package com.jwy.action;
import java.awt.Color;
import java.io.OutputStream;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFRichTextString;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.hssf.util.CellRangeAddress;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.actions.DispatchAction;
import com.jwy.dao.ICourseDao;
import com.jwy.dao.ISpecialtyDao;
import com.jwy.dto.Course;
import com.jwy.dto.Specialty;
import com.jwy.dto.StuUser;
import com.lowagie.text.Document;
import com.lowagie.text.Element;
import com.lowagie.text.Font;
import com.lowagie.text.PageSize;
import com.lowagie.text.Paragraph;
import com.lowagie.text.pdf.BaseFont;
import com.lowagie.text.pdf.PdfPCell;
import com.lowagie.text.pdf.PdfPTable;
import com.lowagie.text.pdf.PdfWriter;
public class StatInfoAction extends DispatchAction {
private ISpecialtyDao specialtyDao;
private ICourseDao courseDao;
/**
* @param specialtyDao
* the specialtyDao to set
*/
public void setSpecialtyDao(ISpecialtyDao specialtyDao) {
this.specialtyDao = specialtyDao;
}
/**
* @param courseDao
* the courseDao to set
*/
public void setCourseDao(ICourseDao courseDao) {
this.courseDao = courseDao;
}
/**
* 按照专业编号,课程名称,授课教师姓名进行搜索
* @param mapping
* @param form
* @param request
* @param response
* @return
*/
public ActionForward findBySearch(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
List<Specialty> sList = specialtyDao.findByAll();
Map<String, String> map = new HashMap<String, String>();
if (request.getParameter("specialtyId") != null
&& !request.getParameter("specialtyId").equals("-1")) {
map.put("specialtyId", request.getParameter("specialtyId"));
}
if (request.getParameter("name") != null
&& !request.getParameter("name").equals("")) {
map.put("name", request.getParameter("name"));
}
if (request.getParameter("teacherName") != null
&& !request.getParameter("teacherName").equals("")) {
map.put("teacherName", request.getParameter("teacherName"));
}
List<Object[]> clist = courseDao.findByStat(map);
request.setAttribute("sList", sList);
request.setAttribute("cList", clist);
return mapping.findForward("showStat");
}
/**
* 跟据课程编号查询选择该门课程的学生
* @param mapping
* @param form
* @param request
* @param response
* @return
*/
public ActionForward stuList(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
Integer specialtyId = Integer.valueOf(request
.getParameter("specialtyId"));
Specialty specialty = specialtyDao.findById(specialtyId);// 专业信息
Integer courseId = Integer.valueOf(request.getParameter("courseId"));
List<Object[]> list = courseDao.findSelectStu(courseId);
Course course = courseDao.findByID(courseId);
System.out.println(courseId);
request.setAttribute("specialty", specialty);
request.setAttribute("stuList", list);
request.setAttribute("course", course);
return mapping.findForward("stuList");
}
/**
* 将上课学生名单导出PDF文档
*
* @param mapping
* @param form
* @param request
* @param response
* @return
* @throws Exception
*/
public ActionForward exPDF(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
Integer specialtyId = Integer.valueOf(request.getParameter("specialtyId"));
Specialty specialty = specialtyDao.findById(specialtyId);// 专业信息
Integer courseId = Integer.valueOf(request.getParameter("courseId"));
List<Object[]> list = courseDao.findSelectStu(courseId);
Course course = courseDao.findByID(courseId);
response.setContentType("text/html;charset=GBK");
response.setContentType("application/xml");
response.setHeader("Content-Disposition", "attachment;filename="
+ new String(course.getName().getBytes(), "iso-8859-1")+".pdf");
OutputStream outs = response.getOutputStream(); // 获取输出流
Document document = new Document(PageSize.A4);
PdfWriter.getInstance(document, outs);
document.open();
// 设置中文字体
BaseFont bfChinese = BaseFont.createFont("STSong-Light",
"UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
Font t1 = new Font(bfChinese, 20, Font.BOLD); // 设置一级标题字体
Font t2 = new Font(bfChinese, 15, Font.BOLD); // 设置二级标题字体
Font f1 = new Font(bfChinese, 12, Font.NORMAL); // 设置正文字体
Paragraph pragraph = new Paragraph(
specialty.getEnterYear() + "届" + specialty.getLangthYear()
+ "年制" + specialty.getName() + "专业", t1);
pragraph.setAlignment(Paragraph.ALIGN_CENTER);
document.add(pragraph);
pragraph = new Paragraph(course.getName() + "课程听课人员名单"+" 听课总人数:"+list.size()+" 人", t2);
pragraph.setAlignment(Paragraph.ALIGN_CENTER);
document.add(pragraph);
pragraph = new Paragraph("授课教师" + course.getTeacherName(), t2);
pragraph.setAlignment(Paragraph.ALIGN_CENTER);
document.add(pragraph);
// 建立一个表格
PdfPTable table = new PdfPTable(4);
table.setSpacingBefore(40f);// 设置表格上面空白宽度
// 生成表头
String[] bt = new String[] { "学生姓名", "学号", "性别", "联系电话" };
for (int i = 0; i < bt.length; i++) {
PdfPCell cell = new PdfPCell(new Paragraph(bt[i], f1)); // 建立一个单元格
cell.setBackgroundColor(Color.GRAY);
cell.setHorizontalAlignment(Element.ALIGN_CENTER); // 设置内容水平居中显示
cell.setVerticalAlignment(Element.ALIGN_MIDDLE); // 设置垂直居中
table.addCell(cell);
}
for (int i = 0; i < list.size(); i++) {
Object[] o = list.get(i);
StuUser stuUser = (StuUser) o[0];
PdfPCell nameCell = new PdfPCell(new Paragraph(stuUser.getStuName(), f1)); // 建立一个单元格
nameCell.setHorizontalAlignment(Element.ALIGN_CENTER); // 设置内容水平居中显示
nameCell.setVerticalAlignment(Element.ALIGN_MIDDLE); // 设置垂直居中
PdfPCell stuNoCell = new PdfPCell(new Paragraph(stuUser.getStuNo(),f1)); // 建立一个单元格
stuNoCell.setHorizontalAlignment(Element.ALIGN_CENTER); // 设置内容水平居中显示
stuNoCell.setVerticalAlignment(Element.ALIGN_MIDDLE); // 设置垂直居中
PdfPCell stuSexCell = new PdfPCell(new Paragraph(stuUser.getStuSex(), f1)); // 建立一个单元格
stuSexCell.setHorizontalAlignment(Element.ALIGN_CENTER); // 设置内容水平居中显示
stuSexCell.setVerticalAlignment(Element.ALIGN_MIDDLE); // 设置垂直居中
PdfPCell telCell = new PdfPCell(new Paragraph(stuUser.getTel(), f1)); // 建立一个单元格
telCell.setHorizontalAlignment(Element.ALIGN_CENTER); // 设置内容水平居中显示
telCell.setVerticalAlignment(Element.ALIGN_MIDDLE); // 设置垂直居中
table.addCell(nameCell);
table.addCell(stuNoCell);
table.addCell(stuSexCell);
table.addCell(telCell);
}
document.add(table);
document.close();
outs.close();
return null;
}
/**
* 将上课学生信息导出为Excel文档
* @param mapping
* @param form
* @param request
* @param response
* @return
* @throws Exception
*/
public ActionForward exExcel(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)throws Exception {
Integer specialtyId = Integer.valueOf(request.getParameter("specialtyId"));
Specialty specialty = specialtyDao.findById(specia
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
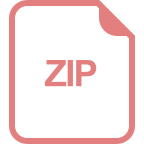
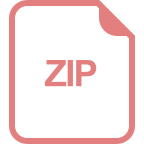
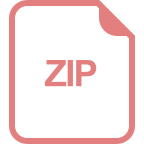
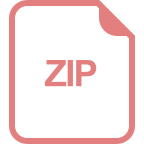
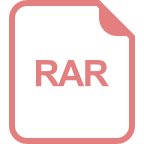
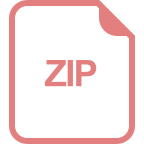
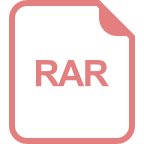
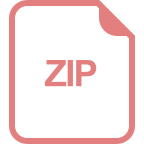
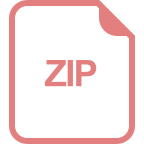
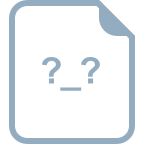
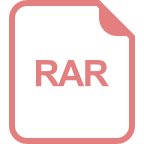
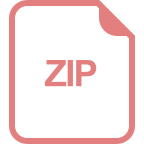
收起资源包目录

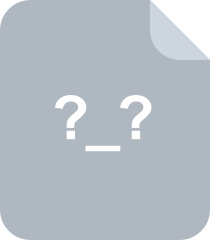
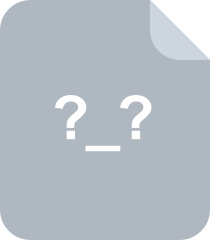
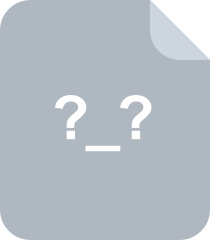
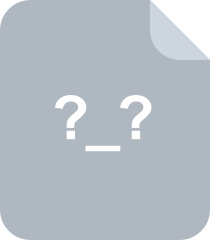
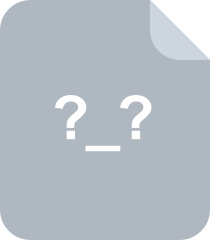
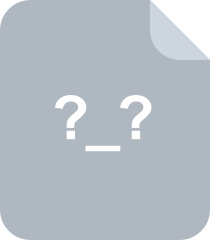
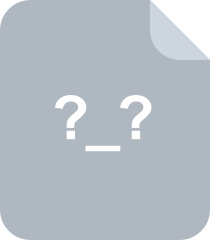
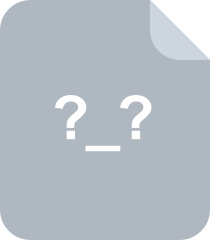
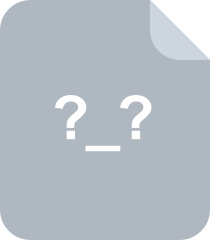
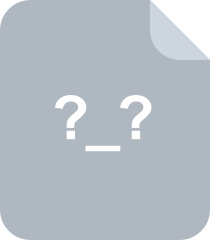
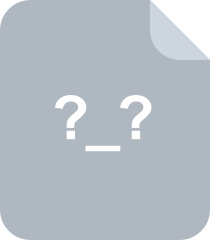
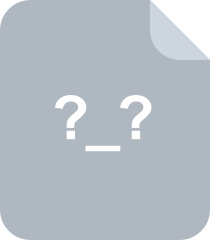
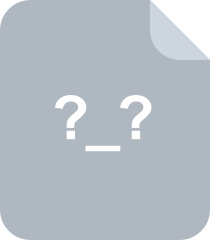
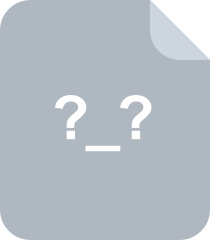
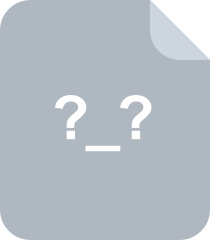
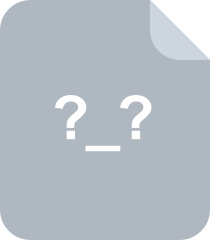
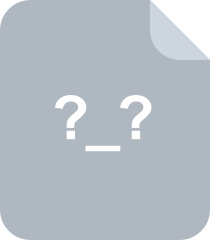
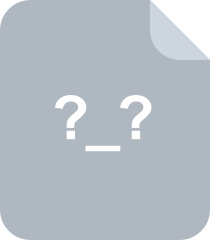
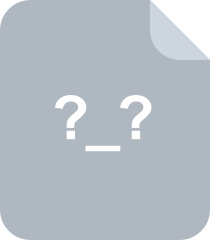
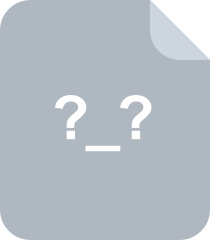
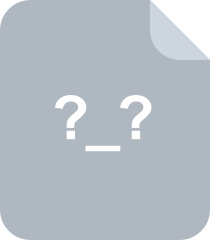
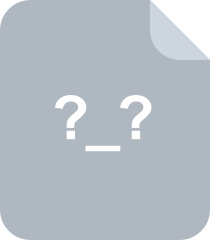
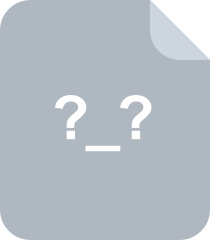
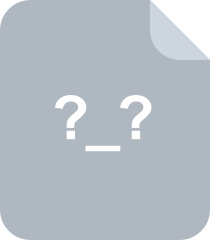
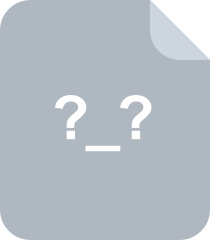
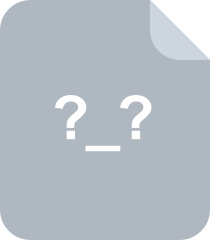
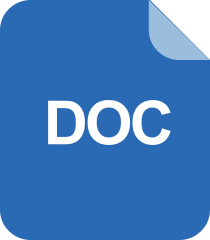
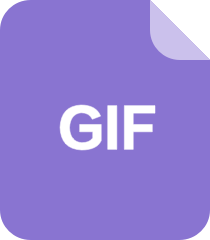
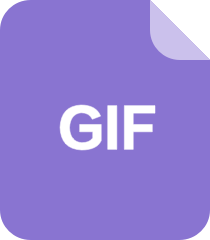
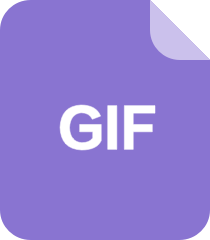
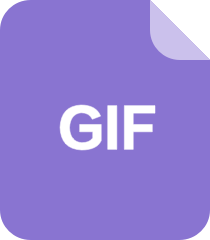
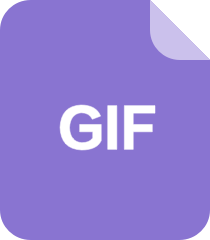
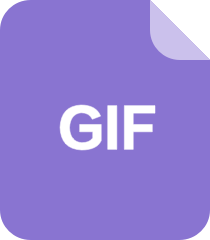
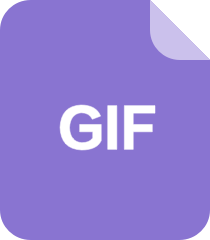
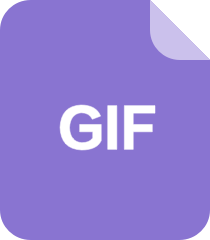
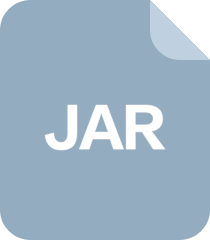
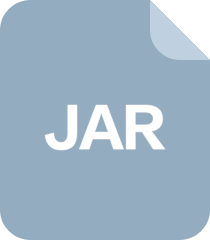
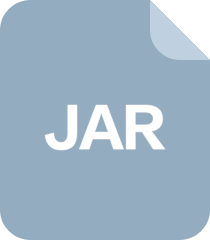
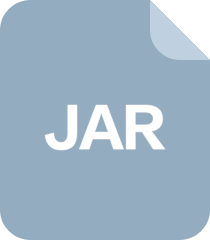
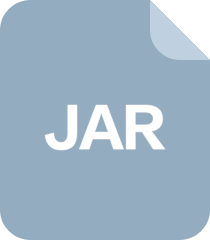
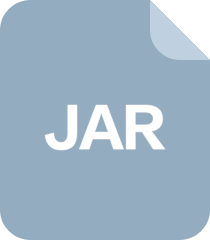
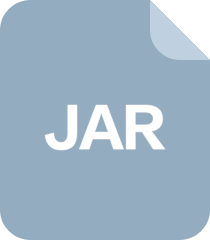
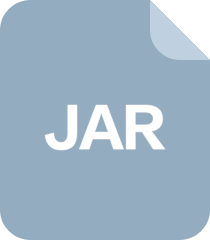
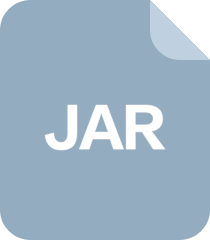
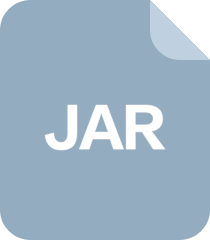
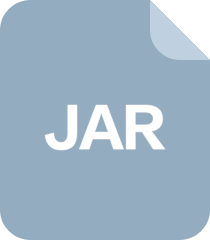
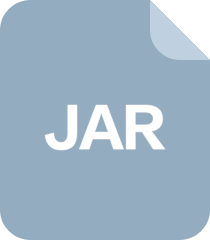
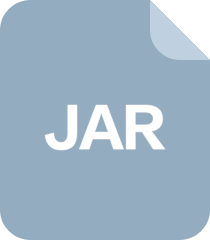
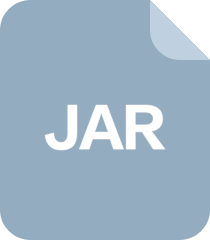
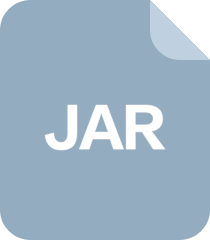
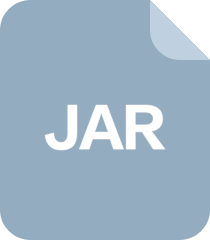
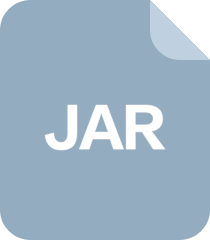
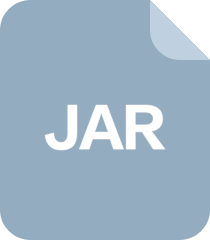
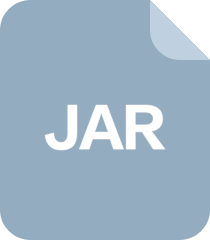
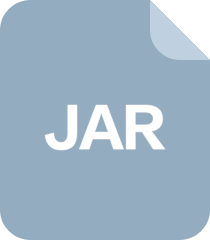
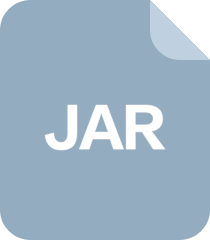
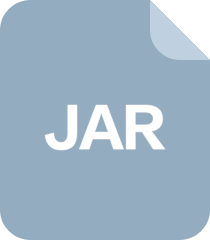
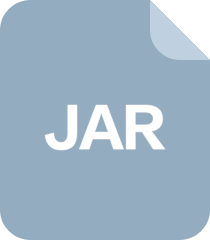
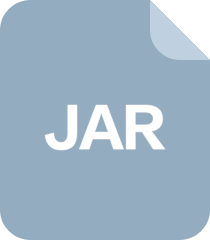
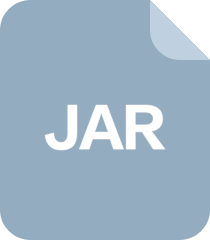
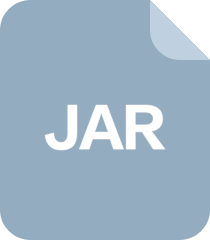
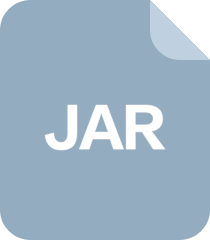
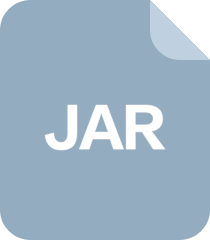
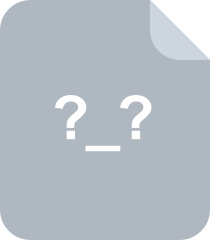
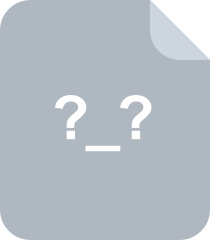
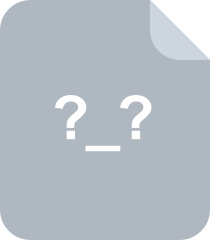
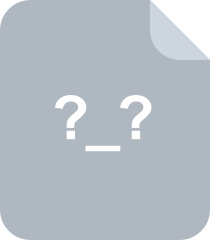
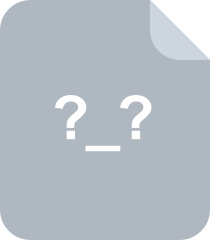
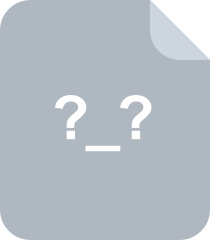
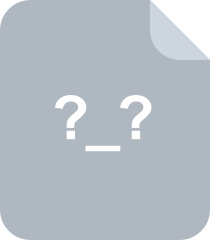
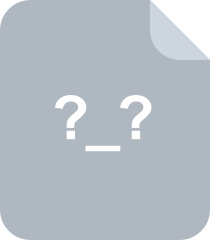
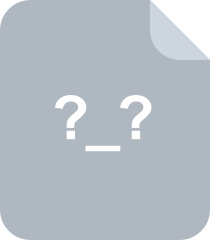
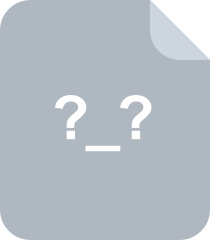
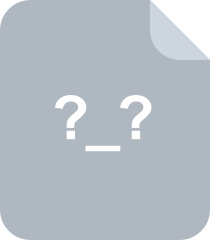
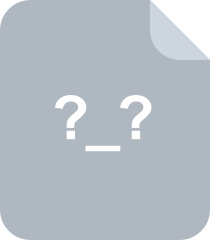
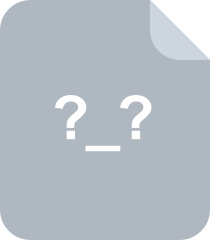
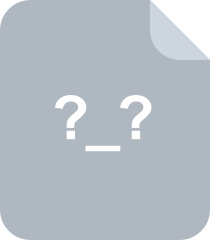
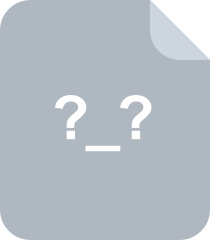
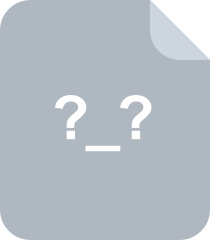
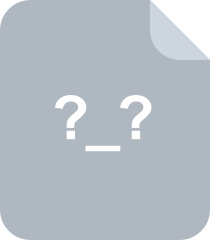
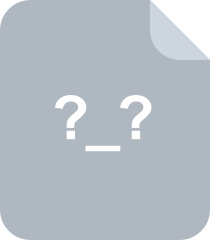
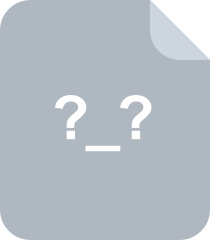
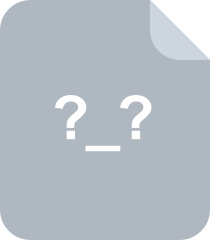
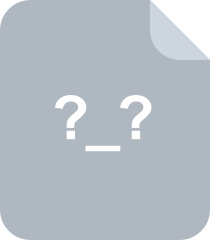
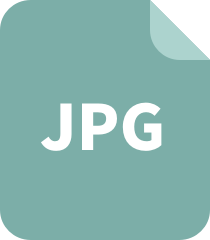
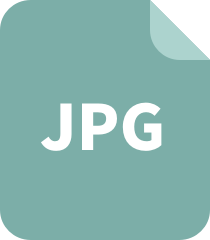
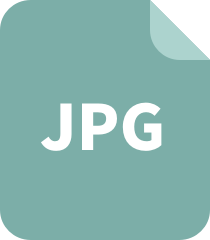
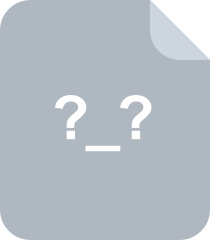
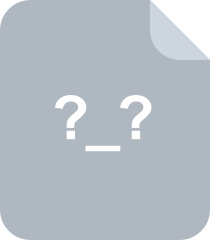
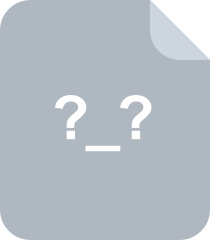
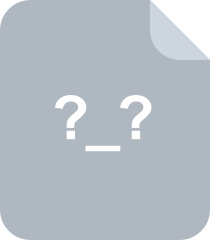
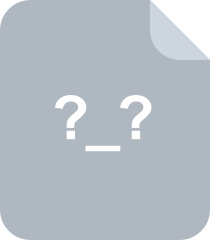
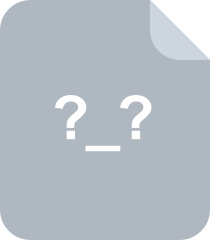
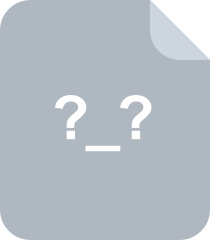
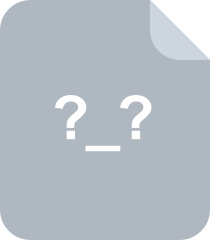
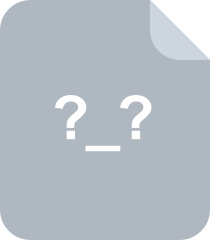
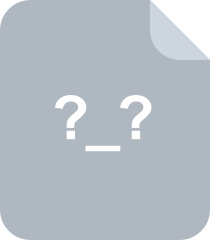
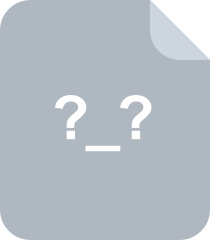
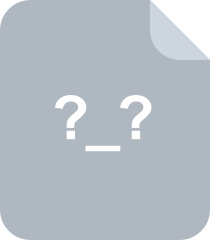
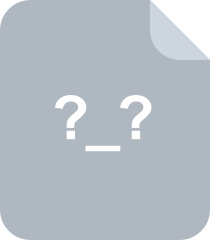
共 139 条
- 1
- 2
资源评论
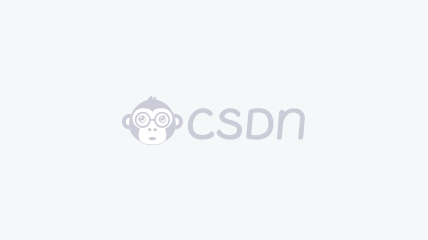

yimeixiaolangzai
- 粉丝: 1723
- 资源: 1130
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

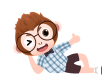
最新资源
- 2021新消费品牌数字化报告.pdf
- 2021中国新锐品牌发展研究:食品饮料行业报告.pdf
- 2021新消费后浪黑马品牌发展洞察-易观.pdf
- 解决 “今天中午吃什么” 的超实用帮手来袭
- 2021中国自主品牌汽车市场研究报告.pdf
- 2022抖音电商新品牌成长报告.pdf
- 华润线下门店引流实操案例复盘.pdf
- 国产彩妆品牌社媒营销案例研究.pdf
- 国货彩妆品牌Q1社媒营销投放分析报告.pdf
- 瑞幸私域案例拆解.pdf
- 品牌私域流量体系运营sop.xlsx
- 奈雪的茶私域案例拆解.pdf
- 新品牌层出不穷,咖啡品牌当如何以消费者为核心,破局而出?202111.pdf
- 瑞幸咖啡企业微信群话术及人设搭建SOP.xlsx
- 完美日记企业微信群发售话术及人设搭建SOP.xlsx
- 企业微信最全养号、防封、加人机制.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


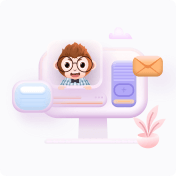
安全验证
文档复制为VIP权益,开通VIP直接复制
