#!/usr/bin/env python 3.7
# -*- coding: utf-8 -*-
# @Time : 2019/5/8 14:18
# @Author : wkend
# @File : charge_category_SVM.py
# @Software: PyCharm
import numpy as np
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
from predict.get_DataSets import get_dataSets
from sklearn.metrics import precision_recall_curve, roc_curve, roc_auc_score
import matplotlib.pyplot as plt
def charge_category_SVM(X, y):
"""利用数据集进行分类"""
X = np.array(X, dtype=float)
y = np.array(y)
# 分离数据集,得到训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=666)
# 对训练、测试数据进行归一化处理
standardScaler = StandardScaler()
standardScaler.fit(X_train)
X_train = standardScaler.transform(X_train) # 对训练数据集进行归一化
X_test_standrad = standardScaler.transform(X_test) # 对测试数据进行归一化
# 寻找超参数
param_grid = [
{
'kernel': ['linear'],
'C': [i for i in range(1, 101)]
},
{
'kernel': ['rbf'],
'gamma': [i for i in range(1, 11)],
'C': [i for i in range(1, 101)]
}
]
# svc = SVC()
# grid_search = GridSearchCV(svc,param_grid,cv=5)
# grid_search.fit(X_train, y_train)
#
# print(grid_search.best_estimator_)
# print(grid_search.best_params_)
#
# svc = grid_search.best_estimator_
# 使用归一化的数据进行分类
svc = SVC(kernel='rbf',C=2,gamma=25)
svc.fit(X_train, y_train)
y_predict = svc.predict(X_test_standrad) # 预测结果向量
decision_scores = svc.decision_function(X_test_standrad) # 决策分数值
# score = svc.score(X_test_standrad, y_test) # 预测准确率
# print('预测准确率:' + str(score))
return y_test, y_predict, decision_scores
def TN(y_true, y_predict):
"""
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回TN值
"""
assert len(y_true) == len(y_predict)
return np.sum((y_true == -1) & (y_predict == -1))
def FP(y_true, y_predict):
"""
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回FP值
"""
assert len(y_true) == len(y_predict)
return np.sum((y_true == -1) & (y_predict == 1))
def FN(y_true, y_predict):
"""
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回FN值
"""
assert len(y_true) == len(y_predict)
return np.sum((y_true == 1) & (y_predict == -1))
def TP(y_true, y_predict):
"""
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回TP值
"""
assert len(y_true) == len(y_predict)
return np.sum((y_true == 1) & (y_predict == 1))
def confusion_matrix(y_true, y_predict):
"""
求混淆矩阵
:param y_true:样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict:样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回混淆矩阵
"""
return np.array([
[TN(y_true, y_predict), FP(y_true, y_predict)],
[FN(y_true, y_predict), TP(y_true, y_predict)],
])
def accuracy_score(y_true, y_predict):
"""
求准确率
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回预测结果的准确率
"""
assert len(y_true) == len(y_predict)
return sum(y_true == y_predict) / len(y_true)
def precision_score(y_true, y_predict):
"""
求精准率
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回预测结果的精准率
"""
tp = TN(y_true, y_predict)
fp = TN(y_true, y_predict)
try:
return tp / (tp + fp)
except:
return 0.0
def recall_score(y_true, y_predict):
"""
求精准率
:param y_true: 样本真实值,为-1表示为恶意软件,为1表示为良性软件
:param y_predict: 样本预测值,为-1表示预测为恶意软件,为1表示预测为良性软件
:return: 返回预测结果的精准率
"""
tp = TN(y_true, y_predict)
fn = FN(y_true, y_predict)
try:
return tp / (tp + fn)
except:
return 0.0
def f1_score(precision, recall):
"""
求精准率和召回率的调和平均值
:param precision: 精准率
:param recall: 召回率
:return: 精准率和召回率的调和平均值
"""
try:
return 2 * precision * recall / (precision + recall)
except:
return 0.0
def make_PR_curve(y_test, decision_scores):
"""绘制PR曲线"""
precisions, recalls, thresholds = precision_recall_curve(y_test, decision_scores)
plt.plot(thresholds, precisions[:-1], label="precisions",linestyle='--')
plt.plot(thresholds, recalls[:-1], label="recalls")
plt.legend()
plt.show()
def make_PR_balance_curve(y_test, decision_scores):
"""绘制PR平衡曲线"""
precisions, recalls, thresholds = precision_recall_curve(y_test, decision_scores)
plt.plot(precisions, recalls)
plt.xlabel('precision')
plt.ylabel('recall')
plt.show()
def make_roc_curve(y_test, decision_scores):
"""绘制ROC曲线"""
fprs, tprs, thresholds = roc_curve(y_test, decision_scores)
plt.plot(fprs, tprs)
plt.xlabel('FPR')
plt.ylabel('TPR')
plt.show()
if __name__ == '__main__':
malware_path = 'G:/毕设/软件样本库/恶意样本/decompile'
benign_path = 'G:/毕设/软件样本库/良性样本/decompile'
# path = 'E:/毕设/软件样本库/恶意软件/test'
malware_dataSets = get_dataSets(malware_path)
# print(len(malware_dataSets)) # 1260
benign_dataSets = get_dataSets(benign_path)
# print(len(benign_dataSets)) # 1184
malware_dataSets.extend(benign_dataSets)
dataSets = malware_dataSets
# print(dataSets)
category = [-1] * 1260 + [1] * 1184
# print(category)
y_test, y_predict, decision_scores = charge_category_SVM(dataSets, category)
# print(y_test)
# print(y_predict)
# print(decision_scores)
confusion_matrix = confusion_matrix(y_test, y_predict)
print(confusion_matrix)
accuracy_score = accuracy_score(y_test, y_predict)
print('SVM准确率:' + str(accuracy_score))
precision_score = precision_score(y_test, y_predict)
print('SVM精准率:' + str(precision_score))
recall_score = recall_score(y_test, y_predict)
print('SVM召回率:' + str(recall_score))
f1_score = f1_score(precision_score, recall_score)
print('SVM F1 Score:' + str(recall_score))
# 绘制PR曲线
make_PR_curve(y_test, decision_scores)
# 绘制PR平衡曲线
# make_PR_balance_curve(y_test, decision_scores)
# # 绘制ROC曲线
# make_roc_curve(y_test, decision_scores)
# ROC曲线面积
roc_auc_score = roc_auc_score(y_test, decision_scores)
print('ROC曲线面积:

龙年行大运
- 粉丝: 1384
- 资源: 3960
最新资源
- 汇川H3U标准程序,程序有本体脉冲控制的三轴定位,有总线控制的汇川伺服定位,轴点动,回零,相对定位绝对定位,程序结构清晰,分模块控制,是工控者学习的好案例
- 松下6轴程序模板 1:plc采用FP-XHC60T ,标准可带6轴程序 2:昆仑通态触摸屏程序(触摸屏附带配方功能,以及产能统计:) 3:项目各种功能完整 该程序为标准框架, a.故障, b.复
- MATLAB Elman神经网络数据预测,BP神经网络预测,电力负荷预测模型研究 负荷预测的核心问题是预测的技术问题,或者说是预测的数学模型 传统的数学模型是用显示的数学表达式加以描述,具有计算量小
- 驱动FOC 电机学习FOC控制 高频注入 推理过程和代码实现以及原理图 FOC矢量控制 FOC驱动无刷驱动foc无刷电机驱动方式学习 可用于驱动无刷电机,永磁同步电机 FOC框架、坐标变、SVPWM
- MATLAB基于卡尔曼滤波的锂蓄电池SOC设计 用自适应卡尔曼滤波方法,基于锂离子动力电池等效电路模型,在未知干扰噪声环境下,在线估计电动汽车锂离子动力电池荷电状态 (SOC) 采用基本卡尔曼滤波和
- 风光储交流微网(双向储能变流器) 含: 1.永磁直驱风机+mppt+整流+并网逆变 mppt采用扫描搜索法 整流采用转速外环电流内环双闭环控制 并网逆变采用电压外环电流内环控制 满功率运行 2.PV+
- 西门子200smart模拟量处理模块带滤波,带报警 好用的200smart模拟量输入处理程序,已经封装成可直接调用程序 功能: 处理电压,电流或者RTD模拟量输入信号,把输入通道值转化为模拟量值,且
- 纯电动汽车动力经济性仿真,Cruise和Simulink联合仿真,提供Cruise整车模型和simuink策略模型,策略主要为BMS、再生制动和电机驱动策略,内含注释模型和详细解析文档
- 微观孔隙建模插件,可指定生成任意孔隙率的随机孔隙模型 模型可导入comsol、abaqus、ansys、fluent、ls dyna等有限元软件进行模拟 孔隙渗流、多孔介质电流模拟、多孔材料等
- 汇川四轴机械手电芯上下料程序 汇川ROBOT机器人电芯自动上料程序,与PLC信号对接,进行电芯取放料控制,待机位,安全位,矩阵料盘控制,多个NG位,扫码位,运动控制,左手势,右手势,程序注释齐全 附
- MATLAB代码:基于二阶锥优化及OLTC档位选择的配电网优化调度 关键词:OLTC档位选择 二阶锥优化 动态优化 最优潮流 参考文档:《主动配电网最优潮流研究及其应用实例》仅参考部分模型,非完全复
- Abaqus三维随机生成圆形骨料python代码~ 随机圆形骨料-互不相交,随机生成半径不同的圆形实体(符合混凝土当中包含圆形骨料等现实问题) 可调节混凝土边界,长,宽,高 ,随机生成圆形骨料 ,半
- MATLAB代码:基于多目标粒子群算法冷热电联供综合能源系统运行优化 关键词:综合能源 冷热电三联供 粒子群算法 多目标优化 参考文档:《基于多目标算法的冷热电联供型综合能源系统运行优化》 仿真平台
- MATLAB代码:基于蒙特卡洛算法的电动汽车充电负荷预测 关键词:蒙特卡洛 电动汽车 充电负荷预测 参考文档:《电动汽车充电负荷预测方法及应用研究》参考第3.2节,完全复现; 仿真平台:MATL
- MATLAB代码:基于多目标遗传算法的分布式电源选址定容研究 关键词:分布式电源 选址定容 多目标遗传算法 参考文档:《店主自写文档》基本复现; 仿真平台:MATLAB 主要内容:代码主要做的
- 四轮轮毂电机驱动智能电动汽车转向失效容错控制研究 上层控制器首先基于时变线性模型预测控制方法求解期望前轮转角和附加横摆力矩,然后考虑转向执行机构建模不确定性以及路面干扰,设计基于滑模变
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


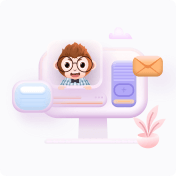