/**
* <p>Title: Sprite.java</p>
*
* <p>Description: Sprite MIDP2.0</p>
*
* <p>Copyright: Copyright (c) 2009</p>
*
* @author fengsheng.yang
*
* @version 1.0
*
* @Date 2009-5-14
*/
package javax.microedition.lcdui.game;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Rect;
public class Sprite extends Layer {
// ----- definitions for the various transformations possible -----
/**
* No transform is applied to the Sprite. This constant has a value of
* <code>0</code>.
*/
public static final int TRANS_NONE = 0;
/**
* Causes the Sprite to appear rotated clockwise by 90 degrees. This
* constant has a value of <code>5</code>.
*/
public static final int TRANS_ROT90 = 5;
/**
* Causes the Sprite to appear rotated clockwise by 180 degrees. This
* constant has a value of <code>3</code>.
*/
public static final int TRANS_ROT180 = 3;
/**
* Causes the Sprite to appear rotated clockwise by 270 degrees. This
* constant has a value of <code>6</code>.
*/
public static final int TRANS_ROT270 = 6;
/**
* Causes the Sprite to appear reflected about its vertical center. This
* constant has a value of <code>2</code>.
*/
public static final int TRANS_MIRROR = 2;
/**
* Causes the Sprite to appear reflected about its vertical center and then
* rotated clockwise by 90 degrees. This constant has a value of
* <code>7</code>.
*/
public static final int TRANS_MIRROR_ROT90 = 7;
/**
* Causes the Sprite to appear reflected about its vertical center and then
* rotated clockwise by 180 degrees. This constant has a value of
* <code>1</code>.
*/
public static final int TRANS_MIRROR_ROT180 = 1;
/**
* Causes the Sprite to appear reflected about its vertical center and then
* rotated clockwise by 270 degrees. This constant has a value of
* <code>4</code>.
*/
public static final int TRANS_MIRROR_ROT270 = 4;
// --- member variables
/**
* If this bit is set, it denotes that the transform causes the axes to be
* interchanged
*/
private static final int INVERTED_AXES = 0x4;
/**
* If this bit is set, it denotes that the transform causes the x axis to be
* flipped.
*/
private static final int X_FLIP = 0x2;
/**
* If this bit is set, it denotes that the transform causes the y axis to be
* flipped.
*/
private static final int Y_FLIP = 0x1;
/**
* Bit mask for channel value in ARGB pixel.
*/
private static final int ALPHA_BITMASK = 0xff000000;
/**
* Source image
*/
Bitmap sourceImage;
/**
* The number of frames
*/
int numberFrames; // = 0;
/**
* list of X coordinates of individual frames
*/
int[] frameCoordsX;
/**
* list of Y coordinates of individual frames
*/
int[] frameCoordsY;
/**
* Width of each frame in the source image
*/
int srcFrameWidth;
/**
* Height of each frame in the source image
*/
int srcFrameHeight;
/**
* The sequence in which to display the Sprite frames
*/
int[] frameSequence;
/**
* The sequence index
*/
private int sequenceIndex; // = 0
/**
* Set to true if custom sequence is used.
*/
private boolean customSequenceDefined; // = false;
// -- reference point
/**
* Horizontal offset of the reference point from the top left of the sprite.
*/
int dRefX; // =0
/**
* Vertical offset of the reference point from the top left of the sprite.
*/
int dRefY; // =0
// --- collision rectangle
/**
* Horizontal offset of the top left of the collision rectangle from the top
* left of the sprite.
*/
int collisionRectX; // =0
/**
* Vertical offset of the top left of the collision rectangle from the top
* left of the sprite.
*/
int collisionRectY; // =0
/**
* Width of the bounding rectangle for collision detection.
*/
int collisionRectWidth;
/**
* Height of the bounding rectangle for collision detection.
*/
int collisionRectHeight;
// --- transformation(s)
// --- values that may change on setting transformations
// start with t_
/**
* The current transformation in effect.
*/
int t_currentTransformation;
/**
* Horizontal offset of the top left of the collision rectangle from the top
* left of the sprite.
*/
int t_collisionRectX;
/**
* Vertical offset of the top left of the collision rectangle from the top
* left of the sprite.
*/
int t_collisionRectY;
/**
* Width of the bounding rectangle for collision detection, with the current
* transformation in effect.
*/
int t_collisionRectWidth;
/**
* Height of the bounding rectangle for collision detection, with the
* current transformation in effect.
*/
int t_collisionRectHeight;
// ----- Constructors -----
/**
* Creates a new non-animated Sprite using the provided Image. This
* constructor is functionally equivalent to calling
* <code>new Sprite(image, image.getWidth(), image.getHeight())</code>
* <p>
* By default, the Sprite is visible and its upper-left corner is positioned
* at (0,0) in the painter's coordinate system. <br>
*
* @param image
* the <code>Image</code> to use as the single frame for the
* </code>Sprite
* @throws NullPointerException
* if <code>img</code> is <code>null</code>
*/
public Sprite(Bitmap image) {
super(image.getWidth(), image.getHeight());
initializeFrames(image, image.getWidth(), image.getHeight(), false);
// initialize collision rectangle
initCollisionRectBounds();
// current transformation is TRANS_NONE
this.setTransformImpl(TRANS_NONE);
}
/**
* Creates a new animated Sprite using frames contained in the provided
* Image. The frames must be equally sized, with the dimensions specified by
* <code>frameWidth</code> and <code>frameHeight</code>. They may be laid
* out in the image horizontally, vertically, or as a grid. The width of the
* source image must be an integer multiple of the frame width, and the
* height of the source image must be an integer multiple of the frame
* height. The values returned by {@link Layer#getWidth} and
* {@link Layer#getHeight} will reflect the frame width and frame height
* subject to the Sprite's current transform.
* <p>
* Sprites have a default frame sequence corresponding to the raw frame
* numbers, starting with frame 0. The frame sequence may be modified with
* {@link #setFrameSequence(int[])}.
* <p>
* By default, the Sprite is visible and its upper-left corner is positioned
* at (0,0) in the painter's coordinate system.
* <p>
*
* @param image
* the <code>Image</code> to use for <code>Sprite</code>
* @param frameWidth
* the <code>width</code>, in pixels, of the individual raw
* frames
* @param frameHeight
* the <code>height</code>, in pixels, of the individual raw
* frames
* @throws NullPointerException
* if <code>img</code> is <code>null</code>
* @throws IllegalArgumentException
* if <code>frameHeight</code> or <code>frameWidth</code> is
* less than <code>1</code>
* @throws IllegalArgumentException
* if the <code>image</code> width is not an integer multiple of
* the <code>frameWidth</code>
* @throws IllegalArgumentException
* if the <code>image</code> height is not an integer multiple
* of the <code>frameHeight</code>
*/
public Sprite(Bitmap image, int frameWidth, int frameHeight) {
super(frameWidth, frameHeight);
// if img is null img.getWidth() will throw NullPointerException
if ((frameWidth < 1 || frameHeight < 1)
|| ((image.getWidth() % frameWidth) != 0)
|| ((image.getHeight() % frameHe
ME上Layer,LayerManager,Sprite,TiledLayer移植到android


zhongdaxia520520
- 粉丝: 5
- 资源: 8
最新资源
- tensorflow-gpu-2.7.4-cp37-cp37m-manylinux2010-x86-64.whl
- 多段线、 圆、弧转多段线(仅我可见)
- tensorflow-2.7.2-cp38-cp38-manylinux2010-x86-64.whl
- yeyue-p8Yi4-ve4a83792.apk
- tensorflow-gpu-2.7.3-cp38-cp38-manylinux2010-x86-64.whl
- 五相感应电机矢量控制模型MATLAB
- RGLED (1) (1).circ
- IMG_20240427_215747.jpg
- python下前端WEB学习笔记
- 田间种植行排号自动生成工具
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


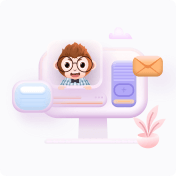
- 1
- 2
前往页