#include "mainwindow.h"
#include "bottleDefectDetector.h"
#include "ui_mainwindow.h"
#include <QTime>
void sleep(unsigned int msec)
{
QTime dieTime = QTime::currentTime().addMSecs(msec);
while( QTime::currentTime() < dieTime )
QCoreApplication::processEvents(QEventLoop::AllEvents, 100);
}
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
QString title = QString::fromLocal8Bit("啤酒瓶口缺陷检测");
setWindowTitle(title);
//初始化
foldPath = "";
isOpen = false;
isStart = false;
isStop = false;
count = 0;
}
MainWindow::~MainWindow()
{
delete ui;
}
/**
* @brief MainWindow::MyRunner 检测控制函数
* @author JueXiao
* @date 2019-4-30 20:05
*/
int MainWindow::MyRunner(){
isStart = true;
std::string pattern_jpg = foldPath +"/*.png";
//获取目录下所有图片文件名
std::vector<cv::String> image_files;
cv::glob(pattern_jpg, image_files);
if (image_files.size() == 0) {
std::cout << "No image files[png]" << std::endl;
return 0;
}
cout<<"image_files.size():"<<image_files.size()<<endl;
cout<<"image_files[0]:"<<image_files.at(0)<<endl;
cout<<"image_files[1]:"<<image_files.at(1)<<endl;
cout<<"image_files[2]:"<<image_files.at(2)<<endl;
bottleDefectDetector bottleDetector; // 创建类对象
for (unsigned int frame = 0; frame < image_files.size(); ++frame) {//
//for(int i = 0; i< filesList.size();++i){
//QString tmp = filesList.at(i);
//qDebug()<<"tmp ="<< tmp;
cout << "当前处理图像:["<<frame<<"]"<< image_files[frame] << endl;
srcFrame = imread(image_files[frame],1);
//srcFrame = imread(foldPath+tmp.toStdString(),1);
count++;
if(srcFrame.empty() || isStop == true){
ui->label_video->clear(); //检测结束或按下结束按钮,清理播放窗口,退出
break;
}
//将图像统一缩放至600x600大小
cv::resize(srcFrame, srcFrame, Size(600, 600), 0, 0, INTER_LINEAR);
//imshow("srcFrame",srcFrame);
//waitKey(2000);
sleep(1000);
//执行检测算法,调用瓶口缺陷检测
bottleDetector.myDetector(srcFrame);
cout << "返回成功,开始显示结果" <<endl;
cvtColor(srcFrame, srcFrame, COLOR_BGR2RGB);
//cvtColor(srcFrame, srcFrame, COLOR_RGB2BGR);
//imshow("Lane Detection Result",srcFrame);
//waitKey(500);
//将Mat转换为QImage进行显示
qimg = QImage((const uchar*)(srcFrame.data),srcFrame.cols,srcFrame.rows, QImage::Format_RGB888);
//ui->label_video->setPixmap(QPixmap::fromImage(qimg));
ui->label_video->setScaledContents(true);
ui->label_video->resize(ui->label_video->width(),ui->label_video->height());
ui->label_video->setPixmap(QPixmap::fromImage(qimg)); //显示图像
ui->label_video->repaint();
//ui->label_video->show();
}
return 0;
}
/**
* @brief MainWindow::on_pushButton_start_detect_clicked 点击开始检测瓶口缺陷
* @author JueXiao
* @date 2019-4-30 19:00
*/
void MainWindow::on_pushButton_start_detect_clicked(){
//正在运行检测中
if(isStart == true){
cout << "debug:正在检测中" << endl;
return;
}
QDir dir(srcDirPath);
QStringList nameFilters;
nameFilters << "*.jpg" << "*.png";
filesList = dir.entryList(nameFilters, QDir::Files|QDir::Readable, QDir::Name);
qDebug()<< "list ="<< filesList;
//初次点击开始,检查是否已经选择目标文件夹
if(isOpen == true){
if(!MyRunner()){
//成功开始检测
//MyRunner();
cout << "debug:检测成功" << endl;
isOpen = false;
isStart = false;
isStop = false;
}
else{
//检测失败
cout << "debug:检测失败" << endl;
}
}
else{
cout << "debug:未选择文件夹" << endl;
QMessageBox box(QMessageBox::Warning,"warning",QString::fromLocal8Bit("请先选择文件夹"));
box.setStandardButtons (QMessageBox::Ok|QMessageBox::Cancel);
box.setButtonText (QMessageBox::Ok,QString::fromLocal8Bit("打开文件夹"));
box.setButtonText (QMessageBox::Cancel,QString::fromLocal8Bit("取消"));
auto select = box.exec ();
if(select == QMessageBox::Ok){
}
return;
}
cout << "debug:结束检测事件" << endl;
}
/**
* @brief MainWindow::on_pushButton_stop_clicked 点击结束
* @author JueXiao
* @date 2019-5-12 19:00
*/
void MainWindow::on_pushButton_stop_clicked(){
if(cap.isOpened())
{
cap.release(); //关闭摄像头
}
//未在运行检测,无法结束
if(isStart == false){
QMessageBox::warning(NULL, "warning", QString::fromLocal8Bit("未在运行检测"), QMessageBox::Yes);
cout << "debug:未在运行检测,无法结束" << endl;
}
else{
//执行结束事件
isOpen = false;
isStart = false;
isStop = true;
cout << "debug:执行结束事件" << endl;
}
}
/**
* @brief MainWindow::closeEvent 关闭窗口事件
* @param event 事件
* @author JueXiao
* @date 2019-4-30 20:20
*/
void MainWindow::closeEvent(QCloseEvent *event){
auto isExit = QMessageBox::information(this, "Tips", QString::fromLocal8Bit("确认退出?"), QMessageBox::Yes | QMessageBox::No);
if(isExit == QMessageBox::Yes)
event->accept();
else
event->ignore();
}
void MainWindow::on_pushButton_openCamera_clicked()
{
cout << "debug:开始打开摄像头事件" << endl;
//VideoCapture cap(0);
cap.open(0);
if(!cap.isOpened())
{
cout << "debug:打开摄像头失败" << endl;
return;
}
while(1)
{
cap >> srcFrame;
cvtColor(srcFrame, srcFrame, COLOR_BGR2RGB);
qimg = QImage((const uchar*)(srcFrame.data),srcFrame.cols,srcFrame.rows, QImage::Format_RGB888);
//ui->label_video->setPixmap(QPixmap::fromImage(qimg));
ui->label_video->setScaledContents(true);
ui->label_video->resize(ui->label_video->width(),ui->label_video->height());
ui->label_video->setPixmap(QPixmap::fromImage(qimg)); //显示图像
ui->label_video->repaint();
}
cout << "debug:结束打开摄像头事件" << endl;
}
void MainWindow::on_pushButton_openFold_clicked()
{
cout << "debug:开始打开文件夹事件" << endl;
srcDirPath = QFileDialog::getExistingDirectory(
this, "choose src Directory",
"/");
if (srcDirPath.isEmpty())
{
cout << "debug:打开文件夹失败" << endl;
return;
}
else
{
qDebug() << "srcDirPath=" << srcDirPath;
srcDirPath += "/";
cout << "debug:打开文件成功" << endl;
foldPath = srcDirPath.toStdString();
cout << "debug:路径是:" << foldPath << endl;
isOpen = true;
}
cout << "debug:结束打开文件夹事件,请点击开始检测!" << endl;
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
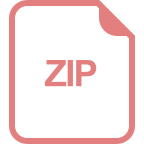
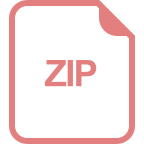
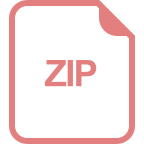
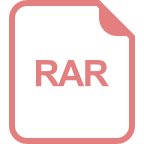
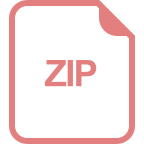
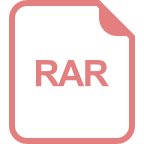
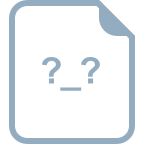
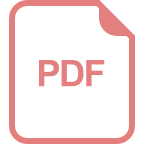
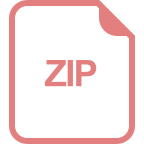
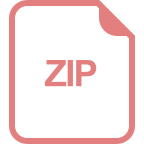
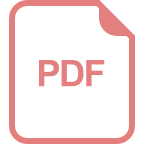
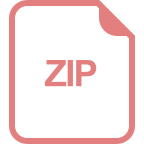
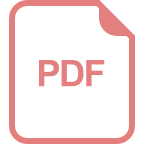
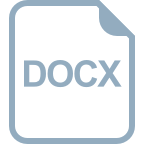
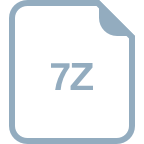
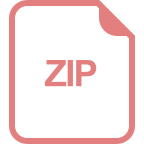
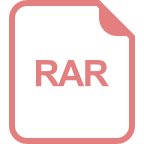
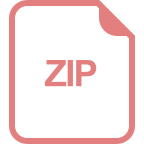
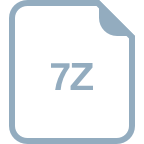
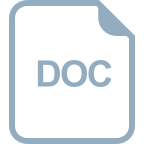
收起资源包目录


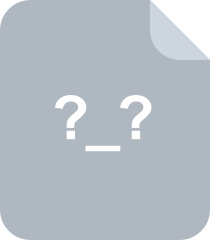
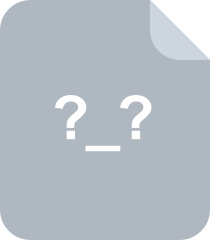
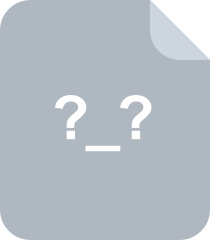
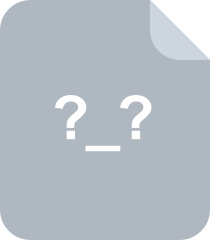
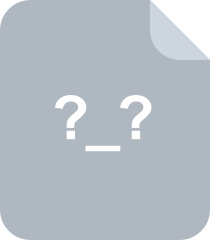
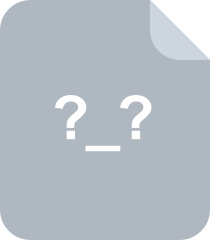
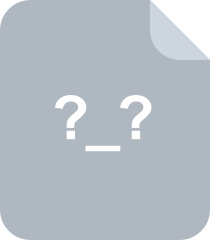
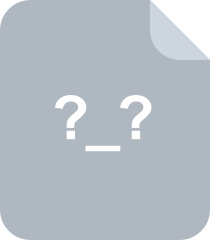
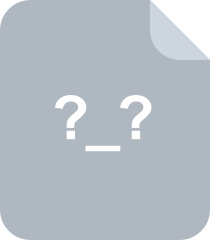
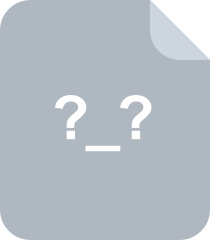
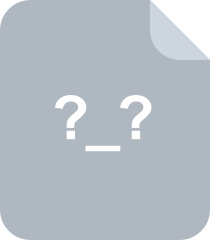

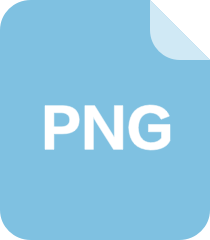
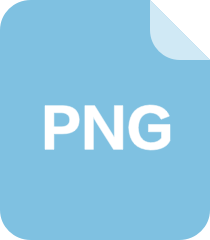
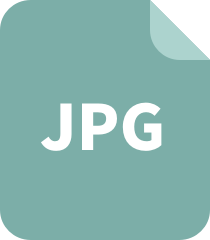
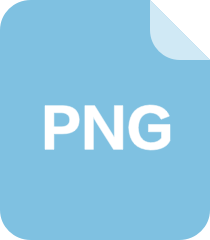
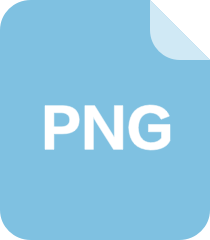
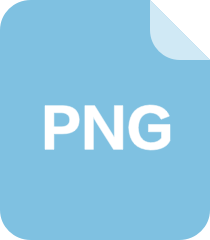
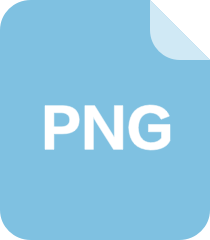
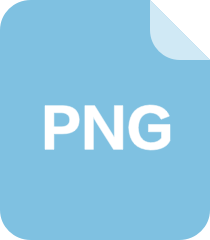
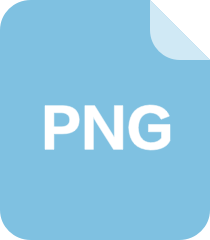
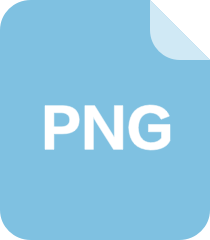
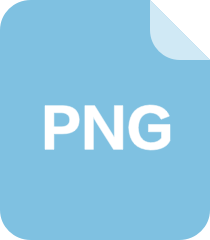
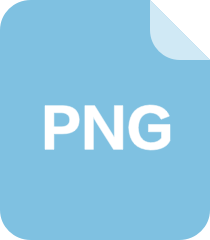
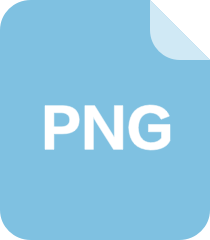
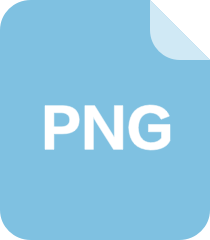
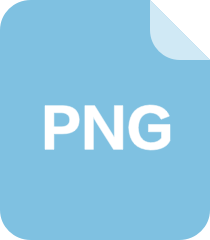
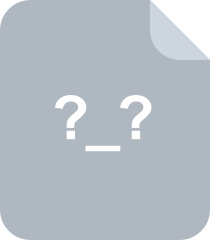
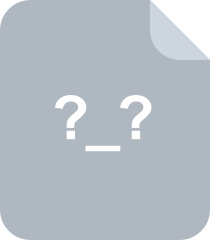
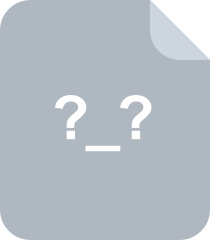
共 29 条
- 1
资源评论
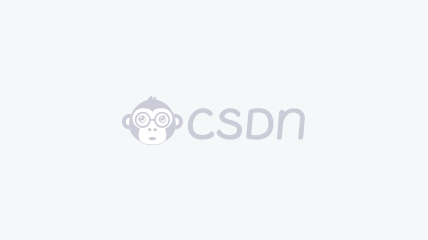

诗眼天涯
- 粉丝: 31
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

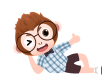
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


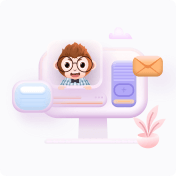
安全验证
文档复制为VIP权益,开通VIP直接复制
