package cn.mldn.lxh.Dao.Impl;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import cn.mldn.lxh.Dao.userDAO;
import cn.mldn.lxh.Dao.dbcon.User;
import cn.mldn.lxh.Dao.dbcon.dbConnection;
public class userDAOImpl implements userDAO{
public void insert(User user) throws Exception {
System.out.println("-----insert----");
dbConnection conn=new dbConnection();
PreparedStatement pstmt=null;
String sql="insert into user_information(name,password,age,email) values(?,?,?,?)";
System.out.println(sql);
System.out.println(user.getUserName());
try{
System.out.println("start insert");
pstmt=conn.getConnection().prepareStatement(sql);
System.out.println(sql);
pstmt.setString(1, user.getUserName());
System.out.println("insert beginning");
pstmt.setString(2, user.getPassword());
pstmt.setInt(3, user.getAge());
pstmt.setString(4, user.getEmail());
pstmt.executeUpdate();
System.out.println("insert implement....");
pstmt.close();
}
catch(Exception e)
{
System.out.println("insert fail");
e.printStackTrace();
throw new Exception("操作中出现错误");
}
finally{
conn.close();
}
}
public void update(User user) throws Exception {
dbConnection conn=null;
PreparedStatement pstmt=null;
String sql="update user_information set name=?,password=?,age=?,email=? where id=?";
try{
pstmt=conn.getConnection().prepareStatement(sql);
pstmt.setString(1, user.getUserName());
pstmt.setString(2, user.getPassword());
pstmt.setInt(3, user.getAge());
pstmt.setString(4, user.getEmail());
pstmt.setInt(5, user.getId());
pstmt.executeUpdate();
pstmt.close();
}
catch(Exception e)
{
throw new Exception("操作中出现错误");
}
finally{
conn.close();
}
}
public void delete(int id) throws Exception {
dbConnection conn=null;
PreparedStatement pstmt=null;
String sql="delete from user_information where id=?";
try{
pstmt=conn.getConnection().prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
}
catch(Exception e)
{
throw new Exception("操作中出现错误");
}
finally{
conn.close();
}
}
public User queryById(int id) throws Exception {
dbConnection conn=null;
User user=null;
PreparedStatement pstmt=null;
String sql="select * from user_information where id=?";
try{
pstmt=conn.getConnection().prepareStatement(sql);
pstmt.setInt(1, id);
ResultSet rs=pstmt.executeQuery();
if(rs.next())
{
user=new User();
user.setUserName(rs.getString("name"));
user.setPassword(rs.getString("password"));
user.setAge(rs.getInt("age"));
user.setEmail(rs.getString("email"));
}
pstmt.close();
}
catch(Exception e)
{
throw new Exception("操作中出现错误");
}
finally{
conn.close();
}
return user;
}
public List queryAll() throws Exception {
List all=null;
dbConnection conn=null;
String sql="select * from user_information";
PreparedStatement pstmt=null;
try{
conn=new dbConnection();
pstmt=conn.getConnection().prepareStatement(sql);
ResultSet rs=pstmt.executeQuery();
while(rs.next())
{
User user=new User();
user.setId(rs.getInt("id"));
user.setUserName(rs.getString("name"));
user.setPassword(rs.getString("password"));
user.setAge(rs.getInt("age"));
user.setEmail(rs.getString("email"));
all.add(user);
}
}catch(Exception e){}
finally{conn.close();}
return all;
}
public List queryByLike(String cond) throws Exception {
List all=new ArrayList();
dbConnection conn=null;
PreparedStatement pstmt=null;
String sql="select * from user_information where name like ? or remark like ?";
try{
conn=new dbConnection();
pstmt=conn.getConnection().prepareStatement(sql);
pstmt.setString(1, cond);
pstmt.setString(2, cond);
ResultSet rs=pstmt.executeQuery();
while(rs.next())
{
User user=new User();
user.setId(rs.getInt("id"));
user.setUserName(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setAge(rs.getInt("age"));
user.setEmail(rs.getString("email"));
all.add(user);
}
}catch(Exception e){}
finally{conn.close();}
return all;
}
}

风/xin云
- 粉丝: 52
- 资源: 9
最新资源
- 【JCR一区级】鸽群算法PIO-Transformer-GRU负荷数据回归预测【含Matlab源码 6315期】.zip
- 【LSTM回归预测】粒子群优化注意力机制的长短时记忆神经网络PSO-attention-LSTM数据回归预测【含Matlab源码 3196期】.zip
- 【独家首发】麻雀搜索算法SSA优化Transformer-BiLSTM负荷数据回归预测【含Matlab源码 6564期】.zip
- 【独家首发】凌日算法TSOA优化Transformer-BiLSTM负荷数据回归预测【含Matlab源码 6562期】.zip
- 【独家首发】能量谷算法EVO优化Transformer-BiLSTM负荷数据回归预测【含Matlab源码 6565期】.zip
- 【JCR一区级】豪猪算法CPO-Transformer-GRU负荷数据回归预测【含Matlab源码 6319期】.zip
- 【JCR一区级】雪融算法SAO-Transformer-GRU负荷数据回归预测【含Matlab源码 6351期】.zip
- 【JCR一区级】黑猩猩算法Chimp-Transformer-GRU负荷数据回归预测【含Matlab源码 6320期】.zip
- 【独家首发】粒子群算法PSO优化Transformer-BiLSTM负荷数据回归预测【含Matlab源码 6561期】.zip
- 【JCR1区】阿基米德算法AOA-CNN-SVM故障诊断分类预测【含Matlab源码 5772期】.zip
- 【JCR一区级】蝗虫算法GOA-Transformer-GRU负荷数据回归预测【含Matlab源码 6322期】.zip
- 【JCR一区级】星雀算法NOA-Transformer-GRU负荷数据回归预测【含Matlab源码 6350期】.zip
- 【JCR一区级】花朵授粉算法FPA-Transformer-GRU负荷数据回归预测【含Matlab源码 6321期】.zip
- 【JCR1区】蝗虫算法GOA-CNN-SVM故障诊断分类预测【含Matlab源码 5794期】.zip
- 【JCR一区级】混沌博弈算法CGO-Transformer-GRU负荷数据回归预测【含Matlab源码 6324期】.zip
- 【JCR一区级】减法平均算法SABO-Transformer-GRU负荷数据回归预测【含Matlab源码 6325期】.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


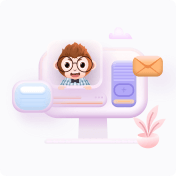