package com.hyq.hm.landmarksticker;
import android.Manifest;
import android.content.Context;
import android.content.pm.PackageManager;
import android.graphics.Rect;
import android.hardware.Camera;
import android.opengl.GLES20;
import android.os.Build;
import android.os.Environment;
import android.os.Handler;
import android.os.HandlerThread;
import android.support.annotation.NonNull;
import android.support.v4.app.ActivityCompat;
import android.support.v4.content.PermissionChecker;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.SurfaceHolder;
import android.view.SurfaceView;
import android.widget.CheckBox;
import android.widget.SeekBar;
import android.widget.Toast;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import trackingsoft.tracking.Face;
import trackingsoft.tracking.FaceTracking;
public class MainActivity extends AppCompatActivity {
private String modelPath = Environment.getExternalStorageDirectory()
+ File.separator + "FaceTracking";
public void copyFilesFromAssets(Context context, String oldPath, String newPath) {
try {
String[] fileNames = context.getAssets().list(oldPath);
if (fileNames.length > 0) {
// directory
File file = new File(newPath);
if (!file.mkdir()) {
Log.d("mkdir", "can't make folder");
}
for (String fileName : fileNames) {
copyFilesFromAssets(context, oldPath + "/" + fileName,
newPath + "/" + fileName);
}
} else {
// file
InputStream is = context.getAssets().open(oldPath);
FileOutputStream fos = new FileOutputStream(new File(newPath));
byte[] buffer = new byte[1024];
int byteCount;
while ((byteCount = is.read(buffer)) != -1) {
fos.write(buffer, 0, byteCount);
}
fos.flush();
is.close();
fos.close();
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
void InitModelFiles() {
String assetPath = "FaceTracking";
// String sdcardPath = modelPath;
// Log.e("TAG","sdcardPath=====>"+sdcardPath);
copyFilesFromAssets(this, assetPath, modelPath);
}
private String[] denied;
private String[] permissions = {Manifest.permission.WRITE_EXTERNAL_STORAGE, Manifest.permission.CAMERA};
public static FaceTracking mMultiTrack106 = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
ArrayList<String> list = new ArrayList<>();
for (int i = 0; i < permissions.length; i++) {
if (PermissionChecker.checkSelfPermission(this, permissions[i]) == PackageManager.PERMISSION_DENIED) {
list.add(permissions[i]);
}
}
if (list.size() != 0) {
denied = new String[list.size()];
for (int i = 0; i < list.size(); i++) {
denied[i] = list.get(i);
}
ActivityCompat.requestPermissions(this, denied, 5);
} else {
init();
}
} else {
init();
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
if (requestCode == 5) {
boolean isDenied = false;
for (int i = 0; i < denied.length; i++) {
String permission = denied[i];
for (int j = 0; j < permissions.length; j++) {
if (permissions[j].equals(permission)) {
if (grantResults[j] != PackageManager.PERMISSION_GRANTED) {
isDenied = true;
break;
}
}
}
}
if (isDenied) {
Toast.makeText(this, "Please give permission.", Toast.LENGTH_SHORT).show();
} else {
init();
}
}
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
}
private HandlerThread mHandlerThread;
private Handler mHandler;
private final byte[] mNv21Data = new byte[CameraOverlap.PREVIEW_WIDTH * CameraOverlap.PREVIEW_HEIGHT * 2];
private CameraOverlap cameraOverlap;
private SurfaceView mSurfaceView;
private EGLUtils mEglUtils;
private GLFramebuffer mFramebuffer;
private GLFrame mFrame;
private SeekBar seekBarA;
private SeekBar seekBarB;
private SeekBar seekBarC;
private CheckBox checkBox;
private final int maxFace = 10;
private final int bitmapCount = 3;
private List<GLPoints> mPoints;
private List<GLBitmap> mBitmaps;
private int index = 0;
private void init() {
InitModelFiles();
cameraOverlap = new CameraOverlap(this);
mFramebuffer = new GLFramebuffer();
mFrame = new GLFrame();
mPoints = new ArrayList<>();
mBitmaps = new ArrayList<>();
for (int i = 0; i < maxFace; i++) {
mPoints.add(new GLPoints());
}
for (int i = 0; i < maxFace * bitmapCount; i++) {
mBitmaps.add(new GLBitmap(this, R.drawable.face_005));
}
mHandlerThread = new HandlerThread("DrawFacePointsThread");
mHandlerThread.start();
mHandler = new Handler(mHandlerThread.getLooper());
Log.e("TAG","=========");
cameraOverlap.setPreviewCallback(new Camera.PreviewCallback() {
@Override
public void onPreviewFrame(byte[] data, Camera camera) {
// Log.e("TAG","======onPreviewFrame========"+index);
index++;
synchronized (mNv21Data) {
System.arraycopy(data, 0, mNv21Data, 0, data.length);
}
mHandler.post(new Runnable() {
@Override
public void run() {
// if (mEglUtils == null) {
// return;
// }
mFrame.setS(seekBarA.getProgress() / 100.0f);
mFrame.setH(seekBarB.getProgress() / 360.0f);
mFrame.setL(seekBarC.getProgress() / 100.0f - 1);
if (mMultiTrack106 == null) {
mMultiTrack106 = new FaceTracking(modelPath + File.separator + "models");
mMultiTrack106.FaceTrackingInit(mNv21Data, CameraOverlap.PREVIEW_HEIGHT, CameraOverlap.PREVIEW_WIDTH);
} else {
mMultiTrack106.Update(mNv21Data, CameraOverlap.PREVIEW_HEIGHT, CameraOverlap.PREVIEW_WIDTH);
// Log.e("TAG", "=====Update=====");
}
boolean rotate270 = cameraOverlap.getOrientation() == 270;
List<Face> faceActions = mMultiTrack106.getTrackingInfo();
GLES20.glEnable(GLES20.GL_BLEND);
GLES20.glBlendFunc(GLES20.GL_SRC_ALPHA, GLES20.GL_ONE_MINUS_SRC_ALPHA);
mFrame.drawFrame(mFramebuffer.drawFrameBuffer(), mFramebuffer.getMatrix());
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该开源视频人脸跟踪算法项目,基于MTcnn人脸检测与ONet人脸跟踪技术,采用C++为主要开发语言,并包含C、Java语言支持。项目包含389个文件,涵盖215个头文件(.hpp)、76个头文件(.h)、20个Java文件、13个XML文件、13个PNG图片、9个二进制文件、9个参数文件、7个C++源文件、3个文本文件、3个Gradle构建脚本。算法在移动端实现速度高达150fps以上,且基于Android平台,提供JNI底层接口,方便用户移植至其他平台。该项目依赖轻量级的ncnn深度学习计算库,便于集成。
资源推荐
资源详情
资源评论
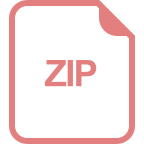
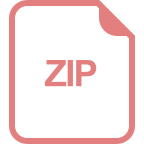
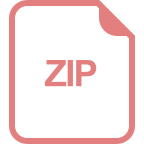
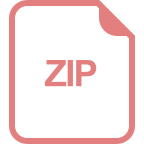
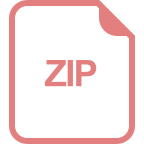
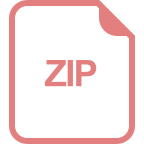
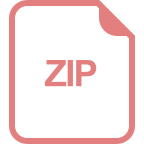
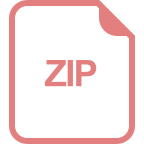
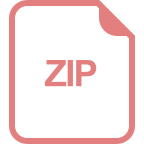
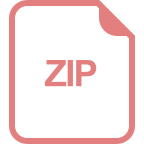
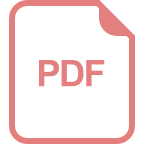
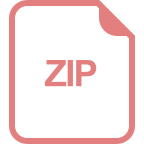
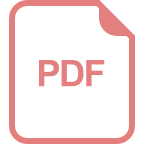
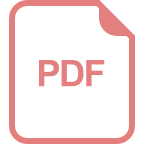
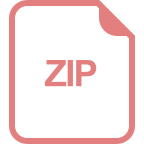
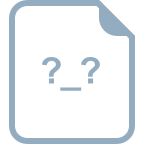
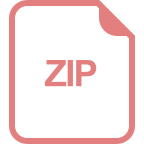
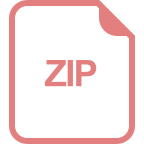
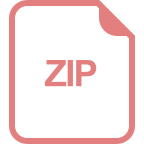
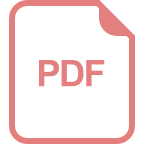
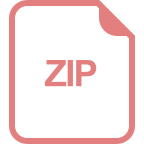
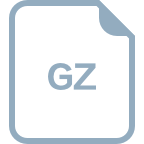
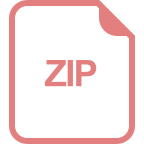
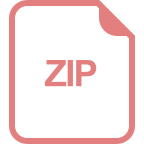
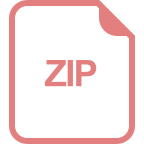
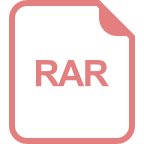
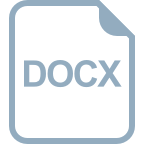
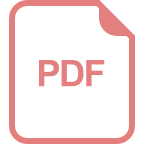
收起资源包目录

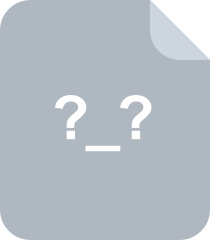
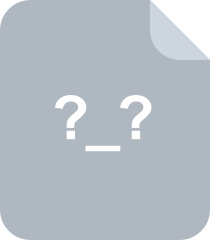
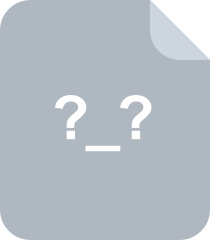
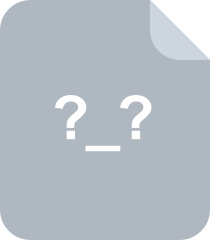
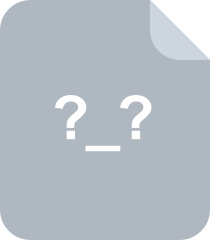
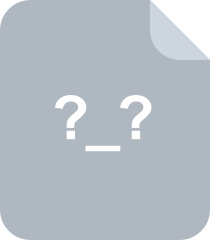
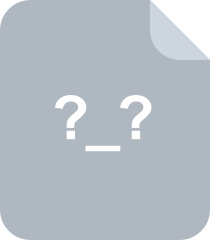
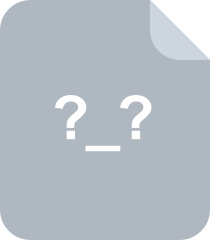
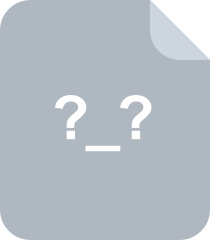
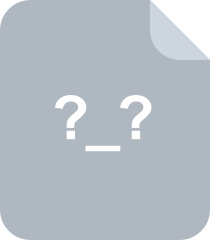
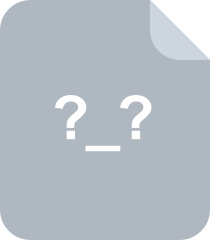
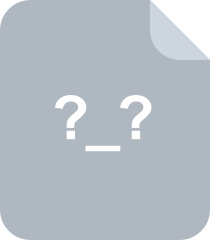
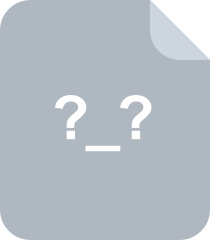
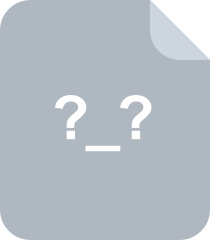
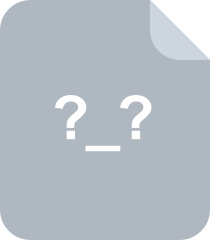
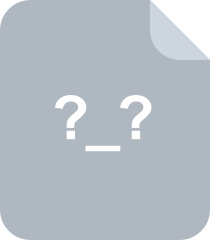
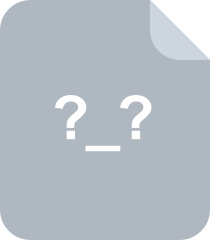
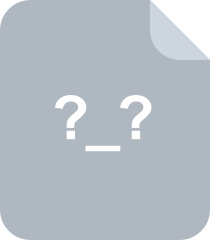
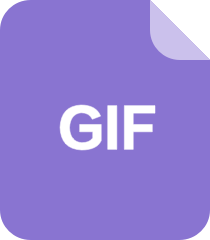
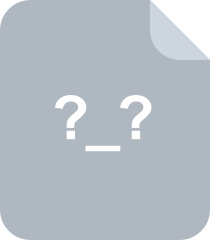
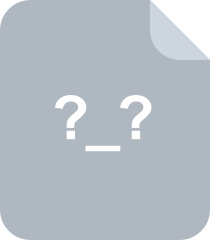
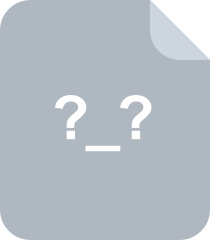
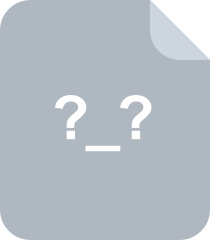
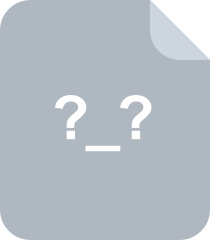
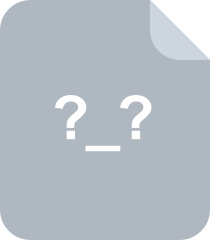
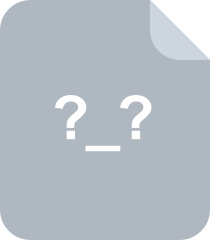
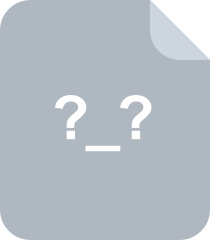
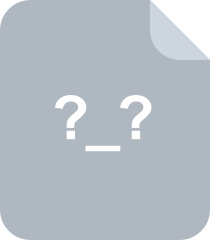
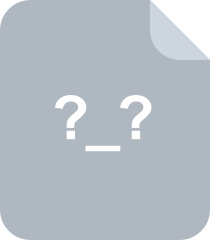
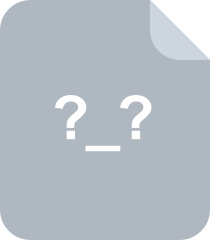
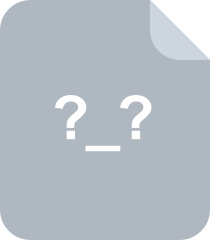
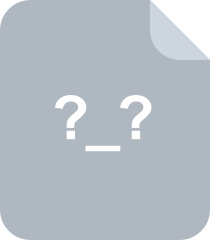
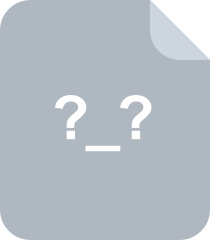
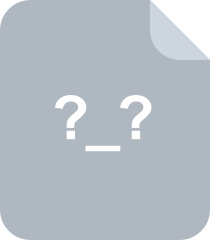
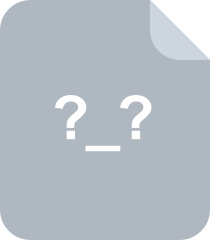
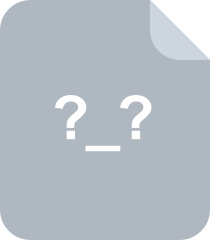
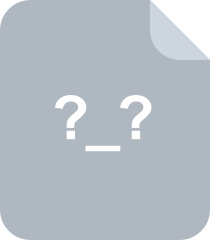
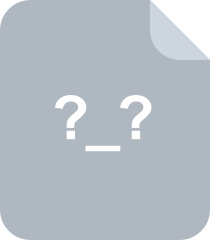
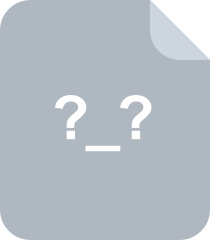
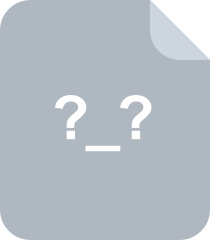
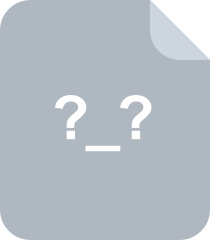
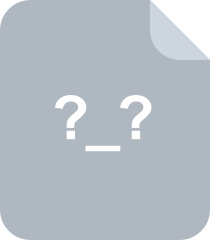
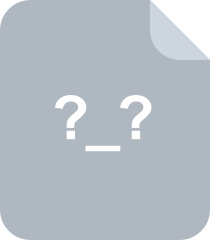
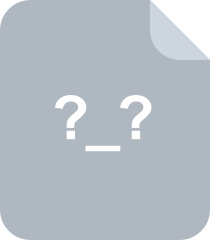
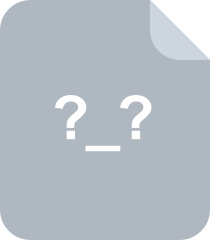
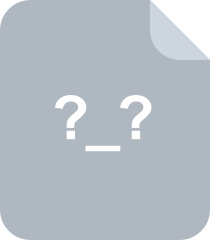
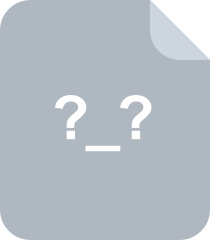
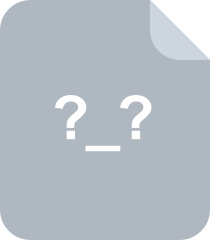
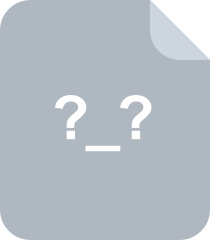
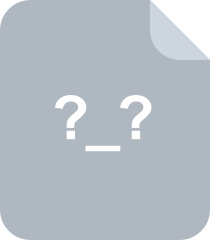
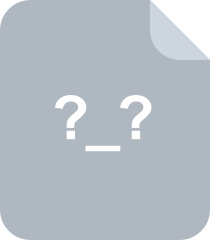
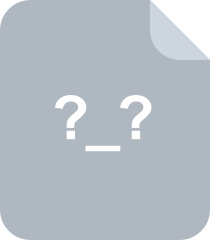
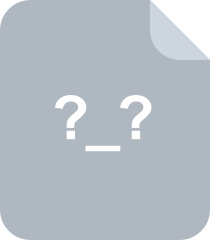
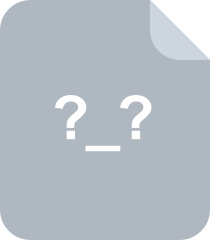
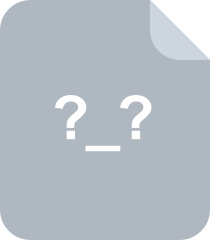
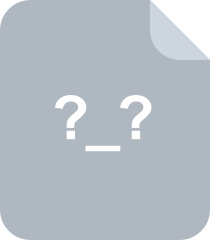
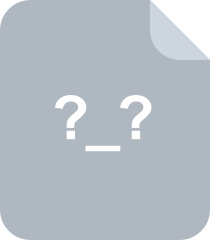
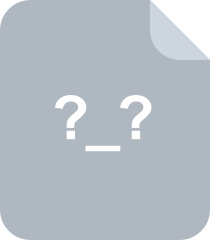
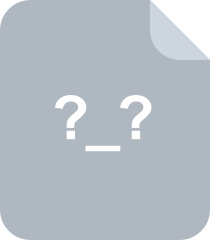
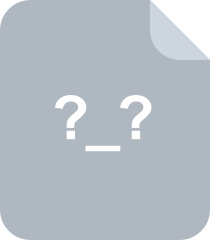
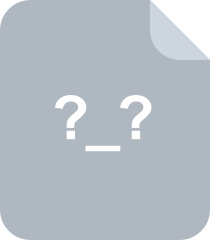
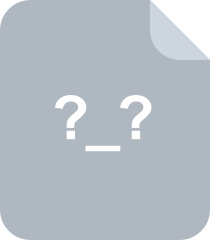
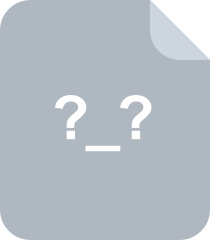
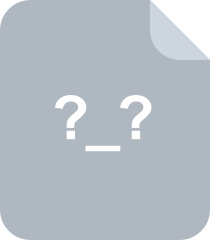
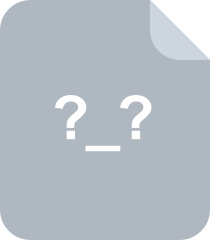
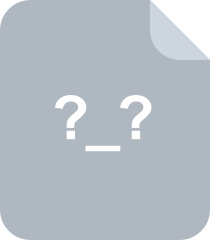
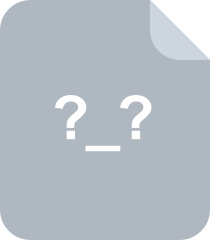
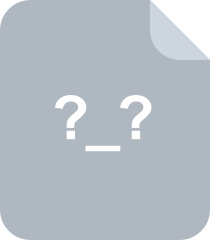
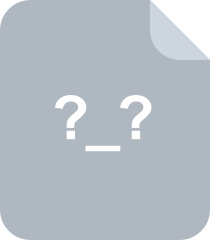
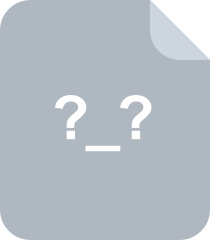
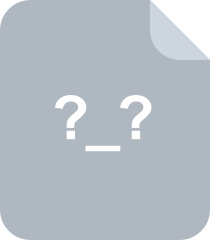
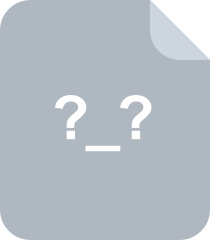
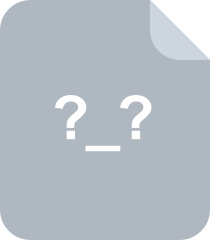
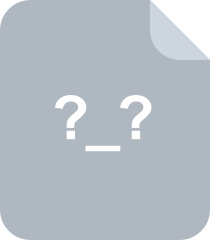
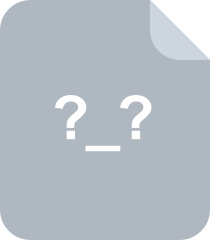
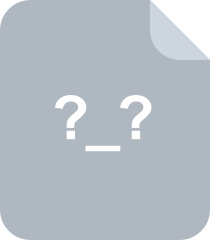
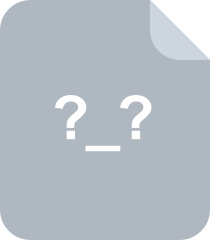
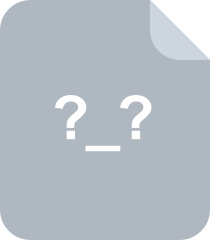
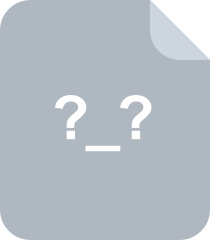
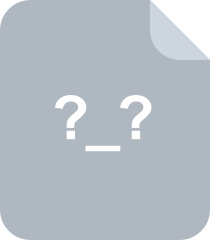
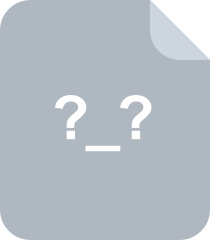
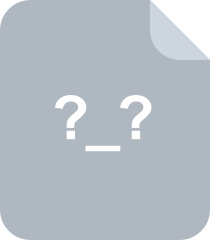
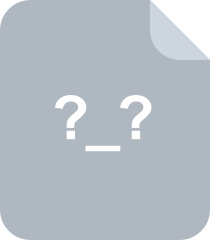
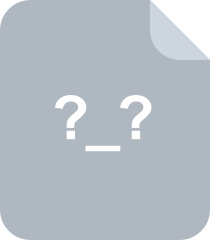
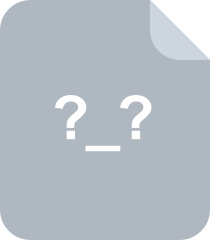
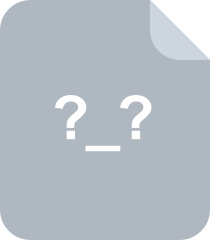
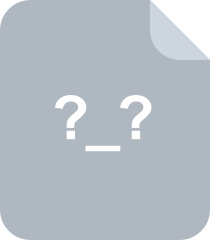
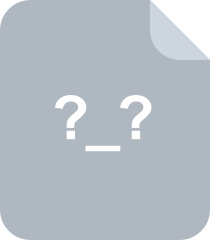
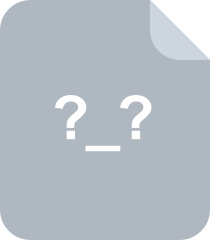
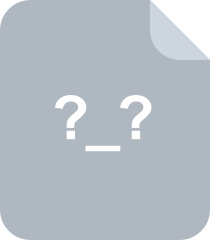
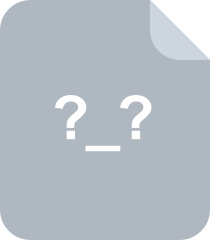
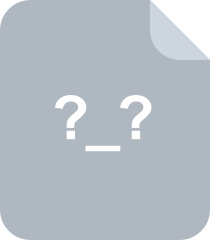
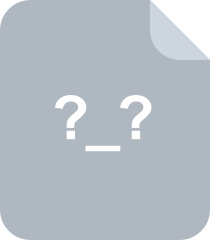
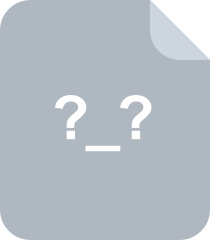
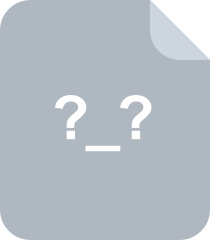
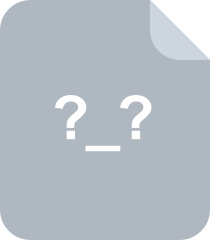
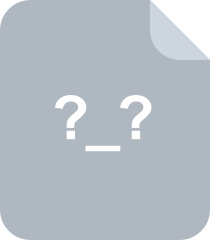
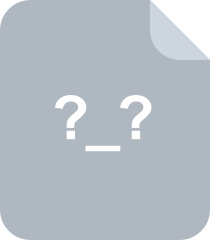
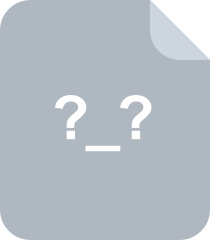
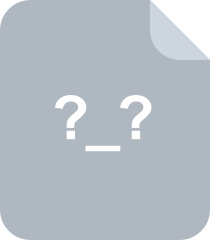
共 387 条
- 1
- 2
- 3
- 4
资源评论
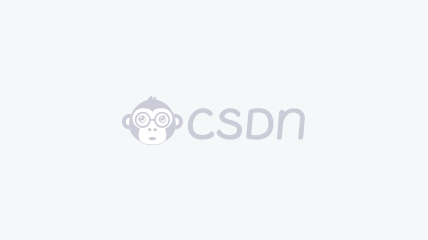

wjs2024
- 粉丝: 2163
- 资源: 5442
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

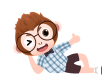
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


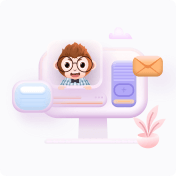
安全验证
文档复制为VIP权益,开通VIP直接复制
