_CFG_INCLUDE_ISR_TRACING == 1)
/*******************************************************************************
* isrstack
*
* Keeps track of nested interrupts.
******************************************************************************/
static traceHandle isrstack[TRC_CFG_MAX_ISR_NESTING];
/*******************************************************************************
* isPendingContextSwitch
*
* Used to indicate if there is a pending context switch.
* If there is a pending context switch the recorder will not create an event
* when returning from the ISR.
******************************************************************************/
int32_t isPendingContextSwitch = 0;
#endif /* (TRC_CFG_INCLUDE_ISR_TRACING == 1) */
/*******************************************************************************
* readyEventsEnabled
*
* This can be used to dynamically disable ready events.
******************************************************************************/
#if !defined TRC_CFG_INCLUDE_READY_EVENTS || TRC_CFG_INCLUDE_READY_EVENTS == 1
static int readyEventsEnabled = 1;
#endif /*!defined TRC_CFG_INCLUDE_READY_EVENTS || TRC_CFG_INCLUDE_READY_EVENTS == 1*/
/*******************************************************************************
* uiTraceTickCount
*
* This variable is should be updated by the Kernel tick interrupt. This does
* not need to be modified when developing a new timer port. It is preferred to
* keep any timer port changes in the HWTC macro definitions, which typically
* give sufficient flexibility.
******************************************************************************/
uint32_t uiTraceTickCount = 0;
/*******************************************************************************
* trace_disable_timestamp
*
* This can be used to disable timestamps as it will cause
* prvTracePortGetTimeStamp() to return the previous timestamp.
******************************************************************************/
uint32_t trace_disable_timestamp = 0;
/*******************************************************************************
* last_timestamp
*
* The most recent timestamp.
******************************************************************************/
static uint32_t last_timestamp = 0;
/*******************************************************************************
* uiTraceSystemState
*
* Indicates if we are currently performing a context switch or just running application code.
******************************************************************************/
volatile uint32_t uiTraceSystemState = TRC_STATE_IN_STARTUP;
/*******************************************************************************
* recorder_busy
*
* Flag that shows if inside a critical section of the recorder.
******************************************************************************/
volatile int recorder_busy = 0;
/*******************************************************************************
* timestampFrequency
*
* Holds the value set by vTraceSetFrequency.
******************************************************************************/
uint32_t timestampFrequency = 0;
/*******************************************************************************
* nISRactive
*
* The number of currently active (including preempted) ISRs.
******************************************************************************/
int8_t nISRactive = 0;
/*******************************************************************************
* handle_of_last_logged_task
*
* The current task.
******************************************************************************/
traceHandle handle_of_last_logged_task = 0;
/*******************************************************************************
* vTraceStopHookPtr
*
* Called when the recorder is stopped, set by vTraceSetStopHook.
******************************************************************************/
TRACE_STOP_HOOK vTraceStopHookPtr = (TRACE_STOP_HOOK)0;
/*******************************************************************************
* init_hwtc_count
*
* Initial TRC_HWTC_COUNT value, for detecting if the time-stamping source is
* enabled. If using the OS periodic timer for time-stamping, this might not
* have been configured on the earliest events during the startup.
******************************************************************************/
uint32_t init_hwtc_count;
/*******************************************************************************
* CurrentFilterMask
*
* The filter mask that will be checked against each object's FilterGroup to see
* if they should be included in the trace or not.
******************************************************************************/
uint16_t CurrentFilterMask TRC_CFG_RECORDER_DATA_ATTRIBUTE;
/*******************************************************************************
* CurrentFilterGroup
*
* The current filter group that will be assigned to newly created objects.
******************************************************************************/
uint16_t CurrentFilterGroup TRC_CFG_RECORDER_DATA_ATTRIBUTE;
/*******************************************************************************
* objectHandleStacks
*
* A set of stacks that keeps track of available object handles for each class.
* The stacks are empty initially, meaning that allocation of new handles will be
* based on a counter (for each object class). Any delete operation will
* return the handle to the corresponding stack, for reuse on the next allocate.
******************************************************************************/
objectHandleStackType objectHandleStacks TRC_CFG_RECORDER_DATA_ATTRIBUTE;
/*******************************************************************************
* traceErrorMessage
*
* The last error message of the recorder. NULL if no error message.
******************************************************************************/
const char* traceErrorMessage TRC_CFG_RECORDER_DATA_ATTRIBUTE;
#if defined(TRC_CFG_ENABLE_STACK_MONITOR) && (TRC_CFG_ENABLE_STACK_MONITOR == 1) && (TRC_CFG_SCHEDULING_ONLY == 0)
/*******************************************************************************
* tasksInStackMonitor
*
* Keeps track of all stack low marks for tasks.
******************************************************************************/
TaskStackMonitorEntry_t tasksInStackMonitor[TRC_CFG_STACK_MONITOR_MAX_TASKS] TRC_CFG_RECORDER_DATA_ATTRIBUTE;
/*******************************************************************************
* tasksNotIncluded
*
* The number of tasks that did not fit in the stack monitor.
******************************************************************************/
int tasksNotIncluded TRC_CFG_RECORDER_DATA_ATTRIBUTE;
#endif /* defined(TRC_CFG_ENABLE_STACK_MONITOR) && (TRC_CFG_ENABLE_STACK_MONITOR == 1) && (TRC_CFG_SCHEDULING_ONLY == 0) */
/*******************************************************************************
* RecorderData
*
* The main data structure in snapshot mode, when using the default static memory
* allocation (TRC_RECORDER_BUFFER_ALLOCATION_STATIC). The recorder uses a pointer
* Rec
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
RTOS 操作系统跟踪工具Tracealyzer Percepio http://percepio.com Percepio是一家位于瑞典的嵌入式操作系统系统辅助开发工具供应商,是Amazon Web Services合作伙伴,嵌入式视觉联盟成员。 Tracealyzer是Percepio 公司开发的一款用于RTOS或基于linux的嵌入式软件系统的可视化跟踪工具,对系统运行时的行为提供了前所未有的洞察方法。帮助开发人员加快固件的开发,减少对系统验证和性能优化所需要的时间。 目前Tracealyzer提供了30多种相互关联的运行时行为视图,包括任务调度、中断、任务之间的相互作用,以及从应用程序代码中记录的用户事件。Tracealyzer作为传统调试的补充,提供更高层次的调试视图,非常适合理解典型的实时问题。
资源推荐
资源详情
资源评论
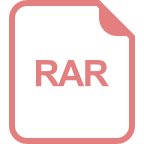
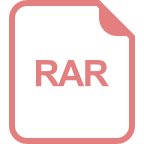
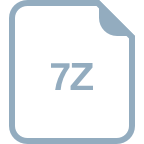
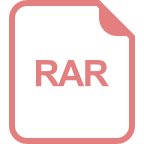
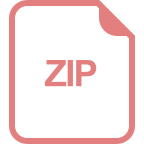
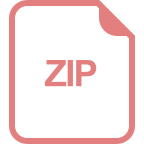
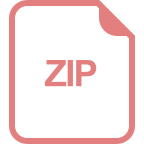
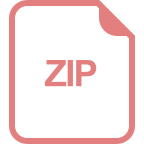
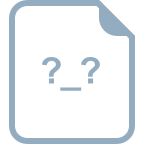
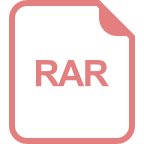
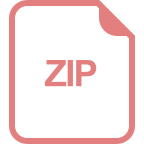
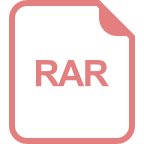
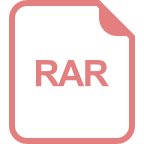
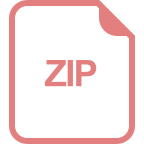
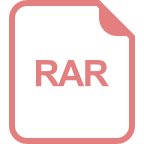
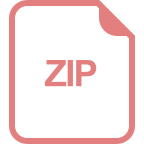
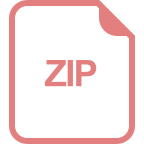
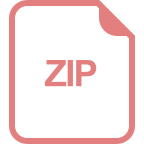
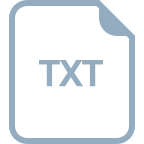
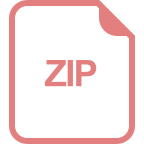
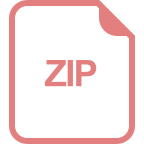
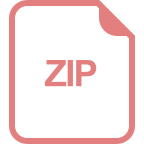
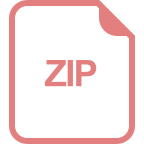
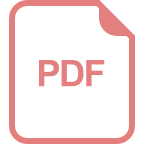
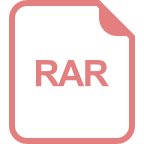
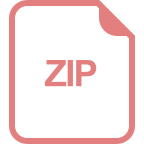
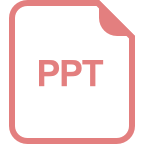
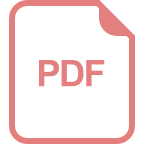
收起资源包目录

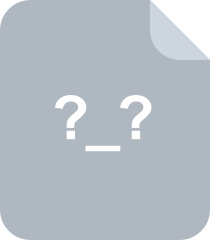
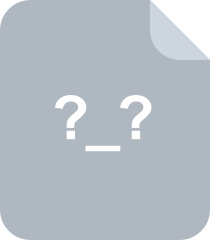
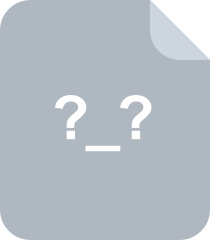
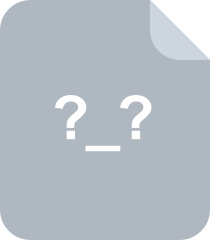
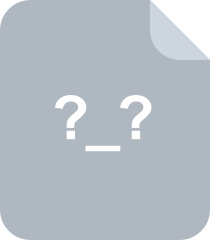
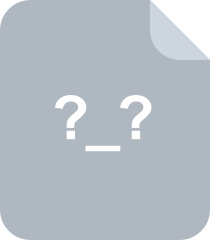
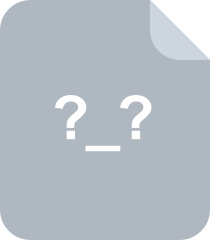
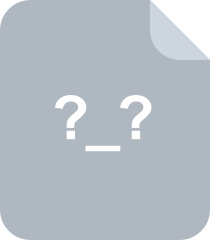
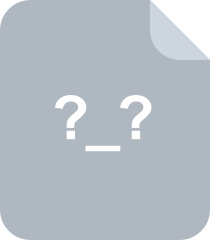
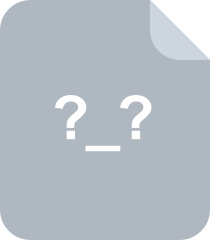
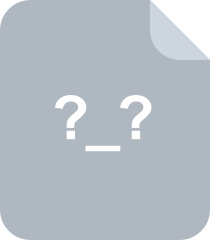
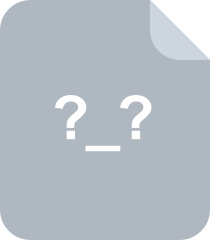
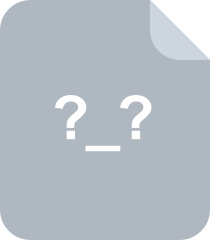
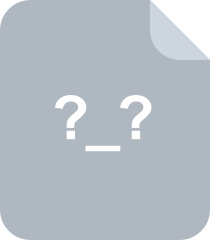
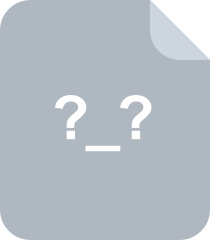
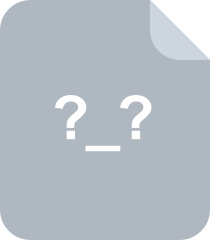
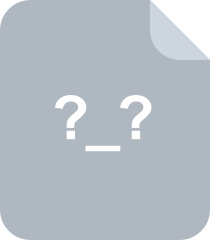
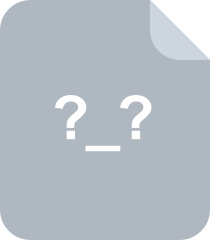
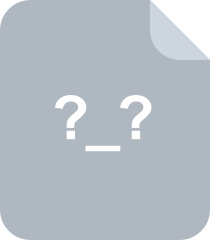
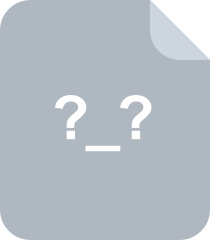
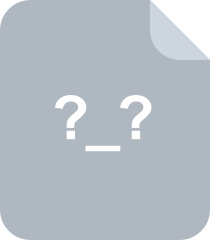
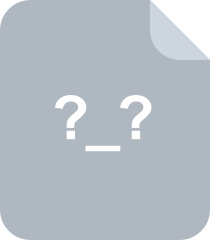
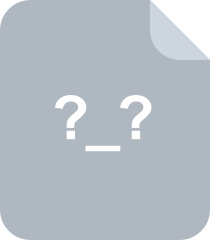
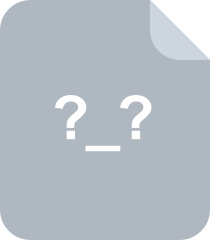
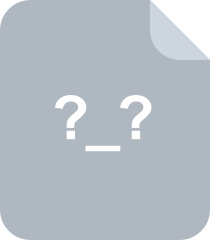
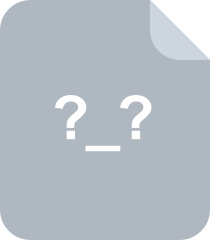
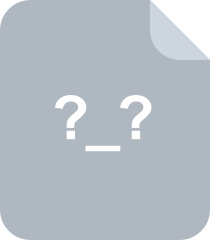
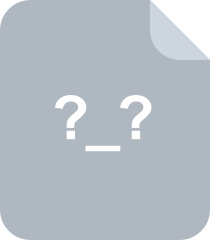
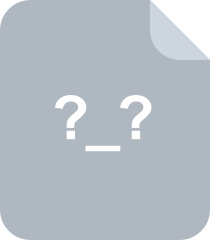
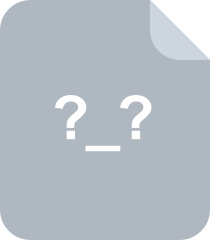
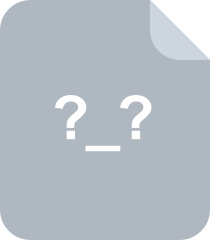
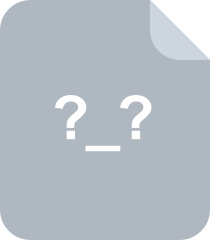
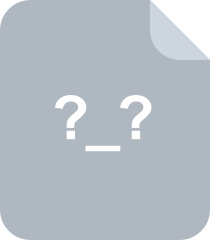
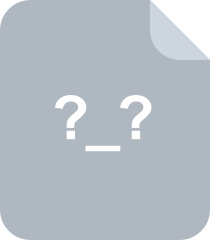
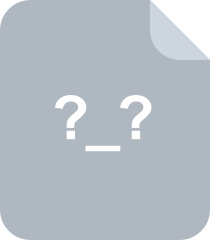
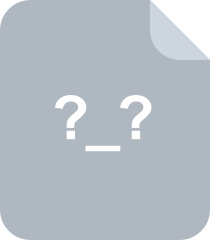
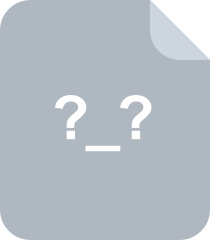
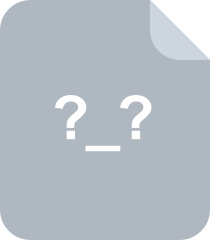
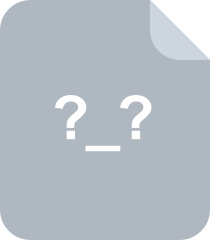
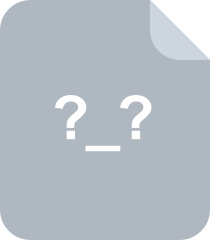
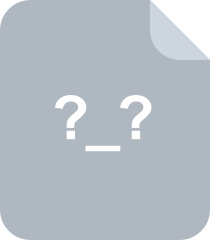
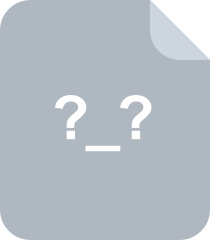
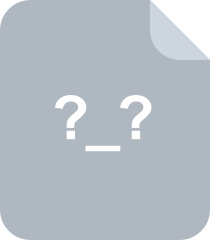
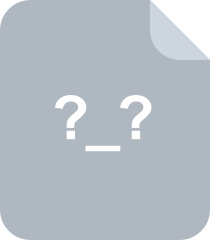
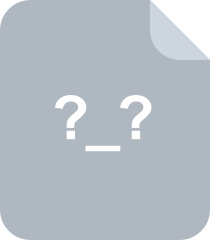
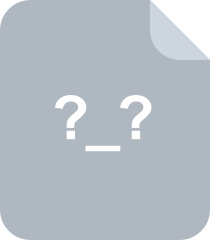
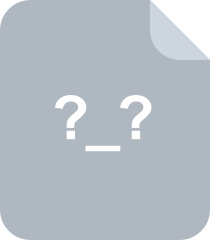
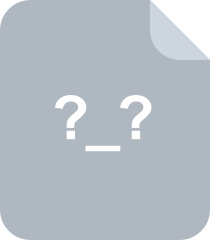
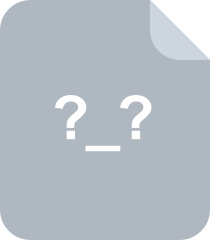
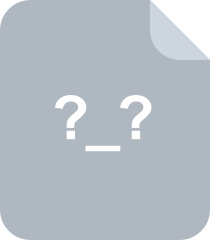
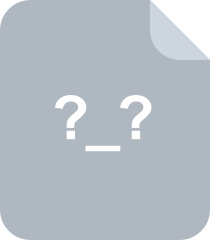
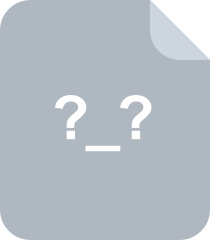
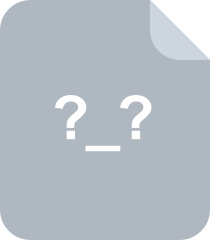
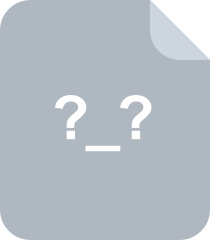
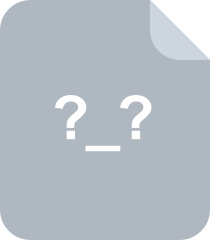
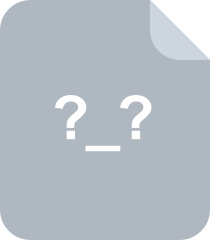
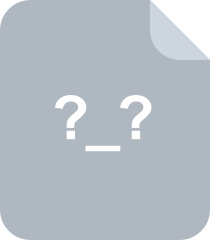
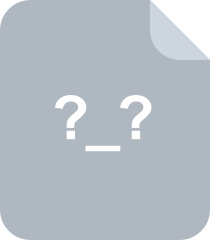
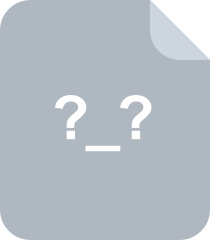
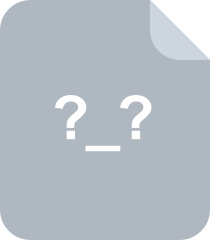
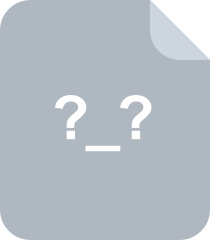
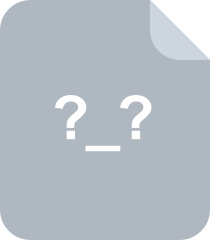
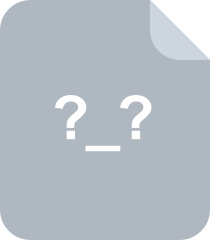
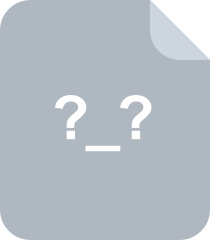
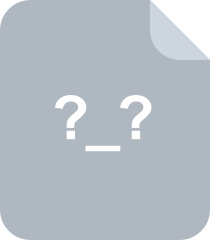
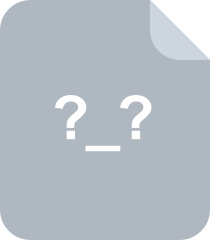
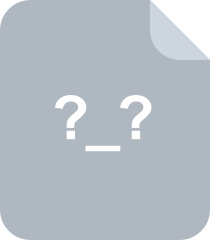
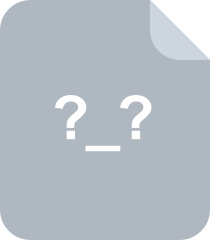
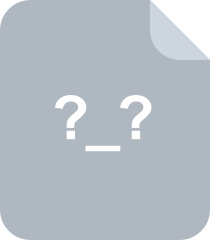
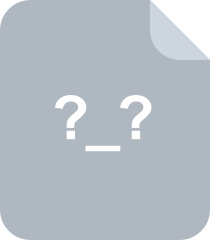
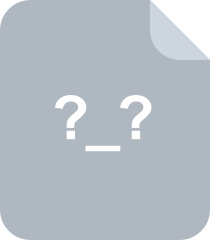
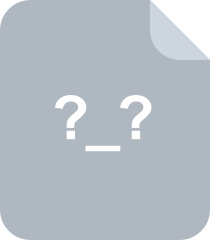
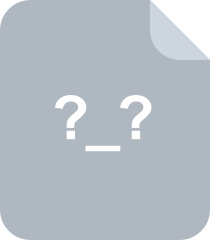
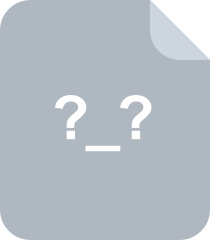
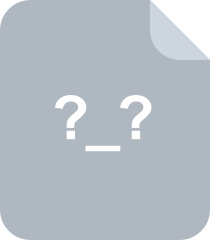
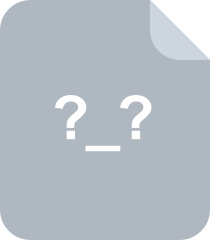
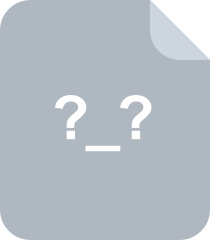
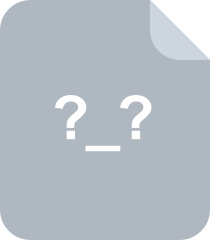
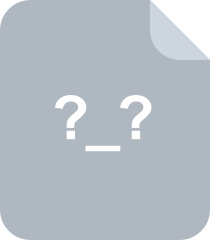
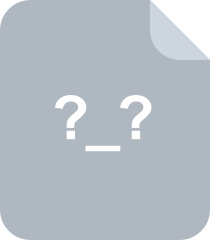
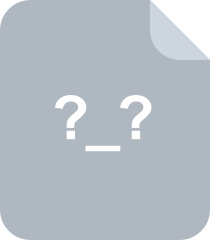
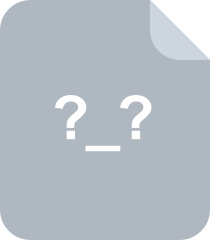
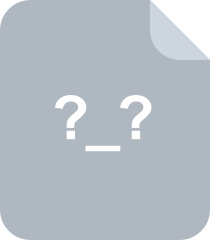
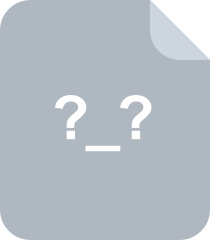
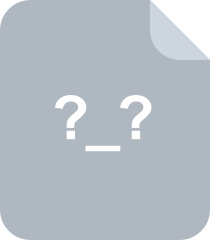
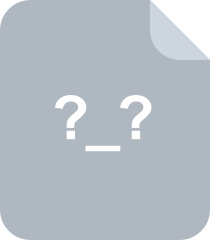
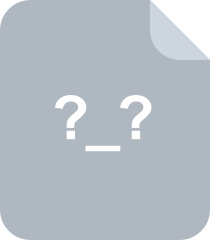
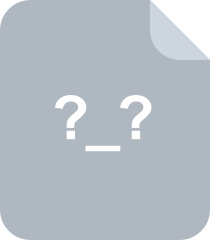
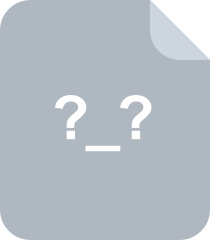
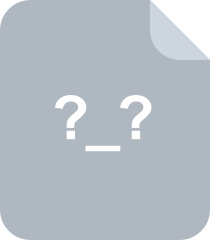
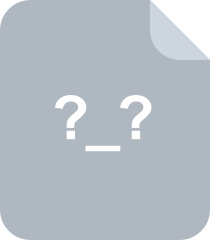
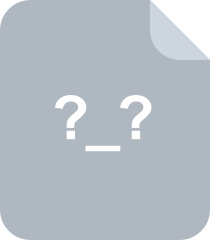
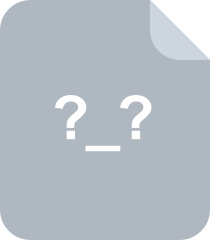
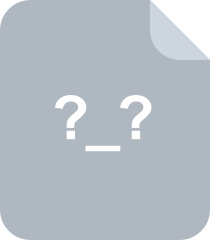
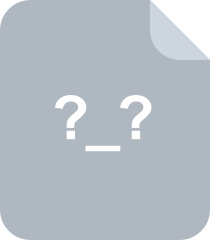
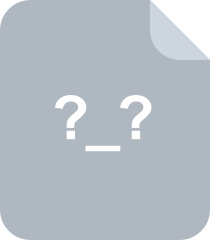
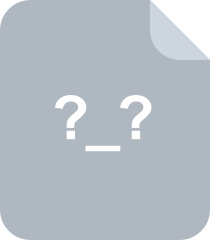
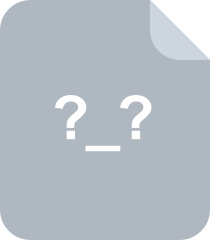
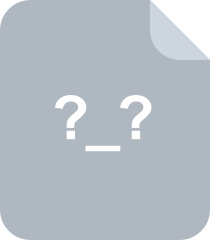
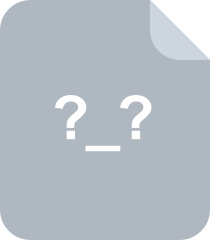
共 619 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
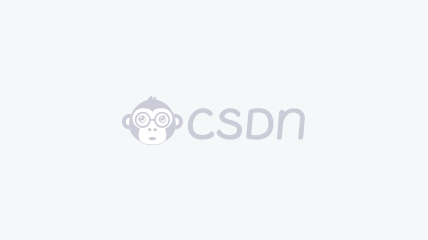

ken2232
- 粉丝: 1w+
- 资源: 25
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

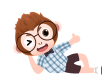
最新资源
- 雅居乐地产置业有限公司企业文化与福利制度培训教材(PPT 60页).ppt
- 人力资源--伊利集团岗前培训手册(PPT 67页).ppt
- 人力资源-培训积分制度(PPT).ppT
- 某某不动产新人培训手册-新人工作培训手册(PPT 38页).ppt
- HR工作者的心理素质完全手册.ppt
- 蓝月亮-人事专员培训操作手册(PPT 33页).ppt
- 人力资源部管理手册-培训管理办法(doc 20).doc
- 山西通达摩托车集团公司培训管理制度(doc 6页).doc
- 山东省对外经济贸易明达公司人事管理培训工作细则(DOC 7页).doc
- 人力资源开发与培训管理制度.doc
- 永泰鑫公司员工培训手册(DOC 27页).doc
- 员工培训计划表.doc
- 美的集团空调事业部人力资源开发与培训制度.doc
- 内部培训评估表7.7.doc
- 康佳集團培訓管理辦法.doc
- 培训需求调查表7.7.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


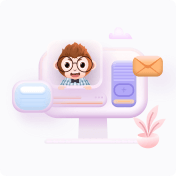
安全验证
文档复制为VIP权益,开通VIP直接复制
