### Java五子棋程序知识点解析 #### 一、程序结构与设计 本Java五子棋程序采用图形用户界面(GUI)的方式实现,主要利用了`java.awt`和`javax.swing`包中的类来构建游戏界面,并通过事件监听器处理用户的鼠标点击事件。整个程序的核心逻辑包括棋盘绘制、棋子放置以及胜负判断。 #### 二、关键代码分析 1. **初始化棋盘和棋子状态** ```java int chess[][] = new int[11][11]; boolean Is_Black_True; mypanel() { Is_Black_True = true; for (int i = 0; i < 11; i++) { for (int j = 0; j < 11; j++) { chess[i][j] = 0; } } addMouseListener(this); setBackground(Color.BLUE); setBounds(0, 0, 360, 360); setVisible(true); } ``` - **说明**:这部分代码用于初始化棋盘和棋子的状态。`chess[][]`数组用于记录每个位置上的棋子情况,其中`0`表示该位置为空,`1`表示黑棋,`2`表示白棋。`Is_Black_True`变量用于标记当前轮到哪一方落子,初始为`true`表示先手是黑棋。 2. **鼠标点击事件处理** ```java public void mousePressed(MouseEvent e) { int x = e.getX(); int y = e.getY(); if (x < 25 || x > 330 + 25 || y < 25 || y > 330 + 25) { return; } if (chess[x / 30 - 1][y / 30 - 1] != 0) { return; } // 根据当前玩家,放置棋子并更新状态 // ... repaint(); // 重绘界面 Justisewiner(); // 判断是否有赢家 } ``` - **说明**:当用户在界面上点击时,此方法会被调用。它首先检查点击的位置是否合法(即位于棋盘内且该位置未被占用),然后根据当前玩家放置棋子,并更新界面显示及玩家状态。 3. **棋盘绘制** ```java void Drawline(Graphics g) { for (int i = 30; i <= 330; i += 30) { for (int j = 30; j <= 330; j += 30) { g.setColor(Color.WHITE); g.drawLine(i, j, i, 330); } } for (int j = 30; j <= 330; j += 30) { g.setColor(Color.WHITE); g.drawLine(30, j, 330, j); } } void Drawchess(Graphics g) { for (int i = 0; i < 11; i++) { for (int j = 0; j < 11; j++) { if (chess[i][j] == 1) { g.setColor(Color.BLACK); g.fillOval((i + 1) * 30 - 8, (j + 1) * 30 - 8, 16, 16); } else if (chess[i][j] == 2) { g.setColor(Color.WHITE); g.fillOval((i + 1) * 30 - 8, (j + 1) * 30 - 8, 16, 16); } } } } ``` - **说明**:这两个方法分别用于绘制棋盘线和棋子。`Drawline()`方法通过循环绘制水平和垂直线条形成棋盘网格;`Drawchess()`方法则遍历`chess[][]`数组,根据数组中的值绘制相应颜色的棋子。 4. **胜负判断** ```java void Justisewiner() { int black_count = 0; int white_count = 0; int i = 0; // 检查行和列 // ... // 检查对角线 for (i = 0; i < 7; i++) { for (int j = 0; j < 7; j++) { // 检查主对角线 // ... // 检查副对角线 // ... } } } ``` - **说明**:这部分代码用于判断当前是否有玩家赢得比赛。它通过遍历`chess[][]`数组,检查每一行、每一列以及两条对角线上的连续棋子数量,若某一方连续五个棋子,则该方获胜。 #### 三、总结 以上是对“java五子棋”程序的主要知识点进行的详细解析。通过这段代码,我们不仅可以看到如何使用Java AWT和Swing包构建图形用户界面,还可以了解到如何处理鼠标事件、绘制图形元素以及实现基本的游戏逻辑。这对于学习Java GUI编程和游戏开发都是很好的实践案例。
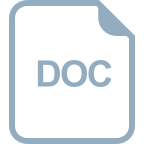
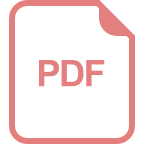
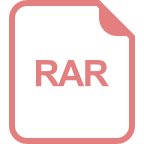
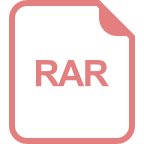
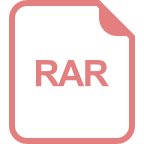
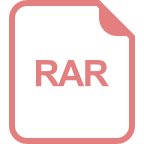
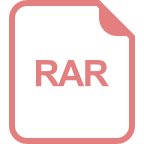
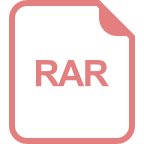
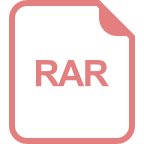
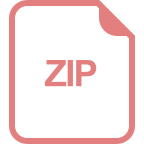
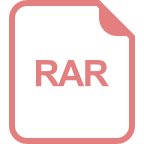
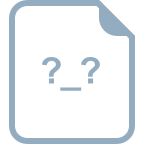
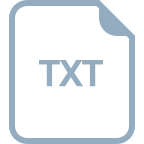
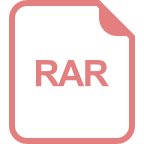
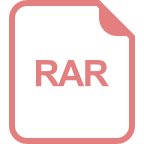
import java.awt.event.*;
import javax.swing.*;
class mypanel extends Panel implements MouseListener
{
int chess[][] = new int[11][11];
boolean Is_Black_True;
mypanel()
{
Is_Black_True = true;
for(int i = 0;i < 11;i++)
{
for(int j = 0;j < 11;j++)
{
chess[i][j] = 0;
}
}
addMouseListener(this);
setBackground(Color.BLUE);
setBounds(0, 0, 360, 360);
setVisible(true);
}
public void mousePressed(MouseEvent e)
{
int x = e.getX();
int y = e.getY();
if(x < 25 || x > 330 + 25 ||y < 25 || y > 330+25)
{
}
if(chess[x/30-1][y/30-1] != 0)
{
return;
}
if(Is_Black_True == true)
{
chess[x/30-1][y/30-1] = 1;
Is_Black_True = false;
repaint();
Justisewiner();
return;
}
if(Is_Black_True == false)
{
chess[x/30-1][y/30-1] = 2;
Is_Black_True = true;
repaint();
Justisewiner();
return;
}
}
void Drawline(Graphics g)
{
for(int i = 30;i <= 330;i += 30)
{
for(int j = 30;j <= 330; j+= 30)
{
g.setColor(Color.WHITE);
剩余10页未读,继续阅读
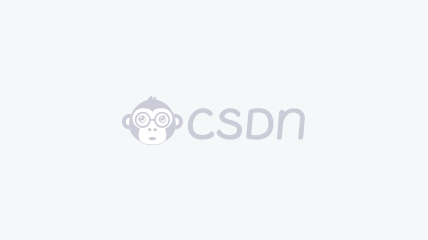

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

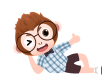
最新资源
- 【java毕业设计】直销模式下家具工厂自建网站源码(ssm+mysql+说明文档+LW).zip
- <项目代码>YOLOv8 遥感航拍飞机识别<目标检测>
- 基础入门:30多种加密编码技术及应用场景详解
- 网络抓包与封包技术解析-应用于各类应用及系统的详细指南
- 【java毕业设计】在线心理评测与咨询系统源码(ssm+mysql+说明文档+LW).zip
- 中国2012-2022年各地区新质生产力水平测算数据(王珏版)【重磅,更新!】
- Web应用架构与安全漏洞基础教程
- 【java毕业设计】尤文图斯足球俱乐部网上商城系统源码(ssm+mysql+说明文档+LW).zip
- 正则表达式 python
- 网络安全渗透测试:操作系统命令与技巧用于文件操作及反弹Shell

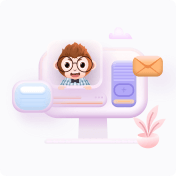
