function CrankMechanism(action,value)
% Example showing a simple animation of a crank mechanism
% Stefan Bj�rklund 2013-02-26
global GEO I_ V_ H_ N_ UIP_ VERSION STEP PARTS RUN
if nargin==0
GEO=[5 12]; %GEO=[CrankRadius PistonRodLength];
STEP(1)=1.5; % Step for changing speed
STEP(2)=1.2; % Step for changing piston rod length
VERSION='Version 1.1 Februari 2013';
action='start';
N_=1000; % Number of positions in the animation(sets the animation speed)
I_=1; % Animation index
V_=(-pi:2*pi/N_:pi-2*pi/N_)'; % Animation crank positions
end
if strcmp(action,'start') % Initializing figure for animation and GUI-bottons (Graphical User Interface)
figure(1)
set(1,'Units','Norm','Position',[0.50 0.10 0.4 0.8],'MenuBar','No','NumberTitle','Off',...
'Name',['Crank Mechanism Animation (' VERSION ')'],'Color',[1 1 0.5]);
clf
UIP_(1)=uicontrol('style','PushButton','Units','Normalized','position',[0.78 0.03 0.18 0.1],...
'callback','CrankMechanism(''RunOrStop'')','string','Run',...
'ForeGroundColor',[0 0.6 0],'FontSize',16);
UIP_(2)=uicontrol('style','PushButton','Units','Normalized','position',[0.89 0.43 0.05 0.05],...
'callback','CrankMechanism(''ChangeSpeed'',1)','string','\/',...
'ForeGroundColor',[0 0.6 0],'FontSize',16);
UIP_(3)=uicontrol('style','pushbutton','Units','Normalized','position',[0.89 0.57 0.05 0.05],...
'callback','CrankMechanism(''ChangeSpeed'',-1)','string','/\',...
'ForeGroundColor',[0 0.6 0],'FontSize',16);
UIP_(4)=uicontrol('style','PushButton','Units','Normalized','position',[0.12 0.38 0.05 0.05],...
'callback','CrankMechanism(''ChangePistonRodLength'',-1)','string','\/',...
'ForeGroundColor',[0 0.6 0],'FontSize',16);
UIP_(5)=uicontrol('style','pushbutton','Units','Normalized','position',[0.12 0.57 0.05 0.05],...
'callback','CrankMechanism(''ChangePistonRodLength'',1)','string','/\',...
'ForeGroundColor',[0 0.6 0],'FontSize',16);
UIP_(6)=uicontrol('style','Edit','Units','Normalized','position',[0.105 0.45 0.08 0.04],...
'callback','CrankMechanism(''ChangePistonRodLength'',2)','string',num2str(GEO(2)),...
'ForeGroundColor','k','FontSize',12);
uicontrol('Style','Text','Units','Normalized','position',[0.84 0.5 0.15 0.05],'BackGroundColor',[1 1 0.5],'FontSize',12,...
'String','Speed','HorizontalAlignment','Center');
uicontrol('Style','Text','Units','Normalized','position',[0.02 0.5 0.25 0.04],'BackGroundColor',[1 1 0.5],'FontSize',12,...
'String','Piston Rod Length','HorizontalAlignment','Center');
CrankMechanism('DrawGeometry',1)
elseif strcmp(action,'DrawGeometry') %Draw animation parts at their initial position
I_=value; % I_ is a global variable indexing to the current position of the animation
figure(1)
AX1=gca;
R=GEO(1); %Crank radius
L=GEO(2); %Piston rod length
T=R/8; %Link thickness
% PARTS is a global variable defining x- and y-coordinates to the parts
% that shall move in the animation. Each part has a reference point (a
% point in the part for which coordinates are calculated) and this
% point should be at the origin. The part should be oriented in the
% angular position that is defined as zero. Equal parts are only
% defined once.
PARTS.R_X=T*[-1 1 1 -1 -1]; %Crank link
PARTS.R_Y=R*[0 0 1 1 0];
PARTS.L_X=T*[-1 1 1 -1 -1]; %Piston rod
PARTS.L_Y=L*[0 0 1 1 0];
PARTS.Piston_X=0.5*R*[-1 1 1 -1 -1]; %Piston
PARTS.Piston_Y=0.6*R*[-1/4 -1/4 1.75 1.75 -1/4];
PARTS.Bearing_X=T*cos(0:pi/10:2*pi); %Bearings
PARTS.Bearing_Y=T*sin(0:pi/10:2*pi);
%Plot fixed and moving PARTS. If the parts intersect the last plotted
%will be placed above the previously plotted.
v1=0:pi/30:2*pi;
fill((R+T)*cos(v1),(R+T)*sin(v1),[1 1 1]*0.7) %Draw a circle representing the crank shaft
hold on
plot([0 0],[0 R+L+max(PARTS.Piston_Y)],'k-.') %Plot the cylinder centre line
c='grbmm'; % Part colors
%Create empty patchobjects to the moving parts (Here two links, piston and two bearings)
H_(1)=patch(0,0,c(1)); %Piston
set(H_(1),'FaceColor',[0 0.6 0])
H_(2)=patch(0,0,c(2)); %Crank link
fill(PARTS.Bearing_X,PARTS.Bearing_Y,c(5)) %Draw circle representing crank bearing (fixed)
H_(3)=patch(0,0,c(3)); %Piston rod
H_(4)=patch(0,0,c(4)); %Lower Piston Rod Bearing
H_(5)=patch(0,0,c(5)); %Upper Piston Rod Bearing
hold off
axis('image')
set( AX1,'Xlim',1.05*(R+T)*[-1 1]);
set( AX1,'Ylim',[-1.05*(R+T) 1.5*R+L+max(PARTS.Piston_Y)]);
CrankMechanism('MoveParts')
elseif strcmp(action,'MoveParts')
fi=V_(I_);R=GEO(1);L=GEO(2);
[x,y,yk,B]=CalcPos(fi,R,L);
xk=0;
[R_X,R_Y]=rotxy(0,0,-fi,PARTS.R_X,PARTS.R_Y);
[L_X,L_Y]=rotxy(0,0,B,PARTS.L_X,PARTS.L_Y);
set(H_(1),'Xdata',xk+PARTS.Piston_X,'Ydata',yk+PARTS.Piston_Y) %Piston
set(H_(2),'Xdata',R_X,'Ydata',R_Y) %Crank
set(H_(3),'Xdata',x+L_X,'Ydata',y+L_Y) %Piston Rod
set(H_(4),'Xdata',x+PARTS.Bearing_X,'Ydata',y+PARTS.Bearing_Y) %Lower Piston Rod Bearing
set(H_(5),'Xdata',xk+PARTS.Bearing_X,'Ydata',yk+PARTS.Bearing_Y) %Upper Piston Rod Bearing
drawnow
elseif strcmp(action,'RunOrStop')
if strcmp(get(UIP_(1),'String'),'Run')
set(UIP_(1),'String','Stop','ForeGroundColor','r');
RUN=1;
CrankMechanism('run');
else
set(UIP_(1),'String','Run','ForeGroundColor',[0 0.6 0]);
RUN=0;
end
elseif strcmp(action,'ChangeSpeed')
if RUN==1
N_=round(N_*(STEP(1))^value);
if N_<120
N_=120;
end
V=V_(I_);
V_=(-pi:2*pi/N_:pi-2*pi/N_)';
[tmp I_]=min(abs(V_-V));
end
elseif strcmp(action,'ChangePistonRodLength')
R=GEO(1);L=GEO(2);
if value==2
L=str2num(get(UIP_(6),'String'));
else
L=round(L*(STEP(2))^value);
end
if L<R
L=R;
end
set(UIP_(6),'String',num2str(L,3))
GEO(2)=L;
CrankMechanism('DrawGeometry',I_)
if RUN==1
CrankMechanism('MoveParts')
end
elseif strcmp(action,'run')
CONT=1;
while CONT==1
CrankMechanism('MoveParts')
if RUN==1
I_=I_+1;
else
CONT=0;
end
if I_==length(V_)
I_=1;
end
end
end
function [x,y,yk,B]=CalcPos(fi,R,L)
B=asin(R/L*sin(fi));
x=R*sin(fi);
y=R*cos(fi);
yk=y+L*cos(B);
function [xn,yn]=rotxy(x0,y0,alfa,x,y)
% [xn,yn]=rotxy(x0,y0,alfa,x,y);
% Rotates the points (x,y) the angle alfa around the point (x0,y0).
xn=x0+(x-x0)*cos(alfa)-(y-y0)*sin(alfa);
yn=y0+(x-x0)*sin(alfa)+(y-y0)*cos(alfa);

matlab科研社
- 粉丝: 2w+
- 资源: 2208
最新资源
- 机械设计硅钢片自动压装熔接机stp全套设计资料100%好用.zip.zip
- 基于springboot的人才公寓管理系统源码(java毕业设计完整源码).zip
- 基于springboot的亿时网上书店系统源码(java毕业设计完整源码).zip
- 基于springboot的仁和机构的体检预约系统的设计与实现源码(java毕业设计完整源码).zip
- 机械设计桁架自动化定子上下料设备sw16可编辑全套设计资料100%好用.zip.zip
- 基于springboot的仓库管理系统源码(java毕业设计完整源码).zip
- 基于springboot的付费自习室管理系统源码(java毕业设计完整源码).zip
- 机械设计混凝土搅拌站砖厂用砂筛机带式输送机sw20可编辑全套设计资料100%好用.zip.zip
- 基于springboot的企业员工薪酬管理系统源码(java毕业设计完整源码).zip
- 基于springboot的企业客源关系管理系统的设计与实现源码(java毕业设计完整源码).zip
- 基于springboot的企业档案管理信息系统的设计与实现源码(java毕业设计完整源码+LW).zip
- 基于springboot的候鸟监测数据管理系统源码(java毕业设计完整源码).zip
- 基于springboot的公寓出租系统的设计与实现源码(java毕业设计完整源码).zip
- 机械设计环形光学胶检测机sw21全套设计资料100%好用.zip.zip
- 机械设计货车底板支架焊接线sw16可编辑全套设计资料100%好用.zip.zip
- 基于springboot的兰州市出租车服务管理系统源码(java毕业设计完整源码).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


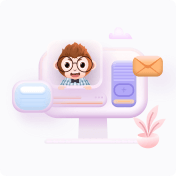