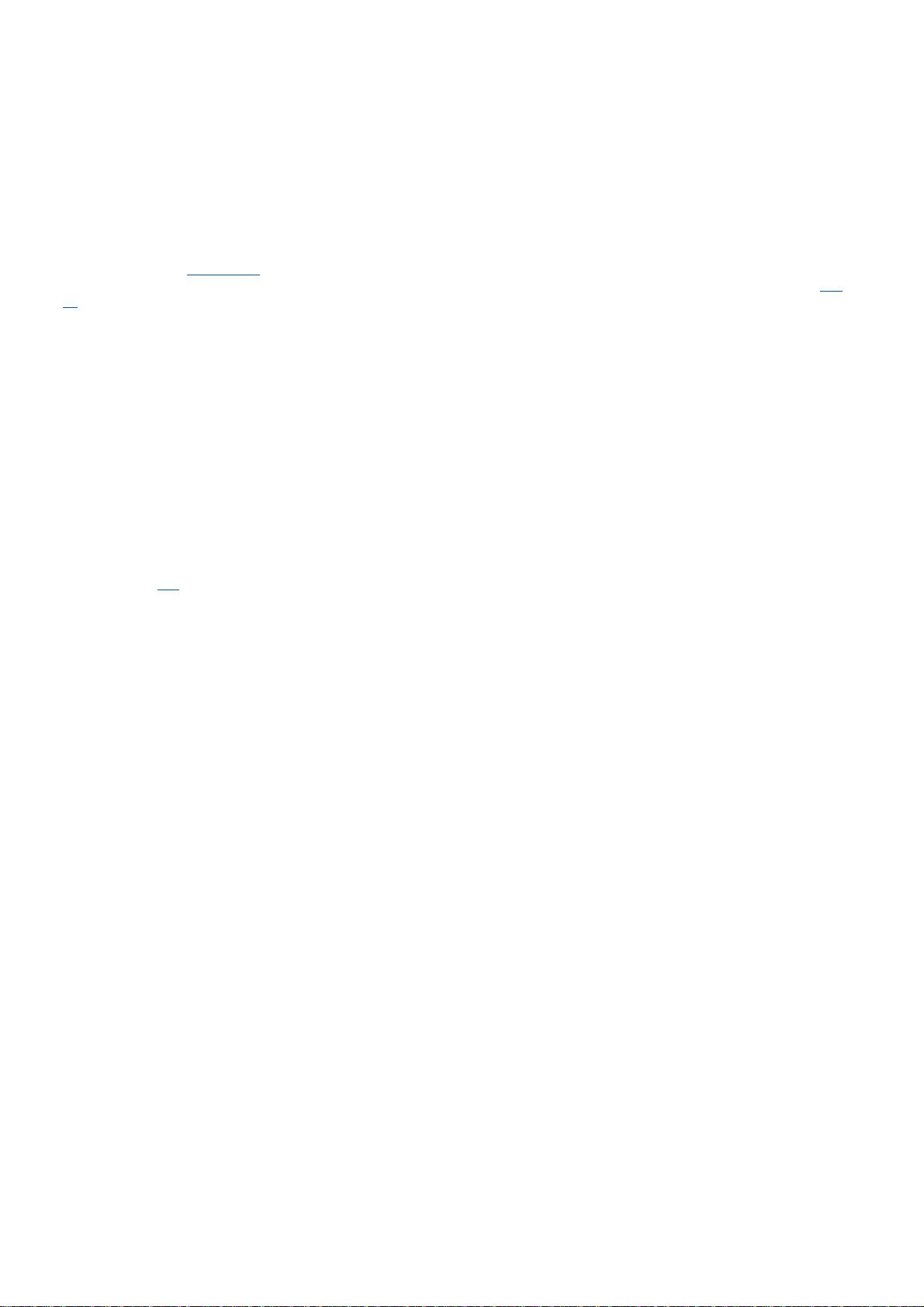
Using Matlab with TinyOS
Last updated 28 April 2005
This tutorial explains how to interact with a mote or sensor network using Matlab. Matlab is matrix-based
programming language with a number of libraries available for plotting and analyzing data. It provides a
command-line like environment (like your bash shell) from which you can send/receive messages to/from your
sensor network and analyze data. You can also use all of TinyOS's Java tools from within the Matlab
environment.
This tutorial will show how to write a simple Oscilloscope application similar to the Java Oscope application
that you used in Tutorial 6
. Before you begin this tutorial, be sure that you have read Lesson 6, that you
have a node connected to your PC that is running the TinyOS Oscilloscope application, and that you have set
up your Matlab environment to work with TinyOS.
Understanding the Basics
The first step in using Matlab with TinyOS is to connect to your Matlab session to your sensor network using
the connect command. This command takes a single argument using the same notation that serialForwarder uses
to indicate how it should connect. For example, you can connect directly to your serial port, to a
serialForwarder, or to an instance of TOSSIM.
connect('serial@COM1:telosb');
connect('sf@localhost:9001);
This command can be called multiple times to connect your Matlab session to several different ports. For
example, you could be connected to a real network as well as an instance of TOSSIM.
The second step is to send a message to the network using the send command, which takes two arguments: 1) a
node ID and 2) a MIG message (MIG is a tool that automatically generates Java representations of TinyOS
packets. see MIG
). We will be using the Oscope MIG objects, so make sure you compile them in the tinyos-
1.x/tools/java/net/tinyos/oscope directory. Then, we need to instantiate the MIG message, which is a Java
class that is a subclass of net.tinyos.message.Message. In Matlab, we can instantiate java objects on the
command line as follows:
msg=net.tinyos.oscope.OscopeResetMsg
Once the java object is instantiated, we can send it to the network:
send(1, msg)
This command automatically addresses the message to node 1. However, it could also be addressed to all nodes
(just as in TinyOS) using TOS_BCAST_ADDR, which is available in a predefined global struct called COMM:
send(COMM.TOS_BCAST_ADDR, msg)
When used with only two arguments, the send command sends to all ports to which the Matlab session is
connected. You may also pass optional parameters to specify that the packet should only be send to certain
ports:
send(COMM.TOS_BCAST_ADDR, msg, serial@COM1:telosb, sf@localhost:9001, ...);
The next step is to receive messages from our network using the receive command, which takes two parameters:
1) the Matlab function that will handle the incoming message events and 2) an example instance of the MIG
object that should be received. We would like to receive the OscopeMsg, which is the message type that the
TinyOS Oscilloscope application uses to transmit its data. Therefore, we first instantiate a OscopeMsg
object.
omsg = net.tinyos.oscope.OscopeMsg
Then, we create a Matlab function that takes two parameters: an address and a message. (Look at tools/matlab
/util/printMsg.m as an example). Finally, we register the function as an event handler for messages of this
type:
receive('printMsg', omsg);
Whenever a message of type OscopeMsg is received, the printMsg function will be called with the message
passed to it as a parameter. If you have an Oscilloscope mote attached to serial@COM1 or sf@localhost:9001,
the messages will start appearing on the screen.
Similar to send, receive takes a port, e.g. serial@COM1 or sf@localhost:9001, as an optional third argument
to indicate that this Matlab function should only serve as a message handler for messages on these ports.
TinyOS Tutorial: Using Matlab with TinyOS http://www.tinyos.net/tinyos-1.x/doc/tutorial/matlab.html
第1页 共4页 2011-11-1 23:35