classdef Particle
properties
x
l
u
v
cost
infeasablity
pBest
pBestCost
pBestinfeasablity
GridIndex
isDominated
end
methods
function obj = Particle(lower,upper,problem)
if nargin > 0
obj.GridIndex = 0;
obj.isDominated = false;
obj.x = unifrnd(lower,upper);
obj.l = lower;
obj.u = upper;
obj.v = zeros(1,max(length(lower),length(upper)));
[obj.cost, obj.infeasablity] = problem(obj.x);
obj.pBest = obj.x;
obj.pBestCost = obj.cost;
obj.pBestinfeasablity = obj.infeasablity;
end
end
function obj = update(obj,w,c,pm,gBest,problem)
obj = obj.updateV(w,c,gBest);
obj = obj.updateX();
[obj.cost, obj.infeasablity] = problem(obj.x);
obj = obj.applyMutatation(pm,problem);
obj = obj.updatePbest();
end
function obj = updateV(obj,w,c,gBest)
obj.v = w.*obj.v + c(1).*rand.*(obj.pBest-obj.x) + c(2).*rand.*(gBest.x-obj.x);
end
function obj = updateX(obj)
i=find(or(obj.x+obj.v>obj.u,obj.x+obj.v<obj.l));
obj.v(i) = -obj.v(i);
obj.x = max(min(obj.x+obj.v,obj.u),obj.l);
end
function obj = updatePbest(obj)
if obj.infeasablity == 0
if obj.pBestinfeasablity > 0
obj.pBest = obj.x;
obj.pBestCost = obj.cost;
obj.pBestinfeasablity = obj.infeasablity;
elseif all(obj.pBestCost >= obj.cost) && any(obj.pBestCost > obj.cost)
obj.pBest = obj.x;
obj.pBestCost = obj.cost;
obj.pBestinfeasablity = obj.infeasablity;
end
else
if obj.pBestinfeasablity >= obj.infeasablity
obj.pBest = obj.x;
obj.pBestCost = obj.cost;
obj.pBestinfeasablity = obj.infeasablity;
end
end
end
function obj = applyMutatation(obj,pm,problem)
if rand<pm
X=obj.Mutate(pm);
[X.cost,X.infeasablity]=problem(X.x);
if X.dominates(obj)
obj=X;
elseif ~obj.dominates(X)
if rand<0.5
obj=X;
end
end
end
end
function obj=Mutate(obj,pm)
nVar=numel(obj.x);
j=randi([1 nVar]);
dx=pm*(obj.u(j)-obj.l(j));
lb=max(obj.x(j)-dx,obj.l(j));
ub=min(obj.x(j)+dx,obj.u(j));
obj.x(j)=unifrnd(lb,ub);
end
function d = dominates(obj,obj1)
if obj.infeasablity == 0
if obj1.infeasablity == 0
if all(obj.cost <= obj1.cost) && any(obj.cost < obj1.cost)
d = true;
else
d = false;
end
else
d = true;
end
elseif obj1.infeasablity == 0
d = false;
elseif obj.infeasablity < obj1.infeasablity
d = true;
else
d = false;
end
end
function obj=updateGridIndex(obj,Grid)
nObj=length(obj.cost);
nGrid=length(Grid(1).LB);
GridSubIndex=zeros(1,nObj);
for j=1:nObj
GridSubIndex(j)=find(obj.cost(j)<Grid(j).UB,1,'first');
end
obj.GridIndex=GridSubIndex(1);
for j=2:nObj
obj.GridIndex=obj.GridIndex-1;
obj.GridIndex=nGrid*obj.GridIndex;
obj.GridIndex=obj.GridIndex+GridSubIndex(j);
end
end
end
methods (Static)
function swarm = updateDomination(swarm)
for index = 1:length(swarm)
swarm(index).isDominated = false;
for i = 1:length(swarm)
if i == index
continue
end
if swarm(i).dominates(swarm(index))
swarm(index).isDominated = true;
break
end
end
end
end
end
end
没有合适的资源?快使用搜索试试~ 我知道了~
基于多目标粒子群优化算法的冷热电联供型综合能源系统运行优化Matlab代码.rar
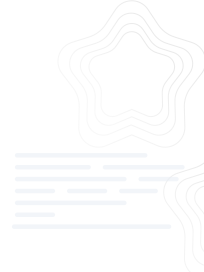
共23个文件
mat:10个
m:7个
jpg:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 160 浏览量
2024-09-22
19:13:24
上传
评论
收藏 2.55MB RAR 举报
温馨提示
1.版本:matlab2014/2019a/2024a 2.附赠案例数据可直接运行matlab程序。 3.代码特点:参数化编程、参数可方便更改、代码编程思路清晰、注释明细。 4.适用对象:计算机,电子信息工程、数学等专业的大学生课程设计、期末大作业和毕业设计。 替换数据可以直接使用,注释清楚,适合新手
资源推荐
资源详情
资源评论
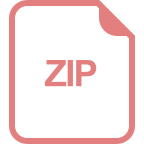
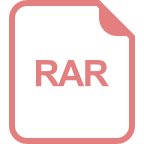
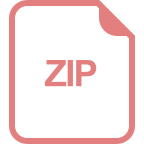
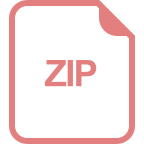
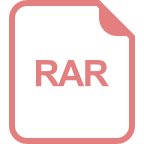
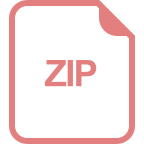
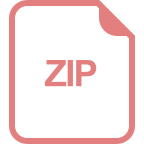
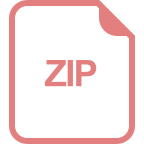
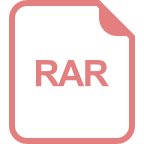
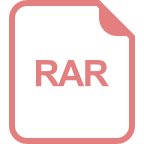
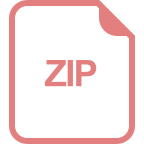
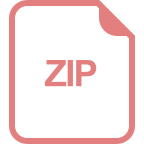
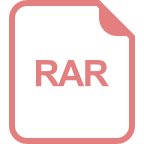
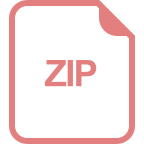
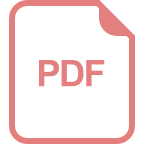
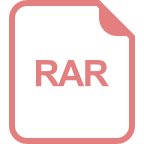
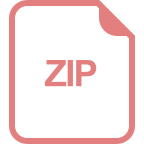
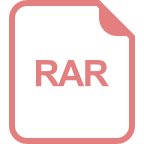
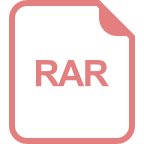
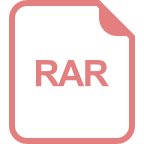
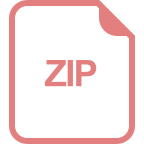
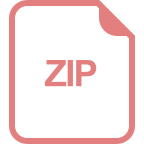
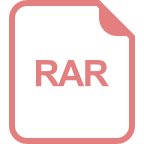
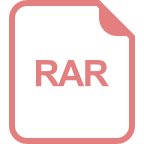
收起资源包目录


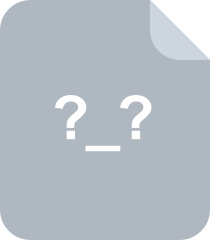
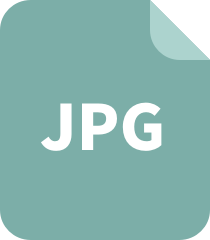
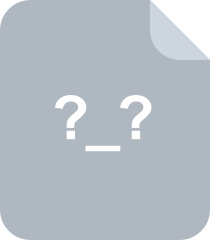
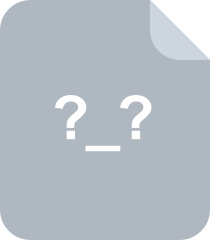
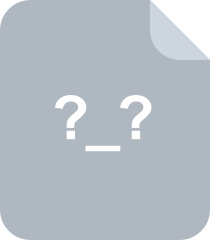
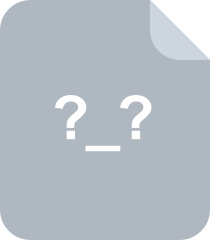
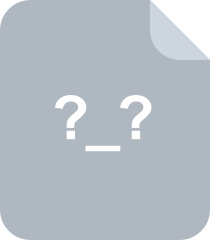
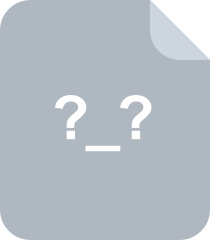
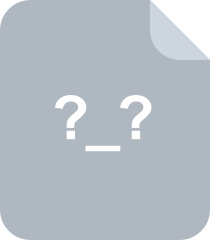
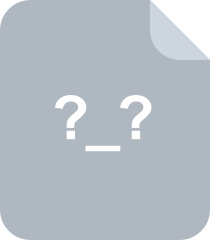
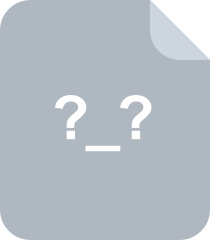
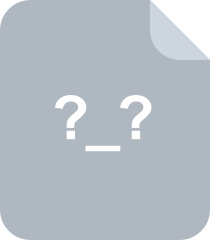
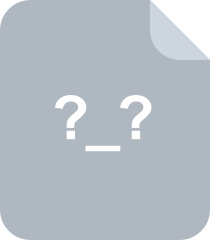
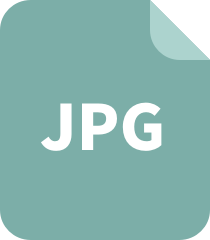
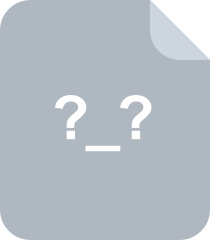
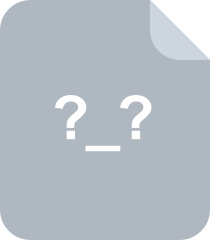
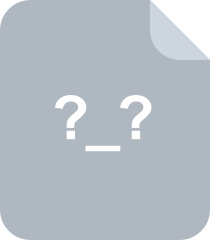
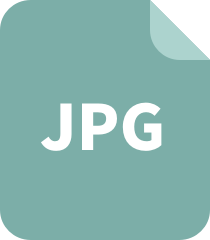
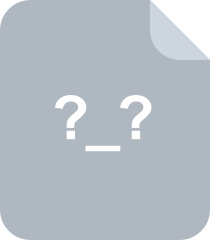
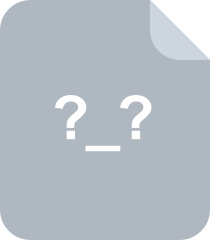
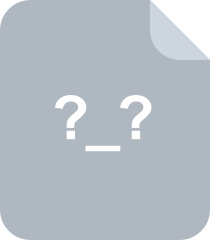
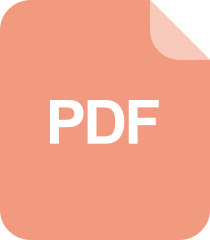
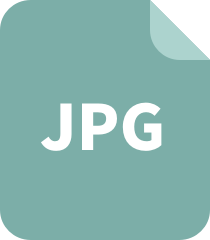
共 23 条
- 1
资源评论
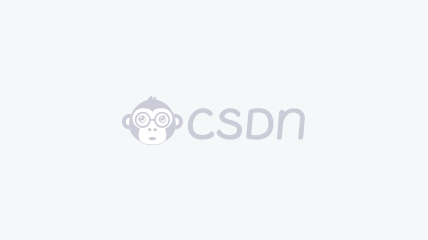

matlab科研社
- 粉丝: 2w+
- 资源: 2041
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

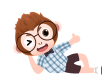
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


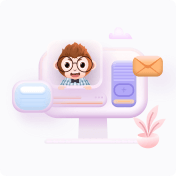
安全验证
文档复制为VIP权益,开通VIP直接复制
