#!/bin/sh
# Uncomment the following line to override the JVM search sequence
# INSTALL4J_JAVA_HOME_OVERRIDE=
# Uncomment the following line to add additional VM parameters
# INSTALL4J_ADD_VM_PARAMS=
INSTALL4J_JAVA_PREFIX=""
GREP_OPTIONS=""
fill_version_numbers() {
if [ "$ver_major" = "" ]; then
ver_major=0
fi
if [ "$ver_minor" = "" ]; then
ver_minor=0
fi
if [ "$ver_micro" = "" ]; then
ver_micro=0
fi
if [ "$ver_patch" = "" ]; then
ver_patch=0
fi
}
is_headless_only() {
if [ "$ver_major" = "1" ]; then
if [ -f "$test_dir/lib/amd64/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/amd64/libsplashscreen.so" ] || [ -f "$test_dir/lib/i386/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/i386/libsplashscreen.so" ]; then
return 1
elif [ -f "$test_dir/lib/aarch64/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/aarch64/libsplashscreen.so" ] || [ -f "$test_dir/lib/aarch32/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/aarch32/libsplashscreen.so" ]; then
return 1
elif [ -f "$test_dir/lib/ppc64le/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/ppc64le/libsplashscreen.so" ] || [ -f "$test_dir/lib/ppc64/libsplashscreen.so" ] || [ -f "$test_dir/jre/lib/ppc64/libsplashscreen.so" ]; then
return 1
fi
elif [ -f "$test_dir/lib/libsplashscreen.so" ]; then
return 1
fi
return 0
}
read_db_entry() {
if [ -n "$INSTALL4J_NO_DB" ]; then
return 1
fi
if [ ! -f "$db_file" ]; then
return 1
fi
if [ ! -x "$java_exc" ]; then
return 1
fi
found=1
exec 7< $db_file
while read r_type r_dir r_ver_major r_ver_minor r_ver_micro r_ver_patch r_ver_vendor<&7; do
if [ "$r_type" = "JRE_VERSION" ]; then
if [ "$r_dir" = "$test_dir" ]; then
ver_major=$r_ver_major
ver_minor=$r_ver_minor
ver_micro=$r_ver_micro
ver_patch=$r_ver_patch
fill_version_numbers
fi
elif [ "$r_type" = "JRE_INFO" ]; then
if [ "$r_dir" = "$test_dir" ]; then
is_64bit=$r_ver_micro
if [ "W$r_ver_minor" = "W$modification_date" ] && [ "W$is_64bit" != "W" ]; then
found=0
break
fi
fi
fi
r_ver_micro=""
done
exec 7<&-
return $found
}
create_db_entry() {
tested_jvm=true
version_output=`"$bin_dir/java" $1 -version 2>&1`
is_gcj=`expr "$version_output" : '.*gcj'`
is_64bit=`expr "$version_output" : '.*64-Bit\|.*amd64'`
if [ "$is_gcj" = "0" ]; then
java_version=`expr "$version_output" : '.*"\(.*\)".*'`
ver_major=`expr "$java_version" : '\([0-9][0-9]*\).*'`
ver_minor=`expr "$java_version" : '[0-9][0-9]*\.\([0-9][0-9]*\).*'`
ver_micro=`expr "$java_version" : '[0-9][0-9]*\.[0-9][0-9]*\.\([0-9][0-9]*\).*'`
ver_patch=`expr "$java_version" : '[0-9][0-9]*\.[0-9][0-9]*\.[0-9][0-9]*[\._]\([0-9][0-9]*\).*'`
fi
fill_version_numbers
if [ -n "$INSTALL4J_NO_DB" ]; then
return
fi
db_new_file=${db_file}_new
if [ -f "$db_file" ]; then
awk '$2 != "'"$test_dir"'" {print $0}' $db_file > $db_new_file
cp "$db_new_file" "$db_file"
rm "$db_new_file" 2> /dev/null
fi
dir_escaped=`echo "$test_dir" | sed -e 's/ /\\\\ /g'`
echo "JRE_VERSION $dir_escaped $ver_major $ver_minor $ver_micro $ver_patch" >> $db_file
echo "JRE_INFO $dir_escaped 1 $modification_date $is_64bit" >> $db_file
chmod g+w $db_file
}
check_date_output() {
if [ -n "$date_output" -a $date_output -eq $date_output 2> /dev/null ]; then
modification_date=$date_output
fi
}
test_jvm() {
tested_jvm=na
test_dir=$1
bin_dir=$test_dir/bin
java_exc=$bin_dir/java
if [ -z "$test_dir" ] || [ ! -d "$bin_dir" ] || [ ! -f "$java_exc" ] || [ ! -x "$java_exc" ]; then
return
fi
modification_date=0
date_output=`date -r "$java_exc" "+%s" 2>/dev/null`
if [ $? -eq 0 ]; then
check_date_output
fi
if [ $modification_date -eq 0 ]; then
stat_path=`command -v stat 2> /dev/null`
if [ "$?" -ne "0" ] || [ "W$stat_path" = "W" ]; then
stat_path=`which stat 2> /dev/null`
if [ "$?" -ne "0" ]; then
stat_path=""
fi
fi
if [ -f "$stat_path" ]; then
date_output=`stat -f "%m" "$java_exc" 2>/dev/null`
if [ $? -eq 0 ]; then
check_date_output
fi
if [ $modification_date -eq 0 ]; then
date_output=`stat -c "%Y" "$java_exc" 2>/dev/null`
if [ $? -eq 0 ]; then
check_date_output
fi
fi
fi
fi
tested_jvm=false
read_db_entry || create_db_entry $2
if [ $full_awt_required = "true" ] && is_headless_only; then
return;
fi
if [ "$ver_major" = "" ]; then
return;
fi
if [ "$ver_major" -lt "1" ]; then
return;
elif [ "$ver_major" -eq "1" ]; then
if [ "$ver_minor" -lt "8" ]; then
return;
fi
fi
if [ "$ver_major" = "" ]; then
return;
fi
if [ "$ver_major" -gt "11" ]; then
return;
fi
app_java_home=$test_dir
}
add_class_path() {
if [ -n "$1" ] && [ `expr "$1" : '.*\*'` -eq "0" ]; then
local_classpath="$local_classpath${local_classpath:+:}${1}${2}"
fi
}
read_vmoptions() {
vmoptions_file=`eval echo "$1" 2>/dev/null`
if [ ! -r "$vmoptions_file" ]; then
vmoptions_file="$prg_dir/$vmoptions_file"
fi
if [ -r "$vmoptions_file" ] && [ -f "$vmoptions_file" ]; then
exec 8< "$vmoptions_file"
while read cur_option<&8; do
is_comment=`expr "W$cur_option" : 'W *#.*'`
if [ "$is_comment" = "0" ]; then
vmo_classpath=`expr "W$cur_option" : 'W *-classpath \(.*\)'`
vmo_classpath_a=`expr "W$cur_option" : 'W *-classpath/a \(.*\)'`
vmo_classpath_p=`expr "W$cur_option" : 'W *-classpath/p \(.*\)'`
vmo_include=`expr "W$cur_option" : 'W *-include-options \(.*\)'`
if [ ! "W$vmo_include" = "W" ]; then
if [ "W$vmo_include_1" = "W" ]; then
vmo_include_1="$vmo_include"
elif [ "W$vmo_include_2" = "W" ]; then
vmo_include_2="$vmo_include"
elif [ "W$vmo_include_3" = "W" ]; then
vmo_include_3="$vmo_include"
fi
fi
if [ ! "$vmo_classpath" = "" ]; then
local_classpath="$i4j_classpath:$vmo_classpath"
elif [ ! "$vmo_classpath_a" = "" ]; then
local_classpath="${local_classpath}:${vmo_classpath_a}"
elif [ ! "$vmo_classpath_p" = "" ]; then
local_classpath="${vmo_classpath_p}:${local_classpath}"
elif [ "W$vmo_include" = "W" ]; then
needs_quotes=`expr "W$cur_option" : 'W.* .*'`
if [ "$needs_quotes" = "0" ]; then
vmoptions_val="$vmoptions_val $cur_option"
else
if [ "W$vmov_1" = "W" ]; then
vmov_1="$cur_option"
elif [ "W$vmov_2" = "W" ]; then
vmov_2="$cur_option"
elif [ "W$vmov_3" = "W" ]; then
vmov_3="$cur_option"
elif [ "W$vmov_4" = "W" ]; then
vmov_4="$cur_option"
elif [ "W$vmov_5" = "W" ]; then
vmov_5="$cur_option"
fi
fi
fi
fi
done
exec 8<&-
if [ ! "W$vmo_include_1" = "W" ]; then
vmo_include="$vmo_include_1"
unset vmo_include_1
read_vmoptions "$vmo_include"
fi
if [ ! "W$vmo_include_2" = "W" ]; then
vmo_include="$vmo_include_2"
unset vmo_include_2
read_vmoptions "$vmo_include"
fi
if [ ! "W$vmo_include_3" = "W" ]; then
vmo_include="$vmo_include_3"
unset vmo_include_3
read_vmoptions "$vmo_include"
fi
fi
}
unpack_file() {
if [ -f "$1" ]; then
jar_file=`echo "$1" | awk '{ print substr($0,1,length($0)-5) }'`
bin/unpack200 -r "$1" "$jar_file" > /dev/null 2>&1
if [ $? -ne 0 ]; then
echo "Error unpacking jar files. The architecture or bitness (32/64)"
echo "of the bundled JVM might not match your machine."
returnCode=1
cd "$old_pwd"
if [ ! "W $INSTALL4J_KEEP_TEMP" = "W yes" ]; then
rm -R -f "$sfx_dir_name"
fi
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
SNAP(Sentinel Application Platform)是欧洲空间局(ESA)开发的一款软件,主要用于处理和分析遥感数据,特别是来自其Sentinel卫星系列的数据。SNAP提供了多种工具和功能,支持用户进行数据的预处理、可视化、分析和产品生成。 软件来自于欧空局官网,本资源提供的是Sentinel Toolbox V10.0版本,包含了esa-snap_sentinel_linux-10.0.0.sh,Linux操作系统可用,可用于哨兵2号数据的处理。
资源推荐
资源详情
资源评论
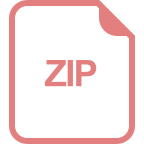
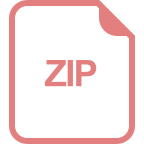
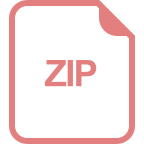
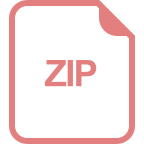
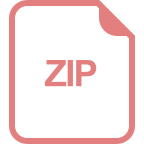
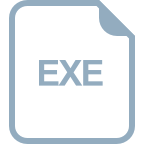
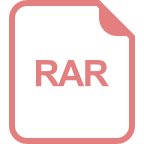
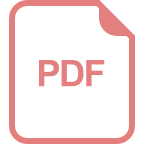
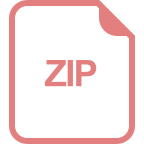
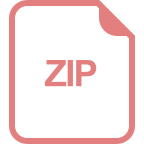
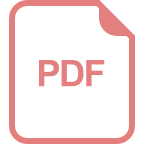
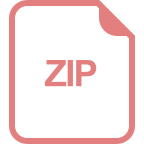
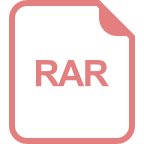
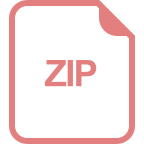
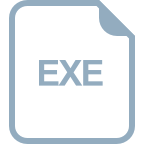
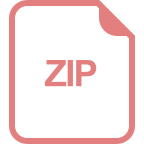
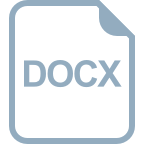
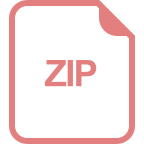
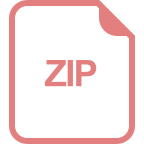
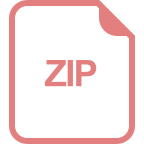
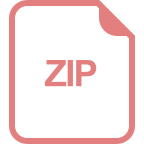
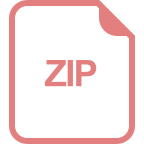
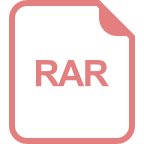
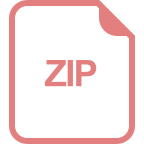
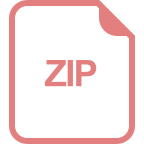
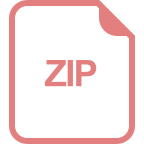
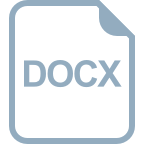
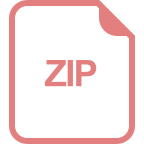
收起资源包目录


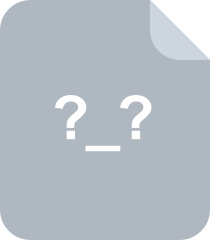
共 1 条
- 1
资源评论
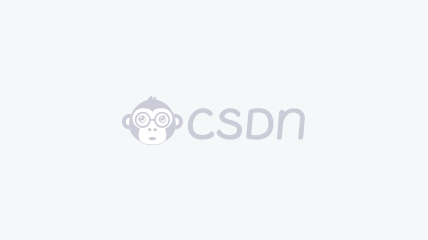


浩瀚地学
- 粉丝: 5834
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

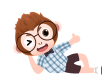
最新资源
- 获取CPU的序列号的Python脚本
- 4354图446546546546546
- 邮箱管理技巧:减少垃圾邮件的9项实用措施
- 三汇SMG 系列D 型模拟网关用户手册,用于三汇SMG系列网关配置
- Siemens Automation Framework V1.2
- 单个IO口检测多个按键
- 汇川EASY32x固件6.3.0.0
- 高分成品毕业设计《基于SSM(Spring、Spring MVC、MyBatis)+MySQL开发个人财务管理系统》+源码+论文+说明文档+数据库
- 高分成品毕业设计《基于SSM(Spring、Spring MVC、MyBatis)+MySQL开发B2C电子商务平台》+源码+论文+说明文档+数据库
- HKJC_3in1_TR_PROD_L3.0R1An_Build10229.apk
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


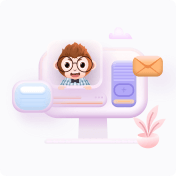
安全验证
文档复制为VIP权益,开通VIP直接复制
