springboot + mybatis
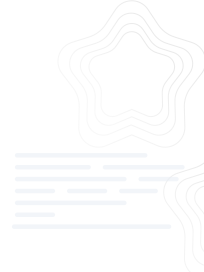

SpringBoot和MyBatis是Java开发中常用的两个框架,它们结合使用可以构建高效、简洁的Web应用程序。SpringBoot简化了Spring应用的初始搭建以及开发过程,而MyBatis则是一个优秀的持久层框架,它支持定制化SQL、存储过程以及高级映射。下面将详细介绍这两个框架的集成与使用。 SpringBoot的核心思想是“约定优于配置”,它默认配置了许多常见的功能,如嵌入式Servlet容器、自动配置、Starter依赖等,大大减少了开发者在项目初始化时的工作量。在SpringBoot中引入MyBatis,我们通常会使用`spring-boot-starter-mybatis`这个Starter依赖,它包含了MyBatis和Spring的整合模块,使得MyBatis可以无缝地融入到SpringBoot的环境中。 集成步骤如下: 1. 添加依赖:在`pom.xml`文件中添加SpringBoot对MyBatis的支持,包括`spring-boot-starter-data-jpa`(虽然这里不是JPA,但SpringBoot默认包含这个依赖)和`mybatis-spring-boot-starter`。 ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.4</version> </dependency> ``` 2. 配置数据库连接:在`application.properties`或`application.yml`中配置数据源的相关信息,例如数据库驱动、URL、用户名和密码。 ```properties spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.jdbc.Driver ``` 3. 创建Mapper接口和XML文件:MyBatis中的Mapper接口用于定义SQL操作,每个方法对应一个SQL语句。在`src/main/resources/mapper`目录下创建对应的XML文件,编写SQL语句。 4. 配置Mapper:在SpringBoot的主配置类上添加`@MapperScan`注解,指定扫描Mapper接口的包名。 ```java @SpringBootApplication @MapperScan("com.example.demo.mapper") // 替换为你的Mapper接口包名 public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } } ``` 5. 实现业务逻辑:创建Service层,注入Mapper接口,通过调用接口的方法实现业务逻辑。 示例项目中的`keep-hello`可能表示一个简单的HelloWorld示例,这通常会包含一个用户实体(User)、对应的Mapper接口(UserMapper)、Mapper的XML文件(UserMapper.xml)以及一个展示问候信息的Controller。在`UserMapper`中定义查询用户的方法,例如: ```java public interface UserMapper { User selectUserById(Long id); } ``` 在`UserMapper.xml`中编写对应的SQL: ```xml <select id="selectUserById" resultType="com.example.demo.entity.User"> SELECT * FROM user WHERE id = #{id} </select> ``` 然后在Controller中注入`UserMapper`并调用该方法: ```java @RestController public class HelloController { @Autowired private UserMapper userMapper; @GetMapping("/hello") public String hello() { User user = userMapper.selectUserById(1L); return "Hello, " + user.getName(); } } ``` 通过这种方式,SpringBoot和MyBatis可以轻松地构建出一个完整的CRUD应用。MyBatis允许开发者自由编写SQL,提供良好的灵活性,而SpringBoot的自动化配置和 Starter 依赖让项目的搭建变得简单快捷。这个示例项目是初学者学习SpringBoot和MyBatis整合的绝佳起点,也是开发人员快速搭建新项目的模板。
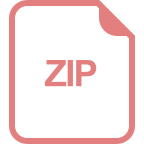
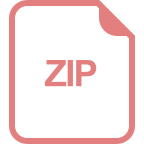
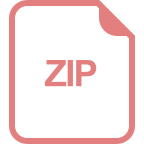
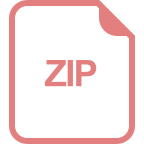
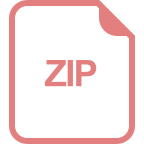
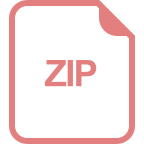
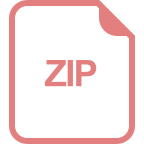
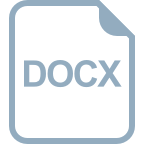
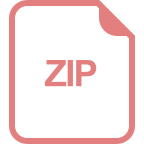
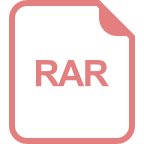
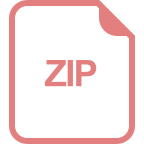
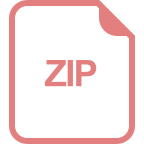
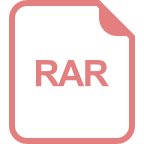
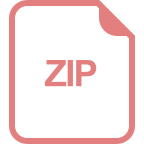
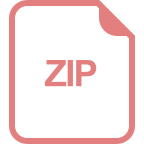
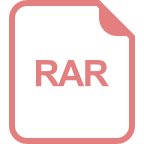
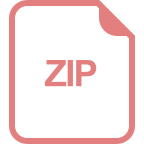
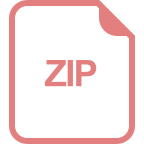


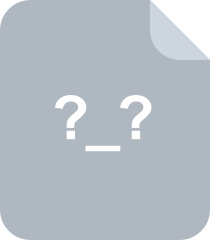



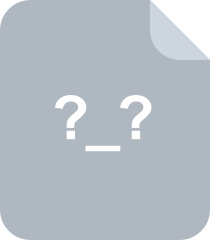

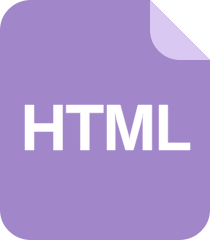
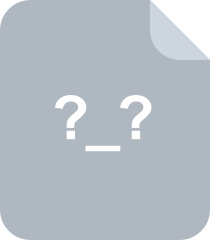

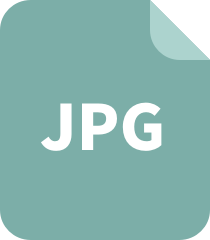
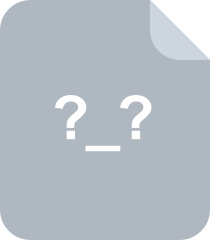





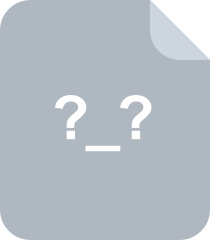
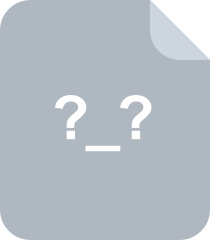
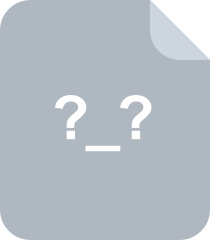

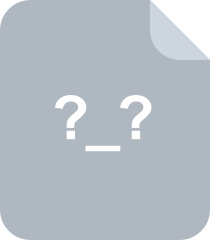


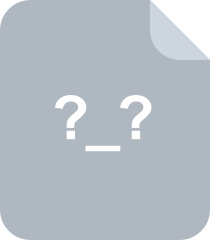




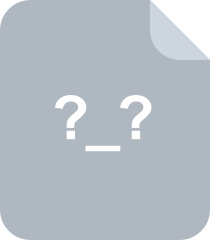



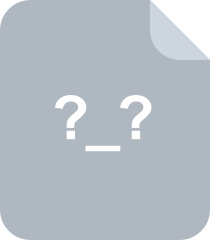

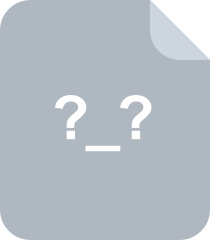
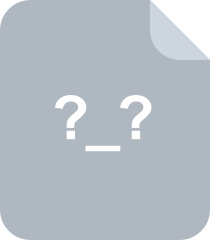
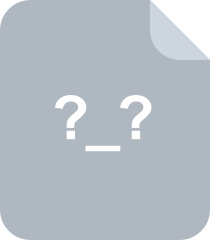

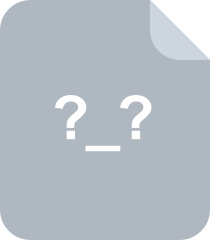

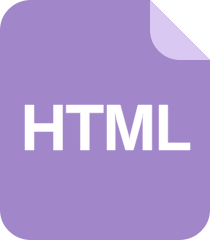
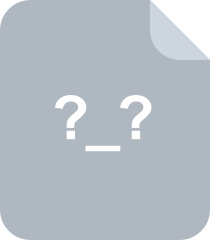

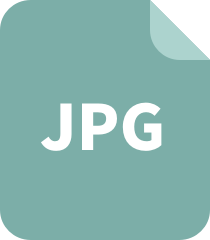
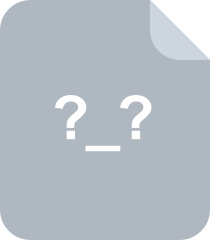
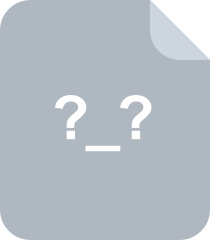
- 1
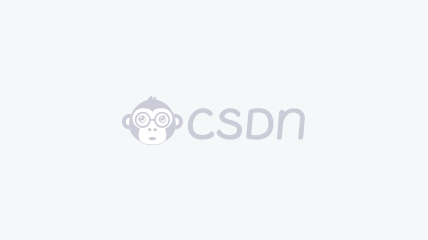

- 粉丝: 1
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

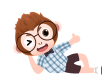
最新资源

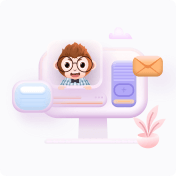
