function [vlist,edgeq,flist,info]=upolyhedron(w,md)
% UPOLYHEDRON calculate uniform polyhedron characteristics
%
% Inputs: W Specifies the desired polyhedron in one of three forms:
% (a) name e.g. W='cube'; precede by 'dual' for dual or
% 'laevo' or 'dextro' [default] for chiral polyhedra
% (b) index in the list given below e.g. W=6 is the cube; negative for dual
% n.{1,2,3,4,5} gives n-sided prism, antiprism, grammic prism, grammic antiprism or grammic crossed antiprism
% (c) Wythoff symbol (see below) e.g. W=[3 0 2 4] is the cube
% |p q r, p|q r, p q|r or p q r| respectively with 0 for |
% using -1 instead of 0 gives the dual of the polyhedron
% using -2 (or -3 for dual) gives a reflected version of a snub polyhedron
% MD specifies a mode string
% 'g' plot image
% 'w' plot wireframe
% 'f' plot coloured faces
% 'v' number vertices
% 't' plot vertex figure (a slice centred on a vertex)
%
% ? homogeneous output coordinates
% ? create net
% ? segment faces to remove internal portions of the surfaces
% ? size: [max] diameter=1, [longest] edge=1, include anisotropic normalization
% to minimize variance of vertex radius or edge vector length
% ? orientation: vertex at the top, largest stable base at bottom
%
% Outputs:
% VLIST(:,7) gives the [x y z d n e t] for each vertex
% x,y,z = position, d=distance from origin, n=valency, e=edge index, t=type (-ve for reflected)
% EDGEQ(:,9) has one row for each direction of each edge:
% 1 v1 first vertex (normally start)
% 2 v2 second vertex
% 3 f1 first face (normally on left)
% 4 f2 second face
% 5 ev1 next edge around vertex 1 (normally anticlockwise)
% 6 ef1 next edge around f1 (normally anticlockwise)
% 7 er reverse edge
% 8 z twisted edge: clockwise neighbours around v1 and v2 are on the same face
% 9 sf swap face order: ???
% 10 sv swap vertex order: v2 preceeds v1 around f1
% FLIST(:,7) gives the [x y z d n e t] for each face
% x,y,z = unit normal, d=distance from origin, n=valency, e=edge index, t=type (-ve for reflected)
% INFO structure containing the following fields:
% hemi true if faces are hemispherical (i.e. pass through the origin)
% onesided true is one-sided (like a moebius strip)
% snub true if a snub polyhedron
% This software is based closely on a Mathmatica program described in [1] which, in turn, was based on a
% C program described in [2].
%
% Wythoff Symbol
% p,q,r define a spherical triangle whose angles at the corners are pi/p, pi/q and pi/r;
% this triangle tiles the sphere if repeatedly reflected in its sides. The
% polyhedron vertices are at the reflections of a seed vertex as follows
% where the Vertex configuration gives the polygon orders of the faces around each vertex:
% |p q r : Vertex at a point such that when rotated around any of p,q,r by twice the angle at that
% corner is displaced by the same distance for each corner. This is a snub polyhedron and
% only even numbers of reflections are used to generate vertices. Configuration {3 p 3 q 3 r}
% p|q r : Vertex at p. Configuration = {q r q r ... q r} with 2n terms where n is the numerator of p
% p q|r : Vertex on pq and on the bisector of angle r. Configuration {p 2r q 2r}
% p q r| : Vertex at incentre: meeting point of all the angle bisectors. Configuration {2p 2q 2r}
% |3/2 5/3 3 5/2 This special case is the great dirhombicosidodecahedron. It is a bit weird because
% many of the edges are shared by four faces instead of the usual two.
% If two of p,q,r = 2 then the third is arbitrary (prisms and antiprisms), otherwise only the numerators
% 1:5 can occur, and 4 and 5 cannot occur together. If all are integers then the poyhedron is convex.
%
% References:
% [1] R. E. Maeder. Uniform polyhedra. The Mathematica Journal, 3 (4): 4857, 1993.
% [2] Z. HarEl. Uniform solution for uniform polyhedra. Geometriae Dedicata, 47: 57110, 1993.
% [3] H. S. M. Coxeter, M. S. Longuet-Higgins, and J. C. P. Miller. Uniform polyhedra.
% Philosophical Transactions of the Royal Society A, 246 (916): 401450, May 1954.
% [4] P. W. Messer. Closed-form expressions for uniform polyhedra and their duals.
% Discrete and Computational Geometry, 27 (3): 353375, Jan. 2002.
%%%% BUGS and SUGGESTIONS %%%%%%
% (1) we should ensure the "first" edges of the vertices and faces are consistent
% (2) need to sort faces and vertices into a type order
% (3) should ensure that for a non-chiral polyhedron, the vertex polarity alternates
% (4) w=75 does not work
% (5) dual of henispherical poyhedron
% (6) flist not calculated correctly for duals
% (7) add additional stuff into info.*
% (8) vertex figures seem to include additional (duplicated) lines
% (9) sort out when reflected vertices are really rotationally congruent
% (10) could optionally colour by face type
% (11) order alphabetically by noun
% (12) allow abbreviated names + prisms with preceding decimal number
% (13) calculate correct names
% (14) include names for duals
% (15) make "names" etc persistent
% Example slide show:
% for i=1:74, disp(num2str(i)); upolyhedron(i); pause(2); end
% for i=5:10, disp(num2str(i)); for j=1:5, upolyhedron(i+j/10); pause(2); end, end
% Copyright (C) Mike Brookes 1997
% Version: $Id: upolyhedron.m,v 1.4 2010/04/16 07:44:01 dmb Exp $
%
% VOICEBOX is a MATLAB toolbox for speech processing.
% Home page: http://www.ee.ic.ac.uk/hp/staff/dmb/voicebox/voicebox.html
%
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% This program is free software; you can redistribute it and/or modify
% it under the terms of the GNU General Public License as published by
% the Free Software Foundation; either version 2 of the License, or
% (at your option) any later version.
%
% This program is distributed in the hope that it will be useful,
% but WITHOUT ANY WARRANTY; without even the implied warranty of
% MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
% GNU General Public License for more details.
%
% You can obtain a copy of the GNU General Public License from
% http://www.gnu.org/copyleft/gpl.html or by writing to
% Free Software Foundation, Inc.,675 Mass Ave, Cambridge, MA 02139, USA.
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% [line nn] referes to the kaleido Mathematica program on which this is based
% see http://www.mathconsult.ch/showroom/unipoly/unipoly.html#Images
% Variables used
% adjacent adjacency matrix
% chi characteristic
% cosa cos of the angle subtended by a half edge
% d density
% e number of edges
% even number of even faces to remove
% f number of faces
% fi(n) number of faces of type i
% g order of group
% gamma(n) included spherical triangle angle between centre of face,
% vertex and edge for face type i
% hemiQ =1 for hemispherical faces (includes polyhedron centre)
% incidence faces incident at vertex
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
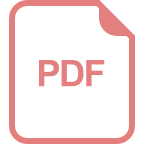
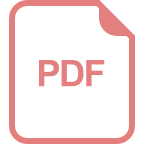
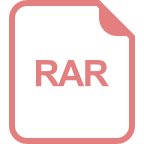
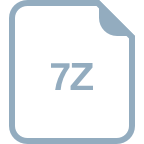
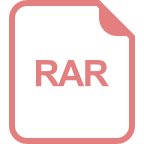
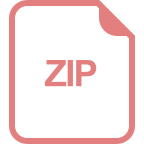
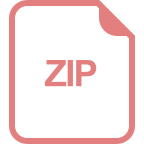
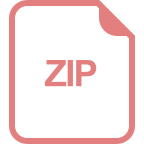
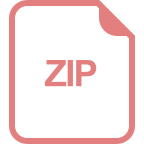
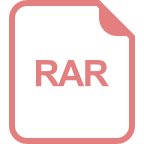
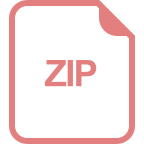
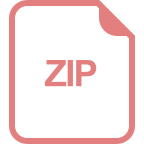
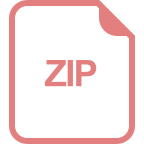
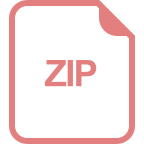
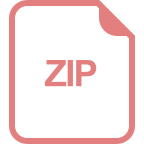
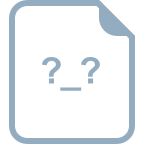
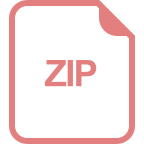
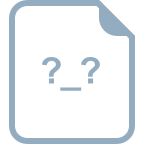
收起资源包目录

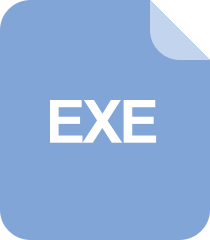
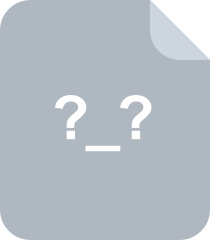
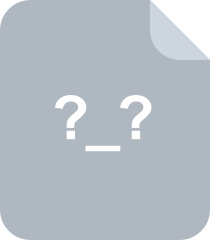
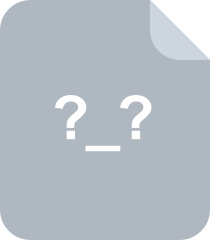
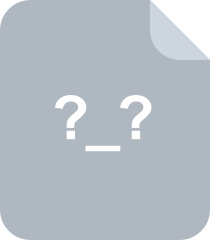
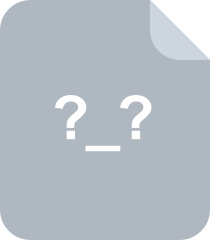
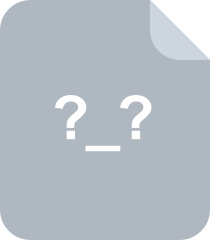
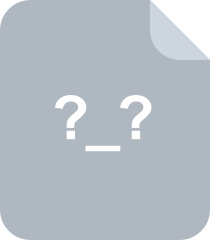
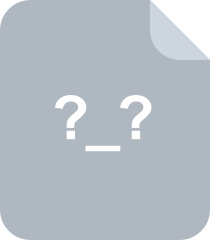
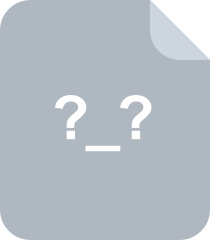
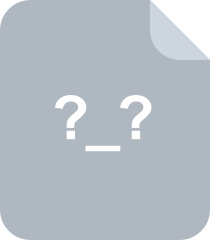
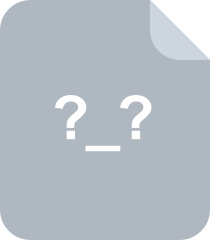
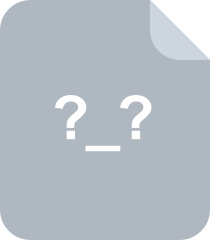
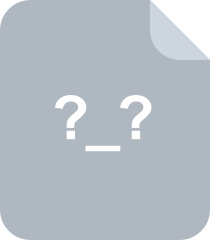
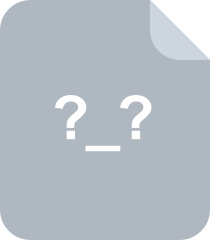
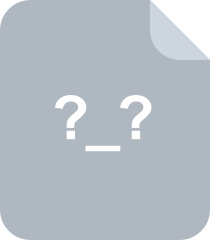
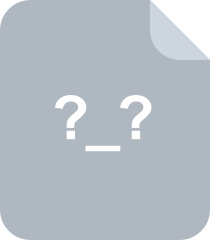
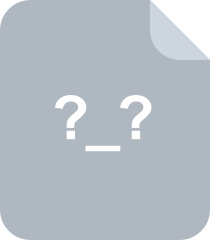
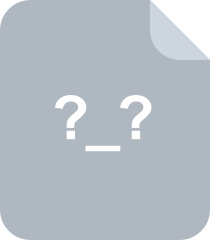
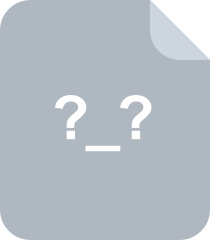
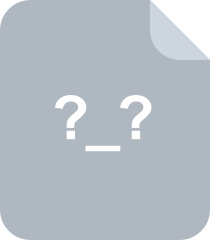
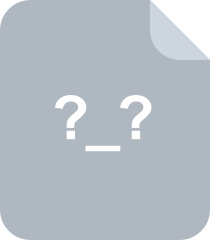
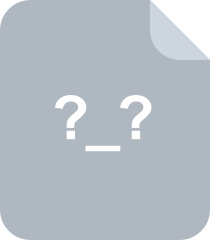
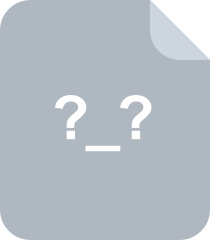
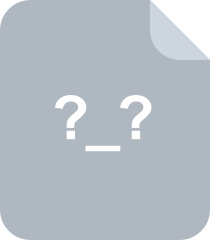
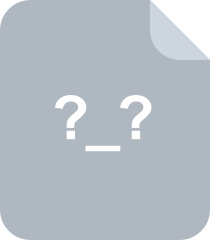
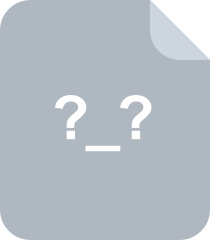
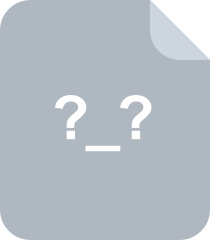
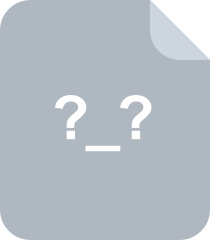
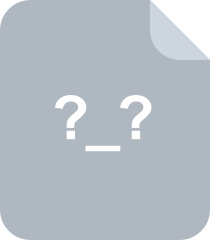
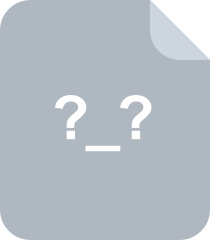
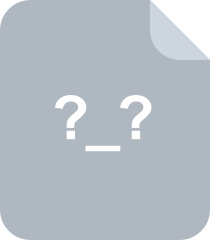
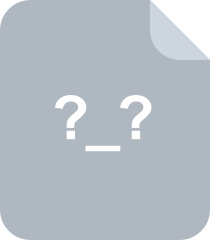
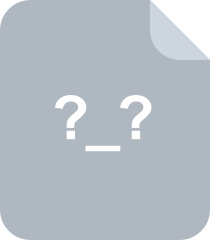
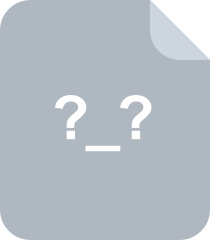
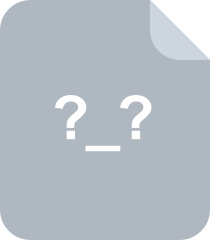
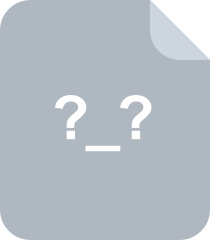
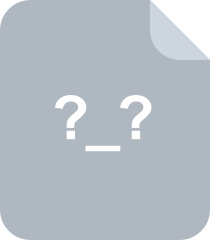
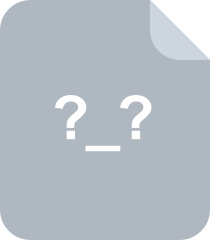
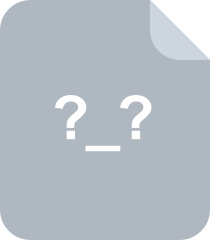
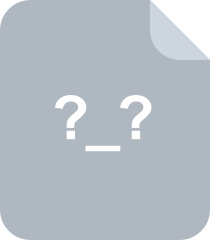
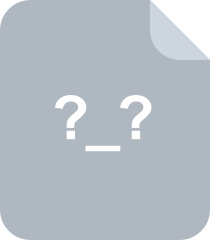
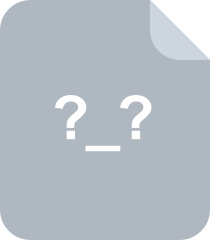
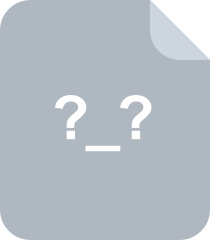
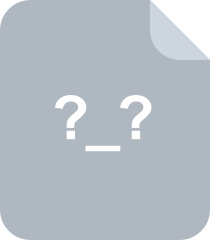
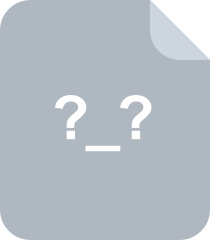
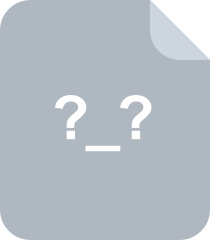
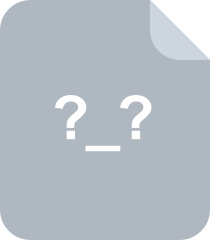
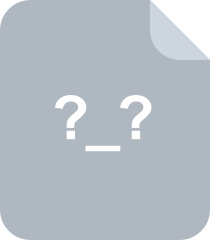
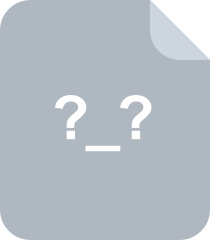
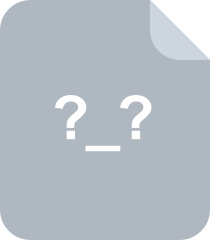
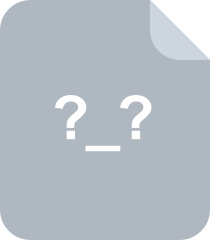
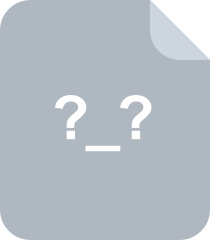
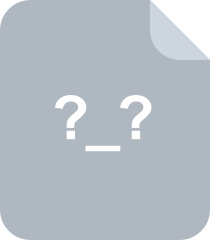
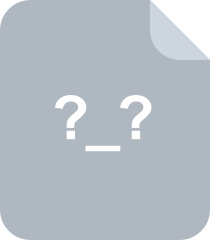
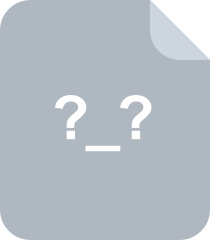
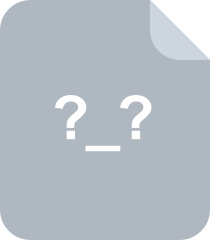
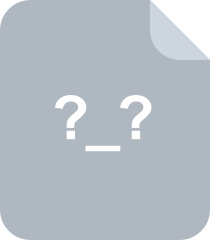
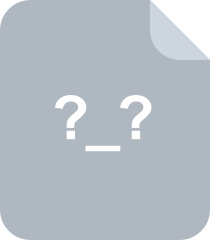
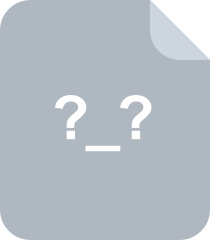
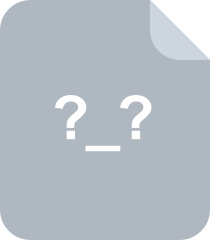
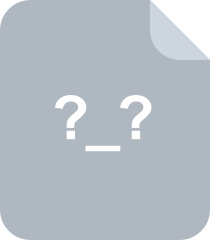
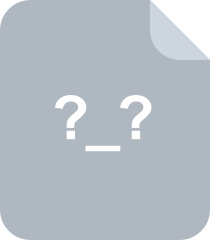
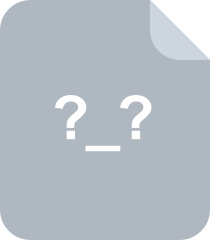
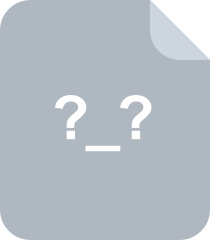
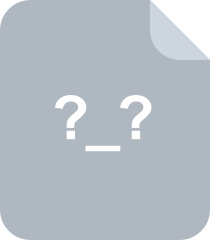
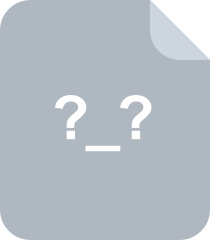
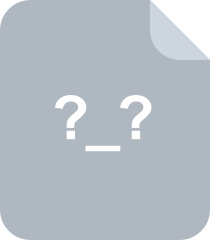
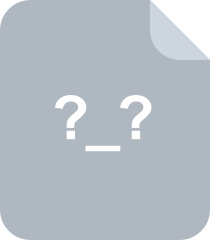
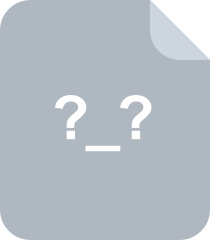
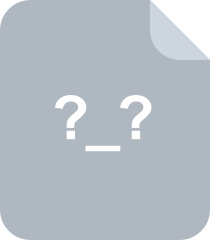
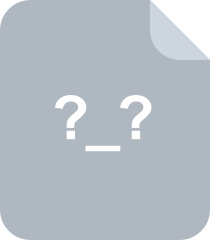
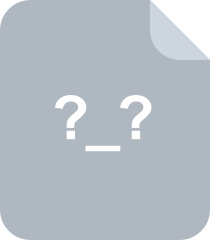
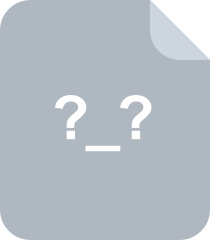
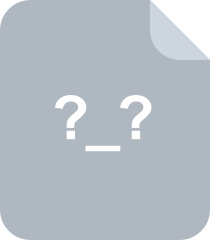
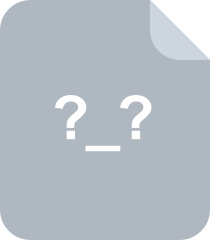
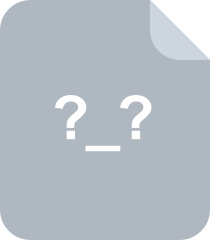
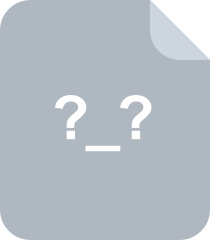
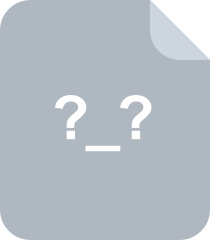
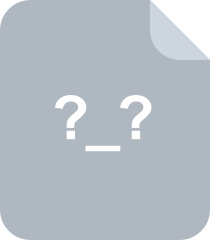
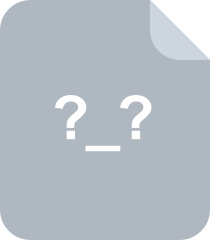
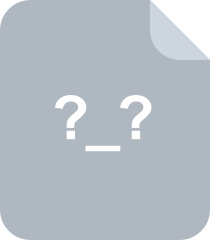
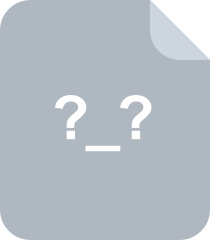
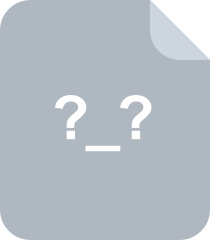
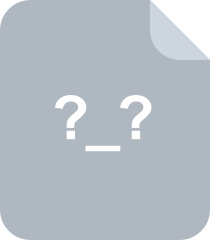
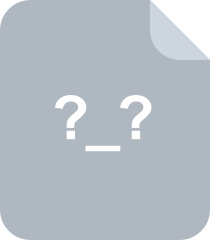
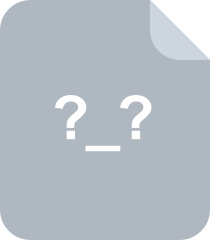
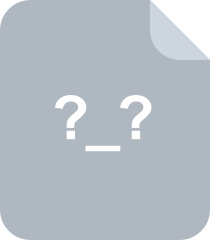
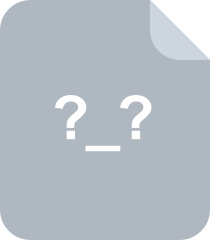
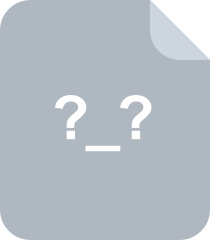
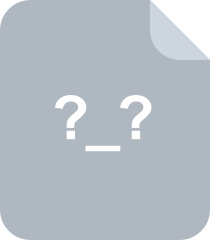
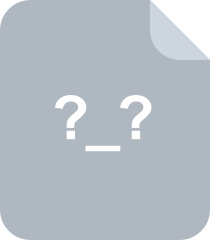
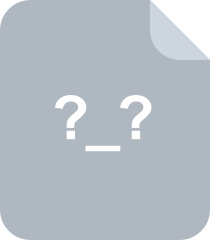
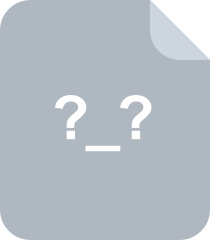
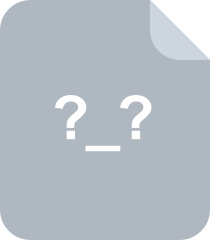
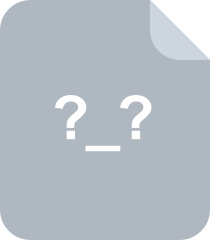
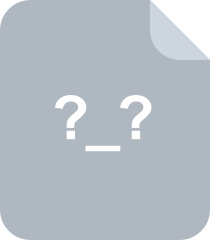
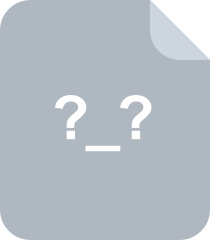
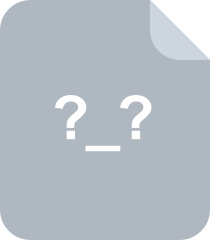
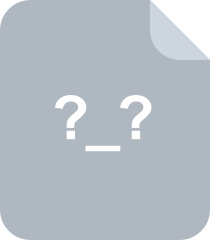
共 262 条
- 1
- 2
- 3

海宝7号
- 粉丝: 9705
- 资源: 124

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

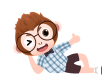
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页