# Simple OnOff model
The Mesh Model Specification specifies a Generic OnOff Model to be used in real applications
with the mesh. This vendor-specific model is a simplified version of the Generic OnOff Model.
It is an introductory example for @ref md_doc_libraries_how_to_models, but you can also use it
in your applications.
See the following sections for information about how to implement a vendor-specific
Simple OnOff model that turns something On or Off, for example a light bulb, a heater,
or a washing machine.
@note
For brevity, some important features such as error handling are not discussed on this page.
When writing your application, check the error codes returned by all API functions
to avoid bugs in your application.
**Table of contents**
- [Properties and features](@ref simple_onoff_model_overview)
- [Supported opcodes](@ref simple_onoff_model_overview_opcodes)
- [Identifiers](@ref simple_onoff_model_overview_identifiers)
- [Implementing the model](@ref simple_onoff_model_implementing)
- [Implementing the server model](@ref simple_onoff_model_implementing_server)
- [Implementing the client model](@ref simple_onoff_model_implementing_client)
You can check the complete model implementation and its layout
in the `models/vendor/simple_on_off` directory.
If you want to see this model integrated into a complete application, take a look at
the @ref md_examples_light_switch_README and at the `examples/light_switch` directory.
---
## Properties and features @anchor simple_onoff_model_overview
A mesh application is specified using a client-server architecture, where client and server
models use publish and subscribe mechanism to communicate with each other.
For this reason, the intended functionality of this model will be realized using two parts:
- the server model, used for maintaining the OnOff state;
- a client model, used for manipulating the OnOff state on the server.
When the server model receives a GET or a (reliable) SET message from a client model, it sends the
current value of the OnOff state as response. This keeps the client up-to-date about the server
state.
For more details about setting up publication and subscription,
see [Creating new models](@ref creating_models_publication_subscription).
### Supported opcodes @anchor simple_onoff_model_overview_opcodes
The following table shows the opcodes that are supported by this model.
| Name | Definition | Opcode | Description | Parameter | Parameter size |
| ------------------ | -----------------------------------------| ------------:| ----------------------------- | ------------- | --------------:|
| SET | `::SIMPLE_ON_OFF_OPCODE_SET` | 0xc1 | Sets the current on/off state | New state | 1 byte |
| GET | `::SIMPLE_ON_OFF_OPCODE_GET` | 0xc2 | Gets the current on/off state | N/A | No parameter |
| SET UNRELIABLE | `::SIMPLE_ON_OFF_OPCODE_SET_UNRELIABLE` | 0xc3 | Sets the current on/off state | New state | 1 byte |
| Status | `::SIMPLE_ON_OFF_OPCODE_STATUS` | 0xc4 | Contains the current state | Current state | 1 byte |
The opcodes sent on-air are three bytes for the vendor-specific models. The
complete opcode is the combination of the vendor-specific opcode and the company identifier.
For more information, see the `access_opcode_t` documentation.
### Identifiers @anchor simple_onoff_model_overview_identifiers
For this model, the following identifiers are used.
| Description | Value |
| ------------------ | ---------:|
| Company identifier | 0x0059 |
| Server identifier | 0x0000 |
| Client identifier | 0x0001 |
The company identifier used in this table is Nordic Semiconductor's assigned Bluetooth
company ID. In a real application, use your own company's assigned ID.
---
## Implementing the model @anchor simple_onoff_model_implementing
As described earlier, a model comprises of two entities that together implement
the complete behavior:
- Server model, which typically has states and exposes messages to control
value of these states and trigger behaviors.
- Client model, which sends the messages to control and observe the states on the server model.
Implement both the [server](@ref simple_onoff_model_implementing_server) and the [client](@ref simple_onoff_model_implementing_client) models
for the Simple OnOff model to work.
### Implementing the server model @anchor simple_onoff_model_implementing_server
The behavior of the simple OnOff server is illustrated by the following message sequence chart.

When the OnOff server receives SET and GET messages:
- It calls a callback function provided by the application.
- It shares or requests the data through callback function parameters.
To implement the server model:
-# Define a model context structure that contains pointers to the callback functions.
This context structure gets passed to all message handlers.
- The following code snippet shows the context structure required
for the server model (`simple_on_off_server_t`) and associated callbacks:
```C
/** Forward declaration. */
typedef struct __simple_on_off_server simple_on_off_server_t;
/**
* Get callback type.
* @param[in] p_self Pointer to the Simple OnOff Server context structure.
* @returns @c true if the state is On, @c false otherwise.
*/
typedef bool (*simple_on_off_get_cb_t)(const simple_on_off_server_t * p_self);
/**
* Set callback type.
* @param[in] p_self Pointer to the Simple OnOff Server context structure.
* @param[in] on_off Desired state
* @returns @c true if the set operation was successful, @c false otherwise.
*/
typedef bool (*simple_on_off_set_cb_t)(const simple_on_off_server_t * p_self, bool on_off);
/** Simple OnOff Server state structure. */
struct __simple_on_off_server
{
/** Model handle assigned to the server. */
access_model_handle_t model_handle;
/** Get callback. */
simple_on_off_get_cb_t get_cb;
/** Set callback. */
simple_on_off_set_cb_t set_cb;
};
```
-# Define the opcodes and create the necessary opcode handler functions to handle
the incoming messages for the server model.
- You need three opcode handlers in the server to handle `::SIMPLE_ON_OFF_OPCODE_GET`,
`::SIMPLE_ON_OFF_OPCODE_SET`, and `::SIMPLE_ON_OFF_OPCODE_SET_UNRELIABLE` messages. Each of
these opcode handlers calls the corresponding user callback function from the context
structure. This context structure gets passed to the opcode handlers via the `p_args` parameter.
- All opcode handlers of all the models must use the same function prototype:
```C
typedef void (*access_opcode_handler_cb_t)(access_model_handle_t handle,
const access_message_rx_t * p_message,
void * p_args);
```
- The following snippet shows the opcode handlers defined for the Simple OnOff server model:
```C
static void handle_set_cb(access_model_handle_t handle, const access_message_rx_t * p_message, void * p_args)
{
simple_on_off_server_t * p_server = p_args;
NRF_MESH_ASSERT(p_server->set_cb != NULL);
bool value = (((simple_on_off_msg_set_t*) p_message->p_data)->on_off) > 0;
value = p_server->set_cb(p_server, value);
reply_status(p_server, p_message, value);
(void) simple_on_off_server_status_publish(p_server, value); /* We don't care about status */
}
static void handle_get_cb(access_model_handle_t handle, const access_message_rx_t * p_message, void * p_args)
{
simple_on_off_server_t * p_server = p_args;
NRF_MESH_ASSERT(p_server->get
没有合适的资源?快使用搜索试试~ 我知道了~
nrf5_SDK_for_Mesh_v4.0.0_src.zip
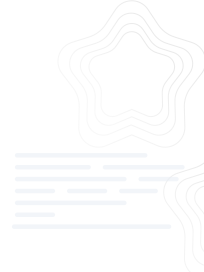
共1950个文件
h:375个
c:303个
sct:222个

需积分: 10 6 下载量 122 浏览量
2020-05-18
08:50:41
上传
评论
收藏 55.8MB ZIP 举报
温馨提示
--基于SES开发的蓝牙MESH SDK --nRF52832 目前最新版本 蓝牙 MESH SDK --内嵌开发例程 --SDK为SDK16.0 --自带代理功能,可直接使用手机app配置,比V2.0好太多
资源推荐
资源详情
资源评论
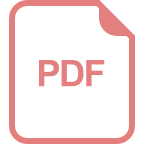
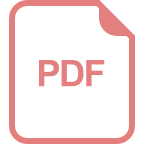
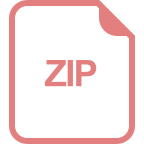
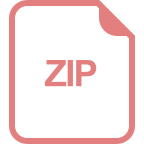
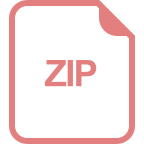
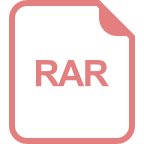
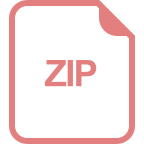
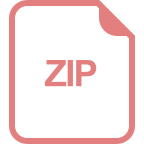
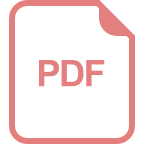
收起资源包目录

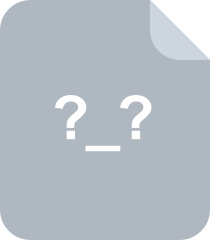
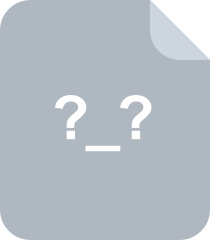
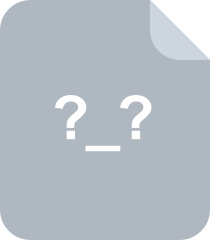
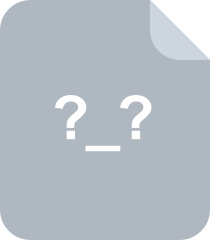
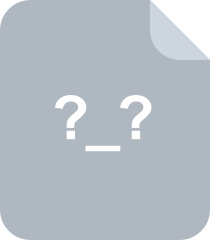
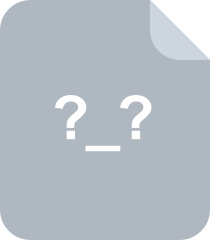
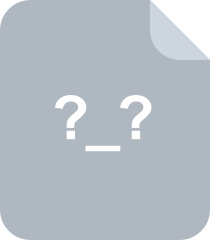
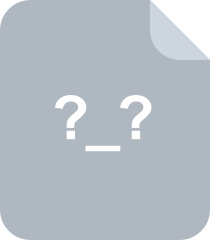
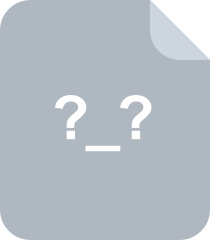
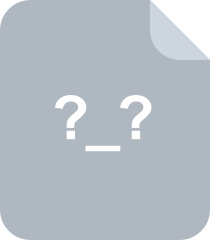
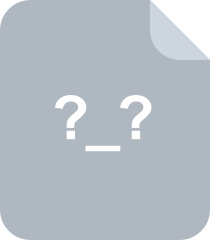
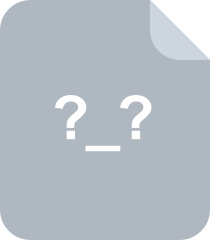
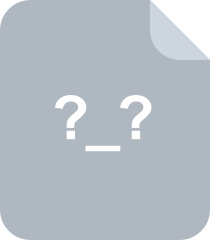
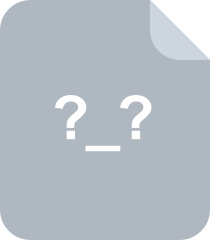
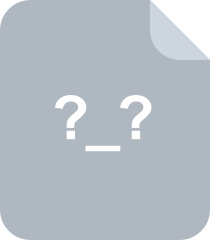
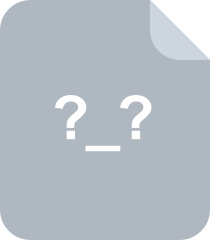
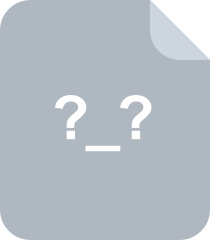
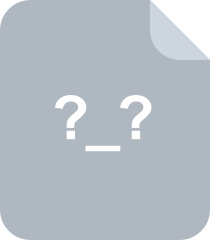
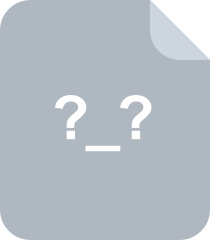
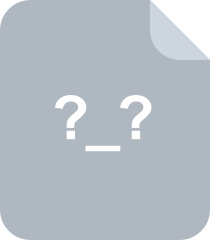
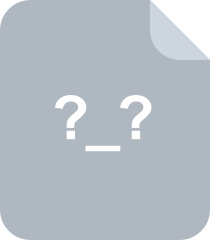
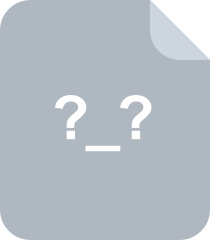
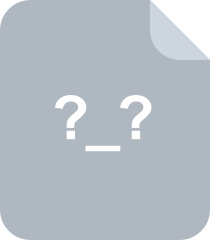
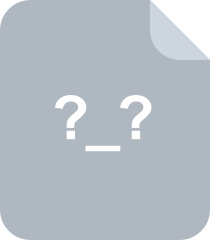
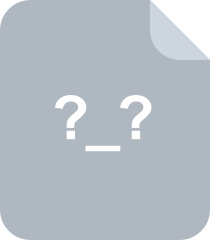
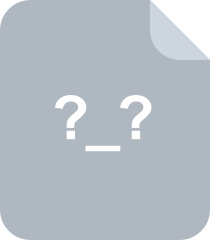
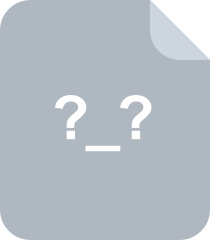
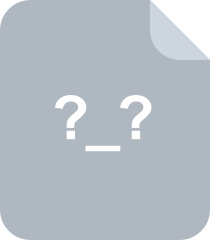
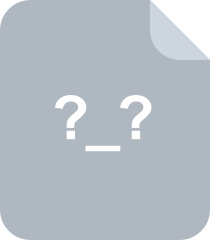
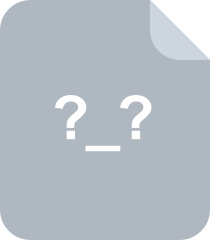
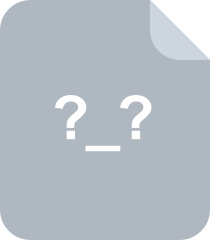
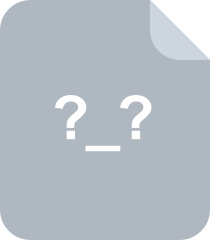
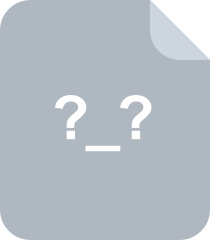
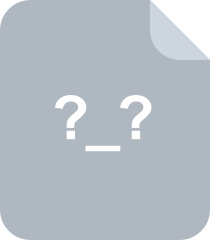
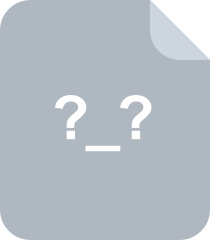
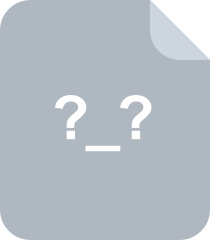
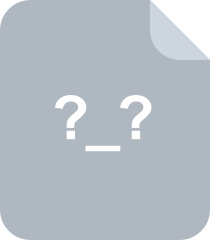
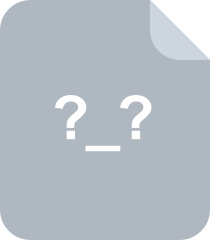
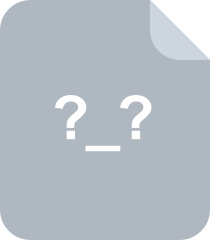
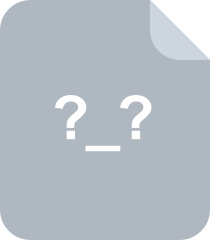
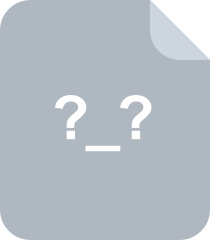
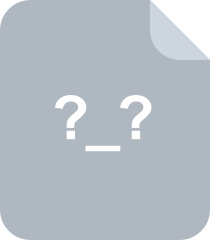
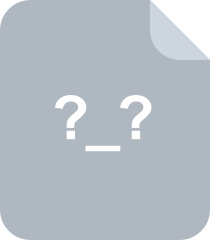
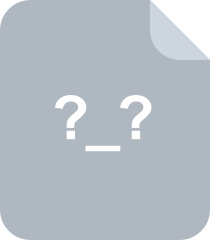
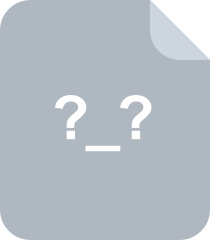
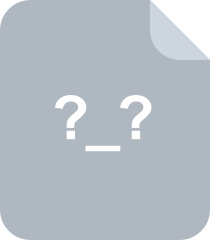
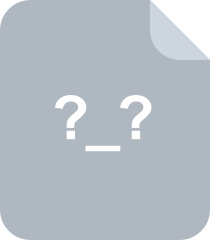
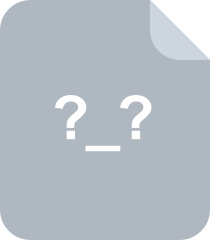
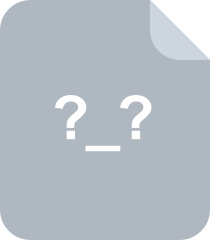
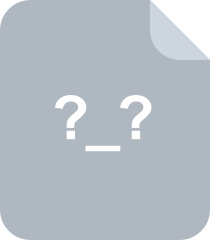
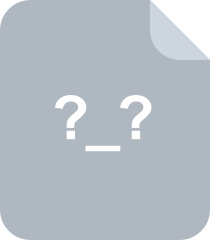
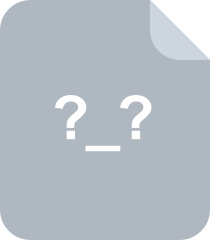
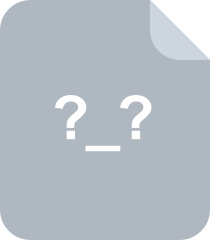
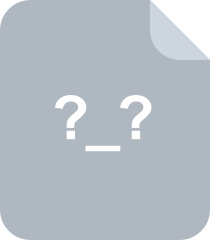
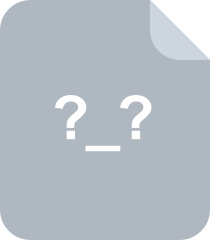
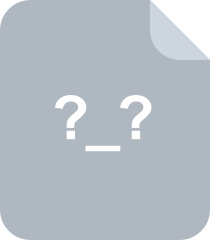
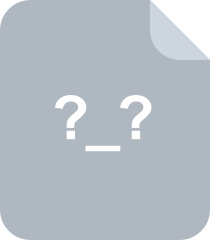
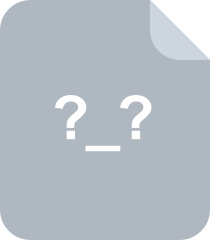
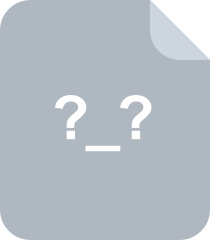
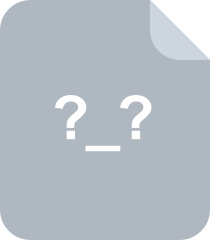
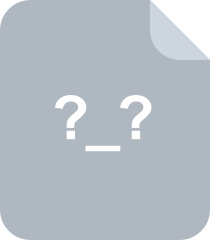
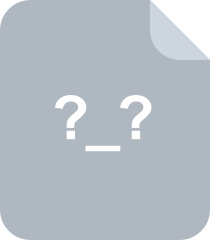
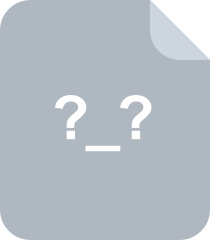
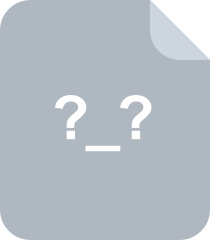
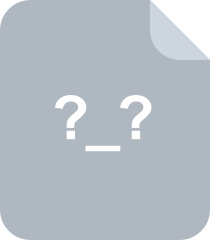
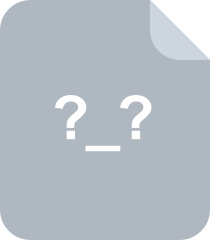
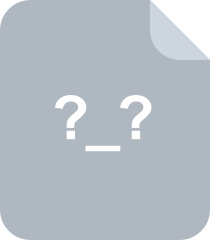
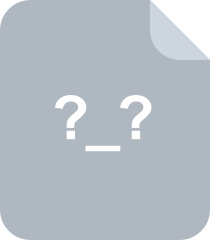
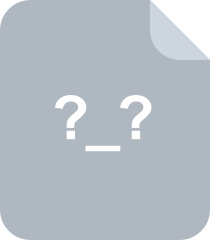
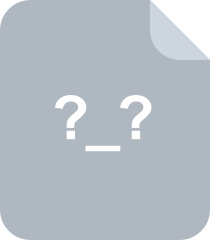
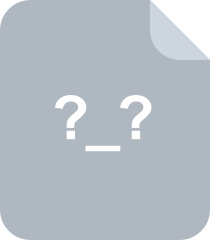
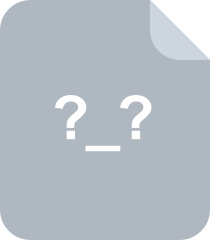
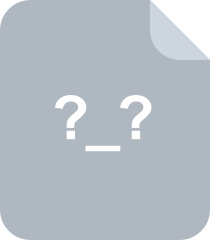
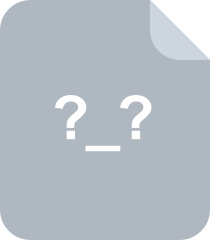
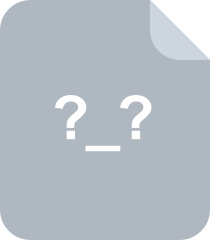
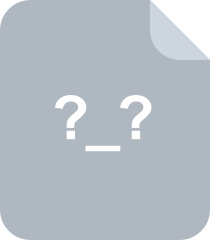
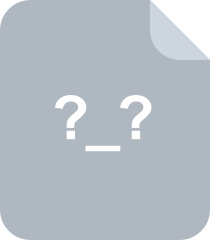
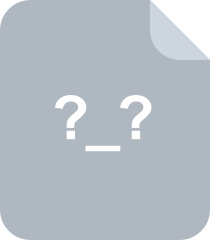
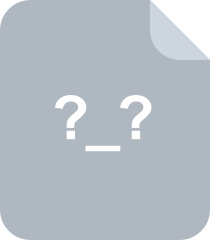
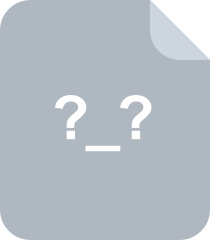
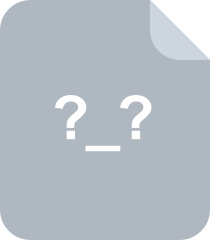
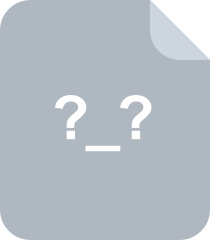
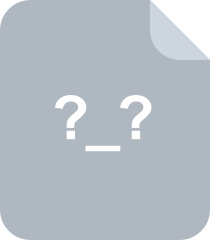
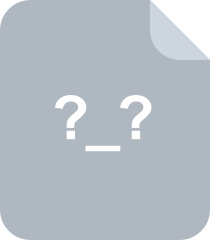
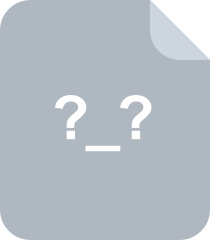
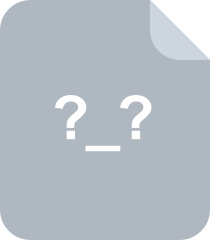
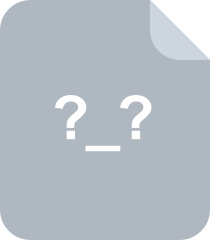
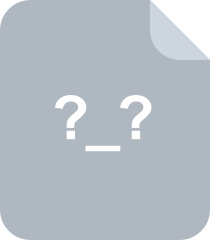
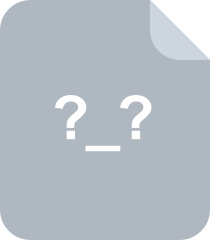
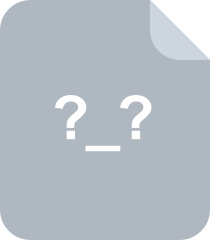
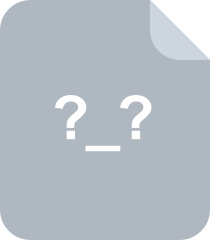
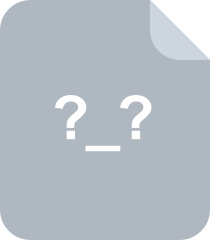
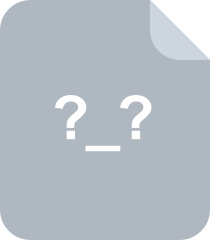
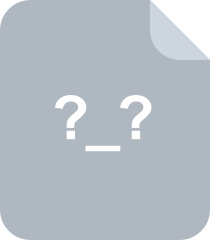
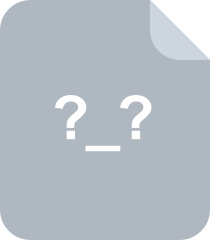
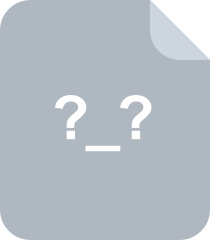
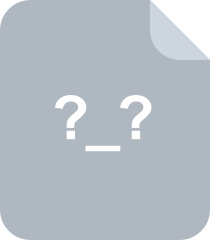
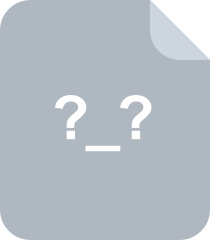
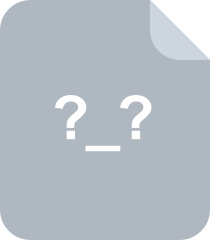
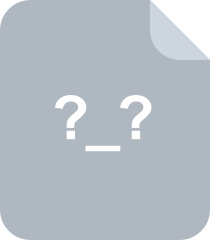
共 1950 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
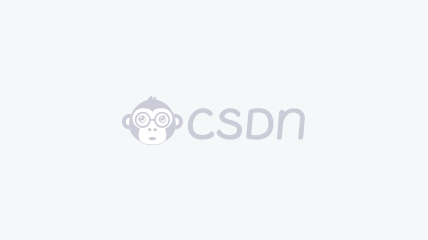


jiaozao6542
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

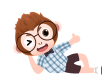
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


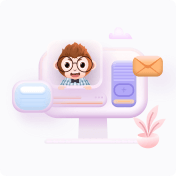
安全验证
文档复制为VIP权益,开通VIP直接复制
