**Android BLE 聊天案例:BLE服务端详解** Bluetooth Low Energy(BLE),也被称为蓝牙智能,是一种低功耗的无线通信技术,广泛应用于移动设备、物联网设备间的短距离通信。在Android平台上,开发BLE应用涉及到对Android Bluetooth API的深入理解和使用。本案例将详细介绍如何构建一个基于BLE的服务端,实现与客户端的聊天功能。 ### 1. Android BLE API Android系统提供了`BluetoothGatt`和`BluetoothGattServer`接口来支持BLE通信。`BluetoothGatt`用于客户端连接到BLE设备,而`BluetoothGattServer`则用于创建并管理BLE服务端。 ### 2. 创建BLE服务 BLE服务由一系列特征值(Characteristics)组成,每个特征值都有一个唯一的UUID。服务端需要定义服务和特征值,然后通过`BluetoothGattServer`接口的`addService`方法将它们添加到服务器中。 ```java BluetoothGattService service = new BluetoothGattService(SERVICE_UUID, BluetoothGattService.SERVICE_TYPE_PRIMARY); BluetoothGattCharacteristic characteristic = new BluetoothGattCharacteristic(CHARACTERISTIC_UUID, BluetoothGattCharacteristic.PROPERTY_WRITE_NO_RESPONSE | BluetoothGattCharacteristic.PROPERTY_NOTIFY, BluetoothGattCharacteristic.PERMISSION_WRITE | BluetoothGattCharacteristic.PERMISSION_READ); service.addCharacteristic(characteristic); bluetoothGattServer.addService(service); ``` ### 3. 特征值的读写处理 服务端需要监听特征值的读写请求,并做出响应。`BluetoothGattServerCallback`回调中包含了处理这些请求的方法: ```java @Override public void onCharacteristicReadRequest(BluetoothDevice device, int requestId, int offset, BluetoothGattCharacteristic characteristic) { // 处理读请求 } @Override public void onCharacteristicWriteRequest(BluetoothDevice device, int requestId, BluetoothGattCharacteristic characteristic, boolean preparedWrite, boolean responseNeeded, int offset, byte[] value) { // 处理写请求 } ``` ### 4. 通知和指示 BLE服务端可以向客户端发送数据,通过设置特征值的`PROPERTY_NOTIFY`或`PROPERTY_INDICATE`属性。当客户端订阅了特征值的更新时,服务端可以通过`BluetoothGattCharacteristic.setNotificationValue`开启通知,并调用`BluetoothGattServer.notifyCharacteristicChanged`发送数据。 ```java characteristic.setValue(data); boolean success = bluetoothGattServer.notifyCharacteristicChanged(device, characteristic, true); ``` ### 5. 连接和断开管理 服务端需要监听客户端的连接和断开事件,以便进行资源管理和错误处理。`BluetoothGattServerCallback`提供了`onConnectionStateChange`方法来处理这些事件。 ```java @Override public void onConnectionStateChange(BluetoothDevice device, int status, int newState) { if (newState == BluetoothGatt.GATT_CONNECTED) { // 客户端已连接 } else if (newState == BluetoothGatt.GATT_DISCONNECTED) { // 客户端已断开 } } ``` ### 6. 性能优化和兼容性 由于BLE协议的复杂性和不同Android设备间的差异,服务端需要考虑兼容性问题,例如连接超时、重连策略等。同时,为了提高性能,需要合理设置数据传输的大小和频率,避免因数据量过大或过于频繁导致的连接中断。 ### 7. 安全性考虑 BLE通信的安全性也是一个重要的方面。服务端应确保只有授权的客户端才能连接和访问数据。可以通过加密特征值或使用安全认证机制来增强安全性。 ### 结论 通过以上步骤,我们可以创建一个基本的BLE服务端,实现与客户端的通信。在实际应用中,还需要根据具体需求进行功能扩展,例如添加更多服务、特征值,或者实现更复杂的业务逻辑。理解并熟练运用Android的BLE API是构建高效、稳定且安全的BLE应用的关键。
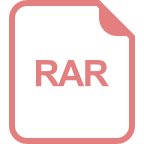
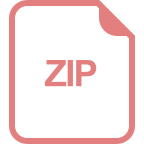
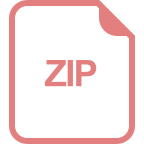
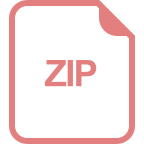
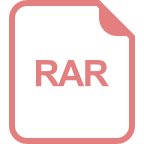
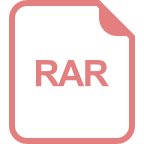
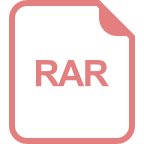
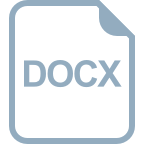
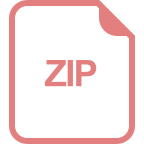
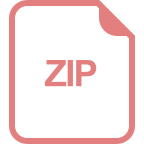
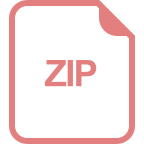
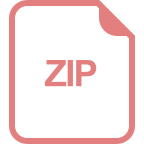
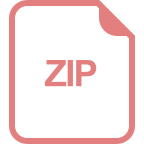
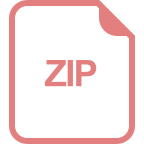
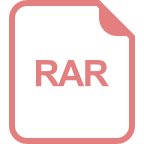
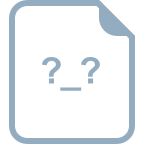
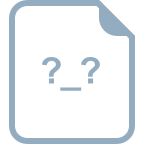
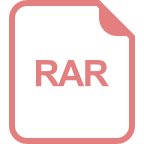
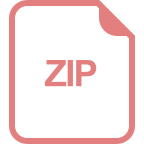
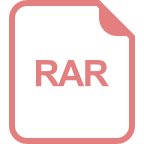
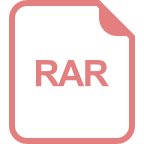

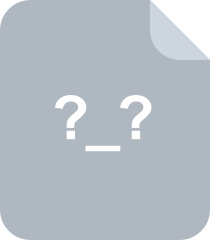
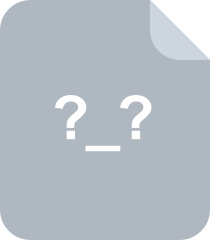
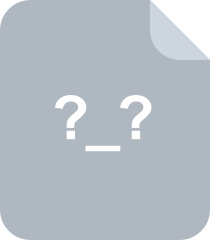
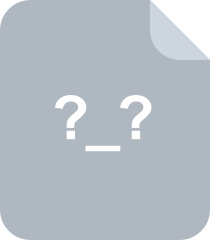
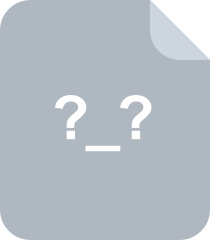
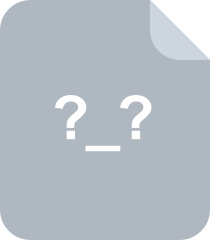
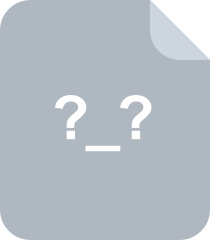
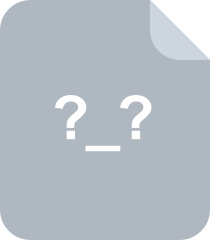
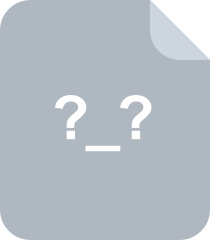
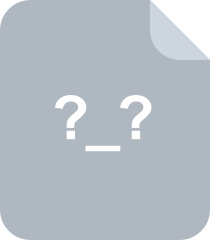
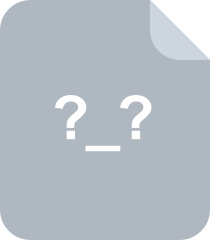
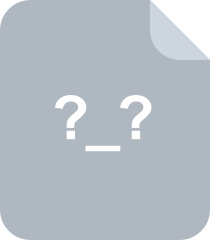
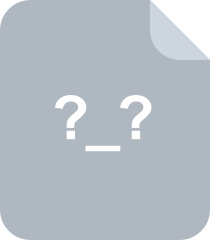
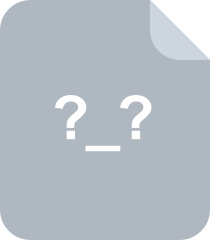
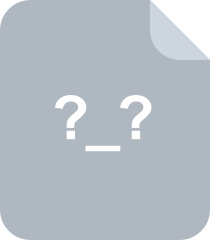
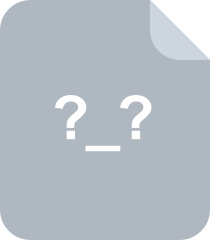
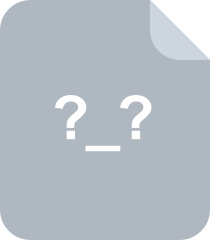
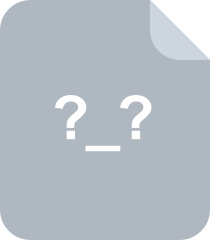
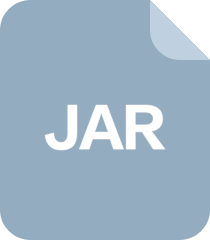
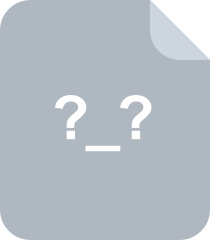
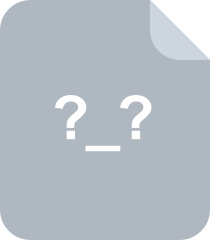
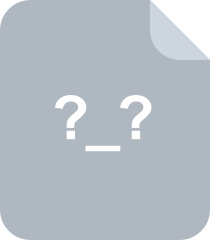
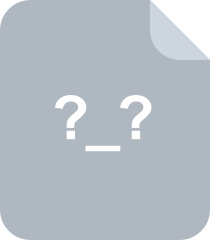
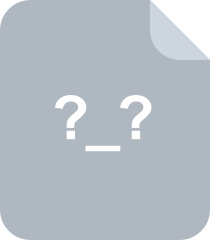
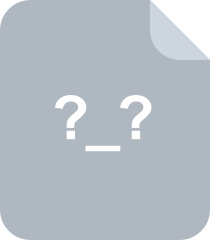
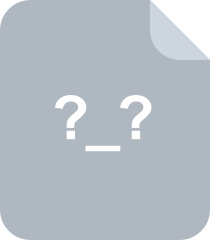
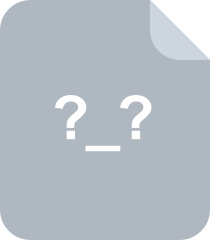
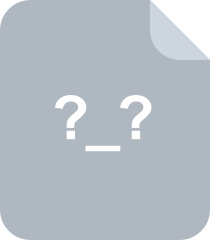
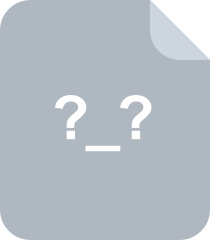
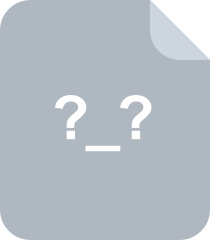
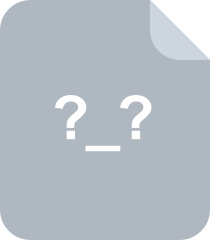
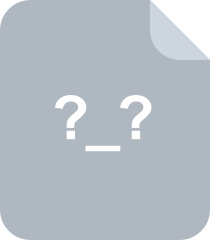
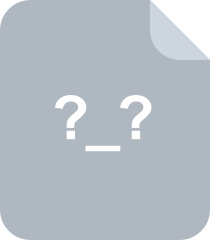
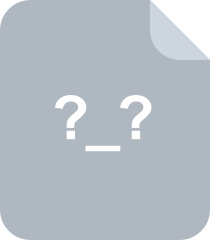
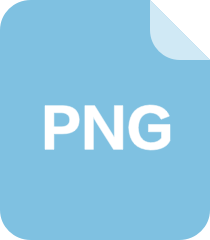
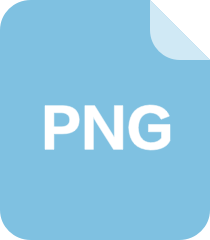
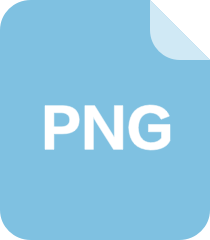
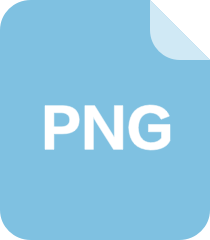
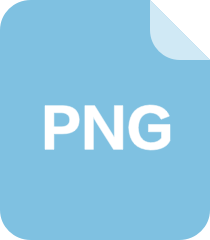
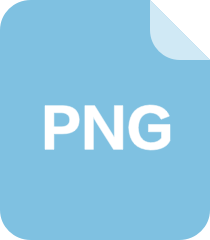
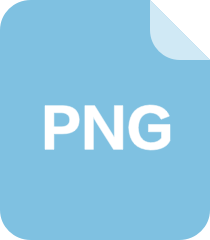
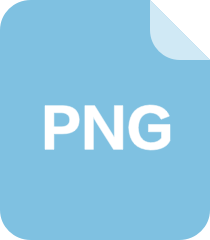
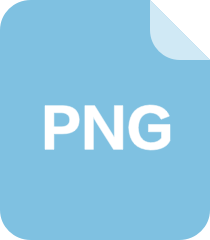
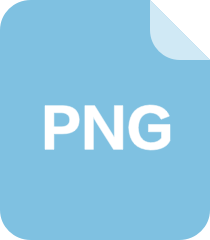
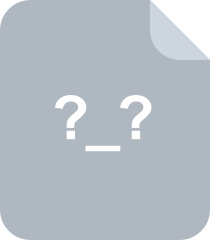
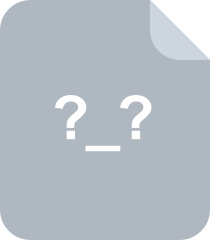
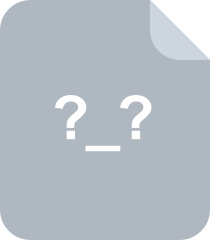
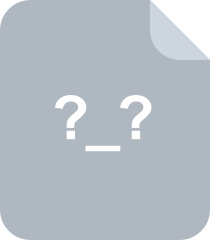
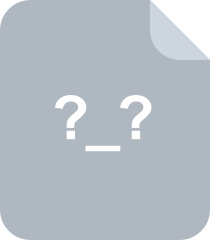
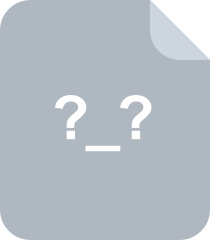
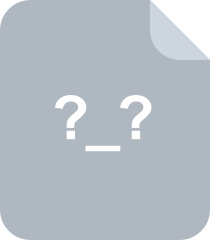
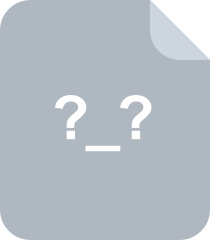
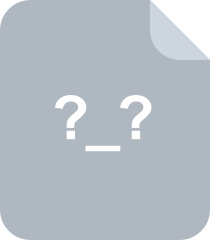
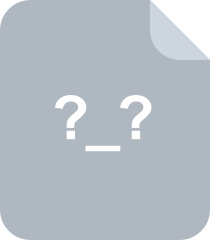
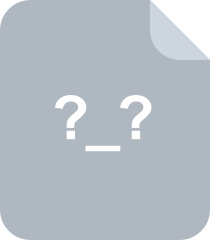
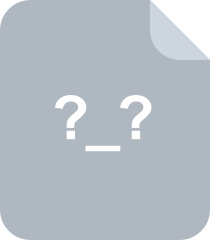
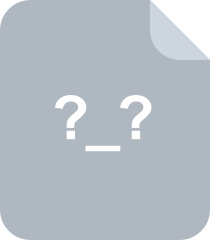
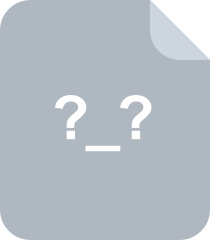
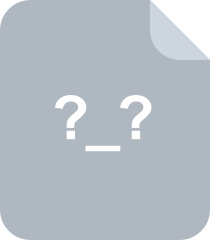
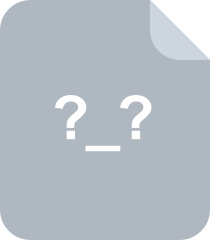
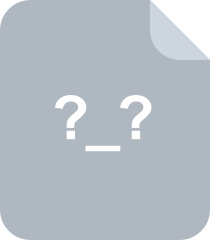
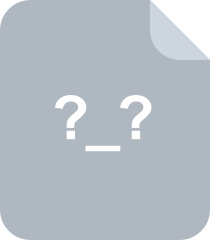
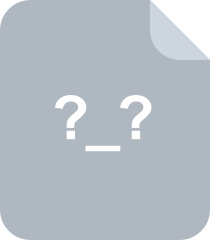
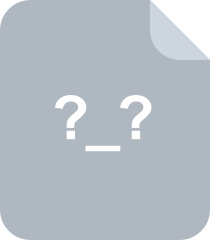
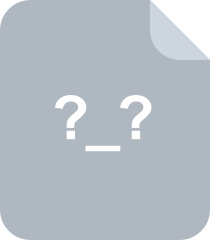
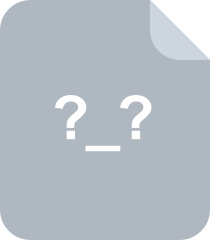
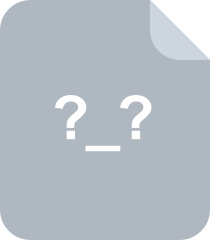
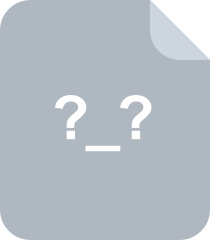
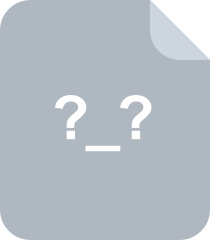
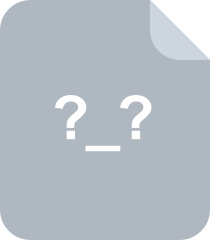
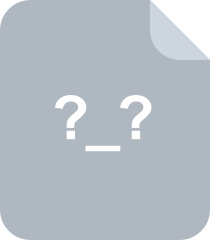
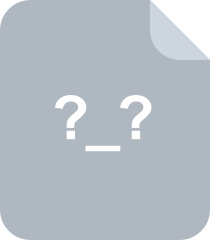
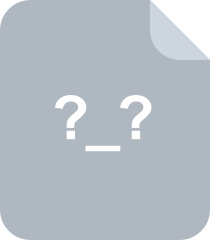
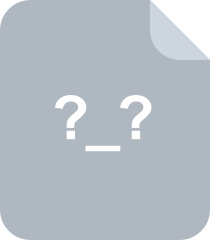
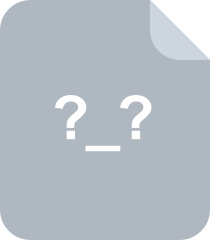
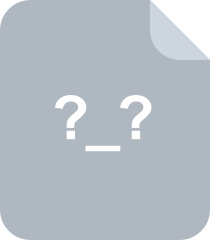
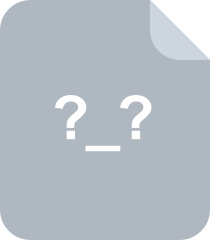
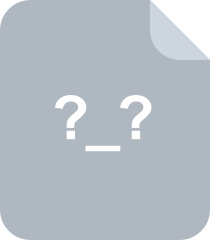
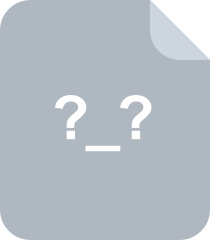
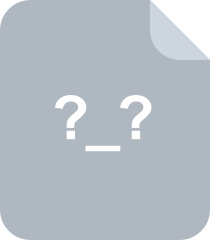
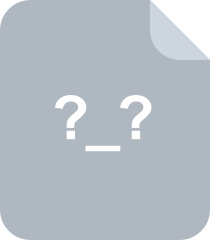
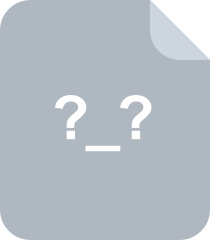
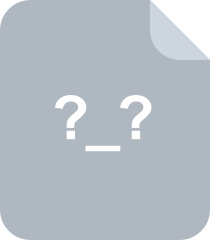
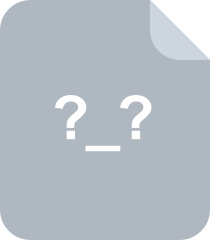
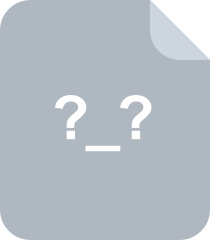
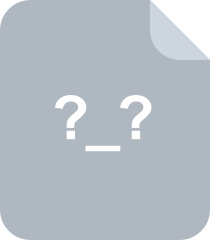
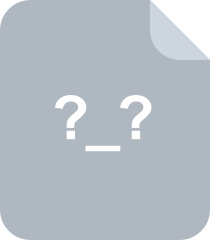
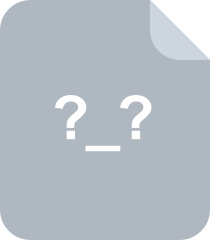
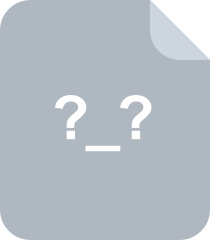
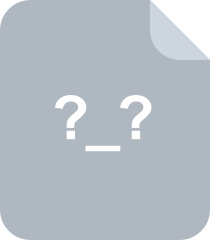
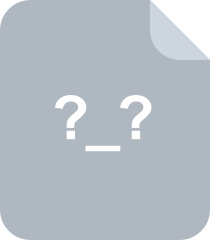
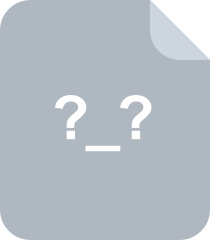
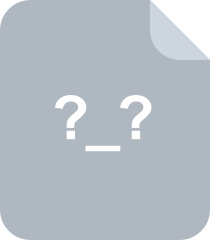
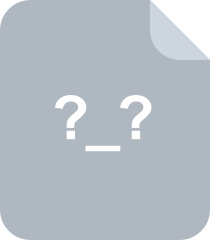
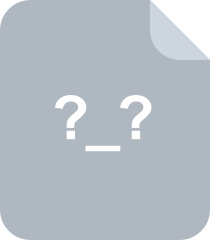
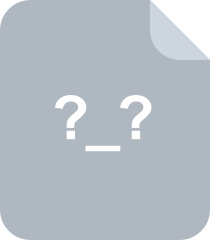
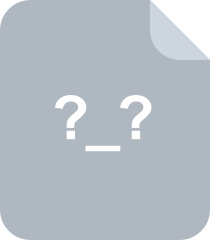
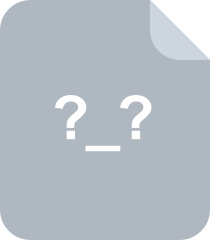
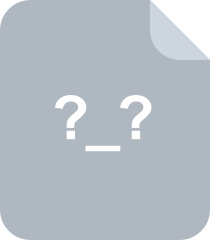
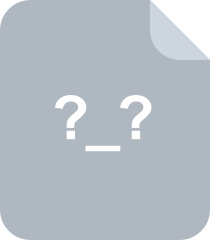
- 1
- 2
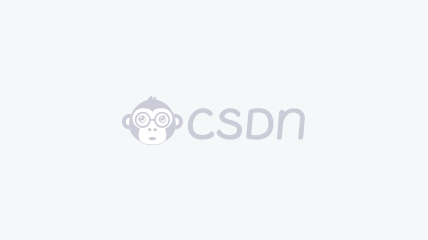
- 小学语文助教2019-10-28好用,五星了
- 白云竹海2019-12-08有学习价值,你值得研究!
- Bruce_zhu_hangzhou2024-06-24好用,是要找的东东

- 粉丝: 1w+
- 资源: 43
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

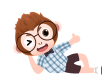
最新资源
- Python爬虫资源:全面掌握爬虫技术的综合指南
- 人工智能-智能助手-凤希AI伴侣:多功能整合,效率提升利器
- 新冠病毒密接者跟踪系统设计与实现(代码+数据库+LW)
- Xmind-for-Windows-x64bit-24.01.13311-畅享版
- XMind2023-v23.11.04336-x64-Repack-畅享版
- XMind2024-mac畅享版
- 基于Spring Boot的夕阳红公寓管理系统的设计与实现(代码+数据库+LW)
- 基于神经音频编解码器的高效语音分离技术研究-Codecformer模型
- 源感知神经音频编解码器SD-Codec的技术与应用
- 导师信息评价-导师评价(包含全国各大高校各个专业).xls
- 源码程序,前端vue,后端php,前后端全开源
- 手边酒店V25.0.22小程序前端+后端.zip
- 基于java的简易版营业厅宽带系统+jsp源码(java毕业设计完整源码+LW).zip
- 11基于java的旅游管理系统设计新版源码+数据库+说明
- mmexport1735574041820.jpg

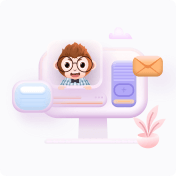
