/*********************************************************************
*
* Zigbee MAC layer
*
*********************************************************************
* FileName: zMAC.c
* Dependencies:
* Processor: PIC18F
* Complier: MCC18 v2.30 or higher
* HITECH PICC-18 V8.10PL1 or higher
* Company: Microchip Technology, Inc.
*
* Software License Agreement
*
* The software supplied herewith by Microchip Technology Incorporated
* (the Company) for its PICmicro® Microcontroller is intended and
* supplied to you, the Companys customer, for use solely and
* exclusively on Microchip PICmicro Microcontroller products. The
* software is owned by the Company and/or its supplier, and is
* protected under applicable copyright laws. All rights are reserved.
* Any use in violation of the foregoing restrictions may subject the
* user to criminal sanctions under applicable laws, as well as to
* civil liability for the breach of the terms and conditions of this
* license.
*
* THIS SOFTWARE IS PROVIDED IN AN AS IS CONDITION. NO WARRANTIES,
* WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED
* TO, IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A
* PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE COMPANY SHALL NOT,
* IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR
* CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER.
*
*
* Author Date Comment
*~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
* Nilesh Rajbharti 7/12/04 Rel 0.9
* Nilesh Rajbharti 11/1/04 Pre-release version
* David Flowers 3/10/05 add MLME_DISASSOCIATE_request
* DF/KO 04/29/05 Microchip ZigBee Stack v1.0-2.0
* DF/KO 07/18/05 Microchip ZigBee Stack v1.0-3.0
* DF/KO 07/27/05 Microchip ZigBee Stack v1.0-3.1
* DF/KO 08/19/05 Microchip ZigBee Stack v1.0-3.2
* DF/KO 09/08/05 Microchip ZigBee Stack v1.0-3.3
********************************************************************/
// Uncomment ENABLE_DEBUG line to enable debug mode for this file.
// Or you may also globally enable debug by defining this macro
// in zigbee.def file or from compiler command-line.
#ifndef ENABLE_DEBUG
//#define ENABLE_DEBUG
#endif
#include <string.h>
#include "Compiler.h"
#include "zigbee.h"
#include "Tick.h"
#include "Debug.h"
#include "zMAC.h"
#include "zPHY.h"
#include "sralloc.h"
#include "NeighborTable.h"
#define RANDOM_LSB (TMR0L)
#include "zNWK.h"
void MACBufferPacket(void);
// Association State Machine states.
typedef enum _SM_ASSOCIATION
{
SM_SEND_ASSOCIATE_REQ,
SM_WAIT_FOR_TX_COMPLETE,
SM_WAIT_FOR_ACK,
SM_SEND_DATA_REQ,
SM_DATA_REQ_ACK_WAIT,
SM_WAIT_FOR_ASSOC_RESP,
SM_SEND_DISASSOCIATE_REQ,
SM_SEND_ORPHAN_NOTICE,
SM_WAIT_FOR_COORD_ALIGNMENT
} SM_ASSOCIATION;
#if defined(I_AM_END_DEVICE) || defined(I_AM_ROUTER)
static SM_ASSOCIATION smAssociation;
// Running count of transmit retries used to association/disassociation
BYTE macFrameRetryCount;
#endif
// MAC Module state info.
MAC_STATE macState;
// Potential coordinator description - used when Associate process is started.
PAN_DESC PANDesc;
// Number of coordinators found operating in radius.
BYTE PANDescCount;
// Current channel of operation.
BYTE macCurrentChannel;
// Running counter of MAC frame DSN.
BYTE macDSN;
BYTE lastMACDSN;
// Length of transmit packet that is being current loaded.
BYTE macPacketLen;
// MAC address info.
NODE_INFO macInfo;
// Coordinator address info.
NODE_INFO macCoordInfo;
// A temp NODE_INFO to store out going commands from the MAC layer
NODE_INFO macDestinationNode;
// RF channel energy as of last energy scan.
BYTE macCurrentEnergy;
// Current MAC frame header.
MAC_HEADER macCurrentFrame;
BYTE macCurrentHeaderLength;
// Array of recently transmitted frames that are yet to be acknowledged
// by remote nodes.
MAC_FRAME_STATUS macFrameStatusQ[MAX_MAC_FRAME_STATUS];
BYTE macFrameStatusQLen;
// Tick value used to calculate timeout conditions.
TICK macStartTick;
// Handle to a frame - used by many command state machines
HFRAME hFrame;
BOOL retransmitPacket;
RX_BUFFER RXBuffer[RX_BUFFER_SIZE];
BYTE currentBuffer;
extern SHORT_ADDR BroadcastSrcAddress;
// This is the frame that we will be operating on next call to MACTask().
// This was done to reduce total time taken by MACTask().
// As per the logic, MACTask() needs to go through all of frames
// in queue and do timeout and retry operations. To reduce total time
// taken by MACTask(), the logic is modified to operate on one frame
// at a time.
// This variable keepds track of frame that needs to be operated
// on next call.
BYTE currentQueueItem;
// Only coordinator will process orphan notice.
#if defined(I_AM_COORDINATOR) || defined(I_AM_ROUTER)
// A orphan notice is said to be valid if there is no data bytes in addition to
// the command identifier itself.
#define MACProcessOrphanNotice() (macCurrentFrame.frameLength == 0)
#endif
#if defined(MAC_USE_TX_INDIRECT_BUFFER)
// MAC_USE_TX_INDIRECT_BUFFER is used by coordinator only to hold outgoing (indirect) frames until
// remote end device request it.
#define TX_INDIRECT_BUFFERS (40)
#define INVALID_INDIRECT_BUFFER (0xFF)
typedef struct
{
unsigned char *pBuffer; // Pointer to dynamically allocated TX buffer
struct // Flags about current frame.
{
unsigned int hFrame:7;
unsigned int bIsSent:1;
unsigned int bIsInUse:1;
unsigned int bIsBroadcast:1;
} Flags;
BYTE frameDSN; // DSN assigned to this frame.
SHORT_ADDR destShortAddr; // Destination that it is addressed to.
TICK lastTick; // Tick to calculate timeout condition.
BYTE headerLength;
} TX_INDIRECT_BUFFER;
#if defined(MCHP_C18)
// Array to hold individual tx indirect buffer entries.
#pragma udata MultiBankTxBuffers=0x100
TX_INDIRECT_BUFFER txIndirectBuffer[TX_INDIRECT_BUFFERS];
#pragma udata
#else
TX_INDIRECT_BUFFER txIndirectBuffer[TX_INDIRECT_BUFFERS];
#endif
// Private table to keep track of the order in which the
// txIndirectBuffer[] array is filled (and needs to be
// retransmitted)
BYTE OrderingTable[TX_INDIRECT_BUFFERS];
// Private pointer to access current tx indirect buffer.
BYTE currentTxIndirectBuffer;
BYTE *pCurrentTxIndirectData;
static void CompressOrderingTable(void);
static void ProcessTxIndirectBuffer(void);
static BOOL MACProcessDataReq(void);
static void _MACIndirectTransmit(TX_INDIRECT_BUFFER *pIndirectTxBuffer, BOOL markAsSent);
#endif
// When tx indirect buffer is used, tx data can be placed in indirect buffer
// as required by higher level.
#if defined(MAC_USE_TX_INDIRECT_BUFFER)
#define PHYClearTxFIFO() \
if ( !macState.bits.bUseTxRAM ) \
{ \
while (!PHYIsIdle()) \
{ \
CLRWDT(); \
} \
PHYBegin(); \
PHYFlushTx(); \

yelangchnl
- 粉丝: 0
- 资源: 6
最新资源
- 基于PyTorch的声音信号识别:Mel特征提取与训练集验证集构建技术实现(含代码及解释)
- 永磁同步电机模型预测控制,电流预测控制,单矢量双矢量三矢量模型预测控制,pi控制,foc控制,转矩控制
- springboot+vue+redis前后端分离网上商城项目003(源码+sql)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 毕业设计-嵌入式智能宠物小屋,自动喂食等-主要是基于STM32的多个传感器上报信息,使用阿里云进行远距离操控以及监视,然后控制继电器实现全自动照顾宠物屋的小项目
- springboot美食菜谱分享平台优化版(源码+sql+论文报告)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot婚纱摄影系统(源码+sql)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot SSM 宠物医院管理系统(源码+论文)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- jiffyReader谷歌插件
- 基于WordPress开发的高颜值cms主题,支持白天与黑夜模式v2.8.2
- springboot+redis水果超市商城系统(源码+sql+论文报告)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot博客论坛系统(源码+数据库+设计报告)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- Java定制前后端分离学生信息管理系统(spring boot+vue)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- Java springboot+vue疫情防疫管理系统系统(源码+完整论文以及各种报告)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot 学生成绩请假信息管理系统002(源码+sql)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot 酒庄内部管理系统(源码+sql+论文)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- springboot+layui仓库管理系统(源码+sql)-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


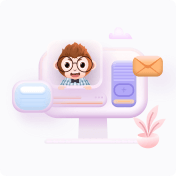
- 1
- 2
- 3
前往页