/*
Copyright 2013 Daniel Wirtz <dcode@dcode.io>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
/**
* @license protobuf.js (c) 2013 Daniel Wirtz <dcode@dcode.io>
* Released under the Apache License, Version 2.0
* see: https://github.com/dcodeIO/protobuf.js for details
*/
(function(global, factory) {
/* AMD */ if (typeof define === 'function' && define["amd"])
define(["bytebuffer"], factory);
/* CommonJS */ else if (typeof require === "function" && typeof module === "object" && module && module["exports"])
module["exports"] = factory(require("bytebuffer"), true);
/* Global */ else
(global["dcodeIO"] = global["dcodeIO"] || {})["ProtoBuf"] = factory(global["dcodeIO"]["ByteBuffer"]);
})(this, function(ByteBuffer, isCommonJS) {
"use strict";
/**
* The ProtoBuf namespace.
* @exports ProtoBuf
* @namespace
* @expose
*/
var ProtoBuf = {};
/**
* @type {!function(new: ByteBuffer, ...[*])}
* @expose
*/
ProtoBuf.ByteBuffer = ByteBuffer;
/**
* @type {?function(new: Long, ...[*])}
* @expose
*/
ProtoBuf.Long = ByteBuffer.Long || null;
/**
* ProtoBuf.js version.
* @type {string}
* @const
* @expose
*/
ProtoBuf.VERSION = "5.0.1";
/**
* Wire types.
* @type {Object.<string,number>}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES = {};
/**
* Varint wire type.
* @type {number}
* @expose
*/
ProtoBuf.WIRE_TYPES.VARINT = 0;
/**
* Fixed 64 bits wire type.
* @type {number}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES.BITS64 = 1;
/**
* Length delimited wire type.
* @type {number}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES.LDELIM = 2;
/**
* Start group wire type.
* @type {number}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES.STARTGROUP = 3;
/**
* End group wire type.
* @type {number}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES.ENDGROUP = 4;
/**
* Fixed 32 bits wire type.
* @type {number}
* @const
* @expose
*/
ProtoBuf.WIRE_TYPES.BITS32 = 5;
/**
* Packable wire types.
* @type {!Array.<number>}
* @const
* @expose
*/
ProtoBuf.PACKABLE_WIRE_TYPES = [
ProtoBuf.WIRE_TYPES.VARINT,
ProtoBuf.WIRE_TYPES.BITS64,
ProtoBuf.WIRE_TYPES.BITS32
];
/**
* Types.
* @dict
* @type {!Object.<string,{name: string, wireType: number, defaultValue: *}>}
* @const
* @expose
*/
ProtoBuf.TYPES = {
// According to the protobuf spec.
"int32": {
name: "int32",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: 0
},
"uint32": {
name: "uint32",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: 0
},
"sint32": {
name: "sint32",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: 0
},
"int64": {
name: "int64",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: ProtoBuf.Long ? ProtoBuf.Long.ZERO : undefined
},
"uint64": {
name: "uint64",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: ProtoBuf.Long ? ProtoBuf.Long.UZERO : undefined
},
"sint64": {
name: "sint64",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: ProtoBuf.Long ? ProtoBuf.Long.ZERO : undefined
},
"bool": {
name: "bool",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: false
},
"double": {
name: "double",
wireType: ProtoBuf.WIRE_TYPES.BITS64,
defaultValue: 0
},
"string": {
name: "string",
wireType: ProtoBuf.WIRE_TYPES.LDELIM,
defaultValue: ""
},
"bytes": {
name: "bytes",
wireType: ProtoBuf.WIRE_TYPES.LDELIM,
defaultValue: null // overridden in the code, must be a unique instance
},
"fixed32": {
name: "fixed32",
wireType: ProtoBuf.WIRE_TYPES.BITS32,
defaultValue: 0
},
"sfixed32": {
name: "sfixed32",
wireType: ProtoBuf.WIRE_TYPES.BITS32,
defaultValue: 0
},
"fixed64": {
name: "fixed64",
wireType: ProtoBuf.WIRE_TYPES.BITS64,
defaultValue: ProtoBuf.Long ? ProtoBuf.Long.UZERO : undefined
},
"sfixed64": {
name: "sfixed64",
wireType: ProtoBuf.WIRE_TYPES.BITS64,
defaultValue: ProtoBuf.Long ? ProtoBuf.Long.ZERO : undefined
},
"float": {
name: "float",
wireType: ProtoBuf.WIRE_TYPES.BITS32,
defaultValue: 0
},
"enum": {
name: "enum",
wireType: ProtoBuf.WIRE_TYPES.VARINT,
defaultValue: 0
},
"message": {
name: "message",
wireType: ProtoBuf.WIRE_TYPES.LDELIM,
defaultValue: null
},
"group": {
name: "group",
wireType: ProtoBuf.WIRE_TYPES.STARTGROUP,
defaultValue: null
}
};
/**
* Valid map key types.
* @type {!Array.<!Object.<string,{name: string, wireType: number, defaultValue: *}>>}
* @const
* @expose
*/
ProtoBuf.MAP_KEY_TYPES = [
ProtoBuf.TYPES["int32"],
ProtoBuf.TYPES["sint32"],
ProtoBuf.TYPES["sfixed32"],
ProtoBuf.TYPES["uint32"],
ProtoBuf.TYPES["fixed32"],
ProtoBuf.TYPES["int64"],
ProtoBuf.TYPES["sint64"],
ProtoBuf.TYPES["sfixed64"],
ProtoBuf.TYPES["uint64"],
ProtoBuf.TYPES["fixed64"],
ProtoBuf.TYPES["bool"],
ProtoBuf.TYPES["string"],
ProtoBuf.TYPES["bytes"]
];
/**
* Minimum field id.
* @type {number}
* @const
* @expose
*/
ProtoBuf.ID_MIN = 1;
/**
* Maximum field id.
* @type {number}
* @const
* @expose
*/
ProtoBuf.ID_MAX = 0x1FFFFFFF;
/**
* If set to `true`, field names will be converted from underscore notation to camel case. Defaults to `false`.
* Must be set prior to parsing.
* @type {boolean}
* @expose
*/
ProtoBuf.convertFieldsToCamelCase = false;
/**
* By default, messages are populated with (setX, set_x) accessors for each field. This can be disabled by
* setting this to `false` prior to building messages.
* @type {boolean}
* @expose
*/
ProtoBuf.populateAccessors = true;
/**
* By default, messages are populated with default values if a field is not present on the wire. To disable
* this behavior, set this setting to `false`.
* @type {boolean}
* @expose
*/
ProtoBuf.populateDefaults = true;
/**
* @alias ProtoBuf.Util
* @expose
*/
ProtoBuf.Util = (function() {
"use strict";
/**
* ProtoBuf utilities.
* @exports ProtoBuf.Util
* @namespace
*/
var Util = {};
/**
* Flag if running in node or not.
* @type {boolean}
* @const
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
万豪.zip源码cocos creator项目源码下载万豪.zip源码cocos creator项目源码下载 1.上线产品适合个人学习技术做项目参考 2.开发脚本为javsScropt或者typeScript 3.上线产品适合小公司开发游戏项目参考
资源推荐
资源详情
资源评论
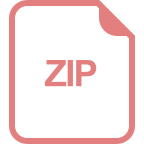
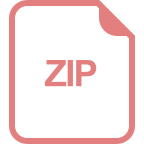
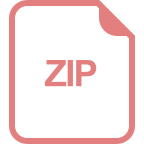
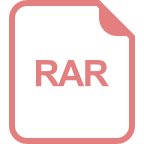
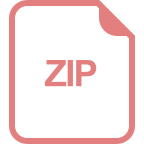
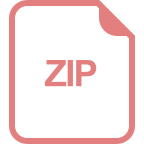
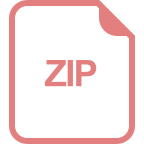
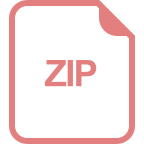
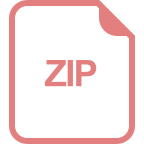
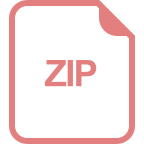
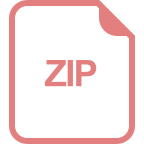
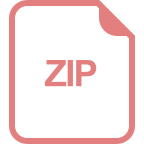
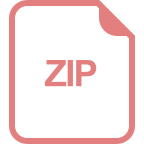
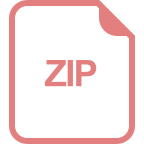
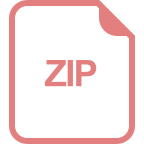
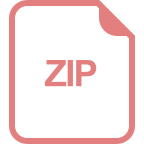
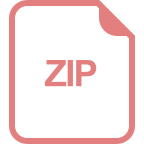
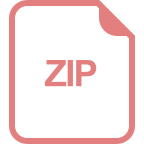
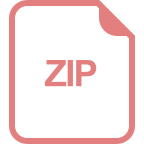
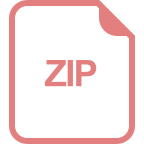
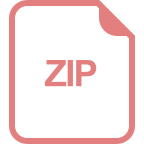
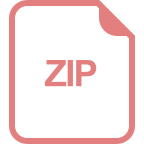
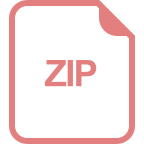
收起资源包目录

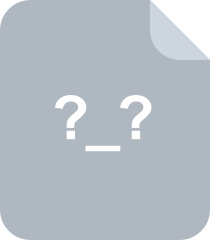
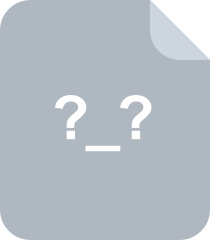
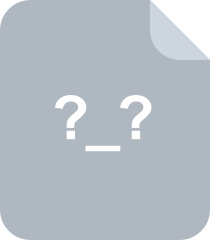
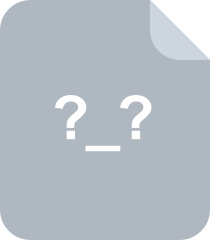
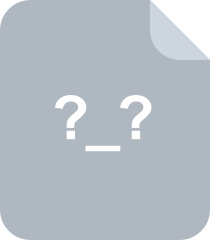
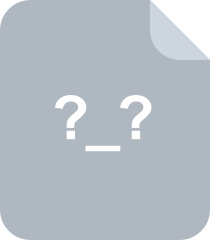
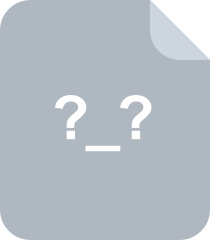
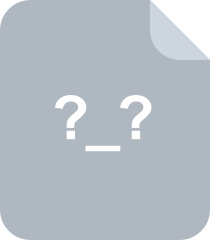
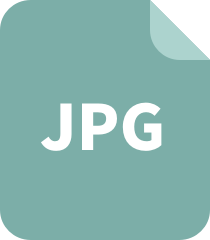
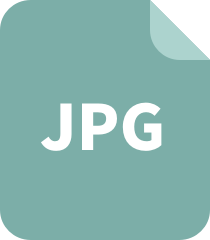
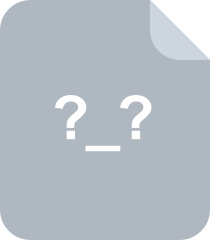
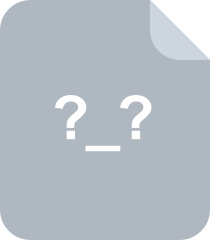
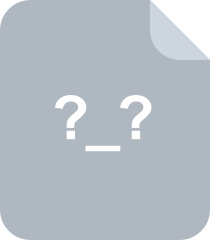
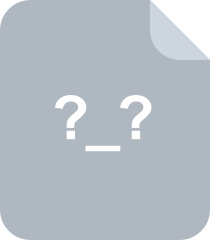
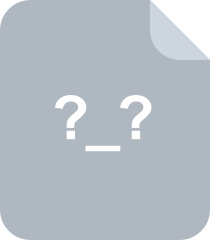
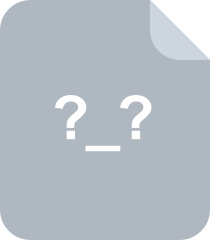
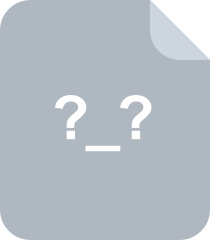
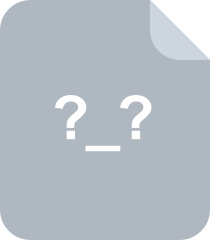
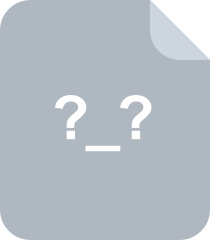
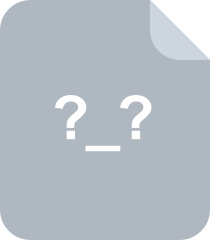
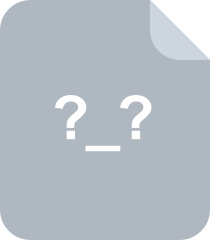
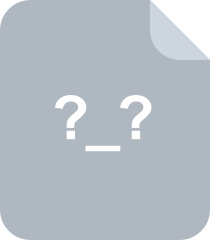
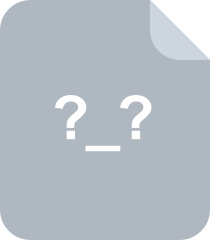
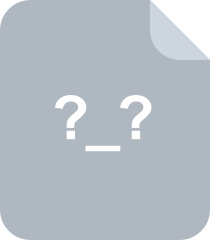
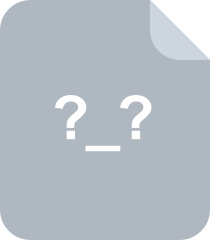
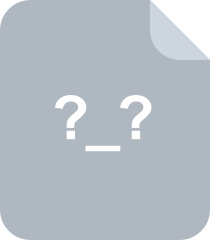
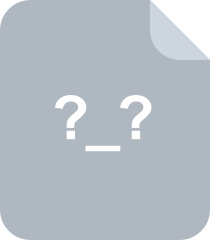
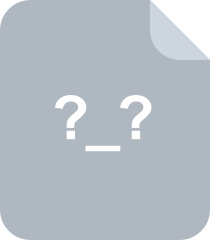
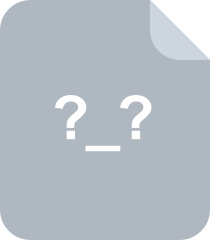
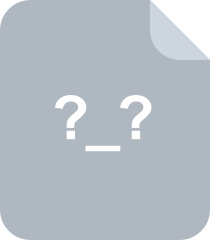
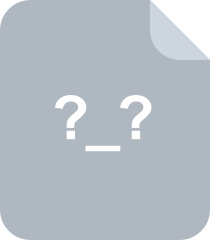
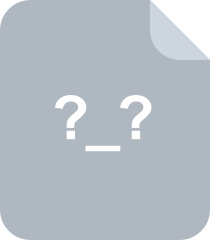
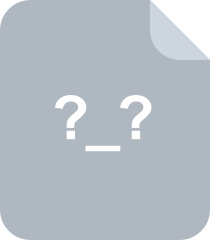
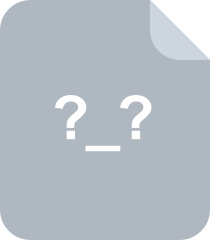
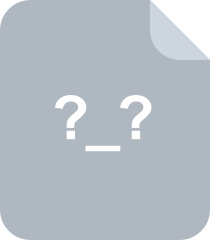
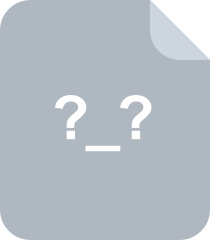
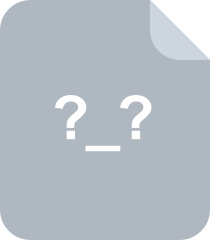
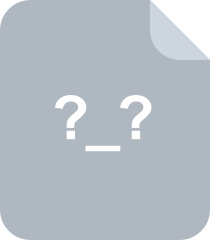
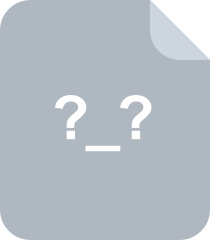
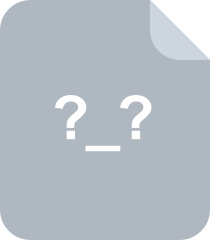
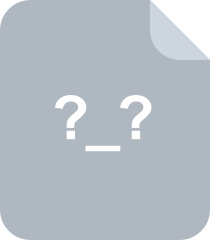
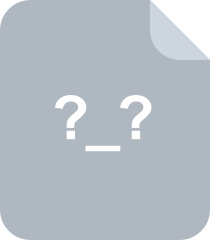
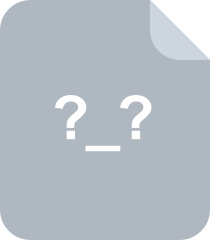
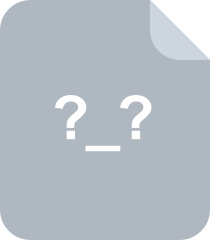
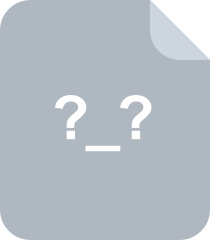
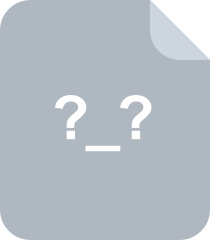
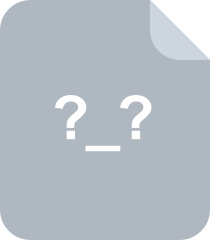
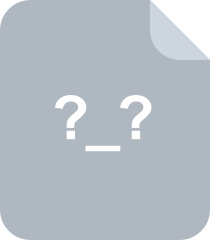
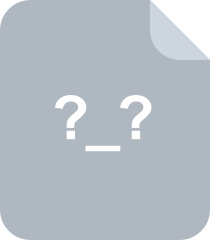
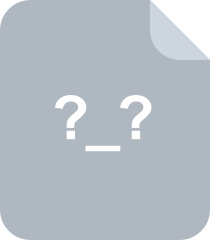
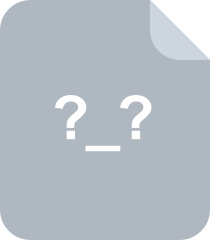
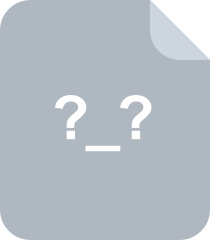
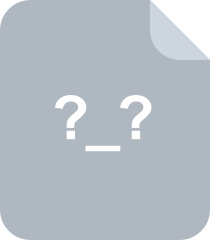
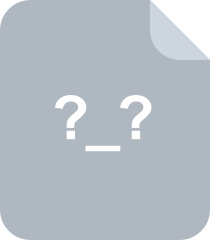
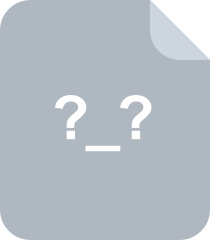
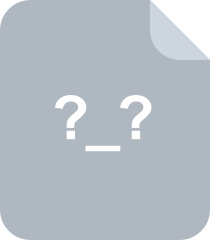
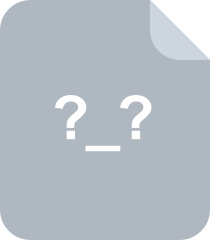
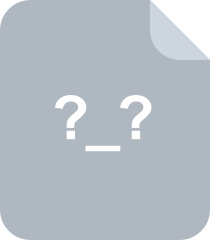
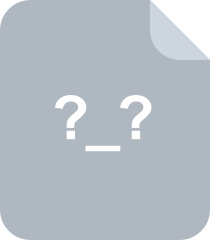
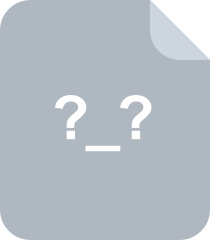
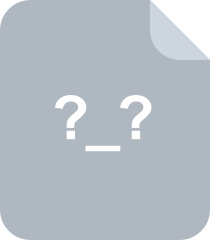
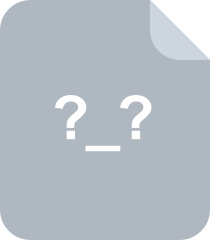
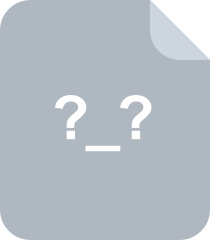
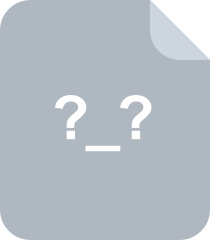
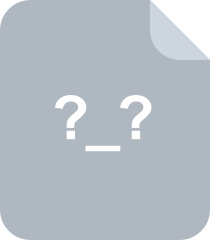
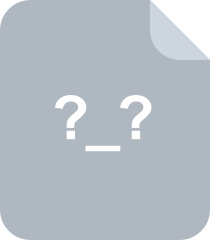
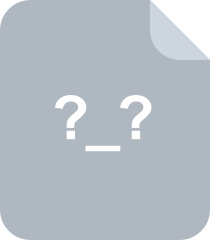
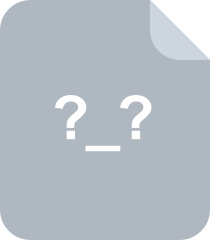
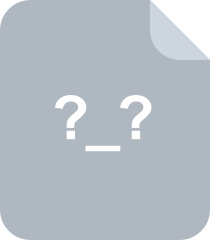
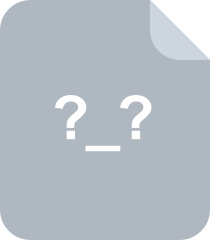
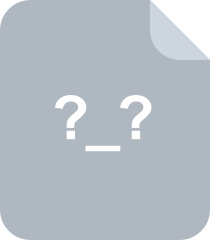
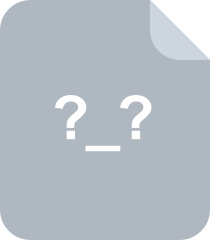
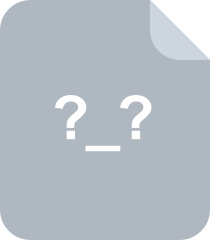
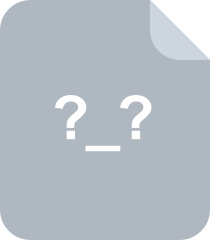
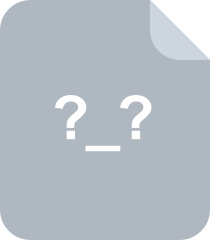
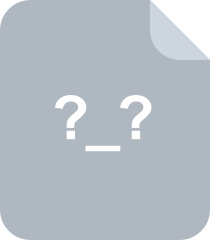
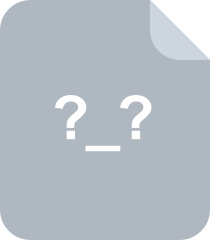
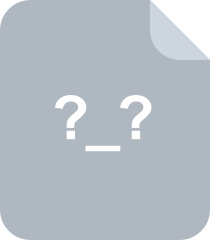
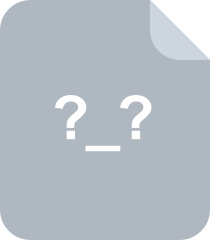
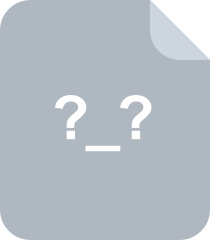
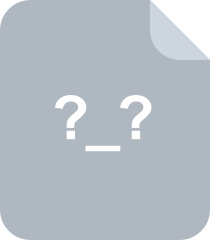
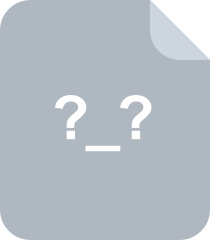
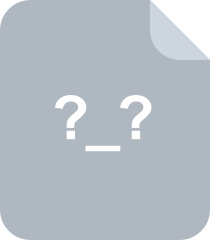
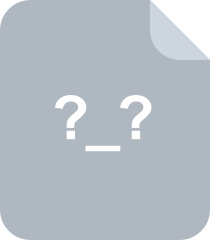
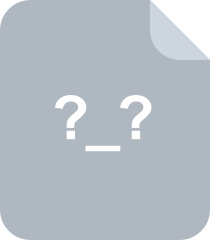
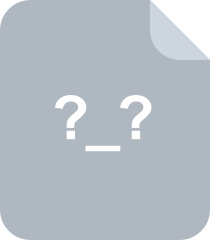
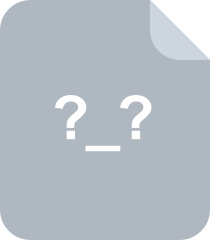
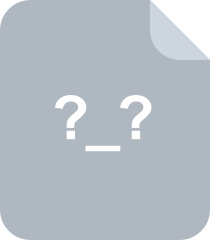
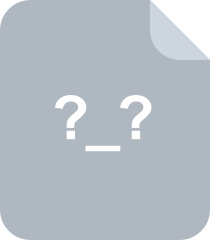
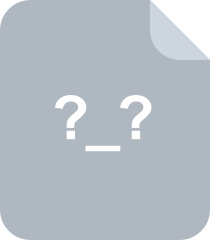
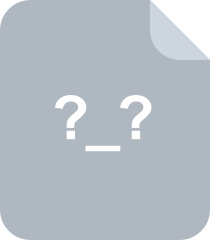
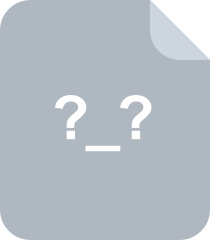
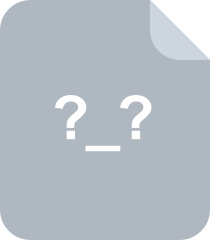
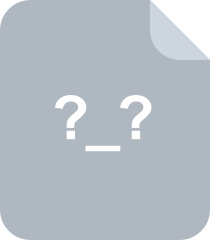
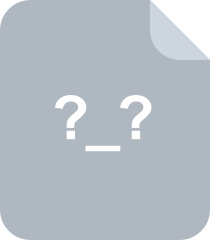
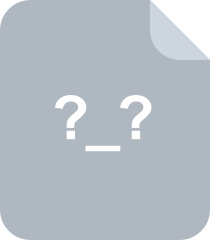
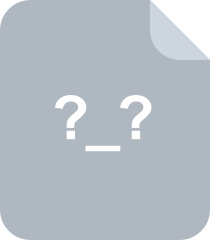
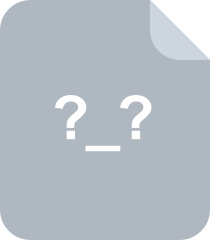
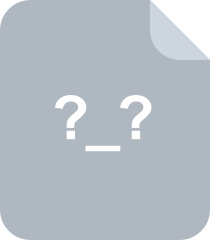
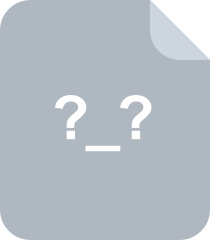
共 402 条
- 1
- 2
- 3
- 4
- 5
资源评论
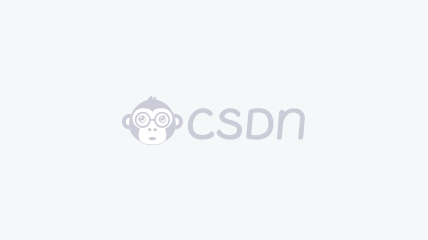
- vivien03182024-12-05发现一个宝藏资源,赶紧冲冲冲!支持大佬~

yxkfw
- 粉丝: 82
- 资源: 2万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

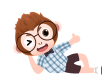
最新资源
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 注册程序源码-样式优化
- 【创新无忧】基于星雀优化算法NOA优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于雪融优化算法SAO优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于遗传算法GA优化极限学习机KELM实现故障诊断附matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


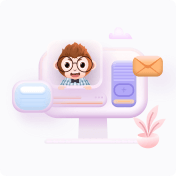
安全验证
文档复制为VIP权益,开通VIP直接复制
