WinPcap ARP欺骗简单源码
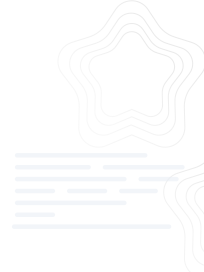

WinPcap ARP欺骗简单源码是一个关于网络安全领域的实践示例,主要涉及了两个核心技术:WinPcap和ARP协议。WinPcap是Windows平台下的一款开源网络数据包捕获和网络分析库,它允许程序员深入到网络层,获取并处理网络流量。而ARP(Address Resolution Protocol)是TCP/IP协议栈中的一个重要组成部分,用于将IP地址转换为物理(MAC)地址,这是网络通信的基础。 在ARP欺骗中,攻击者通过发送虚假的ARP响应,误导网络设备将特定IP地址的流量路由到错误的MAC地址,从而实现中间人攻击。这种攻击可能导致数据泄露、会话劫持甚至整个网络瘫痪。这个简单的源码示例旨在演示如何使用WinPcap库来创建和发送伪造的ARP包,帮助学习者理解ARP欺骗的工作原理。 WinPcap.cpp文件是源代码的主要部分,其中包含了使用WinPcap API进行数据包捕获和注入的函数。开发者可能在这里定义了打开网络接口、设置过滤器、读取数据包以及发送自定义ARP包的函数。例如,`pcap_open_live()`函数用于打开一个网络接口,`pcap_compile()`和`pcap_setfilter()`用于设定过滤规则,只捕获或发送特定类型的包。此外,`pcap_sendpacket()`函数则用于发送构造好的ARP欺骗包。 WinPcapTest.exe是编译后的可执行程序,可以直接运行来测试源码的功能。它可能包含了一些测试用例,模拟不同类型的ARP欺骗场景。 WinPcapTest.vcxproj.*文件是一系列Visual Studio项目文件,包括解决方案文件(.sln)、项目文件(.vcxproj)、项目过滤文件(.vcxproj.filters)以及用户特定的项目设置文件(.vcxproj.user)。这些文件用于在Visual Studio环境中管理和构建项目,确保源码能够正确编译和链接到WinPcap库。 通过这个源码示例,学习者可以深入了解WinPcap库的使用,包括如何打开网络接口、设置捕获参数、解析和构建数据包,以及如何利用ARP协议的特性进行欺骗。同时,这也是一个很好的起点,帮助开发者理解网络攻击机制,并为构建更复杂的网络安全防护系统打下基础。在实际应用中,应严格遵守法律法规,仅用于合法的网络安全研究和防御目的。
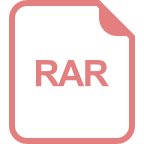
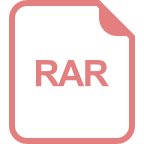
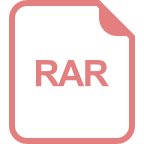
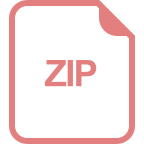
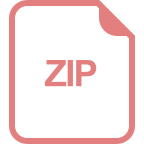
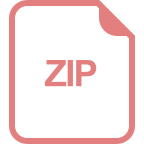
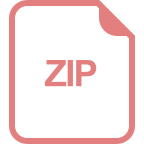
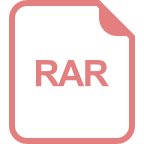
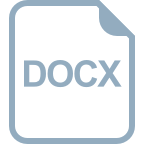
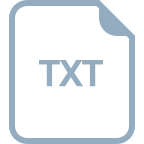
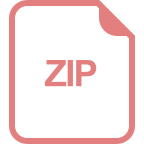
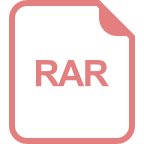
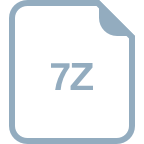
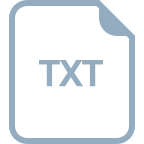
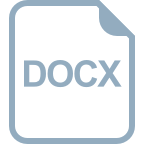
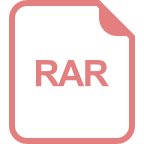
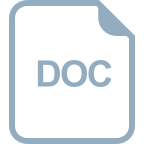
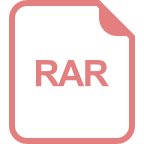
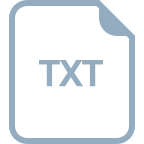
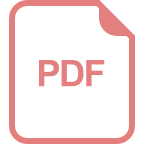
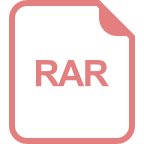
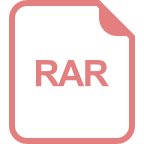

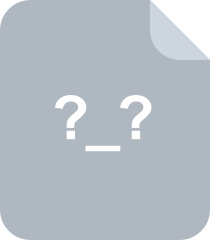
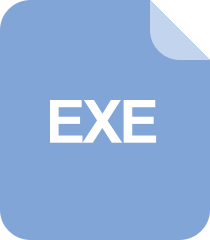
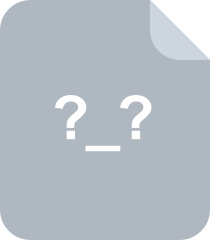
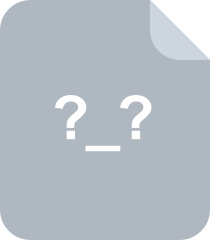
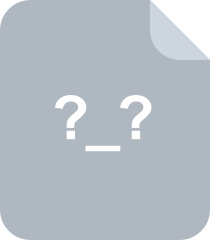
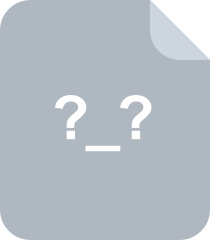
- 1
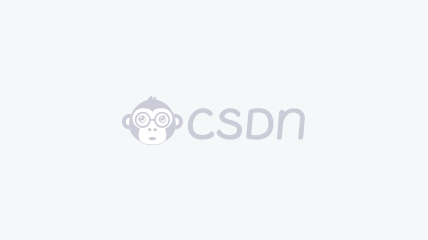

- 粉丝: 33
- 资源: 9
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

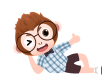
最新资源
- 9.30 SWKJ 男头7张+女头2张.zip
- 1734967319584.png
- TG-2024-12-23-194506126.mp4
- 计算机导论之软件工程-公司员工内部培训
- 网络唤醒++安装包,可以直接安装到所有openwrt设备
- Example10_1.java
- MATLAB空数组(empty array)的深刻理解与运用
- 群接龙脚本autojs总结and精美ui.zip
- jhaghjgfhgsdhghsdh
- 2023-04-06-项目笔记 - 第三百五十七阶段 - 4.4.2.355全局变量的作用域-355 -2025.12.24
- 通过apache+aliyuncli管理阿里云子用户
- 快递公司送货策略.doc
- 2023-04-06-项目笔记 - 第三百五十七阶段 - 4.4.2.355全局变量的作用域-355 -2025.12.24
- ISO15118-1-2013 Road vehicles - Vehicle to grid communication interface General information
- Android+课程设计不是梦+音乐播放器
- 期末上机考试第三题.py

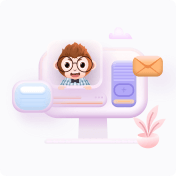
