# melody

[](https://app.codecov.io/github/olahol/melody)
[](https://goreportcard.com/report/github.com/olahol/melody)
[](https://godoc.org/github.com/olahol/melody)
> :notes: Minimalist websocket framework for Go.
Melody is websocket framework based on [github.com/gorilla/websocket](https://github.com/gorilla/websocket)
that abstracts away the tedious parts of handling websockets. It gets out of
your way so you can write real-time apps. Features include:
* [x] Clear and easy interface similar to `net/http` or Gin.
* [x] A simple way to broadcast to all or selected connected sessions.
* [x] Message buffers making concurrent writing safe.
* [x] Automatic handling of sending ping/pong heartbeats that timeout broken sessions.
* [x] Store data on sessions.
## Install
```bash
go get github.com/olahol/melody
```
## [Example: chat](https://github.com/olahol/melody/tree/master/examples/chat)
[](https://github.com/olahol/melody/tree/master/examples/chat)
```go
package main
import (
"net/http"
"github.com/olahol/melody"
)
func main() {
m := melody.New()
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
http.ServeFile(w, r, "index.html")
})
http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) {
m.HandleRequest(w, r)
})
m.HandleMessage(func(s *melody.Session, msg []byte) {
m.Broadcast(msg)
})
http.ListenAndServe(":5000", nil)
}
```
## [Example: gophers](https://github.com/olahol/melody/tree/master/examples/gophers)
[](https://github.com/olahol/melody/tree/master/examples/gophers)
```go
package main
import (
"fmt"
"net/http"
"sync/atomic"
"github.com/olahol/melody"
)
var idCounter atomic.Int64
func main() {
m := melody.New()
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
http.ServeFile(w, r, "index.html")
})
http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) {
m.HandleRequest(w, r)
})
m.HandleConnect(func(s *melody.Session) {
id := idCounter.Add(1)
s.Set("id", id)
s.Write([]byte(fmt.Sprintf("iam %d", id)))
})
m.HandleDisconnect(func(s *melody.Session) {
if id, ok := s.Get("id"); ok {
m.BroadcastOthers([]byte(fmt.Sprintf("dis %d", id)), s)
}
})
m.HandleMessage(func(s *melody.Session, msg []byte) {
if id, ok := s.Get("id"); ok {
m.BroadcastOthers([]byte(fmt.Sprintf("set %d %s", id, msg)), s)
}
})
http.ListenAndServe(":5000", nil)
}
```
### [More examples](https://github.com/olahol/melody/tree/master/examples)
## [Documentation](https://godoc.org/github.com/olahol/melody)
## Contributors
<a href="https://github.com/olahol/melody/graphs/contributors">
<img src="https://contrib.rocks/image?repo=olahol/melody" />
</a>
## FAQ
If you are getting a `403` when trying to connect to your websocket you can [change allow all origin hosts](http://godoc.org/github.com/gorilla/websocket#hdr-Origin_Considerations):
```go
m := melody.New()
m.Upgrader.CheckOrigin = func(r *http.Request) bool { return true }
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
旋律 适用于 Go 的极简 websocket 框架。Melody 是基于github.com/gorilla/websocket 的websocket 框架 ,它抽象了处理 websocket 的繁琐部分。它让您可以编写实时应用程序。功能包括清晰且简单的界面类似于net/http或 Gin。向所有或选定的连接会话进行广播的简单方法。消息缓冲区使得并发写入变得安全。自动处理发送超时中断会话的 ping/pong 心跳。存储会话数据。安装go get github.com/olahol/melody例如聊天package mainimport ( "net/http" "github.com/olahol/melody")func main() { m := melody.New() http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "index.html") }) http.HandleF
资源推荐
资源详情
资源评论
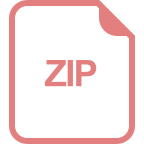
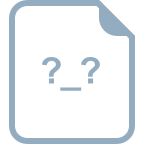
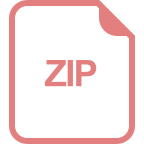
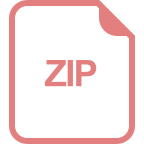
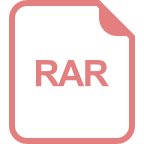
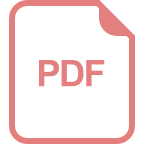
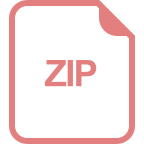
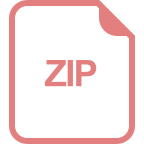
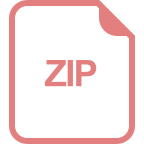
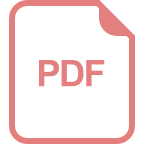
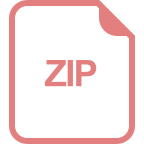
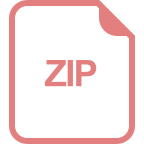
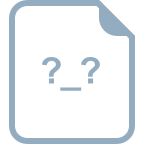
收起资源包目录

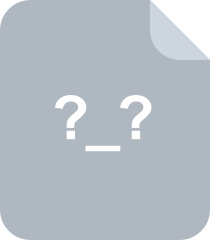
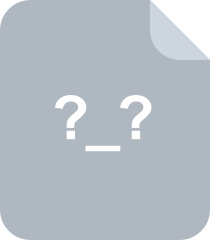


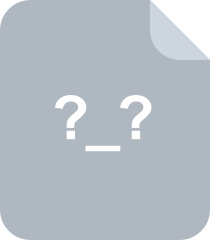
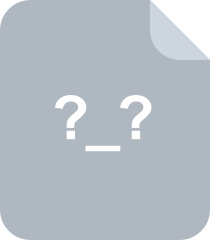
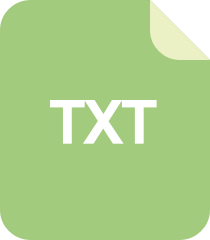
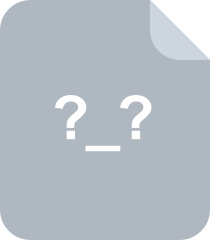
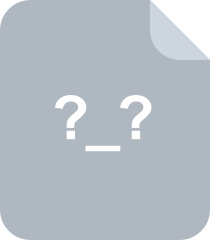
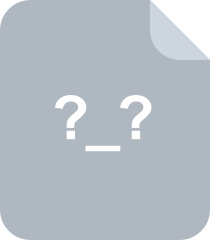

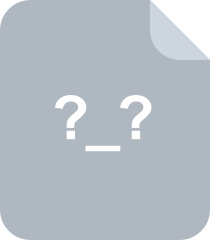
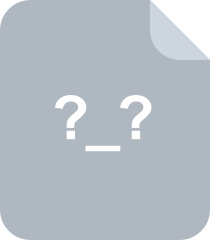

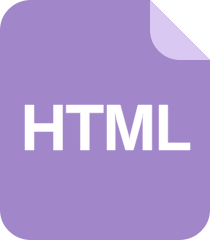
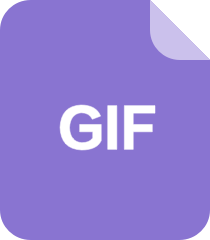
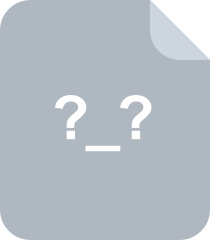

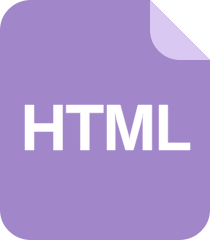
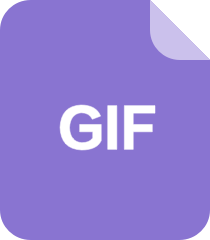
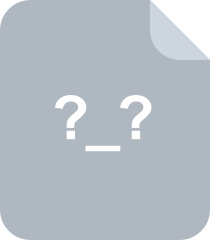

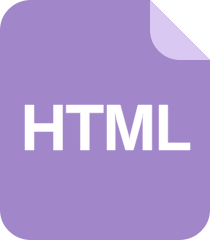
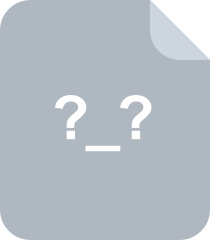

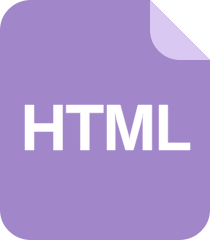
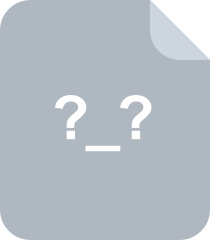

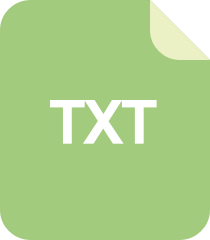
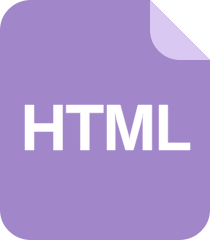
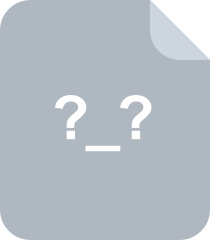

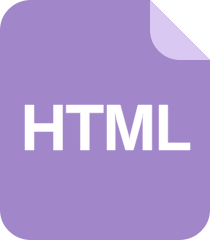
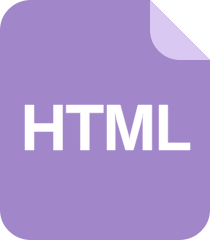
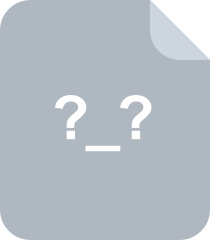
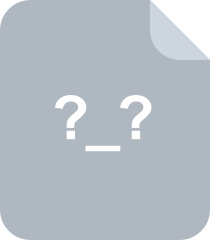
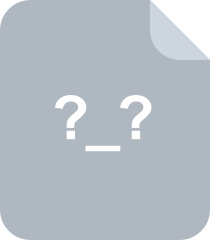
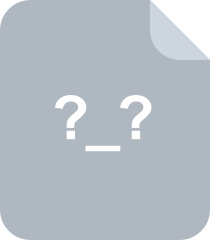
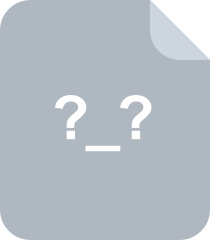
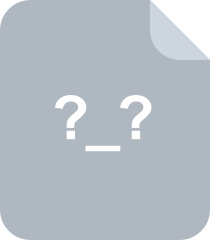
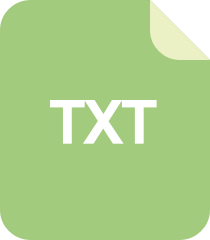
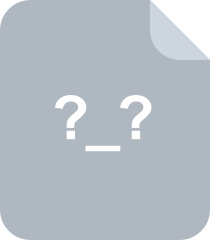
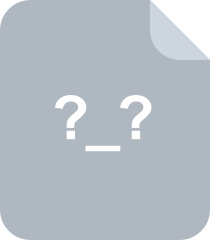
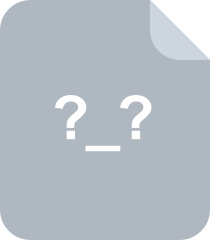
共 35 条
- 1
资源评论
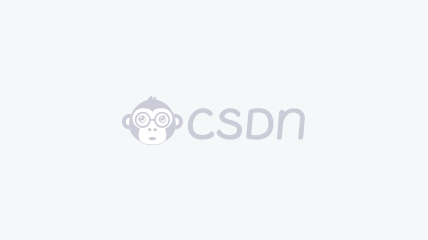

赵闪闪168
- 粉丝: 1658
- 资源: 5391
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

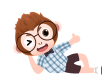
最新资源
- base(1).apk.1
- K618977005_2012-12-6_beforeP_000.txt.PRM
- 秋招信息获取与处理基础教程
- 程序员面试笔试面经技巧基础教程
- Python实例-21个自动办公源码-数据处理技术+Excel+自动化脚本+资源管理
- 全球前8GDP数据图(python动态柱状图)
- 汽车检测7-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 检测高压线电线-YOLO(v5至v9)、COCO、Darknet、VOC数据集合集.rar
- 检测行路中的人脸-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- Image_17083039753012.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


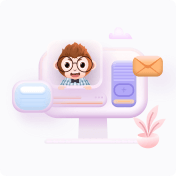
安全验证
文档复制为VIP权益,开通VIP直接复制
