#include <iostream>
#include <iomanip>
#include <stdlib.h>
#include <windows.h>
#include <string>
#include "folder.h"
using namespace std;
string UserName[8] = {"xiaomai", "user1", "user2", "user3",
"user4", "user5","user6", "user7"};
string PassWord[8] = {"12345","12345","12345","12345",
"12345","12345","12345","12345"};
string const Methods[14] = {"create", "open", "read", "write", "copy",
"append", "close", "fdelete",
"mkdir", "cd", "dir", "rmdir",
"help", "logout"};
////static bool file_state = false;
void change()
{
for(int a =0; a<4; a++)
{
Sleep(500);
cout<<'.';
}
}
/*退出*/
void logout()
{
cout<<endl;
cout<<setw(8)<<" Logouting";
change();
system("cls");
}
/*帮助文档函数*/
void Help()
{
system("cls");
system("title 命令帮助 ");
cout<<endl;
char *help = " ★输入命令对照表★\n"
" ☆输入 help 即可查询☆\n"
" →→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→\n"
" \n"
" ★ create <filename> 创建文件 \n"
" ★ open/close <filename> 打开/关闭文件 \n"
" ★ read <filename> 读文件 \n"
" ★ write <filename> 写文件 \n"
" ★ copy <filenameA> <filenameB> 复制文件 \n"
" ★ append <filenameA> <filenameB> 将一个文件内容附加到 \n"
" ★ 另一个文件尾部 \n"
" ★ fdelete <filename> 删除文件 \n"
" \n"
" ★ mkdir <foldername> 创建目录 \n"
" ★ dir 列出文件目录 \n"
" ★ rmdir <foldername> 删除目录 \n"
" \n"
" ★ help 操作帮助 \n"
" ★ logout 用户退出 \n"
" \n"
" →→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→\n";
for(int n=0; n<strlen(help); n++)
{
if(help[n]!=' ')
{
Sleep(1);
}
cout<<help[n];
}
cout<<" 按 enter 键返回!"<<endl;
getchar();
getchar();
system("cls");
return;
}
/*用户操作选择函数*/
void Chose(Folder* &list, Folder* &Target, int Unum, string path)
{
system("title 用户操作界面 ");
string method;
cout<<path;
cin>>method;
//getline(method, '\0');
string A_Name, B_Name;
// const char *o_method;
// o_method = c_method.data();
while("logout" != method)
{
int num = 0;
for(; num<13; num++)
{
if(0 == method.compare (Methods[num]))
{
break;
}
}
/* if((true == file_state))
{
cout<<"非法操作,请先关闭文件!"<<endl;
cin>>method;
continue;
}*/
switch(num)
{
case 0:
cin>>A_Name;
Create(Target, A_Name, Unum);
break;
case 1:
cin>>A_Name;
Open(Target, A_Name);
break;
case 2:
cin>>A_Name;
Read(Target, A_Name);
break;
case 3:
cin>>A_Name;
Write(Target, A_Name, Unum);
break;
case 4:
cin>>A_Name;
cin>>B_Name;
Copy(Target, A_Name, B_Name, Unum);
break;
case 5:
cin>>A_Name;
cin>>B_Name;
Append(Target, A_Name, B_Name, Unum);
break;
case 6:
cin>>A_Name;
Close(Target, A_Name);
break;
case 7:
cin>>A_Name;
Delete(Target, A_Name, Unum);
break;
case 8:
cin>>A_Name;
Mkdir(Target, A_Name, Unum);
break;
case 9:
cin>>A_Name;
Cd(list, Target, A_Name, path);
break;
case 10:
// cin>>A_Name;
Dir(Target);
break;
case 11:
cin>>A_Name;
Rmdir(Target, A_Name, Unum);
break;
case 12:
Help();
break;
default:
cout<<"Input ERROR. Please input correctly again!"<<endl;
break;
}
cout<<path;
cin>>method;
// getline(method, '\0');
//o_method = c_method.data();
}
logout();
return;
}
/*登陆*/
void login(Folder* &list, Folder* &Target, int Unum, string path)
{
// cout<<'\n'<<" 请选择登录用户名,或选择退出系统!"<<endl;
// cout<<
system("title 用户登录界面 ");
cout<<endl<<endl;
cout<<" UserName: ";
string User;
cin>>User;
cout<<'\n'<<" PassWord: ";
string PassW;
cin>>PassW;
int i = 0;
int rest = 3;
BOOL OK = FALSE;
while((OK == FALSE) && (1 != rest))
{
for(; i < 8; i++)
{
if((0 == User.compare (UserName[i])) &&
(0 == PassW.compare (PassWord[i])))
{
OK = TRUE;
Unum = i;
cout<<" LOGIN SUCCEED!"<<endl;
cout<<" Page is changing";
change();
break;
}
}
if(8 == i)
{
rest--;
cout<<setw(8)
<<" UserName OR PassWord ERROR!"<<endl;
cout<<setw(8)
<<" Please input again!"<<"("<<rest<<")"<<endl;
Sleep(1000);
system("cls");
cout<<endl<<endl;
cout<<" UserName: ";
cin>>User;
cout<<'\n'<<" PassWord: ";
cin>>PassW;
i = 0;
}
}
if((OK == FALSE) && (1 == rest))
{
cout<<" You have tried more than 3 times!"
<<'\n'
<<" So, system is locked!"<<endl;
cout<<" Logouting";
change();
system("cls");
logout();
}
else
{
system("cls");
Help();
Chose(list, Target,Unum, path);
}
return;
}
/*程序初始化函数*/
void Begin(Folder* &list, Folder* &Target, string &path)
{
static Folder *tp = new Folder;
Folder *q;
///********C盘********
// tp->Utype = Efolder;
tp->Owner = -1;
// Time(tp->m_time);
tp->m_time = Time();
tp->foldername = "C";
tp->next = NULL;
tp->file = NULL;
tp->folders = NULL;
tp->fparent = NULL;
list = tp;
q = tp;
///********D盘********
tp = new Folder;
// tp->Utype = Efolder;
tp->Owner = -1;
// Time(tp->m_time);
tp->m_time = Time();
tp->foldername = "D";
tp->next = NULL;
tp->file = NULL;
tp->folders = NULL;
tp->fparent = NULL;
q->next = tp;
q = tp;
///********E盘********
tp = new Folder;
// tp->Utype = Efolder;
tp->Owner = -1;
// Time(tp->m_time);
tp->m_time = Time();
tp->foldername = "E";
tp->next = NULL;
tp->file = NULL;
tp->folders = NULL;
tp->fparent = NULL;
q->next = tp;
//q = tp;
Target = list;
path = "C:\\>";
// cout<<path;
}
/*用户登陆选择*/
void Run(Folder* &list, Folder* &Target, int Unum, string path)
{
Label:
system("title 用户选择界面 ");
string enOex;
cout<<'\n'<<" 请选择登录用户名,或选择退出系统!"<<endl;
cout<<'\n'<<" 登录:enter 退出:exit "<<endl;
cout<<'\n'<<" 请键入: ";
cin>>enOex;
while(enOex == "enter")
{
system("cls");
Target = list;
login(list, Target, Unum, path);
system("title 用户选择界面 ");
cout<<'\n'<<" 请选择登录用户名,或选择退出系统!"<<endl;
cout<<'\n'<<" 登录:enter 退出:exit "<<endl;
cout<<'\n'<<" 请键入: ";
cin>>enOex;
}
if(enOex != "exit")
{
system("cls");
system("title 用户选择界面 ");
cout<<endl<<endl
<<endl<<" 警告: �
没有合适的资源?快使用搜索试试~ 我知道了~
模拟UNIX文件系统的设计及实现
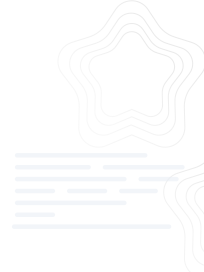
共18个文件
cpp:3个
obj:3个
sbr:2个


温馨提示
即:多用户、多目录的文件系统的设计------用VC或Delphi编程模拟文件系统的管理 多用户的多级目录的文件系统设计。 1. 多用户 :usr1,usr2,usr3,……,usr8 (1-8个用户) 2. 多级目录:可有多级子目录; 3. 具有login (用户登录) 4. 系统初始化(分配内存空间,创建文件卷,初始化根目录) 5. 文件的创建: create 6. 文件的打开:open 7. 文件的读:read 8. 文件的写:write 9. 拷贝文件内容:copy 10. 将一个文件内容附加到另一个文件尾部:append 11. 文件关闭:close 12. 删除文件:delete 13. 创建目录(建立子目录):mkdir 14. 改变当前目录:cd 15. 列出文件目录:dir(包括文件属性) 16. 删除目录:rmdir 17. 退出:logout
资源推荐
资源详情
资源评论
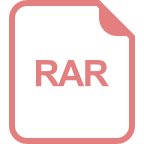
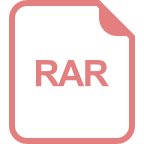
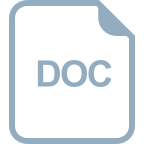
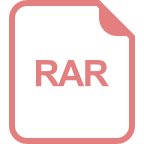
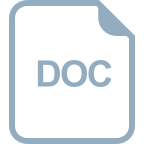
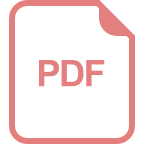
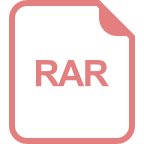
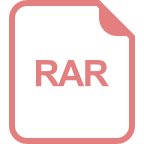
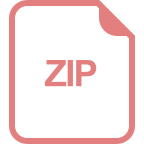
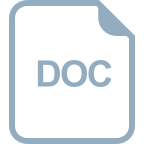
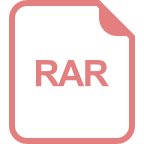
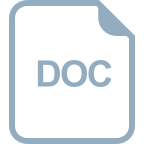
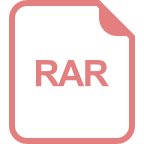
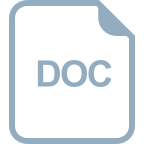
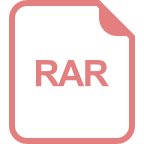
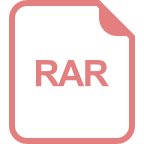
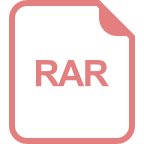
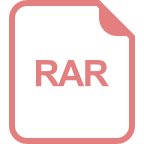
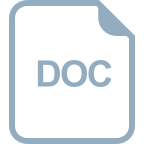
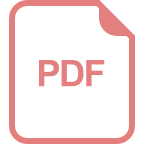
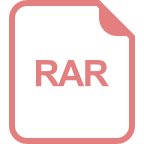
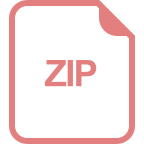
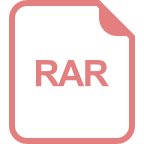
收起资源包目录



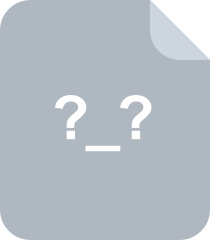
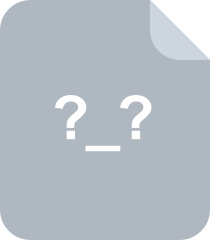
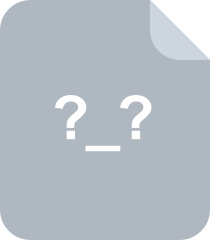
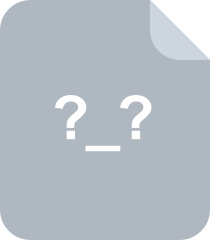
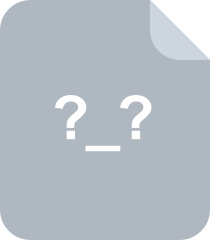
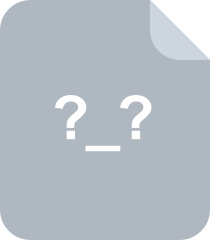

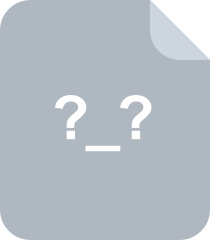
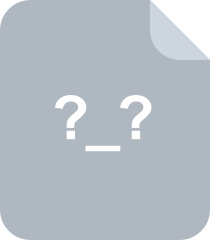
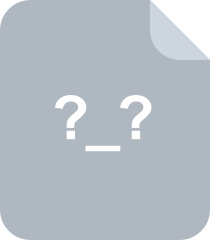
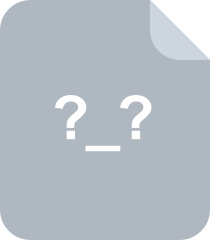
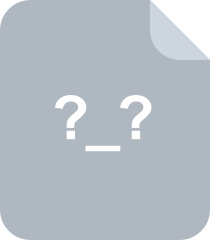
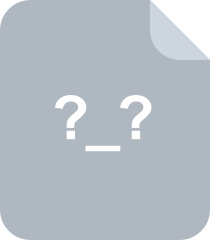
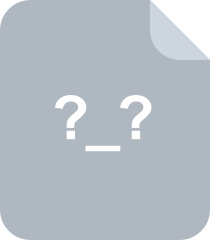
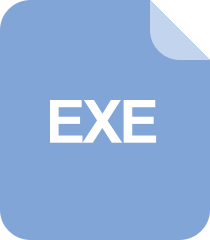
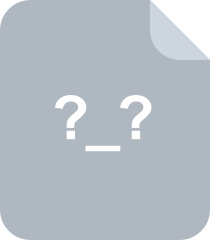
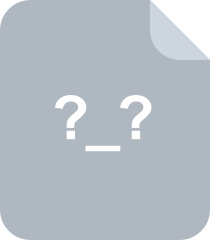
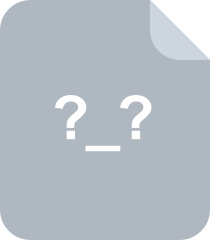
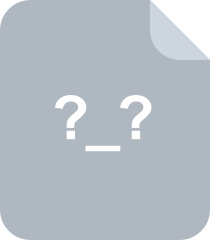
共 18 条
- 1
资源评论
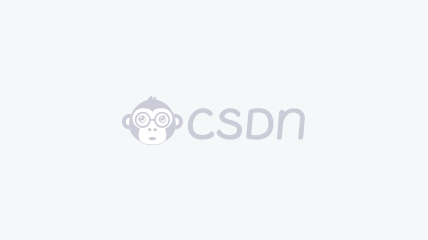
- tylerboy2012-12-12可以,用于参考还是不错的。
- toby27228262012-12-30还行,但是和要求的有点区别
- 提车黄2015-01-22编译过的,一般般
- q11355176172012-03-08模拟的很好,但不是unix文件系统
- Escape__2014-01-03用来参考挺好的

xiao_maijia
- 粉丝: 2
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

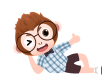
安全验证
文档复制为VIP权益,开通VIP直接复制
