#include "axbopemglwidget.h"
#include "vertices.h"
const unsigned int timeOutmSec=50;
QVector3D viewInitPos(0.0f,5.0f,20.0f);
float _near=0.1f,_far=100.0f;
QMatrix4x4 model;
QMatrix4x4 view;
QMatrix4x4 projection;
QPoint lastPos;
vector<QVector3D> windows;
map<float, QVector3D> sorted;
AXBOpemglWidget::AXBOpemglWidget(QWidget *parent) : QOpenGLWidget(parent)
{
connect(&m_timer,SIGNAL(timeout()),this,SLOT(on_timeout()));
m_timer.start(timeOutmSec);
m_time.start();
m_camera.Position=viewInitPos;
setFocusPolicy(Qt::StrongFocus);
setMouseTracking(true);
windows.push_back(QVector3D(-1.5f, 0.0f, -0.48f));
windows.push_back(QVector3D( 1.5f, 0.0f, 0.51f));
windows.push_back(QVector3D( 0.0f, 0.0f, 0.7f));
windows.push_back(QVector3D(-0.3f, 0.0f, -2.3f));
windows.push_back(QVector3D( 0.5f, 0.0f, -0.6f));
foreach(auto item,windows) {
float distance = m_camera.Position.distanceToPoint(item);
sorted[distance] = item;
}
}
AXBOpemglWidget::~AXBOpemglWidget()
{
for(auto iter=m_Models.begin();iter!=m_Models.end();iter++){
ModelInfo *modelInfo=&iter.value();
delete modelInfo->model;
}
}
void AXBOpemglWidget::loadModel(string path)
{
static int i=0;
makeCurrent();
Model * _model=new Model(QOpenGLContext::currentContext()->versionFunctions<QOpenGLFunctions_3_3_Core>()
,path.c_str());
//m_camera.Position=cameraPosInit(_model->m_maxY,_model->m_minY);
m_Models["Julian"+QString::number(i++)]=
ModelInfo{_model,QVector3D(0,0-_model->m_minY,0)
,0.0,0.0,0.0,false,QString::fromLocal8Bit("张三")+QString::number(i++)};
doneCurrent();
}
void AXBOpemglWidget::initializeGL()
{
initializeOpenGLFunctions();
//创建VBO和VAO对象,并赋予ID
bool success;
m_ShaderProgram.addShaderFromSourceFile(QOpenGLShader::Vertex,":/shaders/shaders/shapes.vert");
m_ShaderProgram.addShaderFromSourceFile(QOpenGLShader::Fragment,":/shaders/shaders/shapes.frag");
success=m_ShaderProgram.link();
if(!success) qDebug()<<"ERR:"<<m_ShaderProgram.log();
m_BoxDiffuseTex=new
QOpenGLTexture(QImage(":/images/images/container2.png").mirrored());
m_WindowDiffuseTex=new
QOpenGLTexture(QImage(":/images/images/blending_transparent_window.png"));
m_PlaneDiffuseTex=new
QOpenGLTexture(QImage(":/images/images/wall.jpg").mirrored());
glEnable(GL_BLEND);
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
m_PlaneMesh=processMesh(planeVertices,6,m_PlaneDiffuseTex->textureId());
m_WindowMesh=processMesh(transparentVertices,6,m_WindowDiffuseTex->textureId());
}
void AXBOpemglWidget::resizeGL(int w, int h)
{
Q_UNUSED(w);
Q_UNUSED(h);
}
void AXBOpemglWidget::paintGL()
{
model.setToIdentity();
view.setToIdentity();
projection.setToIdentity();
// float time=m_time.elapsed()/50.0;
projection.perspective(m_camera.Zoom,(float)width()/height(),_near,_far);
view=m_camera.GetViewMatrix();
glClearColor(0.2f, 0.3f, 0.3f, 1.0f);
glEnable(GL_DEPTH_TEST);
glDepthFunc(GL_LESS);
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
m_ShaderProgram.bind();
m_ShaderProgram.setUniformValue("projection", projection);
m_ShaderProgram.setUniformValue("view", view);
//model.rotate(time, 1.0f, 1.0f, 0.5f);
m_ShaderProgram.setUniformValue("viewPos",m_camera.Position);
// light properties, note that all light colors are set at full intensity
m_ShaderProgram.setUniformValue("light.ambient", 0.7f, 0.7f, 0.7f);
m_ShaderProgram.setUniformValue("light.diffuse", 0.9f, 0.9f, 0.9f);
m_ShaderProgram.setUniformValue("light.specular", 1.0f, 1.0f, 1.0f);
// material properties
m_ShaderProgram.setUniformValue("material.shininess", 32.0f);
m_ShaderProgram.setUniformValue("light.direction", -0.2f, -1.0f, -0.3f);
m_ShaderProgram.setUniformValue("model", model);
m_PlaneMesh->Draw(m_ShaderProgram);
foreach(auto modelInfo,m_Models){
model.setToIdentity();
model.translate(modelInfo.worldPos);
model.rotate(modelInfo.pitch,QVector3D(1.0,0.0,0.0));
model.rotate(modelInfo.yaw,QVector3D(0.0,1.0,0.0));
model.rotate(modelInfo.roll,QVector3D(0.0,0.0,1.0));
m_ShaderProgram.setUniformValue("model", model);
modelInfo.model->Draw(m_ShaderProgram);
}
for(map<float,QVector3D>::reverse_iterator riter=sorted.rbegin();
riter!=sorted.rend();riter++){
model.setToIdentity();
model.translate(riter->second);
m_ShaderProgram.setUniformValue("model", model);
m_WindowMesh->Draw(m_ShaderProgram);
}
}
void AXBOpemglWidget::wheelEvent(QWheelEvent *event)
{
m_camera.ProcessMouseScroll(event->angleDelta().y()/120);
}
void AXBOpemglWidget::keyPressEvent(QKeyEvent *event)
{
float deltaTime=timeOutmSec/1000.0f;
switch (event->key()) {
case Qt::Key_W: m_camera.ProcessKeyboard(FORWARD,deltaTime);break;
case Qt::Key_S: m_camera.ProcessKeyboard(BACKWARD,deltaTime);break;
case Qt::Key_D: m_camera.ProcessKeyboard(RIGHT,deltaTime);break;
case Qt::Key_A: m_camera.ProcessKeyboard(LEFT,deltaTime);break;
case Qt::Key_Q: m_camera.ProcessKeyboard(DOWN,deltaTime);break;
case Qt::Key_E: m_camera.ProcessKeyboard(UP,deltaTime);break;
case Qt::Key_Space: m_camera.Position=viewInitPos;break;
default:break;
}
}
void AXBOpemglWidget::mouseMoveEvent(QMouseEvent *event)
{
makeCurrent();
if(m_modelMoving){
for(auto iter=m_Models.begin();iter!=m_Models.end();iter++){
ModelInfo *modelInfo=&iter.value();
if(!modelInfo->isSelected) continue;
modelInfo->worldPos=
QVector3D(worldPosFromViewPort(event->pos().x(),event->pos().y()));
}
}else
if(event->buttons() & Qt::RightButton
|| event->buttons() & Qt::LeftButton
|| event->buttons() & Qt::MiddleButton){
auto currentPos=event->pos();
QPoint deltaPos=currentPos-lastPos;
lastPos=currentPos;
if(event->buttons() & Qt::RightButton)
m_camera.ProcessMouseMovement(deltaPos.x(),-deltaPos.y());
else
for(auto iter=m_Models.begin();iter!=m_Models.end();iter++){
ModelInfo *modelInfo=&iter.value();
if(!modelInfo->isSelected) continue;
if(event->buttons() & Qt::MiddleButton){
modelInfo->roll+=deltaPos.x();
}
else if(event->buttons() & Qt::LeftButton){
modelInfo->yaw+=deltaPos.x();
modelInfo->pitch+=deltaPos.y();
}
}
}
doneCurrent();
}
void AXBOpemglWidget::mousePressEvent(QMouseEvent *event)
{
bool hasSelected=false;
makeCurrent();
lastPos=event->pos();
if(event->buttons()&Qt::LeftButton){
QVector4D wolrdPostion=worldPosFromViewPort(event->pos().x(),
event->pos().y());
mousePickingPos(QVector3D(wolrdPostion));
for(QMap<QString, ModelInfo>::iterator iter=m_Models.begin();iter!=m_Models.end();iter++){
ModelInfo *modelInfo=&iter.value();
float r=(modelInfo->model->m_maxY-modelInfo->model->m_minY)/2;
if(modelInfo->worldPos.distanceToPoint(QVector3D(wolrdPostion))<r
&&!hasSelected){
modelInfo->isSelected=true;
hasSelected=true;
}
else
modelInfo->isSelected=false;
// qDebug()<<model
没有合适的资源?快使用搜索试试~ 我知道了~
OpenGL 混合
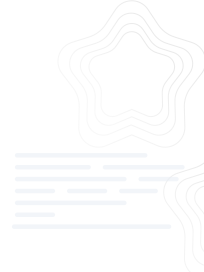
共121个文件
h:76个
hpp:9个
inl:8个

2 下载量 195 浏览量
2023-06-19
20:48:40
上传
评论
收藏 9.02MB ZIP 举报
温馨提示
OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenGL 混合OpenG
资源推荐
资源详情
资源评论
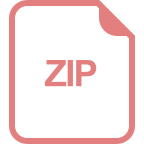
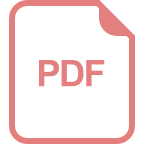
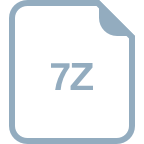
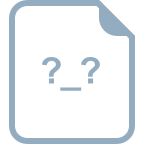
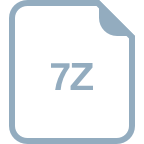
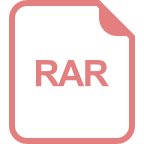
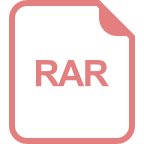
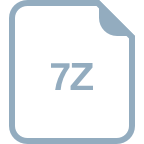
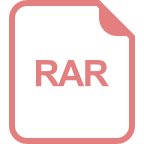
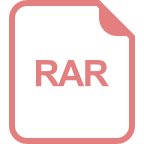
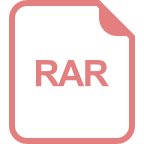
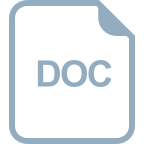
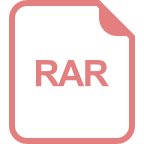
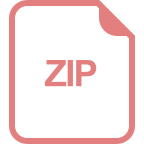
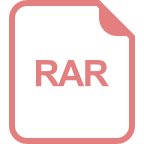
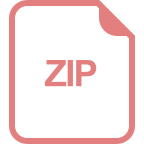
收起资源包目录

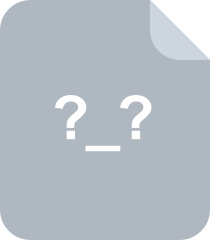
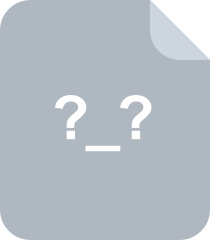
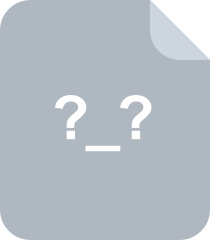
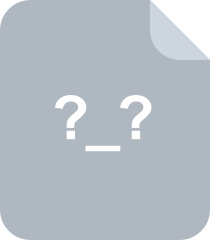
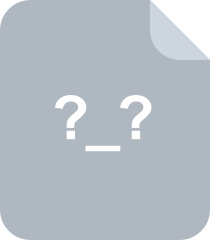
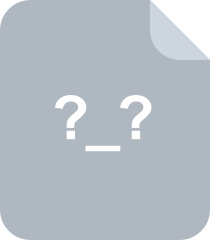
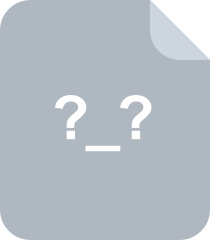
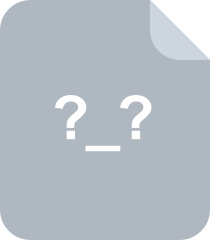
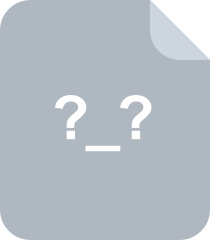
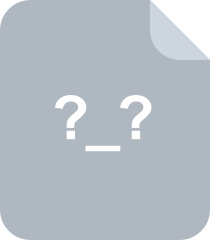
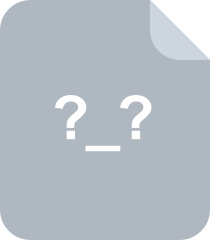
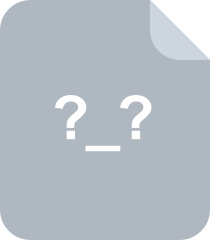
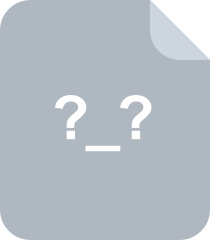
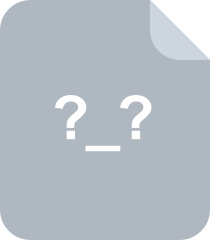
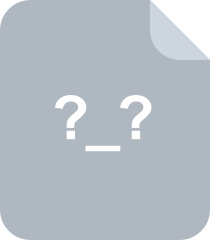
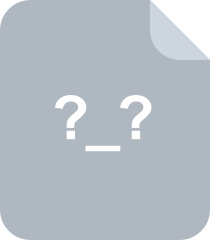
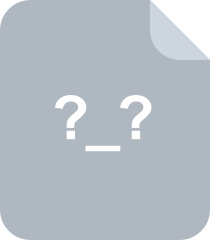
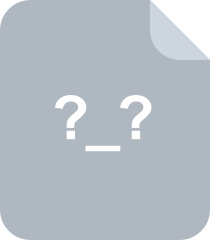
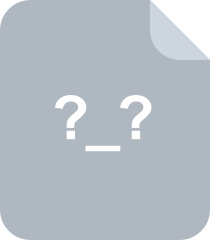
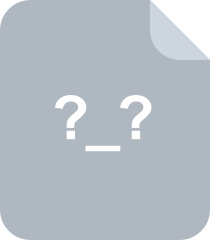
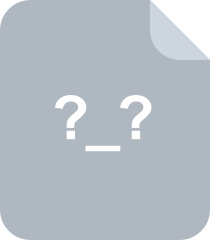
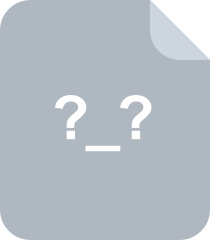
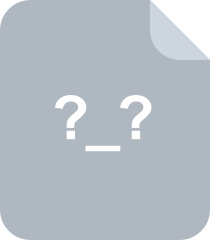
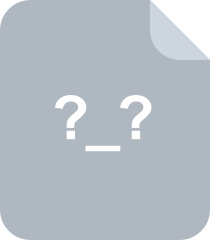
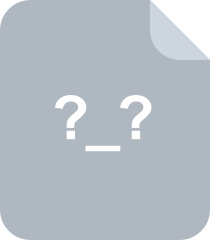
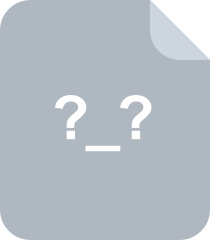
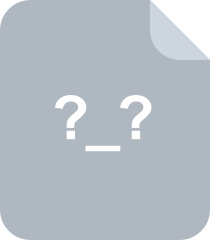
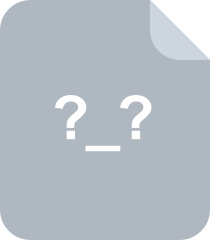
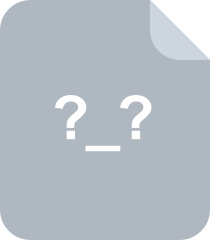
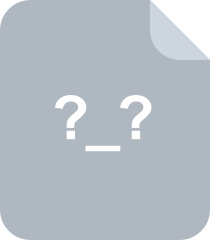
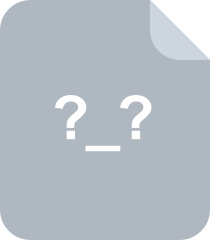
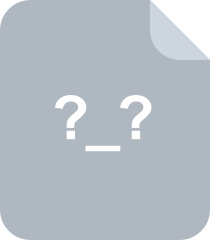
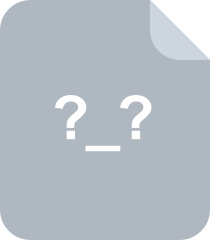
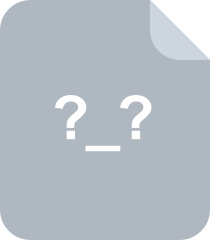
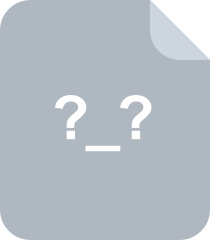
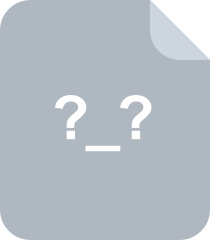
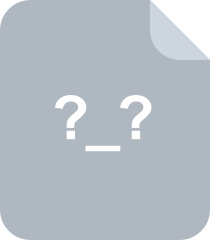
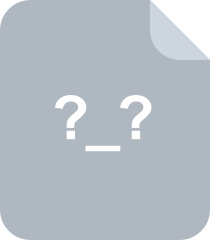
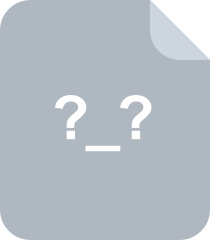
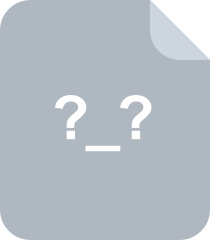
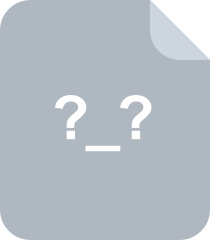
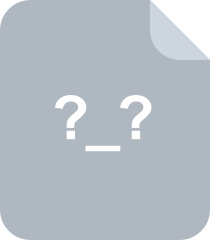
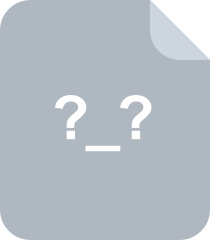
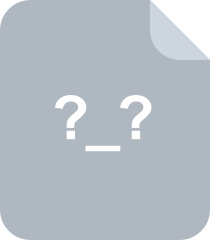
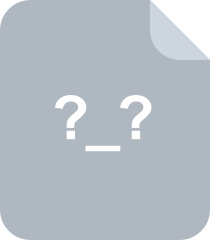
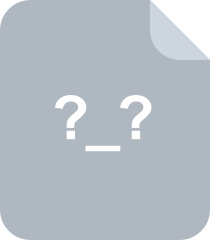
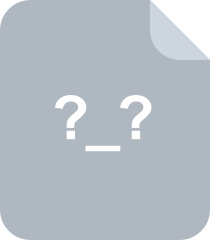
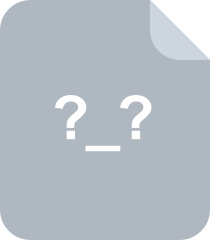
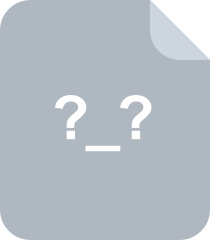
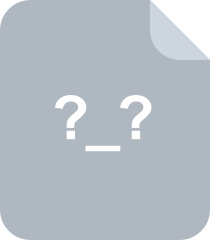
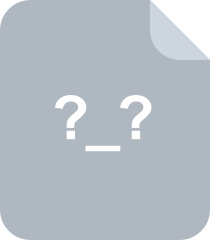
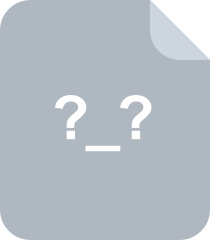
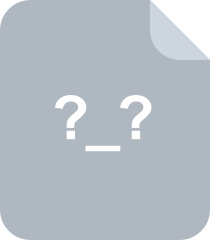
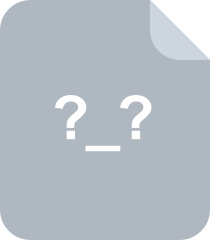
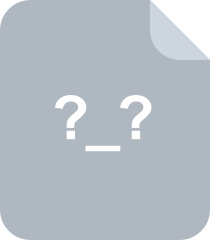
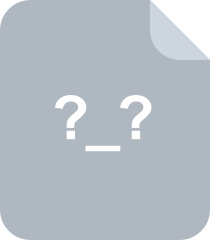
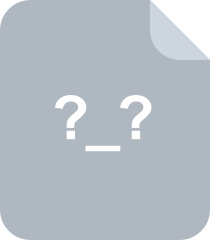
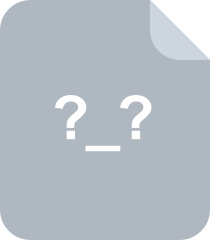
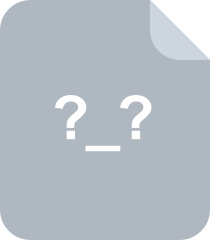
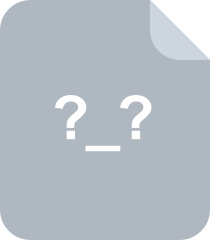
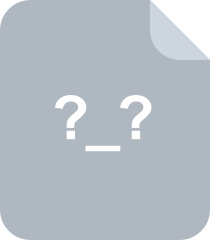
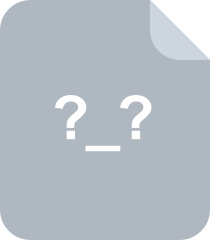
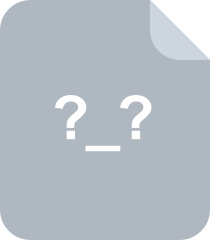
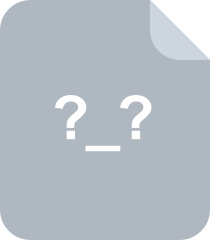
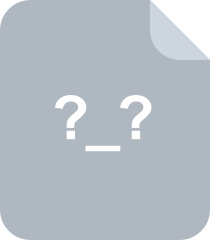
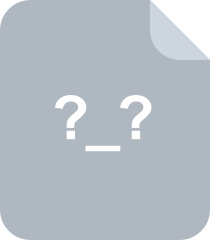
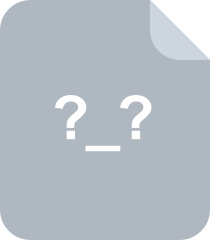
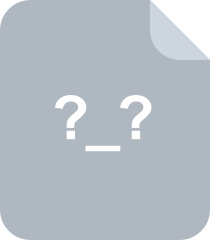
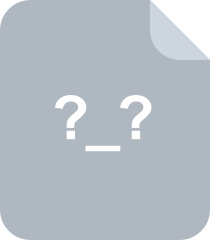
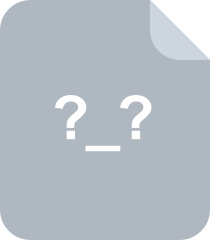
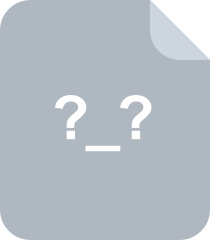
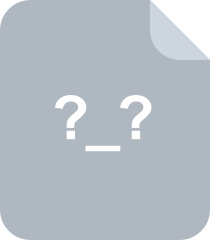
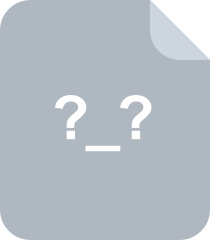
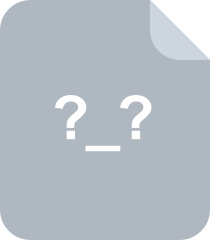
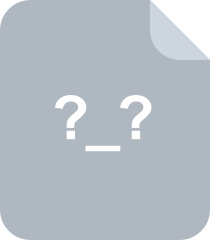
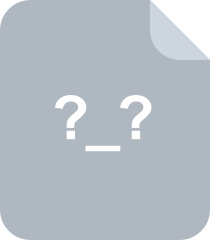
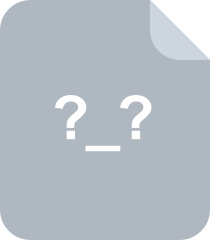
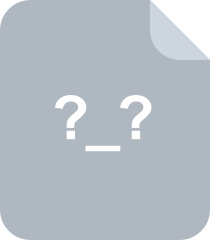
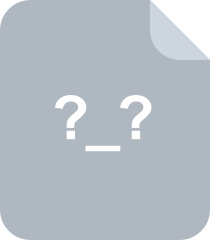
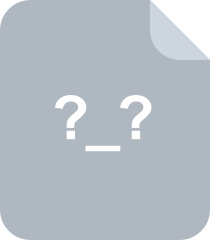
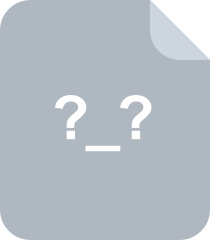
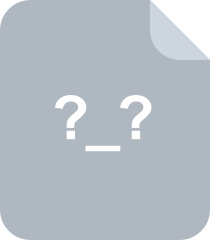
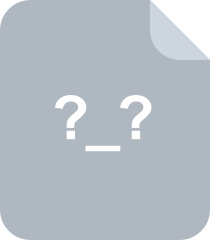
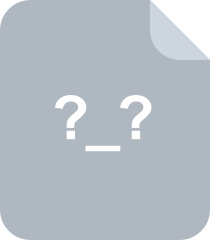
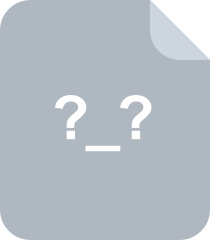
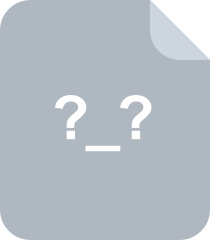
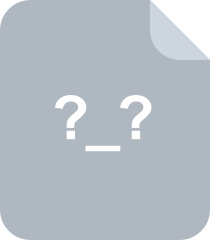
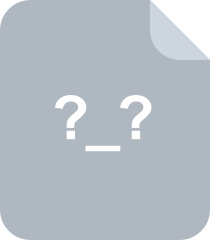
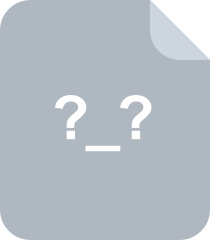
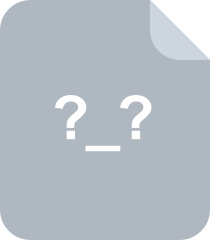
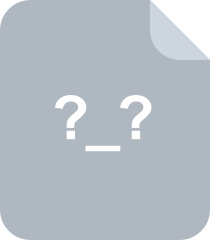
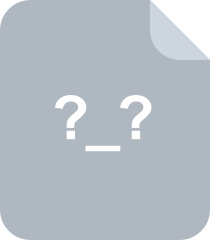
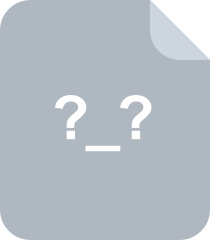
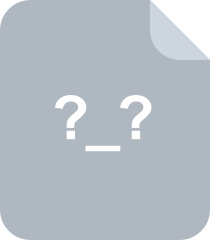
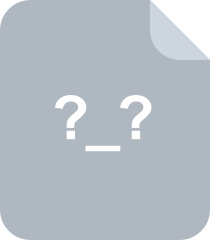
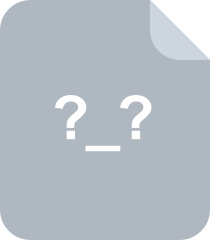
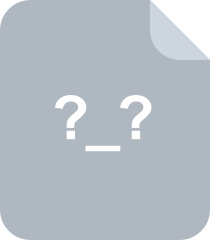
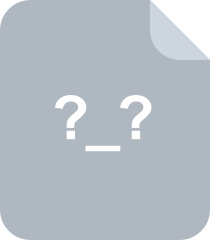
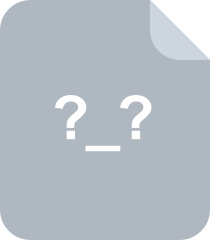
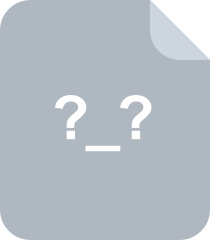
共 121 条
- 1
- 2
资源评论
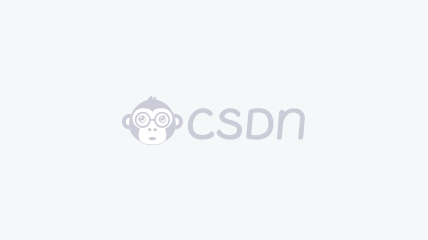

灬Sunnnnn
- 粉丝: 3w+
- 资源: 88
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

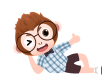
最新资源
- 基于Java开发的日程管理FlexTime应用设计源码
- SM2258XT-BGA144-4BGA180-6L-R1019 三星KLUCG4J1CB B0B1颗粒开盘工具 , EC, 3A, 94, 43, A4, CA 七彩虹SL300这个固件有用
- GJB 5236-2004 军用软件质量度量
- 30天开发操作系统 第 8 天 - 鼠标控制与切换32模式
- spice vd interface接口
- 安装Git时遇到找不到`/dev/null`的问题
- 标量(scalar)、向量(vector)、矩阵(matrix)、数组(array)等概念的深入理解与运用
- 数值计算复习内容,涵盖多种方法,内容为gpt生成
- 标量(scalar)、向量(vector)、矩阵(matrix)、数组(array)等概念的深入理解与运用
- 网络综合项目实验12.19
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


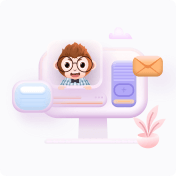
安全验证
文档复制为VIP权益,开通VIP直接复制
