# GoWorld
_**Scalable Distributed Game Server Engine with Hot Reload in Golang**_
[](https://godoc.org/github.com/xiaonanln/goworld)
[](https://travis-ci.org/xiaonanln/goworld) [](https://goreportcard.com/report/github.com/xiaonanln/goworld) [](https://codecov.io/gh/xiaonanln/goworld)
[](https://raw.githubusercontent.com/xiaonanln/goworld/master/LICENSE)
* [Features](#features)
* [Architecture](#architecture)
* [Introduction](#introduction)
* [Get GoWorld](#get-goworld)
* [Manage GoWorld Servers](#manage-goworld-servers)
* [Demos](#demos)
* [Chatroom Demo](#chatroom-demo)
* [Unity Demo](#unity-demo): [GoWorldUnityDemo.zip](https://drive.google.com/file/d/1A1CJCVWFQWa-iMuAoAdHZ4JoXTtU5Q7z/view?usp=sharing)
---------------------------------------
#### 中文资料
> [中文文档](https://godoc.org/github.com/xiaonanln/goworld/cn)
> [游戏服务器介绍](http://www.cnblogs.com/isaiah/p/7259036.html)
> [目录结构说明](https://github.com/xiaonanln/goworld/wiki/GoWorld%E6%B8%B8%E6%88%8F%E6%9C%8D%E5%8A%A1%E5%99%A8%E5%BC%95%E6%93%8E%E7%9B%AE%E5%BD%95%E7%BB%93%E6%9E%84)
> [使用GoWorld轻松实现分布式聊天服务器](https://github.com/xiaonanln/goworld/wiki/%E4%BD%BF%E7%94%A8GoWorld%E6%B8%B8%E6%88%8F%E6%9C%8D%E5%8A%A1%E5%99%A8%E5%BC%95%E6%93%8E%E8%BD%BB%E6%9D%BE%E5%AE%9E%E7%8E%B0%E5%88%86%E5%B8%83%E5%BC%8F%E8%81%8A%E5%A4%A9%E6%9C%8D%E5%8A%A1%E5%99%A8)
#### 游戏服务端开源引擎GoWorld教程
1.[安装和运行](https://zhuanlan.zhihu.com/p/66304813 "安装和运行")
2.[Unity示例双端联调](https://zhuanlan.zhihu.com/p/67065981 "Unity示例双端联调")
3.[手把手写一个聊天室](https://zhuanlan.zhihu.com/p/67951379 "手把手写一个聊天室")
4.[多个频道的聊天室](https://zhuanlan.zhihu.com/p/68901701 "多个频道的聊天室")
5.[登录注册和存储](https://zhuanlan.zhihu.com/p/70039615 "登录注册和存储")
6.[移动同步和AOI](https://zhuanlan.zhihu.com/p/70778081 "移动同步和AOI")
7.[源码解析之启动流程和热更新](https://zhuanlan.zhihu.com/p/72093172 "源码解析之启动流程和热更新")
8.[码解析之gate](https://zhuanlan.zhihu.com/p/73727839 "码解析之gate")
9.[源码解析之dispatcher](https://zhuanlan.zhihu.com/p/73906406 "源码解析之dispatcher")
10.[源码解析之entity](https://zhuanlan.zhihu.com/p/74736032 "源码解析之entity")
---------------------------------------
> **欢迎加入Go服务端开发交流群:[662182346](http://shang.qq.com/wpa/qunwpa?idkey=f2a99bd9bd9e6df3528174180aad753d05b372a8828e1b8e5c1ec5df42b301db)**
---------------------------------------
## Features
* **Spaces & Entities**: manage multiple spaces and entities with AOI support
* **Distributed**: increase server capacity by using more machines
* **Hot-Swappable**: update game logic by restarting server process
* **Multiple Communication Protocols**: supports TCP, [KCP](https://github.com/skywind3000/kcp) and WebSocket
* **Traffic Compression & Encryption**: traffic between clients and servers can be compressed and encrypted
## Architecture

## Introduction
GoWorld server adopts an entity framework, in which entities represent all players, monsters, NPCs.
Entities in the same space can visit each other directly by calling methods or access attributes.
Entities in different spaces can call each over using RPC.
A GoWorld server consists of one dispatcher, one or more games and one or more gates.
The gates are responsible for handling client connections and receive/send packets from/to clients.
The games manages all entities and runs all game logic.
The dispatcher is responsible for redirecting packets among games and between games and gates.
The game processes are **hot-swappable**.
We can swap a game by sending `SIGHUP` to the process and restart the process with **-restore** parameter to bring game
back to work but with the latest executable image. This feature enables updating server-side logic or fixing server bugs
transparently without significant interference of online players.
## Installing GoWorld
GoWorld requries Go 1.11+ to install.
```bash
go install github.com/xiaonanln/goworld/cmd/...
```
## Manage GoWorld Servers
Use command `goworld` to build, start, stop and reload game servers.
**Build Example Chatroom Server:**
```bash
$ goworld build examples/chatroom_demo
```
**Start Example Chatroom Server: (dispatcher -> game -> gate)**
```bash
$ goworld start examples/chatroom_demo
```
**Stop Game Server (gate -> game -> dispatcher):**
```bash
$ goworld stop examples/chatroom_demo
```
**Reload Game Servers:**
```bash
$ goworld reload examples/chatroom_demo
```
Reload will reboot game processes with the current executable while preserving all game server states.
**However, it does not work on Windows.**
**List Server Processes:**
```bash
$ goworld status examples/chatroom_demo
> 1 dispatcher running, 1/1 gates running, 1/1 games (examples/chatroom_demo) running
> 2763 dispatcher /home/ubuntu/go/src/github.com/xiaonanln/goworld/components/dispatcher/dispatcher -dispid 1
> 2770 chatroom_demo /home/ubuntu/go/src/github.com/xiaonanln/goworld/examples/chatroom_demo/chatroom_demo -gid 1
> 2779 gate /home/ubuntu/go/src/github.com/xiaonanln/goworld/components/gate/gate -gid 1
```
## Demos
### Chatroom Demo
The chatroom demo is a simple implementation of chatroom server and client. It illustrates how
GoWorld can also be used for games which don't divide players by spaces.
The chatroom demo provides following features:
* register & login
* send chat message
* switch chatrooms
**Build Server:**
```bash
goworld build examples/chatroom_demo
```
**Run Server:**
```bash
goworld start examples/chatroom_demo
```
**Chatroom Demo Client:**
Chatroom demo client implements the client-server protocol in Javascript.
The client for chatroom demo is hosted at [github.com/xiaonanln/goworld-chatroom-demo-client](https://github.com/xiaonanln/goworld-chatroom-demo-client).
The project was created and built in [Cocos Creater 1.5](http://www.cocos2d-x.org/).
### Unity Demo
Unity Demo is a simple multiple player monster shooting game to show how spaces and entities of GoWorld
can be used to create multiple player online games.
* register & login
* move players in space
* summon monsters
* player shoot monsters
* monsters attack players
Developing features:
* Hit effects
* Players migrate among multiple spaces
* Server side map navigation
**Build Server:**
```bash
goworld build examples/unity_demo
```
**Run Server:**
```bash
goworld start examples/unity_demo
```
**Unity Demo Client:**
Unity demo client implements the client-server protocol in C#.
The client for unity demo is hosted at [https://github.com/xiaonanln/goworld-unity-demo](https://github.com/xiaonanln/goworld-unity-demo).
The project was created and built in [Unity 2017.1](https://unity3d.com/).
You can try the demo by downloading [GoWorldUnityDemo.zip](https://drive.google.com/file/d/1A1CJCVWFQWa-iMuAoAdHZ4JoXTtU5Q7z/view?usp=sharing).
The demo connects to a goworld server on Huawei Cloud instance.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
围棋世界Golang 中具有热重载功能的可扩展分布式游戏服务器引擎 特征建筑学介绍获取 GoWorld管理 GoWorld 服务器演示聊天室演示Unity 演示GoWorldUnityDemo.zip中文资料中文文档游戏服务器介绍目录结构说明使用GoWorld轻松实现全球聊天服务器游戏服务端开源引擎GoWorld教程1.安装和运行2. Unity例子双端联调3.手部分写一个聊天室4.多个频道的聊天室5.登录注册和存储6.移动同步和AOI7.源码解析之启动流程和热更新8.码解析之门9 .解析之dispatcher源码10.源码解析之entity欢迎加入Go服务端开发交流群662182346特征空间和实体通过 AOI 支持管理多个空间和实体分布式通过使用更多机器来增加服务器容量热插拔通过重启服务器进程来更新游戏逻辑多种通信协议支持TCP、KCP和WebSocket流量压缩和加密客户端和服务器之间的流量可以压缩和加密建筑学介绍GoWorld服务器采用实体框架,实体代表所有玩家、怪物、NPC。同一
资源推荐
资源详情
资源评论
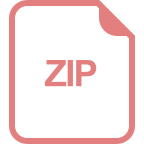
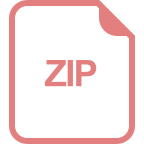
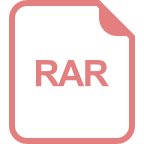
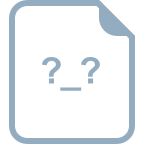
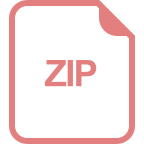
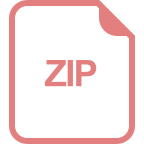
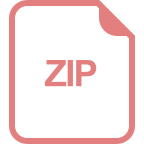
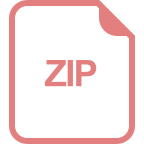
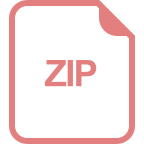
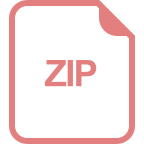
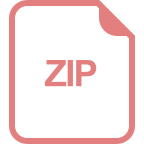
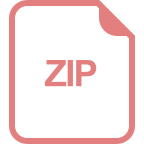
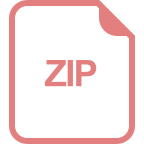
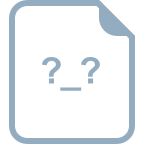
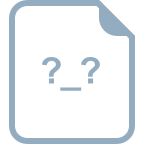
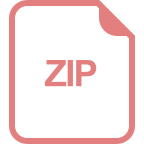
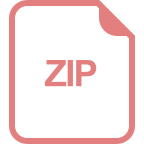
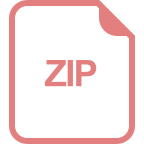
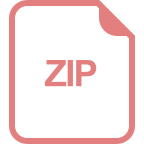
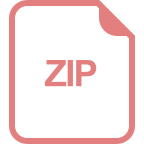
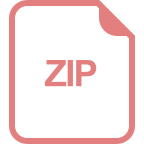
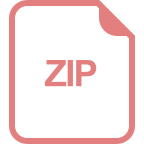
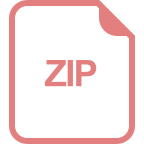
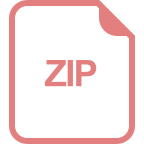
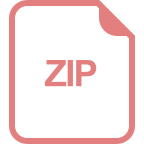
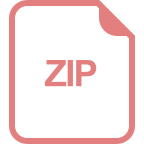
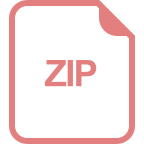
收起资源包目录

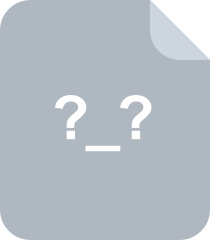
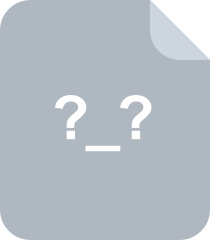
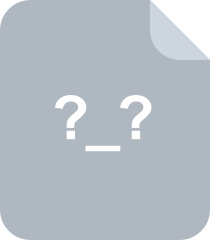
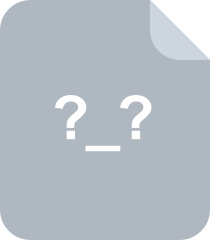
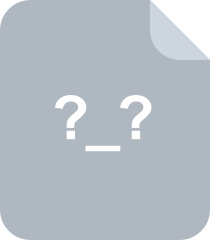
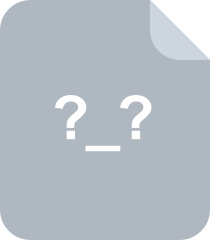
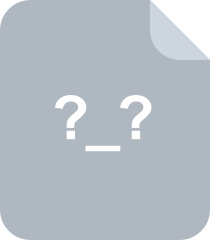
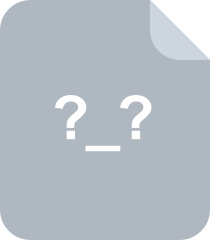
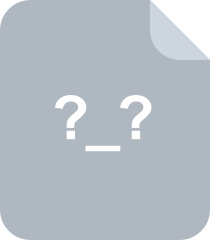
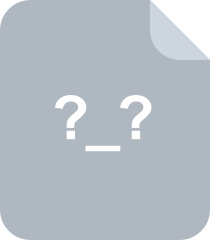
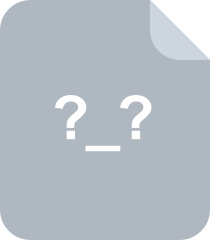
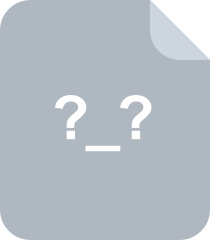
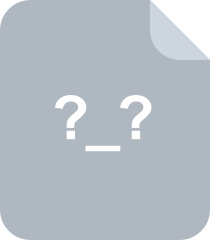
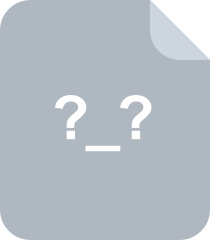
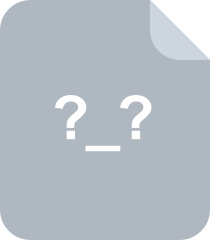
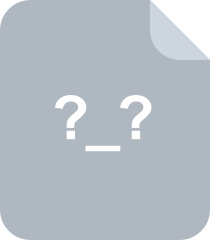
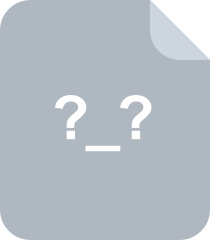
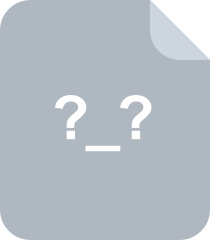
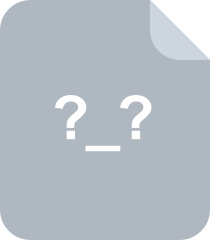
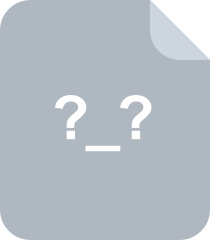
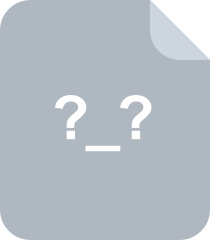
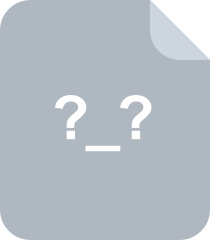
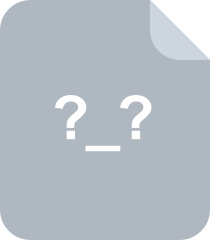
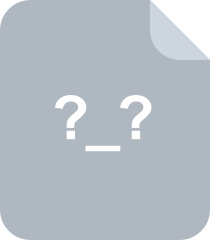
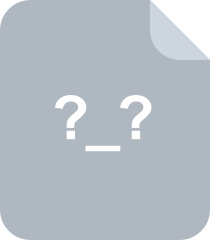
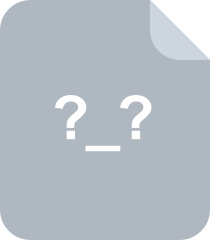
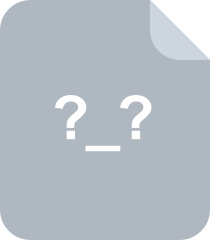
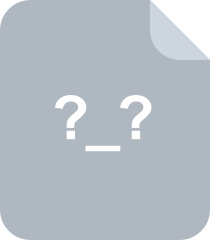
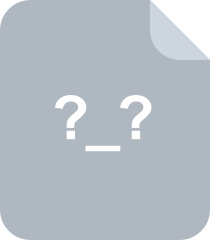
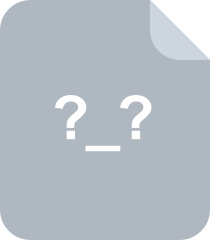
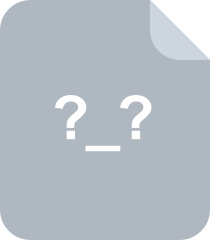
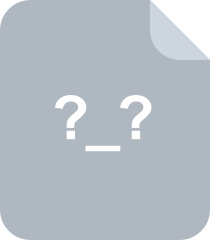
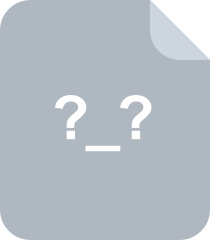
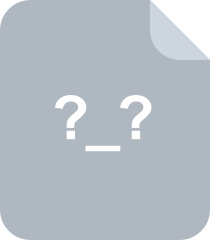
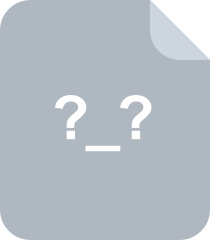
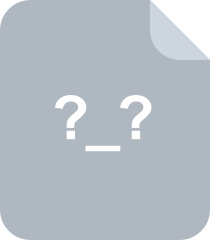
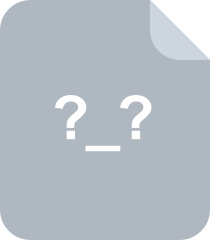
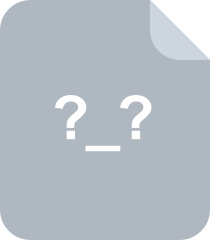
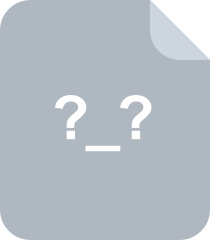
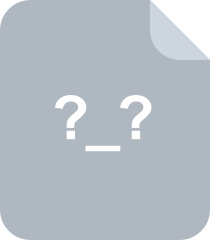
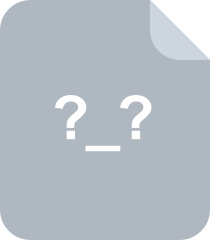
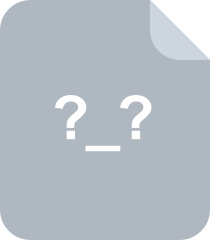
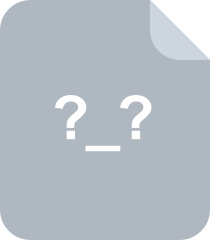
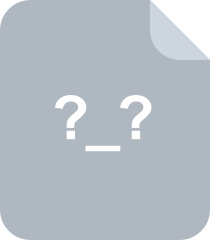
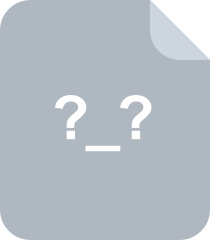
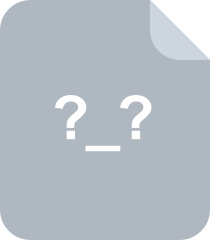
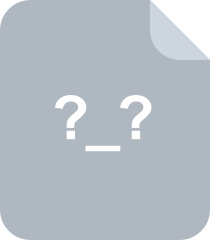
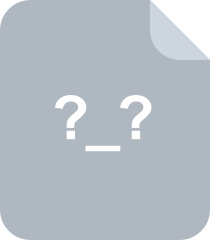
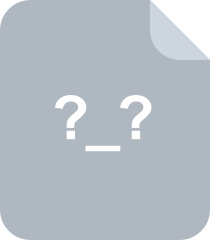
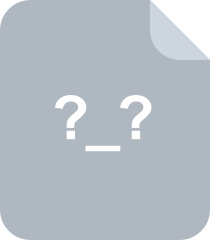
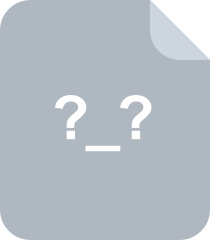
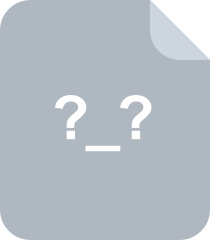
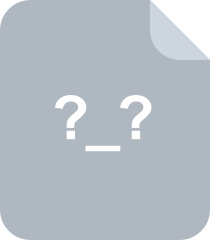
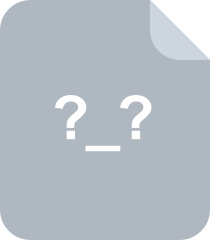
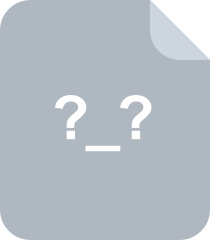
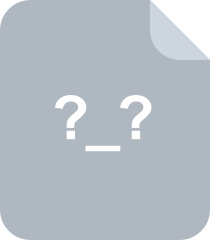
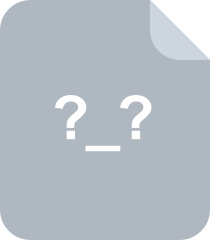
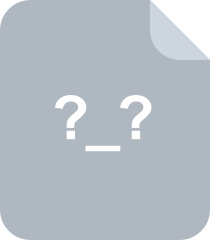
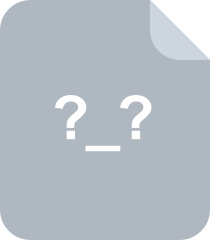
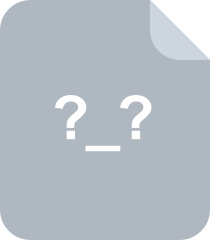
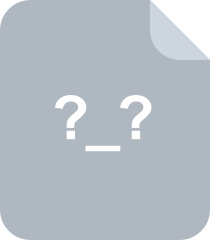
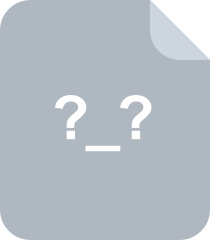
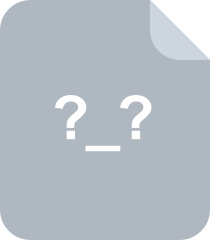
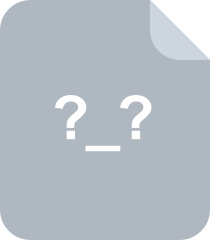
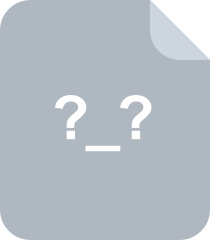
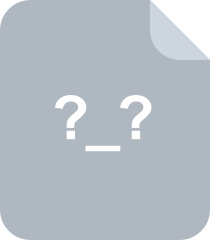
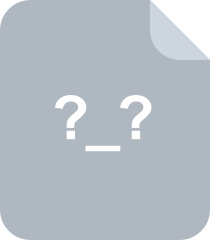
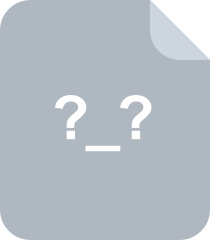
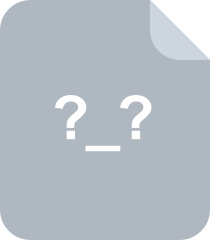
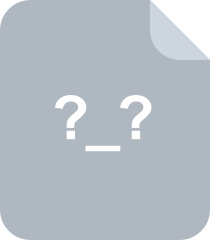
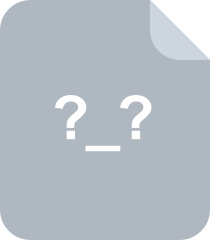
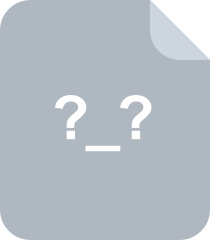
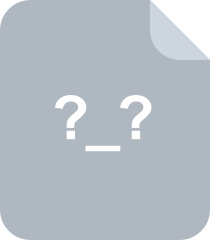
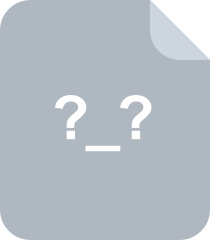
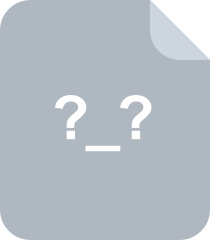
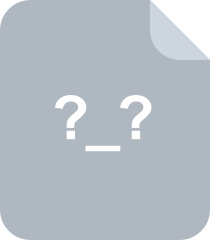
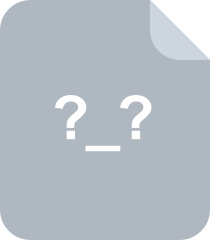
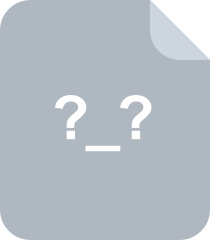
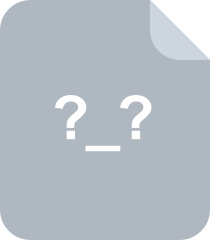
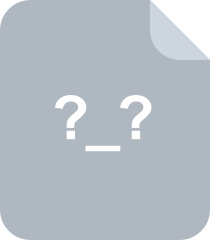
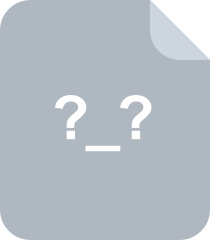
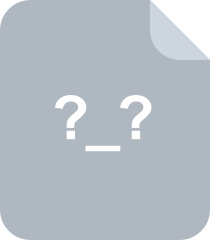
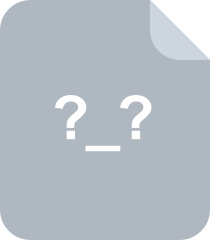
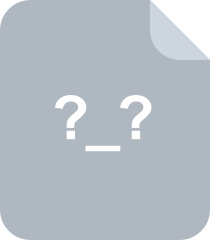
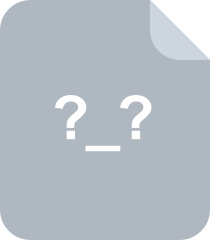
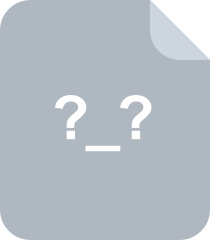
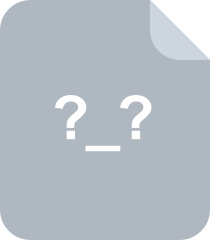
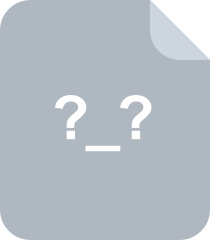
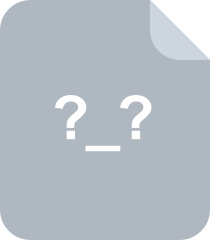
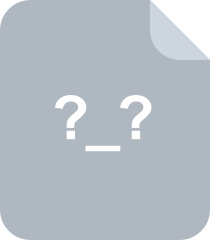
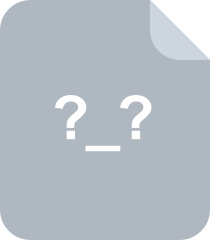
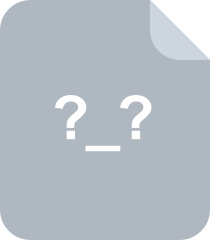
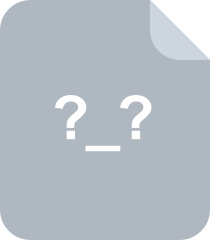
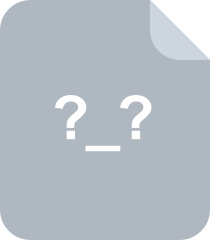
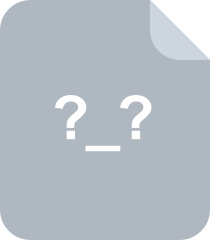
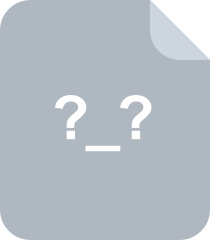
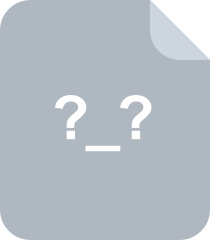
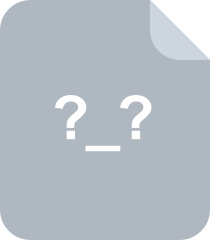
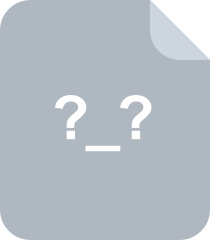
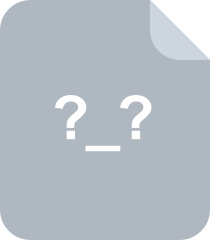
共 157 条
- 1
- 2
资源评论
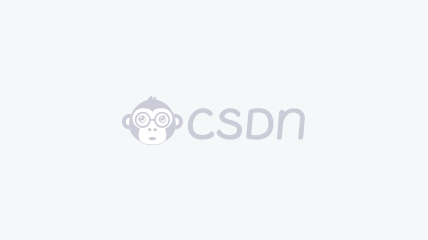

赵闪闪168
- 粉丝: 1726
- 资源: 6171
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

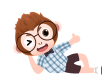
最新资源
- Universal Scanner Portable 可扫描附近设备IP
- c#语言winforms开发 使用devexpress控件DocumentManager进行多文档管理,在父窗口打开多个子窗口的实例,有详细中文解释
- zigbee CC2530无线自组网协议栈系统代码实现串口打印数据.zip
- Oracle语句优化规则汇总pdf版最新版本
- 华硕B85 pro gamer 刷NVME的bin文件,直接用工具就能用
- VSCode-win32-x64-1.96.0
- zigbee CC2530无线自组网协议栈系统代码实现带路由器的多终端点播通信例程.zip
- zigbee CC2530无线自组网协议栈系统代码实现协调器、路由器、终端的点播无线通讯.zip
- Objective-C语言教程:从基础语法到高级特性全面解析
- 888482540328469DreamFace_4.9.0.apk
- IMG_5950.jpg
- zigbee CC2530无线自组网协议栈系统代码实现协调器按键控制终端LED灯和继电器动作.zip
- zigbee CC2530无线自组网协议栈系统代码实现协调器将串口接收的指令无线发给终端并控制终端LED灯.zip
- zigbee CC2530无线自组网协议栈系统代码实现协调器与多终端的组播组网及多终端的控制.zip
- zigbee CC2530无线自组网协议栈系统代码实现协调器与终端的TI Sensor实验和Monitor使用.zip
- zigbee CC2530无线自组网协议栈系统代码实现协调器与终端的广播组网与数据传输.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


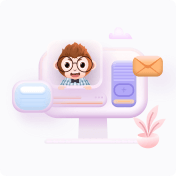
安全验证
文档复制为VIP权益,开通VIP直接复制
