# -*- coding: utf-8 -*-
"""
Created on Tue Sep 26 16:03:41 2017
@author: 董玮
"""
import argparse
import time
import copy
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.examples.tutorials.mnist import input_data
"""
类型:抽象类
说明:规则化数据接口,一般用于数据预处理中。
"""
class interface_normalize_data(object):
"""
类型:公有成员变量
说明:规则化数据过程中,定义一个无穷小精度,用来防止数据计算中的非法操作。
"""
epsilon = 1e-8
"""
类型:抽象公有成员函数
说明:用来规则化数据。
参数:
data -- 待处理的数据。
返回值:
data -- 处理后的数据。
"""
def normalize_data(self, data):
pass
"""
类型:实体类,继承自抽象类interface_normalize_data
说明:用于中心化数据,使数据中心在坐标原点上。
"""
class mean_normalization(interface_normalize_data):
def normalize_data(self, data):
#计算数据每个维度的期望,并用每一条数据减去期望。
data = data - np.mean(data, axis = 1, keepdims = True)
return data
"""
类型:实体类,继承自抽象类interface_normalize_data
说明:用于中心化数据,并除以方差,使数据中心在坐标原点上,并且使每个维度之间的跨度相似。
"""
class variance_normalization(interface_normalize_data):
def normalize_data(self, data):
data = data - np.mean(data, axis = 1, keepdims = True)
#计算数据每个维度的方差。
variance = np.mean(np.square(data), axis = 1, keepdims = True)
#除以方差并在除数上加上无穷小精度。
data = data / (variance + self.epsilon)
return data
"""
类型:实体类,继承自抽象类interface_normalize_data
说明:用于Z-Score统计,与上述实体类的区别是除以标准差而不是方差。
"""
class zscore_normalization(interface_normalize_data):
def normalize_data(self, data):
data = data - np.mean(data, axis = 1, keepdims = True)
variance = np.mean(np.square(data), axis = 1, keepdims = True)
#除以标准差并在除数上加上无穷小精度。
data = data / np.sqrt(variance + self.epsilon)
return data
"""
类型:抽象类
说明:神经网络初始化参数接口。
"""
class interface_initialize_parameters(object):
"""
类型:公有成员变量
说明:用来定义输入层、隐藏层、输出层每层的神经元个数。
"""
structure = None
"""
类型:公有成员变量
说明:随机种子,用来产生随机数。
"""
seed = 1
"""
类型:抽象公有成员函数
说明:用来初始化参数。
"""
def initialize_parameters(self):
pass
"""
类型:实体类
说明:标准的x-avier参数初始化,继承自抽象类interface_initialize_parameters
"""
class xavier_initialize_parameters(interface_initialize_parameters):
"""
类型:公有成员函数
说明:用来初始化参数。
参数:无
返回值:
parameters -- 返回初始化后的参数。
"""
def initialize_parameters(self):
np.random.seed(self.seed)
parameters = {}
#初始化两类参数,一种是W1、W2、W3……,另一种是b1、b2、b3……。其中数字代表层数。
#W的维度为(当前层神经元数,前一层神经元数)。b的维度为(当前层神经元数,1)。
for l in range(1, len(self.structure)):
parameters["W" + str(l)] = np.random.randn(self.structure[l], self.structure[l-1]) / np.sqrt(self.structure[l-1]/2)
parameters["b" + str(l)] = np.zeros((self.structure[l], 1))
return parameters
"""
类型:实体类
说明:具有batch normalization功能的x-avier参数初始化,继承自抽象类interface_initialize_parameters
"""
class xavier_initialize_parameters_BN(interface_initialize_parameters):
"""
类型:公有成员函数
说明:用来初始化参数。
参数:无
返回值:
parameters -- 返回初始化后的参数。
"""
def initialize_parameters(self):
np.random.seed(self.seed)
parameters = {}
#因batch normalization需要,初始化三类参数,W1、W2、W3……,gamma1、gamma2、gamma3……,beta1、beta2、beta3……。其中数字代表层数。
#W的维度为(当前层神经元数,前一层神经元数)。gamma与beta的维度均为(当前层神经元数,1)。
for l in range(1, len(self.structure)):
parameters["W" + str(l)] = np.random.randn(self.structure[l], self.structure[l-1]) / np.sqrt(self.structure[l-1]/2)
parameters["gamma" + str(l)] = np.ones((self.structure[l], 1))
#parameters["gamma" + str(l)] = np.random.randn(self.structure[l], 1) / np.sqrt(self.structure[l]/2)
parameters["beta" + str(l)] = np.zeros((self.structure[l], 1))
return parameters
"""
类型:抽象类
说明:计算激活函数的值和激活函数的梯度值
"""
class interface_activation(object):
"""
类型:公有成员变量
说明:规则化数据过程中,定义一个无穷小精度,用来防止数据计算中的非法操作。
"""
epsilon = 1e-8
"""
类型:抽象公有成员函数
说明:计算激活函数的值。
"""
def activate_function(self, *arguments):
pass
"""
类型:抽象公有成员函数
说明:计算激活函数的梯度值。
"""
def derivation_activate_function(self, *arguments):
pass
"""
类型:抽象类
说明:计算代价函数的值和代价函数的梯度值
"""
class interface_cost(object):
"""
类型:公有成员变量
说明:规则化数据过程中,定义一个无穷小精度,用来防止数据计算中的非法操作。
"""
epsilon = 1e-8
"""
类型:抽象公有成员函数
说明:计算代价函数并返回代价值。
"""
def cost_function(self, *arguments):
pass
"""
类型:抽象公有成员函数
说明:计算代价函数的梯度并返回梯度值。
"""
def derivation_cost_function(self, *arguments):
pass
"""
类型:具体类
说明:relu激活函数,继承自interface_activation。
"""
class relu(interface_activation):
"""
类型:公有成员函数
说明:计算relu函数的值。
参数:
Z -- 每一层(不包括最后一层)的线性值。
返回值:
A -- 激活值。
"""
def activate_function(self, *arguments):
Z = arguments[0]
A = np.maximum(Z, 0)
return A
"""
类型:公有成员函数
说明:计算relu函数的梯度值。
参数:
dA -- 每一层(不包括最后一层)激活值的梯度值。
返回值:
A -- 线性值的梯度值。
"""
def derivation_activate_function(self, *arguments):
dA = arguments[0]
A = arguments[1]
dZ = dA * np.where(A > 0, 1, 0)
return dZ
"""
类型:具体类
说明:softmax代价函数,继承自interface_activation。
"""
class softmax(interface_cost):
"""
类型:公有成员函数
说明:计算softmax代价函数的代价值与输出层(最后一层)的激活值。
参数�

dongweiweiwei
- 粉丝: 5
- 资源: 4
最新资源
- Linux Shell 特殊符号及其用法详解
- 基于STM32的交流电流测量系统(程序+电路资料全)
- “戏迷导航”:戏剧推广网站的个性化推荐系统
- Laser MFP 133 136 138不加电如何确认电源板还是主板故障
- STM32F030单片机采集ADC值并从串口2打印.zip
- java版socket NIO实现,包含客户端和服务端
- 21数科-苏秀娟-论文初稿.pdf
- STM32F030单片机串口1、串口2配置及数据打印.zip
- STM32F030单片机串口2发送接收.zip
- 探秘 Docker 网络:高效容器通信的关键
- STM32F030单片机控制LED灯.zip
- 基于 PyQt 的弱口令检测工具程序设计与实现
- 证件照提取矫正,能提取各种证件并矫正
- STM32F103+PWM+DMA精准控制输出脉冲的数量和频率 源程序
- 篡改猴插件中很实用的脚本
- stm32+SCD40二氧化碳传感器源程序
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


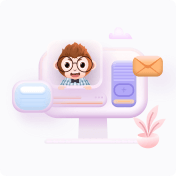