// SPDX-License-Identifier: GPL-2.0
/* Copyright(c) 2013 - 2021 Intel Corporation. */
#ifdef HAVE_XDP_SUPPORT
#include <linux/bpf.h>
#endif
/* Local includes */
#include "i40e.h"
#include "i40e_helper.h"
#include "i40e_diag.h"
#ifdef HAVE_VXLAN_RX_OFFLOAD
#if IS_ENABLED(CONFIG_VXLAN)
#include <net/vxlan.h>
#endif
#endif /* HAVE_VXLAN_RX_OFFLOAD */
#ifdef HAVE_GRE_ENCAP_OFFLOAD
#include <net/gre.h>
#endif /* HAVE_GRE_ENCAP_OFFLOAD */
#ifdef HAVE_GENEVE_RX_OFFLOAD
#if IS_ENABLED(CONFIG_GENEVE)
#include <net/geneve.h>
#endif
#endif /* HAVE_GENEVE_RX_OFFLOAD */
#ifdef HAVE_UDP_ENC_RX_OFFLOAD
#include <net/udp_tunnel.h>
#endif
/* All i40e tracepoints are defined by the include below, which
* must be included exactly once across the whole kernel with
* CREATE_TRACE_POINTS defined
*/
#define CREATE_TRACE_POINTS
#include "i40e_trace.h"
char i40e_driver_name[] = "i40e";
static const char i40e_driver_string[] =
"Intel(R) 40-10 Gigabit Ethernet Connection Network Driver";
#ifndef DRV_VERSION_LOCAL
#define DRV_VERSION_LOCAL
#endif /* DRV_VERSION_LOCAL */
#define DRV_VERSION_DESC ""
#define DRV_VERSION_MAJOR 2
#define DRV_VERSION_MINOR 17
#define DRV_VERSION_BUILD 15
#define DRV_VERSION_SUBBUILD 0
#define DRV_VERSION __stringify(DRV_VERSION_MAJOR) "." \
__stringify(DRV_VERSION_MINOR) "." \
__stringify(DRV_VERSION_BUILD) \
DRV_VERSION_DESC __stringify(DRV_VERSION_LOCAL)
const char i40e_driver_version_str[] = DRV_VERSION;
static const char i40e_copyright[] = "Copyright(c) 2013 - 2021 Intel Corporation.";
/* a bit of forward declarations */
static void i40e_vsi_reinit_locked(struct i40e_vsi *vsi);
static void i40e_handle_reset_warning(struct i40e_pf *pf, bool lock_acquired);
static int i40e_add_vsi(struct i40e_vsi *vsi);
static int i40e_add_veb(struct i40e_veb *veb, struct i40e_vsi *vsi);
static int i40e_setup_pf_switch(struct i40e_pf *pf, bool reinit);
static int i40e_setup_misc_vector(struct i40e_pf *pf);
static void i40e_determine_queue_usage(struct i40e_pf *pf);
static int i40e_setup_pf_filter_control(struct i40e_pf *pf);
static void i40e_clear_rss_config_user(struct i40e_vsi *vsi);
static void i40e_prep_for_reset(struct i40e_pf *pf);
static void i40e_reset_and_rebuild(struct i40e_pf *pf, bool reinit,
bool lock_acquired);
static int i40e_reset(struct i40e_pf *pf);
static void i40e_rebuild(struct i40e_pf *pf, bool reinit, bool lock_acquired);
static int i40e_setup_misc_vector_for_recovery_mode(struct i40e_pf *pf);
static int i40e_restore_interrupt_scheme(struct i40e_pf *pf);
static bool i40e_check_recovery_mode(struct i40e_pf *pf);
static int i40e_init_recovery_mode(struct i40e_pf *pf, struct i40e_hw *hw);
static i40e_status i40e_force_link_state(struct i40e_pf *pf, bool is_up);
static bool i40e_is_total_port_shutdown_enabled(struct i40e_pf *pf);
static void i40e_fdir_sb_setup(struct i40e_pf *pf);
static int i40e_veb_get_bw_info(struct i40e_veb *veb);
static int i40e_get_capabilities(struct i40e_pf *pf,
enum i40e_admin_queue_opc list_type);
/* i40e_pci_tbl - PCI Device ID Table
*
* Last entry must be all 0s
*
* { Vendor ID, Device ID, SubVendor ID, SubDevice ID,
* Class, Class Mask, private data (not used) }
*/
static const struct pci_device_id i40e_pci_tbl[] = {
{PCI_VDEVICE(INTEL, I40E_DEV_ID_SFP_XL710), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_QEMU), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_KX_B), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_KX_C), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_QSFP_A), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_QSFP_B), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_QSFP_C), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_5G_BASE_T_BC), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_BASE_T), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_BASE_T4), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_BASE_T_BC), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_SFP), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_B), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_20G_KR2), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_20G_KR2_A), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_X710_N3000), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_XXV710_N3000), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_KX_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_QSFP_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_SFP_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_1G_BASE_T_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_10G_BASE_T_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_SFP_I_X722), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_25G_B), 0},
{PCI_VDEVICE(INTEL, I40E_DEV_ID_25G_SFP28), 0},
/* required last entry */
{0, }
};
MODULE_DEVICE_TABLE(pci, i40e_pci_tbl);
#define I40E_MAX_VF_COUNT 128
#define OPTION_UNSET -1
#define I40E_PARAM_INIT { [0 ... I40E_MAX_NIC] = OPTION_UNSET}
#define I40E_MAX_NIC 64
#if !defined(HAVE_SRIOV_CONFIGURE) && !defined(HAVE_RHEL6_SRIOV_CONFIGURE)
#ifdef CONFIG_PCI_IOV
static int max_vfs[I40E_MAX_NIC+1] = I40E_PARAM_INIT;
module_param_array_named(max_vfs, max_vfs, int, NULL, 0);
MODULE_PARM_DESC(max_vfs,
"Number of Virtual Functions: 0 = disable (default), 1-"
__stringify(I40E_MAX_VF_COUNT) " = enable "
"this many VFs");
#endif /* CONFIG_PCI_IOV */
#endif /* HAVE_SRIOV_CONFIGURE */
static int debug = -1;
module_param(debug, int, 0);
MODULE_PARM_DESC(debug, "Debug level (0=none,...,16=all)");
static int l4mode = L4_MODE_DISABLED;
module_param(l4mode, int, 0000);
MODULE_PARM_DESC(l4mode, "L4 cloud filter mode: 0=UDP,1=TCP,2=Both,-1=Disabled(default)");
MODULE_AUTHOR("Intel Corporation, <e1000-devel@lists.sourceforge.net>");
MODULE_DESCRIPTION("Intel(R) 40-10 Gigabit Ethernet Connection Network Driver");
MODULE_LICENSE("GPL");
MODULE_VERSION(DRV_VERSION);
static struct workqueue_struct *i40e_wq;
bool i40e_is_l4mode_enabled(void)
{
return l4mode > L4_MODE_DISABLED;
}
/**
* i40e_get_lump - find a lump of free generic resource
* @pf: board private structure
* @pile: the pile of resource to search
* @needed: the number of items needed
* @id: an owner id to stick on the items assigned
*
* Returns the base item index of the lump, or negative for error
**/
static int i40e_get_lump(struct i40e_pf *pf, struct i40e_lump_tracking *pile,
u16 needed, u16 id)
{
int ret = -ENOMEM;
u16 i, j;
if (!pile || needed == 0 || id >= I40E_PILE_VALID_BIT) {
dev_info(&pf->pdev->dev,
"param err: pile=%s needed=%d id=0x%04x\n",
pile ? "<valid>" : "<null>", needed, id);
return -EINVAL;
}
/* start from beginning because earlier areas may have been freed */
i = 0;
while (i < pile->num_entries) {
/* skip already allocated entries */
if (pile->list[i] & I40E_PILE_VALID_BIT) {
i++;
continue;
}
/* do we have enough in this lump? */
for (j = 0; (j < needed) && ((i+j) < pile->num_entries); j++) {
if (pile->list[i+j] & I40E_PILE_VALID_BIT)
break;
}
if (j == needed) {
/* there was enough, so assign it to the requestor */
for (j = 0; j < needed; j++)
pile->list[i+j] = id | I40E_PILE_VALID_BIT;
ret = i;
break;
}
/* not enough, so skip over it and continue looking */
i += j;
}
return ret;
}
/**
* i40e_put_lump - return a lump of generic resource
* @pile: the pile of resource to search
* @index: the base item index
* @id: the owner id of the items assigned
*
* Returns the count of items in the lump
**/
static int i40e_put_lump(struct i40e_lump_tracking *pile, u16 index, u16 id)
{
int valid_id = (id | I40E_PILE_VALID_BIT);
int count = 0;
u16 i;
if (!pile || index >= pile->num_entries)
return -EINVAL;
for (i = index;
i < pile->num_entries && pile->list[i] == valid_id;
i++) {
pile->list[i] = 0;
count++;
}
return count;
}
/**
* i40e_max_lump_qp - find a biggest size of lump available in qp_pile
* @pf: pointer to private device data structure
*
* Returns the max size of lump in a qp_pile, or negative for error
*/
int i40e_max_lump_qp(struct i40e_pf *pf)
{
struct i40e_lump_tracking *pile = pf->qp_pile;
int pool_size, max_size;
u16 i;
if (!pile) {
dev_info(&pf->pdev->dev,
"param err: pile=%s\n",
pile ? "<valid>" : "<null>");
retur
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
此版本包括适用于英特以太网网络连接的 i40e Linux* 基础驱动程序。 i40e 驱动程序支持基于以下控制器的设备: 英特尔 Ethernet Controller X710 英特尔Ethernet Controller XL710 英特尔以太网 网络连接 X722 英特尔Ethernet Controller XXV710 英特尔Ethernet Controller V710 i40e-x.x.x.tar.gz
资源详情
资源评论
资源推荐
收起资源包目录


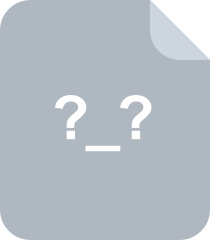
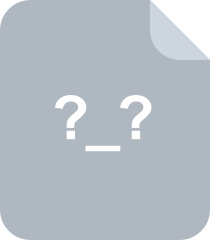

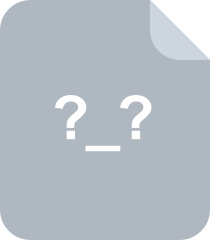
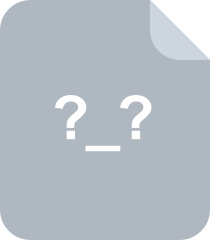
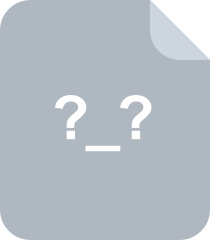
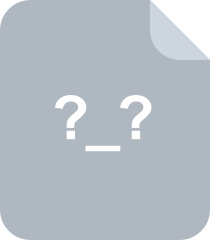
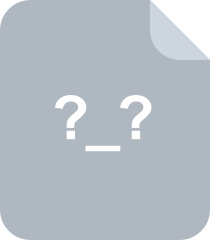
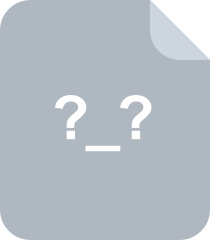
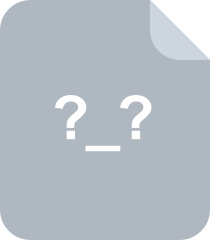
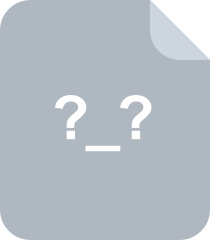
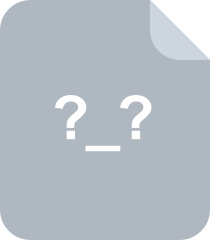
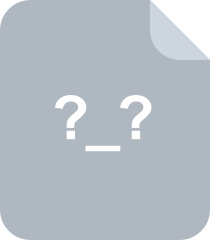
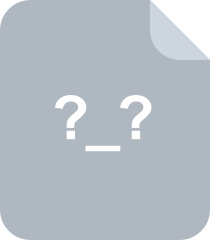
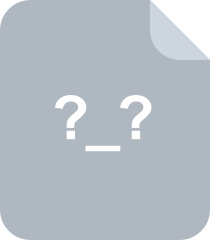
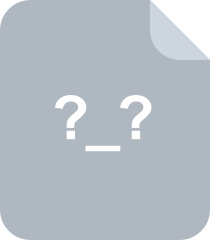
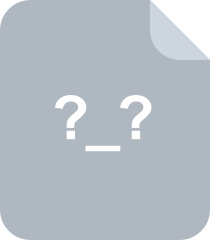
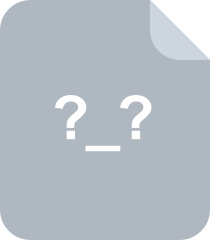
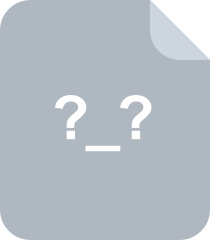
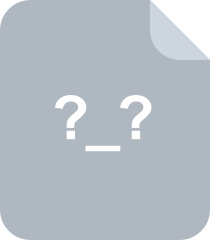
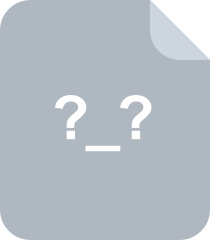
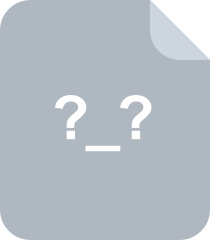
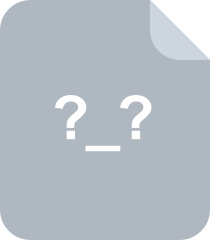
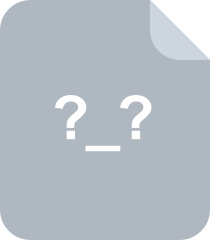
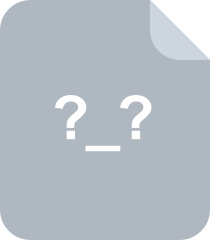
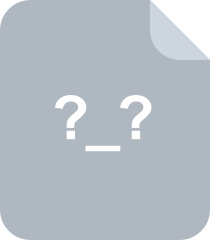
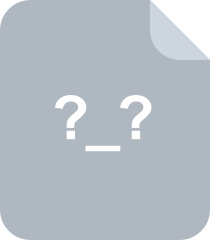
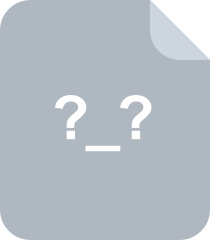
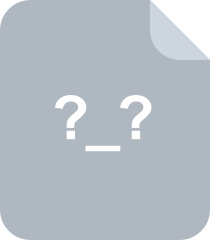
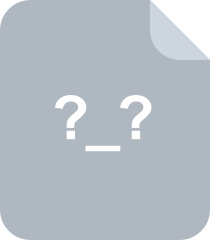
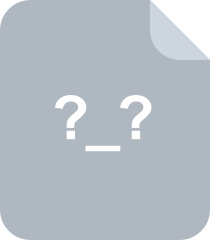
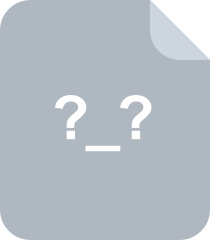
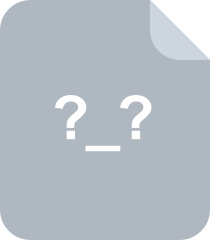
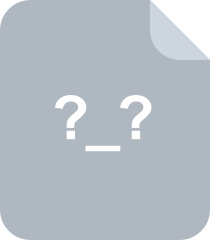
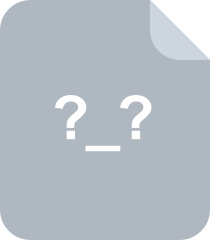
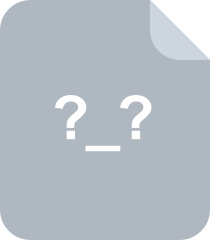
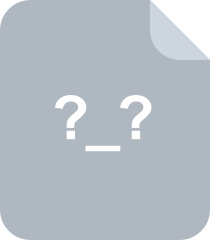
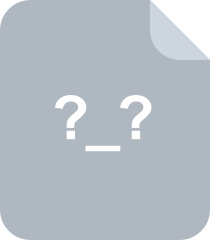
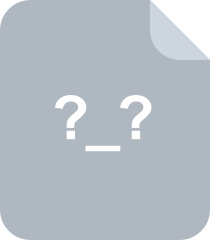
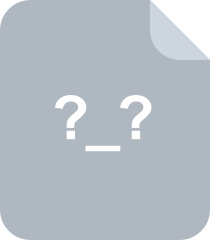
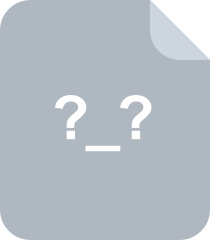
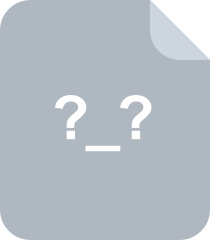
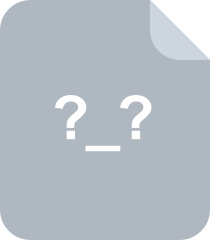
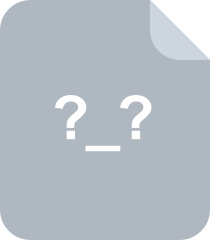
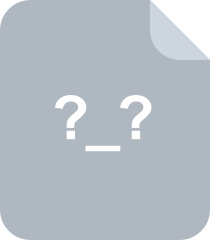

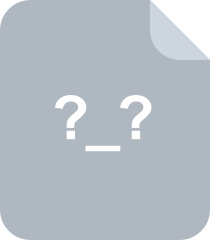
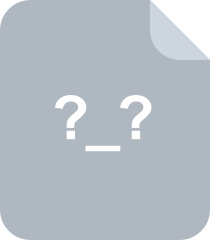
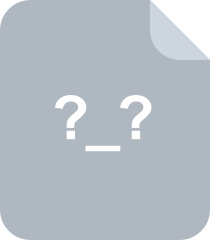
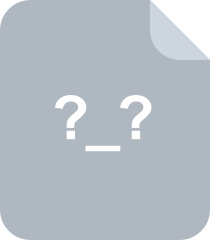
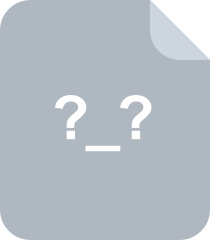
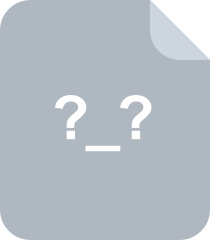
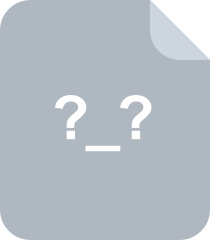
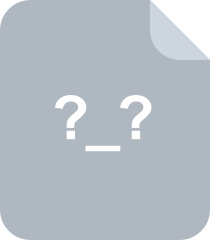
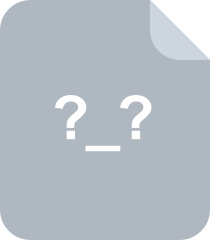
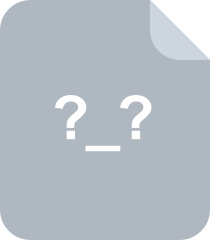
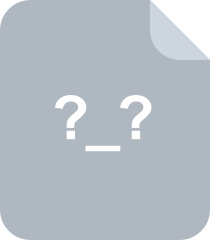
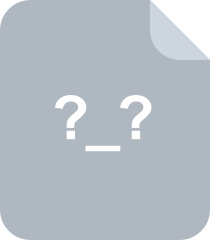
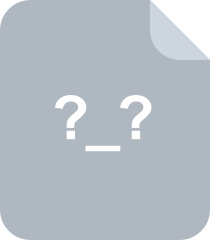
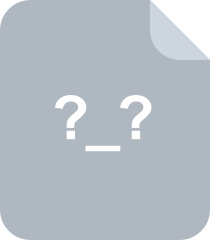
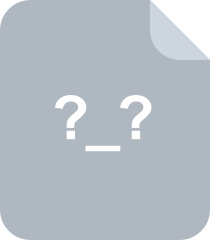
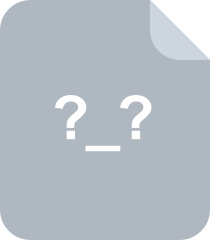
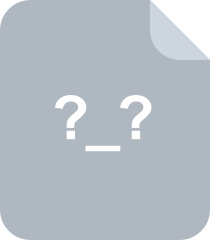
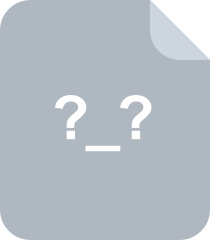
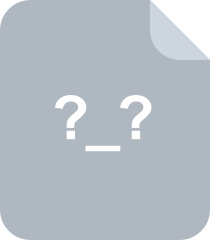

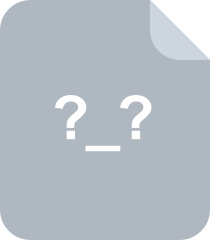
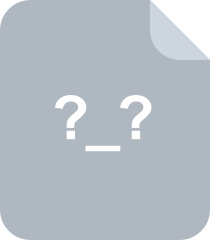
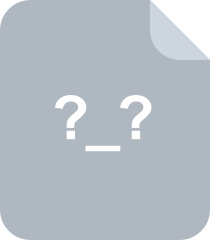
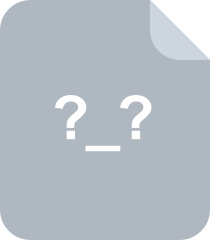
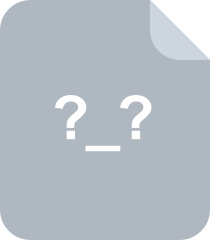
共 68 条
- 1
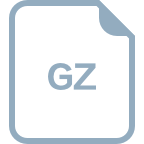
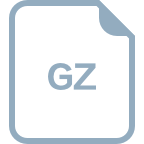
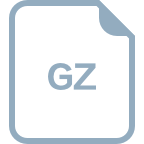
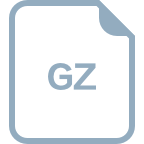
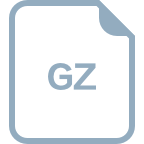
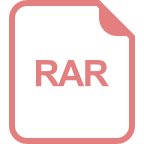
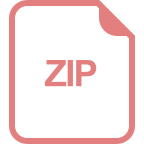
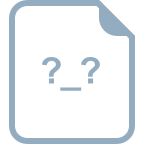
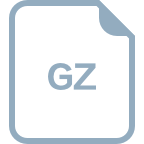
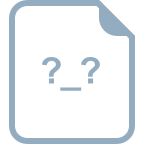
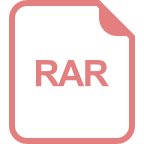
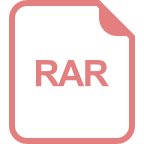
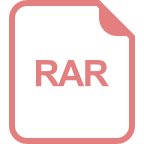
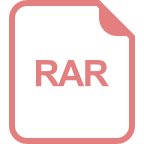
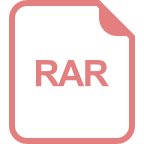
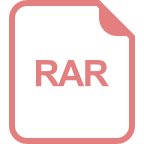
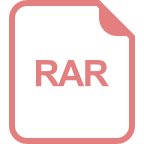
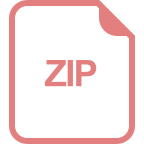
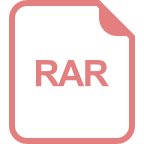
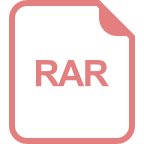
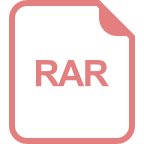
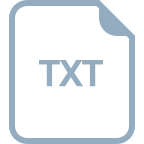

yal179
- 粉丝: 44
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

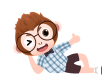
最新资源
- 二维码图形检测6-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- Matlab绘制绚丽烟花动画迎新年
- 厚壁圆筒弹性应力计算,过盈干涉量计算
- 网络实践11111111111111
- GO编写图片上传代码.txt
- LabVIEW采集摄像头数据,实现图像数据存储和浏览
- 几种不同方式生成音乐的 Python 源码示例.txt
- python红包打开后出现烟花代码.txt
- 嵌入式 imx6 linux gdb工具
- 乒乓球检测22-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


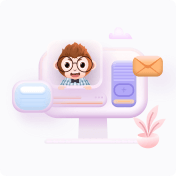
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0