package com.updateapp;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONObject;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.pm.PackageManager.NameNotFoundException;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class UpdateAppActivity extends Activity {
/** Called when the activity is first created. */
private static final String TAG = "Update";
private Button btnUpdateApp;
private ProgressDialog pBar;
private String downPath = "http://10.0.2.2:8080/";
private String appName = "NewAppSample.apk";
private String appVersion = "version.json";
private int newVerCode = 0;
private String newVerName = "";
private Handler handler=new Handler();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
try {
if(isNetworkAvailable(this) == false){
return;
}else{
checkToUpdate();
}
} catch (NameNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
btnUpdateApp = (Button)findViewById(R.id.btnUpdateApp);
btnUpdateApp.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
}
});
}
//check the Network is available
private static boolean isNetworkAvailable(Context context) {
// TODO Auto-generated method stub
try{
ConnectivityManager cm = (ConnectivityManager)context
.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo netWorkInfo = cm.getActiveNetworkInfo();
return (netWorkInfo != null && netWorkInfo.isAvailable());//妫�祴缃戠粶鏄惁鍙敤
}catch(Exception e){
e.printStackTrace();
return false;
}
}
//check new version and update
private void checkToUpdate() throws NameNotFoundException {
// TODO Auto-generated method stub
if(getServerVersion()){
int currentCode = CurrentVersion.getVerCode(this);
if(newVerCode > currentCode)
{//Current Version is old
//寮瑰嚭鏇存柊鎻愮ず瀵硅瘽妗� showUpdateDialog();
}
}
}
//show Update Dialog
private void showUpdateDialog() throws NameNotFoundException {
// TODO Auto-generated method stub
StringBuffer sb = new StringBuffer();
sb.append("");
sb.append(CurrentVersion.getVerName(this));
sb.append("VerCode:");
sb.append(CurrentVersion.getVerCode(this));
sb.append("\n");
sb.append("鍙戠幇鏂扮増鏈細");
sb.append(newVerName);
sb.append("NewVerCode:");
sb.append(newVerCode);
sb.append("\n");
sb.append("");
Dialog dialog = new AlertDialog.Builder(UpdateAppActivity.this)
.setTitle("杞欢鏇存柊")
.setMessage(sb.toString())
.setPositiveButton("鏇存柊", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
showProgressBar();//鏇存柊褰撳墠鐗堟湰
}
})
.setNegativeButton("鏆備笉鏇存柊", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
}
}).create();
dialog.show();
}
protected void showProgressBar() {
// TODO Auto-generated method stub
pBar = new ProgressDialog(UpdateAppActivity.this);
pBar.setTitle("姝e湪涓嬭浇");
pBar.setMessage("璇风◢鍚�..");
pBar.setProgressStyle(ProgressDialog.STYLE_SPINNER);
downAppFile(downPath + appName);
}
//Get ServerVersion from GetUpdateInfo.getUpdateVerJSON
private boolean getServerVersion() {
// TODO Auto-generated method stub
try{
String newVerJSON = GetUpdateInfo.getUpdataVerJSON(downPath + appVersion);
JSONArray jsonArray = new JSONArray(newVerJSON);
if(jsonArray.length() > 0){
JSONObject obj = jsonArray.getJSONObject(0);
try{
newVerCode = Integer.parseInt(obj.getString("verCode"));
newVerName = obj.getString("verName");
}catch(Exception e){
Log.e(TAG, e.getMessage());
newVerCode = -1;
newVerName = "";
return false;
}
}
}catch(Exception e){
Log.e(TAG, e.getMessage());
return false;
}
return true;
}
protected void downAppFile(final String url) {
pBar.show();
new Thread(){
public void run(){
HttpClient client = new DefaultHttpClient();
HttpGet get = new HttpGet(url);
HttpResponse response;
try {
response = client.execute(get);
HttpEntity entity = response.getEntity();
long length = entity.getContentLength();
Log.isLoggable("DownTag", (int) length);
InputStream is = entity.getContent();
FileOutputStream fileOutputStream = null;
if(is == null){
throw new RuntimeException("isStream is null");
}
File file = new File(Environment.getExternalStorageDirectory(),appName);
fileOutputStream = new FileOutputStream(file);
byte[] buf = new byte[1024];
int ch = -1;
do{
ch = is.read(buf);
if(ch <= 0)break;
fileOutputStream.write(buf, 0, ch);
}while(true);
is.close();
fileOutputStream.close();
haveDownLoad();
}catch(ClientProtocolException e){
e.printStackTrace();
}catch(IOException e){
e.printStackTrace();
}
}
}.start();
}
//cancel progressBar and start new App
protected void haveDownLoad() {
// TODO Auto-generated method stub
handler.post(new Runnable(){
public void run(){
pBar.cancel();
//寮瑰嚭璀﹀憡妗�鎻愮ず鏄惁瀹夎鏂扮殑鐗堟湰
Dialog installDialog = new AlertDialog.Builder(UpdateAppActivity.this)
.setTitle("涓嬭浇瀹屾垚")
.setMessage("鏄惁瀹夎鏂扮殑搴旂敤")
.setPositiveButton("纭畾", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
installNewApk();
finish();
}
})
.setNegativeButton("鍙栨秷", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
finish();
}
})
.create();
installDialog.show();
}
});
}
//瀹夎鏂扮殑搴旂敤
protected void installNewApk() {
// TODO Auto-generated method stub
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(
new File(Environment.getExternalStorageDirectory(),appName)),
"application/vnd.android.package-archive");
startActivity(intent);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Android 应用软件自动更新源码.zip
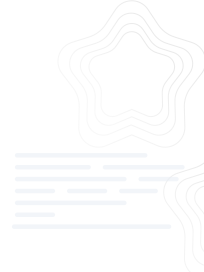
共43个文件
class:17个
png:6个
java:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 6 浏览量
2022-06-18
17:03:42
上传
评论
收藏 2.03MB ZIP 举报
温馨提示
Android 应用软件自动更新源码.zip
资源推荐
资源详情
资源评论
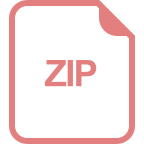
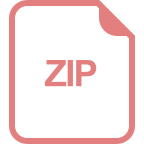
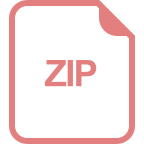
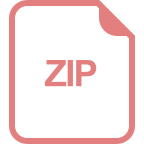
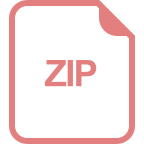
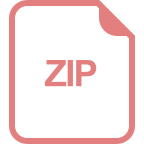
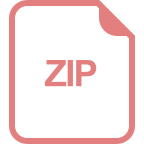
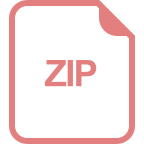
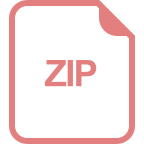
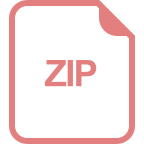
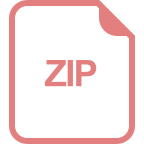
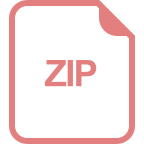
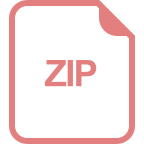
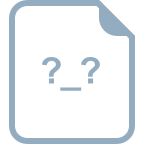
收起资源包目录




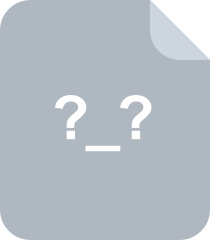
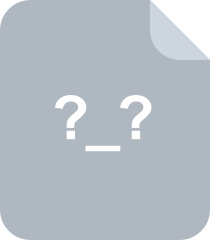


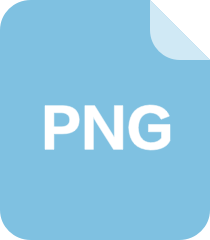

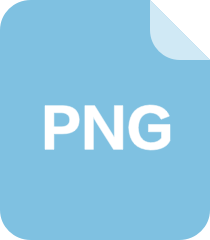

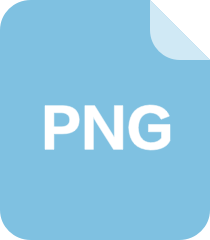

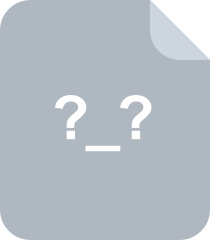
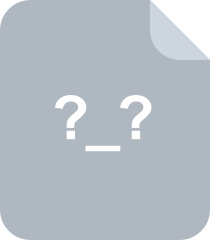


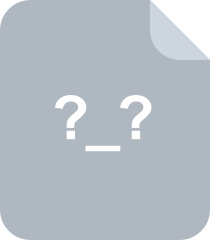
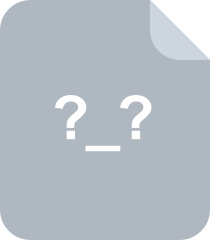
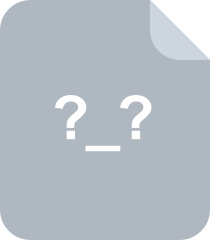
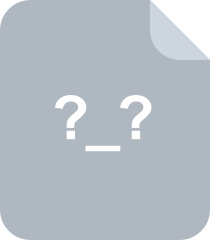
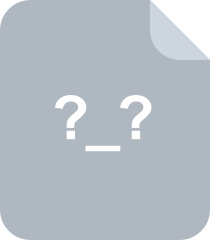
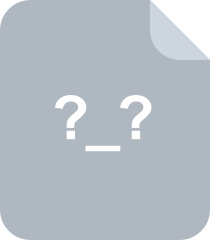
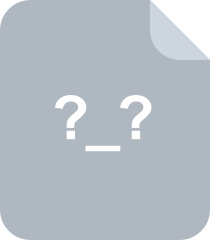
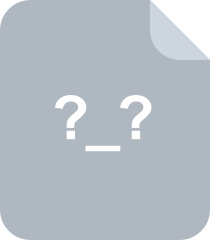
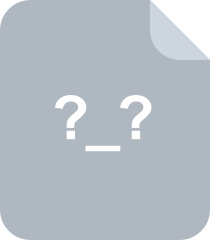
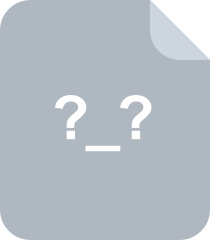
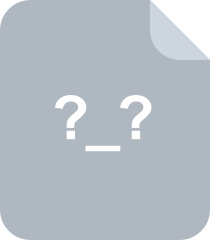
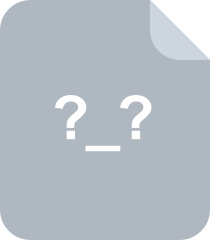
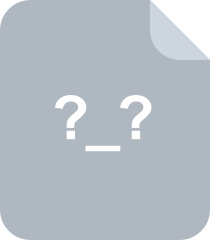
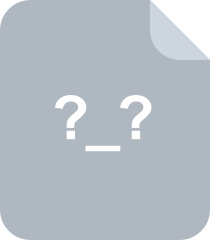
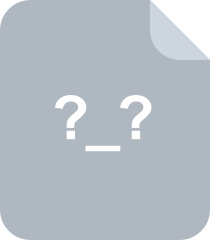
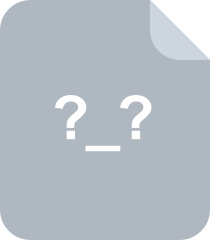
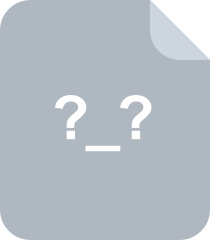
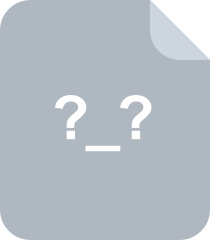
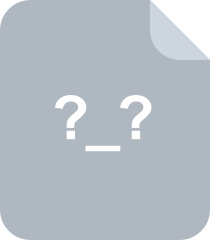


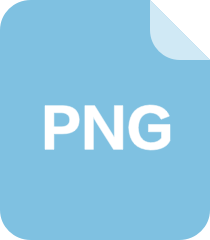

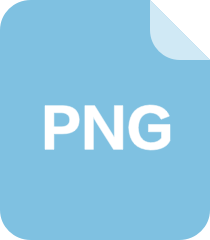

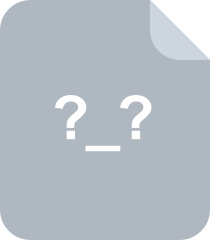

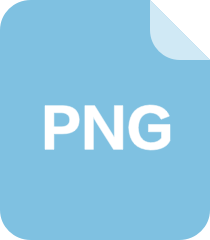

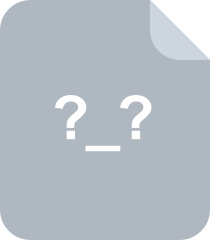

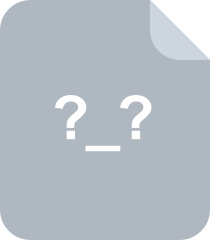



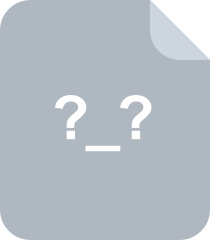
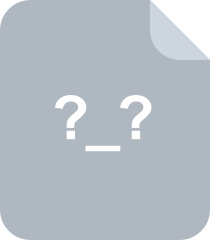

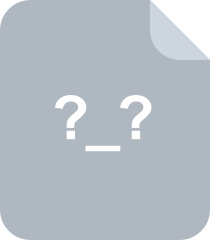



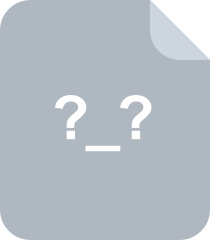
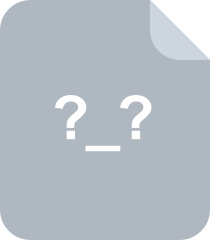
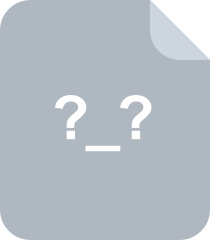
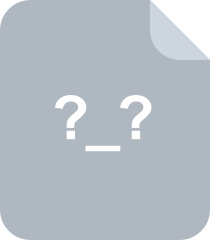
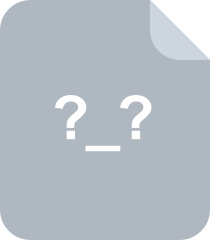
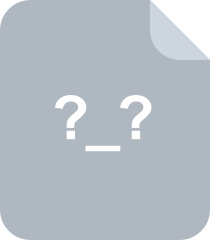
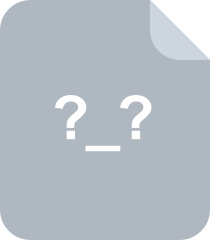
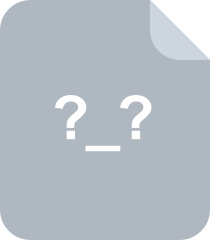
共 43 条
- 1
资源评论
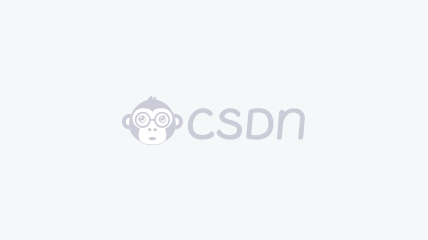

BryanDing
- 粉丝: 301
- 资源: 5582
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

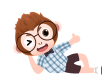
最新资源
- elasticsearch数据库下载、配置、使用案例
- springboot的概要介绍与分析
- C语言的概要介绍与分析
- 第一个较大的Android项目,基于Android平台的图书管理系统(Android studio).zip
- Cisco Packet Tracer 6.2 for Windows Instructor Version
- 使⽤pyIAST计算⽓体吸附选择性
- tmp_b056727e59b8123365486983f32baa9732607ec3c6137b12.pdf
- C代码实现文件的拆分和合并,本质上就是文件的读写操作.zip
- TVMP3player.apk.1
- 出马出马出马出马出马出马出马
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


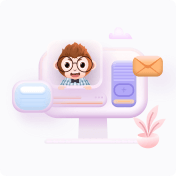
安全验证
文档复制为VIP权益,开通VIP直接复制
