# redis-rb [![Build Status][gh-actions-image]][gh-actions-link] [![Inline docs][rdoc-master-image]][rdoc-master-link]
A Ruby client that tries to match [Redis][redis-home]' API one-to-one, while still providing an idiomatic interface.
See [RubyDoc.info][rubydoc] for the API docs of the latest published gem.
## Getting started
Install with:
```
$ gem install redis
```
You can connect to Redis by instantiating the `Redis` class:
```ruby
require "redis"
redis = Redis.new
```
This assumes Redis was started with a default configuration, and is
listening on `localhost`, port 6379. If you need to connect to a remote
server or a different port, try:
```ruby
redis = Redis.new(host: "10.0.1.1", port: 6380, db: 15)
```
You can also specify connection options as a [`redis://` URL][redis-url]:
```ruby
redis = Redis.new(url: "redis://:p4ssw0rd@10.0.1.1:6380/15")
```
The client expects passwords with special characters to be URL-encoded (i.e.
`CGI.escape(password)`).
To connect to Redis listening on a Unix socket, try:
```ruby
redis = Redis.new(path: "/tmp/redis.sock")
```
To connect to a password protected Redis instance, use:
```ruby
redis = Redis.new(password: "mysecret")
```
To connect a Redis instance using [ACL](https://redis.io/topics/acl), use:
```ruby
redis = Redis.new(username: 'myname', password: 'mysecret')
```
The Redis class exports methods that are named identical to the commands
they execute. The arguments these methods accept are often identical to
the arguments specified on the [Redis website][redis-commands]. For
instance, the `SET` and `GET` commands can be called like this:
```ruby
redis.set("mykey", "hello world")
# => "OK"
redis.get("mykey")
# => "hello world"
```
All commands, their arguments, and return values are documented and
available on [RubyDoc.info][rubydoc].
## Connection Pooling and Thread safety
The client does not provide connection pooling. Each `Redis` instance
has one and only one connection to the server, and use of this connection
is protected by a mutex.
As such it is heavily recommended to use the [`connection_pool` gem](https://github.com/mperham/connection_pool), e.g.:
```ruby
module MyApp
def self.redis
@redis ||= ConnectionPool::Wrapper.new do
Redis.new(url: ENV["REDIS_URL"])
end
end
end
MyApp.redis.incr("some-counter")
```
## Sentinel support
The client is able to perform automatic failover by using [Redis
Sentinel](http://redis.io/topics/sentinel). Make sure to run Redis 2.8+
if you want to use this feature.
To connect using Sentinel, use:
```ruby
SENTINELS = [{ host: "127.0.0.1", port: 26380 },
{ host: "127.0.0.1", port: 26381 }]
redis = Redis.new(name: "mymaster", sentinels: SENTINELS, role: :master)
```
* The master name identifies a group of Redis instances composed of a master
and one or more slaves (`mymaster` in the example).
* It is possible to optionally provide a role. The allowed roles are `master`
and `slave`. When the role is `slave`, the client will try to connect to a
random slave of the specified master. If a role is not specified, the client
will connect to the master.
* When using the Sentinel support you need to specify a list of sentinels to
connect to. The list does not need to enumerate all your Sentinel instances,
but a few so that if one is down the client will try the next one. The client
is able to remember the last Sentinel that was able to reply correctly and will
use it for the next requests.
To [authenticate](https://redis.io/docs/management/sentinel/#configuring-sentinel-instances-with-authentication) Sentinel itself, you can specify the `sentinel_username` and `sentinel_password`. Exclude the `sentinel_username` option if you're using password-only authentication.
```ruby
SENTINELS = [{ host: '127.0.0.1', port: 26380},
{ host: '127.0.0.1', port: 26381}]
redis = Redis.new(name: 'mymaster', sentinels: SENTINELS, sentinel_username: 'appuser', sentinel_password: 'mysecret', role: :master)
```
If you specify a username and/or password at the top level for your main Redis instance, Sentinel *will not* using thouse credentials
```ruby
# Use 'mysecret' to authenticate against the mymaster instance, but skip authentication for the sentinels:
SENTINELS = [{ host: '127.0.0.1', port: 26380 },
{ host: '127.0.0.1', port: 26381 }]
redis = Redis.new(name: 'mymaster', sentinels: SENTINELS, role: :master, password: 'mysecret')
```
So you have to provide Sentinel credential and Redis explicitly even they are the same
```ruby
# Use 'mysecret' to authenticate against the mymaster instance and sentinel
SENTINELS = [{ host: '127.0.0.1', port: 26380 },
{ host: '127.0.0.1', port: 26381 }]
redis = Redis.new(name: 'mymaster', sentinels: SENTINELS, role: :master, password: 'mysecret', sentinel_password: 'mysecret')
```
Also the `name`, `password`, `username` and `db` for Redis instance can be passed as an url:
```ruby
redis = Redis.new(url: "redis://appuser:mysecret@mymaster/10", sentinels: SENTINELS, role: :master)
```
## Cluster support
[Clustering](https://redis.io/topics/cluster-spec). is supported via the [`redis-clustering` gem](cluster/).
## Pipelining
When multiple commands are executed sequentially, but are not dependent,
the calls can be *pipelined*. This means that the client doesn't wait
for reply of the first command before sending the next command. The
advantage is that multiple commands are sent at once, resulting in
faster overall execution.
The client can be instructed to pipeline commands by using the
`#pipelined` method. After the block is executed, the client sends all
commands to Redis and gathers their replies. These replies are returned
by the `#pipelined` method.
```ruby
redis.pipelined do |pipeline|
pipeline.set "foo", "bar"
pipeline.incr "baz"
end
# => ["OK", 1]
```
Commands must be called on the yielded objects. If you call methods
on the original client objects from inside a pipeline, they will be sent immediately:
```ruby
redis.pipelined do |pipeline|
pipeline.set "foo", "bar"
redis.incr "baz" # => 1
end
# => ["OK"]
```
### Exception management
The `exception` flag in the `#pipelined` is a feature that modifies the pipeline execution behavior. When set
to `false`, it doesn't raise an exception when a command error occurs. Instead, it allows the pipeline to execute all
commands, and any failed command will be available in the returned array. (Defaults to `true`)
```ruby
results = redis.pipelined(exception: false) do |pipeline|
pipeline.set('key1', 'value1')
pipeline.lpush('key1', 'something') # This will fail
pipeline.set('key2', 'value2')
end
# results => ["OK", #<RedisClient::WrongTypeError: WRONGTYPE Operation against a key holding the wrong kind of value>, "OK"]
results.each do |result|
if result.is_a?(Redis::CommandError)
# Do something with the failed result
end
end
```
### Executing commands atomically
You can use `MULTI/EXEC` to run a number of commands in an atomic
fashion. This is similar to executing a pipeline, but the commands are
preceded by a call to `MULTI`, and followed by a call to `EXEC`. Like
the regular pipeline, the replies to the commands are returned by the
`#multi` method.
```ruby
redis.multi do |transaction|
transaction.set "foo", "bar"
transaction.incr "baz"
end
# => ["OK", 1]
```
### Futures
Replies to commands in a pipeline can be accessed via the *futures* they
emit. All calls on the pipeline object return a
`Future` object, which responds to the `#value` method. When the
pipeline has successfully executed, all futures are assigned their
respective replies and can be used.
```ruby
set = incr = nil
redis.pipelined do |pipeline|
set = pipeline.set "foo", "bar"
incr = pipeline.incr "baz"
end
set.value
# => "OK"
incr.value
# => 1
```
## Error Handling
In general, if something goes wrong you'll get an exception. For example, if
it can't connect to the server a `Redis::CannotConnectError` erro
没有合适的资源?快使用搜索试试~ 我知道了~
Redis 的 Ruby 客户端库.zip
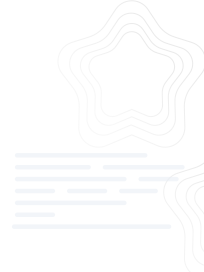
共170个文件
rb:126个
conf:6个
md:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 162 浏览量
2024-12-02
21:08:56
上传
评论
收藏 167KB ZIP 举报
温馨提示
Redis 的 Ruby 客户端库redis-rb Ruby 客户端尝试与Redis的 API 一对一匹配,同时仍提供惯用的接口。请参阅RubyDoc.info了解最新发布的 gem 的 API 文档。入门安装方式$ gem install redis您可以通过实例化类来连接到 Redis Redisrequire "redis"redis = Redis.new假设 Redis 以默认配置启动,并且正在监听localhost端口 6379。如果您需要连接到远程服务器或其他端口,请尝试redis = Redis.new(host: "10.0.1.1", port: 6380, db: 15)您还可以将连接选项指定为redis://URLredis = Redis.new(url: "redis://:p4ssw0rd@10.0.1.1:6380/15")客户端希望带有特殊字符的密码进行URL编码(即 CGI.escape(password))。要连接到在 Unix 套接字上监听的 Redis,请尝试redis = Red
资源推荐
资源详情
资源评论
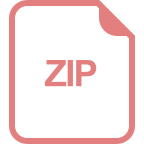
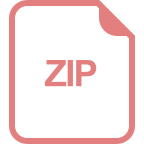
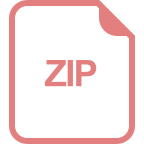
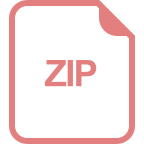
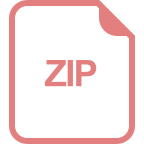
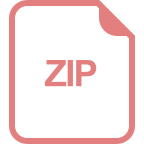
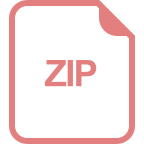
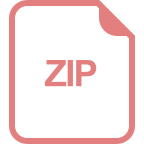
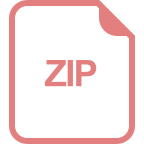
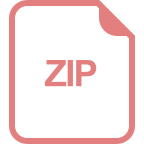
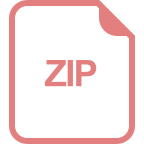
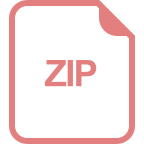
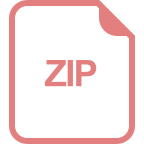
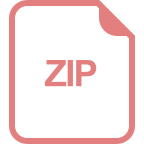
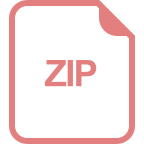
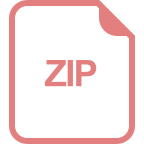
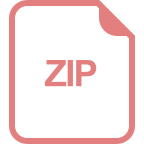
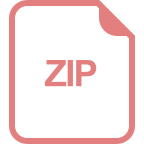
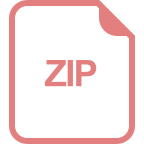
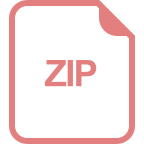
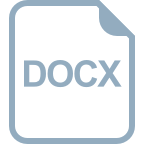
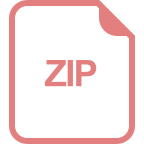
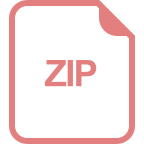
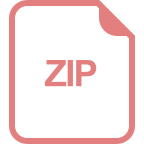
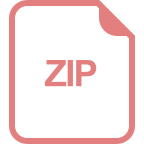
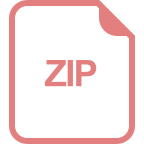
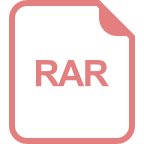
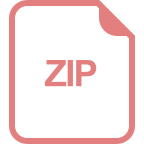
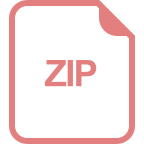
收起资源包目录

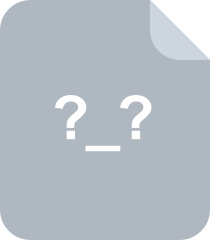
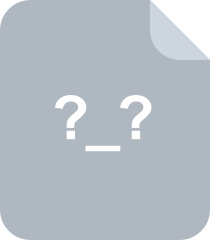
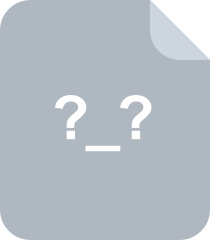
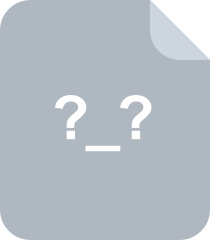
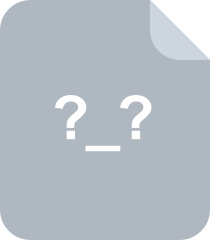
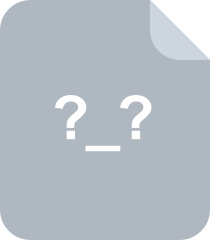
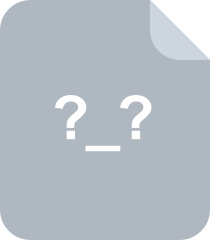
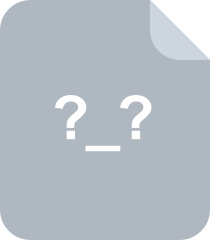
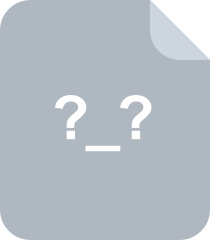
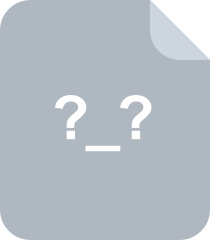
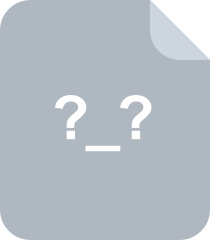
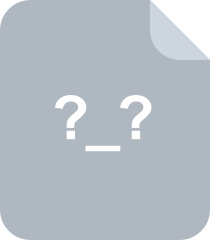
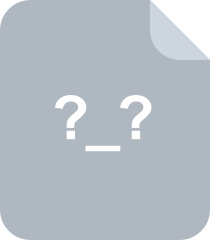
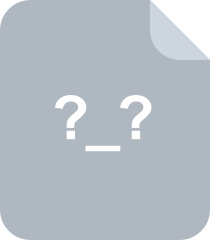
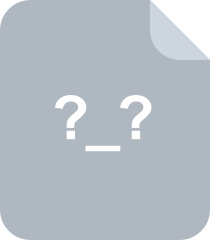
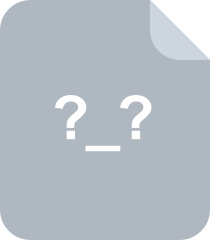
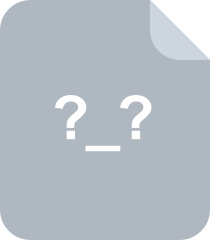
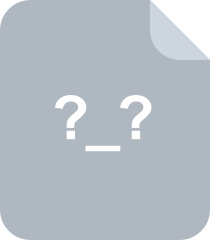
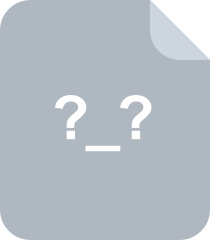
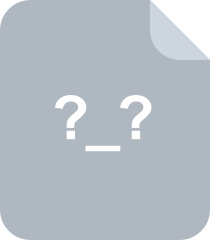
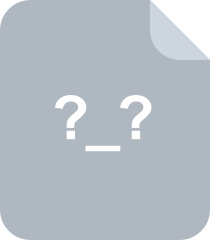
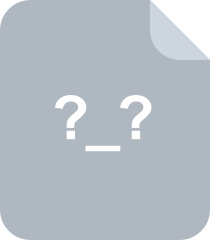
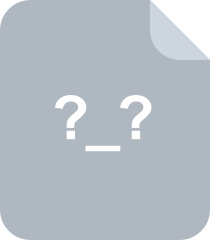
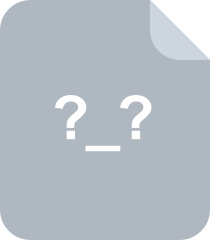
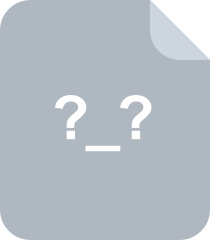
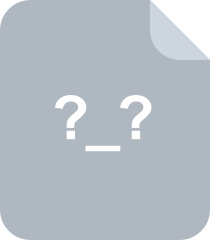
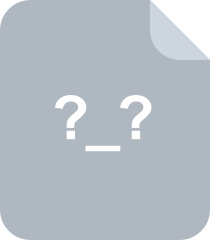
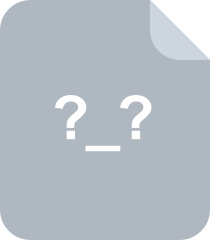
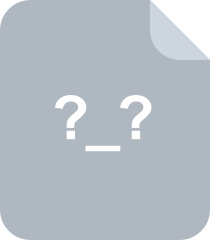
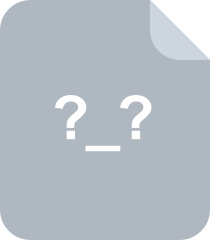
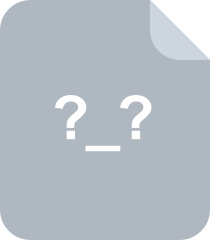
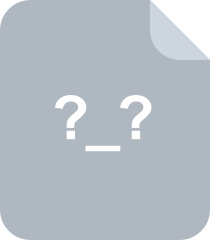
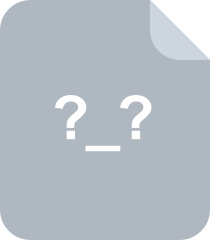
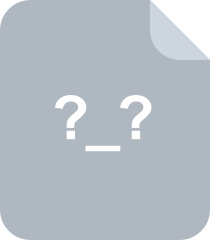
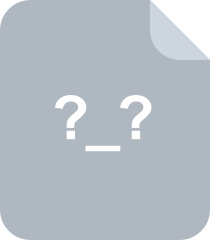
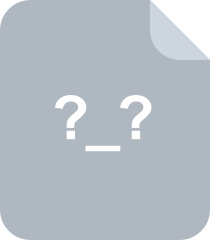
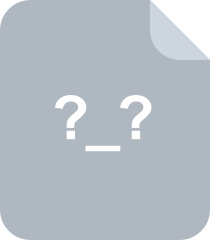
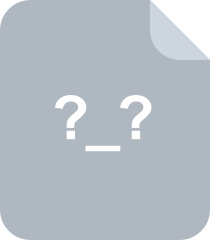
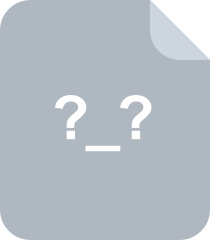
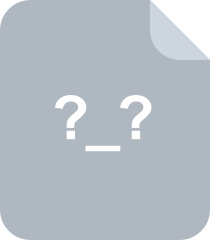
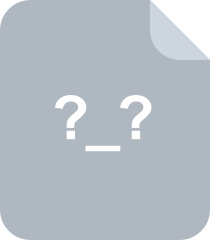
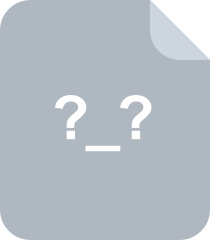
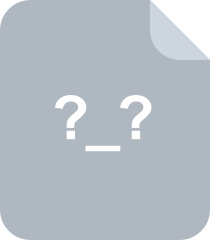
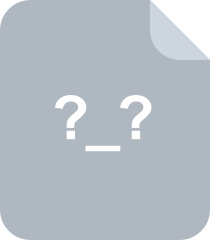
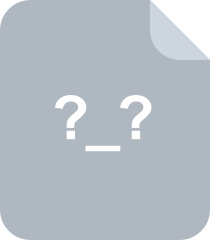
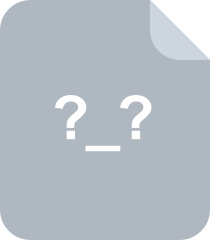
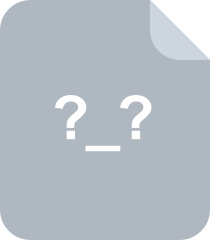
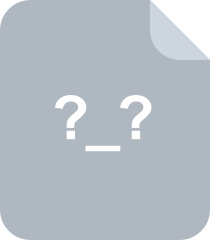
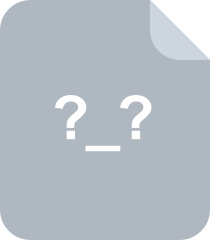
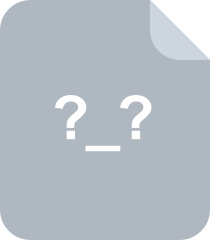
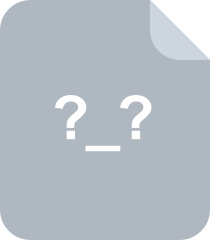
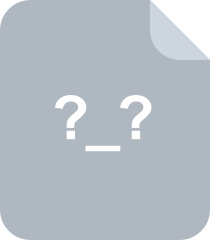
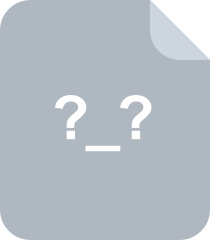
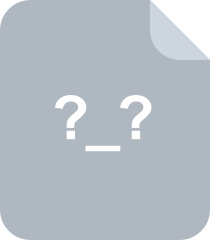
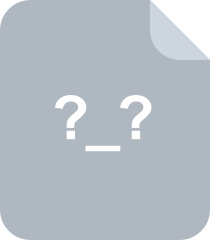
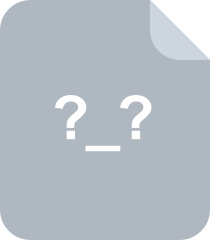
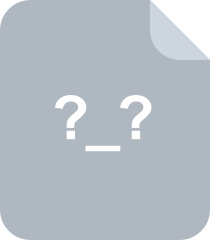
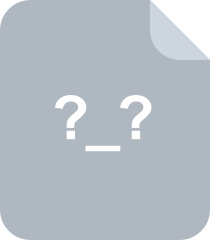
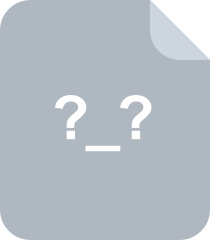
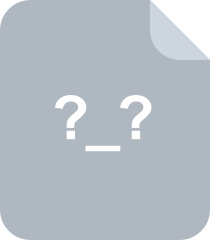
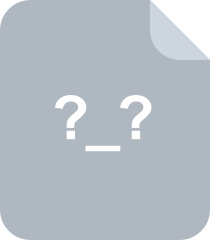
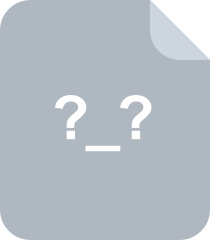
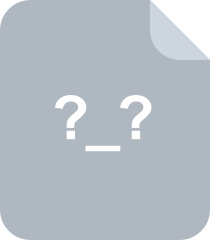
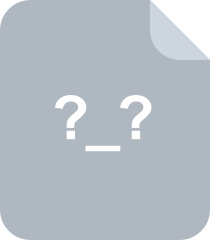
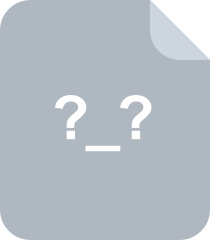
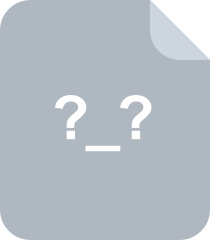
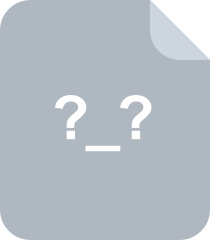
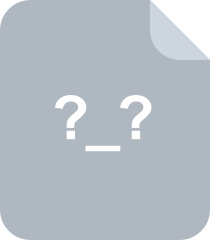
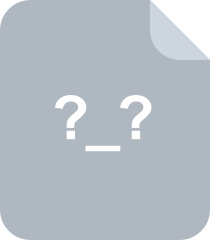
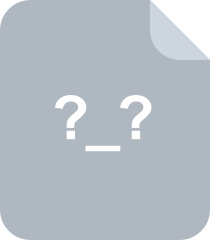
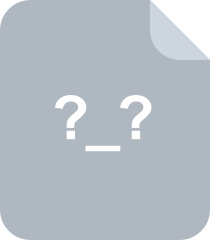
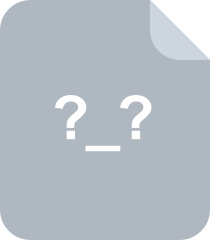
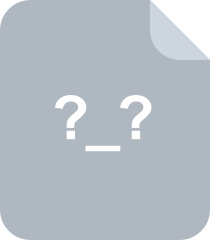
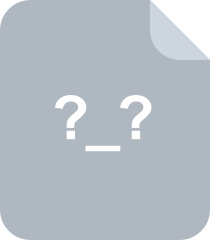
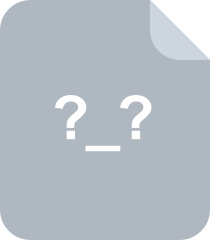
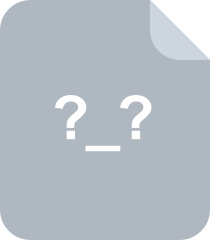
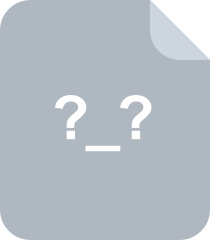
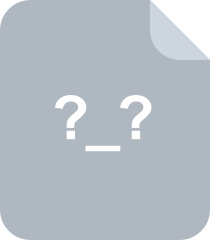
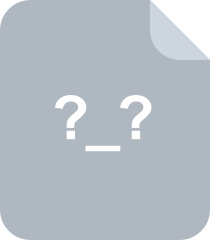
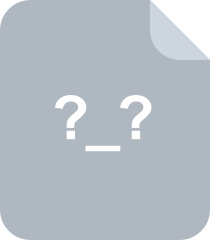
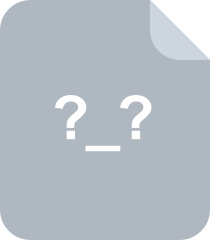
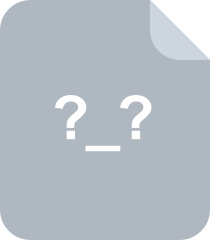
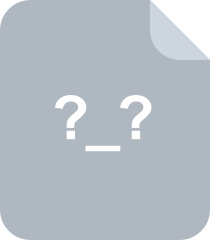
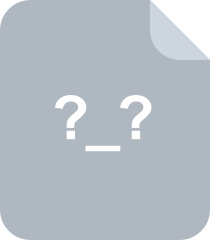
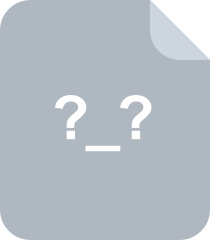
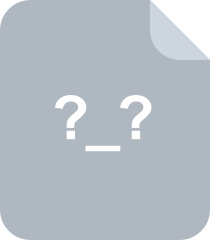
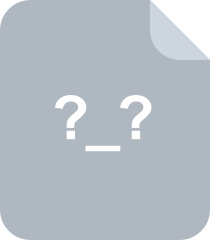
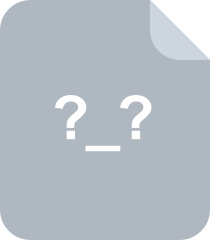
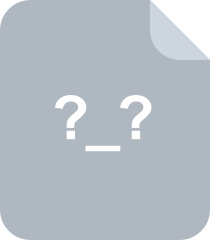
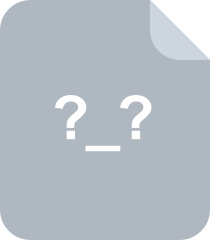
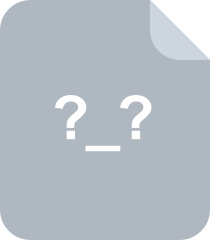
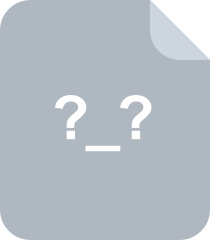
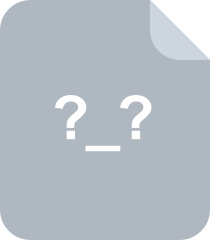
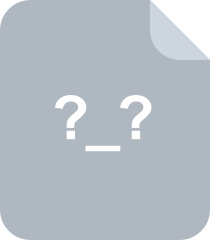
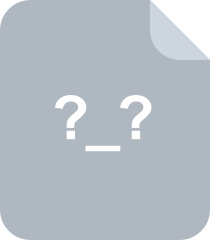
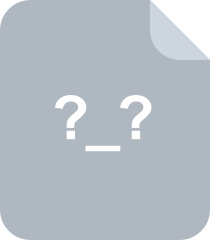
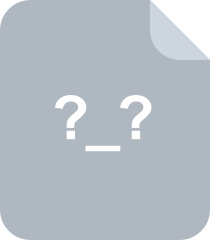
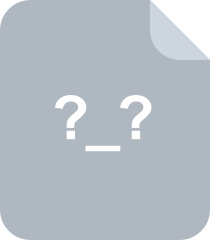
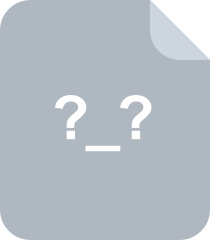
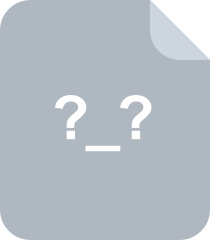
共 170 条
- 1
- 2
资源评论
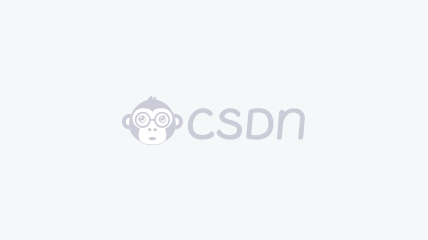

赵闪闪168
- 粉丝: 1646
- 资源: 4872
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

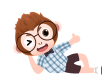
最新资源
- 主要物体检测15-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- Google Maps API Web 服务的 Python 客户端库.zip
- Google Authenticator 服务器端代码.zip
- logo标志检测26-YOLOv7、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- golang 的算法和数据结构.zip
- Vue + SpringBoot前后端项目实例
- Golang 日志库.zip
- DET组件查找器检测15-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- jsp实现增删改查,自行建立数据库和表,表的四个字段分别为 name ,stuid , zhuanye ,id 主键自增,stuid 添加 unique 约束,已解决类爆炸问题
- 第02章 文件与用户管理
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


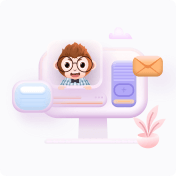
安全验证
文档复制为VIP权益,开通VIP直接复制
