/*++
Copyright (c) Microsoft Corporation. All rights reserved.
Module Name:
HIDPI.H
Abstract:
Public Interface to the HID parsing library.
Environment:
Kernel & user mode
--*/
#ifndef __HIDPI_H__
#define __HIDPI_H__
#include <pshpack4.h>
// Please include "hidsdi.h" to use the user space (dll / parser)
// Please include "hidpddi.h" to use the kernel space parser
//
// Special Link collection values for using the query functions
//
// Root collection references the collection at the base of the link
// collection tree.
// Unspecifies, references all collections in the link collection tree.
//
#define HIDP_LINK_COLLECTION_ROOT ((USHORT) -1)
#define HIDP_LINK_COLLECTION_UNSPECIFIED ((USHORT) 0)
typedef enum _HIDP_REPORT_TYPE
{
HidP_Input,
HidP_Output,
HidP_Feature
} HIDP_REPORT_TYPE;
typedef struct _USAGE_AND_PAGE
{
USAGE Usage;
USAGE UsagePage;
} USAGE_AND_PAGE, *PUSAGE_AND_PAGE;
#define HidP_IsSameUsageAndPage(u1, u2) ((* (PULONG) &u1) == (* (PULONG) &u2))
typedef struct _HIDP_BUTTON_CAPS
{
USAGE UsagePage;
UCHAR ReportID;
BOOLEAN IsAlias;
USHORT BitField;
USHORT LinkCollection; // A unique internal index pointer
USAGE LinkUsage;
USAGE LinkUsagePage;
BOOLEAN IsRange;
BOOLEAN IsStringRange;
BOOLEAN IsDesignatorRange;
BOOLEAN IsAbsolute;
ULONG Reserved[10];
union {
struct {
USAGE UsageMin, UsageMax;
USHORT StringMin, StringMax;
USHORT DesignatorMin, DesignatorMax;
USHORT DataIndexMin, DataIndexMax;
} Range;
struct {
USAGE Usage, Reserved1;
USHORT StringIndex, Reserved2;
USHORT DesignatorIndex, Reserved3;
USHORT DataIndex, Reserved4;
} NotRange;
};
} HIDP_BUTTON_CAPS, *PHIDP_BUTTON_CAPS;
typedef struct _HIDP_VALUE_CAPS
{
USAGE UsagePage;
UCHAR ReportID;
BOOLEAN IsAlias;
USHORT BitField;
USHORT LinkCollection; // A unique internal index pointer
USAGE LinkUsage;
USAGE LinkUsagePage;
BOOLEAN IsRange;
BOOLEAN IsStringRange;
BOOLEAN IsDesignatorRange;
BOOLEAN IsAbsolute;
BOOLEAN HasNull; // Does this channel have a null report union
UCHAR Reserved;
USHORT BitSize; // How many bits are devoted to this value?
USHORT ReportCount; // See Note below. Usually set to 1.
USHORT Reserved2[5];
ULONG UnitsExp;
ULONG Units;
LONG LogicalMin, LogicalMax;
LONG PhysicalMin, PhysicalMax;
union {
struct {
USAGE UsageMin, UsageMax;
USHORT StringMin, StringMax;
USHORT DesignatorMin, DesignatorMax;
USHORT DataIndexMin, DataIndexMax;
} Range;
struct {
USAGE Usage, Reserved1;
USHORT StringIndex, Reserved2;
USHORT DesignatorIndex, Reserved3;
USHORT DataIndex, Reserved4;
} NotRange;
};
} HIDP_VALUE_CAPS, *PHIDP_VALUE_CAPS;
//
// Notes:
//
// ReportCount: When a report descriptor declares an Input, Output, or
// Feature main item with fewer usage declarations than the report count, then
// the last usage applies to all remaining unspecified count in that main item.
// (As an example you might have data that required many fields to describe,
// possibly buffered bytes.) In this case, only one value cap structure is
// allocated for these associtated fields, all with the same usage, and Report
// Count reflects the number of fields involved. Normally ReportCount is 1.
// To access all of the fields in such a value structure would require using
// HidP_GetUsageValueArray and HidP_SetUsageValueArray. HidP_GetUsageValue/
// HidP_SetScaledUsageValue will also work, however, these functions will only
// work with the first field of the structure.
//
//
// The link collection tree consists of an array of LINK_COLLECTION_NODES
// where the index into this array is the same as the collection number.
//
// Given a collection A which contains a subcollection B, A is defined to be
// the parent B, and B is defined to be the child.
//
// Given collections A, B, and C where B and C are children of A, and B was
// encountered before C in the report descriptor, B is defined as a sibling of
// C. (This implies, of course, that if B is a sibling of C, then C is NOT a
// sibling of B).
//
// B is defined as the NextSibling of C if and only if there exists NO
// child collection of A, call it D, such that B is a sibling of D and D
// is a sibling of C.
//
// E is defined to be the FirstChild of A if and only if for all children of A,
// F, that are not equivalent to E, F is a sibling of E.
// (This implies, of course, that the does not exist a child of A, call it G,
// where E is a sibling of G). In other words the first sibling is the last
// link collection found in the list.
//
// In other words, if a collection B is defined within the definition of another
// collection A, B becomes a child of A. All collections with the same parent
// are considered siblings. The FirstChild of the parent collection, A, will be
// last collection defined that has A as a parent. The order of sibling pointers
// is similarly determined. When a collection B is defined, it becomes the
// FirstChild of it's parent collection. The previously defined FirstChild of the
// parent collection becomes the NextSibling of the new collection. As new
// collections with the same parent are discovered, the chain of sibling is built.
//
// With that in mind, the following describes conclusively a data structure
// that provides direct traversal up, down, and accross the link collection
// tree.
//
//
typedef struct _HIDP_LINK_COLLECTION_NODE
{
USAGE LinkUsage;
USAGE LinkUsagePage;
USHORT Parent;
USHORT NumberOfChildren;
USHORT NextSibling;
USHORT FirstChild;
ULONG CollectionType: 8; // As defined in 6.2.2.6 of HID spec
ULONG IsAlias : 1; // This link node is an allias of the next link node.
ULONG Reserved: 23;
PVOID UserContext; // The user can hang his coat here.
} HIDP_LINK_COLLECTION_NODE, *PHIDP_LINK_COLLECTION_NODE;
//
// When a link collection is described by a delimiter, alias link collection
// nodes are created. (One for each usage within the delimiter).
// The parser assigns each capability description listed above only one
// link collection.
//
// If a control is defined within a collection defined by
// delimited usages, then that control is said to be within multiple link
// collections, one for each usage within the open and close delimiter tokens.
// Such multiple link collecions are said to be aliases. The first N-1 such
// collections, listed in the link collection node array, have their IsAlias
// bit set. The last such link collection is the link collection index used
// in the capabilities described above.
// Clients wishing to set a control in an aliased collection, should walk the
// collection array once for each time they see the IsAlias flag set, and use
// the last link collection as the index for the below accessor functions.
//
// NB: if IsAlias is set, then NextSibling should be one more than the current
// link collection node index.
//
typedef PUCHAR PHIDP_REPORT_DESCRIPTOR;
typedef struct _HIDP_PREPARSED_DATA * PHIDP_PREPARSED_DATA;
typedef struct _HIDP_CAPS
{
USAGE Usage;
USAGE UsagePage;
USHORT InputReportByteLength;
USHORT OutputReportByteLength;
USHORT FeatureRe
一些头文件(包括devioctl.h、ntdddisk.h、ntddstor.h)
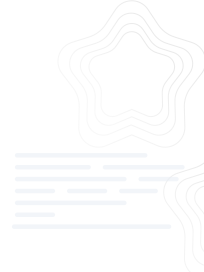

在Windows驱动程序开发中,头文件起着至关重要的作用,它们包含了系统调用、API函数声明、结构体定义以及常量枚举等信息。在这个名为"一些头文件(包括devioctl.h、ntdddisk.h、ntddstor.h)"的资源包中,我们重点关注的是与设备I/O控制和存储设备交互相关的头文件。下面将详细讨论这三个关键头文件的内容和用途。 `devioctl.h`是设备I/O控制请求的头文件。在Windows驱动程序中,设备I/O控制(Device Input/Output Control, IOCTLS)是一种机制,允许用户模式的应用程序或者内核模式的驱动程序向设备驱动发送特定的命令。这些命令通常用于配置设备、查询状态、或者执行设备特有的操作。`devioctl.h`中定义了`IOCTL`宏以及一系列用于构建IOCTL代码的常量,这些代码是驱动识别并处理特定I/O请求的关键。开发者需要依据这些定义来构造自己的设备控制请求。 接下来,`ntdddisk.h`是与磁盘设备交互的头文件。它包含了处理磁盘设备所需的结构体、常量和函数原型。例如,`DRIVE_LAYOUT_INFORMATION_EX`结构体用来表示磁盘分区的信息,`GET_DRIVE_LAYOUT_EX`和`SET_DRIVE_LAYOUT_EX`等IOCTLs用于获取或设置磁盘的分区布局。此外,`ntdddisk.h`还定义了如` IOCTL_DISK_GET_DRIVE_GEOMETRY`这样的命令,用于获取磁盘的几何信息,如磁头数、柱面数和扇区数等。这对于进行磁盘管理、数据恢复或性能优化的驱动程序来说是必不可少的。 `ntddstor.h`是针对存储设备的一般接口头文件,它不仅涵盖了磁盘,还包括其他类型的存储设备,如闪存、光驱等。这个头文件提供了更广泛的数据结构和控制命令,比如`STORAGE_PROPERTY_QUERY`和`STORAGE_DESCRIPTOR_HEADER`等,它们用于查询存储设备的属性和描述符。`ntddstor.h`中的IOCTLs如`IOCTL_STORAGE_QUERY_PROPERTY`可以用来获取设备的属性信息,例如设备类型、容量、支持的特性等。 开发Windows驱动程序时,理解并正确使用这些头文件是至关重要的。它们是驱动程序与操作系统内核通信的桥梁,允许驱动程序根据设备的具体需求执行各种操作。而`winddk`(Windows Driver Development Kit)则是一整套工具和库,为开发、调试和测试Windows驱动程序提供了全面的支持,包括这些头文件在内的所有资源。 总结来说,`devioctl.h`提供了设备I/O控制的基础,`ntdddisk.h`专注于磁盘设备的操作,而`ntddstor.h`则是更广泛的存储设备接口。这三个头文件共同构成了Windows驱动程序开发中的重要组成部分,对于编写能够高效、正确地与硬件交互的驱动程序至关重要。


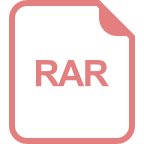
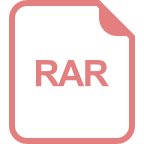













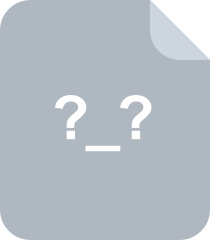
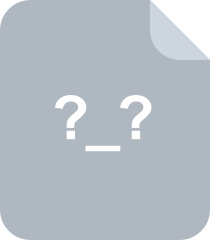
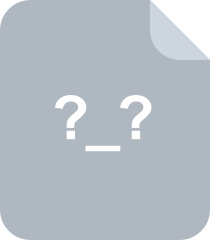
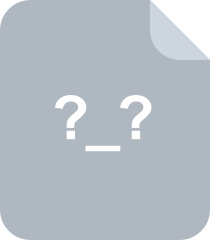
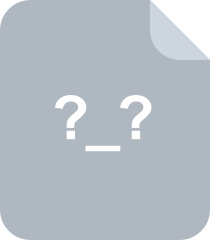
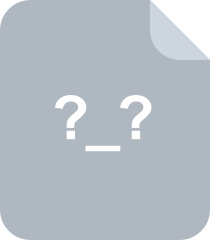
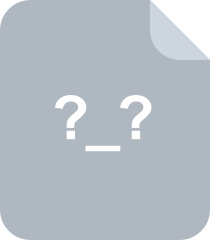
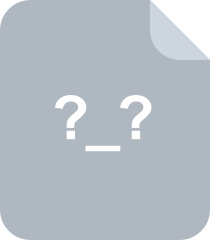
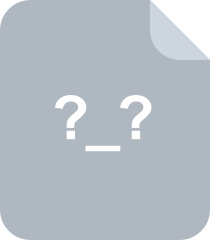
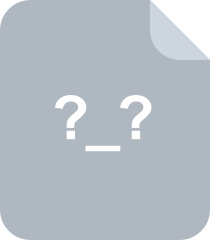
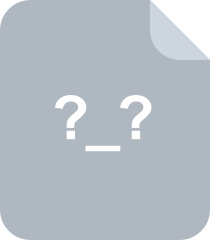
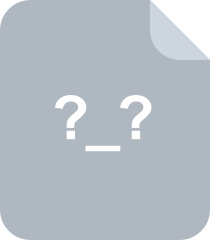
- 1
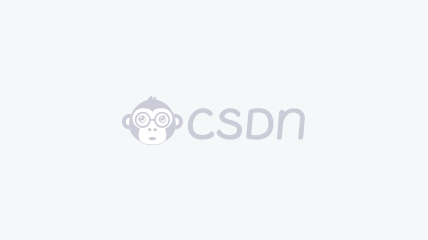
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 1012
- 资源: 38
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

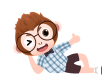
最新资源

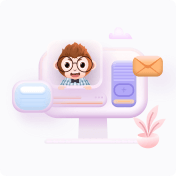
