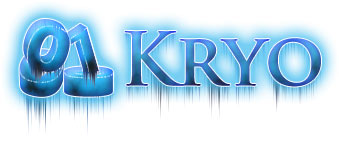
[](https://github.com/EsotericSoftware/kryo/actions/workflows/ci-workflow.yml)
[](http://search.maven.org/#search%7Cga%7C1%7Cg%3A%22com.esotericsoftware%22%20AND%20a%3Akryo)
[](https://gitter.im/EsotericSoftware/kryo)
[](https://bugs.chromium.org/p/oss-fuzz/issues/list?sort=-opened&can=1&q=proj:kryo)
Kryo is a fast and efficient binary object graph serialization framework for Java. The goals of the project are high speed, low size, and an easy to use API. The project is useful any time objects need to be persisted, whether to a file, database, or over the network.
Kryo can also perform automatic deep and shallow copying/cloning. This is direct copying from object to object, not object to bytes to object.
This documentation is for Kryo version 5.x. See [the Wiki](https://github.com/EsotericSoftware/kryo/wiki/Kryo-v4) for version 4.x.
## Contact / Mailing list
Please use the [Kryo mailing list](https://groups.google.com/forum/#!forum/kryo-users) for questions, discussions, and support. Please limit use of the Kryo issue tracker to bugs and enhancements, not questions, discussions, or support.
## Table of contents
- [Recent releases](#recent-releases)
- [Installation](#installation)
* [With Maven](#with-maven)
* [Without Maven](#without-maven)
* [Building from source](#building-from-source)
- [Quickstart](#quickstart)
- [IO](#io)
* [Output](#output)
* [Input](#input)
* [ByteBuffers](#bytebuffers)
* [Unsafe buffers](#unsafe-buffers)
* [Variable length encoding](#variable-length-encoding)
* [Chunked encoding](#chunked-encoding)
* [Buffer performance](#buffer-performance)
- [Reading and writing objects](#reading-and-writing-objects)
* [Round trip](#round-trip)
* [Deep and shallow copies](#deep-and-shallow-copies)
* [References](#references)
+ [ReferenceResolver](#referenceresolver)
+ [Reference limits](#reference-limits)
* [Context](#context)
* [Reset](#reset)
- [Serializer framework](#serializer-framework)
* [Registration](#registration)
+ [ClassResolver](#classresolver)
+ [Optional registration](#optional-registration)
* [Default serializers](#default-serializers)
+ [Serializer factories](#serializer-factories)
* [Object creation](#object-creation)
+ [InstantiatorStrategy](#instantiatorstrategy)
+ [Overriding create](#overriding-create)
* [Final classes](#final-classes)
* [Closures](#closures)
* [Compression and encryption](#compression-and-encryption)
- [Implementing a serializer](#implementing-a-serializer)
* [Serializer references](#serializer-references)
+ [Nested serializers](#nested-serializers)
+ [KryoException](#kryoexception)
+ [Stack size](#stack-size)
* [Accepting null](#accepting-null)
* [Generics](#generics)
* [KryoSerializable](#kryoserializable)
* [Serializer copying](#serializer-copying)
+ [KryoCopyable](#kryocopyable)
+ [Immutable serializers](#immutable-serializers)
- [Kryo versioning and upgrading](#kryo-versioning-and-upgrading)
- [Interoperability](#interoperability)
- [Compatibility](#compatibility)
* [Replacing a class](#replacing-a-class)
- [Serializers](#serializers)
* [FieldSerializer](#fieldserializer)
+ [CachedField settings](#cachedfield-settings)
+ [FieldSerializer annotations](#fieldserializer-annotations)
* [VersionFieldSerializer](#versionfieldserializer)
* [TaggedFieldSerializer](#taggedfieldserializer)
* [CompatibleFieldSerializer](#compatiblefieldserializer)
* [BeanSerializer](#beanserializer)
* [CollectionSerializer](#collectionserializer)
* [MapSerializer](#mapserializer)
* [JavaSerializer and ExternalizableSerializer](#javaserializer-and-externalizableserializer)
- [Logging](#logging)
- [Thread safety](#thread-safety)
* [Pooling](#pooling)
- [Benchmarks](#benchmarks)
- [Links](#links)
* [Projects using Kryo](#projects-using-kryo)
* [Scala](#scala)
* [Clojure](#clojure)
* [Objective-C](#objective-c)
## Recent releases
* [4.0.3](https://github.com/EsotericSoftware/kryo/releases/tag/kryo-parent-4.0.3) - brings bug fixes and performance improvements for chunked encoding.
* [5.6.2](https://github.com/EsotericSoftware/kryo/releases/tag/kryo-parent-5.6.2) - recompiles 5.6.1 to be compatible with Java 8 again
* [5.6.1](https://github.com/EsotericSoftware/kryo/releases/tag/kryo-parent-5.6.1) - brings a bug fix for the Maven coordinates of the versioned artifact
* [5.6.0](https://github.com/EsotericSoftware/kryo/releases/tag/kryo-parent-5.6.0) - brings bug fixes and performance improvements.
* [5.5.0](https://github.com/EsotericSoftware/kryo/releases/tag/kryo-parent-5.5.0) - brings bug fixes and performance improvements.
## Installation
Kryo publishes two kinds of artifacts/jars:
* the default jar (with the usual library dependencies) which is meant for direct usage in applications (not libraries)
* a dependency-free, "versioned" jar which should be used by other libraries. Different libraries shall be able to use different major versions of Kryo.
Kryo JARs are available on the [releases page](https://github.com/EsotericSoftware/kryo/releases) and at [Maven Central](https://search.maven.org/#search|gav|1|g%3Acom.esotericsoftware%20a%3Akryo). The latest snapshots of Kryo, including snapshot builds of master, are in the [Sonatype Repository](https://oss.sonatype.org/content/repositories/snapshots/com/esotericsoftware/kryo/).
### With Maven
To use the latest Kryo release in your application, use this dependency entry in your `pom.xml`:
```xml
<dependency>
<groupId>com.esotericsoftware</groupId>
<artifactId>kryo</artifactId>
<version>5.6.2</version>
</dependency>
```
To use the latest Kryo release in a library you want to publish, use this dependency entry in your `pom.xml`:
```xml
<dependency>
<groupId>com.esotericsoftware.kryo</groupId>
<artifactId>kryo5</artifactId>
<version>5.6.2</version>
</dependency>
```
To use the latest Kryo snapshot, use:
```xml
<repository>
<id>sonatype-snapshots</id>
<name>sonatype snapshots repo</name>
<url>https://oss.sonatype.org/content/repositories/snapshots</url>
</repository>
<!-- for usage in an application: -->
<dependency>
<groupId>com.esotericsoftware</groupId>
<artifactId>kryo</artifactId>
<version>5.6.3-SNAPSHOT</version>
</dependency>
<!-- for usage in a library that should be published: -->
<dependency>
<groupId>com.esotericsoftware.kryo</groupId>
<artifactId>kryo5</artifactId>
<version>5.6.3-SNAPSHOT</version>
</dependency>
```
### Without Maven
Not everyone is a Maven fan. Using Kryo without Maven requires placing the [Kryo JAR](#installation) on your classpath along with the dependency JARs found in [lib](https://github.com/EsotericSoftware/kryo/tree/master/lib).
### Building from source
Building Kryo from source requires JDK11+ and Maven. To build all artifacts, run:
```
mvn clean && mvn install
```
## Quickstart
Jumping ahead to show how the library can be used:
```java
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.io.Input;
import com.esotericsoftware.kryo.io.Output;
import java.io.*;
public class HelloKryo {
static public void main (String[] args) throws Exception {
Kryo kryo = new Kryo();
kryo.register(SomeClass.class);
SomeClass object = new SomeClass();
object.value = "Hello Kryo!";
Output output = new Output(new FileOutputStream("file.bin"));
kryo.writeObject(output,
没有合适的资源?快使用搜索试试~ 我知道了~
Java二进制序列化与克隆快速、高效、自动化.zip
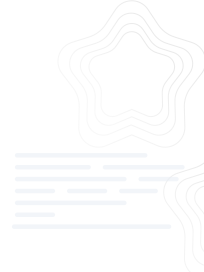
共222个文件
java:133个
html:33个
jar:13个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 162 浏览量
2024-11-25
00:05:36
上传
评论
收藏 8.9MB ZIP 举报
温馨提示
Kryo 是一个快速高效的 Java 二进制对象图序列化框架。该项目的目标是实现高速、小尺寸和易于使用的 API。当需要将对象持久化时,无论是将对象持久化到文件、数据库还是通过网络,该项目都非常有用。Kryo 还可以自动执行深层和浅层复制/克隆。这是从对象到对象的直接复制,而不是从对象到字节再到对象的复制。本文档适用于 Kryo 版本 5.x。请参阅4.x 版本的Wiki 。联系方式/邮件列表请使用Kryo 邮件列表来提问、讨论和获取支持。请将 Kryo 问题跟踪器的使用限制在错误和改进上,而不是提问、讨论或支持上。目录近期发布安装使用 Maven没有 Maven从源代码构建快速入门输入输出输出输入字节缓冲区不安全的缓冲区可变长度编码分块编码缓冲性能读写对象往返深拷贝和浅拷贝参考引用解析器参考限值语境重置序列化器框架登记类解析器可选注册默认序列化器序列化器工厂对象创建实例化策略覆盖创建最后课程闭包压缩和加密实现序列化器序列化器参考嵌套序列化程序Kryo异常堆栈大小接受 null
资源推荐
资源详情
资源评论
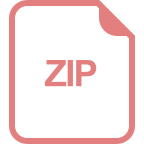
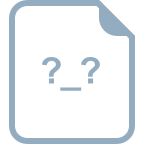
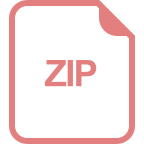
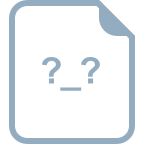
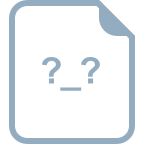
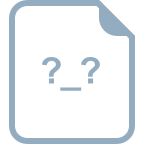
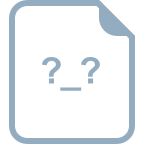
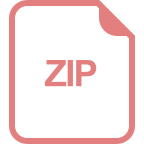
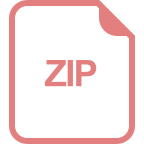
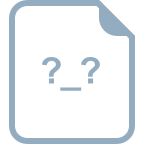
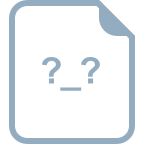
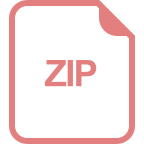
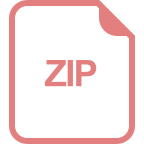
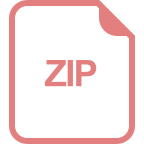
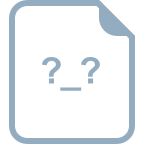
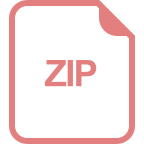
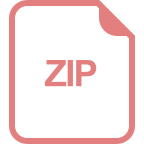
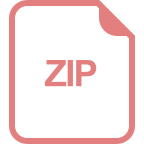
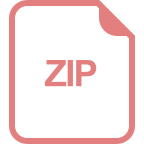
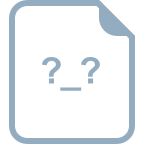
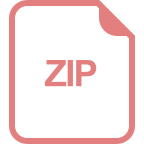
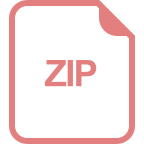
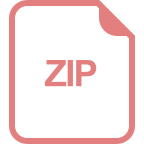
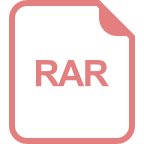
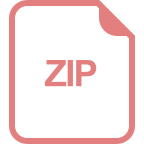
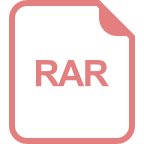
收起资源包目录

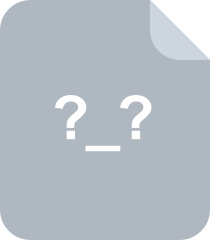
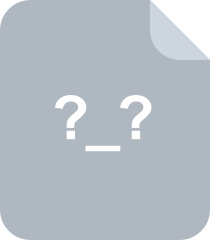
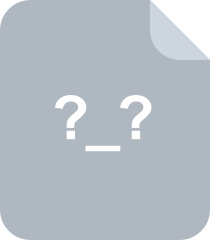
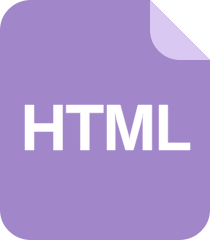
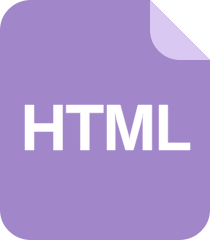
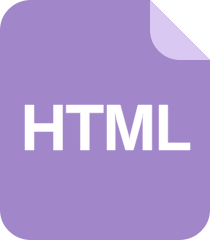
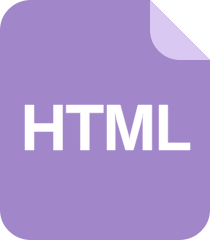
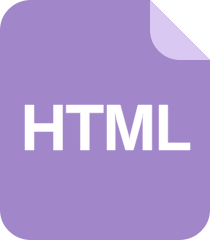
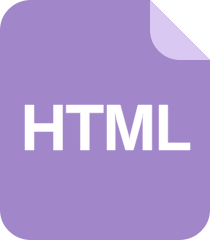
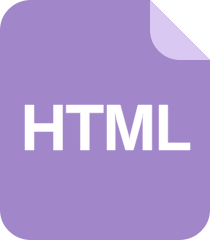
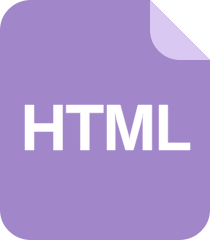
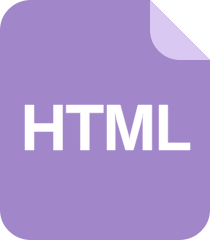
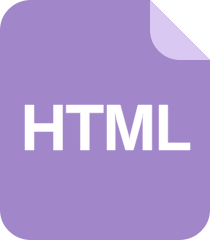
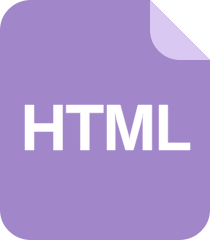
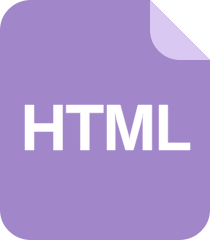
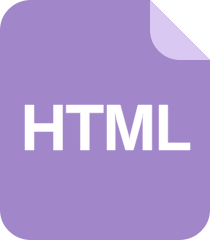
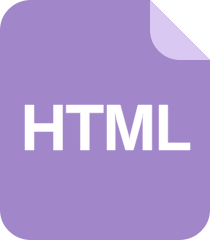
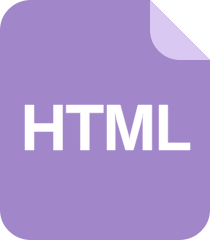
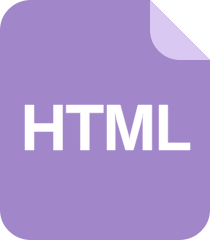
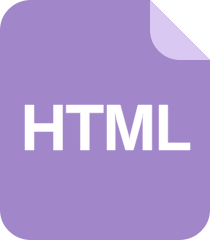
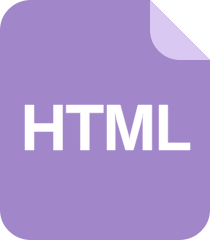
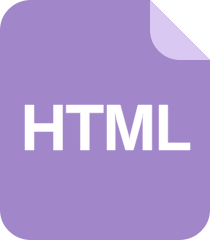
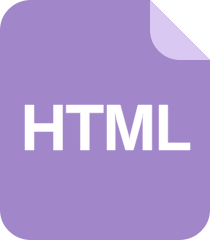
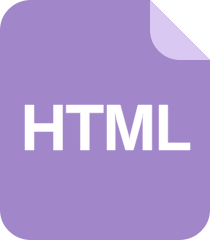
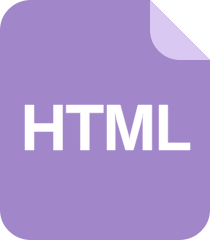
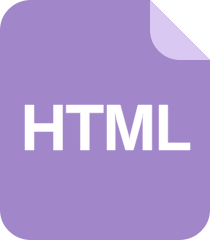
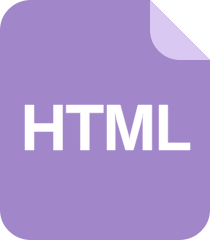
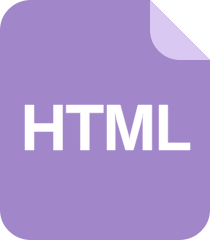
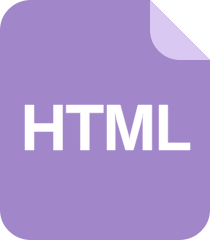
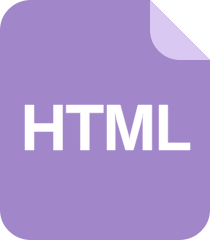
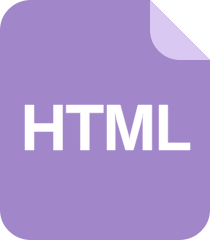
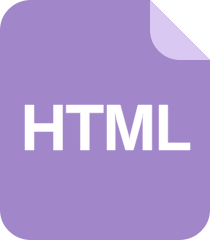
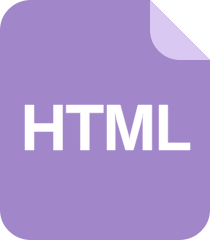
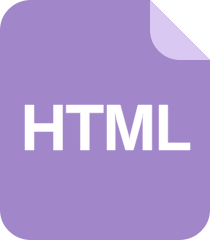
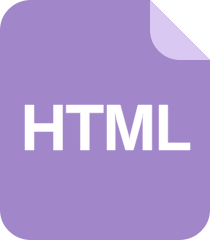
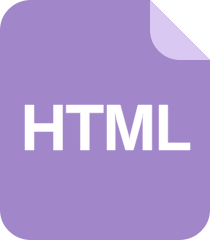
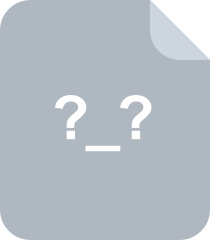
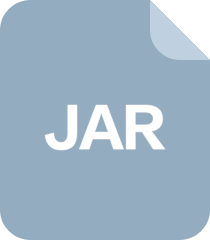
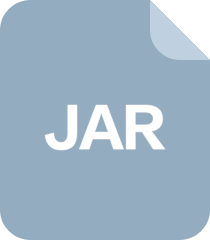
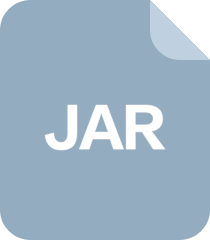
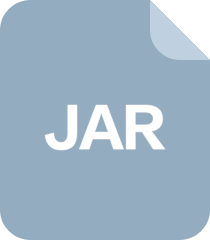
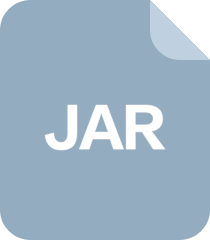
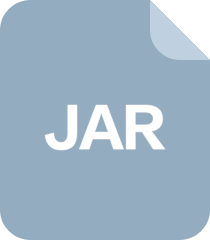
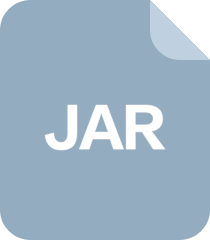
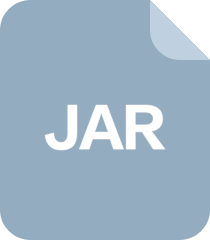
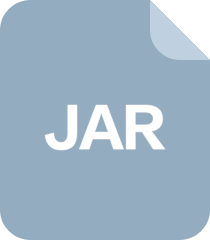
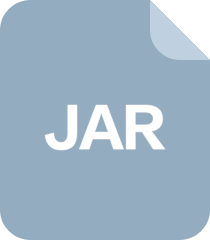
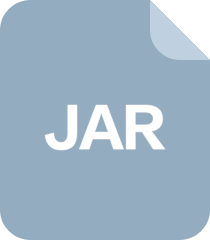
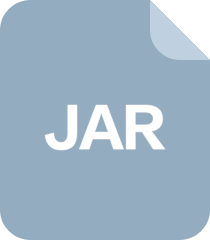
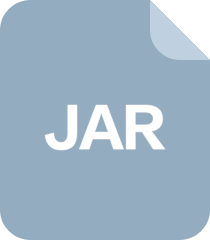
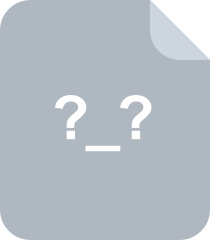
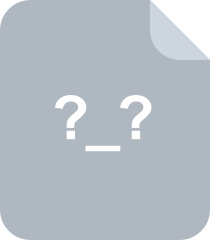
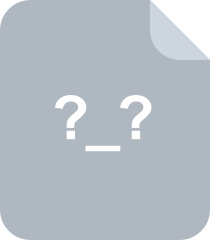
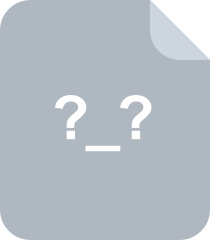
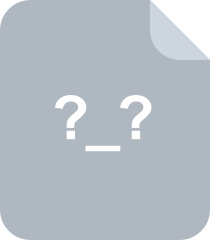
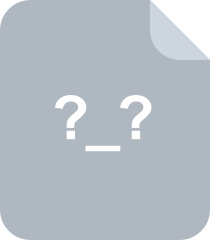
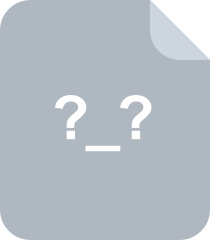
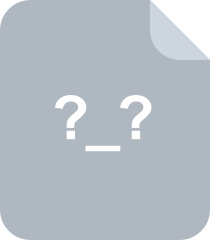
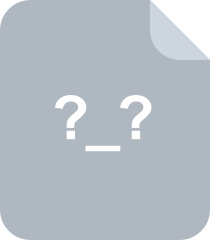
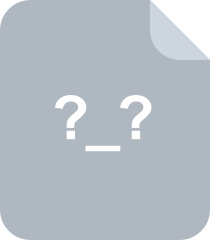
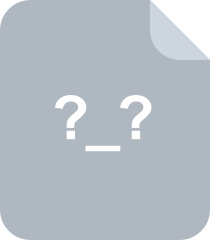
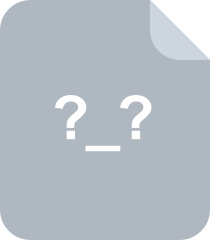
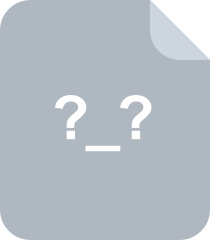
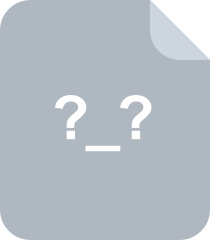
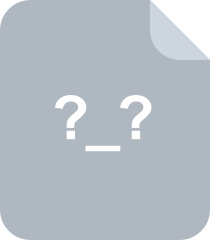
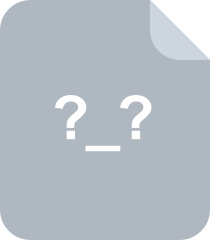
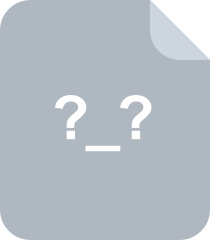
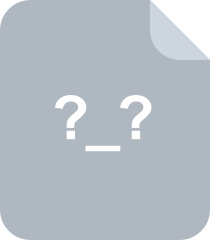
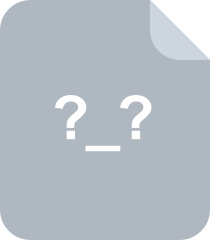
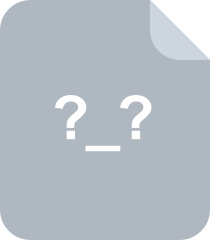
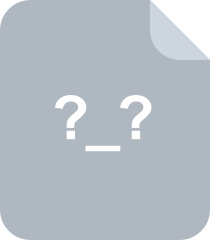
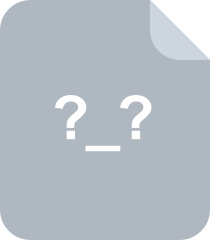
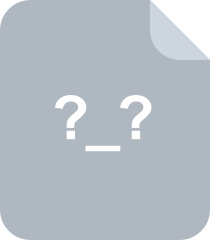
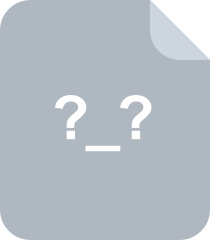
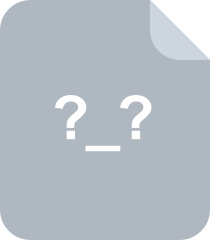
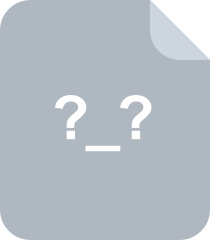
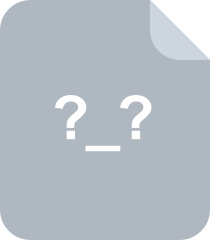
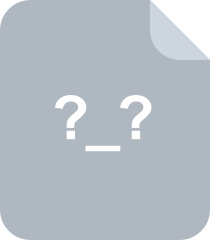
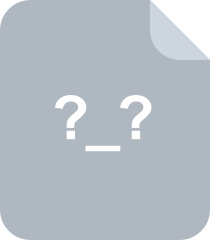
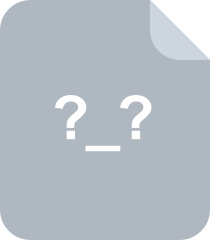
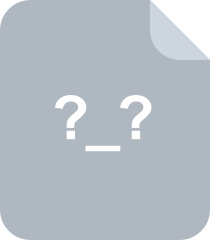
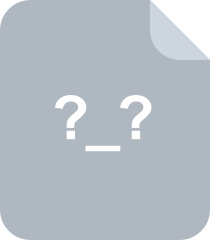
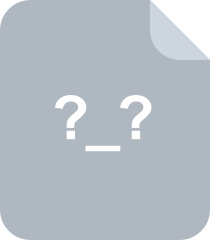
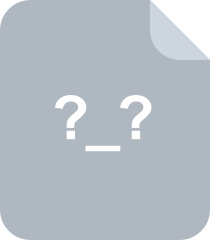
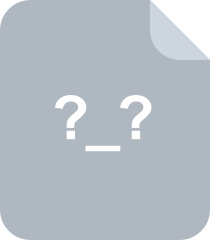
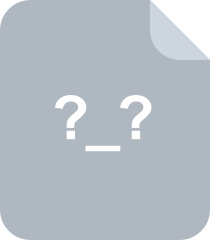
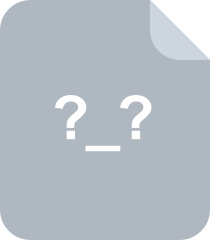
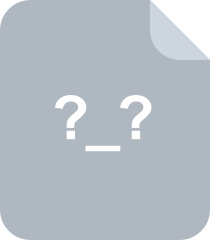
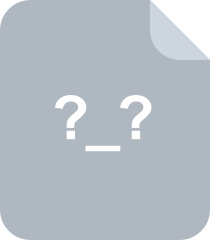
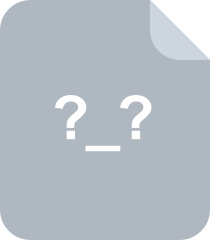
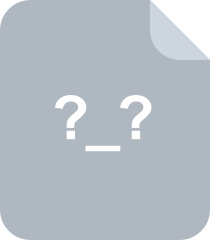
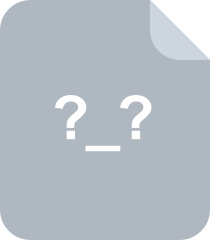
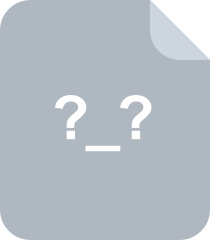
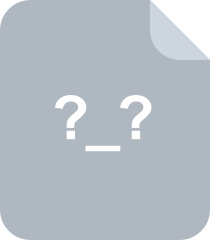
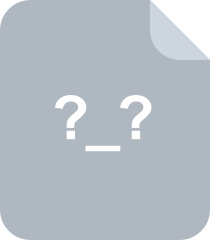
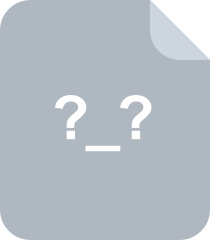
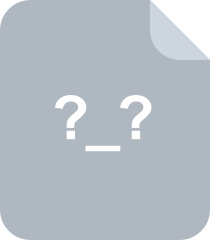
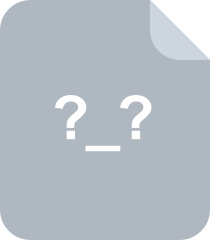
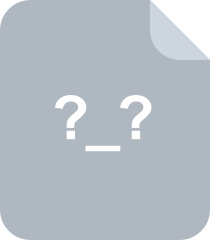
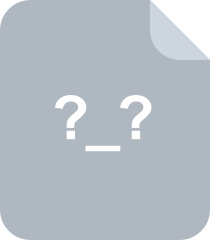
共 222 条
- 1
- 2
- 3
资源评论
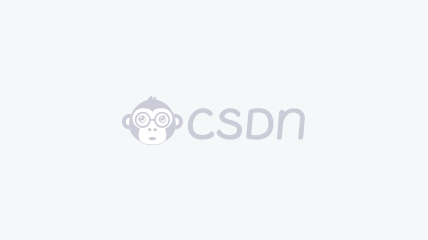

赵闪闪168
- 粉丝: 1533
- 资源: 3077
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

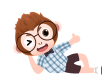
最新资源
- GJB150A-2009军用装备实验室环境试验方法(共19份标准文件)
- 浩辰CAD看图王8.6.0最新版本下载,轻量化CAD看图软件,无需下载专业CAD软件,即可实现CAD看图、CAD图纸编辑、格式转换、三维览图等
- SW materials
- 英雄联盟评论数据集和停用词表
- 整合Springboot shiro jpa mysql 实现权限管理系统(附源码地址)
- 微信小游戏小鸟飞行游戏
- 20190313-100538-非对称电容在变压器油中10kv高压电作用下产生力的现象
- GB材料数据库(!请注意鉴别其中的材料参数并不是完全正确!)
- JAVA商城,支持小程序商城、 供应链商城 小程序商城 H5商城 app商城超全商城模式官网 支持小程序商城 H5商城 APP商城 PC商城
- springboot的在线商城系统设计与开发源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


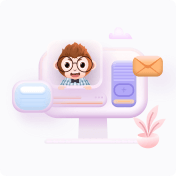
安全验证
文档复制为VIP权益,开通VIP直接复制
