springboot集成Mybatis和quartz
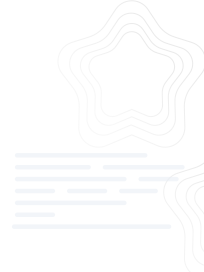

在IT行业中,Spring Boot是一个非常流行的微服务框架,它简化了Spring应用的初始搭建以及开发过程。Spring Boot的核心理念是“约定优于配置”,这使得开发者能够快速构建可运行的应用程序。而MyBatis是一个优秀的持久层框架,它支持定制化SQL、存储过程以及高级映射。Quartz则是Java领域广泛使用的任务调度库,可以用来执行定时任务。 当我们谈论"springboot集成Mybatis和quartz"时,意味着我们要将这三个组件结合在一起,创建一个具有数据库操作和定时任务功能的项目。下面我们将详细讨论如何实现这个集成。 集成Spring Boot和MyBatis。Spring Boot提供了对MyBatis的内置支持,我们只需要添加相应的依赖。在`pom.xml`文件中,我们需要引入Spring Boot的starter-web和starter-data-jpa,以及MyBatis的启动依赖: ```xml <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.4</version> </dependency> <!-- 数据库驱动,例如MySQL --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> </dependencies> ``` 接下来,配置MyBatis。在`application.yml`或`application.properties`中,设置数据源、MyBatis的配置文件路径和Mapper扫描路径: ```yaml spring: datasource: url: jdbc:mysql://localhost:3306/mydb?useUnicode=true&characterEncoding=utf8 username: root password: password driver-class-name: com.mysql.jdbc.Driver mybatis: mapper-locations: classpath:mapper/*.xml configuration: map-underscore-to-camel-case: true ``` 创建MyBatis的Mapper接口和对应的XML文件,定义SQL语句和结果映射。例如,创建一个UserMapper接口和对应的UserMapper.xml文件。 然后,配置Quartz。在`pom.xml`中添加Quartz的依赖: ```xml <dependency> <groupId>org.quartz-scheduler</groupId> <artifactId>quartz</artifactId> <version>2.3.2</version> </dependency> ``` 在`application.yml`或`application.properties`中配置Quartz的基本设置: ```yaml quartz: job-store-type: memory scheduler-instance-name: MyScheduler ``` 创建一个Job类,实现`org.quartz.Job`接口,并在需要执行的任务中注入所需的Service或Repository。例如,创建一个`HelloJob`类: ```java import org.quartz.Job; import org.quartz.JobExecutionContext; import org.quartz.JobExecutionException; public class HelloJob implements Job { @Override public void execute(JobExecutionContext context) throws JobExecutionException { System.out.println("Hello, Quartz!"); } } ``` 配置Job的触发规则,这可以通过Spring的`@Configuration`和`@Bean`注解完成。例如: ```java import org.quartz.*; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class QuartzConfig { @Bean public JobDetail helloJobDetail() { return JobBuilder.newJob(HelloJob.class) .withIdentity("helloJob", "group1") .build(); } @Bean public CronTrigger helloJobTrigger(JobDetail helloJobDetail) { return TriggerBuilder.newTrigger() .forJob(helloJobDetail) .withIdentity("helloJobTrigger", "group1") .withSchedule(CronScheduleBuilder.cronSchedule("0/5 * * * * ?")) .build(); } @Bean public SchedulerFactoryBean schedulerFactoryBean(JobDetail helloJobDetail, CronTrigger helloJobTrigger) { SchedulerFactoryBean factory = new SchedulerFactoryBean(); factory.setJobDetails(helloJobDetail); factory.setTriggers(helloJobTrigger); return factory; } } ``` 以上配置完成后,Spring Boot应用将启动Quartz定时器,按照指定的Cron表达式定期执行`HelloJob`。同时,MyBatis将与数据库进行交互,处理业务逻辑。 这个"springboot集成Mybatis和quartz"的案例提供了一个基础的框架,开发者可以根据实际需求填充业务代码,实现特定的数据库操作和定时任务。通过这样的整合,我们可以构建出一个高效、可扩展且易于维护的系统。
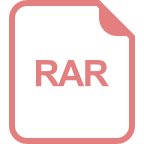
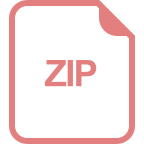
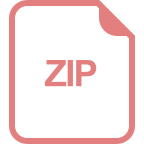
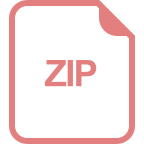
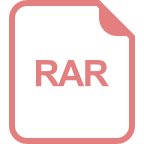
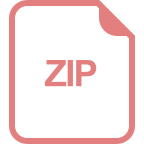
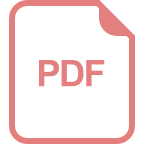
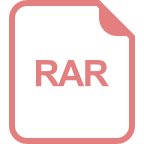
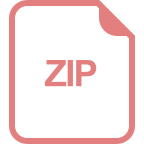
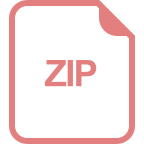
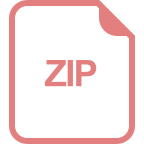
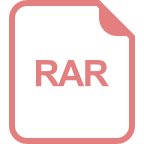
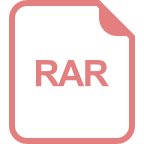
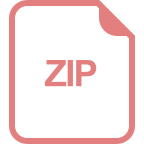
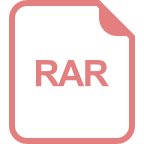
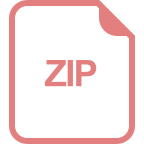
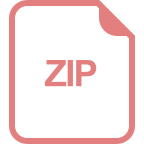
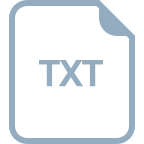
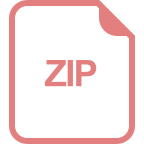
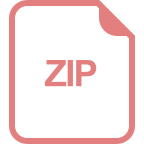

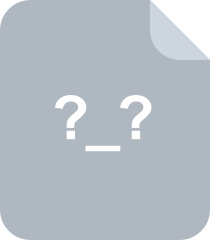
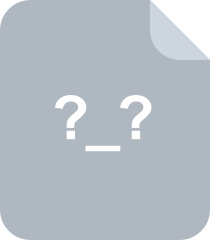
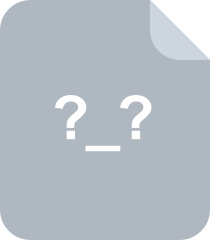
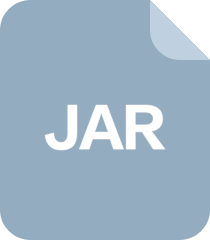
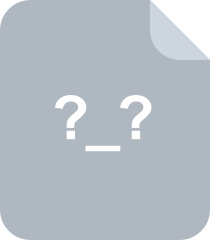
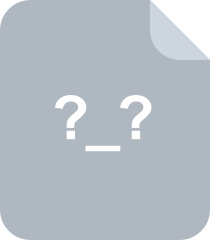
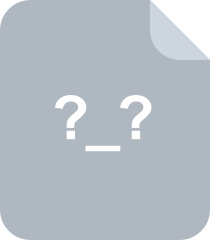
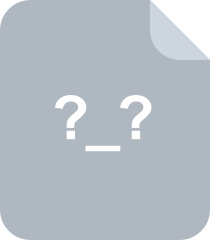
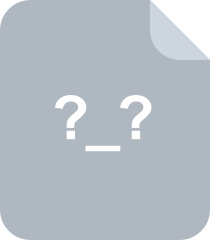
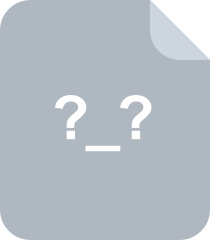
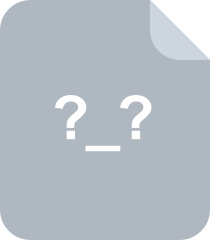
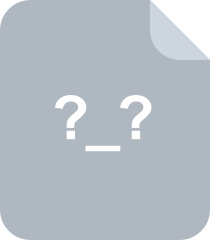
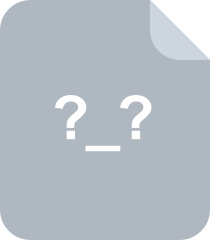
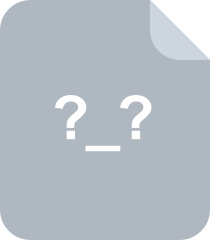
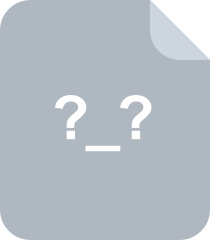
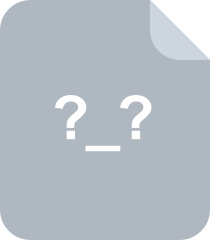
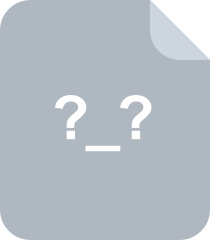
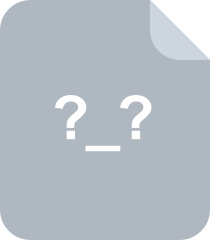
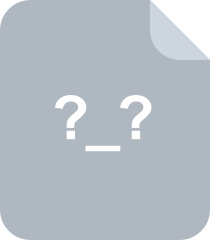
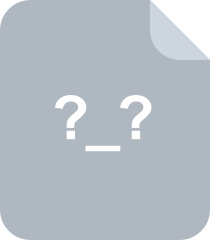
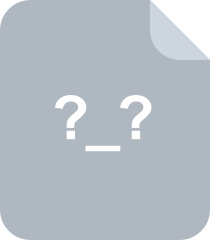
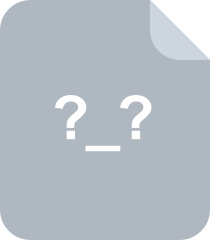
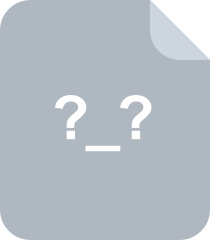
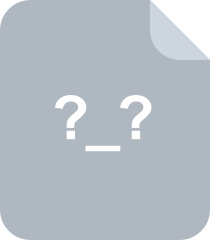
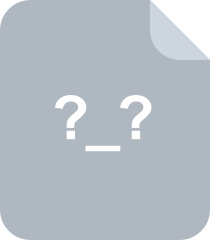
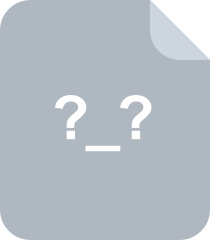
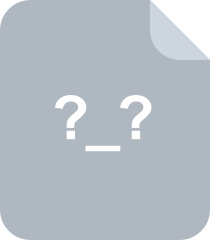
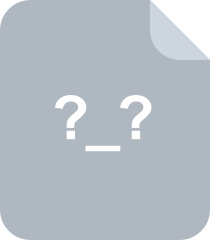
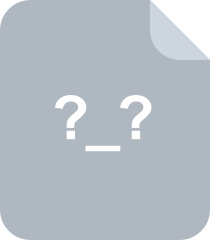
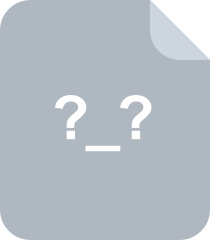
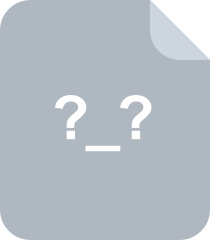
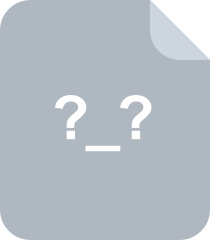
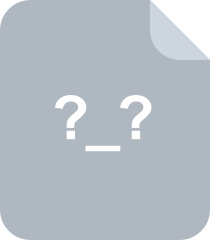
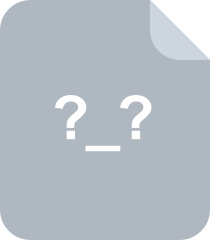
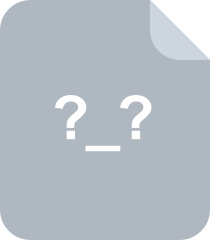
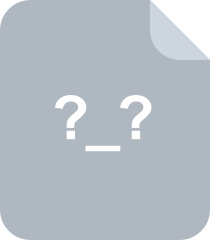
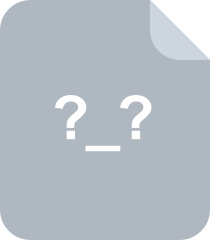
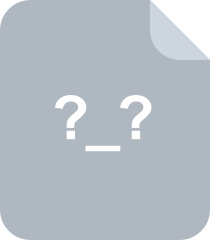
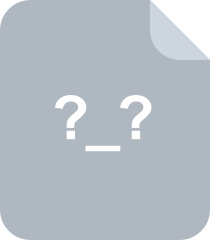
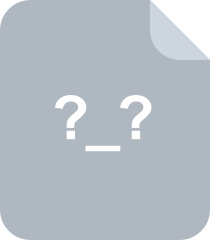
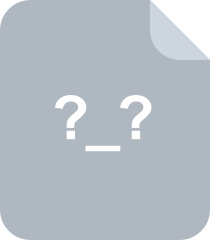
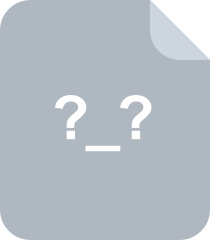
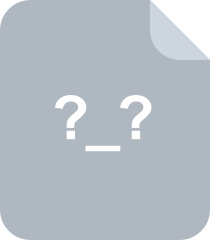
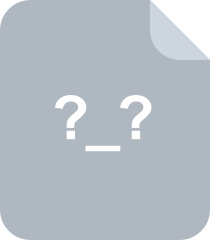
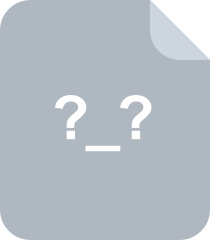
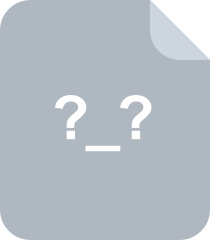
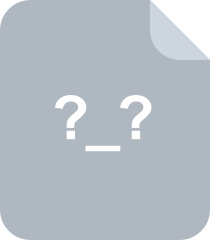
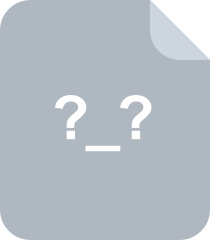
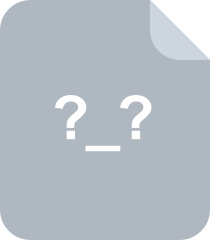
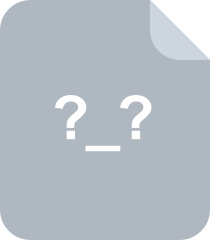
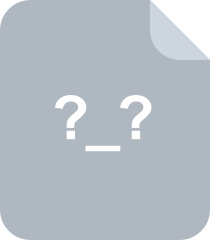
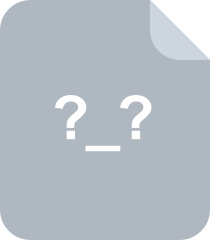
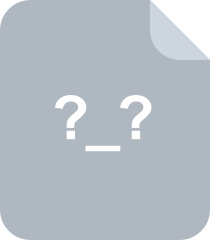
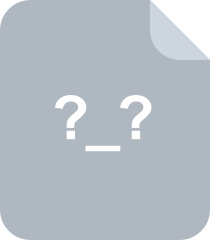
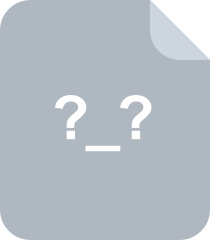
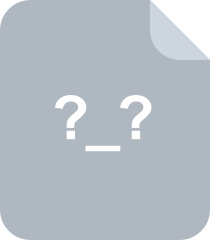
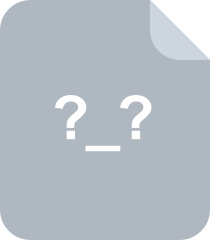
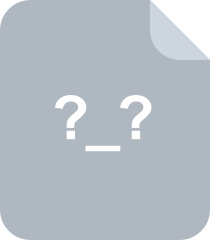
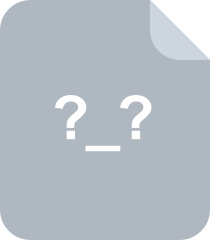
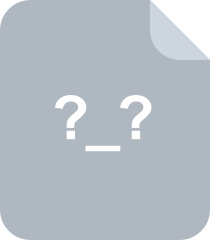
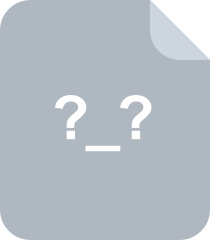
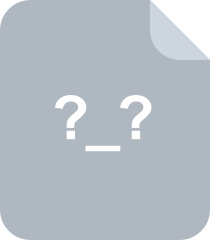
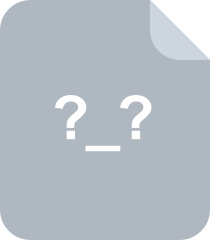
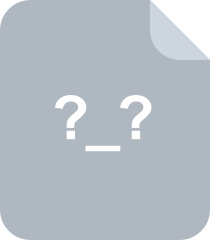
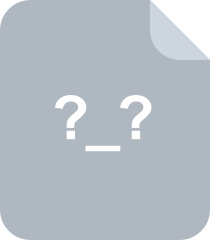
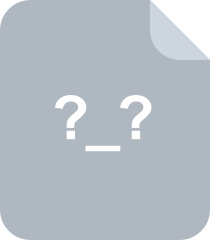
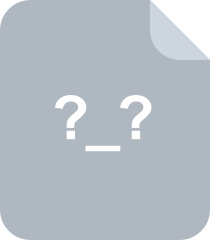
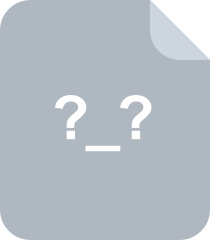
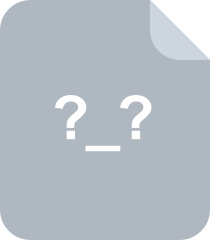
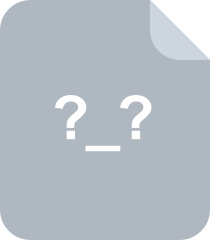
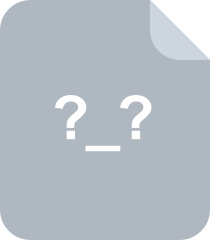
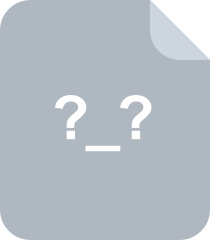
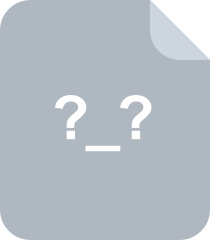
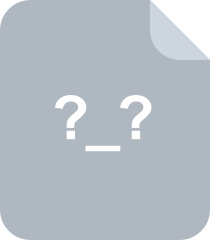
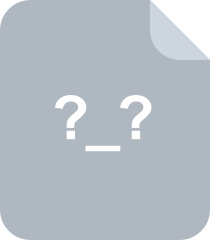
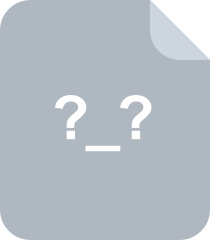
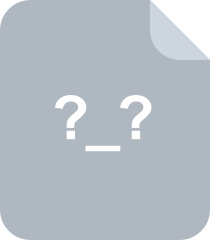
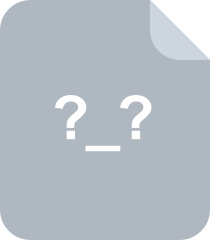
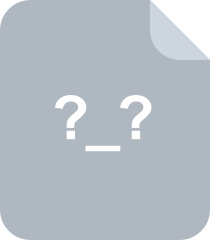
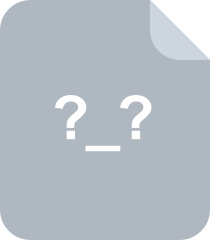
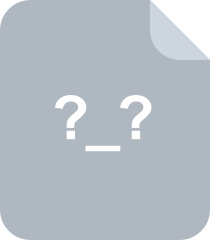
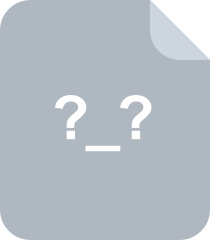
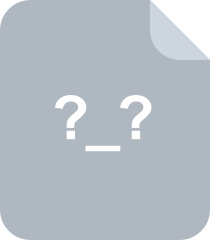
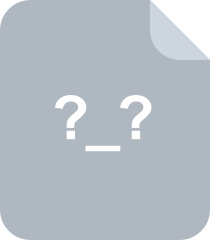
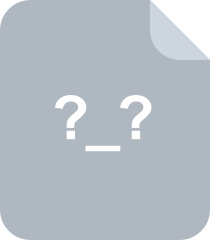
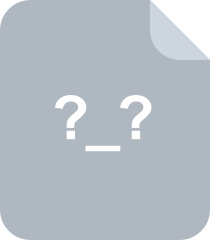
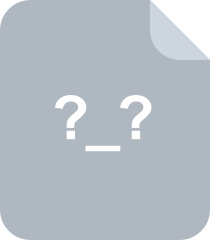
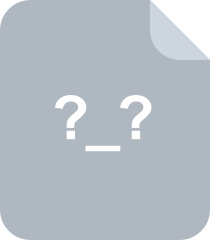
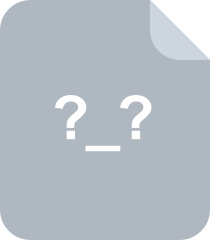
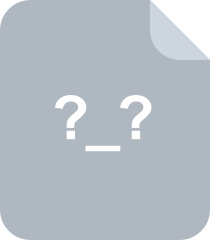
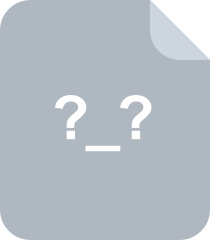
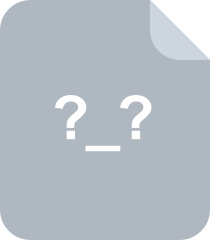
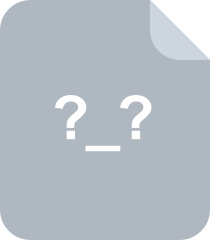
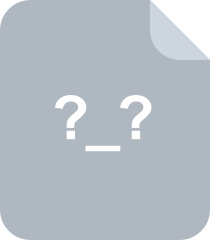
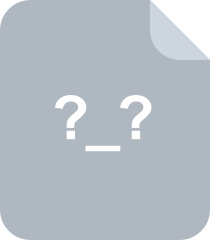
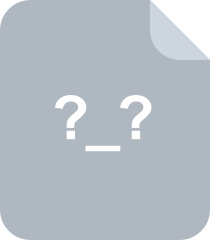
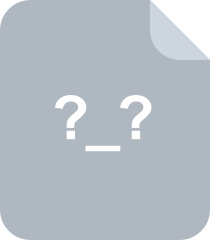
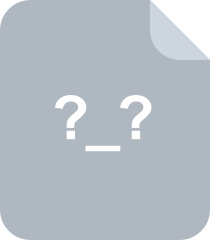
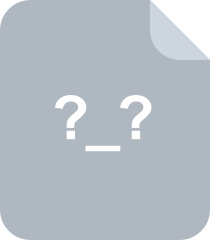
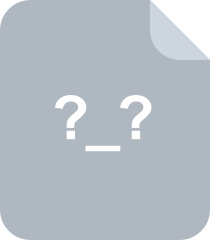
- 1
- 2
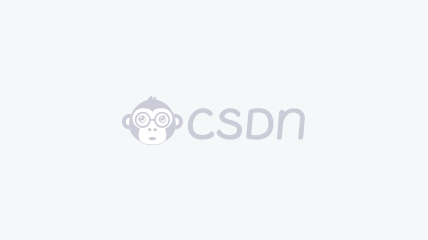

- 粉丝: 43
- 资源: 73
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

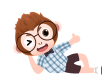
最新资源
- pdf24-creator-11.20.1-x64
- 4米长汽车密封条打孔机sw18可编辑全套技术资料100%好用.zip
- 2000H风量等离子设备(sw18可编辑+工程图+BOM)全套技术资料100%好用.zip
- BMC塑封定子清理机sw18可编辑全套技术资料100%好用.zip
- 编码器箱输送机sw18可编辑全套技术资料100%好用.zip
- 布条镭雕设备(sw17可编辑+工程图+bom)全套技术资料100%好用.zip
- FastReport VCL 6.8.6安装版.rar
- Java项目示例:学生信息系统设计与实现
- GNSS (2).zip
- 玻璃转盘检测机 CCD检测机sw18可编辑全套技术资料100%好用.zip
- 面向对象程序设计课程设计C++链表类应用实例分析与评定
- 插针检测摆盘机 折料摆盘自动机sw16可编辑全套技术资料100%好用.zip
- 冒泡排序算法的基础原理与多语言实现详解
- 多种编程语言下IP地址与整数相互转换的实现方法
- 操作系统资源管理之银行家算法详解与C语言实现
- 车门密封条内水切定长裁断机(sw18可编辑+cad+bom)全套技术资料100%好用.zip

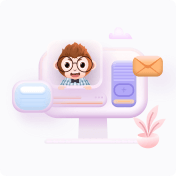
