lexical-core
============
[](https://travis-ci.org/Alexhuszagh/rust-lexical)
[](https://crates.io/crates/lexical-core)
[](https://blog.rust-lang.org/2018/02/15/Rust-1.24.html)
Low-level, lexical conversion routines for use in a `no_std` context. This crate by default does not use the Rust standard library.
- [Getting Started](#getting-started)
- [Features](#features)
- [Format](#format)
- [Configuration](#configuration)
- [Constants](#constants)
- [Documentation](#documentation)
- [Validation](#validation)
- [Implementation Details](#implementation-details)
- [Float to String](#float-to-string)
- [String to Float](#string-to-float)
- [Arbitrary-Precision Arithmetic](#arbitrary-precision-arithmetic)
- [Algorithm Background and Comparison](#algorithm-background-and-comparison)
- [Known Issues](#known-issues)
- [Version Support](#version-support)
- [Changelog](#changelog)
- [License](#license)
- [Contributing](#contributing)
# Getting Started
lexical-core is a low-level API for number-to-string and string-to-number conversions, without requiring a system allocator. If you would like to use a convenient, high-level API, please look at [lexical](../lexical) instead.
Add lexical-core to your `Cargo.toml`:
```toml
[dependencies]
lexical-core = "^0.6.7"
```
And an introduction through use:
```rust
extern crate lexical_core;
// String to number using Rust slices.
// The argument is the byte string parsed.
let f: f32 = lexical_core::parse(b"3.5").unwrap(); // 3.5
let i: i32 = lexical_core::parse(b"15").unwrap(); // 15
// All lexical_core parsers are checked, they validate the
// input data is entirely correct, and stop parsing when invalid data
// is found, or upon numerical overflow.
let r = lexical_core::parse::<u8>(b"256"); // Err(ErrorCode::Overflow.into())
let r = lexical_core::parse::<u8>(b"1a5"); // Err(ErrorCode::InvalidDigit.into())
// In order to extract and parse a number from a substring of the input
// data, use `parse_partial`. These functions return the parsed value and
// the number of processed digits, allowing you to extract and parse the
// number in a single pass.
let r = lexical_core::parse_partial::<i8>(b"3a5"); // Ok((3, 1))
// If an insufficiently long buffer is passed, the serializer will panic.
// PANICS
let mut buf = [b'0'; 1];
//let slc = lexical_core::write::<i64>(15, &mut buf);
// In order to guarantee the buffer is long enough, always ensure there
// are at least `T::FORMATTED_SIZE` bytes, which requires the
// `lexical_core::Number` trait to be in scope.
use lexical_core::Number;
let mut buf = [b'0'; f64::FORMATTED_SIZE];
let slc = lexical_core::write::<f64>(15.1, &mut buf);
assert_eq!(slc, b"15.1");
// When the `radix` feature is enabled, for decimal floats, using
// `T::FORMATTED_SIZE` may significantly overestimate the space
// required to format the number. Therefore, the
// `T::FORMATTED_SIZE_DECIMAL` constants allow you to get a much
// tighter bound on the space required.
let mut buf = [b'0'; f64::FORMATTED_SIZE_DECIMAL];
let slc = lexical_core::write::<f64>(15.1, &mut buf);
assert_eq!(slc, b"15.1");
```
# Features
- **correct** Use a correct string-to-float parser.
<blockquote>Enabled by default, and may be turned off by setting <code>default-features = false</code>. If neither <code>algorithm_m</code> nor <code>bhcomp</code> is enabled while <code>correct</code> is enabled, lexical uses the <code>bigcomp</code> algorithm.</blockquote>
- **trim_floats** Export floats without a fraction as an integer.
<blockquote>For example, <code>0.0f64</code> will be serialized to "0" and not "0.0", and <code>-0.0</code> as "0" and not "-0.0".</blockquote>
- **radix** Allow conversions to and from non-decimal strings.
<blockquote>With radix enabled, any radix from 2 to 36 (inclusive) is valid, otherwise, only 10 is valid.</blockquote>
- **format** Customize accepted inputs for number parsing.
<blockquote>With format enabled, the number format is dictated through the <code>NumberFormat</code> bitflags, which allow you to toggle how to parse a string into a number. Various flags including enabling digit separators, requiring integer or fraction digits, and toggling special values.</blockquote>
- **rounding** Enable custom rounding for IEEE754 floats.
<blockquote>By default, lexical uses round-nearest, tie-even for float rounding (recommended by IEE754).</blockquote>
- **ryu** Use dtolnay's [ryu](https://github.com/dtolnay/ryu/) library for float-to-string conversions.
<blockquote>Enabled by default, and may be turned off by setting <code>default-features = false</code>. Ryu is ~2x as fast as other float formatters.</blockquote>
## Format
Every language has competing specifications for valid numerical input, meaning a number parser for Rust will incorrectly accept or reject input for different programming or data languages. For example:
```rust
extern crate lexical_core;
use lexical_core::*;
// Valid in Rust strings.
// Not valid in JSON.
let f: f64 = parse(b"3.e7").unwrap(); // 3e7
// Let's only accept JSON floats.
let format = NumberFormat::JSON;
let f: f64 = parse_format(b"3.0e7", format).unwrap(); // 3e7
let f: f64 = parse_format(b"3.e7", format).unwrap(); // Panics!
// We can also allow digit separators, for example.
// OCaml, a programming language that inspired Rust,
// accepts digit separators pretty much anywhere.
let format = NumberFormat::OCAML_STRING;
let f: f64 = parse(b"3_4.__0_1").unwrap(); // Panics!
let f: f64 = parse_format(b"3_4.__0_1", format).unwrap(); // 34.01
```
The parsing specification is defined by `NumberFormat`, which provides pre-defined constants for over 40 programming and data languages. However, it also allows you to create your own specification, to dictate parsing.
```rust
extern crate lexical_core;
use lexical_core::*;
// Let's use the standard, Rust grammar.
let format = NumberFormat::standard().unwrap();
// Let's use a permissive grammar, one that allows anything besides
// digit separators.
let format = NumberFormat::permissive().unwrap();
// Let's ignore digit separators and have an otherwise permissive grammar.
let format = NumberFormat::ignore(b'_').unwrap();
// Create our own grammar.
// A NumberFormat is compiled from options into binary flags, each
// taking 1-bit, allowing high-performance, customizable parsing
// once they're compiled. Each flag will be explained while defining it.
// The '_' character will be used as a digit separator.
let digit_separator = b'_';
// Require digits in the integer component of a float.
// `0.1` is valid, but `.1` is not.
let required_integer_digits = false;
// Require digits in the fraction component of a float.
// `1.0` is valid, but `1.` and `1` are not.
let required_fraction_digits = false;
// Require digits in the exponent component of a float.
// `1.0` and `1.0e7` is valid, but `1.0e` is not.
let required_exponent_digits = false;
// Do not allow a positive sign before the mantissa.
// `1.0` and `-1.0` are valid, but `+1.0` is not.
let no_positive_mantissa_sign = false;
// Require a sign before the mantissa.
// `+1.0` and `-1.0` are valid, but `1.0` is not.
let required_mantissa_sign = false;
// Do not allow the use of exponents.
// `300.0` is valid, but `3.0e2` is not.
let no_exponent_notation = false;
// Do not allow a positive sign before the exponent.
// `3.0e2` and 3.0e-2` are valid, but `3.0e+2` is not.
let no_positive_exponent_sign = false;
// Require a sign before the exponent.
// `3.0e+2` and `3.0e-2` are valid, but `3.0e2` is not.
let required_exponent_sign = false;
// Do not allow an exponent without fraction digits.
// `3.0e7` is valid, but `3e7` and `3.e7` are not.
let no_exponent_wi
没有合适的资源?快使用搜索试试~ 我知道了~
将 suricata 检测流程部分编程成.so,外部程序采集数据并调用此.so,获取检测得到的 alert结果
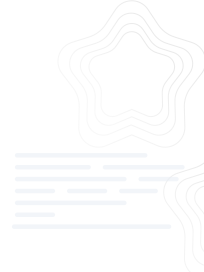
共4284个文件
rs:1758个
c:661个
h:614个

需积分: 0 42 下载量 14 浏览量
2022-02-10
09:30:39
上传
评论 2
收藏 27.63MB ZIP 举报
温馨提示
1、外部调用程序采集网卡数据包,程序调用 libsuri_603.so,将采集到的数据传入。 2、外部程序做线程管理。只在各个子线程中分别调用libsuri_603.so做初始化和检测功能。 3、封装提供给外部程序调用的动态规则加载接口,这个采用单独的线程。 4、至少提供四个接口,分别为初始化,检测数据传入,动态规则加载接口,Destroy。
资源详情
资源评论
资源推荐
收起资源包目录

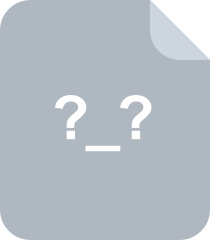
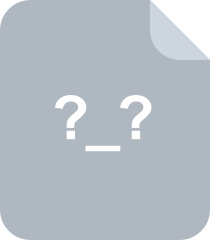
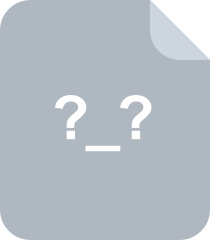
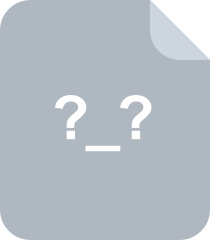
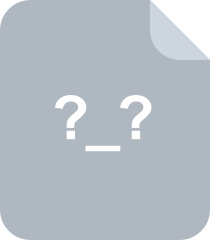
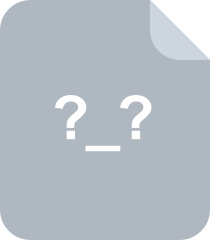
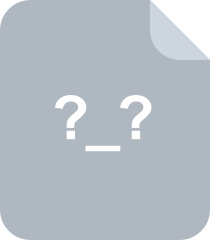
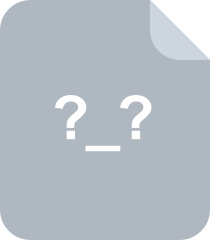
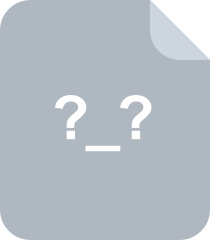
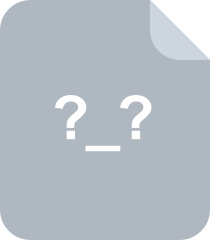
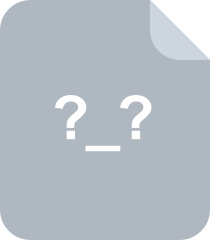
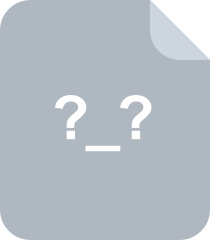
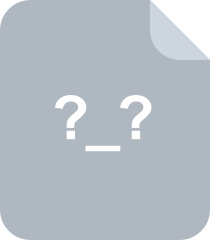
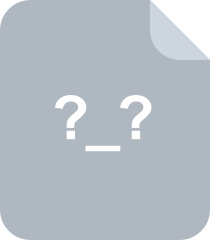
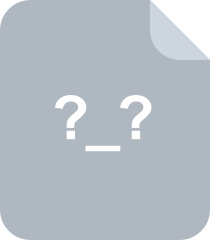
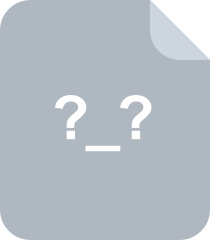
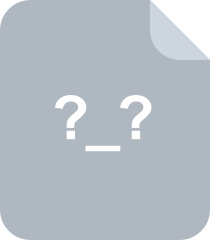
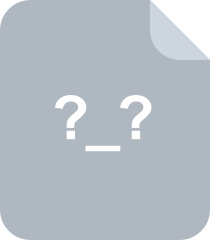
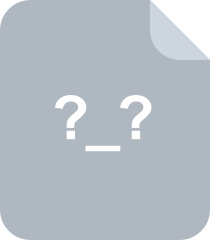
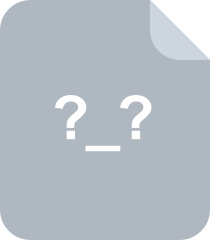
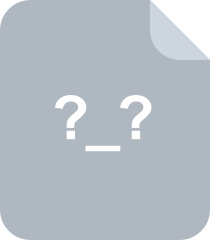
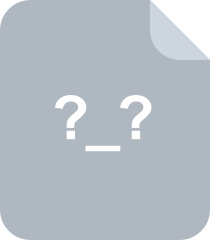
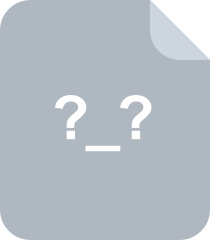
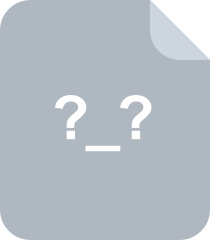
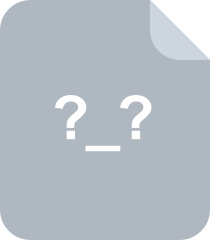
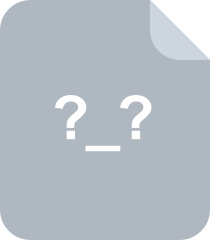
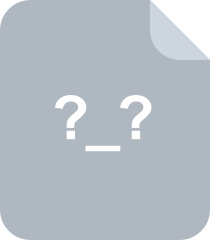
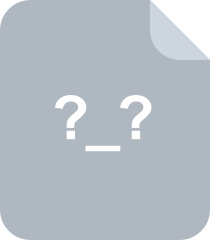
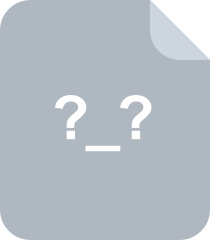
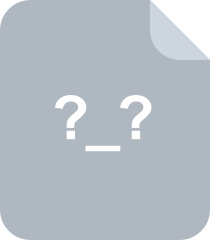
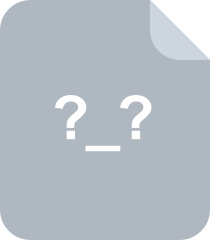
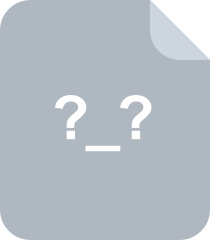
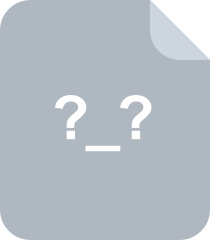
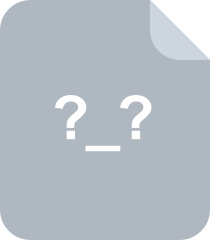
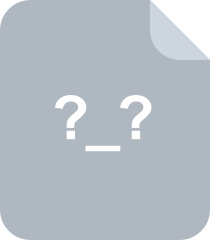
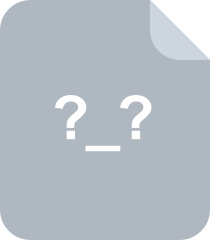
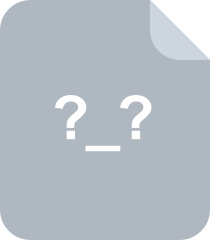
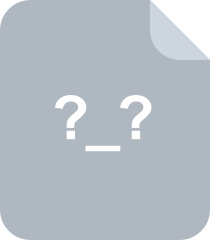
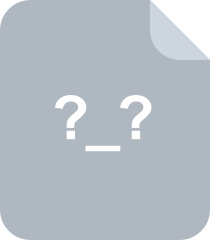
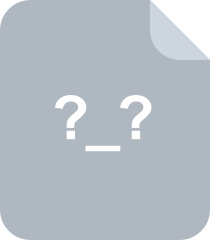
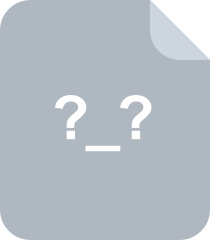
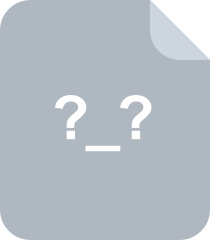
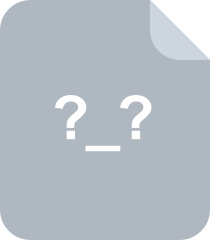
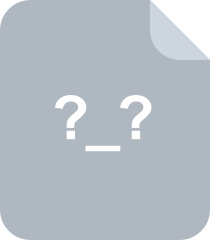
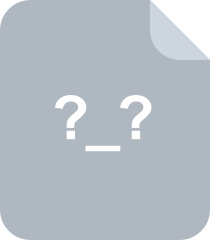
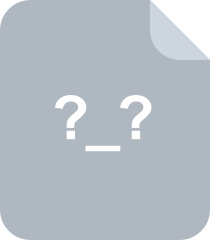
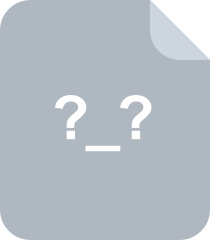
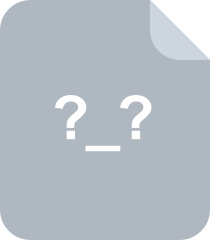
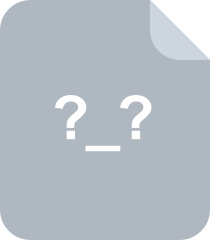
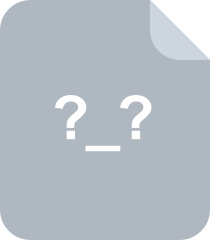
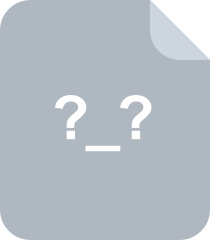
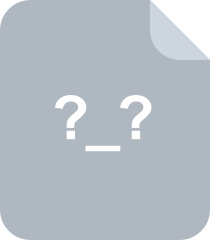
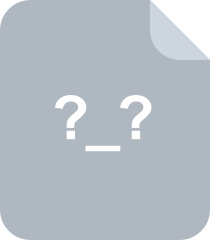
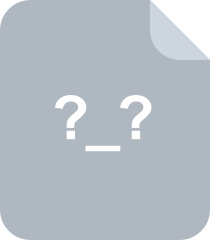
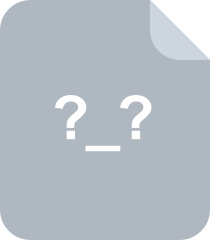
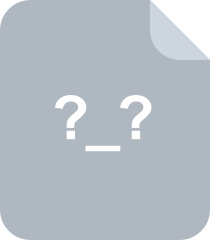
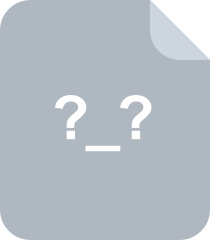
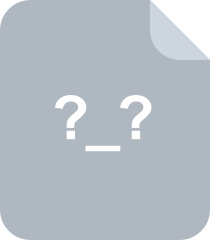
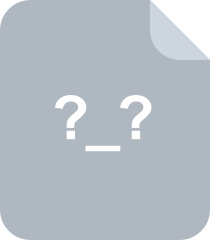
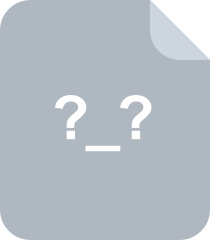
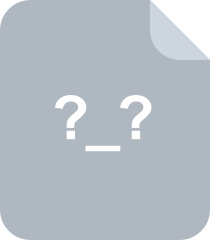
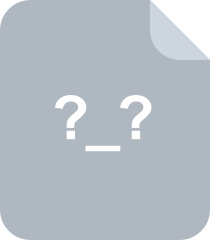
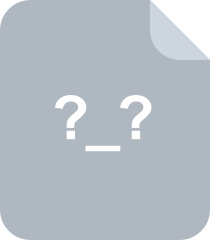
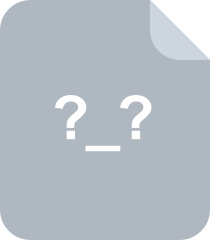
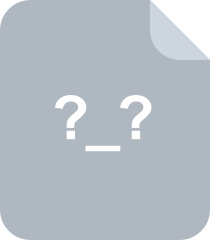
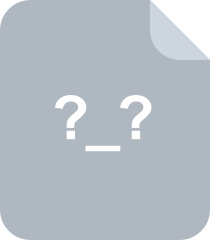
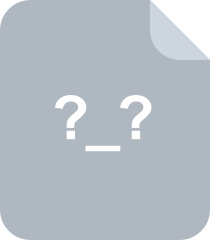
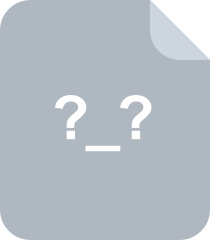
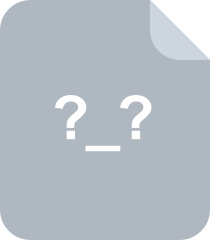
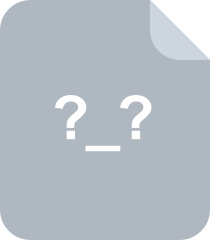
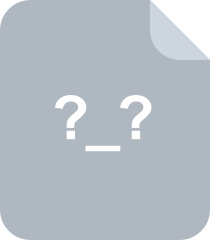
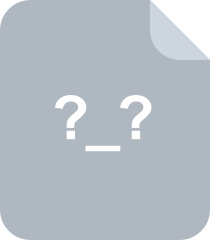
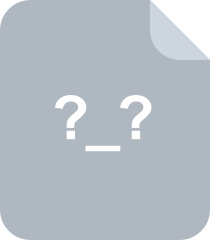
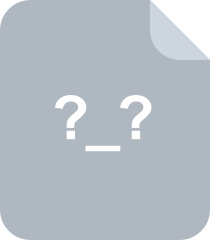
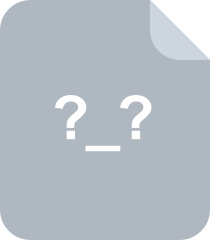
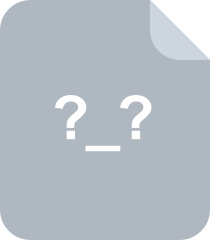
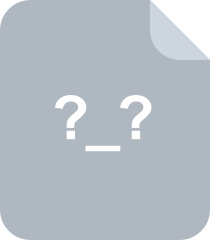
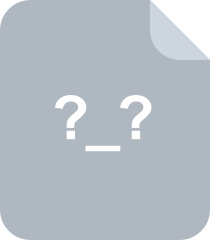
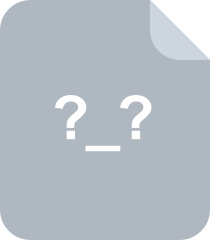
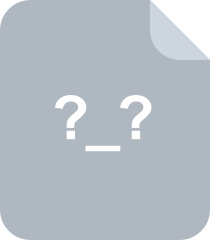
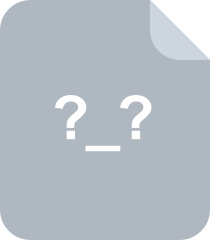
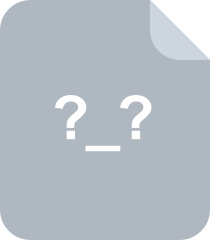
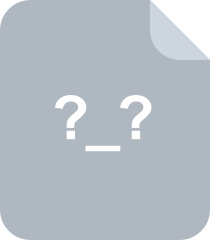
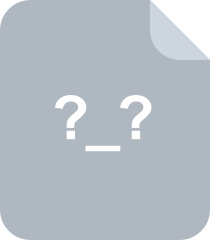
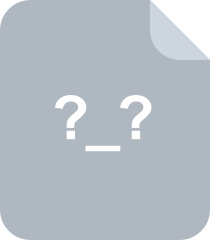
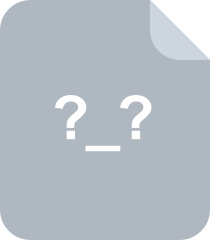
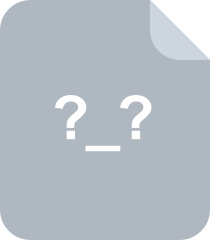
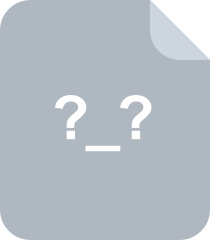
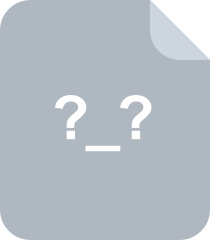
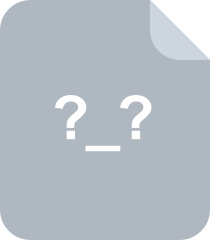
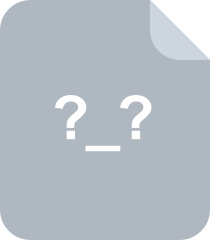
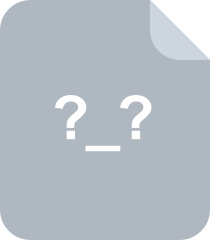
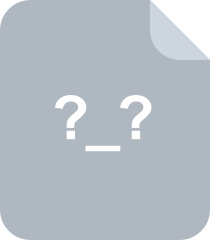
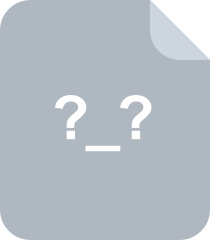
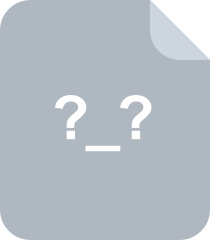
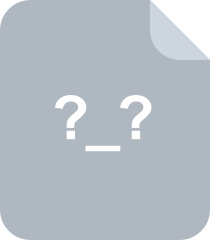
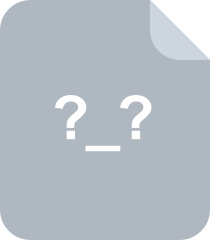
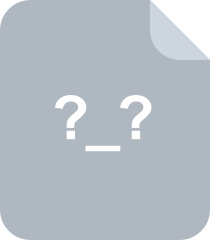
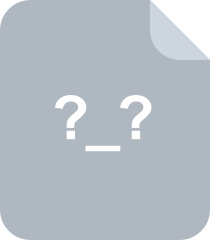
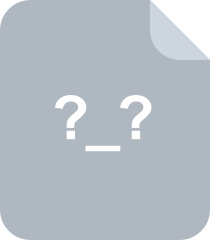
共 4284 条
- 1
- 2
- 3
- 4
- 5
- 6
- 43
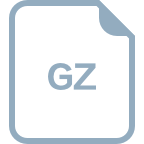
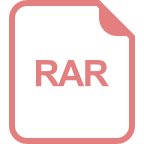
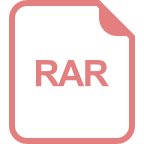
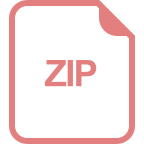
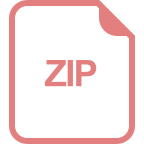
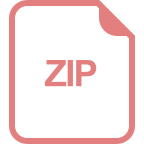
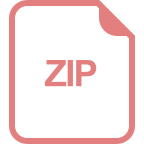
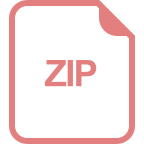
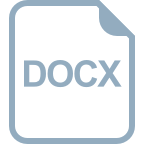
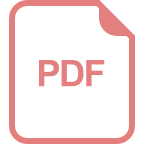
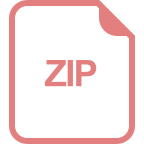
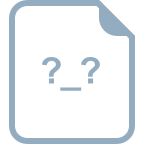
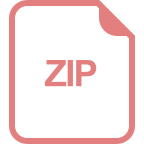
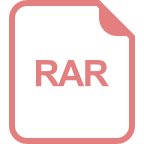
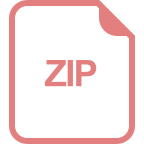
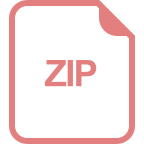
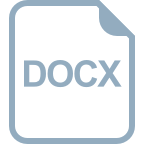

杜兰特的小号
- 粉丝: 4352
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

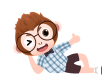
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0