This repository provides multiple pretrained YOLO v8[1] object detection networks for MATLAB®, trained on the COCO 2017[2] dataset. These object detectors can detect 80 different object categories including [person, car, traffic light, etc](/src/%2Bhelper/getCOCOClasess.m). [](https://matlab.mathworks.com/open/github/v1?repo=matlab-deep-learning/Pretrained-YOLOv8-Network-For-Object-Detection)
**Creator**: MathWorks Development
**Includes Codegen support**: ✔
**Includes transfer learning script**: ✔
**Includes Simulink support script**: ✔
## License
The software and model weights are released under the [GNU Affero General Public License v3.0](LICENSE). For alternative licensing, contact [Ultralytics Licensing](https://www.ultralytics.com/license).
## Requirements
- MATLAB® R2024a or later
- Computer Vision Toolbox™
- Deep Learning Toolbox™
- Deep Learning Toolbox Converter for ONNX Model Format
- (optional) Visual Studio C++ compiler for training on Windows
- (optional) MATLAB® Coder for code generation
- (optional) GPU Coder for code generation
## Getting Started
Download or clone this repository to your machine and open it in MATLAB®.
### Download the pretrained network
Use the code below to download the pretrained network.
```matlab
% Load YOLO v8 model
det = yolov8ObjectDetector('yolov8s');
% Analyze loaded model
analyzeNetwork(det.Network);
```
modelName of the pretrained YOLO v8 deep learning model, specified as one of these:
- yolov8n
- yolov8s
- yolov8m
- yolov8l
- yolov8x
Following is the description of various YOLO v8 models available in this repo:
| Model | Description |
|-------------- |--------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| yolov8n | Nano pretrained YOLO v8 model optimized for speed and efficiency. |
| yolov8s | Small pretrained YOLO v8 model balances speed and accuracy, suitable for applications requiring real-time performance with good detection quality. |
| yolov8m | Medium pretrained YOLO v8 model offers higher accuracy with moderate computational demands. |
| yolov8l | Large pretrained YOLO v8 model prioritizes maximum detection accuracy for high-end systems, at the cost of computational intensity. |
| yolov8x | Extra Large YOLOv8 model is the most accurate but requires significant computational resources, ideal for high-end systems prioritizing detection performance. |
### Detect Objects Using Pretrained YOLO v8
To perform object detection on an example image using the pretrained model, utilize the provided code below.
```matlab
% Read test image.
I = imread(fullfile('data','inputTeam.jpg'));
% Load YOLO v8 small network.
det = yolov8ObjectDetector('yolov8s');
% Perform detection using pretrained model.
[bboxes, scores, labels] = detect(det, I);
% Visualize detection results.
annotations = string(labels) + ': ' + string(scores);
Iout = insertObjectAnnotation(I, 'rectangle', bboxes, annotations);
figure, imshow(Iout);
```

## Metrics and Evaluation
### Size and Accuracy Metrics
| Model | Input image resolution | Size (MB) | mAP |
|-------------- |:----------------------:|:---------:|:----:|
| yolov8n | 640 x 640 | 10.7 | 37.3 |
| yolov8s | 640 x 640 | 37.2 | 44.9 |
| yolov8m | 640 x 640 | 85.4 | 50.2 |
| yolov8l | 640 x 640 | 143.3 | 52.9 |
| yolov8x | 640 x 640 | 222.7 | 53.9 |
mAP for models trained on the COCO dataset is computed as average over IoU of .5:.95.
## Transfer learning
Training of a YOLO v8 object detector requires GPU drivers. Additionally, when training on Windows, the Visual Studio C++ compiler is required to facilitate the process.
### Setup
Run `installUltralytics.m` to install the required Python files and set up the Python environment for training YOLOv8. This MATLAB script automates downloading and setting up a standalone Python environment tailored for YOLOv8 training. It determines the system architecture, downloads the appropriate Python build, extracts it, and configures MATLAB settings to use this Python interpreter. Finally, it installs the Ultralytics package and its dependencies using `pip`.
### Obtain data
Use the code below to download the multiclass object detection dataset, or the subsequent steps can be followed to create a custom dataset. The dataset downloaded using the following command will already be in the required format, allowing the [Train YOLO v8 object detector](https://github.com/matlab-deep-learning/Pretrained-YOLOv8-Network-For-Object-Detection/tree/main?tab=readme-ov-file#train-yolo-v8-object-detector) section to be proceeded with directly.
```matlab
helper.downloadMultiClassData()
```
For more information about multiclass object detection dataset, see [Multiclass Object Detection Using YOLO v2 Deep Learning](https://in.mathworks.com/help/vision/ug/multiclass-object-detection-using-yolo-v2-deep-learning.html) example.
### Configure custom data to train YOLO v8 object detector
Refer to `getYOLOFormat.m` to configure custom data to the YOLO format for training the YOLO v8 object detector. The following sections provides more details on the dataset format.
#### Dataset directory structure for custom dataset
When using custom dataset for YOLO v8 training, organize training and validation images and labels as shown in the datasets example directory below. It is mandatory to have both training and validation data to train YOLO v8 network.
<img src="/data/datasetDir.png" alt="datasetDir" width="500"/>
#### Dataset format for custom dataset
Labels for training YOLO v8 must be in YOLO format, with each image having its own *.txt file. If an image contains no objects, a *.txt file is not needed. Each *.txt file should have one row per object in the format: class xCenter yCenter width height, where class numbers start from 0, following a zero-indexed system. Box coordinates should be in normalized xywh format, ranging from 0 to 1. If the coordinates are in pixels, divide xCenter and width by the image width, and yCenter and height by the image height as shown below.
<img src="/data/datasetFormat.png" alt="datasetFormat" width="500"/>
The label file corresponding to the above image contains 3 chairs (class 2).

Refer to the code below to convert bounding box [xCord, yCord, wdt, ht] to [xCenter, yCenter, wdt, ht] format.
```matlab
boxes(:,1) = boxes(:,1) + (boxes(:,3)./2);
boxes(:,2) = boxes(:,2) + (boxes(:,4)./2);
```
Refer to the code below to normalize ground truth bounding box [xCenter, yCenter, wdt, ht] with respect to input image.
```matlab
% Read input image.
[Irow, Icol, ~] = size(inpImage);
% Normalize xCenter and width of ground truth bounding box.
xCenter = xCenter./Icol;
wdt = wdt./Icol;
% Normalize yCenter and height of ground truth bounding box.
yCenter = yCenter./Irow;
ht = ht./Irow;
```
### Train YOLO v8 object detector
Run below code to train YOLO v8 object detector on multiclass object detection dataset. For more information about evaluation, see [Multiclass Object Detection Using YOLO v2 Deep Learning](https://in.mathworks.com/help/vision/ug/multiclass-object-detection-using-yolo-v2-deep-learning.html) example.
```matlab
yolov8Det = trainYOLOv8ObjectDetector('data.yaml','yolov8n.pt', ImageSize=[720 720 3

猰貐的新时代
- 粉丝: 1w+
- 资源: 3014
最新资源
- 21.《银行业数据资产估值指南》.pdf
- 基于有限差分-嵌入式离散裂缝网络(FDM-EDFM)的油气藏地层压力场计算,通过matlab代码实现,可提供理论指导和相关问题,可计算不同裂缝网络的压力分布
- 20.《数字政府一体化建设白皮书》.pdf
- 《算法与数据结构》第四章:栈与队列-循环队列C语言实现
- springboot-vue-在线装修管理网站【源码+sql脚本+29页从零开始图文详解+论文+答辩+环境工具+教程+视频+模板】
- 《算法与数据结构》第四章:栈与队列-链队列C语言实现
- 31.《2024北京“数据要素x”典型案例》.pdf
- 35.《数据资产入表:或成城投转型新出路》.pdf
- 三自由度动力学模型Simulink Carsim; Simulink Carsim联合仿真验证模型; 包括车辆误差跟踪模型; 包括纵滑刚度、侧偏刚度估计方法; 包括详细PPT(22页)流程讲解
- 32.《公共数据授权运营发展洞察》.pdf
- 33.《商业银行数据资产估值研究与入表探索》白皮书.pdf
- Ansys LS-dyna三维混凝土细观骨料模型建模命令(三组连续级配、ITZ0.025mm、体积率可调整,最大趋近于100%)
- mlx90640原厂支持驱动 github少了软硬件IIC的驱动 这边已经补齐
- csdn评论统计工具,exe程序,打开直接使用,防止关进小黑屋
- 考虑实时市场联动的电力零商鲁棒定价策略 考虑电力零商日前定价、日前购电、实时能量管理、电动汽车用户需求响应和电力市场统一出清价格等因素,建立了考虑电动汽车不确定性的电力零商鲁棒定价模型 然后,通过线
- 61节课,零基础Python爬虫48小时速成课-5.7G网盘下载.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


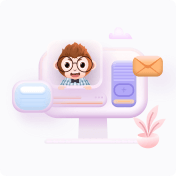