// Test1View.cpp : implementation of the CTest1View class
//
#include "stdafx.h"
#include "Test1.h"
#include "Test1Doc.h"
#include "Test1View.h"
#include "MainFrm.h"
#include "ControlWnd.h"
#include "ProjectView.h"
#include "math.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
GLfloat Databuf[ArrayOne][ArrayTwo];
GLfloat xrof,yrof,zrof;//各坐标轴倾角
/////////////////////////////////////////////////////////////////////////////
// CTest1View
extern bool gbIsGetData;
extern bool gbDataIsEmpty;
IMPLEMENT_DYNCREATE(CTest1View, CView)
BEGIN_MESSAGE_MAP(CTest1View, CView)
//{{AFX_MSG_MAP(CTest1View)
ON_WM_CREATE()
ON_WM_DESTROY()
ON_WM_SIZE()
ON_COMMAND(IDC_TURNX, OnTurnx)
ON_COMMAND(IDC_TURNY, OnTurny)
ON_COMMAND(IDC_TURNZ, OnTurnz)
ON_WM_ERASEBKGND()
ON_COMMAND(IDC_RESUME, OnResume)
ON_WM_MOUSEWHEEL()
ON_WM_LBUTTONDOWN()
ON_WM_LBUTTONUP()
ON_WM_MOUSEMOVE()
ON_WM_CONTEXTMENU()
ON_WM_KILLFOCUS()
//}}AFX_MSG_MAP
// Standard printing commands
ON_COMMAND(ID_FILE_PRINT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW, CView::OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTest1View construction/destruction
CTest1View::CTest1View()
{
m_pDC = NULL;
nWithy = 0;
m_Prox = 60;
m_Proy = 5;
m_Proz = 10;
m_First = 1;
m_Second = 2;
m_Third = 3;
m_bDown = false;
m_bIsMove = true;
m_bGetFileSuccess = true;
m_pFr = NULL;
m_FileName = "data/t56a.3DSF";
m_nMinDepth = 520.00f;
m_nMaxDepth = 3299.875 ;
m_bMoveSel = false;
m_SelType = 0;
m_Zoom = 0;
m_xMove = m_yMove = m_zMove = 0;
m_bLighting = false;
}
CTest1View::~CTest1View()
{
if (m_pDC)
{
delete m_pDC;
m_pDC = NULL;
}
}
BOOL CTest1View::PreCreateWindow(CREATESTRUCT& cs)
{
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
return CView::PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
// CTest1View drawing
void CTest1View::OnDraw(CDC* pDC)
{
CTest1Doc* pDoc = GetDocument();
ASSERT_VALID(pDoc);
//清除颜色缓冲和深度缓冲
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
//用单位矩阵替换当前矩阵
glLoadIdentity();
//旋转角度
glRotatef(xrof,1.0f,0.0f,0.0f);//x轴
glRotatef(yrof,0.0f,1.0f,0.0f);//y轴
glRotatef(zrof,0.0f,0.0f,1.0f);//z轴
//选择框
if( this->m_pFr->m_pCtrlWnd->m_bShowConsult)
this->Draw3DRect();
//画点
this->PutPoint();
//坐标系和文字
if (this->m_pFr->m_pCtrlWnd->m_bShowCoordinate)
{
this->DrawLines();
this->DrawText();
}
//强制绘图完成
glFinish();
//交换缓冲区数据
SwapBuffers(wglGetCurrentDC());
//画选中区域
this->DrawSelArea(pDC);
}
/////////////////////////////////////////////////////////////////////////////
// CTest1View printing
BOOL CTest1View::OnPreparePrinting(CPrintInfo* pInfo)
{
// default preparation
return DoPreparePrinting(pInfo);
}
void CTest1View::OnBeginPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add extra initialization before printing
}
void CTest1View::OnEndPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add cleanup after printing
}
/////////////////////////////////////////////////////////////////////////////
// CTest1View diagnostics
#ifdef _DEBUG
void CTest1View::AssertValid() const
{
CView::AssertValid();
}
void CTest1View::Dump(CDumpContext& dc) const
{
CView::Dump(dc);
}
CTest1Doc* CTest1View::GetDocument() // non-debug version is inline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CTest1Doc)));
return (CTest1Doc*)m_pDocument;
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CTest1View message handlers
int CTest1View::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CView::OnCreate(lpCreateStruct) == -1)
return -1;
return 0;
}
//在此释放OpenGL使用的设备环境
void CTest1View::OnDestroy()
{
CView::OnDestroy();
HGLRC hrc;
hrc = ::wglGetCurrentContext();
::wglMakeCurrent(NULL, NULL);
if (hrc)
::wglDeleteContext(hrc);
}
//设置视口
void CTest1View::OnSize(UINT nType, int cx, int cy)
{
CView::OnSize(nType, cx, cy);
SetViewPort(cx,cy);
}
void CTest1View::SetViewPort(int cx, int cy)
{
this->m_xRect = cx;
this->m_yRect = cy;
//避免除数为0
if(m_yRect==0)
m_yRect=1;
glViewport(0,0,m_xRect,m_yRect);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if(m_xRect>=m_yRect)
{
glOrtho(-20.0,20.0,-20.0*(GLfloat)m_yRect/(GLfloat)m_xRect, 20.0*(GLfloat)m_yRect/(GLfloat)m_xRect,-50.0,50.0);
}
else
{
glOrtho(-20.0*(GLfloat)m_xRect/(GLfloat)m_yRect,20.0*(GLfloat)m_xRect/(GLfloat)m_yRect,-20.0,20.0,-50.0,50.0);
}
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
//从文件中读取数据
bool CTest1View::GetFileData(CString filename)
{
::gbIsGetData = true;
::gbDataIsEmpty = true;
::SendMessage(this->m_pFr->m_pCtrlWnd->m_hWnd,MESSAGE_DISABLEBTN,0,0);
this->ZeroArray();
this->m_pFr->InvalidateAllWnd();//Invalidate();
FILE *stream;
GLfloat Num;
if( (stream = fopen( filename, "r+t" )) != NULL )
{
int n = 0,m = 0;
for (int i=0; i<ArrayOne; i++)
{
if (n == 0 || n == 200)
{
m ++;
::SendMessage(this->m_pFr->m_hWnd,MESSAGE_STEPPRO,m,0);
n = 0;
}
n++;
for(int j=0; j<ArrayTwo; j++)
{
fscanf(stream,"%f",&Num);
Databuf[i][j]=Num;
}
}
fclose( stream );
::gbIsGetData = false;
m_bGetFileSuccess = true;
::gbDataIsEmpty = false;
::SendMessage(this->m_pFr->m_pCtrlWnd->m_hWnd,MESSAGE_ENABLEBTN,0,0);
::SendMessage(this->m_pFr->m_pCtrlWnd->m_hWnd,MESSAGE_GETDATAFINISHED,0,0);
}
else
m_bGetFileSuccess = false;
return m_bGetFileSuccess;
}
//设置适于OpenGL使用的像素格式
bool CTest1View::bSetPixelFormat()
{
//定义一种像素格式
static PIXELFORMATDESCRIPTOR pfd =
{
sizeof(PIXELFORMATDESCRIPTOR), // size of this pfd
1, // version number
PFD_DRAW_TO_WINDOW |// support window
PFD_SUPPORT_OPENGL | // support OpenGL 支持OpenGL
PFD_DOUBLEBUFFER, // double buffered 支持又缓冲
PFD_TYPE_RGBA, // RGBA type使用RGBA模式,不用调色板
24, // 24-bit color depth 使用24位真彩色
0, 0, 0, 0, 0, 0, // color bits ignored
0, // no alpha buffer
0, // shift bit ignored
0, // no accumulation buffer
0, 0, 0, 0, // accum bits ignored
32, // 32-bit z-buffer 32位Z轴缓冲
0, // no stencil buffer
0, // no auxiliary buffer
PFD_MAIN_PLANE, // main layer
0, // reserved
0, 0, 0 // layer masks ignored
};
int pixelformat;
//如果可以得到指定的
if ( (pixelformat = ChoosePixelFormat(m_pDC->GetSafeHdc(), &pfd)) == FALSE )
{
AfxMessageBox("ChoosePixelFormat failed");
return false;
}
//用上面取到的格式设置设备环境
if (SetPixelFormat(m_pDC->GetSafeHdc(), pixelformat, &pfd) == FALSE)
{
AfxMessageBox("SetPixelFormat failed");
return false;
}
return true;
}
//初始化OpenGL环境
void CTest1View::IniOpenGL()
{
m_pDC = new CClientDC(this);
PIXELFORMATDESCRIPTOR pfd;
int n;
//定义一个绘制上下文的句柄
HGLRC hrc;
//初始化过程中主要就是初始化了一个客户区的设备环境指针
ASSERT(m_pDC != NULL);
//建立应用所需的像素格式,并与当前设备上下文相关连
if (!bSetPixelFormat())
return;
//得到指定设备环境的象素模式索引
n = ::GetPixelFormat(m_pDC->GetSafeHdc());
//根据上面得到的索引值来声明一个象素模式
::DescribePixelFormat(m_pDC->GetSafeHdc(), n, sizeof(pfd), &pfd);
//创建一个上下文设备环境
hrc = wglCreateContext(m_pDC->GetSafeHdc());
//将刚生成的设备上下文指针设为当前环境
wglMakeCurrent(m_pDC->GetSafeHdc(), hrc);
//置黑背景
glClearColor(0.0,0.0,0.0,0.0);
glCle
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
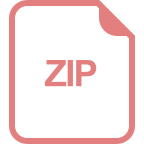
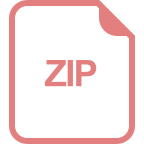
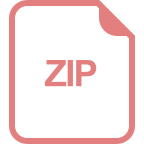
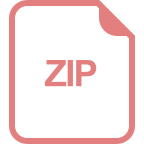
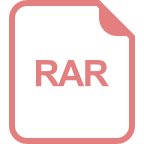
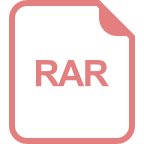
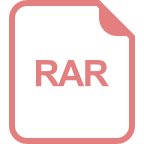
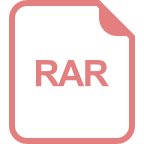
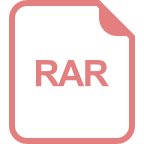
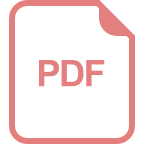
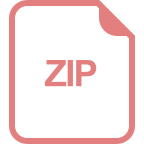
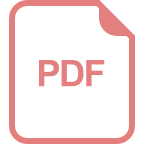
收起资源包目录


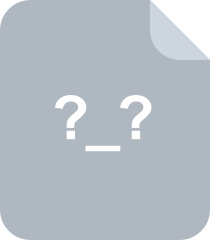

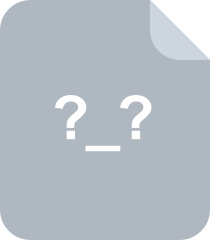
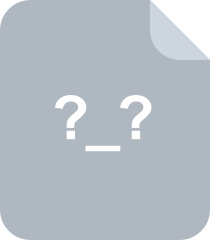
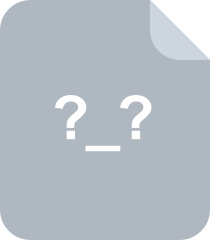
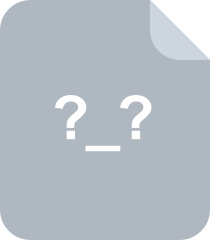

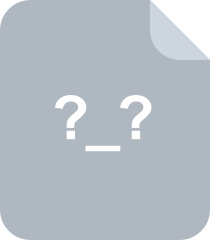
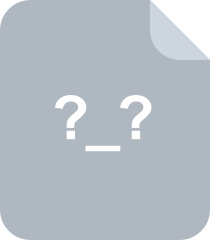
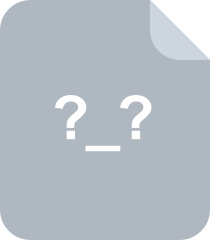
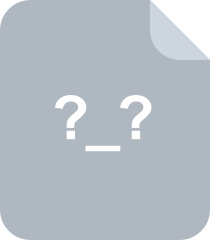

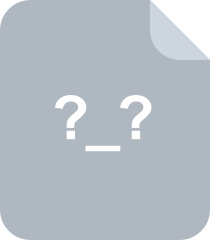
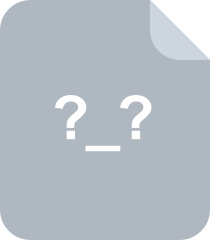
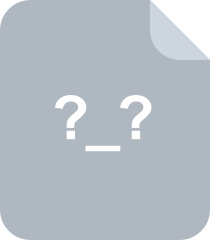
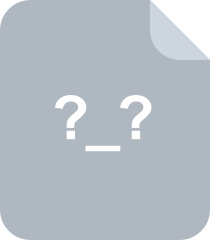
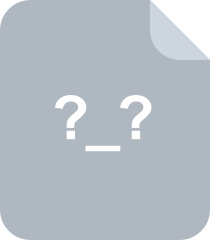
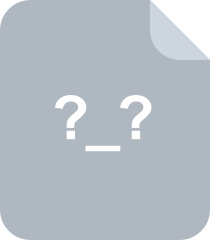
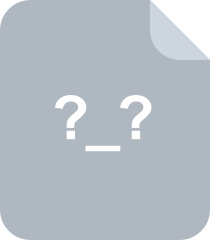
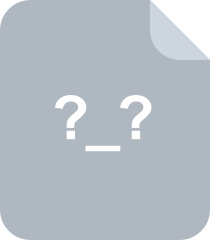
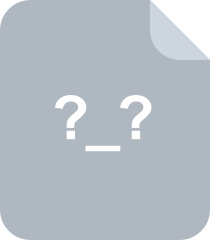
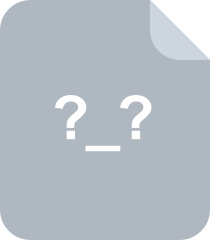
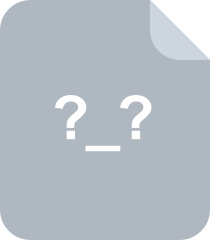
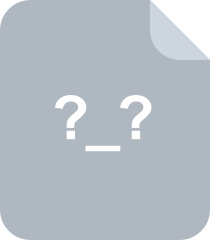

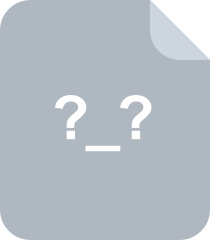
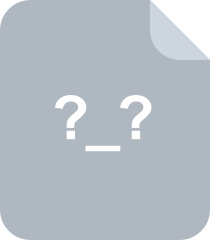
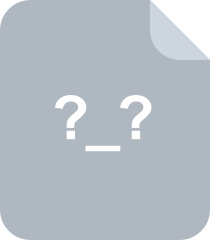

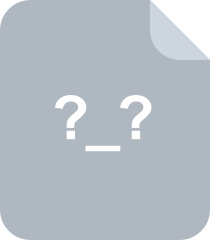
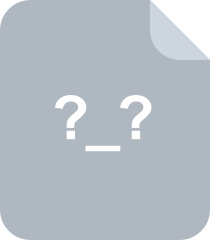
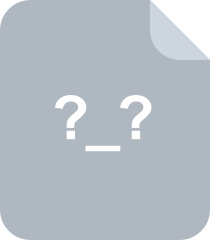
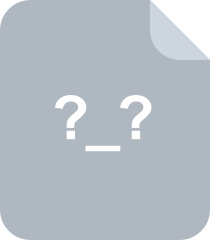
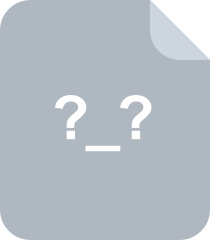
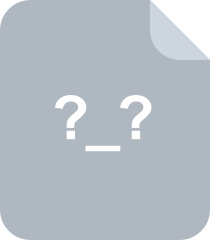
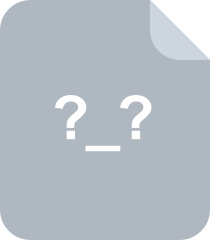
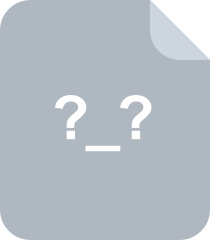
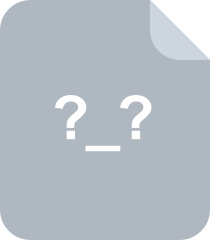
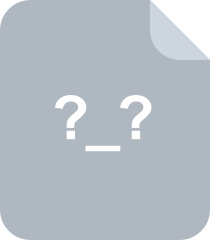
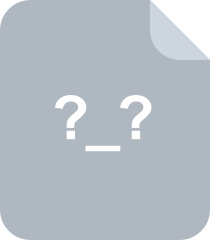
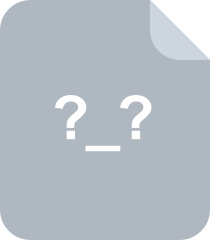

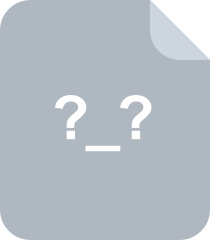
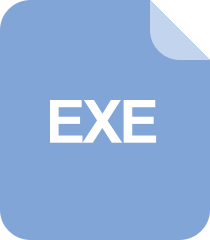
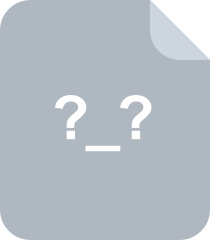
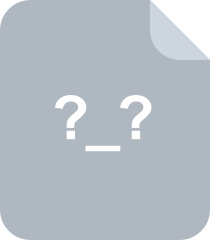
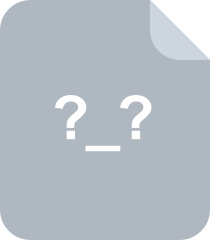
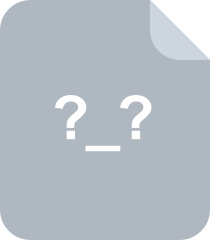
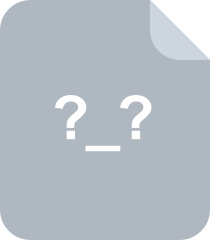
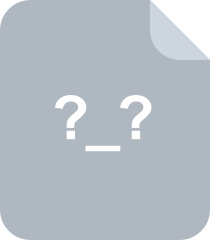
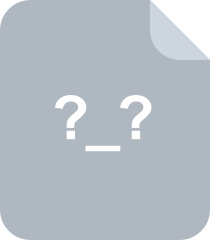
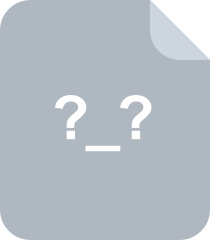
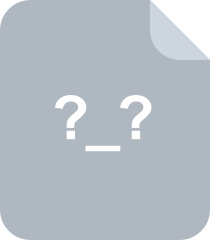
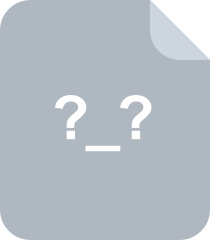
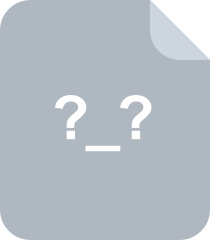
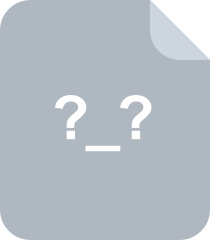
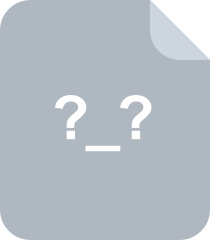
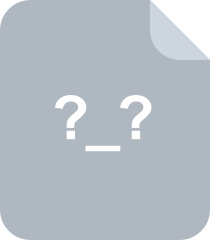
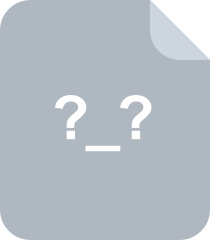
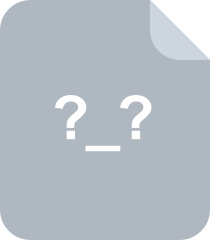
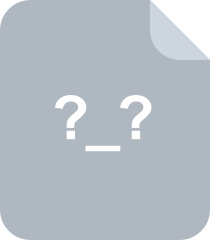
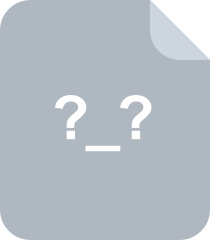
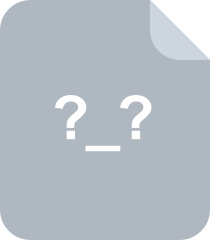
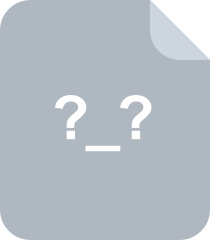

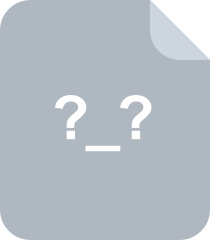
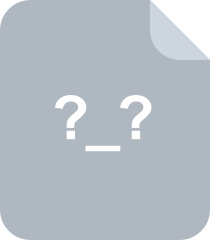
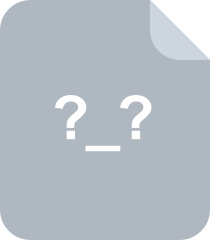

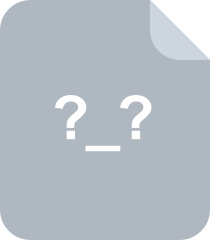
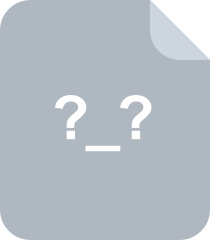
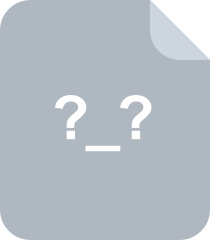
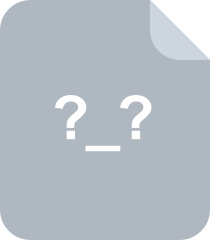
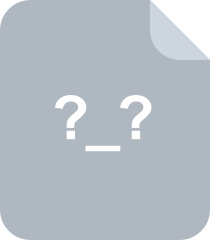
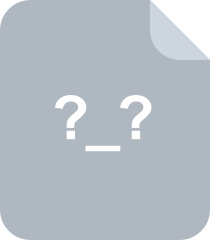
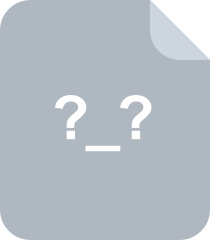
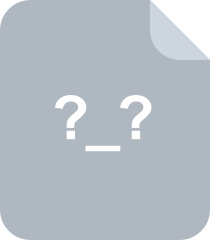
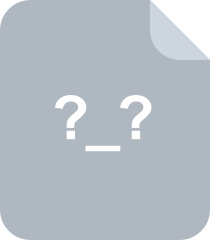
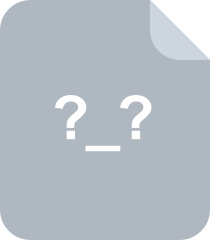
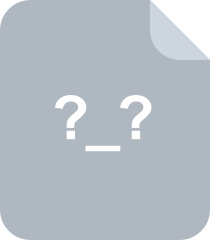
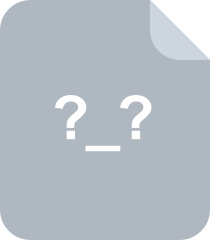
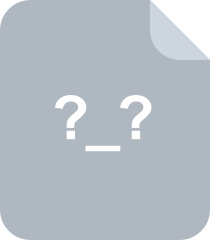

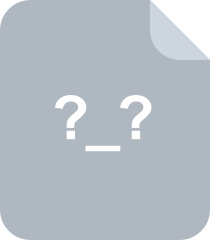
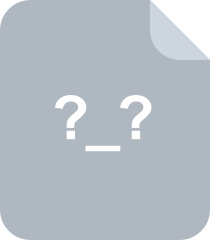
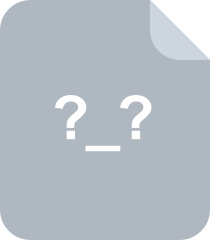
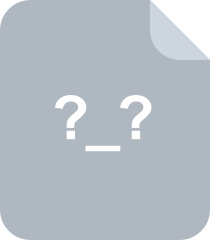
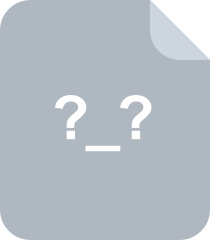
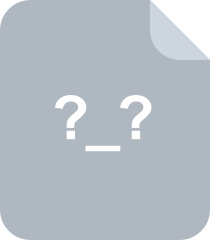
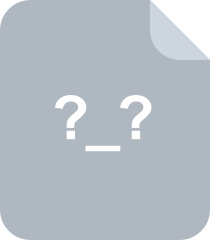
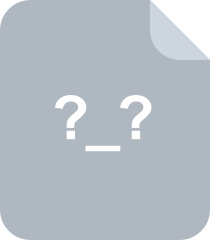
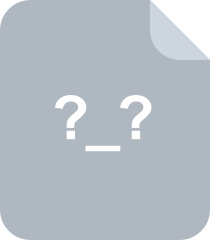
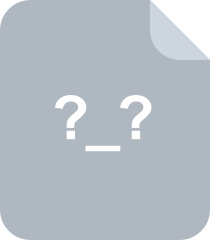
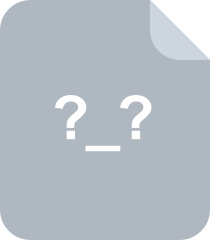
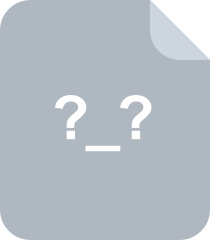
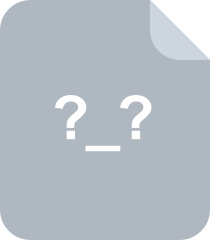
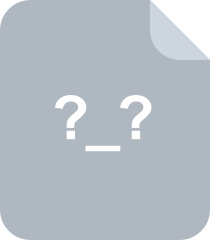
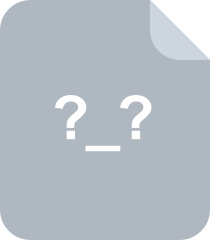

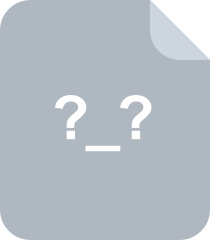
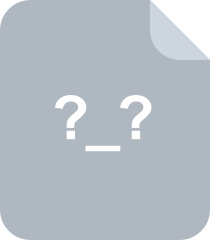
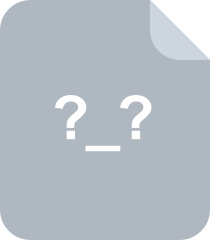
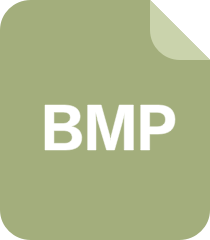
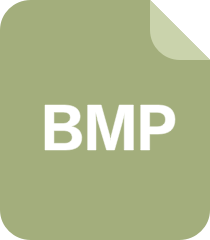
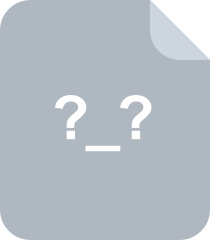
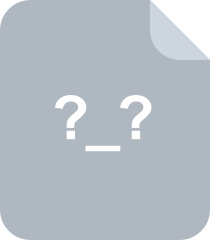
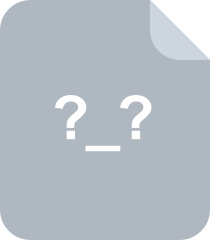
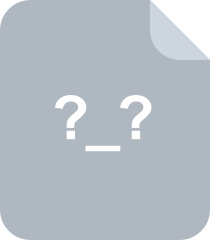
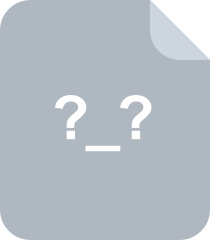
共 99 条
- 1

yujunnb
- 粉丝: 43
- 资源: 105
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

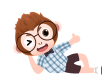
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页