没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论

















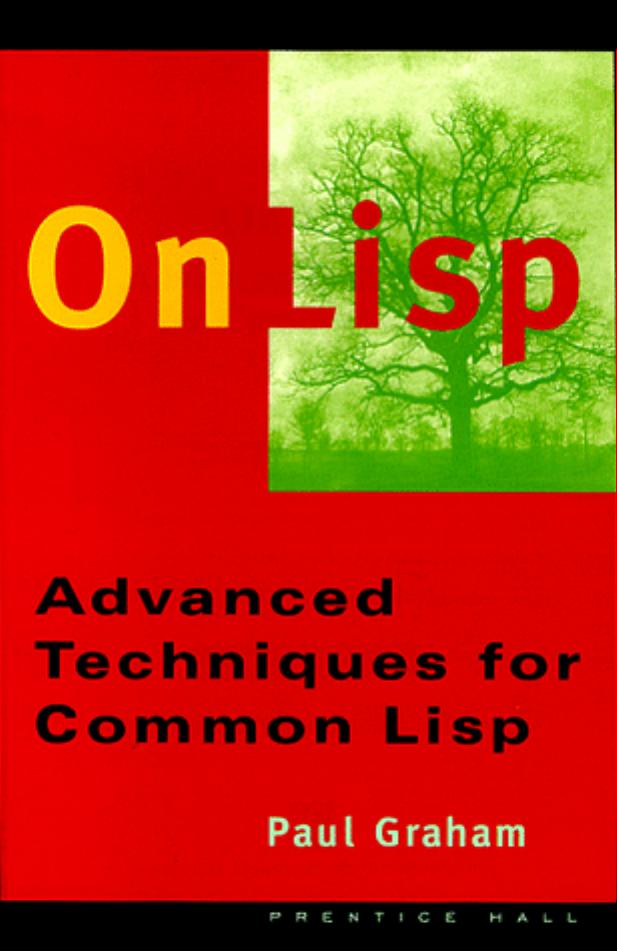
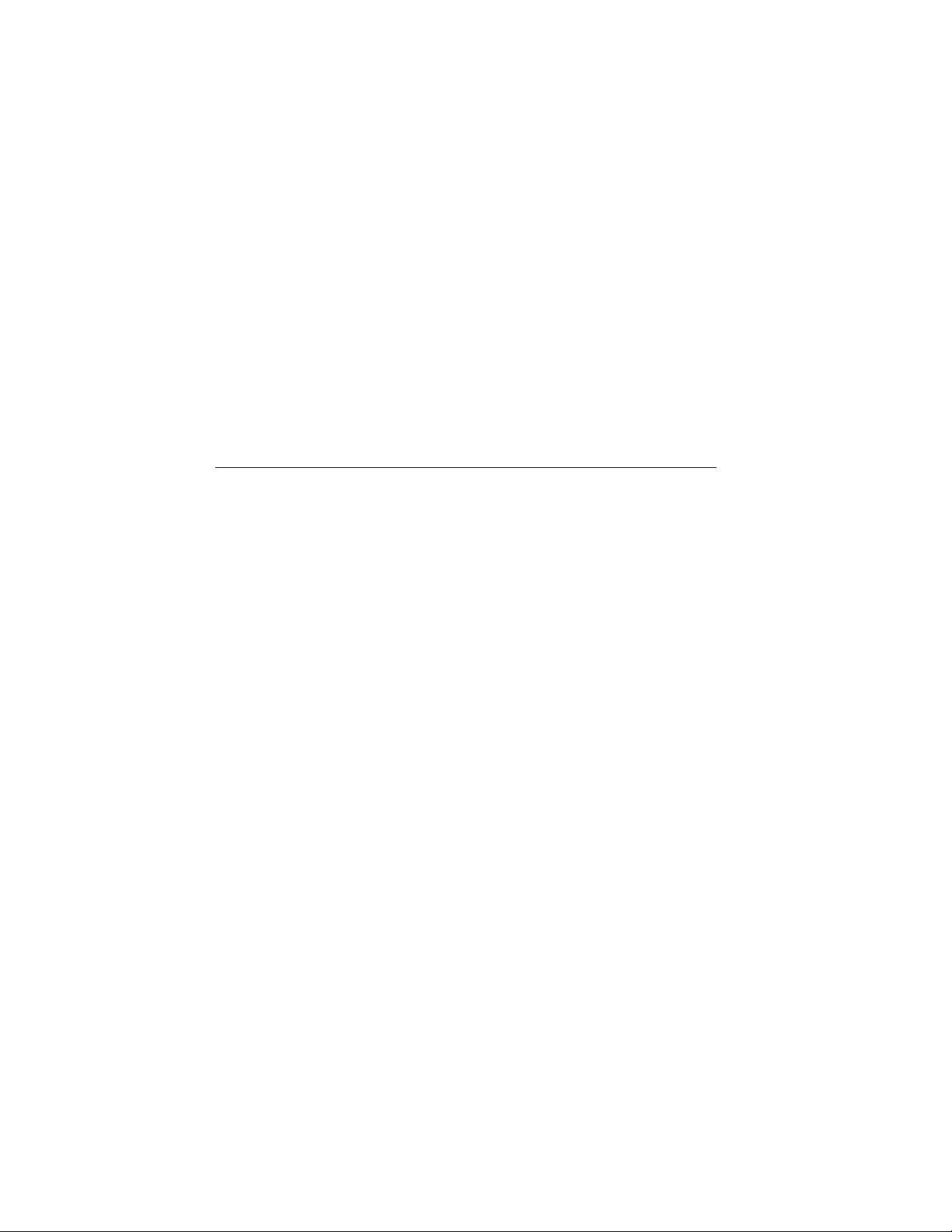
Preface
This book is intended for anyone who wants to become a better Lisp programmer.
It assumes some familiarity with Lisp, but not necessarily extensive programming
experience. The first few chapters contain a fair amount of review. I hope that
these sections will be interesting to more experienced Lisp programmers as well,
because they present familiar subjects in a new light.
It’s difficultto conveythe essence of a programminglanguage in one sentence,
but John Foderaro has come close:
Lisp is a programmable programming language.
There is more to Lisp than this, but the ability to bend Lisp to one’s will is a
large part of what distinguishes a Lisp expert from a novice. As well as writing
their programs down toward the language, experienced Lisp programmers build
the language up toward their programs. This book teaches how to program in the
bottom-up style for which Lisp is inherently well-suited.
Bottom-up Design
Bottom-up design is becoming more important as software grows in complexity.
Programs today may have to meet specifications which are extremely complex,
or even open-ended. Under such circumstances, the traditional top-down method
sometimes breaks down. In its place there has evolved a style of programming
v
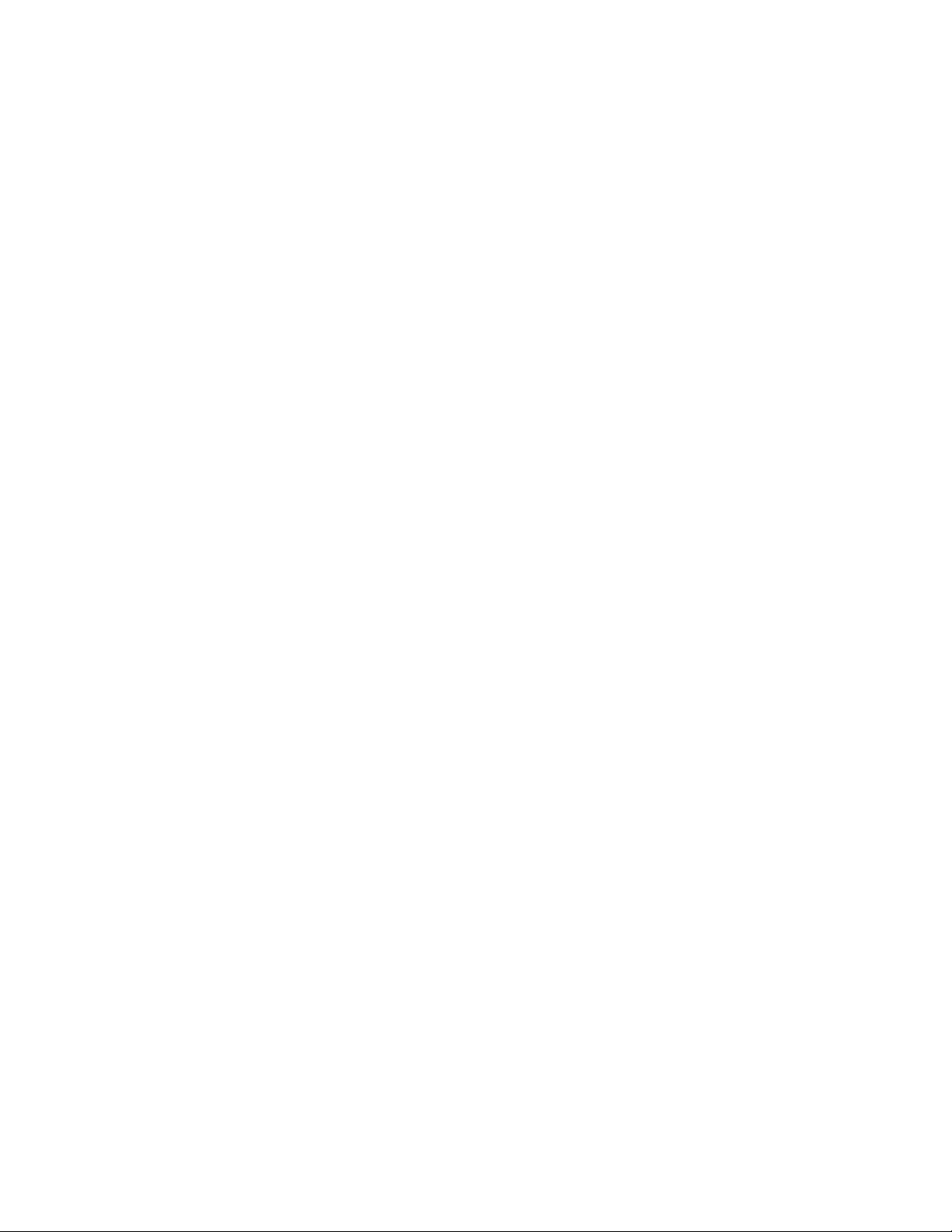
vi PREFACE
quite different from what is currently taught in most computer science courses:
a bottom-up style in which a program is written as a series of layers, each one
acting as a sort of programming language for the one above. X Windows and T
E
X
are examples of programs written in this style.
The theme of this book is twofold: that Lisp is a natural language for programs
written in the bottom-up style, and that the bottom-up style is a natural way to
write Lisp programs. On Lisp will thus be of interest to two classes of readers.
For people interested in writing extensible programs, this book will show what
you can do if you have the right language. For Lisp programmers, this book offers
a practical explanation of how to use Lisp to its best advantage.
The title is intended to stress the importance of bottom-up programming in
Lisp. Instead of just writing your program in Lisp, you can write your own
language on Lisp, and write your program in that.
It is possible to write programs bottom-up in any language, but Lisp is the
most natural vehicle for this style of programming. In Lisp, bottom-up design is
not a special technique reserved for unusually large or difficult programs. Any
substantial program will be written partly in this style. Lisp was meant from the
start to be an extensible language. The language itself is mostly a collection of
Lisp functions, no different from the ones you define yourself. What’s more, Lisp
functions can be expressed as lists, which are Lisp data structures. This means
you can write Lisp functions which generate Lisp code.
A good Lisp programmer must know how to take advantageof this possibility.
The usual way to do so is by defining a kind of operator called a macro. Mastering
macros is one of the most important steps in moving from writing correct Lisp
programs to writing beautiful ones. Introductory Lisp books have room for no
morethan a quickoverviewofmacros: anexplanationofwhat macrosare,together
with a few examples which hint at the strange and wonderful things you can do
with them. Those strange and wonderful things will receive special attention here.
One of the aims of this book is to collect in one place all that people have till now
had to learn from experience about macros.
Understandably, introductory Lisp books do not emphasize the differences
between Lisp and other languages. They have to get their message across to
students who have, for the most part, been schooled to think of programs in Pascal
terms. It would only confuse matters to explain that, while defun looks like a
procedure definition, it is actually a program-writing program that generates code
which builds a functional object and indexes it under the symbol given as the first
argument.
One of the purposes of this book is to explain what makes Lisp different from
other languages. When I began, I knew that, all other things being equal, I would
much rather write programs in Lisp than in C or Pascal or Fortran. I knew also that
this was not merely a question of taste. But I realized that if I was actually going
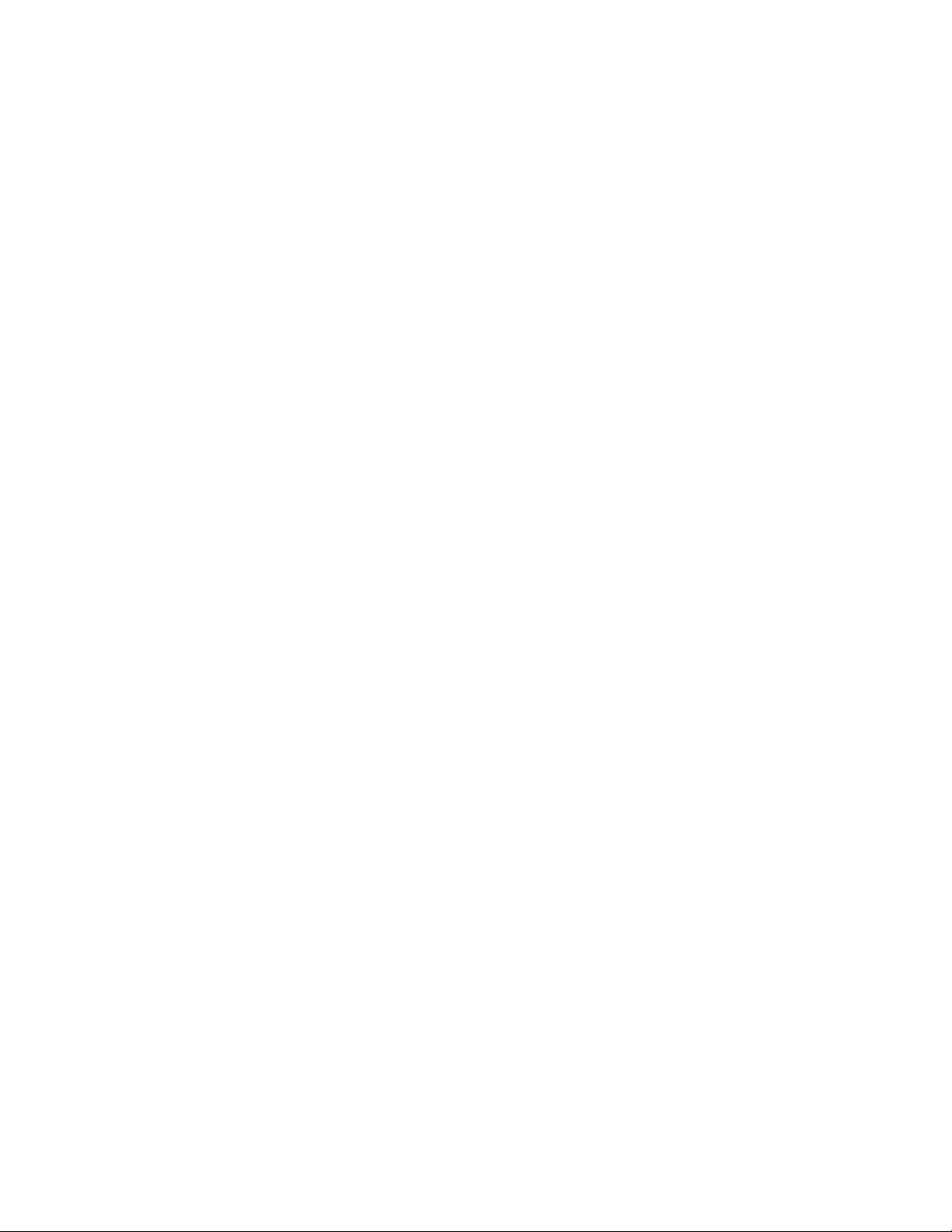
PREFACE vii
to claim that Lisp was in some ways a better language, I had better be prepared to
explain why.
When someone asked Louis Armstrong what jazz was, he replied “If you have
to ask what jazz is, you’ll never know.” But he did answer the question in a way:
he showed people what jazz was. That’s one way to explain the power of Lisp—to
demonstrate techniques that would be difficult or impossible in other languages.
Most books on programming—even books on Lisp programming—deal with the
kinds of programs you could write in any language. On Lisp deals mostly with
the kinds of programs you could only write in Lisp. Extensibility, bottom-up
programming, interactive development, source code transformation, embedded
languages—this is where Lisp shows to advantage.
In principle, of course, any Turing-equivalent programming language can do
the same things as any other. But that kind of power is not what programming
languages are about. In principle, anything you can do with a programming
language you can do with a Turing machine; in practice, programming a Turing
machine is not worth the trouble.
So when I say that this book is about how to do things that are impossible
in other languages, I don’t mean “impossible” in the mathematical sense, but in
the sense that matters for programming languages. That is, if you had to write
some of the programs in this book in C, you might as well do it by writing a Lisp
compiler in C first. Embedding Prolog in C, for example—can you imagine the
amount of work that would take? Chapter 24 shows how to do it in 180 lines of
Lisp.
I hoped to do more than simply demonstrate the power of Lisp, though. I also
wantedto explain why Lisp is different. This turnsout to be a subtle question—too
subtle to be answered with phrases like “symbolic computation.” What I have
learned so far, I have tried to explain as clearly as I can.
Plan of the Book
Since functions are the foundation of Lisp programs, the book begins with sev-
eral chapters on functions. Chapter 2 explains what Lisp functions are and the
possibilities they offer. Chapter 3 then discusses the advantages of functional
programming, the dominant style in Lisp programs. Chapter 4 shows how to use
functions to extend Lisp. Then Chapter 5 suggests the new kinds of abstractions
we can define with functions that return other functions. Finally, Chapter 6 shows
how to use functions in place of traditional data structures.
The remainder of the book deals more with macros than functions. Macros
receive more attention partly because there is more to say about them, and partly
because they have not till now been adequately described in print. Chapters 7–10
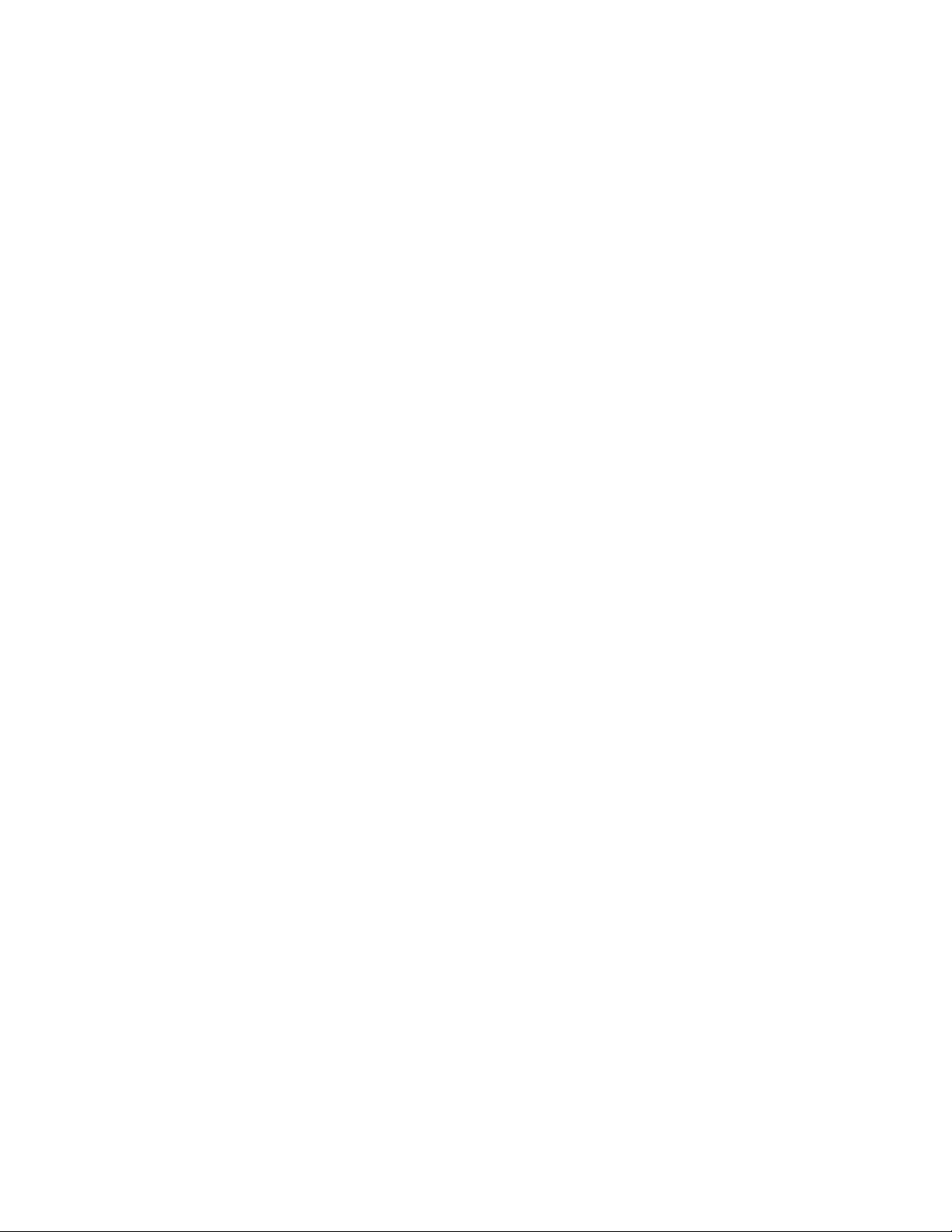
viii PREFACE
form a complete tutorial on macro technique. By the end of it you will know most
of what an experienced Lisp programmer knows about macros: how they work;
how to define, test, and debug them; when to use macros and when not; the major
types of macros; how to write programs which generate macro expansions; how
macro style differs from Lisp style in general; and how to detect and cure each of
the unique problems that afflict macros.
Following this tutorial, Chapters 11–18 show some of the powerful abstrac-
tions you can build with macros. Chapter 11 shows how to write the classic
macros—those which create context, or implement loops or conditionals. Chap-
ter 12 explains the role of macros in operations on generalized variables. Chap-
ter 13 shows how macros can make programs run faster by shifting computation
to compile-time. Chapter 14 introduces anaphoric macros, which allow you to
use pronouns in your programs. Chapter 15 shows how macros provide a more
convenient interface to the function-builders defined in Chapter 5. Chapter 16
shows how to use macro-defining macros to make Lisp write your programs for
you. Chapter 17 discusses read-macros, and Chapter 18, macros for destructuring.
With Chapter 19 begins the fourth part of the book, devoted to embedded
languages. Chapter 19 introduces the subject by showing the same program, a
program to answer queries on a database, implemented first by an interpreter
and then as a true embedded language. Chapter 20 shows how to introduce
into Common Lisp programs the notion of a continuation, an object representing
the remainder of a computation. Continuations are a very powerful tool, and
can be used to implement both multiple processes and nondeterministic choice.
Embedding these control structures in Lisp is discussed in Chapters 21 and 22,
respectively. Nondeterminism, which allows you to write programs as if they
had foresight, sounds like an abstraction of unusual power. Chapters 23 and 24
present two embedded languages which show that nondeterminism lives up to its
promise: a complete
ATN parser and an embedded Prolog which combined total
about 200 lines of code.◦
Thefactthat theseprogramsare shortmeansnothing initself. If youresortedto
writing incomprehensible code, there’s no telling what you could do in 200 lines.
The point is, these programs are not short because they depend on programming
tricks, but because they’re written using Lisp the way it’s meant to be used. The
point of Chapters 23 and 24 is not how to implement
ATNs in one page of code
or Prolog in two, but to show that these programs, when given their most natural
Lisp implementation, simply are that short. The embedded languages in the latter
chapters provide a proof by example of the twin points with which I began: that
Lisp is a natural language for bottom-up design, and that bottom-up design is a
natural way to use Lisp.
The book concludes with a discussion of object-oriented programming, and
particularly
CLOS, the Common Lisp Object System. By saving this topic till
剩余422页未读,继续阅读
资源评论
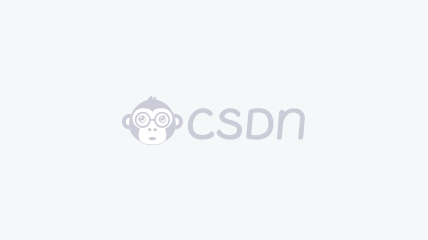

「已注销」
- 粉丝: 6
- 资源: 48
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

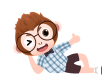
最新资源
- 基于c++、easyx库的玫瑰花效果显示
- PQ控制三相并网逆变器:功率控制、PWM调制与LCL滤波器的应用及仿真模型学习交流,PQ控制三相并网逆变器:功率控制、PWM调制与LCL滤波器的应用及仿真模型学习交流,PQ控制三相并网逆变器,并网有功
- deepseek世上最全最详细的文档,包含10多个pdf文件
- 《绝区零》角色系统拆解
- 模拟退火算法与最低水平线算法结合的矩形排样与下料优化系统:输出最佳剩料率与水平线,支持数据替换优化,程序已调试完成可直接运行,模拟退火与最低水平线算法相结合的矩形排样与下料优化系统-实现剩余材料率优
- 双向全桥LLC谐振变换器与隔离型双向变换器的交流电网仿真研究:变频控制与闭环策略分析,双向全桥LLC谐振变换器与隔离型双向变换器的交流电网仿真研究:变频控制与闭环策略探讨,双向全桥LLC谐振变器并入交
- 《销售漏斗心理学》读书分享PPT
- 基于随机森林算法RF的多输入单输出数据回归预测-含详细注释的代码与性能评估指标(决定系数R2、平均绝对误差MAE、平均相对误差MBE),基于随机森林算法RF的多输入单输出数据回归预测-含详细注释的
- DCP9040CN-MFC9440CN维修手册(维修站)
- 全球商品和服务出口(1960 年至今,17特征)数据集 CSV
- 全球交通事故数据集(10,000 条交通事故报告记录,11特征)CSV
- 欧洲足球联赛的比赛统计数据集(23类赛事,244,038场比赛,7特征)CSV
- Python数据分析实例:基于航空公司客户飞行数据进行忠诚度分析及聚类建模
- 文化旅游数据集(5000 个样本,14特征)CSV
- 陨石登陆地球数据集(34,500+ 陨石着陆的详细记录,10特征)CSV
- 手机型号的详细规格数据集(900+记录,15特征)CSV
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


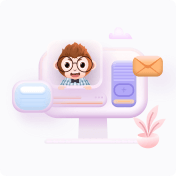
安全验证
文档复制为VIP权益,开通VIP直接复制
