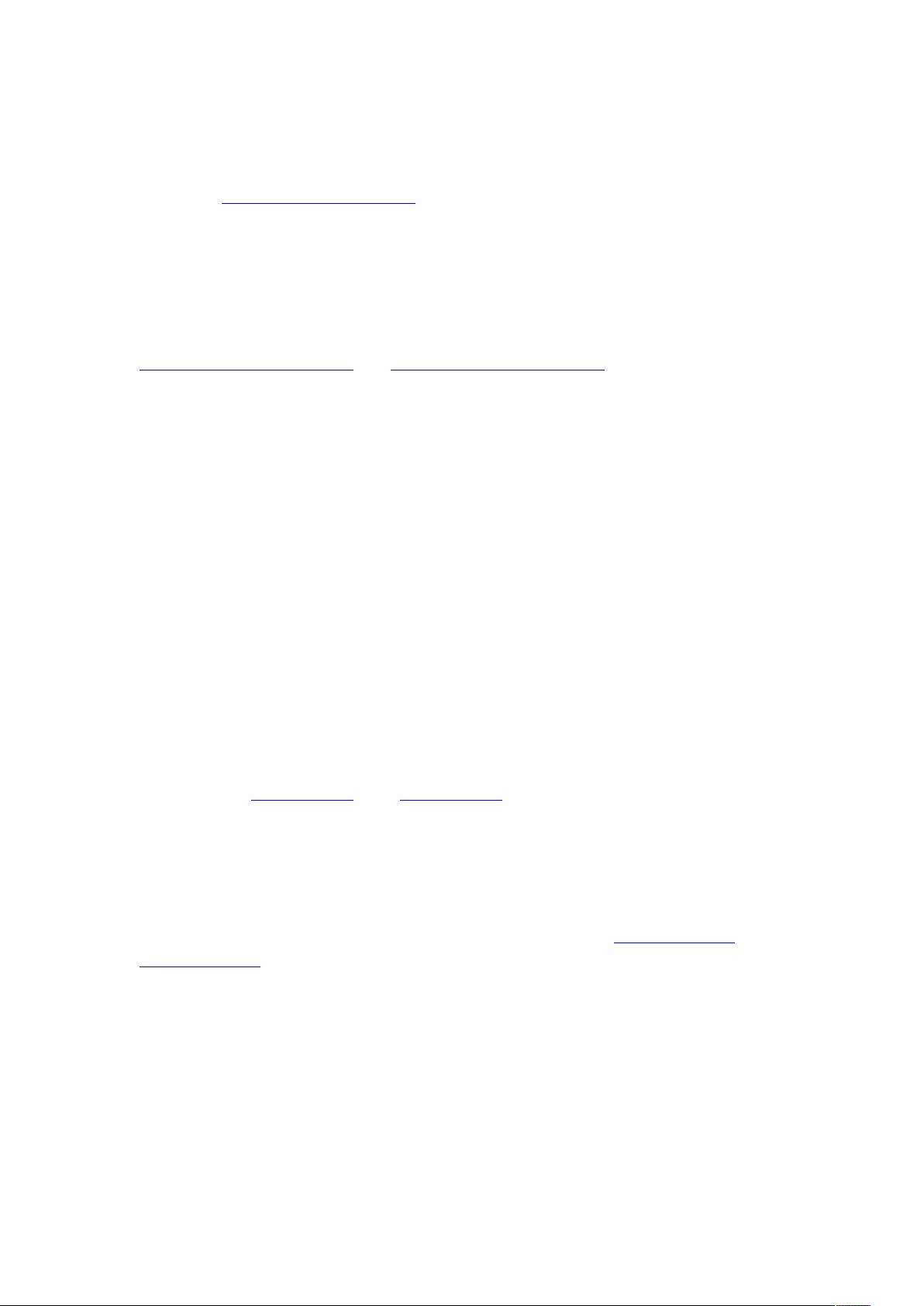
Binding Parameters
To avoid SQL injection attacks and to improve performance of executing
repeatedly used SQL statements, one can "prepare" an SQL statement with
optional parameter placeholders that are to be replaced with the actual
parameters during the parameter binding process.
The parameter placeholders can be either named (represented as unique
tokens) or unnamed (represented as question marks). Call
CDbCommand::bindParam() or CDbCommand::bindValue() to replace these
placeholders with the actual parameters. The parameters do not need to
be quoted: the underlying database driver does it for you. Parameter
binding must be done before the SQL statement is executed.
// an SQL with two placeholders ":username" and ":email"
$sql="INSERT INTO users(username, email) VALUES(:username,:email)";
$command=$connection->createCommand($sql);
// replace the placeholder ":username" with the actual username value
$command->bindParam(":username",$username,PDO::PARAM_STR);
// replace the placeholder ":email" with the actual email value
$command->bindParam(":email",$email,PDO::PARAM_STR);
$command->execute();
// insert another row with a new set of parameters
$command->bindParam(":username",$username2,PDO::PARAM_STR);
$command->bindParam(":email",$email2,PDO::PARAM_STR);
$command->execute();
The methods bindParam() and bindValue() are very similar. The only
difference is that the former binds a parameter with a PHP variable
reference while the latter with a value. For parameters that represent
large block of data memory, the former is preferred for performance
consideration.
For more details about binding parameters, see the relevant PHP
documentation.
Binding Columns
When fetching query results, one can also bind columns with PHP variables
so that they are automatically populated with the latest data each time
a row is fetched.
$sql="SELECT username, email FROM users";
评论0
最新资源