# SSD: Single Shot MultiBox Object Detector, in PyTorch
A [PyTorch](http://pytorch.org/) implementation of [Single Shot MultiBox Detector](http://arxiv.org/abs/1512.02325) from the 2016 paper by Wei Liu, Dragomir Anguelov, Dumitru Erhan, Christian Szegedy, Scott Reed, Cheng-Yang, and Alexander C. Berg. The official and original Caffe code can be found [here](https://github.com/weiliu89/caffe/tree/ssd).
<img align="right" src= "https://github.com/amdegroot/ssd.pytorch/blob/master/doc/ssd.png" height = 400/>
### Table of Contents
- <a href='#installation'>Installation</a>
- <a href='#datasets'>Datasets</a>
- <a href='#training-ssd'>Train</a>
- <a href='#evaluation'>Evaluate</a>
- <a href='#performance'>Performance</a>
- <a href='#demos'>Demos</a>
- <a href='#todo'>Future Work</a>
- <a href='#references'>Reference</a>
## Installation
- Install [PyTorch](http://pytorch.org/) by selecting your environment on the website and running the appropriate command.
- Clone this repository.
* Note: We currently only support Python 3+.
- Then download the dataset by following the [instructions](#datasets) below.
- We now support [Visdom](https://github.com/facebookresearch/visdom) for real-time loss visualization during training!
* To use Visdom in the browser:
```Shell
# First install Python server and client
pip install visdom
# Start the server (probably in a screen or tmux)
python -m visdom.server
```
* Then (during training) navigate to http://localhost:8097/ (see the Train section below for training details).
- Note: For training, we currently support [VOC](http://host.robots.ox.ac.uk/pascal/VOC/) and [COCO](http://mscoco.org/), and aim to add [ImageNet](http://www.image-net.org/) support soon.
## Datasets
To make things easy, we provide bash scripts to handle the dataset downloads and setup for you. We also provide simple dataset loaders that inherit `torch.utils.data.Dataset`, making them fully compatible with the `torchvision.datasets` [API](http://pytorch.org/docs/torchvision/datasets.html).
### COCO
Microsoft COCO: Common Objects in Context
##### Download COCO 2014
```Shell
# specify a directory for dataset to be downloaded into, else default is ~/data/
sh data/scripts/COCO2014.sh
```
### VOC Dataset
PASCAL VOC: Visual Object Classes
##### Download VOC2007 trainval & test
```Shell
# specify a directory for dataset to be downloaded into, else default is ~/data/
sh data/scripts/VOC2007.sh # <directory>
```
##### Download VOC2012 trainval
```Shell
# specify a directory for dataset to be downloaded into, else default is ~/data/
sh data/scripts/VOC2012.sh # <directory>
```
## Training SSD
- First download the fc-reduced [VGG-16](https://arxiv.org/abs/1409.1556) PyTorch base network weights at: https://s3.amazonaws.com/amdegroot-models/vgg16_reducedfc.pth
- By default, we assume you have downloaded the file in the `ssd.pytorch/weights` dir:
```Shell
mkdir weights
cd weights
wget https://s3.amazonaws.com/amdegroot-models/vgg16_reducedfc.pth
```
- To train SSD using the train script simply specify the parameters listed in `train.py` as a flag or manually change them.
```Shell
python train.py
```
- Note:
* For training, an NVIDIA GPU is strongly recommended for speed.
* For instructions on Visdom usage/installation, see the <a href='#installation'>Installation</a> section.
* You can pick-up training from a checkpoint by specifying the path as one of the training parameters (again, see `train.py` for options)
## Evaluation
To evaluate a trained network:
```Shell
python eval.py
```
You can specify the parameters listed in the `eval.py` file by flagging them or manually changing them.
<img align="left" src= "https://github.com/amdegroot/ssd.pytorch/blob/master/doc/detection_examples.png">
## Performance
#### VOC2007 Test
##### mAP
| Original | Converted weiliu89 weights | From scratch w/o data aug | From scratch w/ data aug |
|:-:|:-:|:-:|:-:|
| 77.2 % | 77.26 % | 58.12% | 77.43 % |
##### FPS
**GTX 1060:** ~45.45 FPS
## Demos
### Use a pre-trained SSD network for detection
#### Download a pre-trained network
- We are trying to provide PyTorch `state_dicts` (dict of weight tensors) of the latest SSD model definitions trained on different datasets.
- Currently, we provide the following PyTorch models:
* SSD300 trained on VOC0712 (newest PyTorch weights)
- https://s3.amazonaws.com/amdegroot-models/ssd300_mAP_77.43_v2.pth
* SSD300 trained on VOC0712 (original Caffe weights)
- https://s3.amazonaws.com/amdegroot-models/ssd_300_VOC0712.pth
- Our goal is to reproduce this table from the [original paper](http://arxiv.org/abs/1512.02325)
<p align="left">
<img src="http://www.cs.unc.edu/~wliu/papers/ssd_results.png" alt="SSD results on multiple datasets" width="800px"></p>
### Try the demo notebook
- Make sure you have [jupyter notebook](http://jupyter.readthedocs.io/en/latest/install.html) installed.
- Two alternatives for installing jupyter notebook:
1. If you installed PyTorch with [conda](https://www.continuum.io/downloads) (recommended), then you should already have it. (Just navigate to the ssd.pytorch cloned repo and run):
`jupyter notebook`
2. If using [pip](https://pypi.python.org/pypi/pip):
```Shell
# make sure pip is upgraded
pip3 install --upgrade pip
# install jupyter notebook
pip install jupyter
# Run this inside ssd.pytorch
jupyter notebook
```
- Now navigate to `demo/demo.ipynb` at http://localhost:8888 (by default) and have at it!
### Try the webcam demo
- Works on CPU (may have to tweak `cv2.waitkey` for optimal fps) or on an NVIDIA GPU
- This demo currently requires opencv2+ w/ python bindings and an onboard webcam
* You can change the default webcam in `demo/live.py`
- Install the [imutils](https://github.com/jrosebr1/imutils) package to leverage multi-threading on CPU:
* `pip install imutils`
- Running `python -m demo.live` opens the webcam and begins detecting!
## TODO
We have accumulated the following to-do list, which we hope to complete in the near future
- Still to come:
* [x] Support for the MS COCO dataset
* [ ] Support for SSD512 training and testing
* [ ] Support for training on custom datasets
## Authors
* [**Max deGroot**](https://github.com/amdegroot)
* [**Ellis Brown**](http://github.com/ellisbrown)
***Note:*** Unfortunately, this is just a hobby of ours and not a full-time job, so we'll do our best to keep things up to date, but no guarantees. That being said, thanks to everyone for your continued help and feedback as it is really appreciated. We will try to address everything as soon as possible.
## References
- Wei Liu, et al. "SSD: Single Shot MultiBox Detector." [ECCV2016]((http://arxiv.org/abs/1512.02325)).
- [Original Implementation (CAFFE)](https://github.com/weiliu89/caffe/tree/ssd)
- A huge thank you to [Alex Koltun](https://github.com/alexkoltun) and his team at [Webyclip](http://www.webyclip.com) for their help in finishing the data augmentation portion.
- A list of other great SSD ports that were sources of inspiration (especially the Chainer repo):
* [Chainer](https://github.com/Hakuyume/chainer-ssd), [Keras](https://github.com/rykov8/ssd_keras), [MXNet](https://github.com/zhreshold/mxnet-ssd), [Tensorflow](https://github.com/balancap/SSD-Tensorflow)
没有合适的资源?快使用搜索试试~ 我知道了~
大学生创新创业训练项目-矿井救援机器人.zip
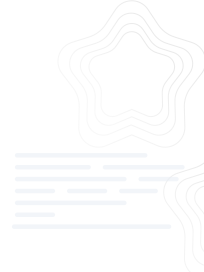
共85个文件
py:35个
jpg:13个
mp4:7个

需积分: 0 2 下载量 184 浏览量
2024-01-17
19:04:52
上传
评论
收藏 150.64MB ZIP 举报
温馨提示
大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机器人.zip大学生创新创业训练项目-矿井救援机
资源推荐
资源详情
资源评论
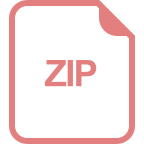
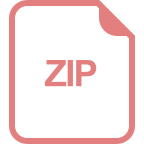
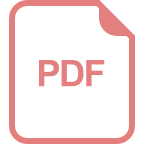
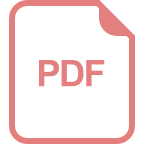
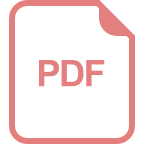
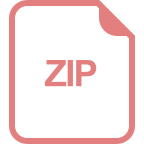
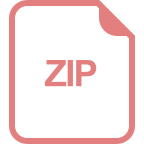
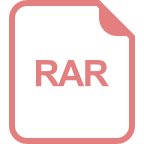
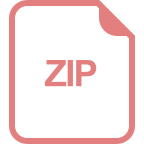
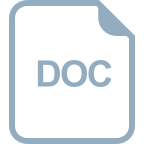
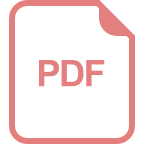
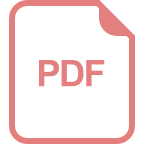
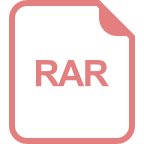
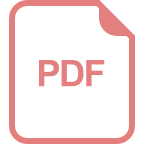
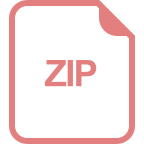
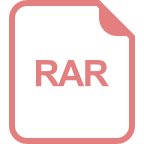
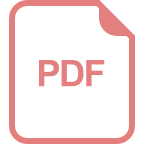
收起资源包目录


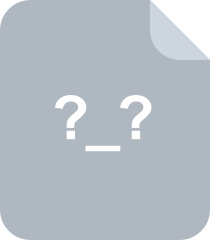
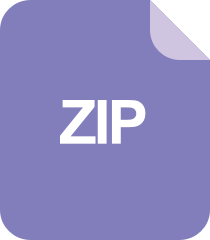

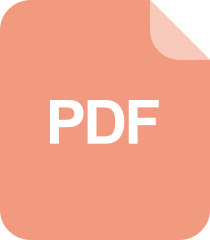
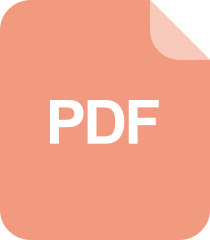
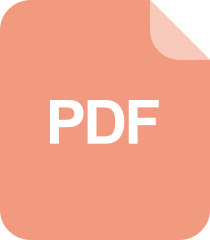
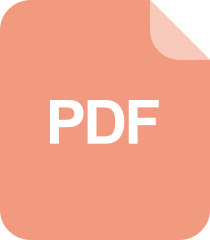


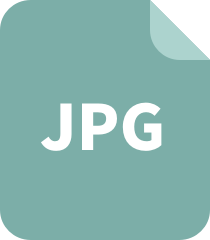
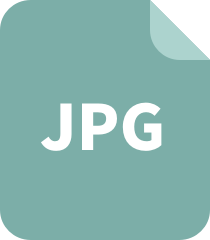
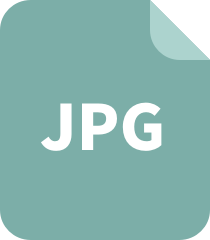
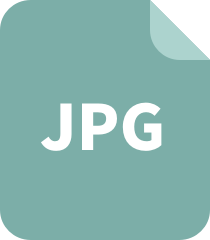
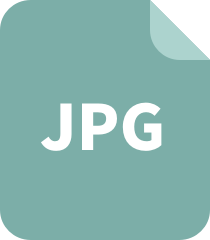
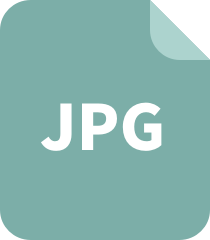
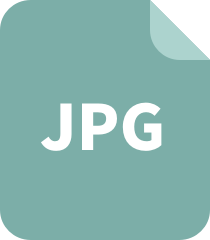
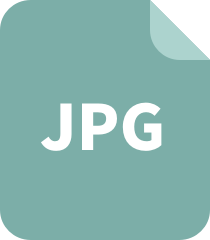
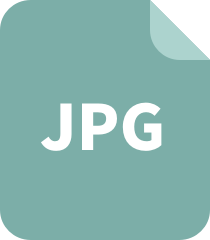
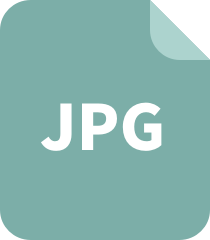
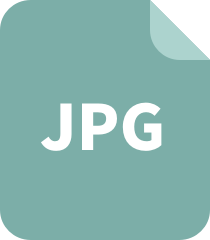

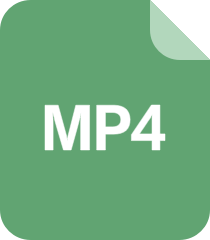
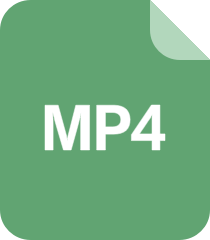
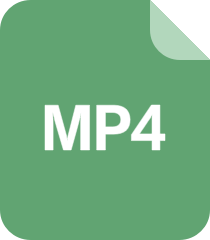
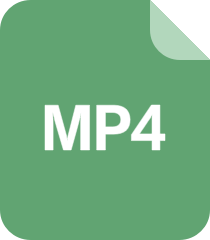
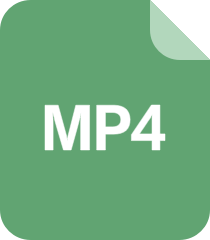
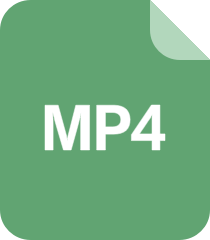
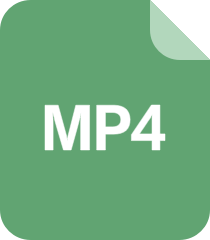


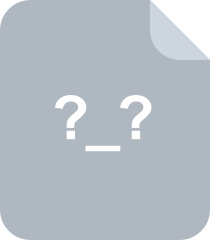
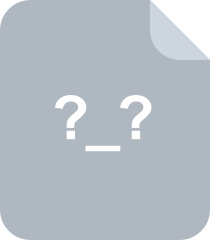
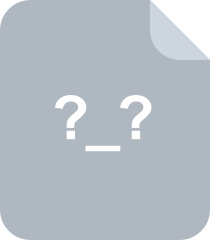
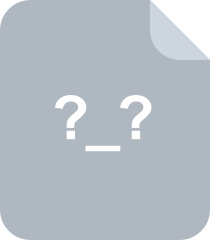
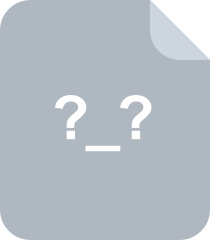

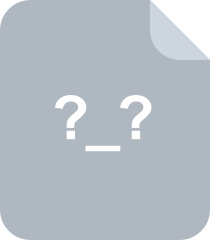

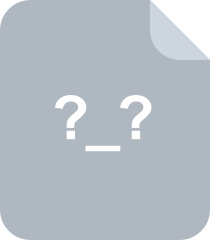
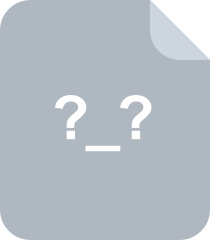
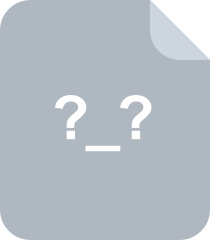

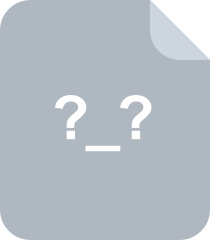
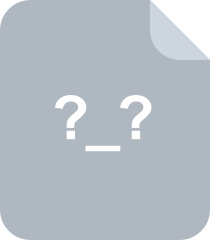
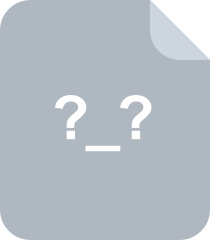
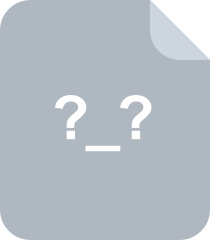

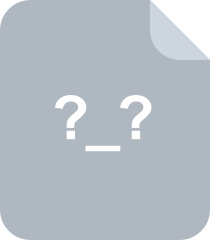


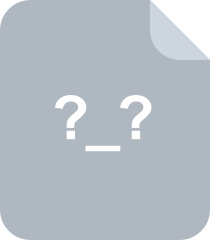

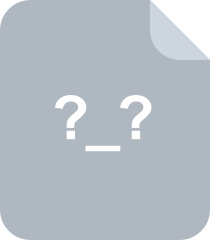
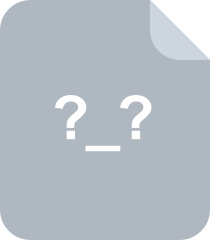
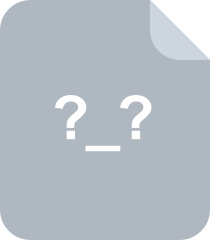
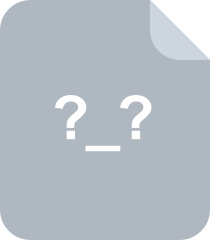
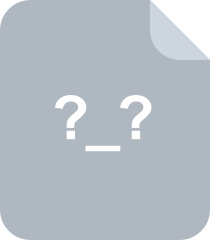
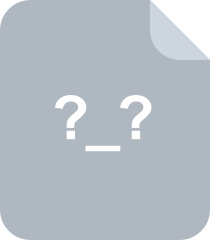

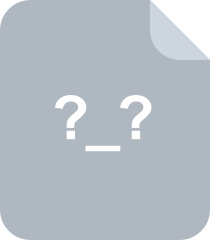
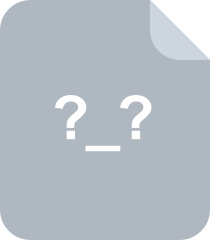
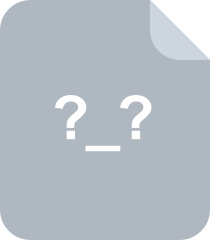
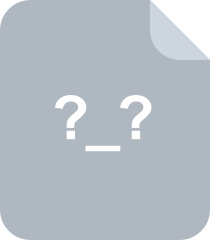
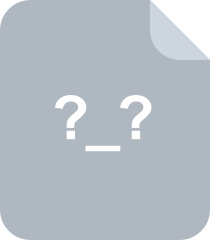

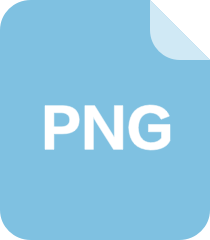
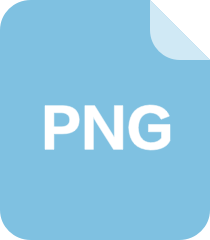
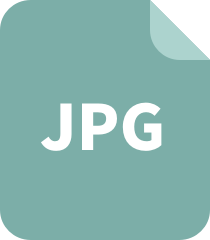
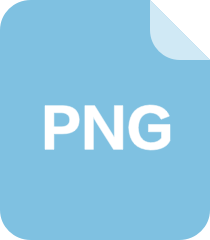
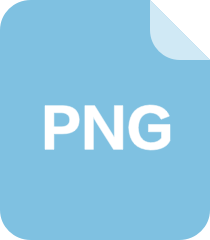

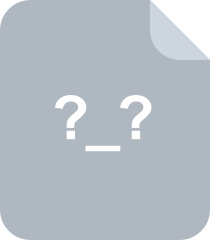
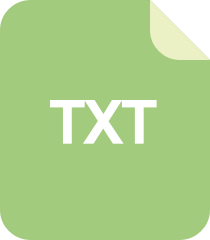
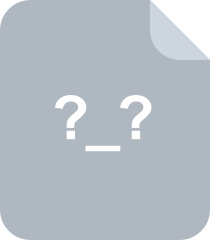
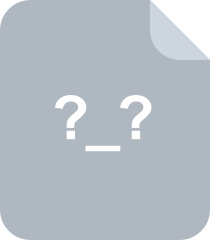
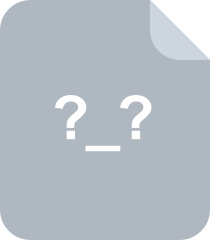

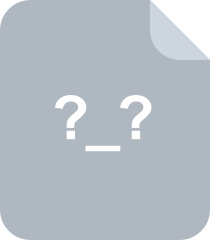

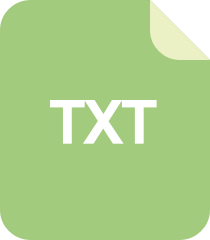
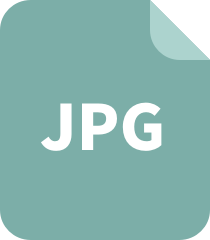
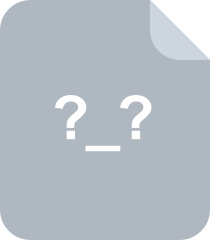

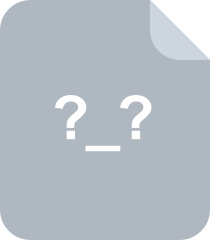
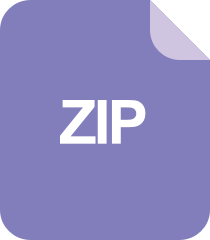
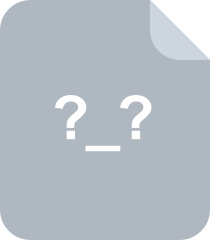
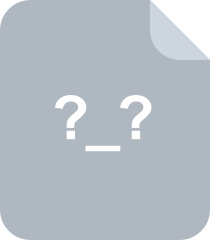
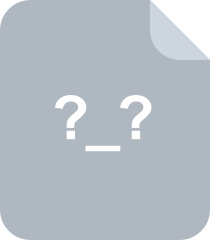
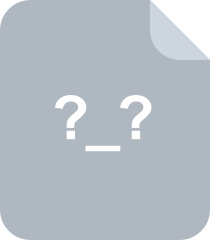

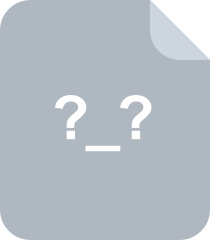
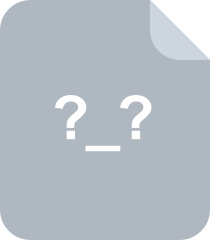
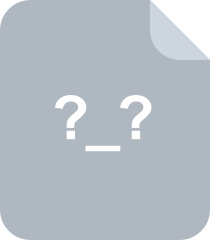

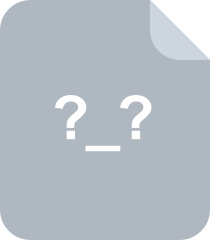
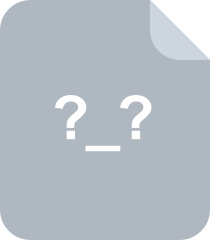

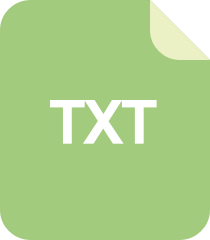
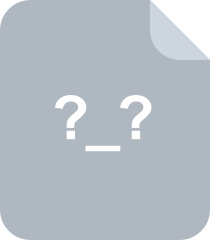
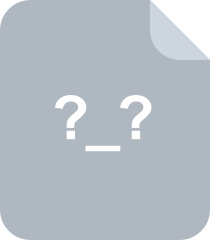
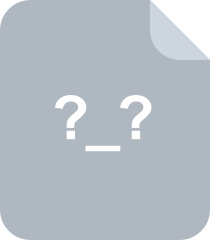
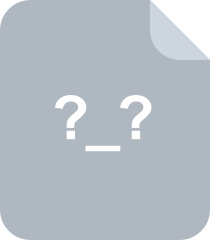
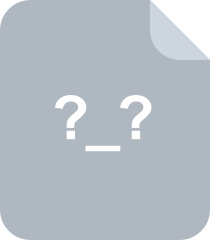
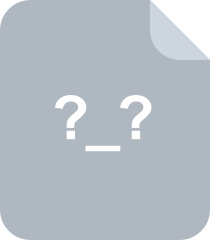
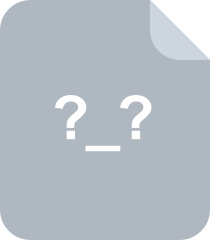
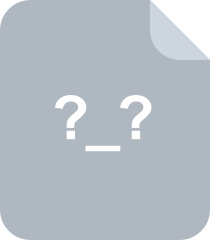
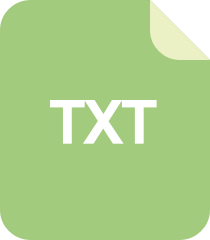
共 85 条
- 1
资源评论
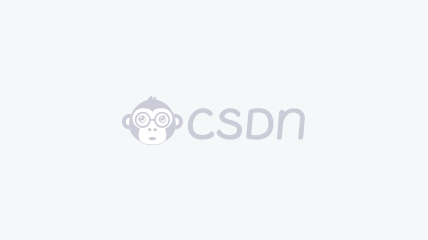

张无忌打怪兽
- 粉丝: 2055
- 资源: 1197
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

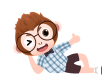
最新资源
- MATLAB界面设计报告.pdf
- 基于PHP实现的学生宿舍管理系统+项目源码+文档说明
- 微信小程序制作方案及流程-微信程序方案.pdf
- 【java毕业设计】家用电器销售网站源码(ssm+jsp+mysql+说明文档+LW).zip
- 【java毕业设计】固定资产管理系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 如何降低电源的待机功耗
- Java基础面试题梳理及其关键知识点解析
- 【java毕业设计】个性化影片推荐系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 课堂作业-基于PHP实现功能简单的学生管理系统+项目源码+文档说明
- 【java毕业设计】个人交友网站源码(ssm+jsp+mysql+说明文档+LW).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


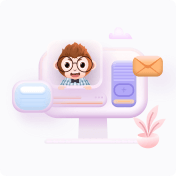
安全验证
文档复制为VIP权益,开通VIP直接复制
