const shareUtil = require('./share.js');
const GameTools = require('GameTools');
const GameManager = cc.Class({
extends: cc.Component,
statics: {
instance: null
},
properties: {
FrameSize: {
default: {}
},
scoreLabel: cc.Label,
score: 0,
exp: 0,
totalExp: 0,
tempExp: 0,
isGameOver: false,
CharacterPrefab: {
default: null,
type: cc.Prefab,
displayName: '主角'
},
JoyStickPrefab: {
default: null,
type: cc.Prefab,
displayName: '摇杆'
},
NormalEnemyPrefab: {
default: null,
type: cc.Prefab,
displayName: '普通敌人Prefab'
},
spawnNode: {
type: cc.Node,
default: null,
displayName: '出生节点'
},
spawn: {
default: [],
type: [cc.Vec2],
displayName: '出生点'
},
gameOverPanelPrefab: {
type: cc.Prefab,
default: null,
displayName: '游戏结束面板'
},
spawnNum: {
type: cc.Integer,
default: 5,
displayName: '敌人出生点数量'
},
character: cc.Node
},
onLoad () {
if (CC_WECHATGAME) {
this.totalExp = GameTools.getLocalData('exp') || 0;
}
this.FrameSize = cc.view.getFrameSize();
this.initShare();
this.gameStart();
},
update () {
if (cc.director.getScene().name === 'game') {
if (!this.isGameOver) {
if (this.score % 1000 === 200) {
this.resetSpawn();
this.spawnEnemy();
}
this.score += 2;
this.scoreLabel.string = this.score;
}
}
},
initShare() {
if (CC_WECHATGAME) {
cc.loader.loadRes('texture/share',(err, data) => {
shareUtil.shareMenu(wx, data);
});
}
},
spawnCharacter () {
this.character = cc.instantiate(this.CharacterPrefab);
this.character.parent = cc.find('Canvas');
this.character.setPosition(cc.v2(0, 0));
},
setJoystick () {
const joyStick = cc.instantiate(this.JoyStickPrefab);
joyStick.parent = cc.find('Canvas');
joyStick.setPosition(cc.v2(0, -200));
},
resetSpawn () {
this.spawn = [];
this.spawn.push(cc.v2(this.getMockPoint('x'), this.FrameSize.height / 2));
this.spawn.push(cc.v2(this.getMockPoint('x'), -this.FrameSize.height / 2));
this.spawn.push(cc.v2(this.FrameSize.width / 2, this.getMockPoint('y')));
this.spawn.push(cc.v2(this.FrameSize.width / 2, this.getMockPoint('y')));
this.spawn.push(cc.v2(-this.FrameSize.width / 2, this.getMockPoint('y')));
this.spawn.push(cc.v2(-this.FrameSize.width / 2, this.getMockPoint('y')));
},
spawnEnemy () {
this.spawn.map(p => {
const enemy = cc.instantiate(this.NormalEnemyPrefab);
enemy.parent = this.spawnNode;
enemy.setPosition(p);
});
},
getMockPoint (point = 'x') {
const span = point === 'x' ? this.FrameSize.width : this.FrameSize.height;
return span / 2 * Math.random() * Math.pow(-1, Math.floor(Math.random() * 10));
},
setGameState (state) {
switch (state) {
case 'GAMEOVER': this.gameOver(); break;
}
},
gameOver () {
this.isGameOver = true;
this.exp = Math.floor(this.score / 50);
cc.director.pause();
const gameOverPanel = cc.instantiate(this.gameOverPanelPrefab);
gameOverPanel.parent = cc.find('Canvas');
// this._gameOverPanel.setPosition(cc.v2(0, 0));
if (CC_WECHATGAME) {
const newExp = GameTools.addExp(this.exp - this.tempExp);
this.totalExp = newExp;
// submit score
wx.postMessage({
messageType: 2,
data: {
score: this.score
}
});
}
},
resgureGame() {
this.cleanGameOverPanel();
this.cleanEnemyies();
this.isGameOver = false;
cc.director.resume();
},
resetGame () {
// if (cc.director.isPaused && this.isGameOver) {
// }
this.score = 0;
this.tempExp = 0;
this.cleanScreenNode();
this.isGameOver = false;
this.gameStart();
cc.director.resume();
},
gameStart () {
this.spawnCharacter();
this.setJoystick();
},
cleanJoyStick() {
const joyStick = cc.find('Canvas/JoyStick');
joyStick.destroy();
},
cleanEnemyies() {
this.spawnNode.destroyAllChildren();
},
cleanGameOverPanel() {
const gamePanel = cc.find('Canvas/GameOver');
gamePanel.destroy();
},
cleanCharacter() {
this.character.destroy();
this.character = null;
},
cleanScreenNode () {
this.cleanGameOverPanel();
this.cleanJoyStick();
this.cleanEnemyies();
this.cleanCharacter();
}
});
if (!GameManager.instance) {
GameManager.instance = new GameManager();
}
module.exports = GameManager;
没有合适的资源?快使用搜索试试~ 我知道了~
逃亡大作战-creator.zip源码cocos creator项目源码下载
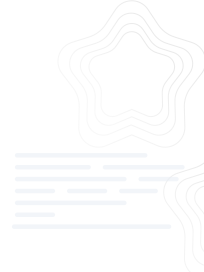
共199个文件
meta:104个
png:45个
js:22个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 12 浏览量
2022-03-18
09:43:00
上传
评论
收藏 2.75MB ZIP 举报
温馨提示
逃亡大作战-creator.zip源码cocos creator项目源码下载逃亡大作战-creator.zip源码cocos creator项目源码下载 1.上线产品适合个人学习技术做项目参考 2.开发脚本为javsScropt或者typeScript 3.上线产品适合小公司开发游戏项目参考
资源推荐
资源详情
资源评论
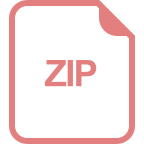
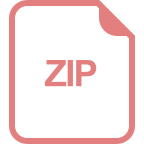
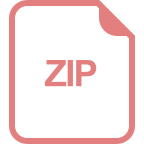
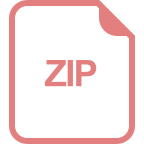
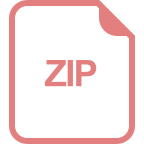
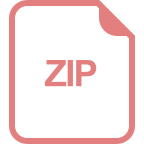
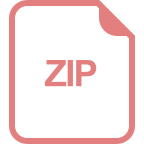
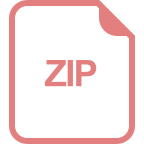
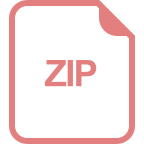
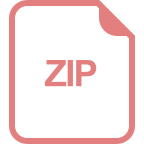
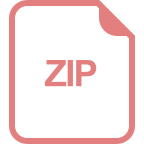
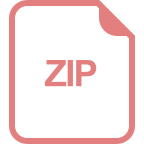
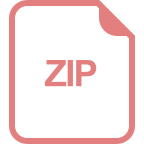
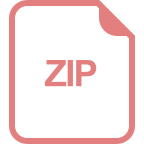
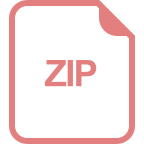
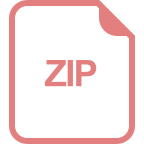
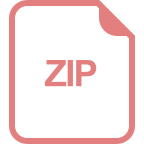
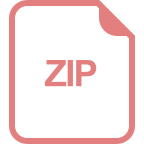
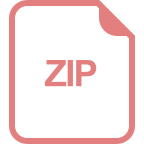
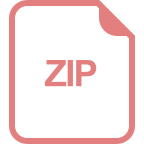
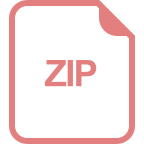
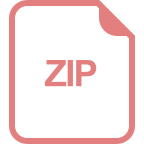
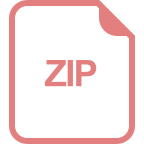
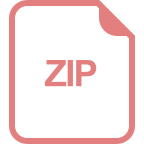
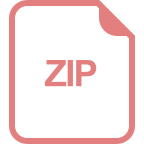
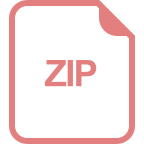
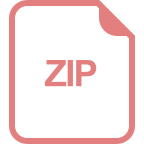
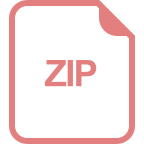
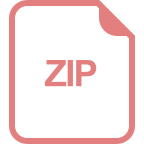
收起资源包目录

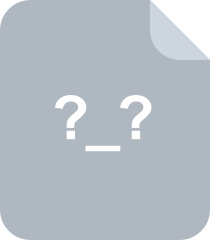
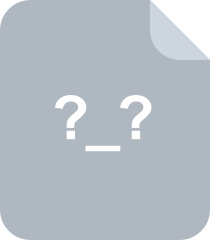
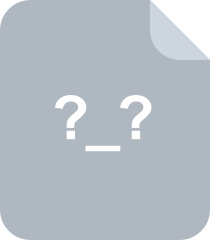
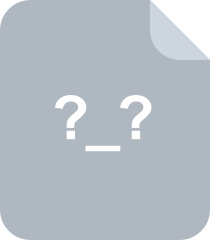
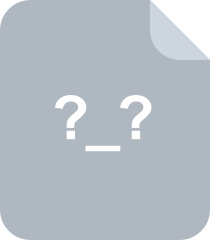
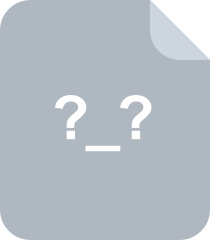
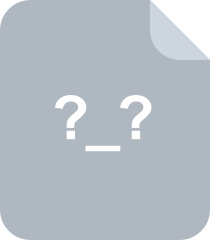
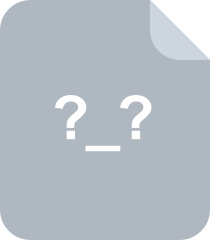
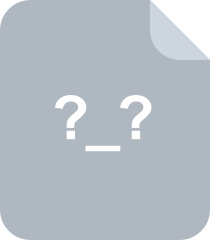
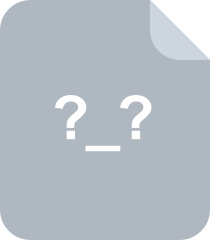
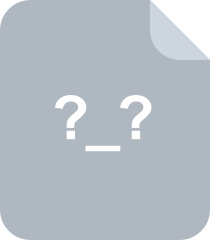
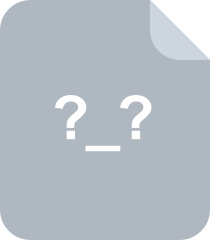
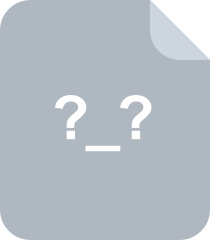
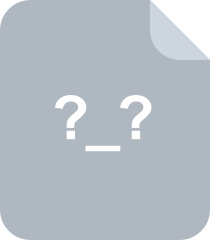
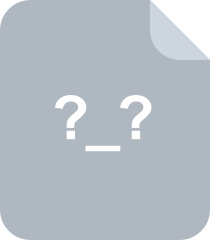
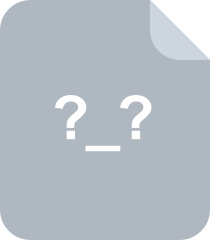
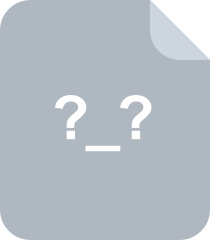
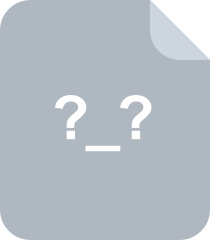
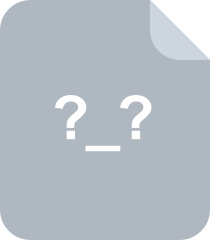
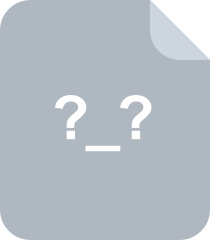
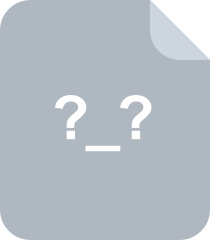
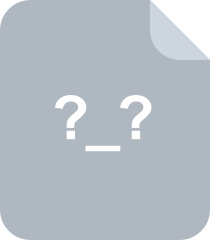
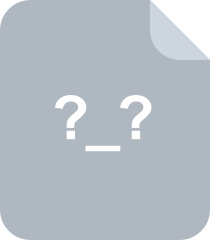
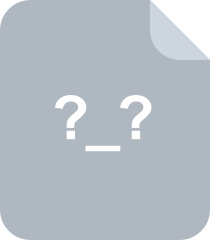
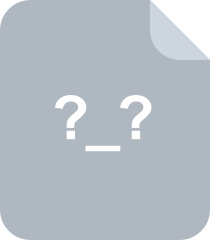
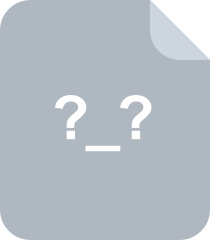
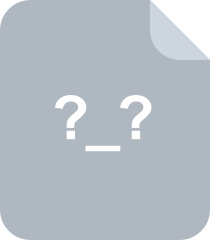
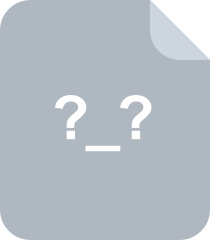
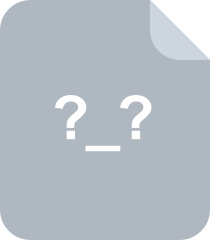
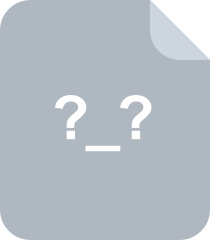
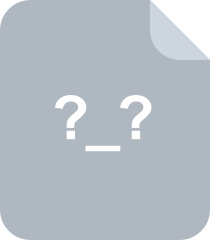
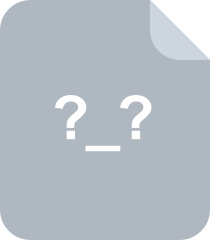
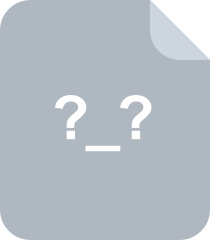
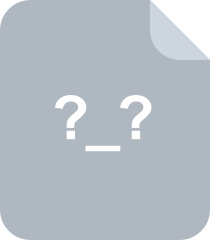
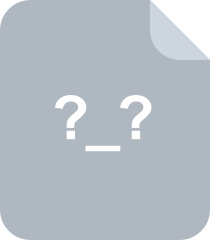
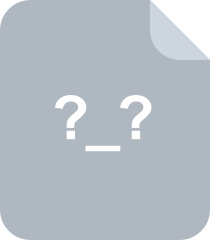
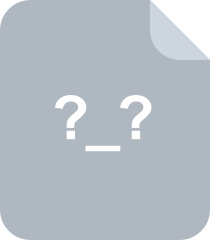
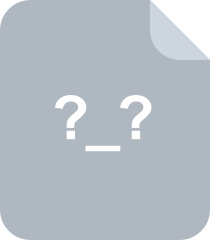
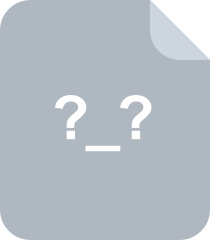
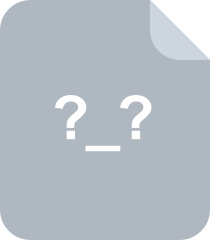
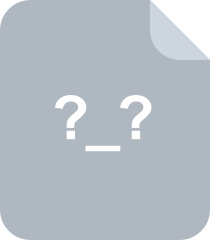
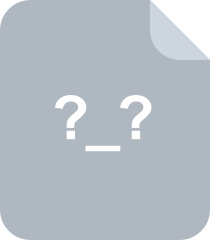
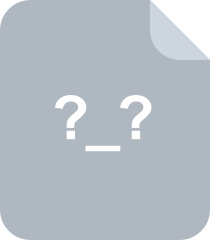
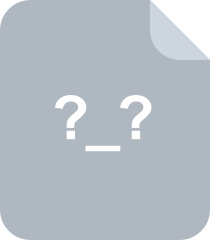
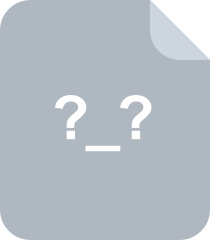
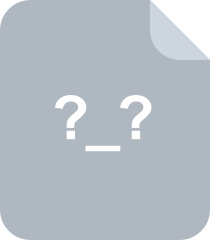
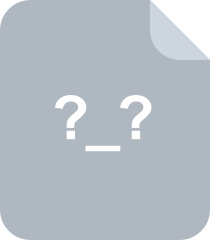
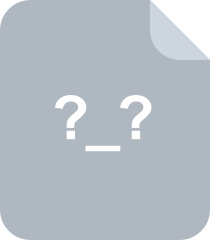
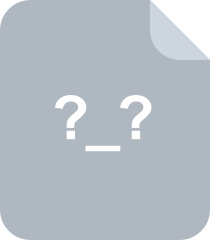
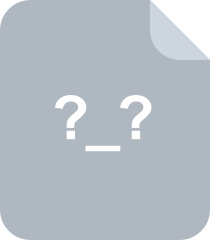
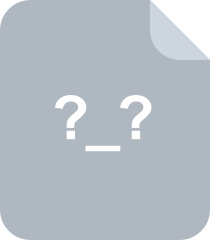
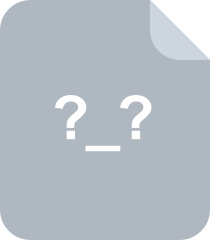
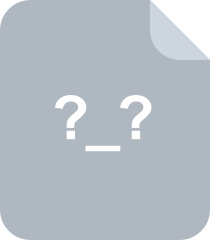
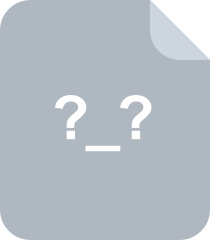
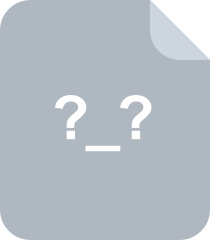
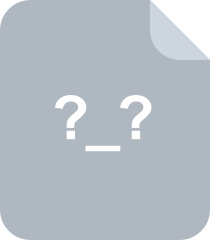
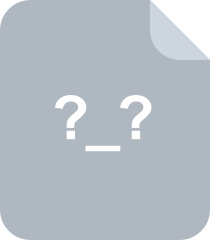
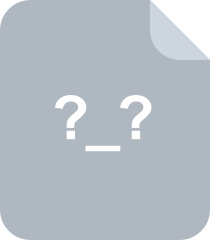
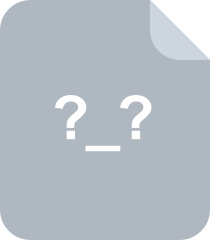
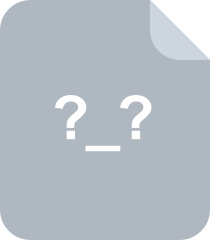
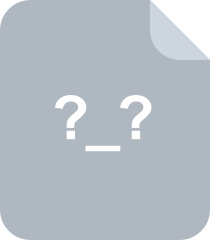
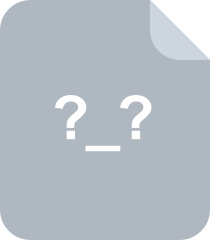
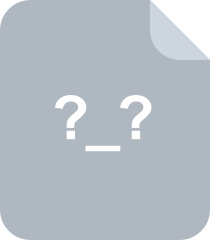
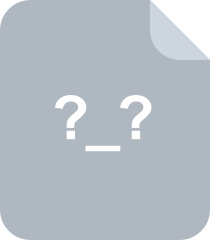
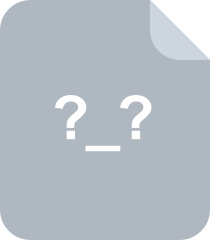
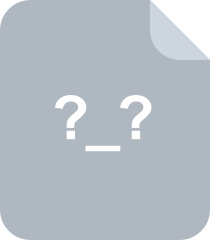
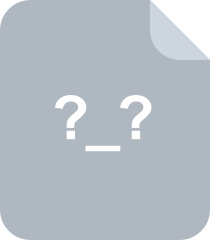
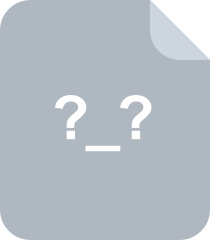
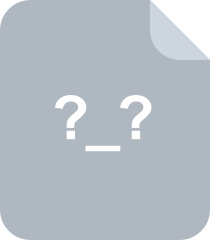
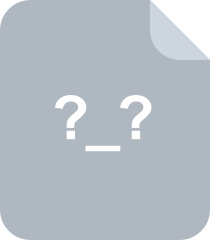
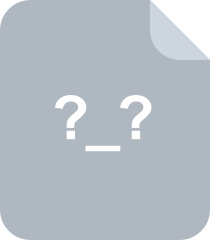
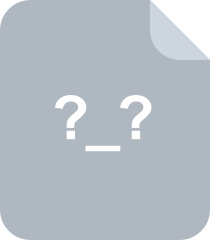
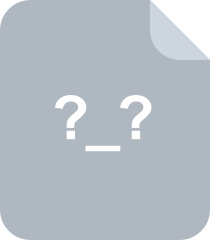
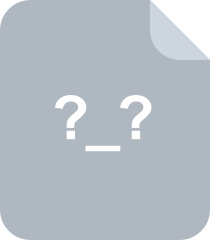
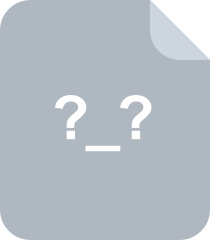
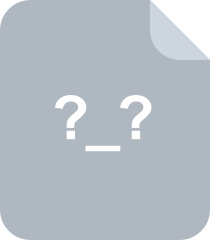
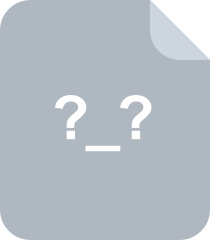
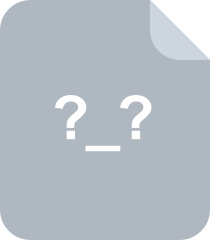
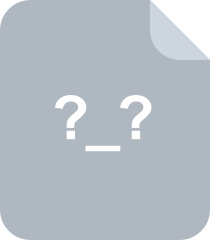
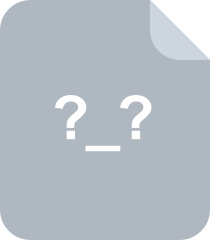
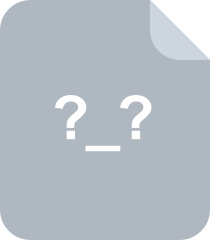
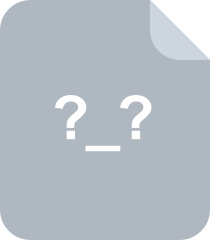
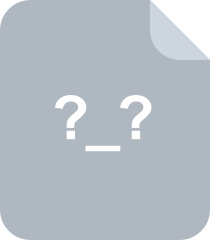
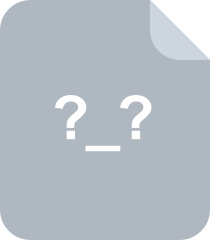
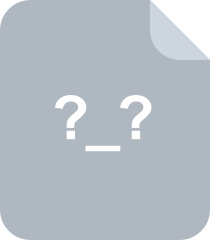
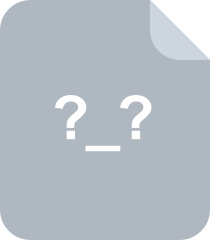
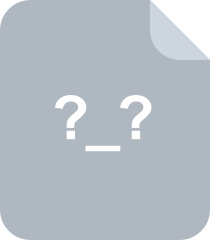
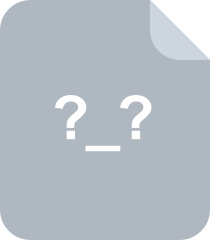
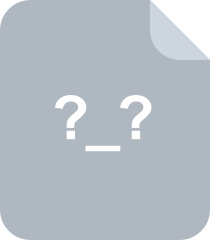
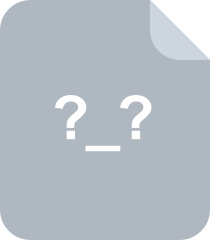
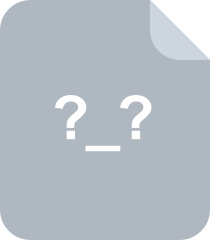
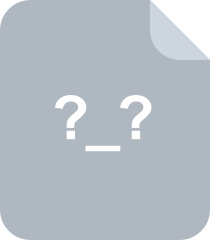
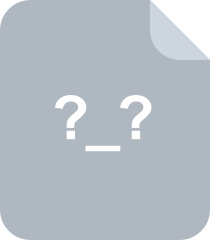
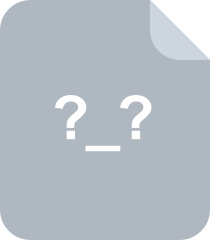
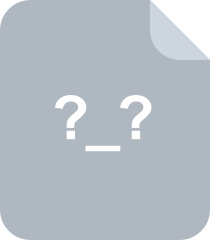
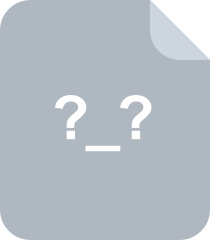
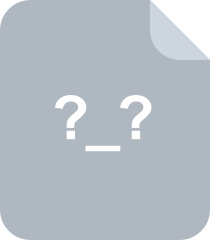
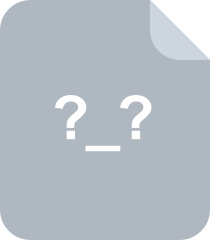
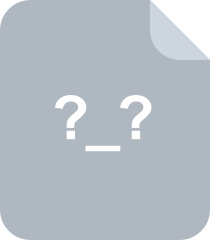
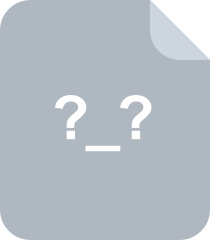
共 199 条
- 1
- 2
资源评论
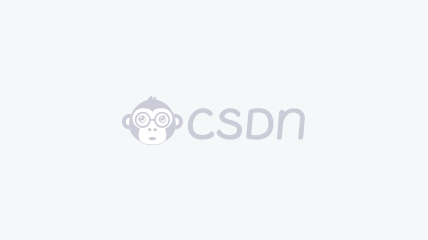

yxkfw
- 粉丝: 81
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

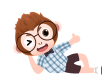
最新资源
- MineAdmin是基于Hyperf框架 和 Vue3+Vite5 开发的前后端分离权限管理系统,自适应多终端 特色:后端 crud 生成 + 前端低代码 json 化配置.zip
- Preact前端框架,一键部署到云开发平台.zip
- bpi flash读ID程序
- Lessgo 是一款简单、稳定、高效、灵活的 golang web 开发框架,支持动态路由、自动化API测试文档、热编译、热更新等,实现前后端分离、系统与业务分离.zip
- 2019计算机联考408代码题
- easyink的前端服务之一,基于企业微信JS-SDK开发的企微客户端侧边栏页面.zip
- DRF-ADMIN后台管理系统项目(端代码).zip
- micro-app-chrome-plugin是基于京东零售推出的一款为micro-app框架而开发的chrome插件.zip
- front-end project template 前端快速开发模版.zip
- LaravelAdmin,简洁、直观、强悍的前端后端开发框架,让全栈开发更迅速的SPA单页面应用.LaravelAdmin,LaravelAdmin官网.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


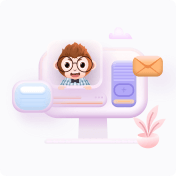
安全验证
文档复制为VIP权益,开通VIP直接复制
