package identification_of_rice.pojo;
import java.util.ArrayList;
import java.util.List;
public class PestExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public PestExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table pest
*
* @mbggenerated Sat Nov 05 20:30:53 CST 2022
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andPestidIsNull() {
addCriterion("pestid is null");
return (Criteria) this;
}
public Criteria andPestidIsNotNull() {
addCriterion("pestid is not null");
return (Criteria) this;
}
public Criteria andPestidEqualTo(Integer value) {
addCriterion("pestid =", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidNotEqualTo(Integer value) {
addCriterion("pestid <>", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidGreaterThan(Integer value) {
addCriterion("pestid >", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidGreaterThanOrEqualTo(Integer value) {
addCriterion("pestid >=", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidLessThan(Integer value) {
addCriterion("pestid <", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidLessThanOrEqualTo(Integer value) {
addCriterion("pestid <=", value, "pestid");
return (Criteria) this;
}
public Criteria andPestidIn(List<Integer> values) {
addCriterion("pestid in", values, "pestid");
return (Criteria) this;
}
public Criteria andPestidNotIn(List<Integer> values) {
addCriterion("pestid not in", values, "pestid");
return (Criteria) this;
}
public Criteria andPestidBetween(Integer value1, Integer value2) {
addCriterion("pestid between", value1, value2, "pestid");
return (Criteria) this;
}
public Criteria andPestidNotBetween(Integer value1, Integer value2) {
addCriterion("pestid not between", value1, value2, "pestid");
return (Criteria) this;
}
public Criteria andKindIsNull() {
addCriterion("kind is null");
return (Criteria) this;
}
public Criteria andKindIsNotNull() {
addCriterion("kind is not null");
return (Criteria) this;
}
public Criteria andKindEqualTo(Integer value) {
addCriterion("kind =", value, "kind");
return (Criteria) this;
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目是一款融合SSM和Flask框架的稻米图像识别系统设计源码,涵盖604个文件,包括299个GIF动画、113个JavaScript脚本、48个JPG图像、31个Java源代码、30个HTML文档、25个CSS样式表、24个PNG图片、10个XML配置文件、3个Python脚本、3个EOT字体文件。系统以Flask处理图像识别任务,Java负责业务逻辑。
资源推荐
资源详情
资源评论
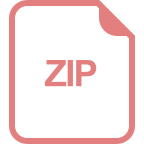
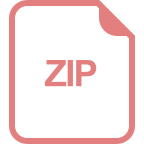
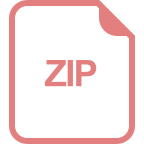
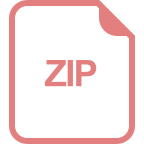
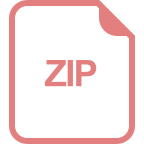
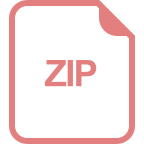
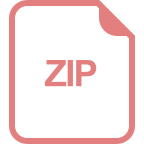
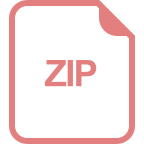
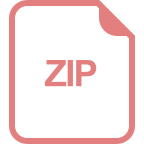
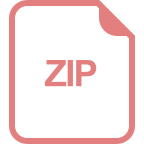
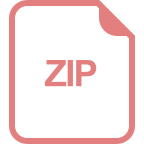
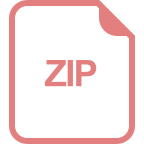
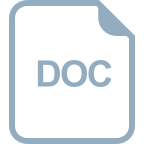
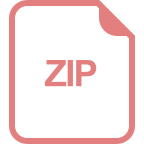
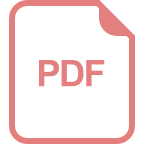
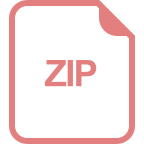
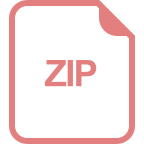
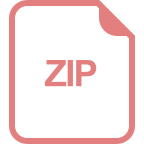
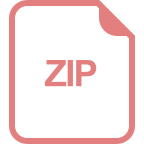
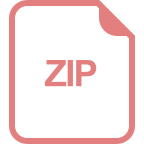
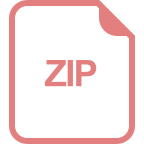
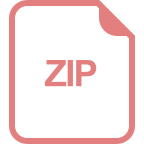
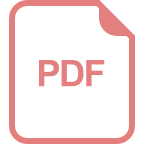
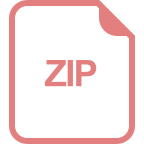
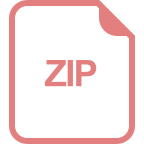
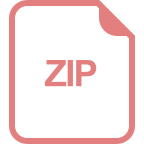
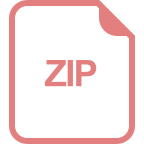
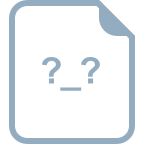
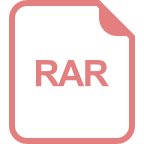
收起资源包目录

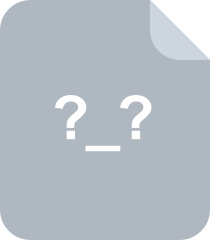
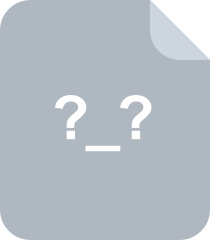
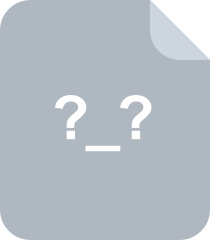
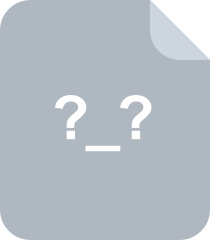
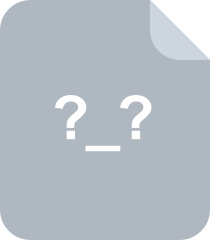
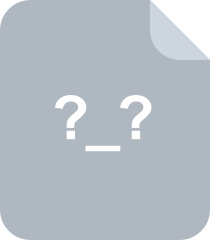
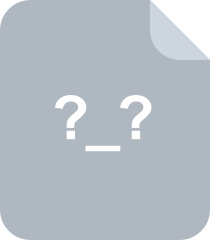
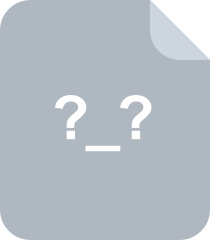
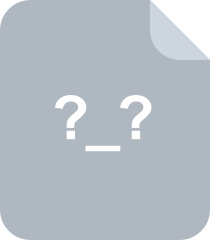
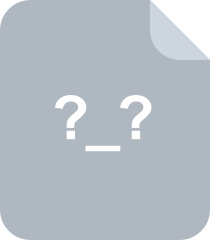
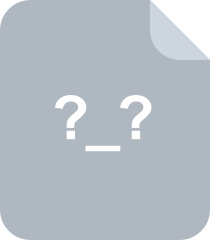
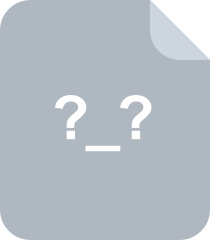
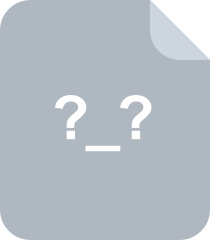
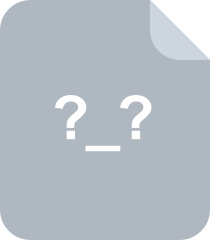
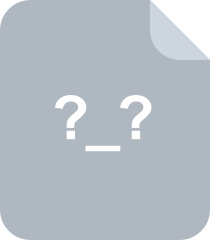
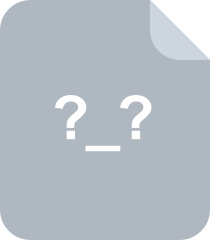
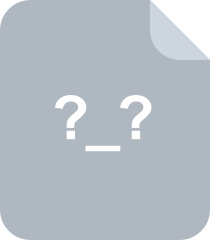
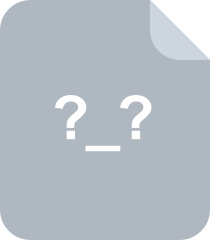
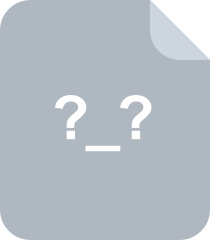
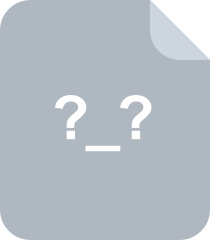
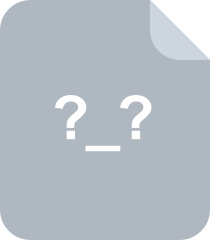
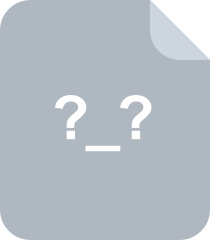
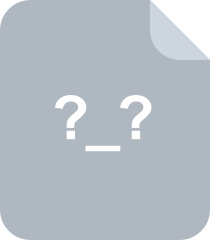
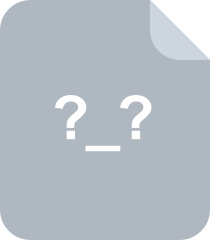
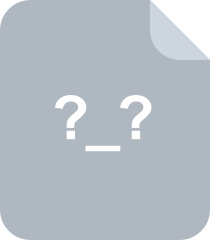
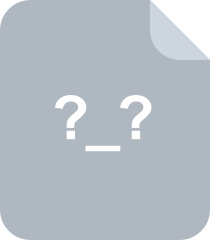
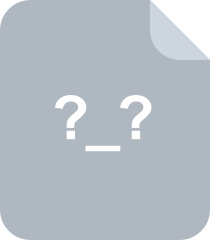
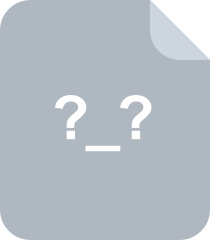
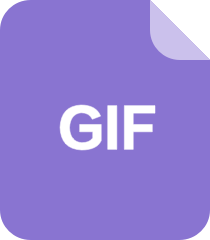
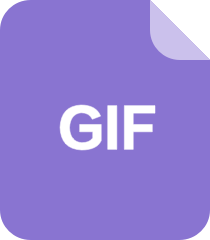
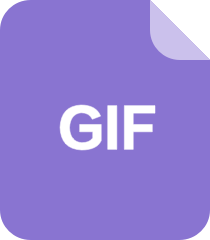
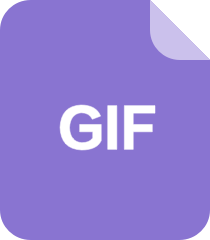
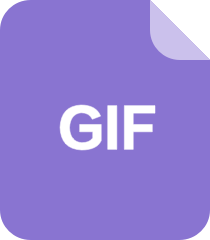
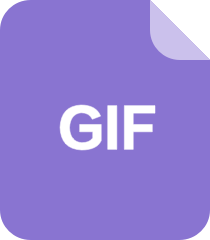
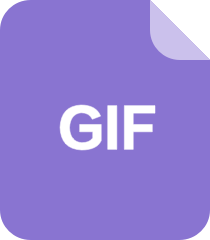
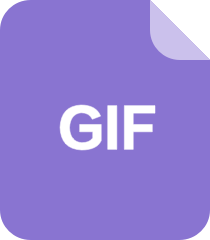
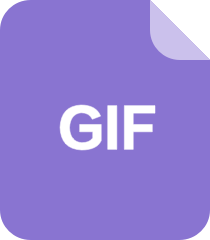
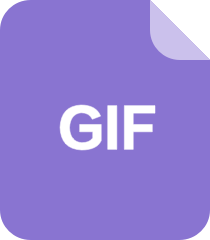
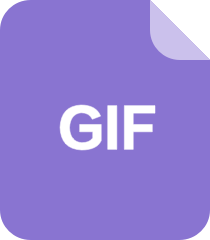
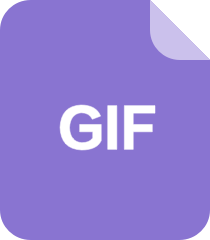
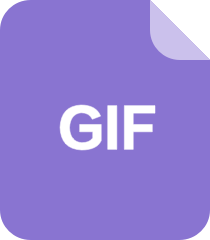
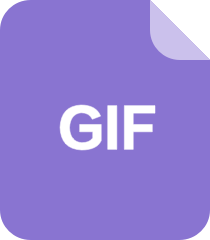
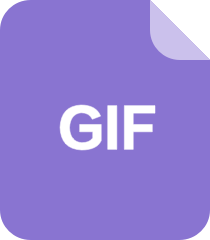
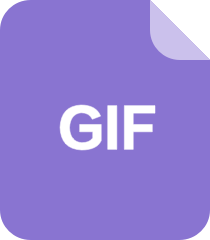
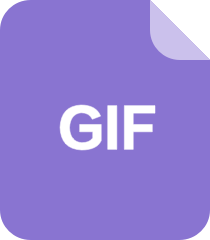
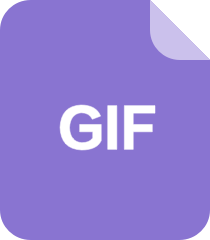
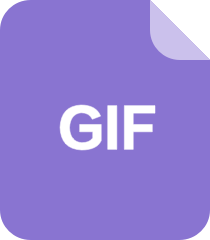
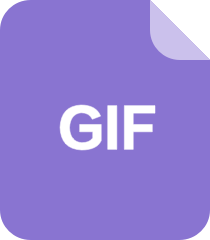
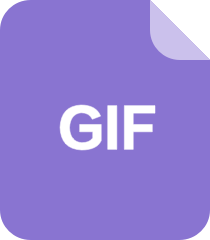
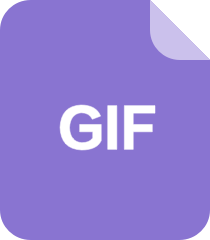
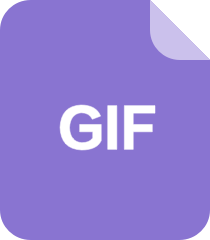
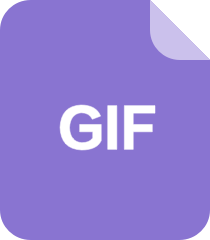
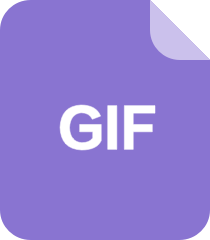
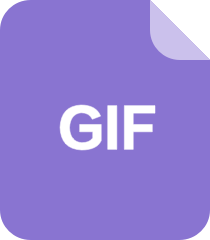
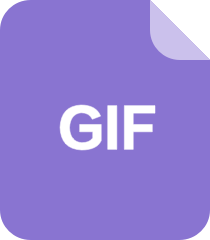
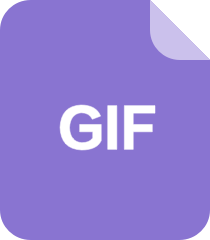
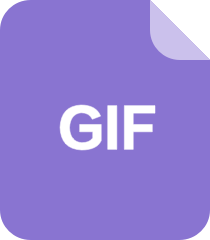
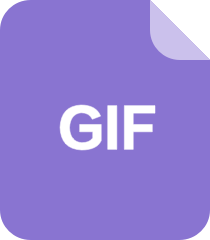
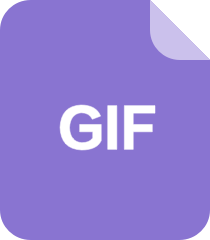
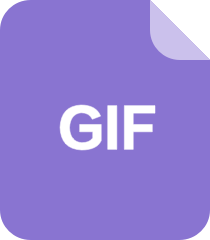
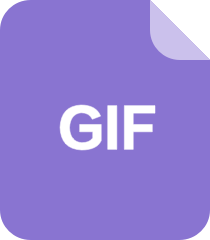
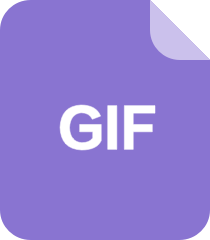
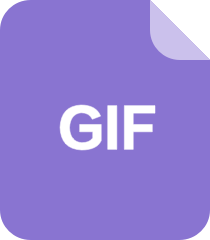
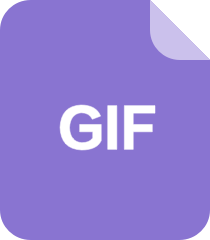
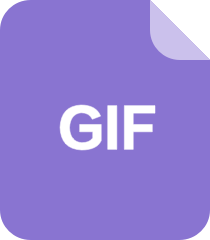
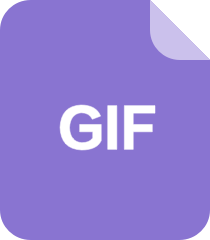
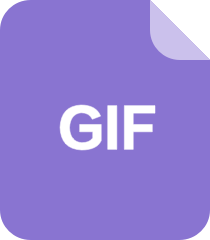
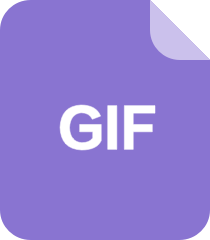
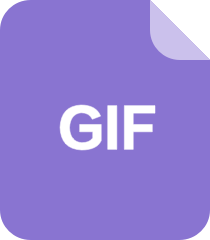
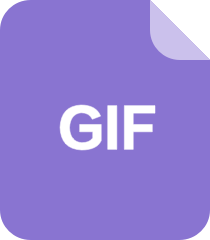
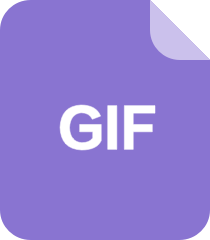
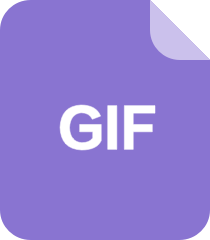
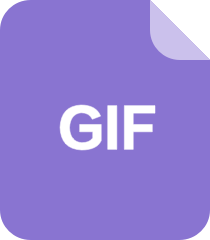
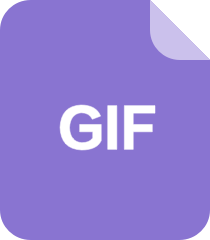
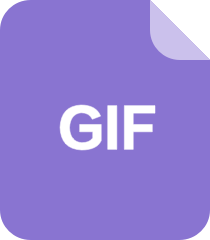
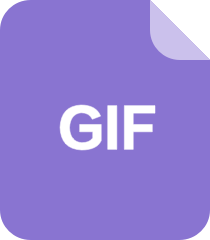
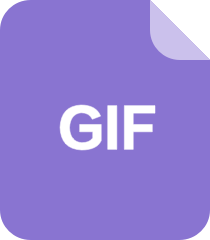
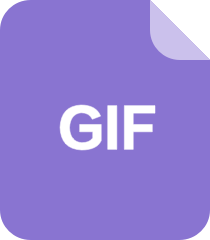
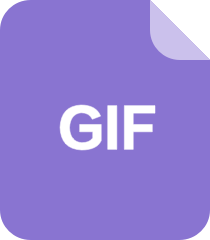
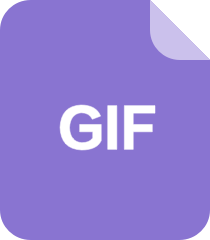
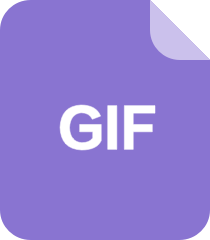
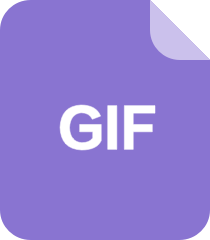
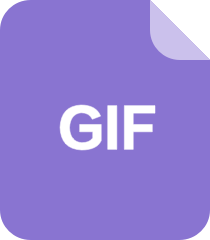
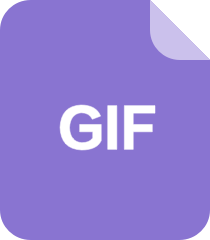
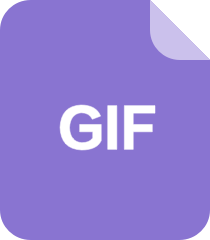
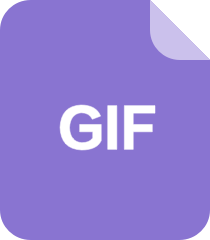
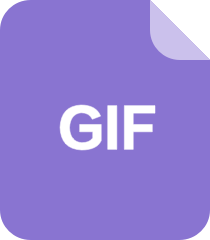
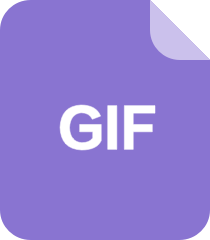
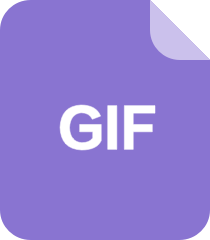
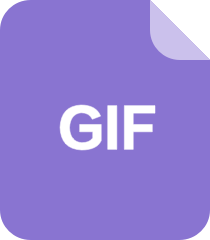
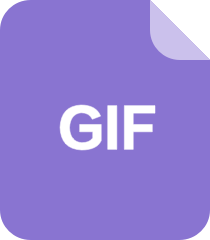
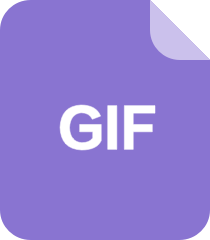
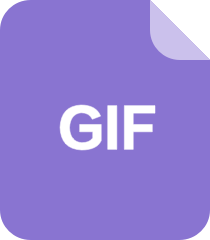
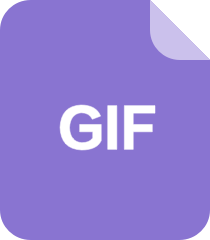
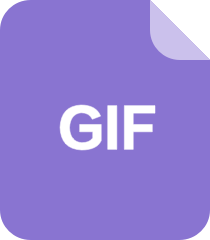
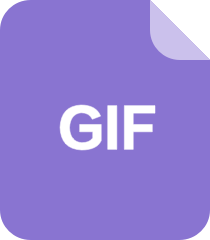
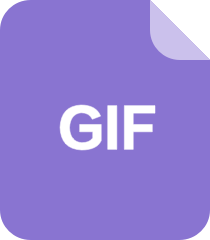
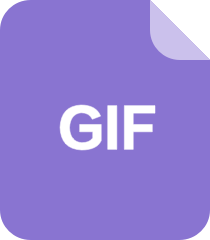
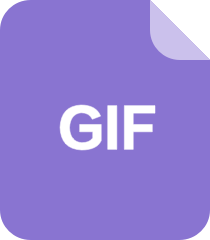
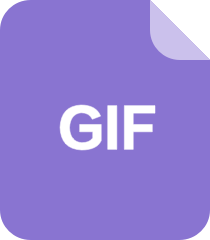
共 605 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
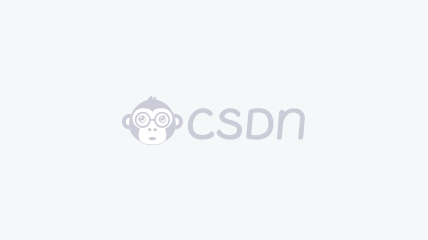

wjs2024
- 粉丝: 2242
- 资源: 5454
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

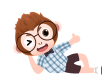
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


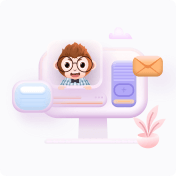
安全验证
文档复制为VIP权益,开通VIP直接复制
