在Android平台上进行Bluetooth Low Energy(BLE)开发,你需要掌握一系列的关键步骤和技术。以下是一份详细的Android BLE开发指南,包括权限管理、蓝牙初始化、设备搜索以及数据传输等方面。 1. **BLE权限**: 在Android应用中使用BLE功能,首先需要在`AndroidManifest.xml`文件中声明必要的权限。至少需要以下两个权限: ```xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> ``` 如果你想确保你的应用只在支持BLE的设备上运行,还需添加: ```xml <uses-feature android:name="android.hardware.bluetooth_le" android:required="true"/> ``` 2. **蓝牙初始化**: 在开始BLE操作之前,需要检查设备是否支持BLE,并初始化蓝牙适配器。通过`PackageManager`的`hasSystemFeature()`方法来检查BLE功能: ```java if (!getPackageManager().hasSystemFeature(PackageManager.FEATURE_BLUETOOTH_LE)) { // BLE不被支持,显示错误信息并结束活动 } ``` 接下来,通过`Context`获取`BluetoothManager`,然后获取`BluetoothAdapter`实例: ```java BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE); BluetoothAdapter mBluetoothAdapter = bluetoothManager.getAdapter(); ``` 确保蓝牙已开启,否则启动一个请求用户开启蓝牙的意图: ```java if (mBluetoothAdapter == null || !mBluetoothAdapter.isEnabled()) { Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT); } ``` 3. **搜索BLE设备**: 要扫描BLE设备,使用`BluetoothAdapter`的`startLeScan()`方法,同时提供一个`LeScanCallback`用于接收扫描结果。当找到目标设备后,应停止扫描以节省电量: ```java private BluetoothAdapter.LeScanCallback mLeScanCallback = new BluetoothAdapter.LeScanCallback() { @Override public void onLeScan(BluetoothDevice device, int rssi, byte[] scanRecord) { // 处理发现的设备 } }; private void scanLeDevice(boolean enable) { if (enable) { mHandler.postDelayed(new Runnable() { @Override public void run() { mScanning = false; mBluetoothAdapter.stopLeScan(mLeScanCallback); } }, SCAN_PERIOD); // 停止扫描的时间,如10秒 mScanning = true; mBluetoothAdapter.startLeScan(mLeScanCallback); } else { mScanning = false; mBluetoothAdapter.stopLeScan(mLeScanCallback); } } ``` 4. **连接BLE设备**: 扫描到设备后,选择一个设备并建立连接。使用`BluetoothGatt`类来代表与BLE设备的连接,并调用`connectGatt()`方法: ```java BluetoothDevice device = ... // 选择的设备 BluetoothGatt mBluetoothGatt = device.connectGatt(this, false, mGattCallback); ``` 提供一个`BluetoothGattCallback`实例以处理连接状态变化、服务发现和数据交换等事件。 5. **服务和特征的发现**: 连接成功后,需要发现BLE设备提供的服务和特征。调用`BluetoothGatt`的`discoverServices()`方法: ```java if (mBluetoothGatt != null) { mBluetoothGatt.discoverServices(); } ``` 在`BluetoothGattCallback`中,监听`onServicesDiscovered()`回调来处理服务发现的结果。 6. **读取和写入数据**: 一旦服务和特征被发现,可以使用`BluetoothGattService`和`BluetoothGattCharacteristic`来读取或写入数据。例如,调用`readCharacteristic()`或`writeCharacteristic()`方法: ```java BluetoothGattCharacteristic characteristic = ... // 选择的特征 boolean success = mBluetoothGatt.readCharacteristic(characteristic); if (success) { // 读取操作成功,等待onCharacteristicRead()回调 } // 或者写入数据 characteristic.setValue(...); // 设置要写入的数据 success = mBluetoothGatt.writeCharacteristic(characteristic); if (success) { // 写入操作成功,等待onCharacteristicWrite()回调 } ``` 7. **通知和指示**: 对于需要实时数据更新的特征,可以设置通知或指示。这通常涉及在特征上调用`setNotification()`或`setIndication()`,并在`BluetoothGattCallback`的`onCharacteristicChanged()`回调中处理数据。 8. **处理连接问题**: 要处理可能的连接丢失或断开,你需要在`BluetoothGattCallback`的`onConnectionStateChange()`回调中检查新的连接状态。如果连接断开,可能需要重新扫描并尝试重新连接。 9. **释放资源**: 当应用不再需要与BLE设备交互时,记得释放资源。调用`BluetoothGatt`的`disconnect()`和`close()`方法来断开连接并关闭Gatt对象: ```java if (mBluetoothGatt != null) { mBluetoothGatt.disconnect(); mBluetoothGatt.close(); mBluetoothGatt = null; } ``` 以上就是Android BLE开发的基本流程。理解这些概念和步骤是开发BLE应用的关键。在实际项目中,可能还需要处理更多的细节,如错误处理、数据解析、UI同步等。同时,由于Android版本和设备之间的差异,可能需要针对特定设备进行兼容性调整。
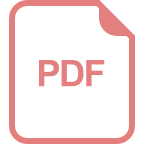
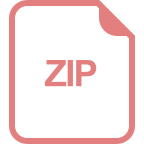
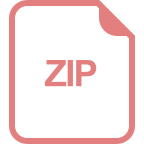
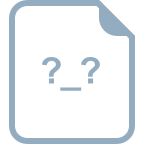
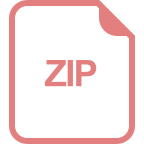
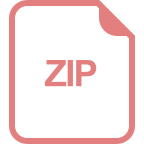
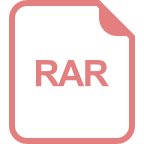
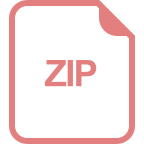
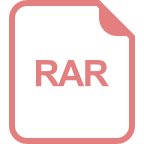
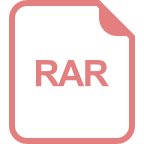
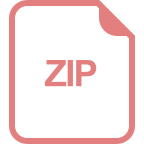
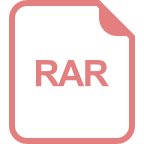
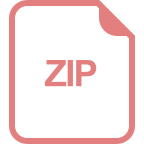
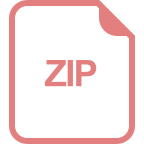
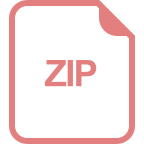
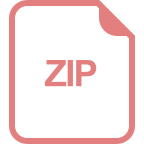
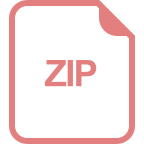
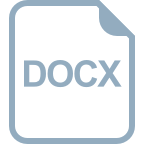
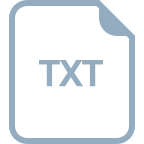
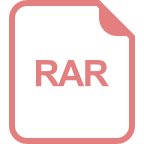
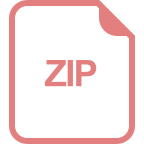
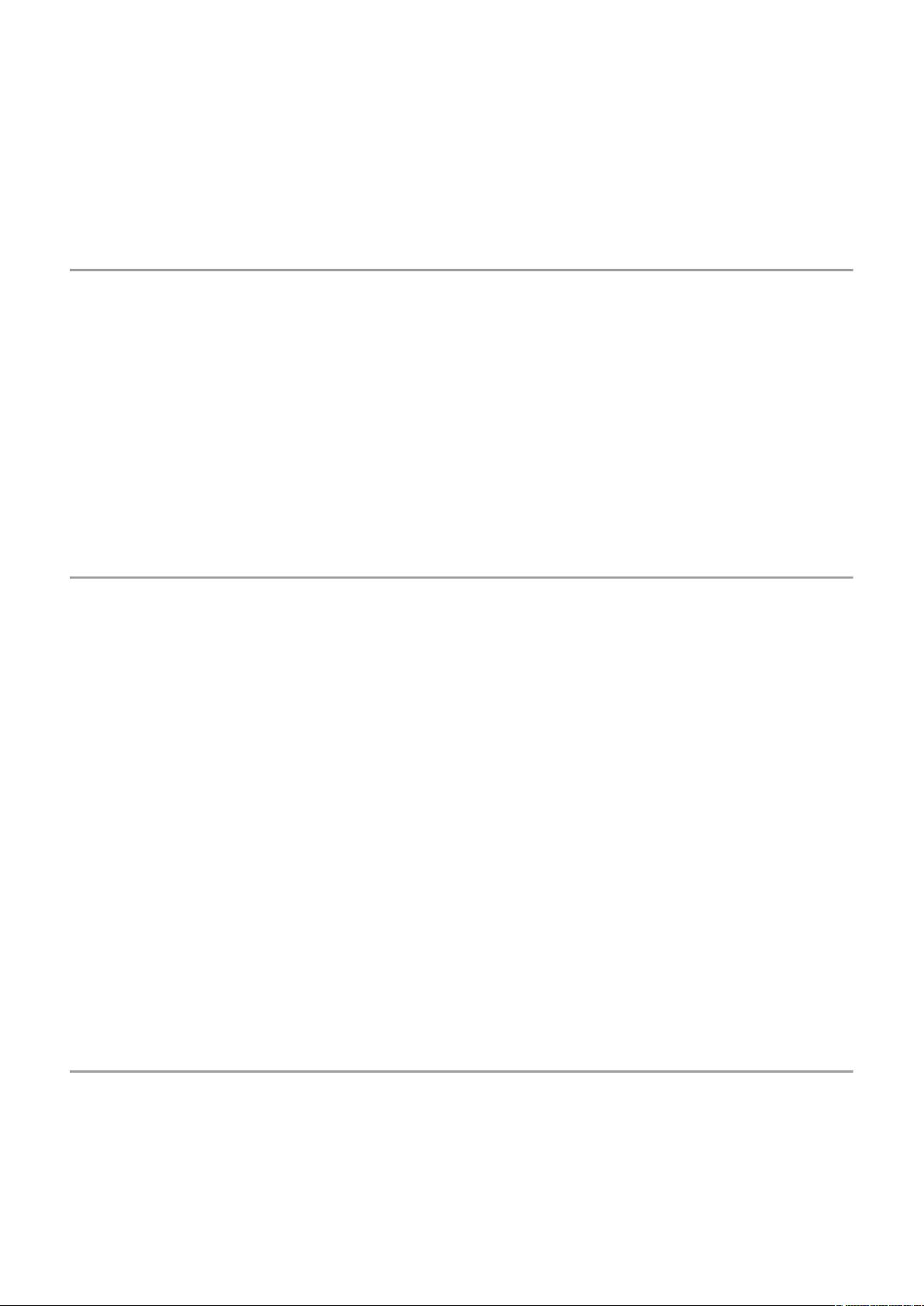
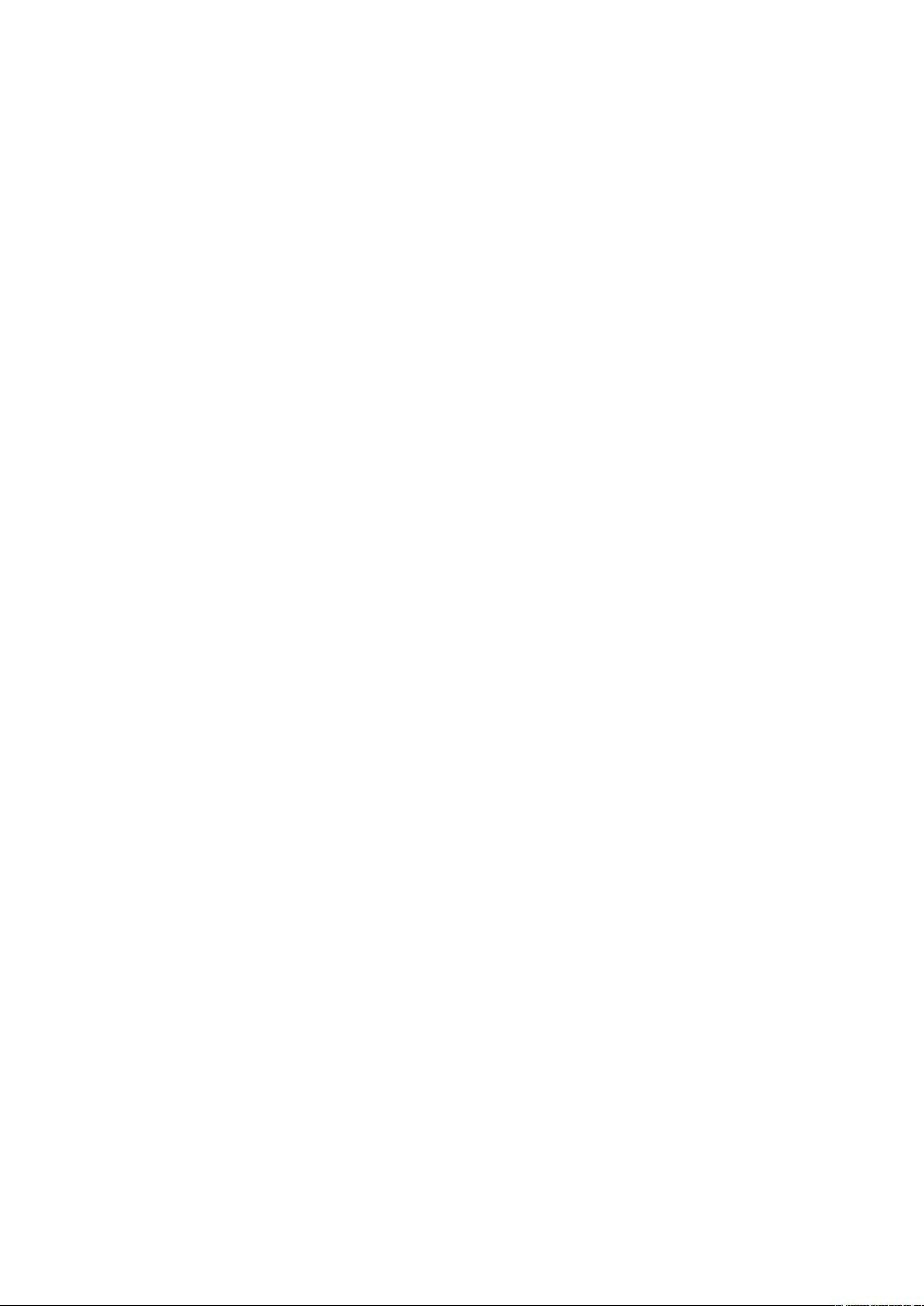
剩余7页未读,继续阅读
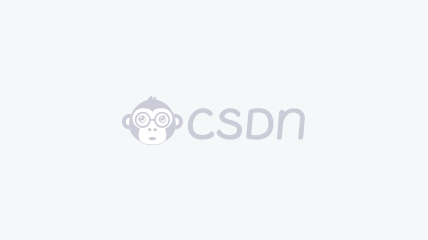

- 粉丝: 0
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

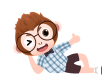
最新资源

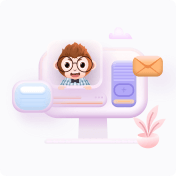
