package five;
import java.awt.BorderLayout;
import java.awt.Button;
import java.awt.Frame;
import java.awt.Label;
import java.awt.TextArea;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.sql.Date;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import game.Game;
import game.GameDao;
import game.Manual;
import game.ManualDao;
import user.User;
import user.UserDao;
public class FiveServer extends Frame implements ActionListener {// 服务器端界面
Label lStatus = new Label("当前连接数:0", Label.LEFT);// 客户端连接数
TextArea taMessage = new TextArea("", 22, 50, TextArea.SCROLLBARS_VERTICAL_ONLY);// 显示客户端行为
Button btServerClose = new Button("关闭服务器");
ServerSocket ss = null;// 服务器套接字
public static final int TCP_PORT = 4801; // 服务器端口号常量
static int clientNum = 0; // 记录当前连接到服务器上的客户端数量
ArrayList<Client> clients = new ArrayList<Client>(); // 保存客户端信息的链表
public static void main(String[] args) {
FiveServer fs = new FiveServer();
fs.startServser();
}
public FiveServer() {
super("JAVA 五子棋服务器");
btServerClose.addActionListener(this);
add(lStatus, BorderLayout.NORTH);
add(taMessage, BorderLayout.CENTER);
add(btServerClose, BorderLayout.SOUTH);
setLocation(500, 200);
pack();
setVisible(true);
setResizable(false);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btServerClose) {
System.exit(0);
}
}
class Client { // 内部类:记录连接到服务器的客户端信息
String name;
Socket s;
String state; // 1、ready 2、playing
Client opponent;
String chessColor;// 该客户端执棋的颜色
int step;// 下棋的手数
int[][] coordinates;// 每一步棋子的坐标
public Client(String name, Socket s) {
this.name = name;
this.s = s;
this.state = "ready";
this.opponent = null;
step = 0;
coordinates = new int[19 * 19][2];
}
}
class ClientThread extends Thread {// 内部线程类:服务器接收客户端消息
private Client c;
private DataInputStream dis;
private DataOutputStream dos;
ClientThread(Client c) {
this.c = c;
}
public void run() {
while (true) {
try {
dis = new DataInputStream(c.s.getInputStream());
String msg = dis.readUTF();
String[] words = msg.split(":");
if (words[0].equals(Command.JOIN)) {// join:+opponentName:+playingTime
String opponentName = words[1];
String playingTime = words[2];
for (int i = 0; i < clients.size(); i++) {
if (clients.get(i).name.equals(opponentName)) {
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.JOIN + ":" + c.name + ":" + playingTime);
break;
}
}
} else if (words[0].equals(Command.REFUSE)) {
// c.state = "palying";
String opponentName = words[1];
for (int i = 0; i < clients.size(); i++) {
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.REFUSE + ":" + c.name);
break;
}
} else if (words[0].equals(Command.AGREE)) {
c.state = "playing";
String opponentName = words[1];
for (int i = 0; i < clients.size(); i++) {
if (clients.get(i).name.equals(opponentName)) {
clients.get(i).state = "playng";
clients.get(i).opponent = c;
c.opponent = clients.get(i);
break;
}
}
for (int i = 0; i < clients.size(); i++) {// 改变所有客户端中这两个客户的状态为playing
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.CHANGE + ":" + c.name + ":playing");
dos.writeUTF(Command.CHANGE + ":" + opponentName + ":playing");
}
int r = (int) (Math.random() * 2);// 随机分配黑旗、白棋
if (r == 0) {
dos = new DataOutputStream(c.s.getOutputStream());
dos.writeUTF(Command.GUESSCOLOR + ":black:" + opponentName);
dos = new DataOutputStream(c.opponent.s.getOutputStream());
dos.writeUTF(Command.GUESSCOLOR + ":white:" + c.name);
c.chessColor = "black";
c.opponent.chessColor = "white";
} else {
dos = new DataOutputStream(c.s.getOutputStream());
dos.writeUTF(Command.GUESSCOLOR + ":white:" + opponentName);
dos = new DataOutputStream(c.opponent.s.getOutputStream());
dos.writeUTF(Command.GUESSCOLOR + ":black:" + c.name);
c.chessColor = "white";
c.opponent.chessColor = "black";
}
taMessage.append(c.name + " playing\n");
taMessage.append(opponentName + " playing\n");
} else if (words[0].equals(Command.GO)) {
dos = new DataOutputStream(c.opponent.s.getOutputStream());
dos.writeUTF(msg);
taMessage.append(c.name + " " + msg + "\n");
String x = words[1];
String y = words[2];
c.coordinates[c.step][0] = Integer.valueOf(x);
c.coordinates[c.step][1] = Integer.valueOf(y);
c.step++;
c.opponent.coordinates[c.opponent.step][0] = Integer.valueOf(x);
c.opponent.coordinates[c.opponent.step][1] = Integer.valueOf(y);
c.opponent.step++;
} else if (words[0].equals(Command.WIN)) {
for (int i = 0; i < clients.size(); i++) {// 改变所有客户端客户列表中这两个客户的状态为”ready“
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.CHANGE + ":" + c.name + ":ready");
dos.writeUTF(Command.CHANGE + ":" + c.opponent.name + ":ready");
}
dos = new DataOutputStream(c.s.getOutputStream());
dos.writeUTF(Command.TELLRESULT + ":win");// 向自己发挥胜利的命令
dos = new DataOutputStream(c.opponent.s.getOutputStream());
dos.writeUTF(Command.TELLRESULT + ":losses");// 向对方发送失败的命令
c.state = "ready";
c.opponent.state = "ready";
taMessage.append(c.name + " win\n");
taMessage.append(c.opponent.name + " losses\n");
Date date = new Date(new java.util.Date().getTime());
recordGame(date);
recordManual(date);
} else if (words[0].equals(Command.GIVEUP)) {
for (int i = 0; i < clients.size(); i++) {
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.CHANGE + ":" + c.name + ":ready");
dos.writeUTF(Command.CHANGE + ":" + c.opponent.name + ":ready");
}
dos = new DataOutputStream(c.s.getOutputStream());
dos.writeUTF(Command.TELLRESULT + ":losses");
dos = new DataOutputStream(c.opponent.s.getOutputStream());
dos.writeUTF(Command.TELLRESULT + ":win");
c.state = "ready";
c.opponent.state = "ready";
taMessage.append(c.name + " loss\n");
taMessage.append(c.opponent.name + " win\n");
} else if (words[0].equals(Command.QUIT)) {
for (int i = 0; i < clients.size(); i++) {
if (clients.get(i) != c) {
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.DELETE + ":" + c.name);
}
}
clients.remove(c);
taMessage.append(c.name + " quit\n");
clientNum--;
lStatus.setText("连接数:" + clientNum);
return;
} else if (words[0].equals(Command.TALK)) {
for (int i = 0; i < clients.size(); i++) {
dos = new DataOutputStream(clients.get(i).s.getOutputStream());
dos.writeUTF(Command.TALK + ":" + c.name + ":" + msg);
}
taMessage.append(c.name + " talk\n");
} else if (words[0].equals(Command.GAME)) {
ArrayList<Game> games;
GameDao gd = new GameDao(
没有合适的资源?快使用搜索试试~ 我知道了~
java 五子棋游戏.zip
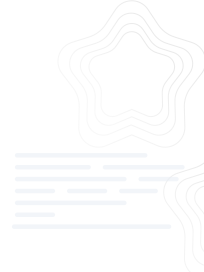
共27个文件
java:24个
jpg:3个

需积分: 5 0 下载量 158 浏览量
2024-03-30
15:34:19
上传
评论
收藏 27KB ZIP 举报
温馨提示
java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zip java 五子棋游戏.zipjava 五子棋游戏.zipjava 五子棋游戏.zipja
资源推荐
资源详情
资源评论
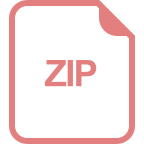
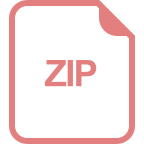
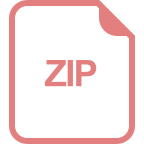
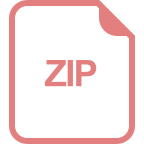
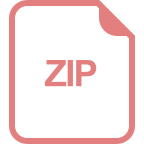
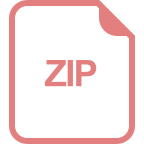
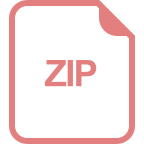
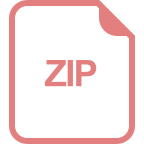
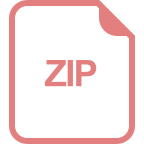
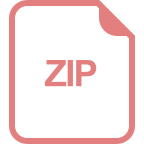
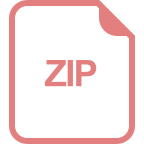
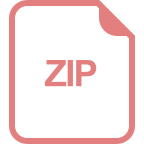
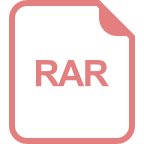
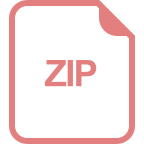
收起资源包目录




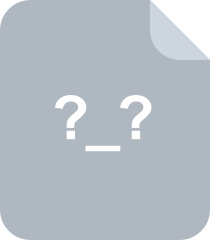
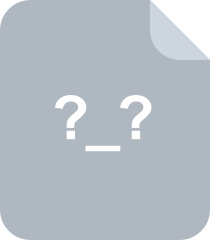
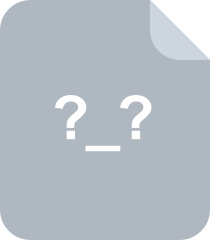
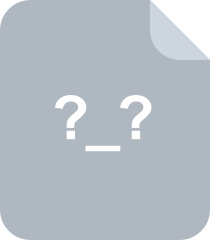
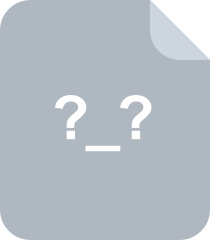
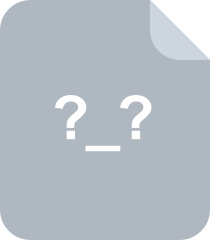

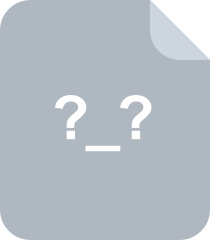
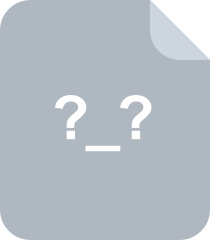
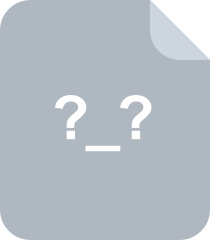
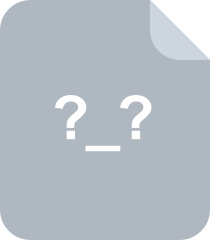
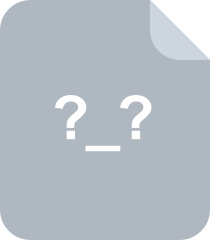
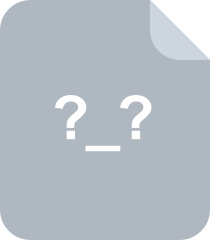
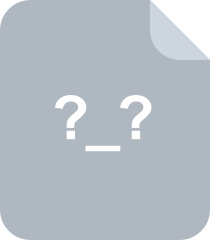
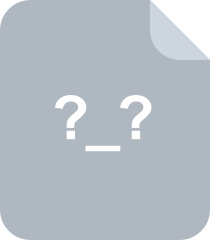
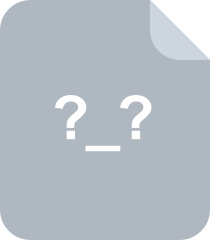
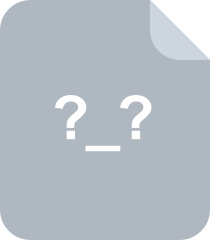
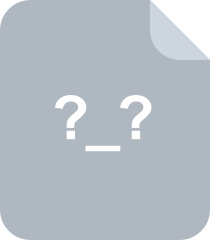
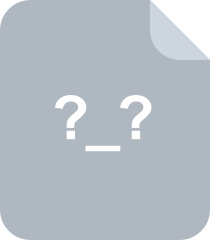

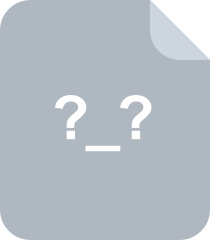
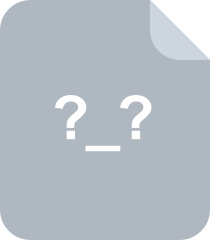
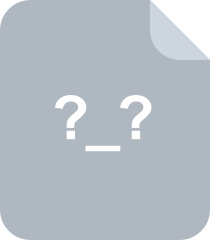
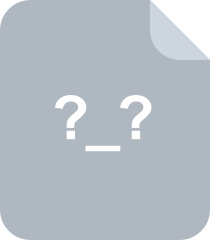
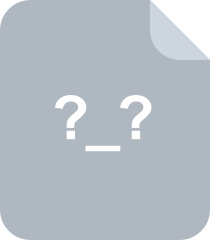
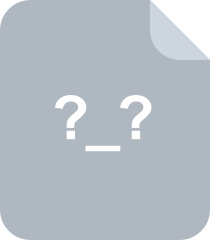

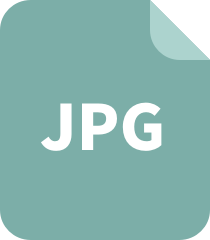
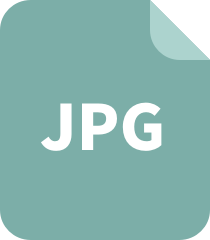
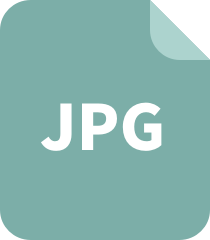
共 27 条
- 1
资源评论
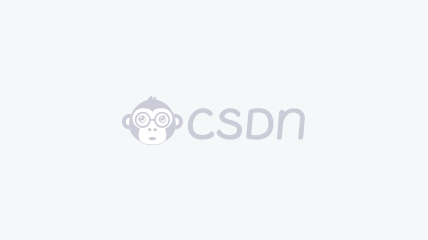

土豆片片
- 粉丝: 1852
- 资源: 5869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

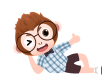
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


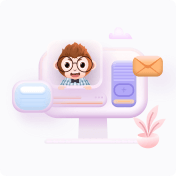
安全验证
文档复制为VIP权益,开通VIP直接复制
