package cn.com.webxml;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.WebEndpoint;
import javax.xml.ws.WebServiceClient;
import javax.xml.ws.WebServiceFeature;
import javax.xml.ws.Service;
/**
* <a href="http://www.webxml.com.cn/" target="_blank">WebXml.com.cn</a> <strong>天气预报 Web 服务,</strong>数据来源于<strong>中国气象局</strong> <a href="http://www.cma.gov.cn/" target="_blank">http://www.cma.gov.cn/</a> ,数据每2.5小时左右自动更新一次,准确可靠。包括 340 多个中国主要城市和 60 多个国外主要城市三日内的天气预报数据。</br>此天气预报Web Services请不要用于任何商业目的,若有需要请<a href="http://www.webxml.com.cn/zh_cn/contact_us.aspx" target="_blank">联系我们</a>,欢迎技术交流。 QQ:8409035<br /><strong>使用本站 WEB 服务请注明或链接本站:http://www.webxml.com.cn/ 感谢大家的支持</strong>!<br /><span style="color:#999999;">通知:天气预报 WEB 服务如原来使用地址 http://www.onhap.com/WebServices/WeatherWebService.asmx 的,请改成现在使用的服务地址 http://www.webxml.com.cn/WebServices/WeatherWebService.asmx ,重新引用即可。</span><br /><br />
*
* This class was generated by Apache CXF 2.7.0
* 2012-11-23T14:44:52.390+08:00
* Generated source version: 2.7.0
*
*/
@WebServiceClient(name = "WeatherWebService",
wsdlLocation = "weather.wsdl",
targetNamespace = "http://WebXml.com.cn/")
public class WeatherWebService extends Service {
public final static URL WSDL_LOCATION;
public final static QName SERVICE = new QName("http://WebXml.com.cn/", "WeatherWebService");
public final static QName WeatherWebServiceHttpPost = new QName("http://WebXml.com.cn/", "WeatherWebServiceHttpPost");
public final static QName WeatherWebServiceHttpGet = new QName("http://WebXml.com.cn/", "WeatherWebServiceHttpGet");
public final static QName WeatherWebServiceSoap = new QName("http://WebXml.com.cn/", "WeatherWebServiceSoap");
public final static QName WeatherWebServiceSoap12 = new QName("http://WebXml.com.cn/", "WeatherWebServiceSoap12");
static {
URL url = WeatherWebService.class.getResource("weather.wsdl");
if (url == null) {
java.util.logging.Logger.getLogger(WeatherWebService.class.getName())
.log(java.util.logging.Level.INFO,
"Can not initialize the default wsdl from {0}", "weather.wsdl");
}
WSDL_LOCATION = url;
}
public WeatherWebService(URL wsdlLocation) {
super(wsdlLocation, SERVICE);
}
public WeatherWebService(URL wsdlLocation, QName serviceName) {
super(wsdlLocation, serviceName);
}
public WeatherWebService() {
super(WSDL_LOCATION, SERVICE);
}
//This constructor requires JAX-WS API 2.2. You will need to endorse the 2.2
//API jar or re-run wsdl2java with "-frontend jaxws21" to generate JAX-WS 2.1
//compliant code instead.
// public WeatherWebService(WebServiceFeature ... features) {
// super(WSDL_LOCATION, SERVICE, features);
// }
//This constructor requires JAX-WS API 2.2. You will need to endorse the 2.2
//API jar or re-run wsdl2java with "-frontend jaxws21" to generate JAX-WS 2.1
//compliant code instead.
// public WeatherWebService(URL wsdlLocation, WebServiceFeature ... features) {
// super(wsdlLocation, SERVICE, features);
// }
//This constructor requires JAX-WS API 2.2. You will need to endorse the 2.2
//API jar or re-run wsdl2java with "-frontend jaxws21" to generate JAX-WS 2.1
//compliant code instead.
// public WeatherWebService(URL wsdlLocation, QName serviceName, WebServiceFeature ... features) {
// super(wsdlLocation, serviceName, features);
// }
/**
*
* @return
* returns WeatherWebServiceHttpPost
*/
@WebEndpoint(name = "WeatherWebServiceHttpPost")
public WeatherWebServiceHttpPost getWeatherWebServiceHttpPost() {
return super.getPort(WeatherWebServiceHttpPost, WeatherWebServiceHttpPost.class);
}
/**
*
* @param features
* A list of {@link javax.xml.ws.WebServiceFeature} to configure on the proxy. Supported features not in the <code>features</code> parameter will have their default values.
* @return
* returns WeatherWebServiceHttpPost
*/
@WebEndpoint(name = "WeatherWebServiceHttpPost")
public WeatherWebServiceHttpPost getWeatherWebServiceHttpPost(WebServiceFeature... features) {
return super.getPort(WeatherWebServiceHttpPost, WeatherWebServiceHttpPost.class, features);
}
/**
*
* @return
* returns WeatherWebServiceHttpGet
*/
@WebEndpoint(name = "WeatherWebServiceHttpGet")
public WeatherWebServiceHttpGet getWeatherWebServiceHttpGet() {
return super.getPort(WeatherWebServiceHttpGet, WeatherWebServiceHttpGet.class);
}
/**
*
* @param features
* A list of {@link javax.xml.ws.WebServiceFeature} to configure on the proxy. Supported features not in the <code>features</code> parameter will have their default values.
* @return
* returns WeatherWebServiceHttpGet
*/
@WebEndpoint(name = "WeatherWebServiceHttpGet")
public WeatherWebServiceHttpGet getWeatherWebServiceHttpGet(WebServiceFeature... features) {
return super.getPort(WeatherWebServiceHttpGet, WeatherWebServiceHttpGet.class, features);
}
/**
*
* @return
* returns WeatherWebServiceSoap
*/
@WebEndpoint(name = "WeatherWebServiceSoap")
public WeatherWebServiceSoap getWeatherWebServiceSoap() {
return super.getPort(WeatherWebServiceSoap, WeatherWebServiceSoap.class);
}
/**
*
* @param features
* A list of {@link javax.xml.ws.WebServiceFeature} to configure on the proxy. Supported features not in the <code>features</code> parameter will have their default values.
* @return
* returns WeatherWebServiceSoap
*/
@WebEndpoint(name = "WeatherWebServiceSoap")
public WeatherWebServiceSoap getWeatherWebServiceSoap(WebServiceFeature... features) {
return super.getPort(WeatherWebServiceSoap, WeatherWebServiceSoap.class, features);
}
/**
*
* @return
* returns WeatherWebServiceSoap
*/
@WebEndpoint(name = "WeatherWebServiceSoap12")
public WeatherWebServiceSoap getWeatherWebServiceSoap12() {
return super.getPort(WeatherWebServiceSoap12, WeatherWebServiceSoap.class);
}
/**
*
* @param features
* A list of {@link javax.xml.ws.WebServiceFeature} to configure on the proxy. Supported features not in the <code>features</code> parameter will have their default values.
* @return
* returns WeatherWebServiceSoap
*/
@WebEndpoint(name = "WeatherWebServiceSoap12")
public WeatherWebServiceSoap getWeatherWebServiceSoap12(WebServiceFeature... features) {
return super.getPort(WeatherWebServiceSoap12, WeatherWebServiceSoap.class, features);
}
}

千骑卷平冈
- 粉丝: 26
- 资源: 6
最新资源
- LC-VCO电感电容压控振荡器,很适合新手入门锁相环 pll cppll 有现成的testbench,新手可以先单独仿真电感L,电容C的各项参考曲线,比如实部,虚部阻抗,Rs,Rp值,还有Q值,容值电
- 10月最新美化更新情侣飞行棋网站源码情侣小游戏含修改教程等
- 基于Python的图书管理系统控制台应用
- 永磁同步电机(PMSM)采用超扭滑模观测器(STSMO)的无差电流预测控制(DPCC)参数扰动观测器方法matlab仿真
- Jsoup 实现的java爬虫
- LC-VCO电感电容压控振荡器,很适合新手入门锁相环 pll cppll 有现成的testbench,新手可以先单独仿真电感L,电容C的各项参考曲线,比如实部,虚部阻抗,Rs,Rp值,还有Q值,容值电
- ISO21111 1-5规范
- 调优-基于鲲鹏平台的软硬件优化实践
- gbaseV8s-JDBC驱动包
- 医疗器械证办理时所需的QM质量手册模版
- 西门子S7-1200 1500动态加密功能块程序,可以设置停机运行时间,时间到达设备停止运行,专门针对不守信用的客户使用 博图V16版本,有具体使用视频教程, 里面有两个功能块,一个是动态加密的,一个
- 通过python和transformers库进行感情分析.zip
- class文件反编译图形化工具
- 2024安装最新版的VMware过程
- 环形振荡器 ring vco oscillator 锁相环 pll PLL 压控振荡器 振荡器 集成电路 芯片设计 模拟ic设计 1没基础的同学,首先学习cadence管方 电路+仿真教学
- 三电平半桥LLC谐振变器电路仿真 采用频率控制方式 引入一定的移相角度(比较小) 驱动信号采用CMPA CMPB方式产生 增计数模式(参照DSP PWM生成) 相比普通半桥LLC开关管电压应力小 输出
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


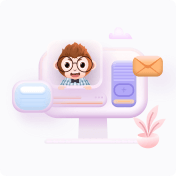
- 1
- 2
- 3
- 4
- 5
前往页