pbm/pgm/ppm图片的读写(Matlab)
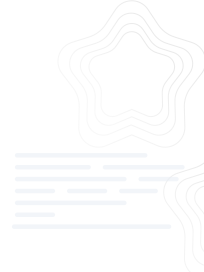


在图像处理领域,PBM、PGM和PPM是一种基于文本格式的简单图像文件类型,统称为PBMPlus或PBM格式。这些格式是最早用于位图图像的标准之一,尤其适用于早期的Unix系统。Matlab作为强大的数值计算和数据可视化环境,提供了处理这种格式图像的功能。本文将详细探讨如何在Matlab中读取和写入PBM、PGM和PPM图片,并介绍相关的编程知识。 PBM(Portable Bitmap)用于黑白图像,PGM(Portable Graymap)用于灰度图像,而PPM(Portable Pixmap)则用于彩色图像。这三种格式都是基于ASCII的,所以它们的内容可以被人类直接阅读。PBM文件以“P1”或“P4”标识,PGM文件以“P2”或“P5”,PPM文件以“P3”或“P6”标识,数字代表其特定的二进制或ASCII编码方式。 在Matlab中,可以使用`imread`和`imwrite`函数来操作这些文件。`imread`用于读取图像,`imwrite`用于保存图像。但需要注意的是,Matlab内置对PBM/PGM/PPM的支持可能有限,因此可能需要自定义函数来处理这些特定格式。 例如,我们可以创建一个自定义的读取函数,如下: ```matlab function img = readPBMPPM(filename) fid = fopen(filename, 'r'); header = fgets(fid); switch header(2) case '1', % PBM ASCII fmt = 'PBM'; data = textscan(fid, '%s', 'HeaderLines', 1, 'Delimiter', ''); img = logical(str2double(data{1})); case '4', % PBM binary fmt = 'PBM'; [width, height] = sscanf(fscanf(fid, '%d %d'), '%d %d'); fseek(fid, 1, 'bof'); % skip "1" img = fread(fid, width*height, 'uint8=>logical') == 1; case '2', % PGM ASCII fmt = 'PGM'; data = textscan(fid, '%s%d%d', 'HeaderLines', 1, 'Delimiter', ''); maxVal = str2double(data{3}); img = reshape(str2double(data{2}'), ... str2double(data{1}(3:end)), []); img = uint8(img / maxVal * 255); case '5', % PGM binary fmt = 'PGM'; [width, height] = sscanf(fscanf(fid, '%d %d'), '%d %d'); maxVal = fscanf(fid, '%d', 1); fseek(fid, 1, 'bof'); % skip "1" img = fread(fid, width*height, 'int16=>uint8') ./ maxVal * 255; case '3', % PPM ASCII fmt = 'PPM'; data = textscan(fid, '%s%d%d%d', 'HeaderLines', 1, 'Delimiter', ''); maxVal = str2double(data{4}); img = reshape(str2double(data{2}'), ... str2double(data{1}(3:end)), str2double(data{1}(1:end-3))); img = uint8(img / maxVal * 255); case '6' % PPM binary fmt = 'PPM'; [width, height] = sscanf(fscanf(fid, '%d %d'), '%d %d'); maxVal = fscanf(fid, '%d', 1); fseek(fid, 1, 'bof'); % skip "1" img = fread(fid, width*height*3, 'uint8') ./ maxVal * 255; img = permute(reshape(img, 3, [], width, height), [2 4 3 1]); end fclose(fid); end ``` 该函数首先读取文件头以确定文件类型,然后根据不同的格式读取图像数据并转换为Matlab中的矩阵表示。对于PPM文件,还需要处理颜色通道。 相应地,我们可以编写一个自定义的写入函数: ```matlab function writePBMPPM(filename, img, fmt) if nargin < 3 fmt = ''; end switch fmt case '' fmt = islogical(img) ? 'PBM' : (max(max(img)) > 255) ? 'PPM' : 'PGM'; case {'PBM', 'PGM', 'PPM'} % nothing to do otherwise error('Unsupported format.'); end fid = fopen(filename, 'w'); fprintf(fid, 'P%i\n', find(strcmp({'P1', 'P2', 'P3', 'P4', 'P5', 'P6'}, [fmt ''])); switch fmt case 'PBM' fprintf(fid, '%d %d\n', size(img, 2), size(img, 1)); if islogical(img) fprintf(fid, '%d ', img(:)'); else fwrite(fid, img(:)', 'uint8'); end case 'PGM' fprintf(fid, '%d %d %d\n', size(img, 2), size(img, 1), max(max(img))); fwrite(fid, img(:)', 'uint8'); case 'PPM' fprintf(fid, '%d %d %d\n', size(img, 3), size(img, 2), size(img, 1)); fwrite(fid, permute(img, [3 2 1]), 'uint8'); end fclose(fid); end ``` 这个函数会自动检测输入图像的数据类型和最大值,然后选择合适的格式进行写入。 在实际使用中,你可以这样调用这两个函数: ```matlab % 读取图像 img = readPBMPPM('input.pbm'); % 处理图像... % 保存图像 writePBMPPM('output.ppm', img, 'PPM'); ``` 总结一下,Matlab虽然内建的图像读写功能可能不直接支持PBM/PGM/PPM格式,但通过编写自定义函数,我们可以轻松地实现这些格式的读取和写入。了解这些基本的图像格式以及如何在Matlab中操作它们,对于进行图像处理和分析是非常有帮助的。
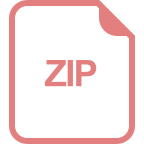
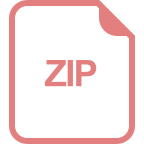
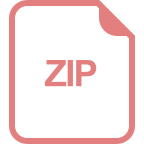
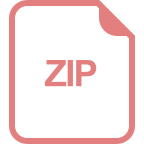
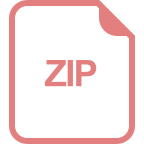
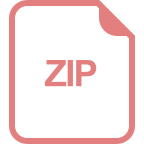
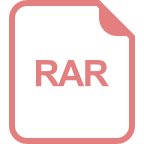
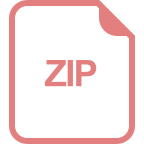
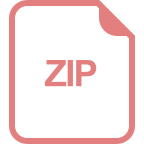
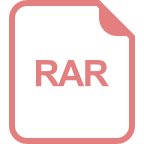
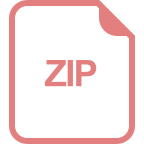
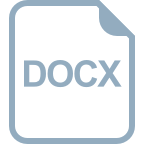
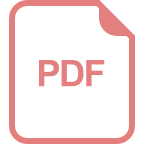
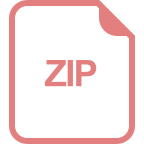
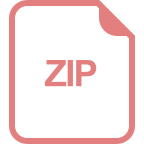


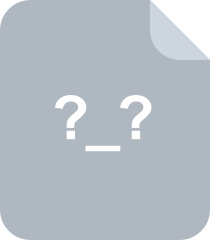
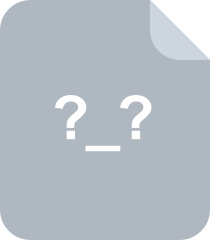
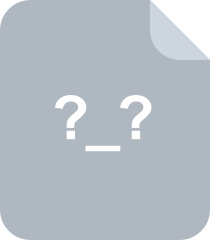
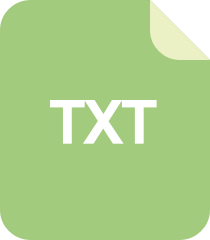
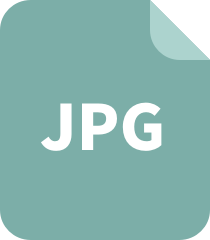
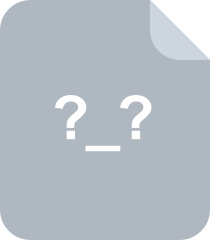
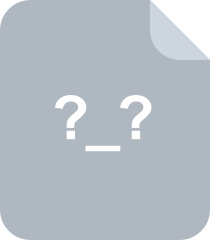
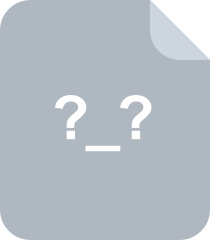
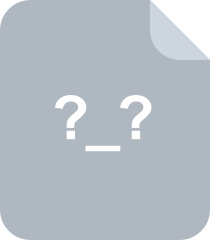
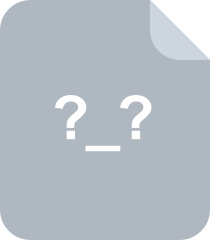
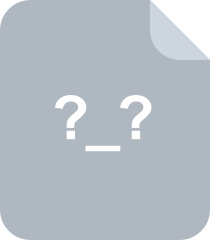
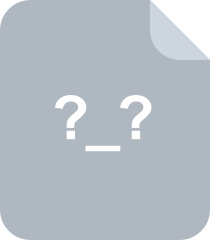
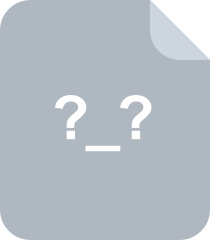
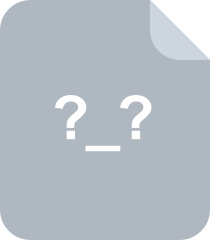
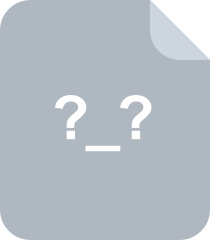
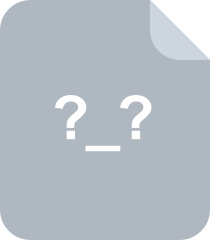
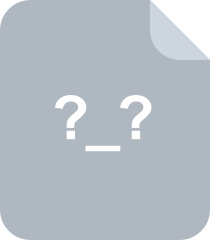
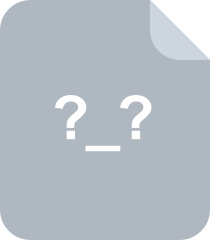
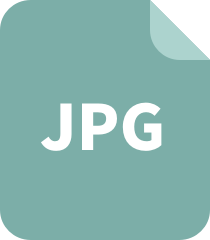
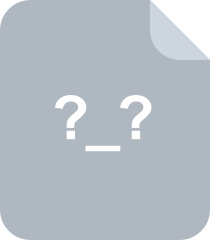
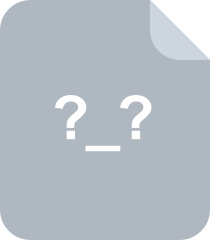
- 1
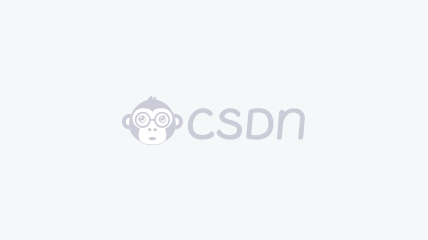
- zhu_yuxiang2017-09-25算法是可用的
- AAA苏打饼干专业扎孔2020-05-15运行不了 错误使用 fread 文件标识符无效。使用 fopen 生成有效的文件标识符。
- weixin_415934182018-05-05个人觉得还是有用的
- myfanmy2017-06-06还算比较有用的
- fanliang122017-05-31还是有用的

- 粉丝: 286
- 资源: 20
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

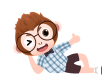
最新资源
- 欧拉方法求解非线性微分方程组及其参数设置与应用
- 华中科技大学 数据库系统原理实践 - 以MySQL为例.zip
- 蜻蜓I系统即时通讯软件v1.0.4版本完整版
- 车辆状态估计,无迹卡尔曼滤波UKF车辆状态估计,无迹卡尔曼滤波UKF 针对乘用车进行车辆运动状态参数估计,采用UKF无迹卡尔曼滤波算法,对车辆的纵向车速、侧向速度、横摆角速度、质心侧偏角、各轮侧向力进
- 南京大学《数据新闻》2017 周一 第3-4节 逸B-210 1-18周.zip
- 传感网应用的开发环境搭建、项目配置及问题解决办法
- 史上最大规模1.4亿知识图谱数据免费下载,知识图谱,通用知识图谱,融合了两千五百多万的实体,拥有亿级别的实体属性关系 .zip
- 车辆稳定域划分,车辆稳定边界拟合 车辆稳定性相平面MATLAB程序绘制 根据确定的简化魔术公式轮胎模型,建立车辆非线性二自由度运动微分方程,并进而对相平面图进行绘制 包括横摆角速度与质心侧偏角的相
- 2-Beaver Notes V3.7.0
- 传感网应用的开发环境搭建、项目配置及问题解决办法
- 分布式光伏结构设计实操班,视频讲解思路清晰,由浅入深,包含PKPM和SAP2000设计,适合新能源光伏设计人员入门及提高,资料难得
- 哈尔滨工业大学《数据结构与算法》、《软件开发实践》作业及实验的Scheme解法 .zip
- 使用python实现空间重构,一维时间序列数据中重建相空间
- 国内首个迁移学习赛题 中国平安前海征信“好信杯”迁移学习大数据算法大赛 FInSight团队作品(算法方案排名第三).zip
- 枝晶生长Comsol仿真模型 锂枝晶生长过程的 枝晶生长Comsol仿真模型 锂枝晶生长过程的枝晶形貌,温度场耦合,应力场,浓度场,电势场 C++程序,基于元胞自动机法模拟枝晶生长,能实现任意角
- STM32使用rtt软件 增加flashdb软件包

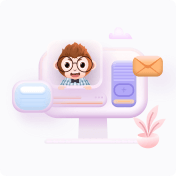
