package com.test.dao.file_dir;
import java.io.File;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
import java.util.Stack;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JOptionPane;
import com.test.db.DBManage;
import com.test.tools.Tools;
import com.test.ui.FilePanel;
/**
* 文件操作管理类
* @author asus
*
*/
public class FileManager {
// 文件分割
public FileSeperate fileSeperate ;
// 文件合并
public FileCombination fileCombination ;
// 删除文件时用到的栈
private Stack<Integer> deleteFileStack = new Stack<Integer>() ;
// 数据库操作
public DBManage dbmanage ;
public Connection connection ;
public PreparedStatement preparedStatement ;
public ResultSet resultSet ;
public HdfsTools hdfsTool ;
public FileManager(DBManage dbmanage) {
this.dbmanage = dbmanage ;
this.hdfsTool = new HdfsTools() ;
}
public FileManager() {
this.dbmanage = new DBManage() ;
this.hdfsTool = new HdfsTools() ;
}
// 显示文件列表
public void showFile(Icon icon , String fileName) {
Tools.loginSuccess.filePanel = new FilePanel(icon , fileName) ;
Tools.loginSuccess.fileBottomPanel.add(Tools.loginSuccess.filePanel) ;
Tools.loginSuccess.repaint() ;
}
// 显示文件列表
public void showFileList(int parentId , int userId) {
try {
String showFile = "select * from file where parent_id = ? and u_id = ? order by f_type DESC" ;
this.connection = this.dbmanage.getConnection() ;
// this.preparedStatement = this.dbmanage.getPreparedStatement(Tools.SHOW_FILE) ;
this.preparedStatement = this.dbmanage.getPreparedStatement(showFile) ;
this.preparedStatement.setInt(1, parentId) ;
this.preparedStatement.setInt(2, userId) ;
this.resultSet = this.preparedStatement.executeQuery() ;
while(this.resultSet.next()) {
// 取得文件名
String fileName = this.resultSet.getString("f_name") ;
String filetype = this.resultSet.getString("f_type") ;
// 显示文件图标
if(filetype.equals("文件")) {
this.showFile(new ImageIcon("image/file.png") , fileName) ;
}else if(filetype.equals("文件夹")) {
this.showFile(new ImageIcon("image/dir.png") , fileName) ;
}
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
// 关闭连接
if(this.resultSet != null) {
try {
this.resultSet.close() ;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
this.dbmanage.closeLink() ;
}
}
// 创建文件夹
public void createDir() {
//随机产生1000以内的命名规则
String filename = "新建文件夹"+ (int)(Math.random()*1000);
Date date = new Date();
String type = "文件夹";
long size = 0 ;
//随机产生的文件名若重名,则继续随机产生,知道不重名为止
while (!checkFileName(filename)) {
filename = "新建文件夹"+ (int)(Math.random()*1000);
}
try {
String createDir = "insert into File (u_id , f_name , f_date , f_type , f_size , parent_id) values(? , ? , ? , ? , ? , ?)" ;
this.connection = this.dbmanage.getConnection() ;
// this.preparedStatement = this.dbmanage.getPreparedStatement(Tools.CREATE_DIR) ;
this.preparedStatement = this.dbmanage.getPreparedStatement(createDir) ;
this.preparedStatement.setInt(1, Tools.userId) ;
this.preparedStatement.setString(2, filename) ;
this.preparedStatement.setString(3, date.toString()) ;
this.preparedStatement.setString(4, type) ;
this.preparedStatement.setLong(5, size) ;
this.preparedStatement.setInt(6, Tools.parentIdStack.lastElement()) ;
this.preparedStatement.execute() ;
// 新建的文件夹在界面上显示出来
Tools.loginSuccess.filePanel = new FilePanel(new ImageIcon("image/dir.png") , filename) ;
Tools.loginSuccess.fileBottomPanel.add(Tools.loginSuccess.filePanel) ;
Tools.loginSuccess.repaint();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
// 关闭连接
if(this.resultSet != null) {
try {
this.resultSet.close() ;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
this.dbmanage.closeLink() ;
}
}
/**
* 检查文件是否重名,通过文件类型和文件名查询
*/
public boolean checkFileName(String filename) {
try {
String checkFileName = "select * from File where u_id = ? and f_name = ? and parent_id = ?" ;
this.connection = this.dbmanage.getConnection() ;
// this.preparedStatement = this.dbmanage.getPreparedStatement(Tools.CHECK_FILE_NAME) ;
this.preparedStatement = this.dbmanage.getPreparedStatement(checkFileName) ;
this.preparedStatement.setInt(1, Tools.userId) ;
this.preparedStatement.setString(2, filename) ;
this.preparedStatement.setInt(3, Tools.parentIdStack.lastElement()) ;
this.resultSet = this.preparedStatement.executeQuery() ;
while(this.resultSet.next()) {
return false ;
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false ;
} finally {
// 关闭连接
if(this.resultSet != null) {
try {
this.resultSet.close() ;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
this.dbmanage.closeLink() ;
}
return true ;
}
/**
* 检查文件是否重名,通过文件ID号查询
*/
public boolean checkPasteFileName() {
try {
String checkPasteFileName = "select * from File where f_name = (select f_name from File where f_id = ?) and u_id = ? and parent_id = ?" ;
this.connection = this.dbmanage.getConnection() ;
// this.preparedStatement = this.dbmanage.getPreparedStatement(Tools.CHECK_PASTE_FILE_NAME) ;
this.preparedStatement = this.dbmanage.getPreparedStatement(checkPasteFileName) ;
this.preparedStatement.setInt(1, Tools.cutFileId) ;
this.preparedStatement.setInt(2, Tools.userId) ;
this.preparedStatement.setInt(3, Tools.parentIdStack.lastElement()) ;
this.resultSet = this.preparedStatement.executeQuery() ;
if(this.resultSet.next()) {
JOptionPane.showMessageDialog(null, "该文件夹已存在,不能粘贴", "警告信息", 1);
return false ;
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false ;
} finally {
// 关闭连接
if(this.resultSet != null) {
try {
this.resultSet.close() ;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
this.dbmanage.closeLink() ;
}
return true ;
}
/**
* 打开文件夹
*/
public boolean openDir(String filename) {
try {
String queryFileId = "select * from File where u_id = ? and f_name = ? and parent_id = ?" ;
this.connection = this.dbmanage.getConnection() ;
// this.preparedStatement = this.dbmanage.getPreparedStatement(Tools.QUERY_FILE_ID) ;
this.preparedStatement = this.dbmanage.getPreparedStatement(queryFileId) ;
this.preparedStatement.setInt(1, Tools.userId) ;
this.preparedStatement.setString(2, filename) ;
this.preparedStatement.setInt(3, Tools.parentIdStack.lastElement()) ;
this.resultSet = this.preparedStatement.executeQuery() ;
int fileId = -1;
String filetype = null ;
if(this.resultSet.next()) {
fileId = this.resultSet.getInt("f_id") ;
filetype = this.resultSet.getString("f_type") ;
}
// 双击打开文件夹
if(filetype != null && filetype.equals("文件夹")) {
Tools.parentIdStack.add(fileId) ;
Tools.loginSuccess.fileBottomPanel.removeAll() ;
this.showFileList(Tools.parentIdStack.lastElement(), Tools.userId) ;
Tools.loginSuccess.fileBottomPanel.repaint() ;
// 设置文件路径
String context = Tools.loginSuccess.search.getText() ;
Tools.loginSuccess.search.setText(context + "\\" + filename) ;
}else if(filet
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Hadoop的分布式文件系统,使用Java语言开发实现了一个本地文件管理系统,其中文件存在于HDFS集群中,通过Java开发的客户端软件进行管理,其功能包括:1、文件分块、加密并上传待HDFS文件系统 2、文件块下载、解密、整合成完整文件 3、文件系统的管理 1、资源内容: 2、代码特点:内含运行结果,不会运行可私信,参数化编程、参数可方便更改、代码编程思路清晰、注释明细,都经过测试运行成功,功能ok的情况下才上传的。 3、适用对象:计算机,电子信息工程、数学等专业的大学生课程设计、期末大作业和毕业设计。 4、作者介绍:某大厂资深算法工程师,从事Matlab、Python、C/C++、Java、YOLO算法仿真工作10年;擅长计算机视觉、 目标检测模型、智能优化算法、神经网络预测、信号处理、元胞自动机、图像处理、智能控制、路径规划、无人机等多种领域的算法仿真实验,更多源码,请上博主主页搜索。 -------------------------------------------------------------------------- -
资源推荐
资源详情
资源评论
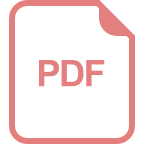
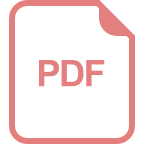
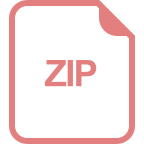
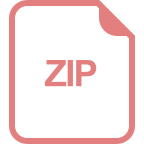
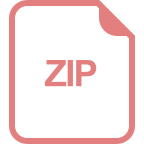
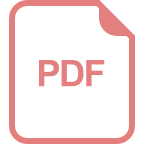
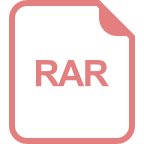
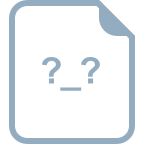
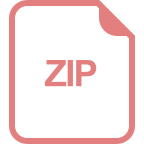
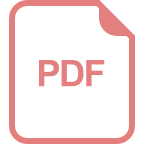
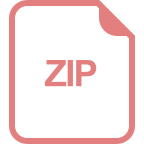
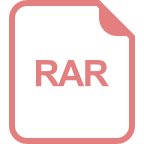
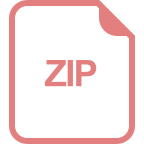
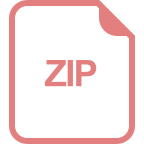
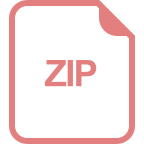
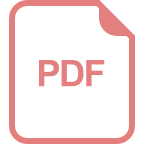
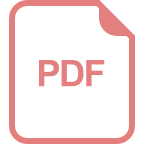
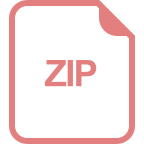
收起资源包目录

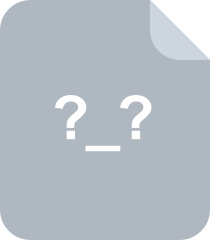
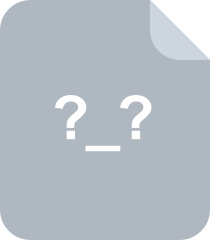
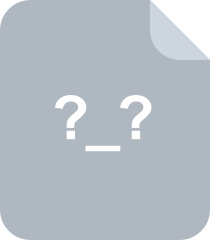
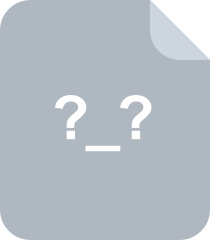
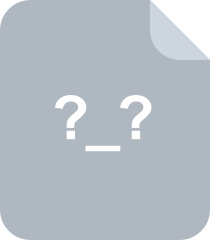
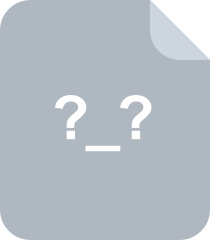
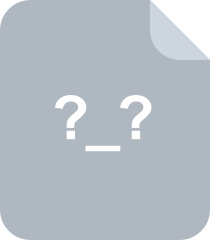
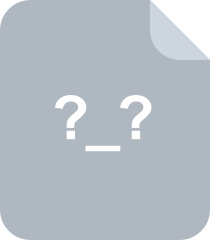
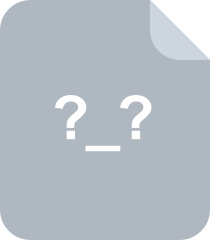
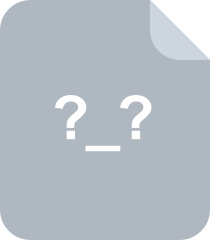
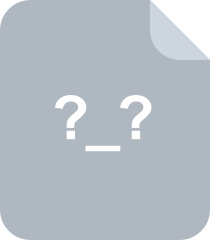
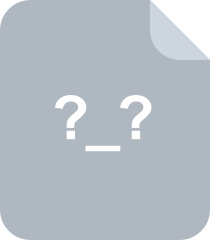
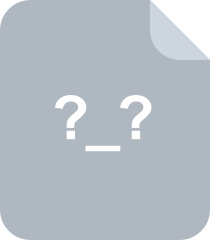
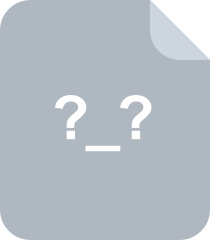
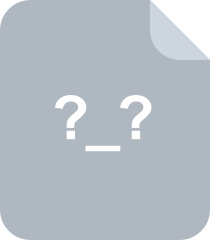
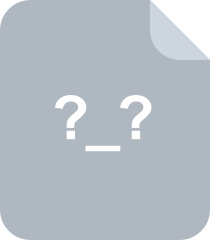
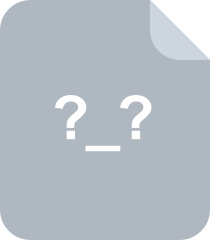
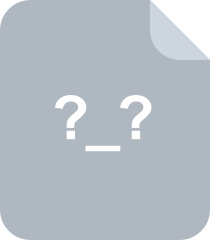
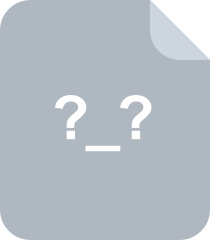
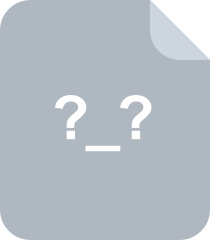
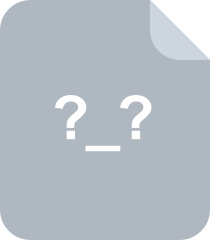
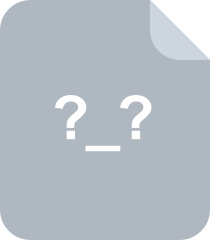
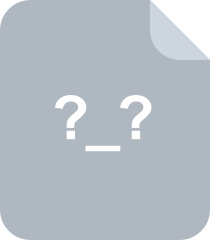
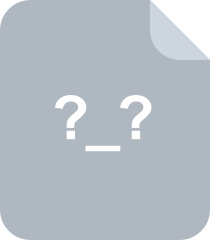
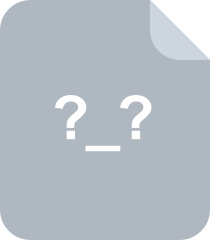
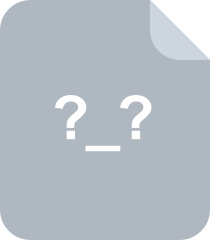
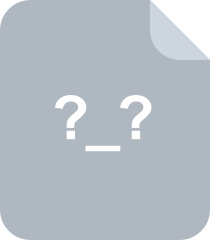
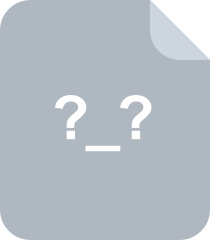
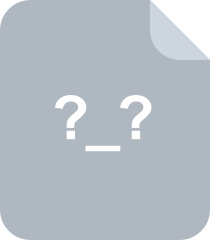
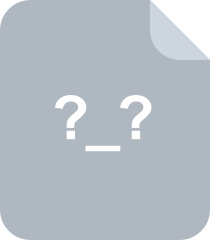
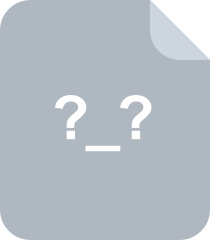
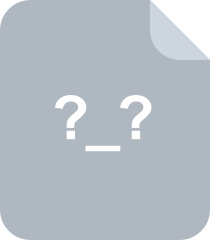
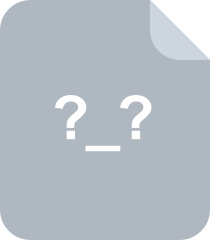
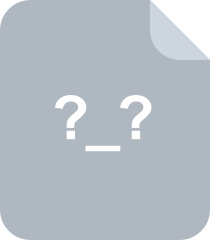
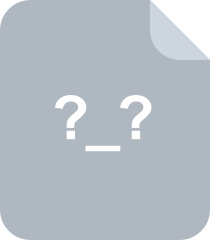
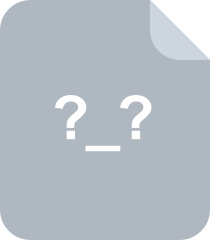
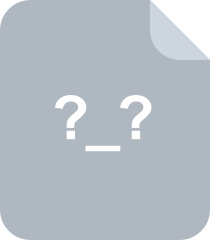
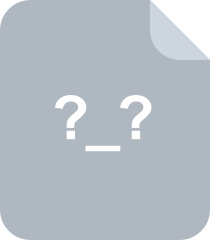
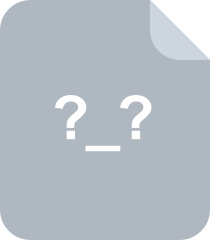
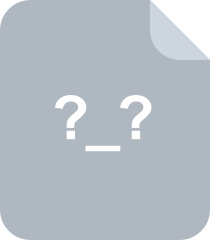
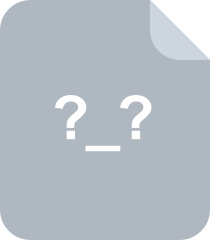
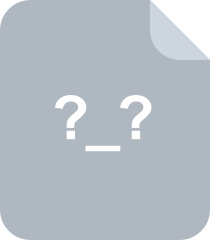
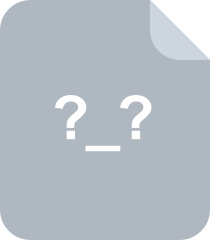
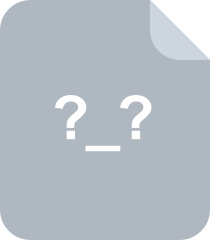
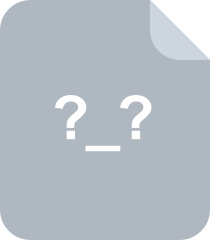
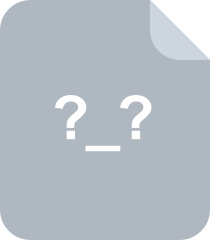
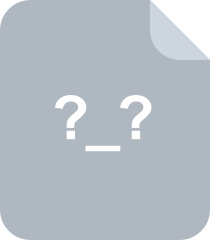
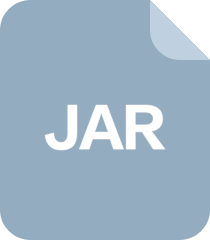
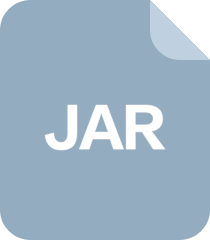
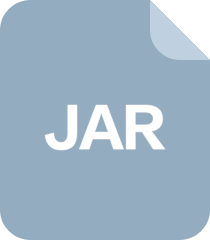
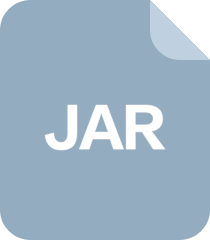
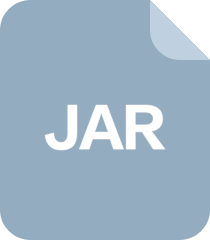
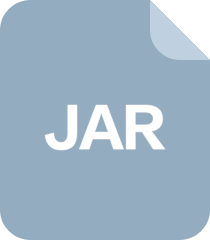
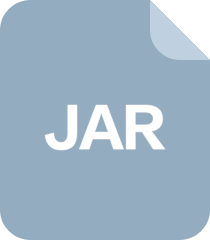
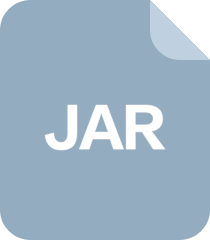
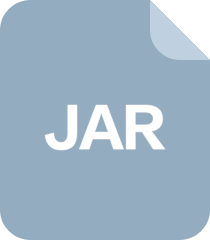
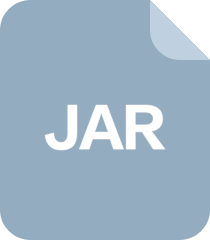
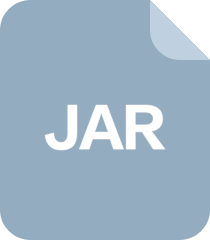
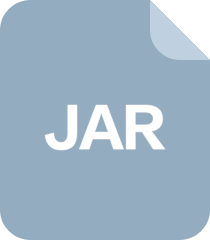
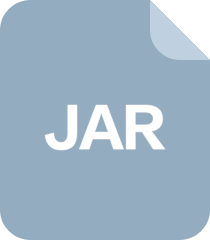
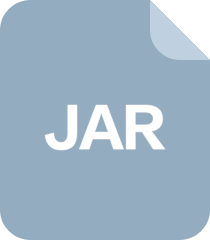
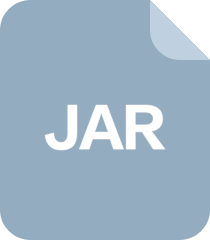
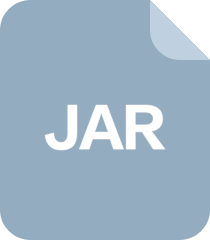
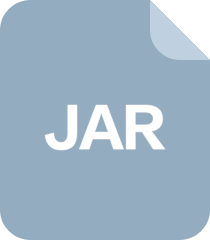
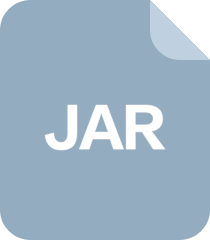
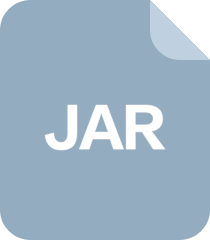
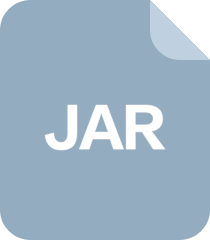
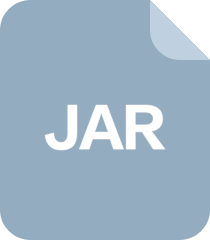
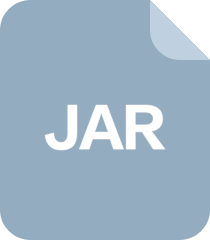
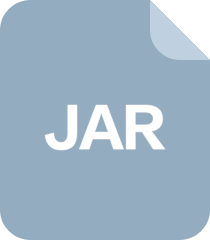
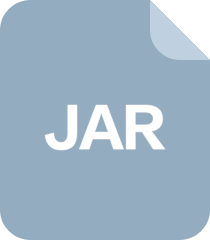
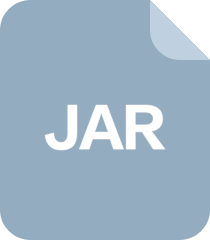
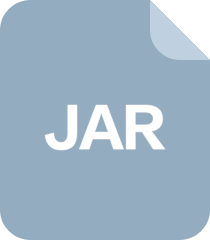
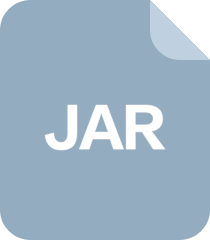
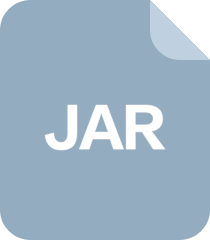
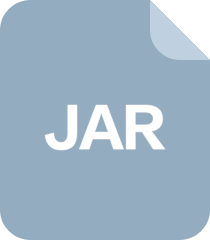
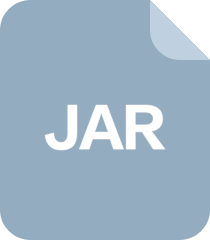
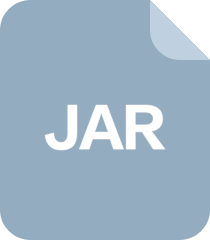
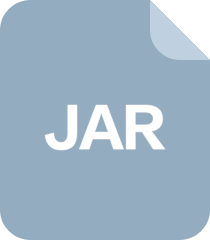
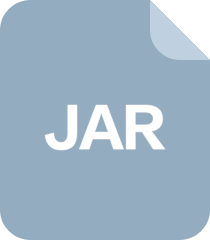
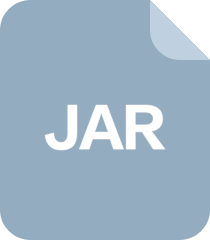
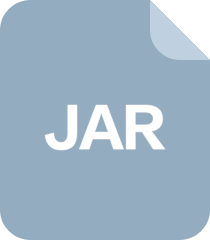
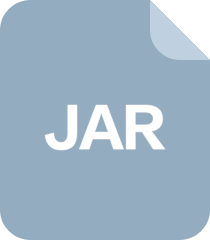
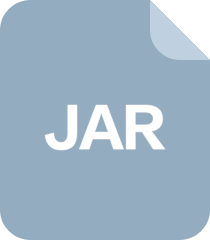
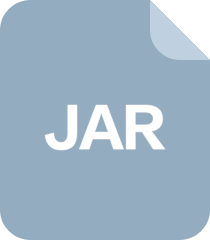
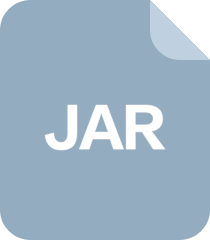
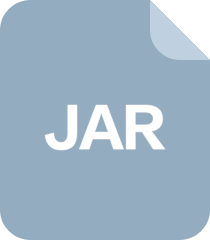
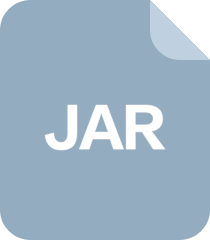
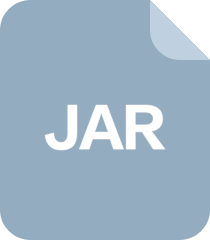
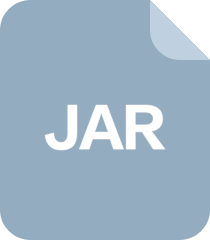
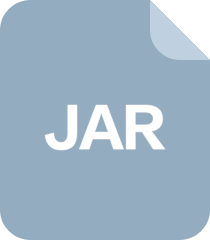
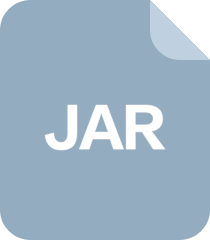
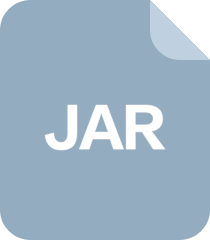
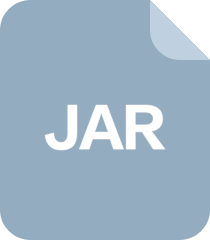
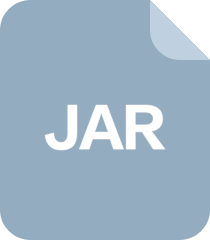
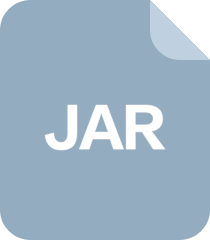
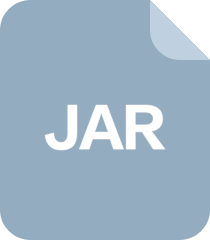
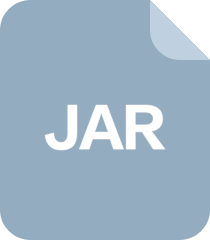
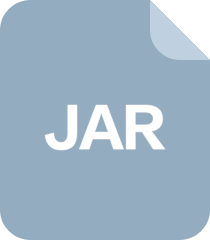
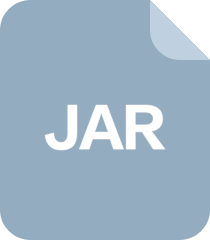
共 191 条
- 1
- 2
资源评论
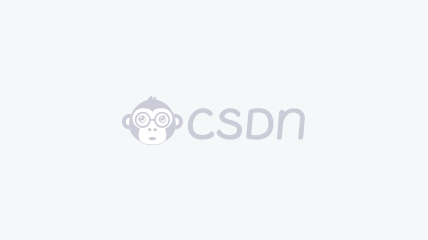

机器学习的喵
- 粉丝: 486
- 资源: 1252
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

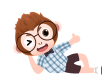
最新资源
- XILINXFPGA源码Xilinxspratan3xcs100E(VGAPS2)
- XILINXFPGA源码XilinxSPARTAN-3E入门开发板实例
- XILINXFPGA源码XilinxSdramVerilog和VHDL版本文档
- 物联网智能家居方案-基于Nucleo-STM32L073&机智云(大赛作品,文档齐全,可直接运行)(文档加Matlab源码)
- XILINXFPGA源码XilinxISE9.xFPGACPLD设计源码
- 成都市地图含高新区(高新南区,高新西区),天府新区,东部新区虚拟行政区划
- XILINXFPGA源码XilinxEDK设计试验
- XILINXFPGA源码XilinxEDKMicroBlaze内置USB固件程序
- 基于 django 的视频点播后台管理系统源代码+数据库
- 基于Java的网上医院预约挂号系统的设计与实现(部署视频)-kaic.mp4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


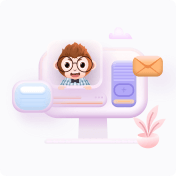
安全验证
文档复制为VIP权益,开通VIP直接复制
