# UIWidgets
[中文](README-ZH.md)
## Introduction
UIWidgets is a plugin package for Unity Editor which helps developers to create, debug and deploy efficient,
cross-platform Apps using the Unity Engine.
UIWidgets is mainly derived from [Flutter](https://github.com/flutter/flutter). However, taking advantage of
the powerful Unity Engine, it offers developers many new features to improve their Apps
as well as the develop workflow significantly.
#### Efficiency
Using the latest Unity rendering SDKs, a UIWidgets App can run very fast and keep >60fps in most times.
#### Cross-Platform
A UIWidgets App can be deployed on all kinds of platforms including PCs, mobile devices and web page directly, like
any other Unity projects.
#### Multimedia Support
Except for basic 2D UIs, developers are also able to include 3D Models, audios, particle-systems to their UIWidgets Apps.
#### Developer-Friendly
A UIWidgets App can be debug in the Unity Editor directly with many advanced tools like
CPU/GPU Profiling, FPS Profiling.
## Example
<div style="text-align: center"><table><tr>
<td style="text-align: center">
<img src="https://connect-prd-cdn.unity.com/20190323/p/images/2a27606f-a2cc-4c9f-9e34-bb39ae64d06c_uiwidgets1.gif" width="200"/>
</td>
<td style="text-align: center">
<img src="https://connect-prd-cdn.unity.com/20190323/p/images/097a7c53-19b3-4e0a-ad27-8ec02506905d_uiwidgets2.gif" width="200" />
</td>
<td style="text-align: center">
<img src="https://connect-prd-cdn.unity.com/20190323/p/images/1f03c1d0-758c-4dde-b3a9-2f5f7216b7d9_uiwidgets3.gif" width="200"/>
</td>
<td style="text-align: center">
<img src="https://connect-prd-cdn.unity.com/20190323/p/images/a8884fbd-9e7c-4bd7-af46-0947e01d01fd_uiwidgets4.gif" width="200"/>
</td>
</tr></table></div>
### Projects using UIWidgets
#### Unity Connect App
The Unity Connect App is created using UIWidgets and available for both Android (https://connect.unity.com/connectApp/download)
and iOS (Searching for "Unity Connect" in App Store). This project is open-sourced @https://github.com/UnityTech/ConnectAppCN.
#### Unity Chinese Doc
The official website of Unity Chinese Documentation (https://connect.unity.com/doc) is powered by UIWidgets and
open-sourced @https://github.com/UnityTech/DocCN.
## Requirements
#### Unity
Install **Unity 2018.4.10f1 (LTS)** or **Unity 2019.1.14f1** and above. You can download the latest Unity on https://unity3d.com/get-unity/download.
#### UIWidgets Package
Visit our Github repository https://github.com/UnityTech/UIWidgets
to download the latest UIWidgets package.
Move the downloaded package folder into the **Package** folder of your Unity project.
Generally, you can make it using a console (or terminal) application by just a few commands as below:
```none
cd <YourProjectPath>/Packages
git clone https://github.com/UnityTech/UIWidgets.git com.unity.uiwidgets
```
## Getting Start
#### i. Overview
In this tutorial, we will create a very simple UIWidgets App as the kick-starter. The app contains
only a text label and a button. The text label will count the times of clicks upon the button.
First of all, please open or create a Unity Project and open it with Unity Editor.
And then open Project Settings, go to Player section and **add "UIWidgets_DEBUG" to the Scripting Define Symbols field.**
This enables the debug mode of UIWidgets for your development. Remove this for your release build afterwards.
#### ii. Scene Build
A UIWidgets App is usually built upon a Unity UI Canvas. Please follow the steps to create a
UI Canvas in Unity.
1. Create a new Scene by "File -> New Scene";
1. Create a UI Canvas in the scene by "GameObject -> UI -> Canvas";
1. Add a Panel (i.e., **Panel 1**) to the UI Canvas by right click on the Canvas and select "UI -> Panel". Then remove the
**Image** Component from the Panel.
#### iii. Create Widget
A UIWidgets App is written in **C# Scripts**. Please follow the steps to create an App and play it
in Unity Editor.
1. Create a new C# Script named "UIWidgetsExample.cs" and paste the following codes into it.
```csharp
using System.Collections.Generic;
using Unity.UIWidgets.animation;
using Unity.UIWidgets.engine;
using Unity.UIWidgets.foundation;
using Unity.UIWidgets.material;
using Unity.UIWidgets.painting;
using Unity.UIWidgets.ui;
using Unity.UIWidgets.widgets;
using UnityEngine;
using FontStyle = Unity.UIWidgets.ui.FontStyle;
namespace UIWidgetsSample {
public class UIWidgetsExample : UIWidgetsPanel {
protected override void OnEnable() {
// if you want to use your own font or font icons.
// FontManager.instance.addFont(Resources.Load<Font>(path: "path to your font"), "font family name");
// load custom font with weight & style. The font weight & style corresponds to fontWeight, fontStyle of
// a TextStyle object
// FontManager.instance.addFont(Resources.Load<Font>(path: "path to your font"), "Roboto", FontWeight.w500,
// FontStyle.italic);
// add material icons, familyName must be "Material Icons"
// FontManager.instance.addFont(Resources.Load<Font>(path: "path to material icons"), "Material Icons");
base.OnEnable();
}
protected override Widget createWidget() {
return new WidgetsApp(
home: new ExampleApp(),
pageRouteBuilder: (RouteSettings settings, WidgetBuilder builder) =>
new PageRouteBuilder(
settings: settings,
pageBuilder: (BuildContext context, Animation<float> animation,
Animation<float> secondaryAnimation) => builder(context)
)
);
}
class ExampleApp : StatefulWidget {
public ExampleApp(Key key = null) : base(key) {
}
public override State createState() {
return new ExampleState();
}
}
class ExampleState : State<ExampleApp> {
int counter = 0;
public override Widget build(BuildContext context) {
return new Column(
children: new List<Widget> {
new Text("Counter: " + this.counter),
new GestureDetector(
onTap: () => {
this.setState(()
=> {
this.counter++;
});
},
child: new Container(
padding: EdgeInsets.symmetric(20, 20),
color: Colors.blue,
child: new Text("Click Me")
)
)
}
);
}
}
}
}
```
1. Save this script and attach it to **Panel 1** as its component.
1. Press the "Play" Button to start the App in Unity Editor.
#### iv. Build App
Finally, the UIWidgets App can be built to packages for any specific platform by the following steps.
1. Open the Build Settings Panel by "File -> Build Settings..."
1. Choose a target platform and click "Build". Then the Unity Editor will automatically assemble
all relevant resources and generate the final App package.
#### How to load images?
1. Put your images files in Resources folder. e.g. image1.png.
2. You can add image1@2.png and image1@3.png in the same folder to support HD screens.
3. Use Image.asset("image1") to load the image. Note: as in Unity, ".png" is not needed.
UIWidgets
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip基于Unity3D和行为树插件制作的AI足球小游戏源码.zip 【备注】 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论

























收起资源包目录

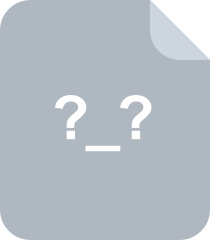
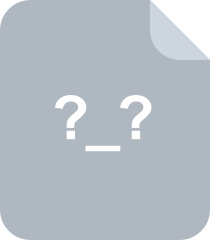
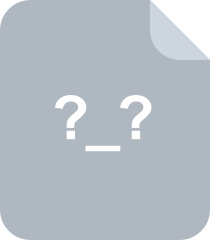
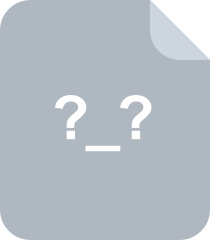
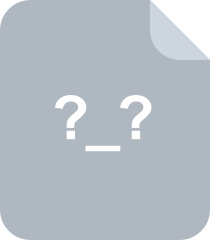
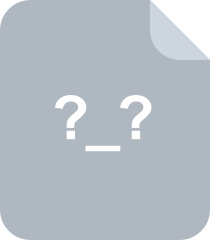
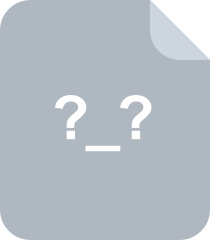
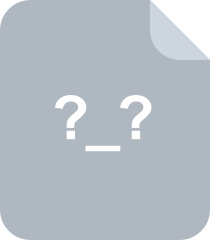
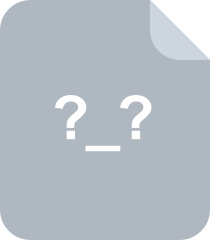
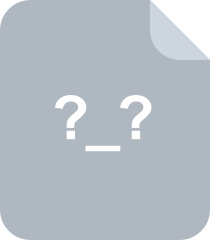
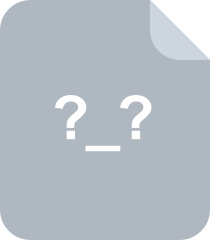
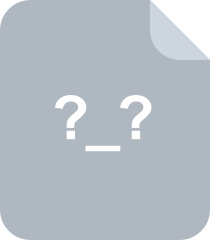
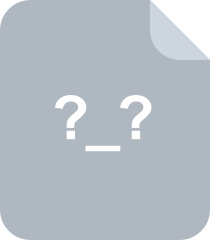
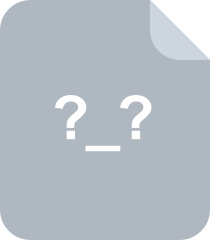
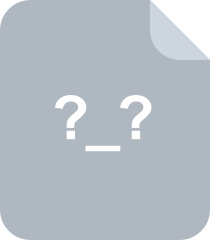
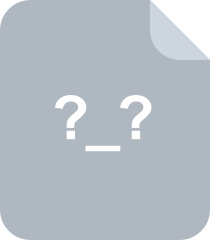
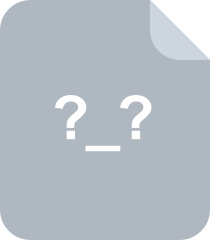
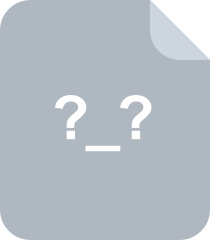
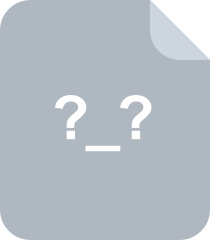
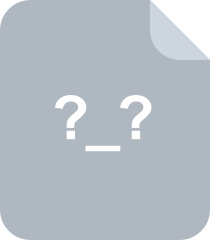
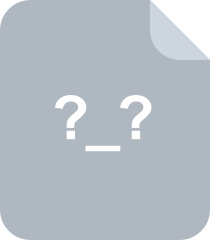
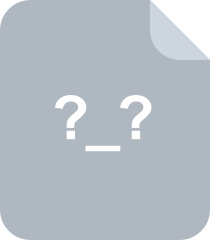
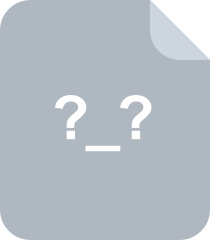
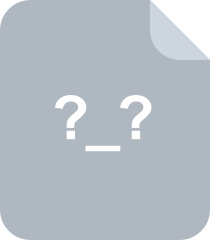
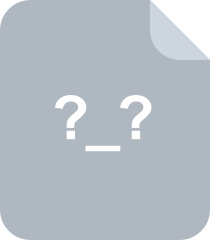
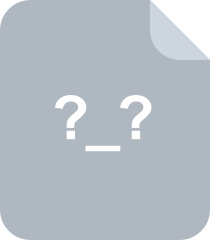
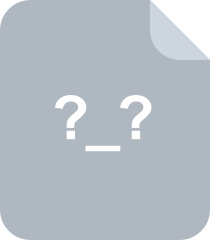
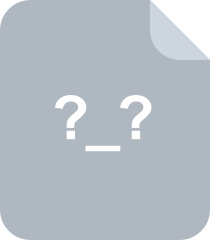
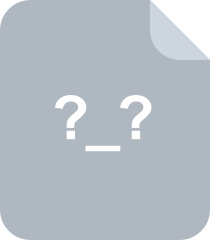
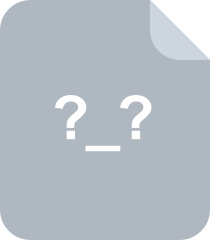
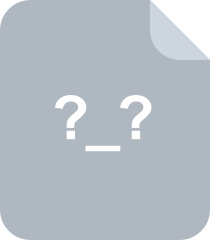
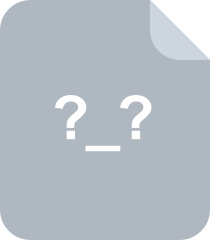
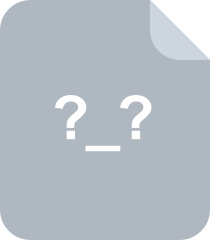
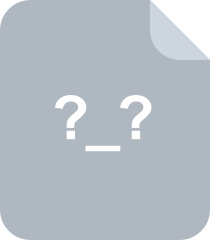
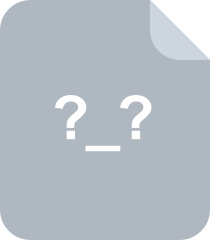
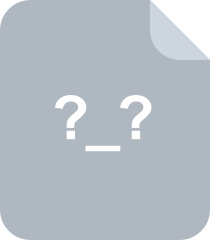
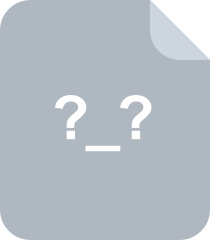
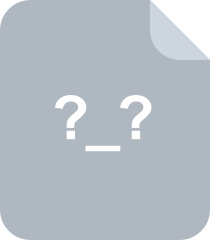
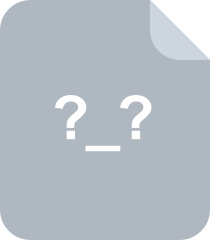
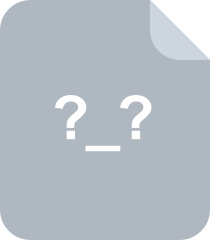
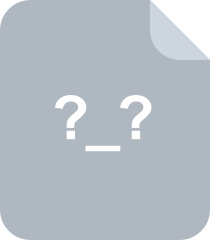
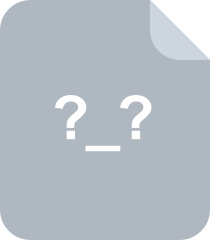
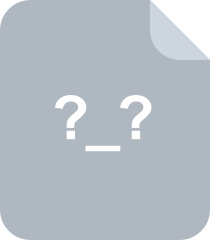
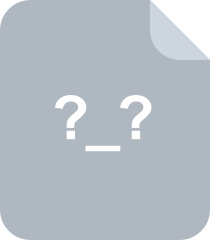
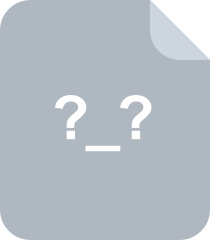
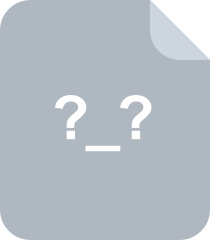
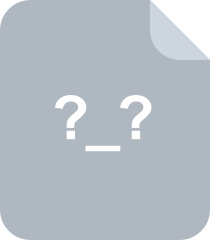
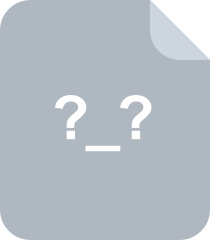
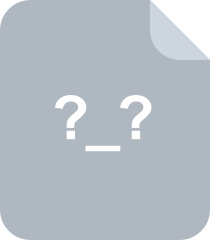
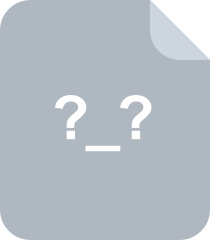
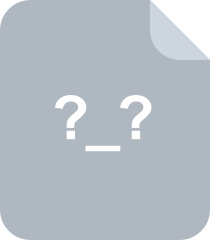
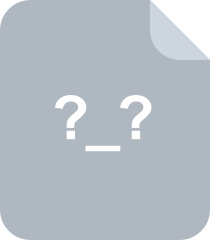
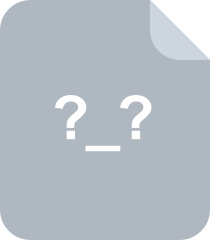
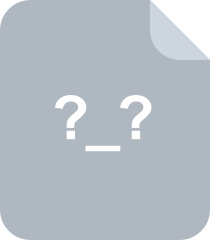
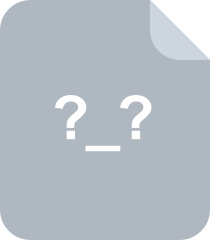
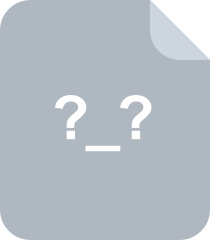
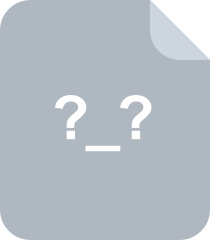
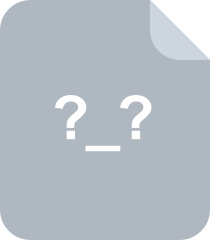
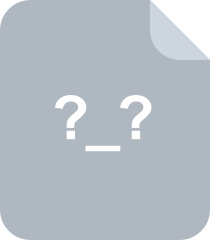
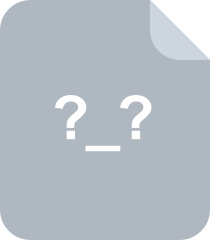
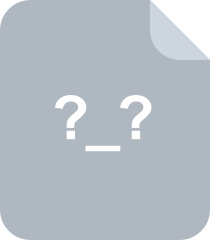
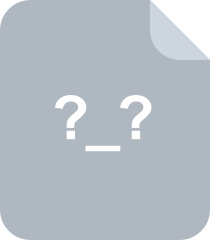
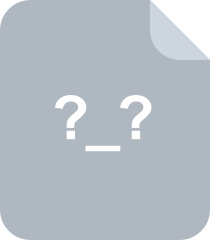
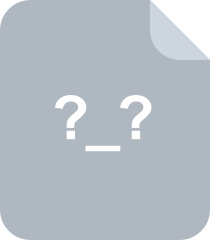
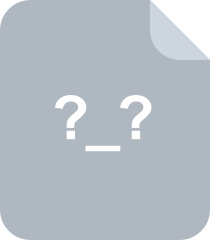
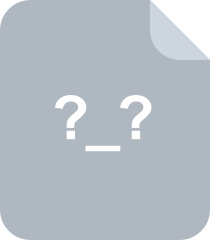
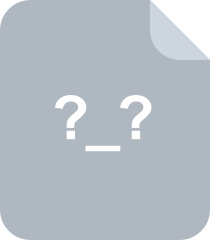
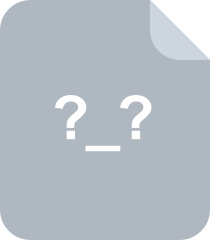
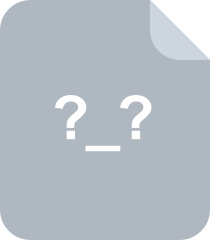
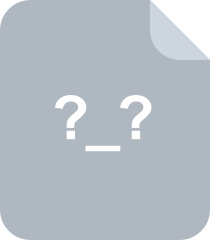
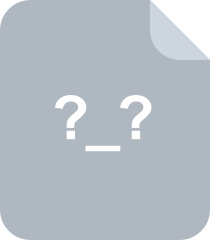
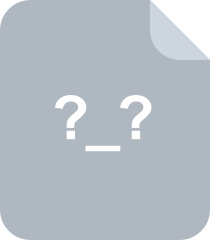
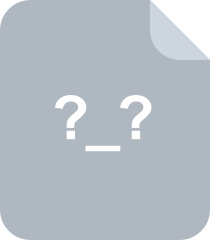
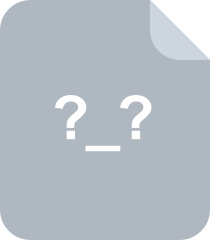
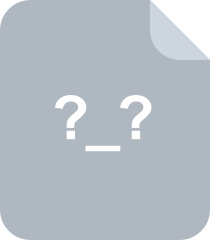
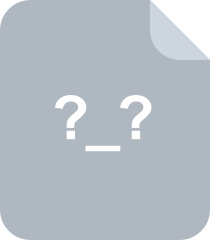
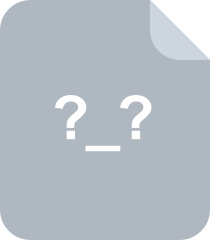
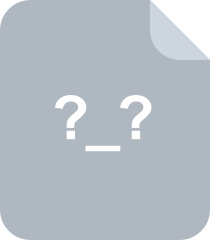
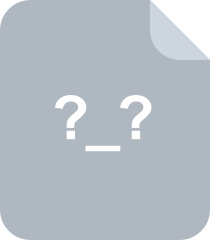
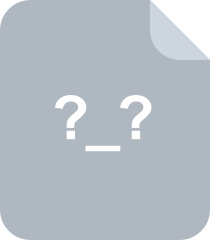
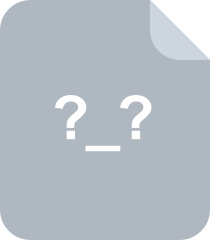
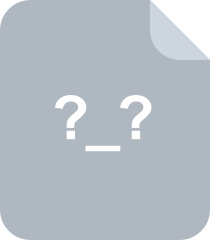
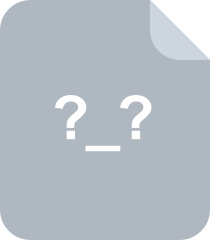
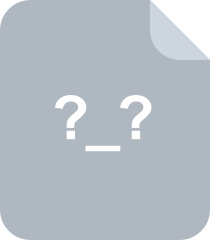
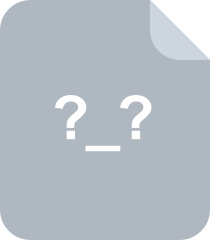
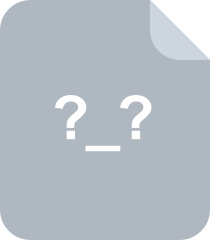
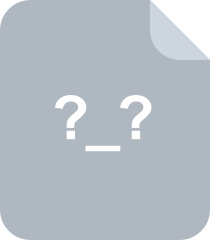
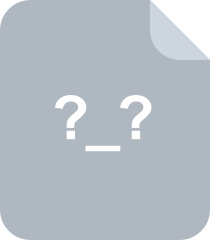
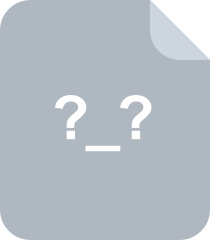
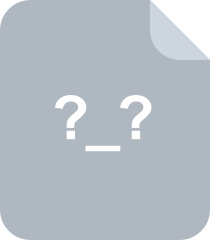
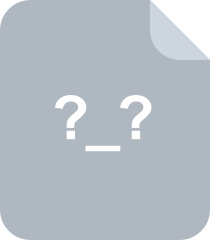
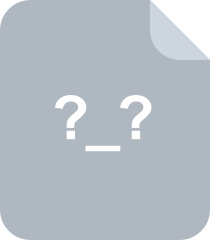
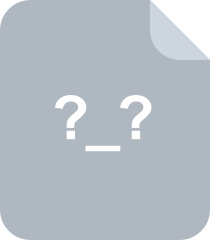
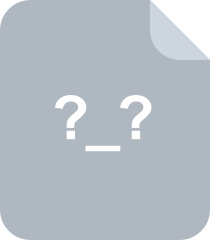
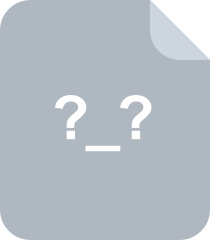
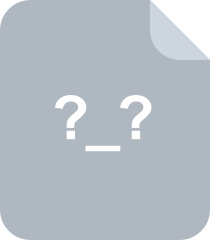
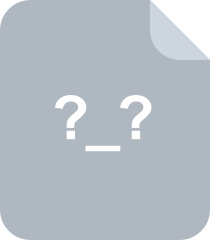
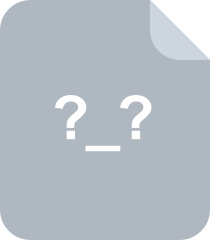
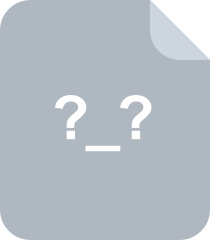
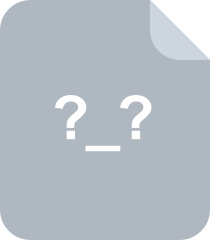
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
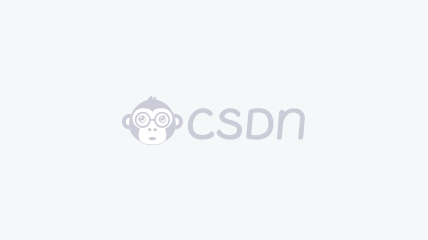
- Dedeleafk2024-02-02资源很赞,希望多一些这类资源。
- individualistloner5132024-01-01资源是宝藏资源,实用也是真的实用,感谢大佬分享~onnx2024-01-24不客气,还有问题直接私信!
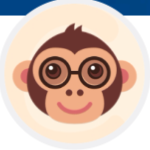
onnx
- 粉丝: 1w+
- 资源: 5637
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

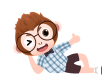
最新资源
- 【毕业设计】Python的Django-html知识图谱的医疗问答系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 工具变量-数字化激励数据集(2012-2023年).xlsx
- 基于麻雀算法优化深度置信网络的分类预测技术研究:详细Matlab代码注释解析,基于麻雀算法优化深度置信网络的分类预测技术研究:详解Matlab代码注释,基于麻雀算法优化深度置信网络(SSA-DBN)的
- 基于CarSim与Simulink联合的四轮独立驱动电动汽车转矩分配控制策略,包含三自由度车辆模型与离散LQR控制方法,详细控制器文档和稳定性控制目标推导(MATLAB 2018b) ,四轮独立驱动电
- 【毕业设计】SpringBoot+Shiro权限管理系统脚手架【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】SpringBoot+vue仓储物流管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】SpringBoot+Vue.js的物流管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】springboot+vue疫情防控管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- AI交互革新:Agentic RAG技术从传统RAG到智能代理的技术演变与突破及其应用场景
- 【毕业设计】SpringBoot酒店管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】springboot-bootstrap 后台管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】springboot+Vue农产品贸易管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】springboot+Vue人力资源管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】vue+springboot教务管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】基于SpringBoot + Mybatis Plus + SaToken + Thymeleaf + Layui的后台管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
- 【毕业设计】基于SpringBoot + Mybatis +Vue商品管理系统【源码+论文+答辩ppt+开题报告+任务书】.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


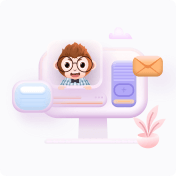
安全验证
文档复制为VIP权益,开通VIP直接复制
